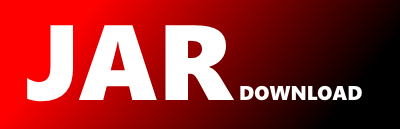
software.amazon.awssdk.services.elasticsearch.model.ServiceSoftwareOptions Maven / Gradle / Ivy
Show all versions of elasticsearch Show documentation
/* * Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved. * * Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with * the License. A copy of the License is located at * * http://aws.amazon.com/apache2.0 * * or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR * CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions * and limitations under the License. */ package software.amazon.awssdk.services.elasticsearch.model; import java.io.Serializable; import java.time.Instant; import java.util.Arrays; import java.util.Collections; import java.util.List; import java.util.Objects; import java.util.Optional; import java.util.function.BiConsumer; import java.util.function.Function; import software.amazon.awssdk.annotations.Generated; import software.amazon.awssdk.core.SdkField; import software.amazon.awssdk.core.SdkPojo; import software.amazon.awssdk.core.protocol.MarshallLocation; import software.amazon.awssdk.core.protocol.MarshallingType; import software.amazon.awssdk.core.traits.LocationTrait; import software.amazon.awssdk.utils.ToString; import software.amazon.awssdk.utils.builder.CopyableBuilder; import software.amazon.awssdk.utils.builder.ToCopyableBuilder; /** *
if you are able to update you service software version.* The current options of an Elasticsearch domain service software options. *
*/ @Generated("software.amazon.awssdk:codegen") public final class ServiceSoftwareOptions implements SdkPojo, Serializable, ToCopyableBuilder{ private static final SdkField CURRENT_VERSION_FIELD = SdkField. builder(MarshallingType.STRING) .memberName("CurrentVersion").getter(getter(ServiceSoftwareOptions::currentVersion)) .setter(setter(Builder::currentVersion)) .traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("CurrentVersion").build()).build(); private static final SdkField NEW_VERSION_FIELD = SdkField. builder(MarshallingType.STRING) .memberName("NewVersion").getter(getter(ServiceSoftwareOptions::newVersion)).setter(setter(Builder::newVersion)) .traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("NewVersion").build()).build(); private static final SdkField UPDATE_AVAILABLE_FIELD = SdkField. builder(MarshallingType.BOOLEAN) .memberName("UpdateAvailable").getter(getter(ServiceSoftwareOptions::updateAvailable)) .setter(setter(Builder::updateAvailable)) .traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("UpdateAvailable").build()).build(); private static final SdkField CANCELLABLE_FIELD = SdkField. builder(MarshallingType.BOOLEAN) .memberName("Cancellable").getter(getter(ServiceSoftwareOptions::cancellable)).setter(setter(Builder::cancellable)) .traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Cancellable").build()).build(); private static final SdkField UPDATE_STATUS_FIELD = SdkField. builder(MarshallingType.STRING) .memberName("UpdateStatus").getter(getter(ServiceSoftwareOptions::updateStatusAsString)) .setter(setter(Builder::updateStatus)) .traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("UpdateStatus").build()).build(); private static final SdkField DESCRIPTION_FIELD = SdkField. builder(MarshallingType.STRING) .memberName("Description").getter(getter(ServiceSoftwareOptions::description)).setter(setter(Builder::description)) .traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Description").build()).build(); private static final SdkField AUTOMATED_UPDATE_DATE_FIELD = SdkField. builder(MarshallingType.INSTANT) .memberName("AutomatedUpdateDate").getter(getter(ServiceSoftwareOptions::automatedUpdateDate)) .setter(setter(Builder::automatedUpdateDate)) .traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("AutomatedUpdateDate").build()) .build(); private static final SdkField OPTIONAL_DEPLOYMENT_FIELD = SdkField. builder(MarshallingType.BOOLEAN) .memberName("OptionalDeployment").getter(getter(ServiceSoftwareOptions::optionalDeployment)) .setter(setter(Builder::optionalDeployment)) .traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("OptionalDeployment").build()) .build(); private static final List > SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(CURRENT_VERSION_FIELD, NEW_VERSION_FIELD, UPDATE_AVAILABLE_FIELD, CANCELLABLE_FIELD, UPDATE_STATUS_FIELD, DESCRIPTION_FIELD, AUTOMATED_UPDATE_DATE_FIELD, OPTIONAL_DEPLOYMENT_FIELD)); private static final long serialVersionUID = 1L; private final String currentVersion; private final String newVersion; private final Boolean updateAvailable; private final Boolean cancellable; private final String updateStatus; private final String description; private final Instant automatedUpdateDate; private final Boolean optionalDeployment; private ServiceSoftwareOptions(BuilderImpl builder) { this.currentVersion = builder.currentVersion; this.newVersion = builder.newVersion; this.updateAvailable = builder.updateAvailable; this.cancellable = builder.cancellable; this.updateStatus = builder.updateStatus; this.description = builder.description; this.automatedUpdateDate = builder.automatedUpdateDate; this.optionalDeployment = builder.optionalDeployment; } /** * * The current service software version that is present on the domain. *
* * @return The current service software version that is present on the domain. */ public final String currentVersion() { return currentVersion; } /** ** The new service software version if one is available. *
* * @return The new service software version if one is available. */ public final String newVersion() { return newVersion; } /** **
* * @return TrueTrue
if you are able to update you service software version.False
if you are not able * to update your service software version. *False */ public final Boolean updateAvailable() { return updateAvailable; } /** *
if you are able to cancel your service software version update.*
* * @return TrueTrue
if you are able to cancel your service software version update.False
if you are * not able to cancel your service software version. *False */ public final Boolean cancellable() { return cancellable; } /** *
if a service software is never automatically updated.* The status of your service software update. This field can take the following values:
*ELIGIBLE
, *PENDING_UPDATE
,IN_PROGRESS
,COMPLETED
, andNOT_ELIGIBLE
. ** If the service returns an enum value that is not available in the current SDK version, {@link #updateStatus} will * return {@link DeploymentStatus#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from * {@link #updateStatusAsString}. *
* * @return The status of your service software update. This field can take the following values: *ELIGIBLE
,PENDING_UPDATE
,IN_PROGRESS
,COMPLETED
, and *NOT_ELIGIBLE
. * @see DeploymentStatus */ public final DeploymentStatus updateStatus() { return DeploymentStatus.fromValue(updateStatus); } /** ** The status of your service software update. This field can take the following values:
*ELIGIBLE
, *PENDING_UPDATE
,IN_PROGRESS
,COMPLETED
, andNOT_ELIGIBLE
. ** If the service returns an enum value that is not available in the current SDK version, {@link #updateStatus} will * return {@link DeploymentStatus#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from * {@link #updateStatusAsString}. *
* * @return The status of your service software update. This field can take the following values: *ELIGIBLE
,PENDING_UPDATE
,IN_PROGRESS
,COMPLETED
, and *NOT_ELIGIBLE
. * @see DeploymentStatus */ public final String updateStatusAsString() { return updateStatus; } /** ** The description of the
* * @return The description of theUpdateStatus
. *UpdateStatus
. */ public final String description() { return description; } /** ** Timestamp, in Epoch time, until which you can manually request a service software update. After this date, we * automatically update your service software. *
* * @return Timestamp, in Epoch time, until which you can manually request a service software update. After this * date, we automatically update your service software. */ public final Instant automatedUpdateDate() { return automatedUpdateDate; } /** **
* * @return TrueTrue
if a service software is never automatically updated.False
if a service software * is automatically updated afterAutomatedUpdateDate
. *False
if a service * software is automatically updated afterAutomatedUpdateDate */ public final Boolean optionalDeployment() { return optionalDeployment; } @Override public Builder toBuilder() { return new BuilderImpl(this); } public static Builder builder() { return new BuilderImpl(); } public static Class extends Builder> serializableBuilderClass() { return BuilderImpl.class; } @Override public final int hashCode() { int hashCode = 1; hashCode = 31 * hashCode + Objects.hashCode(currentVersion()); hashCode = 31 * hashCode + Objects.hashCode(newVersion()); hashCode = 31 * hashCode + Objects.hashCode(updateAvailable()); hashCode = 31 * hashCode + Objects.hashCode(cancellable()); hashCode = 31 * hashCode + Objects.hashCode(updateStatusAsString()); hashCode = 31 * hashCode + Objects.hashCode(description()); hashCode = 31 * hashCode + Objects.hashCode(automatedUpdateDate()); hashCode = 31 * hashCode + Objects.hashCode(optionalDeployment()); return hashCode; } @Override public final boolean equals(Object obj) { return equalsBySdkFields(obj); } @Override public final boolean equalsBySdkFields(Object obj) { if (this == obj) { return true; } if (obj == null) { return false; } if (!(obj instanceof ServiceSoftwareOptions)) { return false; } ServiceSoftwareOptions other = (ServiceSoftwareOptions) obj; return Objects.equals(currentVersion(), other.currentVersion()) && Objects.equals(newVersion(), other.newVersion()) && Objects.equals(updateAvailable(), other.updateAvailable()) && Objects.equals(cancellable(), other.cancellable()) && Objects.equals(updateStatusAsString(), other.updateStatusAsString()) && Objects.equals(description(), other.description()) && Objects.equals(automatedUpdateDate(), other.automatedUpdateDate()) && Objects.equals(optionalDeployment(), other.optionalDeployment()); } /** * Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be * redacted from this string using a placeholder value. */ @Override public final String toString() { return ToString.builder("ServiceSoftwareOptions").add("CurrentVersion", currentVersion()).add("NewVersion", newVersion()) .add("UpdateAvailable", updateAvailable()).add("Cancellable", cancellable()) .add("UpdateStatus", updateStatusAsString()).add("Description", description()) .add("AutomatedUpdateDate", automatedUpdateDate()).add("OptionalDeployment", optionalDeployment()).build(); } public final
if you are able to update you service software version.Optional getValueForField(String fieldName, Class clazz) { switch (fieldName) { case "CurrentVersion": return Optional.ofNullable(clazz.cast(currentVersion())); case "NewVersion": return Optional.ofNullable(clazz.cast(newVersion())); case "UpdateAvailable": return Optional.ofNullable(clazz.cast(updateAvailable())); case "Cancellable": return Optional.ofNullable(clazz.cast(cancellable())); case "UpdateStatus": return Optional.ofNullable(clazz.cast(updateStatusAsString())); case "Description": return Optional.ofNullable(clazz.cast(description())); case "AutomatedUpdateDate": return Optional.ofNullable(clazz.cast(automatedUpdateDate())); case "OptionalDeployment": return Optional.ofNullable(clazz.cast(optionalDeployment())); default: return Optional.empty(); } } @Override public final List > sdkFields() { return SDK_FIELDS; } private static Function False * @return Returns a reference to this object so that method calls can be chained together. */ Builder updateAvailable(Boolean updateAvailable); /** *
if you are able to cancel your service software version update.*
* * @param cancellable * TrueTrue
if you are able to cancel your service software version update.False
if you * are not able to cancel your service software version. *False * @return Returns a reference to this object so that method calls can be chained together. */ Builder cancellable(Boolean cancellable); /** *
if a service software is never automatically updated.* The status of your service software update. This field can take the following values:
* * @param updateStatus * The status of your service software update. This field can take the following values: *ELIGIBLE
, *PENDING_UPDATE
,IN_PROGRESS
,COMPLETED
, andNOT_ELIGIBLE
. *ELIGIBLE
,PENDING_UPDATE
,IN_PROGRESS
,COMPLETED
, * andNOT_ELIGIBLE
. * @see DeploymentStatus * @return Returns a reference to this object so that method calls can be chained together. * @see DeploymentStatus */ Builder updateStatus(String updateStatus); /** ** The status of your service software update. This field can take the following values:
* * @param updateStatus * The status of your service software update. This field can take the following values: *ELIGIBLE
, *PENDING_UPDATE
,IN_PROGRESS
,COMPLETED
, andNOT_ELIGIBLE
. *ELIGIBLE
,PENDING_UPDATE
,IN_PROGRESS
,COMPLETED
, * andNOT_ELIGIBLE
. * @see DeploymentStatus * @return Returns a reference to this object so that method calls can be chained together. * @see DeploymentStatus */ Builder updateStatus(DeploymentStatus updateStatus); /** ** The description of the
* * @param description * The description of theUpdateStatus
. *UpdateStatus
. * @return Returns a reference to this object so that method calls can be chained together. */ Builder description(String description); /** ** Timestamp, in Epoch time, until which you can manually request a service software update. After this date, we * automatically update your service software. *
* * @param automatedUpdateDate * Timestamp, in Epoch time, until which you can manually request a service software update. After this * date, we automatically update your service software. * @return Returns a reference to this object so that method calls can be chained together. */ Builder automatedUpdateDate(Instant automatedUpdateDate); /** **
* * @param optionalDeployment * TrueTrue
if a service software is never automatically updated.False
if a service * software is automatically updated afterAutomatedUpdateDate
. *False
if a service * software is automatically updated afterAutomatedUpdateDate * @return Returns a reference to this object so that method calls can be chained together. */ Builder optionalDeployment(Boolean optionalDeployment); } static final class BuilderImpl implements Builder { private String currentVersion; private String newVersion; private Boolean updateAvailable; private Boolean cancellable; private String updateStatus; private String description; private Instant automatedUpdateDate; private Boolean optionalDeployment; private BuilderImpl() { } private BuilderImpl(ServiceSoftwareOptions model) { currentVersion(model.currentVersion); newVersion(model.newVersion); updateAvailable(model.updateAvailable); cancellable(model.cancellable); updateStatus(model.updateStatus); description(model.description); automatedUpdateDate(model.automatedUpdateDate); optionalDeployment(model.optionalDeployment); } public final String getCurrentVersion() { return currentVersion; } public final void setCurrentVersion(String currentVersion) { this.currentVersion = currentVersion; } @Override public final Builder currentVersion(String currentVersion) { this.currentVersion = currentVersion; return this; } public final String getNewVersion() { return newVersion; } public final void setNewVersion(String newVersion) { this.newVersion = newVersion; } @Override public final Builder newVersion(String newVersion) { this.newVersion = newVersion; return this; } public final Boolean getUpdateAvailable() { return updateAvailable; } public final void setUpdateAvailable(Boolean updateAvailable) { this.updateAvailable = updateAvailable; } @Override public final Builder updateAvailable(Boolean updateAvailable) { this.updateAvailable = updateAvailable; return this; } public final Boolean getCancellable() { return cancellable; } public final void setCancellable(Boolean cancellable) { this.cancellable = cancellable; } @Override public final Builder cancellable(Boolean cancellable) { this.cancellable = cancellable; return this; } public final String getUpdateStatus() { return updateStatus; } public final void setUpdateStatus(String updateStatus) { this.updateStatus = updateStatus; } @Override public final Builder updateStatus(String updateStatus) { this.updateStatus = updateStatus; return this; } @Override public final Builder updateStatus(DeploymentStatus updateStatus) { this.updateStatus(updateStatus == null ? null : updateStatus.toString()); return this; } public final String getDescription() { return description; } public final void setDescription(String description) { this.description = description; } @Override public final Builder description(String description) { this.description = description; return this; } public final Instant getAutomatedUpdateDate() { return automatedUpdateDate; } public final void setAutomatedUpdateDate(Instant automatedUpdateDate) { this.automatedUpdateDate = automatedUpdateDate; } @Override public final Builder automatedUpdateDate(Instant automatedUpdateDate) { this.automatedUpdateDate = automatedUpdateDate; return this; } public final Boolean getOptionalDeployment() { return optionalDeployment; } public final void setOptionalDeployment(Boolean optionalDeployment) { this.optionalDeployment = optionalDeployment; } @Override public final Builder optionalDeployment(Boolean optionalDeployment) { this.optionalDeployment = optionalDeployment; return this; } @Override public ServiceSoftwareOptions build() { return new ServiceSoftwareOptions(this); } @Override public List
> sdkFields() { return SDK_FIELDS; } } }