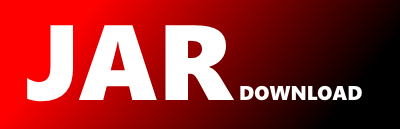
software.amazon.awssdk.services.elasticloadbalancingv2.model.RuleCondition Maven / Gradle / Ivy
Show all versions of elasticloadbalancingv2 Show documentation
/*
* Copyright 2014-2019 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.elasticloadbalancingv2.model;
import java.io.Serializable;
import java.util.Arrays;
import java.util.Collection;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.ListTrait;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.core.util.DefaultSdkAutoConstructList;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* Information about a condition for a rule.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class RuleCondition implements SdkPojo, Serializable, ToCopyableBuilder {
private static final SdkField FIELD_FIELD = SdkField. builder(MarshallingType.STRING)
.getter(getter(RuleCondition::field)).setter(setter(Builder::field))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Field").build()).build();
private static final SdkField> VALUES_FIELD = SdkField
.> builder(MarshallingType.LIST)
.getter(getter(RuleCondition::values))
.setter(setter(Builder::values))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Values").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.STRING)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField HOST_HEADER_CONFIG_FIELD = SdkField
. builder(MarshallingType.SDK_POJO).getter(getter(RuleCondition::hostHeaderConfig))
.setter(setter(Builder::hostHeaderConfig)).constructor(HostHeaderConditionConfig::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("HostHeaderConfig").build()).build();
private static final SdkField PATH_PATTERN_CONFIG_FIELD = SdkField
. builder(MarshallingType.SDK_POJO).getter(getter(RuleCondition::pathPatternConfig))
.setter(setter(Builder::pathPatternConfig)).constructor(PathPatternConditionConfig::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("PathPatternConfig").build()).build();
private static final SdkField HTTP_HEADER_CONFIG_FIELD = SdkField
. builder(MarshallingType.SDK_POJO).getter(getter(RuleCondition::httpHeaderConfig))
.setter(setter(Builder::httpHeaderConfig)).constructor(HttpHeaderConditionConfig::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("HttpHeaderConfig").build()).build();
private static final SdkField QUERY_STRING_CONFIG_FIELD = SdkField
. builder(MarshallingType.SDK_POJO).getter(getter(RuleCondition::queryStringConfig))
.setter(setter(Builder::queryStringConfig)).constructor(QueryStringConditionConfig::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("QueryStringConfig").build()).build();
private static final SdkField HTTP_REQUEST_METHOD_CONFIG_FIELD = SdkField
. builder(MarshallingType.SDK_POJO)
.getter(getter(RuleCondition::httpRequestMethodConfig)).setter(setter(Builder::httpRequestMethodConfig))
.constructor(HttpRequestMethodConditionConfig::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("HttpRequestMethodConfig").build())
.build();
private static final SdkField SOURCE_IP_CONFIG_FIELD = SdkField
. builder(MarshallingType.SDK_POJO).getter(getter(RuleCondition::sourceIpConfig))
.setter(setter(Builder::sourceIpConfig)).constructor(SourceIpConditionConfig::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("SourceIpConfig").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(FIELD_FIELD, VALUES_FIELD,
HOST_HEADER_CONFIG_FIELD, PATH_PATTERN_CONFIG_FIELD, HTTP_HEADER_CONFIG_FIELD, QUERY_STRING_CONFIG_FIELD,
HTTP_REQUEST_METHOD_CONFIG_FIELD, SOURCE_IP_CONFIG_FIELD));
private static final long serialVersionUID = 1L;
private final String field;
private final List values;
private final HostHeaderConditionConfig hostHeaderConfig;
private final PathPatternConditionConfig pathPatternConfig;
private final HttpHeaderConditionConfig httpHeaderConfig;
private final QueryStringConditionConfig queryStringConfig;
private final HttpRequestMethodConditionConfig httpRequestMethodConfig;
private final SourceIpConditionConfig sourceIpConfig;
private RuleCondition(BuilderImpl builder) {
this.field = builder.field;
this.values = builder.values;
this.hostHeaderConfig = builder.hostHeaderConfig;
this.pathPatternConfig = builder.pathPatternConfig;
this.httpHeaderConfig = builder.httpHeaderConfig;
this.queryStringConfig = builder.queryStringConfig;
this.httpRequestMethodConfig = builder.httpRequestMethodConfig;
this.sourceIpConfig = builder.sourceIpConfig;
}
/**
*
* The field in the HTTP request. The following are the possible values:
*
*
* -
*
* http-header
*
*
* -
*
* http-request-method
*
*
* -
*
* host-header
*
*
* -
*
* path-pattern
*
*
* -
*
* query-string
*
*
* -
*
* source-ip
*
*
*
*
* @return The field in the HTTP request. The following are the possible values:
*
* -
*
* http-header
*
*
* -
*
* http-request-method
*
*
* -
*
* host-header
*
*
* -
*
* path-pattern
*
*
* -
*
* query-string
*
*
* -
*
* source-ip
*
*
*/
public String field() {
return field;
}
/**
*
* The condition value. You can use Values
if the rule contains only host-header
and
* path-pattern
conditions. Otherwise, you can use HostHeaderConfig
for
* host-header
conditions and PathPatternConfig
for path-pattern
conditions.
*
*
* If Field
is host-header
, you can specify a single host name (for example,
* my.example.com). A host name is case insensitive, can be up to 128 characters in length, and can contain any of
* the following characters.
*
*
* -
*
* A-Z, a-z, 0-9
*
*
* -
*
* - .
*
*
* -
*
* * (matches 0 or more characters)
*
*
* -
*
* ? (matches exactly 1 character)
*
*
*
*
* If Field
is path-pattern
, you can specify a single path pattern (for example, /img/*).
* A path pattern is case-sensitive, can be up to 128 characters in length, and can contain any of the following
* characters.
*
*
* -
*
* A-Z, a-z, 0-9
*
*
* -
*
* _ - . $ / ~ " ' @ : +
*
*
* -
*
* & (using &)
*
*
* -
*
* * (matches 0 or more characters)
*
*
* -
*
* ? (matches exactly 1 character)
*
*
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* @return The condition value. You can use Values
if the rule contains only host-header
* and path-pattern
conditions. Otherwise, you can use HostHeaderConfig
for
* host-header
conditions and PathPatternConfig
for path-pattern
* conditions.
*
* If Field
is host-header
, you can specify a single host name (for example,
* my.example.com). A host name is case insensitive, can be up to 128 characters in length, and can contain
* any of the following characters.
*
*
* -
*
* A-Z, a-z, 0-9
*
*
* -
*
* - .
*
*
* -
*
* * (matches 0 or more characters)
*
*
* -
*
* ? (matches exactly 1 character)
*
*
*
*
* If Field
is path-pattern
, you can specify a single path pattern (for example,
* /img/*). A path pattern is case-sensitive, can be up to 128 characters in length, and can contain any of
* the following characters.
*
*
* -
*
* A-Z, a-z, 0-9
*
*
* -
*
* _ - . $ / ~ " ' @ : +
*
*
* -
*
* & (using &)
*
*
* -
*
* * (matches 0 or more characters)
*
*
* -
*
* ? (matches exactly 1 character)
*
*
*/
public List values() {
return values;
}
/**
*
* Information for a host header condition. Specify only when Field
is host-header
.
*
*
* @return Information for a host header condition. Specify only when Field
is host-header
* .
*/
public HostHeaderConditionConfig hostHeaderConfig() {
return hostHeaderConfig;
}
/**
*
* Information for a path pattern condition. Specify only when Field
is path-pattern
.
*
*
* @return Information for a path pattern condition. Specify only when Field
is
* path-pattern
.
*/
public PathPatternConditionConfig pathPatternConfig() {
return pathPatternConfig;
}
/**
*
* Information for an HTTP header condition. Specify only when Field
is http-header
.
*
*
* @return Information for an HTTP header condition. Specify only when Field
is
* http-header
.
*/
public HttpHeaderConditionConfig httpHeaderConfig() {
return httpHeaderConfig;
}
/**
*
* Information for a query string condition. Specify only when Field
is query-string
.
*
*
* @return Information for a query string condition. Specify only when Field
is
* query-string
.
*/
public QueryStringConditionConfig queryStringConfig() {
return queryStringConfig;
}
/**
*
* Information for an HTTP method condition. Specify only when Field
is
* http-request-method
.
*
*
* @return Information for an HTTP method condition. Specify only when Field
is
* http-request-method
.
*/
public HttpRequestMethodConditionConfig httpRequestMethodConfig() {
return httpRequestMethodConfig;
}
/**
*
* Information for a source IP condition. Specify only when Field
is source-ip
.
*
*
* @return Information for a source IP condition. Specify only when Field
is source-ip
.
*/
public SourceIpConditionConfig sourceIpConfig() {
return sourceIpConfig;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(field());
hashCode = 31 * hashCode + Objects.hashCode(values());
hashCode = 31 * hashCode + Objects.hashCode(hostHeaderConfig());
hashCode = 31 * hashCode + Objects.hashCode(pathPatternConfig());
hashCode = 31 * hashCode + Objects.hashCode(httpHeaderConfig());
hashCode = 31 * hashCode + Objects.hashCode(queryStringConfig());
hashCode = 31 * hashCode + Objects.hashCode(httpRequestMethodConfig());
hashCode = 31 * hashCode + Objects.hashCode(sourceIpConfig());
return hashCode;
}
@Override
public boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof RuleCondition)) {
return false;
}
RuleCondition other = (RuleCondition) obj;
return Objects.equals(field(), other.field()) && Objects.equals(values(), other.values())
&& Objects.equals(hostHeaderConfig(), other.hostHeaderConfig())
&& Objects.equals(pathPatternConfig(), other.pathPatternConfig())
&& Objects.equals(httpHeaderConfig(), other.httpHeaderConfig())
&& Objects.equals(queryStringConfig(), other.queryStringConfig())
&& Objects.equals(httpRequestMethodConfig(), other.httpRequestMethodConfig())
&& Objects.equals(sourceIpConfig(), other.sourceIpConfig());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public String toString() {
return ToString.builder("RuleCondition").add("Field", field()).add("Values", values())
.add("HostHeaderConfig", hostHeaderConfig()).add("PathPatternConfig", pathPatternConfig())
.add("HttpHeaderConfig", httpHeaderConfig()).add("QueryStringConfig", queryStringConfig())
.add("HttpRequestMethodConfig", httpRequestMethodConfig()).add("SourceIpConfig", sourceIpConfig()).build();
}
public Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "Field":
return Optional.ofNullable(clazz.cast(field()));
case "Values":
return Optional.ofNullable(clazz.cast(values()));
case "HostHeaderConfig":
return Optional.ofNullable(clazz.cast(hostHeaderConfig()));
case "PathPatternConfig":
return Optional.ofNullable(clazz.cast(pathPatternConfig()));
case "HttpHeaderConfig":
return Optional.ofNullable(clazz.cast(httpHeaderConfig()));
case "QueryStringConfig":
return Optional.ofNullable(clazz.cast(queryStringConfig()));
case "HttpRequestMethodConfig":
return Optional.ofNullable(clazz.cast(httpRequestMethodConfig()));
case "SourceIpConfig":
return Optional.ofNullable(clazz.cast(sourceIpConfig()));
default:
return Optional.empty();
}
}
@Override
public List> sdkFields() {
return SDK_FIELDS;
}
private static Function