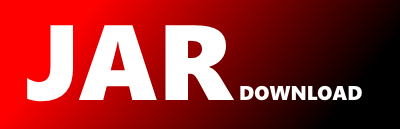
software.amazon.awssdk.services.elastictranscoder.model.AudioParameters Maven / Gradle / Ivy
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.elastictranscoder.model;
import java.beans.Transient;
import java.io.Serializable;
import java.util.Arrays;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* Parameters required for transcoding audio.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class AudioParameters implements SdkPojo, Serializable, ToCopyableBuilder {
private static final SdkField CODEC_FIELD = SdkField. builder(MarshallingType.STRING).memberName("Codec")
.getter(getter(AudioParameters::codec)).setter(setter(Builder::codec))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Codec").build()).build();
private static final SdkField SAMPLE_RATE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("SampleRate").getter(getter(AudioParameters::sampleRate)).setter(setter(Builder::sampleRate))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("SampleRate").build()).build();
private static final SdkField BIT_RATE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("BitRate").getter(getter(AudioParameters::bitRate)).setter(setter(Builder::bitRate))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("BitRate").build()).build();
private static final SdkField CHANNELS_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("Channels").getter(getter(AudioParameters::channels)).setter(setter(Builder::channels))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Channels").build()).build();
private static final SdkField AUDIO_PACKING_MODE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("AudioPackingMode").getter(getter(AudioParameters::audioPackingMode))
.setter(setter(Builder::audioPackingMode))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("AudioPackingMode").build()).build();
private static final SdkField CODEC_OPTIONS_FIELD = SdkField
. builder(MarshallingType.SDK_POJO).memberName("CodecOptions")
.getter(getter(AudioParameters::codecOptions)).setter(setter(Builder::codecOptions))
.constructor(AudioCodecOptions::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("CodecOptions").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(CODEC_FIELD,
SAMPLE_RATE_FIELD, BIT_RATE_FIELD, CHANNELS_FIELD, AUDIO_PACKING_MODE_FIELD, CODEC_OPTIONS_FIELD));
private static final long serialVersionUID = 1L;
private final String codec;
private final String sampleRate;
private final String bitRate;
private final String channels;
private final String audioPackingMode;
private final AudioCodecOptions codecOptions;
private AudioParameters(BuilderImpl builder) {
this.codec = builder.codec;
this.sampleRate = builder.sampleRate;
this.bitRate = builder.bitRate;
this.channels = builder.channels;
this.audioPackingMode = builder.audioPackingMode;
this.codecOptions = builder.codecOptions;
}
/**
*
* The audio codec for the output file. Valid values include aac
, flac
, mp2
,
* mp3
, pcm
, and vorbis
.
*
*
* @return The audio codec for the output file. Valid values include aac
, flac
,
* mp2
, mp3
, pcm
, and vorbis
.
*/
public final String codec() {
return codec;
}
/**
*
* The sample rate of the audio stream in the output file, in Hertz. Valid values include:
*
*
* auto
, 22050
, 32000
, 44100
, 48000
,
* 96000
*
*
* If you specify auto
, Elastic Transcoder automatically detects the sample rate.
*
*
* @return The sample rate of the audio stream in the output file, in Hertz. Valid values include:
*
* auto
, 22050
, 32000
, 44100
, 48000
,
* 96000
*
*
* If you specify auto
, Elastic Transcoder automatically detects the sample rate.
*/
public final String sampleRate() {
return sampleRate;
}
/**
*
* The bit rate of the audio stream in the output file, in kilobits/second. Enter an integer between 64 and 320,
* inclusive.
*
*
* @return The bit rate of the audio stream in the output file, in kilobits/second. Enter an integer between 64 and
* 320, inclusive.
*/
public final String bitRate() {
return bitRate;
}
/**
*
* The number of audio channels in the output file. The following values are valid:
*
*
* auto
, 0
, 1
, 2
*
*
* One channel carries the information played by a single speaker. For example, a stereo track with two channels
* sends one channel to the left speaker, and the other channel to the right speaker. The output channels are
* organized into tracks. If you want Elastic Transcoder to automatically detect the number of audio channels in the
* input file and use that value for the output file, select auto
.
*
*
* The output of a specific channel value and inputs are as follows:
*
*
* -
*
* auto
channel specified, with any input: Pass through up to eight input channels.
*
*
* -
*
* 0
channels specified, with any input: Audio omitted from the output.
*
*
* -
*
* 1
channel specified, with at least one input channel: Mono sound.
*
*
* -
*
* 2
channels specified, with any input: Two identical mono channels or stereo. For more
* information about tracks, see Audio:AudioPackingMode.
*
*
*
*
* For more information about how Elastic Transcoder organizes channels and tracks, see
* Audio:AudioPackingMode
.
*
*
* @return The number of audio channels in the output file. The following values are valid:
*
* auto
, 0
, 1
, 2
*
*
* One channel carries the information played by a single speaker. For example, a stereo track with two
* channels sends one channel to the left speaker, and the other channel to the right speaker. The output
* channels are organized into tracks. If you want Elastic Transcoder to automatically detect the number of
* audio channels in the input file and use that value for the output file, select auto
.
*
*
* The output of a specific channel value and inputs are as follows:
*
*
* -
*
* auto
channel specified, with any input: Pass through up to eight input channels.
*
*
* -
*
* 0
channels specified, with any input: Audio omitted from the output.
*
*
* -
*
* 1
channel specified, with at least one input channel: Mono sound.
*
*
* -
*
* 2
channels specified, with any input: Two identical mono channels or stereo. For
* more information about tracks, see Audio:AudioPackingMode.
*
*
*
*
* For more information about how Elastic Transcoder organizes channels and tracks, see
* Audio:AudioPackingMode
.
*/
public final String channels() {
return channels;
}
/**
*
* The method of organizing audio channels and tracks. Use Audio:Channels
to specify the number of
* channels in your output, and Audio:AudioPackingMode
to specify the number of tracks and their
* relation to the channels. If you do not specify an Audio:AudioPackingMode
, Elastic Transcoder uses
* SingleTrack
.
*
*
* The following values are valid:
*
*
* SingleTrack
, OneChannelPerTrack
, and OneChannelPerTrackWithMosTo8Tracks
*
*
* When you specify SingleTrack
, Elastic Transcoder creates a single track for your output. The track
* can have up to eight channels. Use SingleTrack
for all non-mxf
containers.
*
*
* The outputs of SingleTrack
for a specific channel value and inputs are as follows:
*
*
* -
*
* 0
channels with any input: Audio omitted from the output
*
*
* -
*
* 1, 2, or auto
channels with no audio input: Audio omitted from the output
*
*
* -
*
* 1
channel with any input with audio: One track with one channel, downmixed if necessary
*
*
* -
*
* 2
channels with one track with one channel: One track with two identical channels
*
*
* -
*
* 2 or auto
channels with two tracks with one channel each: One track with two channels
*
*
* -
*
* 2 or auto
channels with one track with two channels: One track with two channels
*
*
* -
*
* 2
channels with one track with multiple channels: One track with two channels
*
*
* -
*
* auto
channels with one track with one channel: One track with one channel
*
*
* -
*
* auto
channels with one track with multiple channels: One track with multiple channels
*
*
*
*
* When you specify OneChannelPerTrack
, Elastic Transcoder creates a new track for every channel in
* your output. Your output can have up to eight single-channel tracks.
*
*
* The outputs of OneChannelPerTrack
for a specific channel value and inputs are as follows:
*
*
* -
*
* 0
channels with any input: Audio omitted from the output
*
*
* -
*
* 1, 2, or auto
channels with no audio input: Audio omitted from the output
*
*
* -
*
* 1
channel with any input with audio: One track with one channel, downmixed if necessary
*
*
* -
*
* 2
channels with one track with one channel: Two tracks with one identical channel each
*
*
* -
*
* 2 or auto
channels with two tracks with one channel each: Two tracks with one channel each
*
*
* -
*
* 2 or auto
channels with one track with two channels: Two tracks with one channel each
*
*
* -
*
* 2
channels with one track with multiple channels: Two tracks with one channel each
*
*
* -
*
* auto
channels with one track with one channel: One track with one channel
*
*
* -
*
* auto
channels with one track with multiple channels: Up to eight tracks with one channel
* each
*
*
*
*
* When you specify OneChannelPerTrackWithMosTo8Tracks
, Elastic Transcoder creates eight single-channel
* tracks for your output. All tracks that do not contain audio data from an input channel are MOS, or Mit Out
* Sound, tracks.
*
*
* The outputs of OneChannelPerTrackWithMosTo8Tracks
for a specific channel value and inputs are as
* follows:
*
*
* -
*
* 0
channels with any input: Audio omitted from the output
*
*
* -
*
* 1, 2, or auto
channels with no audio input: Audio omitted from the output
*
*
* -
*
* 1
channel with any input with audio: One track with one channel, downmixed if necessary,
* plus six MOS tracks
*
*
* -
*
* 2
channels with one track with one channel: Two tracks with one identical channel each, plus
* six MOS tracks
*
*
* -
*
* 2 or auto
channels with two tracks with one channel each: Two tracks with one channel each,
* plus six MOS tracks
*
*
* -
*
* 2 or auto
channels with one track with two channels: Two tracks with one channel each, plus
* six MOS tracks
*
*
* -
*
* 2
channels with one track with multiple channels: Two tracks with one channel each, plus six
* MOS tracks
*
*
* -
*
* auto
channels with one track with one channel: One track with one channel, plus seven MOS
* tracks
*
*
* -
*
* auto
channels with one track with multiple channels: Up to eight tracks with one channel
* each, plus MOS tracks until there are eight tracks in all
*
*
*
*
* @return The method of organizing audio channels and tracks. Use Audio:Channels
to specify the number
* of channels in your output, and Audio:AudioPackingMode
to specify the number of tracks and
* their relation to the channels. If you do not specify an Audio:AudioPackingMode
, Elastic
* Transcoder uses SingleTrack
.
*
* The following values are valid:
*
*
* SingleTrack
, OneChannelPerTrack
, and
* OneChannelPerTrackWithMosTo8Tracks
*
*
* When you specify SingleTrack
, Elastic Transcoder creates a single track for your output. The
* track can have up to eight channels. Use SingleTrack
for all non-mxf
* containers.
*
*
* The outputs of SingleTrack
for a specific channel value and inputs are as follows:
*
*
* -
*
* 0
channels with any input: Audio omitted from the output
*
*
* -
*
* 1, 2, or auto
channels with no audio input: Audio omitted from the output
*
*
* -
*
* 1
channel with any input with audio: One track with one channel, downmixed if
* necessary
*
*
* -
*
* 2
channels with one track with one channel: One track with two identical channels
*
*
* -
*
* 2 or auto
channels with two tracks with one channel each: One track with two
* channels
*
*
* -
*
* 2 or auto
channels with one track with two channels: One track with two channels
*
*
* -
*
* 2
channels with one track with multiple channels: One track with two channels
*
*
* -
*
* auto
channels with one track with one channel: One track with one channel
*
*
* -
*
* auto
channels with one track with multiple channels: One track with multiple
* channels
*
*
*
*
* When you specify OneChannelPerTrack
, Elastic Transcoder creates a new track for every
* channel in your output. Your output can have up to eight single-channel tracks.
*
*
* The outputs of OneChannelPerTrack
for a specific channel value and inputs are as follows:
*
*
* -
*
* 0
channels with any input: Audio omitted from the output
*
*
* -
*
* 1, 2, or auto
channels with no audio input: Audio omitted from the output
*
*
* -
*
* 1
channel with any input with audio: One track with one channel, downmixed if
* necessary
*
*
* -
*
* 2
channels with one track with one channel: Two tracks with one identical channel
* each
*
*
* -
*
* 2 or auto
channels with two tracks with one channel each: Two tracks with one
* channel each
*
*
* -
*
* 2 or auto
channels with one track with two channels: Two tracks with one channel
* each
*
*
* -
*
* 2
channels with one track with multiple channels: Two tracks with one channel each
*
*
* -
*
* auto
channels with one track with one channel: One track with one channel
*
*
* -
*
* auto
channels with one track with multiple channels: Up to eight tracks with one
* channel each
*
*
*
*
* When you specify OneChannelPerTrackWithMosTo8Tracks
, Elastic Transcoder creates eight
* single-channel tracks for your output. All tracks that do not contain audio data from an input channel
* are MOS, or Mit Out Sound, tracks.
*
*
* The outputs of OneChannelPerTrackWithMosTo8Tracks
for a specific channel value and inputs
* are as follows:
*
*
* -
*
* 0
channels with any input: Audio omitted from the output
*
*
* -
*
* 1, 2, or auto
channels with no audio input: Audio omitted from the output
*
*
* -
*
* 1
channel with any input with audio: One track with one channel, downmixed if
* necessary, plus six MOS tracks
*
*
* -
*
* 2
channels with one track with one channel: Two tracks with one identical channel
* each, plus six MOS tracks
*
*
* -
*
* 2 or auto
channels with two tracks with one channel each: Two tracks with one
* channel each, plus six MOS tracks
*
*
* -
*
* 2 or auto
channels with one track with two channels: Two tracks with one channel
* each, plus six MOS tracks
*
*
* -
*
* 2
channels with one track with multiple channels: Two tracks with one channel each,
* plus six MOS tracks
*
*
* -
*
* auto
channels with one track with one channel: One track with one channel, plus
* seven MOS tracks
*
*
* -
*
* auto
channels with one track with multiple channels: Up to eight tracks with one
* channel each, plus MOS tracks until there are eight tracks in all
*
*
*/
public final String audioPackingMode() {
return audioPackingMode;
}
/**
*
* If you specified AAC
for Audio:Codec
, this is the AAC
compression profile
* to use. Valid values include:
*
*
* auto
, AAC-LC
, HE-AAC
, HE-AACv2
*
*
* If you specify auto
, Elastic Transcoder chooses a profile based on the bit rate of the output file.
*
*
* @return If you specified AAC
for Audio:Codec
, this is the AAC
compression
* profile to use. Valid values include:
*
* auto
, AAC-LC
, HE-AAC
, HE-AACv2
*
*
* If you specify auto
, Elastic Transcoder chooses a profile based on the bit rate of the
* output file.
*/
public final AudioCodecOptions codecOptions() {
return codecOptions;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(codec());
hashCode = 31 * hashCode + Objects.hashCode(sampleRate());
hashCode = 31 * hashCode + Objects.hashCode(bitRate());
hashCode = 31 * hashCode + Objects.hashCode(channels());
hashCode = 31 * hashCode + Objects.hashCode(audioPackingMode());
hashCode = 31 * hashCode + Objects.hashCode(codecOptions());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof AudioParameters)) {
return false;
}
AudioParameters other = (AudioParameters) obj;
return Objects.equals(codec(), other.codec()) && Objects.equals(sampleRate(), other.sampleRate())
&& Objects.equals(bitRate(), other.bitRate()) && Objects.equals(channels(), other.channels())
&& Objects.equals(audioPackingMode(), other.audioPackingMode())
&& Objects.equals(codecOptions(), other.codecOptions());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("AudioParameters").add("Codec", codec()).add("SampleRate", sampleRate())
.add("BitRate", bitRate()).add("Channels", channels()).add("AudioPackingMode", audioPackingMode())
.add("CodecOptions", codecOptions()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "Codec":
return Optional.ofNullable(clazz.cast(codec()));
case "SampleRate":
return Optional.ofNullable(clazz.cast(sampleRate()));
case "BitRate":
return Optional.ofNullable(clazz.cast(bitRate()));
case "Channels":
return Optional.ofNullable(clazz.cast(channels()));
case "AudioPackingMode":
return Optional.ofNullable(clazz.cast(audioPackingMode()));
case "CodecOptions":
return Optional.ofNullable(clazz.cast(codecOptions()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
private static Function
*
* auto
, 22050
, 32000
, 44100
, 48000
,
* 96000
*
*
* If you specify auto
, Elastic Transcoder automatically detects the sample rate.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder sampleRate(String sampleRate);
/**
*
* The bit rate of the audio stream in the output file, in kilobits/second. Enter an integer between 64 and 320,
* inclusive.
*
*
* @param bitRate
* The bit rate of the audio stream in the output file, in kilobits/second. Enter an integer between 64
* and 320, inclusive.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder bitRate(String bitRate);
/**
*
* The number of audio channels in the output file. The following values are valid:
*
*
* auto
, 0
, 1
, 2
*
*
* One channel carries the information played by a single speaker. For example, a stereo track with two channels
* sends one channel to the left speaker, and the other channel to the right speaker. The output channels are
* organized into tracks. If you want Elastic Transcoder to automatically detect the number of audio channels in
* the input file and use that value for the output file, select auto
.
*
*
* The output of a specific channel value and inputs are as follows:
*
*
* -
*
* auto
channel specified, with any input: Pass through up to eight input channels.
*
*
* -
*
* 0
channels specified, with any input: Audio omitted from the output.
*
*
* -
*
* 1
channel specified, with at least one input channel: Mono sound.
*
*
* -
*
* 2
channels specified, with any input: Two identical mono channels or stereo. For more
* information about tracks, see Audio:AudioPackingMode.
*
*
*
*
* For more information about how Elastic Transcoder organizes channels and tracks, see
* Audio:AudioPackingMode
.
*
*
* @param channels
* The number of audio channels in the output file. The following values are valid:
*
* auto
, 0
, 1
, 2
*
*
* One channel carries the information played by a single speaker. For example, a stereo track with two
* channels sends one channel to the left speaker, and the other channel to the right speaker. The output
* channels are organized into tracks. If you want Elastic Transcoder to automatically detect the number
* of audio channels in the input file and use that value for the output file, select auto
.
*
*
* The output of a specific channel value and inputs are as follows:
*
*
* -
*
* auto
channel specified, with any input: Pass through up to eight input channels.
*
*
* -
*
* 0
channels specified, with any input: Audio omitted from the output.
*
*
* -
*
* 1
channel specified, with at least one input channel: Mono sound.
*
*
* -
*
* 2
channels specified, with any input: Two identical mono channels or stereo. For
* more information about tracks, see Audio:AudioPackingMode.
*
*
*
*
* For more information about how Elastic Transcoder organizes channels and tracks, see
* Audio:AudioPackingMode
.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder channels(String channels);
/**
*
* The method of organizing audio channels and tracks. Use Audio:Channels
to specify the number of
* channels in your output, and Audio:AudioPackingMode
to specify the number of tracks and their
* relation to the channels. If you do not specify an Audio:AudioPackingMode
, Elastic Transcoder
* uses SingleTrack
.
*
*
* The following values are valid:
*
*
* SingleTrack
, OneChannelPerTrack
, and
* OneChannelPerTrackWithMosTo8Tracks
*
*
* When you specify SingleTrack
, Elastic Transcoder creates a single track for your output. The
* track can have up to eight channels. Use SingleTrack
for all non-mxf
containers.
*
*
* The outputs of SingleTrack
for a specific channel value and inputs are as follows:
*
*
* -
*
* 0
channels with any input: Audio omitted from the output
*
*
* -
*
* 1, 2, or auto
channels with no audio input: Audio omitted from the output
*
*
* -
*
* 1
channel with any input with audio: One track with one channel, downmixed if necessary
*
*
* -
*
* 2
channels with one track with one channel: One track with two identical channels
*
*
* -
*
* 2 or auto
channels with two tracks with one channel each: One track with two channels
*
*
* -
*
* 2 or auto
channels with one track with two channels: One track with two channels
*
*
* -
*
* 2
channels with one track with multiple channels: One track with two channels
*
*
* -
*
* auto
channels with one track with one channel: One track with one channel
*
*
* -
*
* auto
channels with one track with multiple channels: One track with multiple channels
*
*
*
*
* When you specify OneChannelPerTrack
, Elastic Transcoder creates a new track for every channel in
* your output. Your output can have up to eight single-channel tracks.
*
*
* The outputs of OneChannelPerTrack
for a specific channel value and inputs are as follows:
*
*
* -
*
* 0
channels with any input: Audio omitted from the output
*
*
* -
*
* 1, 2, or auto
channels with no audio input: Audio omitted from the output
*
*
* -
*
* 1
channel with any input with audio: One track with one channel, downmixed if necessary
*
*
* -
*
* 2
channels with one track with one channel: Two tracks with one identical channel each
*
*
* -
*
* 2 or auto
channels with two tracks with one channel each: Two tracks with one channel
* each
*
*
* -
*
* 2 or auto
channels with one track with two channels: Two tracks with one channel each
*
*
* -
*
* 2
channels with one track with multiple channels: Two tracks with one channel each
*
*
* -
*
* auto
channels with one track with one channel: One track with one channel
*
*
* -
*
* auto
channels with one track with multiple channels: Up to eight tracks with one channel
* each
*
*
*
*
* When you specify OneChannelPerTrackWithMosTo8Tracks
, Elastic Transcoder creates eight
* single-channel tracks for your output. All tracks that do not contain audio data from an input channel are
* MOS, or Mit Out Sound, tracks.
*
*
* The outputs of OneChannelPerTrackWithMosTo8Tracks
for a specific channel value and inputs are as
* follows:
*
*
* -
*
* 0
channels with any input: Audio omitted from the output
*
*
* -
*
* 1, 2, or auto
channels with no audio input: Audio omitted from the output
*
*
* -
*
* 1
channel with any input with audio: One track with one channel, downmixed if necessary,
* plus six MOS tracks
*
*
* -
*
* 2
channels with one track with one channel: Two tracks with one identical channel each,
* plus six MOS tracks
*
*
* -
*
* 2 or auto
channels with two tracks with one channel each: Two tracks with one channel
* each, plus six MOS tracks
*
*
* -
*
* 2 or auto
channels with one track with two channels: Two tracks with one channel each,
* plus six MOS tracks
*
*
* -
*
* 2
channels with one track with multiple channels: Two tracks with one channel each, plus
* six MOS tracks
*
*
* -
*
* auto
channels with one track with one channel: One track with one channel, plus seven
* MOS tracks
*
*
* -
*
* auto
channels with one track with multiple channels: Up to eight tracks with one channel
* each, plus MOS tracks until there are eight tracks in all
*
*
*
*
* @param audioPackingMode
* The method of organizing audio channels and tracks. Use Audio:Channels
to specify the
* number of channels in your output, and Audio:AudioPackingMode
to specify the number of
* tracks and their relation to the channels. If you do not specify an
* Audio:AudioPackingMode
, Elastic Transcoder uses SingleTrack
.
*
* The following values are valid:
*
*
* SingleTrack
, OneChannelPerTrack
, and
* OneChannelPerTrackWithMosTo8Tracks
*
*
* When you specify SingleTrack
, Elastic Transcoder creates a single track for your output.
* The track can have up to eight channels. Use SingleTrack
for all non-mxf
* containers.
*
*
* The outputs of SingleTrack
for a specific channel value and inputs are as follows:
*
*
* -
*
* 0
channels with any input: Audio omitted from the output
*
*
* -
*
* 1, 2, or auto
channels with no audio input: Audio omitted from the output
*
*
* -
*
* 1
channel with any input with audio: One track with one channel, downmixed if
* necessary
*
*
* -
*
* 2
channels with one track with one channel: One track with two identical channels
*
*
* -
*
* 2 or auto
channels with two tracks with one channel each: One track with two
* channels
*
*
* -
*
* 2 or auto
channels with one track with two channels: One track with two channels
*
*
* -
*
* 2
channels with one track with multiple channels: One track with two channels
*
*
* -
*
* auto
channels with one track with one channel: One track with one channel
*
*
* -
*
* auto
channels with one track with multiple channels: One track with multiple
* channels
*
*
*
*
* When you specify OneChannelPerTrack
, Elastic Transcoder creates a new track for every
* channel in your output. Your output can have up to eight single-channel tracks.
*
*
* The outputs of OneChannelPerTrack
for a specific channel value and inputs are as follows:
*
*
* -
*
* 0
channels with any input: Audio omitted from the output
*
*
* -
*
* 1, 2, or auto
channels with no audio input: Audio omitted from the output
*
*
* -
*
* 1
channel with any input with audio: One track with one channel, downmixed if
* necessary
*
*
* -
*
* 2
channels with one track with one channel: Two tracks with one identical channel
* each
*
*
* -
*
* 2 or auto
channels with two tracks with one channel each: Two tracks with one
* channel each
*
*
* -
*
* 2 or auto
channels with one track with two channels: Two tracks with one channel
* each
*
*
* -
*
* 2
channels with one track with multiple channels: Two tracks with one channel
* each
*
*
* -
*
* auto
channels with one track with one channel: One track with one channel
*
*
* -
*
* auto
channels with one track with multiple channels: Up to eight tracks with one
* channel each
*
*
*
*
* When you specify OneChannelPerTrackWithMosTo8Tracks
, Elastic Transcoder creates eight
* single-channel tracks for your output. All tracks that do not contain audio data from an input channel
* are MOS, or Mit Out Sound, tracks.
*
*
* The outputs of OneChannelPerTrackWithMosTo8Tracks
for a specific channel value and inputs
* are as follows:
*
*
* -
*
* 0
channels with any input: Audio omitted from the output
*
*
* -
*
* 1, 2, or auto
channels with no audio input: Audio omitted from the output
*
*
* -
*
* 1
channel with any input with audio: One track with one channel, downmixed if
* necessary, plus six MOS tracks
*
*
* -
*
* 2
channels with one track with one channel: Two tracks with one identical channel
* each, plus six MOS tracks
*
*
* -
*
* 2 or auto
channels with two tracks with one channel each: Two tracks with one
* channel each, plus six MOS tracks
*
*
* -
*
* 2 or auto
channels with one track with two channels: Two tracks with one channel
* each, plus six MOS tracks
*
*
* -
*
* 2
channels with one track with multiple channels: Two tracks with one channel
* each, plus six MOS tracks
*
*
* -
*
* auto
channels with one track with one channel: One track with one channel, plus
* seven MOS tracks
*
*
* -
*
* auto
channels with one track with multiple channels: Up to eight tracks with one
* channel each, plus MOS tracks until there are eight tracks in all
*
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder audioPackingMode(String audioPackingMode);
/**
*
* If you specified AAC
for Audio:Codec
, this is the AAC
compression
* profile to use. Valid values include:
*
*
* auto
, AAC-LC
, HE-AAC
, HE-AACv2
*
*
* If you specify auto
, Elastic Transcoder chooses a profile based on the bit rate of the output
* file.
*
*
* @param codecOptions
* If you specified AAC
for Audio:Codec
, this is the AAC
* compression profile to use. Valid values include:
*
* auto
, AAC-LC
, HE-AAC
, HE-AACv2
*
*
* If you specify auto
, Elastic Transcoder chooses a profile based on the bit rate of the
* output file.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder codecOptions(AudioCodecOptions codecOptions);
/**
*
* If you specified AAC
for Audio:Codec
, this is the AAC
compression
* profile to use. Valid values include:
*
*
* auto
, AAC-LC
, HE-AAC
, HE-AACv2
*
*
* If you specify auto
, Elastic Transcoder chooses a profile based on the bit rate of the output
* file.
*
* This is a convenience that creates an instance of the {@link AudioCodecOptions.Builder} avoiding the need to
* create one manually via {@link AudioCodecOptions#builder()}.
*
* When the {@link Consumer} completes, {@link AudioCodecOptions.Builder#build()} is called immediately and its
* result is passed to {@link #codecOptions(AudioCodecOptions)}.
*
* @param codecOptions
* a consumer that will call methods on {@link AudioCodecOptions.Builder}
* @return Returns a reference to this object so that method calls can be chained together.
* @see #codecOptions(AudioCodecOptions)
*/
default Builder codecOptions(Consumer codecOptions) {
return codecOptions(AudioCodecOptions.builder().applyMutation(codecOptions).build());
}
}
static final class BuilderImpl implements Builder {
private String codec;
private String sampleRate;
private String bitRate;
private String channels;
private String audioPackingMode;
private AudioCodecOptions codecOptions;
private BuilderImpl() {
}
private BuilderImpl(AudioParameters model) {
codec(model.codec);
sampleRate(model.sampleRate);
bitRate(model.bitRate);
channels(model.channels);
audioPackingMode(model.audioPackingMode);
codecOptions(model.codecOptions);
}
public final String getCodec() {
return codec;
}
public final void setCodec(String codec) {
this.codec = codec;
}
@Override
@Transient
public final Builder codec(String codec) {
this.codec = codec;
return this;
}
public final String getSampleRate() {
return sampleRate;
}
public final void setSampleRate(String sampleRate) {
this.sampleRate = sampleRate;
}
@Override
@Transient
public final Builder sampleRate(String sampleRate) {
this.sampleRate = sampleRate;
return this;
}
public final String getBitRate() {
return bitRate;
}
public final void setBitRate(String bitRate) {
this.bitRate = bitRate;
}
@Override
@Transient
public final Builder bitRate(String bitRate) {
this.bitRate = bitRate;
return this;
}
public final String getChannels() {
return channels;
}
public final void setChannels(String channels) {
this.channels = channels;
}
@Override
@Transient
public final Builder channels(String channels) {
this.channels = channels;
return this;
}
public final String getAudioPackingMode() {
return audioPackingMode;
}
public final void setAudioPackingMode(String audioPackingMode) {
this.audioPackingMode = audioPackingMode;
}
@Override
@Transient
public final Builder audioPackingMode(String audioPackingMode) {
this.audioPackingMode = audioPackingMode;
return this;
}
public final AudioCodecOptions.Builder getCodecOptions() {
return codecOptions != null ? codecOptions.toBuilder() : null;
}
public final void setCodecOptions(AudioCodecOptions.BuilderImpl codecOptions) {
this.codecOptions = codecOptions != null ? codecOptions.build() : null;
}
@Override
@Transient
public final Builder codecOptions(AudioCodecOptions codecOptions) {
this.codecOptions = codecOptions;
return this;
}
@Override
public AudioParameters build() {
return new AudioParameters(this);
}
@Override
public List> sdkFields() {
return SDK_FIELDS;
}
}
}