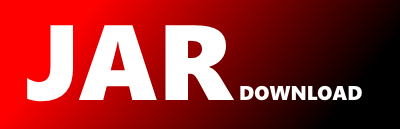
software.amazon.awssdk.services.elasticloadbalancingv2.model.CreateLoadBalancerRequest Maven / Gradle / Ivy
Show all versions of elasticloadbalancingv2 Show documentation
/*
* Copyright 2014-2019 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.elasticloadbalancingv2.model;
import java.util.Arrays;
import java.util.Collection;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import java.util.stream.Collectors;
import java.util.stream.Stream;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.awscore.AwsRequestOverrideConfiguration;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.ListTrait;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.core.util.DefaultSdkAutoConstructList;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*/
@Generated("software.amazon.awssdk:codegen")
public final class CreateLoadBalancerRequest extends ElasticLoadBalancingV2Request implements
ToCopyableBuilder {
private static final SdkField NAME_FIELD = SdkField. builder(MarshallingType.STRING)
.getter(getter(CreateLoadBalancerRequest::name)).setter(setter(Builder::name))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Name").build()).build();
private static final SdkField> SUBNETS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.getter(getter(CreateLoadBalancerRequest::subnets))
.setter(setter(Builder::subnets))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Subnets").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.STRING)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField> SUBNET_MAPPINGS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.getter(getter(CreateLoadBalancerRequest::subnetMappings))
.setter(setter(Builder::subnetMappings))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("SubnetMappings").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(SubnetMapping::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField> SECURITY_GROUPS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.getter(getter(CreateLoadBalancerRequest::securityGroups))
.setter(setter(Builder::securityGroups))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("SecurityGroups").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.STRING)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField SCHEME_FIELD = SdkField. builder(MarshallingType.STRING)
.getter(getter(CreateLoadBalancerRequest::schemeAsString)).setter(setter(Builder::scheme))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Scheme").build()).build();
private static final SdkField> TAGS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.getter(getter(CreateLoadBalancerRequest::tags))
.setter(setter(Builder::tags))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Tags").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(Tag::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField TYPE_FIELD = SdkField. builder(MarshallingType.STRING)
.getter(getter(CreateLoadBalancerRequest::typeAsString)).setter(setter(Builder::type))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Type").build()).build();
private static final SdkField IP_ADDRESS_TYPE_FIELD = SdkField. builder(MarshallingType.STRING)
.getter(getter(CreateLoadBalancerRequest::ipAddressTypeAsString)).setter(setter(Builder::ipAddressType))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("IpAddressType").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(NAME_FIELD, SUBNETS_FIELD,
SUBNET_MAPPINGS_FIELD, SECURITY_GROUPS_FIELD, SCHEME_FIELD, TAGS_FIELD, TYPE_FIELD, IP_ADDRESS_TYPE_FIELD));
private final String name;
private final List subnets;
private final List subnetMappings;
private final List securityGroups;
private final String scheme;
private final List tags;
private final String type;
private final String ipAddressType;
private CreateLoadBalancerRequest(BuilderImpl builder) {
super(builder);
this.name = builder.name;
this.subnets = builder.subnets;
this.subnetMappings = builder.subnetMappings;
this.securityGroups = builder.securityGroups;
this.scheme = builder.scheme;
this.tags = builder.tags;
this.type = builder.type;
this.ipAddressType = builder.ipAddressType;
}
/**
*
* The name of the load balancer.
*
*
* This name must be unique per region per account, can have a maximum of 32 characters, must contain only
* alphanumeric characters or hyphens, must not begin or end with a hyphen, and must not begin with "internal-".
*
*
* @return The name of the load balancer.
*
* This name must be unique per region per account, can have a maximum of 32 characters, must contain only
* alphanumeric characters or hyphens, must not begin or end with a hyphen, and must not begin with
* "internal-".
*/
public String name() {
return name;
}
/**
*
* The IDs of the public subnets. You can specify only one subnet per Availability Zone. You must specify either
* subnets or subnet mappings.
*
*
* [Application Load Balancers] You must specify subnets from at least two Availability Zones.
*
*
* [Network Load Balancers] You can specify subnets from one or more Availability Zones.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* @return The IDs of the public subnets. You can specify only one subnet per Availability Zone. You must specify
* either subnets or subnet mappings.
*
* [Application Load Balancers] You must specify subnets from at least two Availability Zones.
*
*
* [Network Load Balancers] You can specify subnets from one or more Availability Zones.
*/
public List subnets() {
return subnets;
}
/**
*
* The IDs of the public subnets. You can specify only one subnet per Availability Zone. You must specify either
* subnets or subnet mappings.
*
*
* [Application Load Balancers] You must specify subnets from at least two Availability Zones. You cannot specify
* Elastic IP addresses for your subnets.
*
*
* [Network Load Balancers] You can specify subnets from one or more Availability Zones. You can specify one Elastic
* IP address per subnet.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* @return The IDs of the public subnets. You can specify only one subnet per Availability Zone. You must specify
* either subnets or subnet mappings.
*
* [Application Load Balancers] You must specify subnets from at least two Availability Zones. You cannot
* specify Elastic IP addresses for your subnets.
*
*
* [Network Load Balancers] You can specify subnets from one or more Availability Zones. You can specify one
* Elastic IP address per subnet.
*/
public List subnetMappings() {
return subnetMappings;
}
/**
*
* [Application Load Balancers] The IDs of the security groups for the load balancer.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* @return [Application Load Balancers] The IDs of the security groups for the load balancer.
*/
public List securityGroups() {
return securityGroups;
}
/**
*
* The nodes of an Internet-facing load balancer have public IP addresses. The DNS name of an Internet-facing load
* balancer is publicly resolvable to the public IP addresses of the nodes. Therefore, Internet-facing load
* balancers can route requests from clients over the internet.
*
*
* The nodes of an internal load balancer have only private IP addresses. The DNS name of an internal load balancer
* is publicly resolvable to the private IP addresses of the nodes. Therefore, internal load balancers can only
* route requests from clients with access to the VPC for the load balancer.
*
*
* The default is an Internet-facing load balancer.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #scheme} will
* return {@link LoadBalancerSchemeEnum#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available
* from {@link #schemeAsString}.
*
*
* @return The nodes of an Internet-facing load balancer have public IP addresses. The DNS name of an
* Internet-facing load balancer is publicly resolvable to the public IP addresses of the nodes. Therefore,
* Internet-facing load balancers can route requests from clients over the internet.
*
* The nodes of an internal load balancer have only private IP addresses. The DNS name of an internal load
* balancer is publicly resolvable to the private IP addresses of the nodes. Therefore, internal load
* balancers can only route requests from clients with access to the VPC for the load balancer.
*
*
* The default is an Internet-facing load balancer.
* @see LoadBalancerSchemeEnum
*/
public LoadBalancerSchemeEnum scheme() {
return LoadBalancerSchemeEnum.fromValue(scheme);
}
/**
*
* The nodes of an Internet-facing load balancer have public IP addresses. The DNS name of an Internet-facing load
* balancer is publicly resolvable to the public IP addresses of the nodes. Therefore, Internet-facing load
* balancers can route requests from clients over the internet.
*
*
* The nodes of an internal load balancer have only private IP addresses. The DNS name of an internal load balancer
* is publicly resolvable to the private IP addresses of the nodes. Therefore, internal load balancers can only
* route requests from clients with access to the VPC for the load balancer.
*
*
* The default is an Internet-facing load balancer.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #scheme} will
* return {@link LoadBalancerSchemeEnum#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available
* from {@link #schemeAsString}.
*
*
* @return The nodes of an Internet-facing load balancer have public IP addresses. The DNS name of an
* Internet-facing load balancer is publicly resolvable to the public IP addresses of the nodes. Therefore,
* Internet-facing load balancers can route requests from clients over the internet.
*
* The nodes of an internal load balancer have only private IP addresses. The DNS name of an internal load
* balancer is publicly resolvable to the private IP addresses of the nodes. Therefore, internal load
* balancers can only route requests from clients with access to the VPC for the load balancer.
*
*
* The default is an Internet-facing load balancer.
* @see LoadBalancerSchemeEnum
*/
public String schemeAsString() {
return scheme;
}
/**
*
* One or more tags to assign to the load balancer.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* @return One or more tags to assign to the load balancer.
*/
public List tags() {
return tags;
}
/**
*
* The type of load balancer. The default is application
.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #type} will return
* {@link LoadBalancerTypeEnum#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #typeAsString}.
*
*
* @return The type of load balancer. The default is application
.
* @see LoadBalancerTypeEnum
*/
public LoadBalancerTypeEnum type() {
return LoadBalancerTypeEnum.fromValue(type);
}
/**
*
* The type of load balancer. The default is application
.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #type} will return
* {@link LoadBalancerTypeEnum#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #typeAsString}.
*
*
* @return The type of load balancer. The default is application
.
* @see LoadBalancerTypeEnum
*/
public String typeAsString() {
return type;
}
/**
*
* [Application Load Balancers] The type of IP addresses used by the subnets for your load balancer. The possible
* values are ipv4
(for IPv4 addresses) and dualstack
(for IPv4 and IPv6 addresses).
* Internal load balancers must use ipv4
.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #ipAddressType}
* will return {@link IpAddressType#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #ipAddressTypeAsString}.
*
*
* @return [Application Load Balancers] The type of IP addresses used by the subnets for your load balancer. The
* possible values are ipv4
(for IPv4 addresses) and dualstack
(for IPv4 and IPv6
* addresses). Internal load balancers must use ipv4
.
* @see IpAddressType
*/
public IpAddressType ipAddressType() {
return IpAddressType.fromValue(ipAddressType);
}
/**
*
* [Application Load Balancers] The type of IP addresses used by the subnets for your load balancer. The possible
* values are ipv4
(for IPv4 addresses) and dualstack
(for IPv4 and IPv6 addresses).
* Internal load balancers must use ipv4
.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #ipAddressType}
* will return {@link IpAddressType#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #ipAddressTypeAsString}.
*
*
* @return [Application Load Balancers] The type of IP addresses used by the subnets for your load balancer. The
* possible values are ipv4
(for IPv4 addresses) and dualstack
(for IPv4 and IPv6
* addresses). Internal load balancers must use ipv4
.
* @see IpAddressType
*/
public String ipAddressTypeAsString() {
return ipAddressType;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(name());
hashCode = 31 * hashCode + Objects.hashCode(subnets());
hashCode = 31 * hashCode + Objects.hashCode(subnetMappings());
hashCode = 31 * hashCode + Objects.hashCode(securityGroups());
hashCode = 31 * hashCode + Objects.hashCode(schemeAsString());
hashCode = 31 * hashCode + Objects.hashCode(tags());
hashCode = 31 * hashCode + Objects.hashCode(typeAsString());
hashCode = 31 * hashCode + Objects.hashCode(ipAddressTypeAsString());
return hashCode;
}
@Override
public boolean equals(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof CreateLoadBalancerRequest)) {
return false;
}
CreateLoadBalancerRequest other = (CreateLoadBalancerRequest) obj;
return Objects.equals(name(), other.name()) && Objects.equals(subnets(), other.subnets())
&& Objects.equals(subnetMappings(), other.subnetMappings())
&& Objects.equals(securityGroups(), other.securityGroups())
&& Objects.equals(schemeAsString(), other.schemeAsString()) && Objects.equals(tags(), other.tags())
&& Objects.equals(typeAsString(), other.typeAsString())
&& Objects.equals(ipAddressTypeAsString(), other.ipAddressTypeAsString());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public String toString() {
return ToString.builder("CreateLoadBalancerRequest").add("Name", name()).add("Subnets", subnets())
.add("SubnetMappings", subnetMappings()).add("SecurityGroups", securityGroups()).add("Scheme", schemeAsString())
.add("Tags", tags()).add("Type", typeAsString()).add("IpAddressType", ipAddressTypeAsString()).build();
}
public Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "Name":
return Optional.ofNullable(clazz.cast(name()));
case "Subnets":
return Optional.ofNullable(clazz.cast(subnets()));
case "SubnetMappings":
return Optional.ofNullable(clazz.cast(subnetMappings()));
case "SecurityGroups":
return Optional.ofNullable(clazz.cast(securityGroups()));
case "Scheme":
return Optional.ofNullable(clazz.cast(schemeAsString()));
case "Tags":
return Optional.ofNullable(clazz.cast(tags()));
case "Type":
return Optional.ofNullable(clazz.cast(typeAsString()));
case "IpAddressType":
return Optional.ofNullable(clazz.cast(ipAddressTypeAsString()));
default:
return Optional.empty();
}
}
@Override
public List> sdkFields() {
return SDK_FIELDS;
}
private static Function