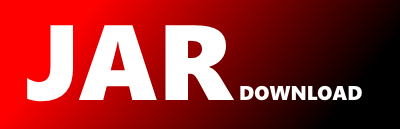
software.amazon.awssdk.services.elastictranscoder.model.Pipeline Maven / Gradle / Ivy
Show all versions of elastictranscoder Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.elastictranscoder.model;
import java.beans.Transient;
import java.io.Serializable;
import java.util.Arrays;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* The pipeline (queue) that is used to manage jobs.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class Pipeline implements SdkPojo, Serializable, ToCopyableBuilder {
private static final SdkField ID_FIELD = SdkField. builder(MarshallingType.STRING).memberName("Id")
.getter(getter(Pipeline::id)).setter(setter(Builder::id))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Id").build()).build();
private static final SdkField ARN_FIELD = SdkField. builder(MarshallingType.STRING).memberName("Arn")
.getter(getter(Pipeline::arn)).setter(setter(Builder::arn))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Arn").build()).build();
private static final SdkField NAME_FIELD = SdkField. builder(MarshallingType.STRING).memberName("Name")
.getter(getter(Pipeline::name)).setter(setter(Builder::name))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Name").build()).build();
private static final SdkField STATUS_FIELD = SdkField. builder(MarshallingType.STRING).memberName("Status")
.getter(getter(Pipeline::status)).setter(setter(Builder::status))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Status").build()).build();
private static final SdkField INPUT_BUCKET_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("InputBucket").getter(getter(Pipeline::inputBucket)).setter(setter(Builder::inputBucket))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("InputBucket").build()).build();
private static final SdkField OUTPUT_BUCKET_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("OutputBucket").getter(getter(Pipeline::outputBucket)).setter(setter(Builder::outputBucket))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("OutputBucket").build()).build();
private static final SdkField ROLE_FIELD = SdkField. builder(MarshallingType.STRING).memberName("Role")
.getter(getter(Pipeline::role)).setter(setter(Builder::role))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Role").build()).build();
private static final SdkField AWS_KMS_KEY_ARN_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("AwsKmsKeyArn").getter(getter(Pipeline::awsKmsKeyArn)).setter(setter(Builder::awsKmsKeyArn))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("AwsKmsKeyArn").build()).build();
private static final SdkField NOTIFICATIONS_FIELD = SdkField. builder(MarshallingType.SDK_POJO)
.memberName("Notifications").getter(getter(Pipeline::notifications)).setter(setter(Builder::notifications))
.constructor(Notifications::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Notifications").build()).build();
private static final SdkField CONTENT_CONFIG_FIELD = SdkField
. builder(MarshallingType.SDK_POJO).memberName("ContentConfig")
.getter(getter(Pipeline::contentConfig)).setter(setter(Builder::contentConfig))
.constructor(PipelineOutputConfig::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ContentConfig").build()).build();
private static final SdkField THUMBNAIL_CONFIG_FIELD = SdkField
. builder(MarshallingType.SDK_POJO).memberName("ThumbnailConfig")
.getter(getter(Pipeline::thumbnailConfig)).setter(setter(Builder::thumbnailConfig))
.constructor(PipelineOutputConfig::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ThumbnailConfig").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(ID_FIELD, ARN_FIELD,
NAME_FIELD, STATUS_FIELD, INPUT_BUCKET_FIELD, OUTPUT_BUCKET_FIELD, ROLE_FIELD, AWS_KMS_KEY_ARN_FIELD,
NOTIFICATIONS_FIELD, CONTENT_CONFIG_FIELD, THUMBNAIL_CONFIG_FIELD));
private static final long serialVersionUID = 1L;
private final String id;
private final String arn;
private final String name;
private final String status;
private final String inputBucket;
private final String outputBucket;
private final String role;
private final String awsKmsKeyArn;
private final Notifications notifications;
private final PipelineOutputConfig contentConfig;
private final PipelineOutputConfig thumbnailConfig;
private Pipeline(BuilderImpl builder) {
this.id = builder.id;
this.arn = builder.arn;
this.name = builder.name;
this.status = builder.status;
this.inputBucket = builder.inputBucket;
this.outputBucket = builder.outputBucket;
this.role = builder.role;
this.awsKmsKeyArn = builder.awsKmsKeyArn;
this.notifications = builder.notifications;
this.contentConfig = builder.contentConfig;
this.thumbnailConfig = builder.thumbnailConfig;
}
/**
*
* The identifier for the pipeline. You use this value to identify the pipeline in which you want to perform a
* variety of operations, such as creating a job or a preset.
*
*
* @return The identifier for the pipeline. You use this value to identify the pipeline in which you want to perform
* a variety of operations, such as creating a job or a preset.
*/
public final String id() {
return id;
}
/**
*
* The Amazon Resource Name (ARN) for the pipeline.
*
*
* @return The Amazon Resource Name (ARN) for the pipeline.
*/
public final String arn() {
return arn;
}
/**
*
* The name of the pipeline. We recommend that the name be unique within the AWS account, but uniqueness is not
* enforced.
*
*
* Constraints: Maximum 40 characters
*
*
* @return The name of the pipeline. We recommend that the name be unique within the AWS account, but uniqueness is
* not enforced.
*
* Constraints: Maximum 40 characters
*/
public final String name() {
return name;
}
/**
*
* The current status of the pipeline:
*
*
* -
*
* Active
: The pipeline is processing jobs.
*
*
* -
*
* Paused
: The pipeline is not currently processing jobs.
*
*
*
*
* @return The current status of the pipeline:
*
* -
*
* Active
: The pipeline is processing jobs.
*
*
* -
*
* Paused
: The pipeline is not currently processing jobs.
*
*
*/
public final String status() {
return status;
}
/**
*
* The Amazon S3 bucket from which Elastic Transcoder gets media files for transcoding and the graphics files, if
* any, that you want to use for watermarks.
*
*
* @return The Amazon S3 bucket from which Elastic Transcoder gets media files for transcoding and the graphics
* files, if any, that you want to use for watermarks.
*/
public final String inputBucket() {
return inputBucket;
}
/**
*
* The Amazon S3 bucket in which you want Elastic Transcoder to save transcoded files, thumbnails, and playlists.
* Either you specify this value, or you specify both ContentConfig
and ThumbnailConfig
.
*
*
* @return The Amazon S3 bucket in which you want Elastic Transcoder to save transcoded files, thumbnails, and
* playlists. Either you specify this value, or you specify both ContentConfig
and
* ThumbnailConfig
.
*/
public final String outputBucket() {
return outputBucket;
}
/**
*
* The IAM Amazon Resource Name (ARN) for the role that Elastic Transcoder uses to transcode jobs for this pipeline.
*
*
* @return The IAM Amazon Resource Name (ARN) for the role that Elastic Transcoder uses to transcode jobs for this
* pipeline.
*/
public final String role() {
return role;
}
/**
*
* The AWS Key Management Service (AWS KMS) key that you want to use with this pipeline.
*
*
* If you use either s3
or s3-aws-kms
as your Encryption:Mode
, you don't need
* to provide a key with your job because a default key, known as an AWS-KMS key, is created for you automatically.
* You need to provide an AWS-KMS key only if you want to use a non-default AWS-KMS key, or if you are using an
* Encryption:Mode
of aes-cbc-pkcs7
, aes-ctr
, or aes-gcm
.
*
*
* @return The AWS Key Management Service (AWS KMS) key that you want to use with this pipeline.
*
* If you use either s3
or s3-aws-kms
as your Encryption:Mode
, you
* don't need to provide a key with your job because a default key, known as an AWS-KMS key, is created for
* you automatically. You need to provide an AWS-KMS key only if you want to use a non-default AWS-KMS key,
* or if you are using an Encryption:Mode
of aes-cbc-pkcs7
, aes-ctr
,
* or aes-gcm
.
*/
public final String awsKmsKeyArn() {
return awsKmsKeyArn;
}
/**
*
* The Amazon Simple Notification Service (Amazon SNS) topic that you want to notify to report job status.
*
*
*
* To receive notifications, you must also subscribe to the new topic in the Amazon SNS console.
*
*
*
* -
*
* Progressing (optional): The Amazon Simple Notification Service (Amazon SNS) topic that you want to notify
* when Elastic Transcoder has started to process the job.
*
*
* -
*
* Complete (optional): The Amazon SNS topic that you want to notify when Elastic Transcoder has finished
* processing the job.
*
*
* -
*
* Warning (optional): The Amazon SNS topic that you want to notify when Elastic Transcoder encounters a
* warning condition.
*
*
* -
*
* Error (optional): The Amazon SNS topic that you want to notify when Elastic Transcoder encounters an error
* condition.
*
*
*
*
* @return The Amazon Simple Notification Service (Amazon SNS) topic that you want to notify to report job
* status.
*
* To receive notifications, you must also subscribe to the new topic in the Amazon SNS console.
*
*
*
* -
*
* Progressing (optional): The Amazon Simple Notification Service (Amazon SNS) topic that you want to
* notify when Elastic Transcoder has started to process the job.
*
*
* -
*
* Complete (optional): The Amazon SNS topic that you want to notify when Elastic Transcoder has
* finished processing the job.
*
*
* -
*
* Warning (optional): The Amazon SNS topic that you want to notify when Elastic Transcoder
* encounters a warning condition.
*
*
* -
*
* Error (optional): The Amazon SNS topic that you want to notify when Elastic Transcoder encounters
* an error condition.
*
*
*/
public final Notifications notifications() {
return notifications;
}
/**
*
* Information about the Amazon S3 bucket in which you want Elastic Transcoder to save transcoded files and
* playlists. Either you specify both ContentConfig
and ThumbnailConfig
, or you specify
* OutputBucket
.
*
*
* -
*
* Bucket: The Amazon S3 bucket in which you want Elastic Transcoder to save transcoded files and playlists.
*
*
* -
*
* Permissions: A list of the users and/or predefined Amazon S3 groups you want to have access to transcoded
* files and playlists, and the type of access that you want them to have.
*
*
* -
*
* GranteeType: The type of value that appears in the Grantee
object:
*
*
* -
*
* Canonical
: Either the canonical user ID for an AWS account or an origin access identity for an
* Amazon CloudFront distribution.
*
*
* -
*
* Email
: The registered email address of an AWS account.
*
*
* -
*
* Group
: One of the following predefined Amazon S3 groups: AllUsers
,
* AuthenticatedUsers
, or LogDelivery
.
*
*
*
*
* -
*
* Grantee
: The AWS user or group that you want to have access to transcoded files and playlists.
*
*
* -
*
* Access
: The permission that you want to give to the AWS user that is listed in Grantee
.
* Valid values include:
*
*
* -
*
* READ
: The grantee can read the objects and metadata for objects that Elastic Transcoder adds to the
* Amazon S3 bucket.
*
*
* -
*
* READ_ACP
: The grantee can read the object ACL for objects that Elastic Transcoder adds to the Amazon
* S3 bucket.
*
*
* -
*
* WRITE_ACP
: The grantee can write the ACL for the objects that Elastic Transcoder adds to the Amazon
* S3 bucket.
*
*
* -
*
* FULL_CONTROL
: The grantee has READ
, READ_ACP
, and WRITE_ACP
* permissions for the objects that Elastic Transcoder adds to the Amazon S3 bucket.
*
*
*
*
*
*
* -
*
* StorageClass: The Amazon S3 storage class, Standard or ReducedRedundancy, that you want Elastic Transcoder
* to assign to the video files and playlists that it stores in your Amazon S3 bucket.
*
*
*
*
* @return Information about the Amazon S3 bucket in which you want Elastic Transcoder to save transcoded files and
* playlists. Either you specify both ContentConfig
and ThumbnailConfig
, or you
* specify OutputBucket
.
*
* -
*
* Bucket: The Amazon S3 bucket in which you want Elastic Transcoder to save transcoded files and
* playlists.
*
*
* -
*
* Permissions: A list of the users and/or predefined Amazon S3 groups you want to have access to
* transcoded files and playlists, and the type of access that you want them to have.
*
*
* -
*
* GranteeType: The type of value that appears in the Grantee
object:
*
*
* -
*
* Canonical
: Either the canonical user ID for an AWS account or an origin access identity for
* an Amazon CloudFront distribution.
*
*
* -
*
* Email
: The registered email address of an AWS account.
*
*
* -
*
* Group
: One of the following predefined Amazon S3 groups: AllUsers
,
* AuthenticatedUsers
, or LogDelivery
.
*
*
*
*
* -
*
* Grantee
: The AWS user or group that you want to have access to transcoded files and
* playlists.
*
*
* -
*
* Access
: The permission that you want to give to the AWS user that is listed in
* Grantee
. Valid values include:
*
*
* -
*
* READ
: The grantee can read the objects and metadata for objects that Elastic Transcoder adds
* to the Amazon S3 bucket.
*
*
* -
*
* READ_ACP
: The grantee can read the object ACL for objects that Elastic Transcoder adds to
* the Amazon S3 bucket.
*
*
* -
*
* WRITE_ACP
: The grantee can write the ACL for the objects that Elastic Transcoder adds to the
* Amazon S3 bucket.
*
*
* -
*
* FULL_CONTROL
: The grantee has READ
, READ_ACP
, and
* WRITE_ACP
permissions for the objects that Elastic Transcoder adds to the Amazon S3 bucket.
*
*
*
*
*
*
* -
*
* StorageClass: The Amazon S3 storage class, Standard or ReducedRedundancy, that you want Elastic
* Transcoder to assign to the video files and playlists that it stores in your Amazon S3 bucket.
*
*
*/
public final PipelineOutputConfig contentConfig() {
return contentConfig;
}
/**
*
* Information about the Amazon S3 bucket in which you want Elastic Transcoder to save thumbnail files. Either you
* specify both ContentConfig
and ThumbnailConfig
, or you specify
* OutputBucket
.
*
*
* -
*
* Bucket
: The Amazon S3 bucket in which you want Elastic Transcoder to save thumbnail files.
*
*
* -
*
* Permissions
: A list of the users and/or predefined Amazon S3 groups you want to have access to
* thumbnail files, and the type of access that you want them to have.
*
*
* -
*
* GranteeType: The type of value that appears in the Grantee object:
*
*
* -
*
* Canonical
: Either the canonical user ID for an AWS account or an origin access identity for an
* Amazon CloudFront distribution.
*
*
*
* A canonical user ID is not the same as an AWS account number.
*
*
* -
*
* Email
: The registered email address of an AWS account.
*
*
* -
*
* Group
: One of the following predefined Amazon S3 groups: AllUsers
,
* AuthenticatedUsers
, or LogDelivery
.
*
*
*
*
* -
*
* Grantee
: The AWS user or group that you want to have access to thumbnail files.
*
*
* -
*
* Access: The permission that you want to give to the AWS user that is listed in Grantee. Valid values include:
*
*
* -
*
* READ
: The grantee can read the thumbnails and metadata for thumbnails that Elastic Transcoder adds
* to the Amazon S3 bucket.
*
*
* -
*
* READ_ACP
: The grantee can read the object ACL for thumbnails that Elastic Transcoder adds to the
* Amazon S3 bucket.
*
*
* -
*
* WRITE_ACP
: The grantee can write the ACL for the thumbnails that Elastic Transcoder adds to the
* Amazon S3 bucket.
*
*
* -
*
* FULL_CONTROL
: The grantee has READ, READ_ACP, and WRITE_ACP permissions for the thumbnails that
* Elastic Transcoder adds to the Amazon S3 bucket.
*
*
*
*
*
*
* -
*
* StorageClass
: The Amazon S3 storage class, Standard
or ReducedRedundancy
,
* that you want Elastic Transcoder to assign to the thumbnails that it stores in your Amazon S3 bucket.
*
*
*
*
* @return Information about the Amazon S3 bucket in which you want Elastic Transcoder to save thumbnail files.
* Either you specify both ContentConfig
and ThumbnailConfig
, or you specify
* OutputBucket
.
*
* -
*
* Bucket
: The Amazon S3 bucket in which you want Elastic Transcoder to save thumbnail files.
*
*
* -
*
* Permissions
: A list of the users and/or predefined Amazon S3 groups you want to have access
* to thumbnail files, and the type of access that you want them to have.
*
*
* -
*
* GranteeType: The type of value that appears in the Grantee object:
*
*
* -
*
* Canonical
: Either the canonical user ID for an AWS account or an origin access identity for
* an Amazon CloudFront distribution.
*
*
*
* A canonical user ID is not the same as an AWS account number.
*
*
* -
*
* Email
: The registered email address of an AWS account.
*
*
* -
*
* Group
: One of the following predefined Amazon S3 groups: AllUsers
,
* AuthenticatedUsers
, or LogDelivery
.
*
*
*
*
* -
*
* Grantee
: The AWS user or group that you want to have access to thumbnail files.
*
*
* -
*
* Access: The permission that you want to give to the AWS user that is listed in Grantee. Valid values
* include:
*
*
* -
*
* READ
: The grantee can read the thumbnails and metadata for thumbnails that Elastic
* Transcoder adds to the Amazon S3 bucket.
*
*
* -
*
* READ_ACP
: The grantee can read the object ACL for thumbnails that Elastic Transcoder adds to
* the Amazon S3 bucket.
*
*
* -
*
* WRITE_ACP
: The grantee can write the ACL for the thumbnails that Elastic Transcoder adds to
* the Amazon S3 bucket.
*
*
* -
*
* FULL_CONTROL
: The grantee has READ, READ_ACP, and WRITE_ACP permissions for the thumbnails
* that Elastic Transcoder adds to the Amazon S3 bucket.
*
*
*
*
*
*
* -
*
* StorageClass
: The Amazon S3 storage class, Standard
or
* ReducedRedundancy
, that you want Elastic Transcoder to assign to the thumbnails that it
* stores in your Amazon S3 bucket.
*
*
*/
public final PipelineOutputConfig thumbnailConfig() {
return thumbnailConfig;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(id());
hashCode = 31 * hashCode + Objects.hashCode(arn());
hashCode = 31 * hashCode + Objects.hashCode(name());
hashCode = 31 * hashCode + Objects.hashCode(status());
hashCode = 31 * hashCode + Objects.hashCode(inputBucket());
hashCode = 31 * hashCode + Objects.hashCode(outputBucket());
hashCode = 31 * hashCode + Objects.hashCode(role());
hashCode = 31 * hashCode + Objects.hashCode(awsKmsKeyArn());
hashCode = 31 * hashCode + Objects.hashCode(notifications());
hashCode = 31 * hashCode + Objects.hashCode(contentConfig());
hashCode = 31 * hashCode + Objects.hashCode(thumbnailConfig());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof Pipeline)) {
return false;
}
Pipeline other = (Pipeline) obj;
return Objects.equals(id(), other.id()) && Objects.equals(arn(), other.arn()) && Objects.equals(name(), other.name())
&& Objects.equals(status(), other.status()) && Objects.equals(inputBucket(), other.inputBucket())
&& Objects.equals(outputBucket(), other.outputBucket()) && Objects.equals(role(), other.role())
&& Objects.equals(awsKmsKeyArn(), other.awsKmsKeyArn()) && Objects.equals(notifications(), other.notifications())
&& Objects.equals(contentConfig(), other.contentConfig())
&& Objects.equals(thumbnailConfig(), other.thumbnailConfig());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("Pipeline").add("Id", id()).add("Arn", arn()).add("Name", name()).add("Status", status())
.add("InputBucket", inputBucket()).add("OutputBucket", outputBucket()).add("Role", role())
.add("AwsKmsKeyArn", awsKmsKeyArn()).add("Notifications", notifications()).add("ContentConfig", contentConfig())
.add("ThumbnailConfig", thumbnailConfig()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "Id":
return Optional.ofNullable(clazz.cast(id()));
case "Arn":
return Optional.ofNullable(clazz.cast(arn()));
case "Name":
return Optional.ofNullable(clazz.cast(name()));
case "Status":
return Optional.ofNullable(clazz.cast(status()));
case "InputBucket":
return Optional.ofNullable(clazz.cast(inputBucket()));
case "OutputBucket":
return Optional.ofNullable(clazz.cast(outputBucket()));
case "Role":
return Optional.ofNullable(clazz.cast(role()));
case "AwsKmsKeyArn":
return Optional.ofNullable(clazz.cast(awsKmsKeyArn()));
case "Notifications":
return Optional.ofNullable(clazz.cast(notifications()));
case "ContentConfig":
return Optional.ofNullable(clazz.cast(contentConfig()));
case "ThumbnailConfig":
return Optional.ofNullable(clazz.cast(thumbnailConfig()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
private static Function