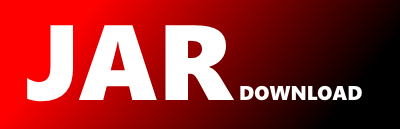
software.amazon.awssdk.services.elasticsearch.model.ChangeProgressStatusDetails Maven / Gradle / Ivy
Show all versions of elasticsearch Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.elasticsearch.model;
import java.io.Serializable;
import java.time.Instant;
import java.util.Arrays;
import java.util.Collection;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import java.util.stream.Collectors;
import java.util.stream.Stream;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.ListTrait;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.core.util.DefaultSdkAutoConstructList;
import software.amazon.awssdk.core.util.SdkAutoConstructList;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* The progress details of a specific domain configuration change.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class ChangeProgressStatusDetails implements SdkPojo, Serializable,
ToCopyableBuilder {
private static final SdkField CHANGE_ID_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("ChangeId").getter(getter(ChangeProgressStatusDetails::changeId)).setter(setter(Builder::changeId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ChangeId").build()).build();
private static final SdkField START_TIME_FIELD = SdkField. builder(MarshallingType.INSTANT)
.memberName("StartTime").getter(getter(ChangeProgressStatusDetails::startTime)).setter(setter(Builder::startTime))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("StartTime").build()).build();
private static final SdkField STATUS_FIELD = SdkField. builder(MarshallingType.STRING).memberName("Status")
.getter(getter(ChangeProgressStatusDetails::statusAsString)).setter(setter(Builder::status))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Status").build()).build();
private static final SdkField> PENDING_PROPERTIES_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("PendingProperties")
.getter(getter(ChangeProgressStatusDetails::pendingProperties))
.setter(setter(Builder::pendingProperties))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("PendingProperties").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.STRING)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField> COMPLETED_PROPERTIES_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("CompletedProperties")
.getter(getter(ChangeProgressStatusDetails::completedProperties))
.setter(setter(Builder::completedProperties))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("CompletedProperties").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.STRING)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField TOTAL_NUMBER_OF_STAGES_FIELD = SdkField. builder(MarshallingType.INTEGER)
.memberName("TotalNumberOfStages").getter(getter(ChangeProgressStatusDetails::totalNumberOfStages))
.setter(setter(Builder::totalNumberOfStages))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("TotalNumberOfStages").build())
.build();
private static final SdkField> CHANGE_PROGRESS_STAGES_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("ChangeProgressStages")
.getter(getter(ChangeProgressStatusDetails::changeProgressStages))
.setter(setter(Builder::changeProgressStages))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ChangeProgressStages").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(ChangeProgressStage::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(CHANGE_ID_FIELD,
START_TIME_FIELD, STATUS_FIELD, PENDING_PROPERTIES_FIELD, COMPLETED_PROPERTIES_FIELD, TOTAL_NUMBER_OF_STAGES_FIELD,
CHANGE_PROGRESS_STAGES_FIELD));
private static final long serialVersionUID = 1L;
private final String changeId;
private final Instant startTime;
private final String status;
private final List pendingProperties;
private final List completedProperties;
private final Integer totalNumberOfStages;
private final List changeProgressStages;
private ChangeProgressStatusDetails(BuilderImpl builder) {
this.changeId = builder.changeId;
this.startTime = builder.startTime;
this.status = builder.status;
this.pendingProperties = builder.pendingProperties;
this.completedProperties = builder.completedProperties;
this.totalNumberOfStages = builder.totalNumberOfStages;
this.changeProgressStages = builder.changeProgressStages;
}
/**
*
* The unique change identifier associated with a specific domain configuration change.
*
*
* @return The unique change identifier associated with a specific domain configuration change.
*/
public final String changeId() {
return changeId;
}
/**
*
* The time at which the configuration change is made on the domain.
*
*
* @return The time at which the configuration change is made on the domain.
*/
public final Instant startTime() {
return startTime;
}
/**
*
* The overall status of the domain configuration change. This field can take the following values:
* PENDING
, PROCESSING
, COMPLETED
and FAILED
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #status} will
* return {@link OverallChangeStatus#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available
* from {@link #statusAsString}.
*
*
* @return The overall status of the domain configuration change. This field can take the following values:
* PENDING
, PROCESSING
, COMPLETED
and FAILED
* @see OverallChangeStatus
*/
public final OverallChangeStatus status() {
return OverallChangeStatus.fromValue(status);
}
/**
*
* The overall status of the domain configuration change. This field can take the following values:
* PENDING
, PROCESSING
, COMPLETED
and FAILED
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #status} will
* return {@link OverallChangeStatus#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available
* from {@link #statusAsString}.
*
*
* @return The overall status of the domain configuration change. This field can take the following values:
* PENDING
, PROCESSING
, COMPLETED
and FAILED
* @see OverallChangeStatus
*/
public final String statusAsString() {
return status;
}
/**
* For responses, this returns true if the service returned a value for the PendingProperties property. This DOES
* NOT check that the value is non-empty (for which, you should check the {@code isEmpty()} method on the property).
* This is useful because the SDK will never return a null collection or map, but you may need to differentiate
* between the service returning nothing (or null) and the service returning an empty collection or map. For
* requests, this returns true if a value for the property was specified in the request builder, and false if a
* value was not specified.
*/
public final boolean hasPendingProperties() {
return pendingProperties != null && !(pendingProperties instanceof SdkAutoConstructList);
}
/**
*
* The list of properties involved in the domain configuration change that are still in pending.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasPendingProperties} method.
*
*
* @return The list of properties involved in the domain configuration change that are still in pending.
*/
public final List pendingProperties() {
return pendingProperties;
}
/**
* For responses, this returns true if the service returned a value for the CompletedProperties property. This DOES
* NOT check that the value is non-empty (for which, you should check the {@code isEmpty()} method on the property).
* This is useful because the SDK will never return a null collection or map, but you may need to differentiate
* between the service returning nothing (or null) and the service returning an empty collection or map. For
* requests, this returns true if a value for the property was specified in the request builder, and false if a
* value was not specified.
*/
public final boolean hasCompletedProperties() {
return completedProperties != null && !(completedProperties instanceof SdkAutoConstructList);
}
/**
*
* The list of properties involved in the domain configuration change that are completed.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasCompletedProperties} method.
*
*
* @return The list of properties involved in the domain configuration change that are completed.
*/
public final List completedProperties() {
return completedProperties;
}
/**
*
* The total number of stages required for the configuration change.
*
*
* @return The total number of stages required for the configuration change.
*/
public final Integer totalNumberOfStages() {
return totalNumberOfStages;
}
/**
* For responses, this returns true if the service returned a value for the ChangeProgressStages property. This DOES
* NOT check that the value is non-empty (for which, you should check the {@code isEmpty()} method on the property).
* This is useful because the SDK will never return a null collection or map, but you may need to differentiate
* between the service returning nothing (or null) and the service returning an empty collection or map. For
* requests, this returns true if a value for the property was specified in the request builder, and false if a
* value was not specified.
*/
public final boolean hasChangeProgressStages() {
return changeProgressStages != null && !(changeProgressStages instanceof SdkAutoConstructList);
}
/**
*
* The specific stages that the domain is going through to perform the configuration change.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasChangeProgressStages} method.
*
*
* @return The specific stages that the domain is going through to perform the configuration change.
*/
public final List changeProgressStages() {
return changeProgressStages;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(changeId());
hashCode = 31 * hashCode + Objects.hashCode(startTime());
hashCode = 31 * hashCode + Objects.hashCode(statusAsString());
hashCode = 31 * hashCode + Objects.hashCode(hasPendingProperties() ? pendingProperties() : null);
hashCode = 31 * hashCode + Objects.hashCode(hasCompletedProperties() ? completedProperties() : null);
hashCode = 31 * hashCode + Objects.hashCode(totalNumberOfStages());
hashCode = 31 * hashCode + Objects.hashCode(hasChangeProgressStages() ? changeProgressStages() : null);
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof ChangeProgressStatusDetails)) {
return false;
}
ChangeProgressStatusDetails other = (ChangeProgressStatusDetails) obj;
return Objects.equals(changeId(), other.changeId()) && Objects.equals(startTime(), other.startTime())
&& Objects.equals(statusAsString(), other.statusAsString())
&& hasPendingProperties() == other.hasPendingProperties()
&& Objects.equals(pendingProperties(), other.pendingProperties())
&& hasCompletedProperties() == other.hasCompletedProperties()
&& Objects.equals(completedProperties(), other.completedProperties())
&& Objects.equals(totalNumberOfStages(), other.totalNumberOfStages())
&& hasChangeProgressStages() == other.hasChangeProgressStages()
&& Objects.equals(changeProgressStages(), other.changeProgressStages());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("ChangeProgressStatusDetails").add("ChangeId", changeId()).add("StartTime", startTime())
.add("Status", statusAsString()).add("PendingProperties", hasPendingProperties() ? pendingProperties() : null)
.add("CompletedProperties", hasCompletedProperties() ? completedProperties() : null)
.add("TotalNumberOfStages", totalNumberOfStages())
.add("ChangeProgressStages", hasChangeProgressStages() ? changeProgressStages() : null).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "ChangeId":
return Optional.ofNullable(clazz.cast(changeId()));
case "StartTime":
return Optional.ofNullable(clazz.cast(startTime()));
case "Status":
return Optional.ofNullable(clazz.cast(statusAsString()));
case "PendingProperties":
return Optional.ofNullable(clazz.cast(pendingProperties()));
case "CompletedProperties":
return Optional.ofNullable(clazz.cast(completedProperties()));
case "TotalNumberOfStages":
return Optional.ofNullable(clazz.cast(totalNumberOfStages()));
case "ChangeProgressStages":
return Optional.ofNullable(clazz.cast(changeProgressStages()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
private static Function