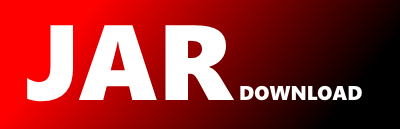
software.amazon.awssdk.services.elastictranscoder.model.JobInput Maven / Gradle / Ivy
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.elastictranscoder.model;
import java.io.Serializable;
import java.util.Arrays;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* Information about the file that you're transcoding.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class JobInput implements SdkPojo, Serializable, ToCopyableBuilder {
private static final SdkField KEY_FIELD = SdkField. builder(MarshallingType.STRING).memberName("Key")
.getter(getter(JobInput::key)).setter(setter(Builder::key))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Key").build()).build();
private static final SdkField FRAME_RATE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("FrameRate").getter(getter(JobInput::frameRate)).setter(setter(Builder::frameRate))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("FrameRate").build()).build();
private static final SdkField RESOLUTION_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("Resolution").getter(getter(JobInput::resolution)).setter(setter(Builder::resolution))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Resolution").build()).build();
private static final SdkField ASPECT_RATIO_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("AspectRatio").getter(getter(JobInput::aspectRatio)).setter(setter(Builder::aspectRatio))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("AspectRatio").build()).build();
private static final SdkField INTERLACED_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("Interlaced").getter(getter(JobInput::interlaced)).setter(setter(Builder::interlaced))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Interlaced").build()).build();
private static final SdkField CONTAINER_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("Container").getter(getter(JobInput::container)).setter(setter(Builder::container))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Container").build()).build();
private static final SdkField ENCRYPTION_FIELD = SdkField. builder(MarshallingType.SDK_POJO)
.memberName("Encryption").getter(getter(JobInput::encryption)).setter(setter(Builder::encryption))
.constructor(Encryption::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Encryption").build()).build();
private static final SdkField TIME_SPAN_FIELD = SdkField. builder(MarshallingType.SDK_POJO)
.memberName("TimeSpan").getter(getter(JobInput::timeSpan)).setter(setter(Builder::timeSpan))
.constructor(TimeSpan::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("TimeSpan").build()).build();
private static final SdkField INPUT_CAPTIONS_FIELD = SdkField
. builder(MarshallingType.SDK_POJO).memberName("InputCaptions")
.getter(getter(JobInput::inputCaptions)).setter(setter(Builder::inputCaptions)).constructor(InputCaptions::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("InputCaptions").build()).build();
private static final SdkField DETECTED_PROPERTIES_FIELD = SdkField
. builder(MarshallingType.SDK_POJO).memberName("DetectedProperties")
.getter(getter(JobInput::detectedProperties)).setter(setter(Builder::detectedProperties))
.constructor(DetectedProperties::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("DetectedProperties").build())
.build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(KEY_FIELD, FRAME_RATE_FIELD,
RESOLUTION_FIELD, ASPECT_RATIO_FIELD, INTERLACED_FIELD, CONTAINER_FIELD, ENCRYPTION_FIELD, TIME_SPAN_FIELD,
INPUT_CAPTIONS_FIELD, DETECTED_PROPERTIES_FIELD));
private static final long serialVersionUID = 1L;
private final String key;
private final String frameRate;
private final String resolution;
private final String aspectRatio;
private final String interlaced;
private final String container;
private final Encryption encryption;
private final TimeSpan timeSpan;
private final InputCaptions inputCaptions;
private final DetectedProperties detectedProperties;
private JobInput(BuilderImpl builder) {
this.key = builder.key;
this.frameRate = builder.frameRate;
this.resolution = builder.resolution;
this.aspectRatio = builder.aspectRatio;
this.interlaced = builder.interlaced;
this.container = builder.container;
this.encryption = builder.encryption;
this.timeSpan = builder.timeSpan;
this.inputCaptions = builder.inputCaptions;
this.detectedProperties = builder.detectedProperties;
}
/**
*
* The name of the file to transcode. Elsewhere in the body of the JSON block is the the ID of the pipeline to use
* for processing the job. The InputBucket
object in that pipeline tells Elastic Transcoder which
* Amazon S3 bucket to get the file from.
*
*
* If the file name includes a prefix, such as cooking/lasagna.mpg
, include the prefix in the key. If
* the file isn't in the specified bucket, Elastic Transcoder returns an error.
*
*
* @return The name of the file to transcode. Elsewhere in the body of the JSON block is the the ID of the pipeline
* to use for processing the job. The InputBucket
object in that pipeline tells Elastic
* Transcoder which Amazon S3 bucket to get the file from.
*
* If the file name includes a prefix, such as cooking/lasagna.mpg
, include the prefix in the
* key. If the file isn't in the specified bucket, Elastic Transcoder returns an error.
*/
public final String key() {
return key;
}
/**
*
* The frame rate of the input file. If you want Elastic Transcoder to automatically detect the frame rate of the
* input file, specify auto
. If you want to specify the frame rate for the input file, enter one of the
* following values:
*
*
* 10
, 15
, 23.97
, 24
, 25
, 29.97
,
* 30
, 60
*
*
* If you specify a value other than auto
, Elastic Transcoder disables automatic detection of the frame
* rate.
*
*
* @return The frame rate of the input file. If you want Elastic Transcoder to automatically detect the frame rate
* of the input file, specify auto
. If you want to specify the frame rate for the input file,
* enter one of the following values:
*
* 10
, 15
, 23.97
, 24
, 25
,
* 29.97
, 30
, 60
*
*
* If you specify a value other than auto
, Elastic Transcoder disables automatic detection of
* the frame rate.
*/
public final String frameRate() {
return frameRate;
}
/**
*
* This value must be auto
, which causes Elastic Transcoder to automatically detect the resolution of
* the input file.
*
*
* @return This value must be auto
, which causes Elastic Transcoder to automatically detect the
* resolution of the input file.
*/
public final String resolution() {
return resolution;
}
/**
*
* The aspect ratio of the input file. If you want Elastic Transcoder to automatically detect the aspect ratio of
* the input file, specify auto
. If you want to specify the aspect ratio for the output file, enter one
* of the following values:
*
*
* 1:1
, 4:3
, 3:2
, 16:9
*
*
* If you specify a value other than auto
, Elastic Transcoder disables automatic detection of the
* aspect ratio.
*
*
* @return The aspect ratio of the input file. If you want Elastic Transcoder to automatically detect the aspect
* ratio of the input file, specify auto
. If you want to specify the aspect ratio for the
* output file, enter one of the following values:
*
* 1:1
, 4:3
, 3:2
, 16:9
*
*
* If you specify a value other than auto
, Elastic Transcoder disables automatic detection of
* the aspect ratio.
*/
public final String aspectRatio() {
return aspectRatio;
}
/**
*
* Whether the input file is interlaced. If you want Elastic Transcoder to automatically detect whether the input
* file is interlaced, specify auto
. If you want to specify whether the input file is interlaced, enter
* one of the following values:
*
*
* true
, false
*
*
* If you specify a value other than auto
, Elastic Transcoder disables automatic detection of
* interlacing.
*
*
* @return Whether the input file is interlaced. If you want Elastic Transcoder to automatically detect whether the
* input file is interlaced, specify auto
. If you want to specify whether the input file is
* interlaced, enter one of the following values:
*
* true
, false
*
*
* If you specify a value other than auto
, Elastic Transcoder disables automatic detection of
* interlacing.
*/
public final String interlaced() {
return interlaced;
}
/**
*
* The container type for the input file. If you want Elastic Transcoder to automatically detect the container type
* of the input file, specify auto
. If you want to specify the container type for the input file, enter
* one of the following values:
*
*
* 3gp
, aac
, asf
, avi
, divx
, flv
,
* m4a
, mkv
, mov
, mp3
, mp4
, mpeg
,
* mpeg-ps
, mpeg-ts
, mxf
, ogg
, vob
,
* wav
, webm
*
*
* @return The container type for the input file. If you want Elastic Transcoder to automatically detect the
* container type of the input file, specify auto
. If you want to specify the container type
* for the input file, enter one of the following values:
*
* 3gp
, aac
, asf
, avi
, divx
,
* flv
, m4a
, mkv
, mov
, mp3
,
* mp4
, mpeg
, mpeg-ps
, mpeg-ts
, mxf
,
* ogg
, vob
, wav
, webm
*/
public final String container() {
return container;
}
/**
*
* The encryption settings, if any, that are used for decrypting your input files. If your input file is encrypted,
* you must specify the mode that Elastic Transcoder uses to decrypt your file.
*
*
* @return The encryption settings, if any, that are used for decrypting your input files. If your input file is
* encrypted, you must specify the mode that Elastic Transcoder uses to decrypt your file.
*/
public final Encryption encryption() {
return encryption;
}
/**
*
* Settings for clipping an input. Each input can have different clip settings.
*
*
* @return Settings for clipping an input. Each input can have different clip settings.
*/
public final TimeSpan timeSpan() {
return timeSpan;
}
/**
*
* You can configure Elastic Transcoder to transcode captions, or subtitles, from one format to another. All
* captions must be in UTF-8. Elastic Transcoder supports two types of captions:
*
*
* -
*
* Embedded: Embedded captions are included in the same file as the audio and video. Elastic Transcoder
* supports only one embedded caption per language, to a maximum of 300 embedded captions per file.
*
*
* Valid input values include: CEA-608 (EIA-608
, first non-empty channel only),
* CEA-708 (EIA-708
, first non-empty channel only), and mov-text
*
*
* Valid outputs include: mov-text
*
*
* Elastic Transcoder supports a maximum of one embedded format per output.
*
*
* -
*
* Sidecar: Sidecar captions are kept in a separate metadata file from the audio and video data. Sidecar
* captions require a player that is capable of understanding the relationship between the video file and the
* sidecar file. Elastic Transcoder supports only one sidecar caption per language, to a maximum of 20 sidecar
* captions per file.
*
*
* Valid input values include: dfxp
(first div element only), ebu-tt
, scc
,
* smpt
, srt
, ttml
(first div element only), and webvtt
*
*
* Valid outputs include: dfxp
(first div element only), scc
, srt
, and
* webvtt
.
*
*
*
*
* If you want ttml or smpte-tt compatible captions, specify dfxp as your output format.
*
*
* Elastic Transcoder does not support OCR (Optical Character Recognition), does not accept pictures as a valid
* input for captions, and is not available for audio-only transcoding. Elastic Transcoder does not preserve text
* formatting (for example, italics) during the transcoding process.
*
*
* To remove captions or leave the captions empty, set Captions
to null. To pass through existing
* captions unchanged, set the MergePolicy
to MergeRetain
, and pass in a null
* CaptionSources
array.
*
*
* For more information on embedded files, see the Subtitles Wikipedia page.
*
*
* For more information on sidecar files, see the Extensible Metadata Platform and Sidecar file Wikipedia pages.
*
*
* @return You can configure Elastic Transcoder to transcode captions, or subtitles, from one format to another. All
* captions must be in UTF-8. Elastic Transcoder supports two types of captions:
*
* -
*
* Embedded: Embedded captions are included in the same file as the audio and video. Elastic
* Transcoder supports only one embedded caption per language, to a maximum of 300 embedded captions per
* file.
*
*
* Valid input values include: CEA-608 (EIA-608
, first non-empty channel only),
* CEA-708 (EIA-708
, first non-empty channel only), and mov-text
*
*
* Valid outputs include: mov-text
*
*
* Elastic Transcoder supports a maximum of one embedded format per output.
*
*
* -
*
* Sidecar: Sidecar captions are kept in a separate metadata file from the audio and video data.
* Sidecar captions require a player that is capable of understanding the relationship between the video
* file and the sidecar file. Elastic Transcoder supports only one sidecar caption per language, to a
* maximum of 20 sidecar captions per file.
*
*
* Valid input values include: dfxp
(first div element only), ebu-tt
,
* scc
, smpt
, srt
, ttml
(first div element only), and
* webvtt
*
*
* Valid outputs include: dfxp
(first div element only), scc
, srt
,
* and webvtt
.
*
*
*
*
* If you want ttml or smpte-tt compatible captions, specify dfxp as your output format.
*
*
* Elastic Transcoder does not support OCR (Optical Character Recognition), does not accept pictures as a
* valid input for captions, and is not available for audio-only transcoding. Elastic Transcoder does not
* preserve text formatting (for example, italics) during the transcoding process.
*
*
* To remove captions or leave the captions empty, set Captions
to null. To pass through
* existing captions unchanged, set the MergePolicy
to MergeRetain
, and pass in a
* null CaptionSources
array.
*
*
* For more information on embedded files, see the Subtitles Wikipedia page.
*
*
* For more information on sidecar files, see the Extensible Metadata Platform and Sidecar file Wikipedia
* pages.
*/
public final InputCaptions inputCaptions() {
return inputCaptions;
}
/**
*
* The detected properties of the input file.
*
*
* @return The detected properties of the input file.
*/
public final DetectedProperties detectedProperties() {
return detectedProperties;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(key());
hashCode = 31 * hashCode + Objects.hashCode(frameRate());
hashCode = 31 * hashCode + Objects.hashCode(resolution());
hashCode = 31 * hashCode + Objects.hashCode(aspectRatio());
hashCode = 31 * hashCode + Objects.hashCode(interlaced());
hashCode = 31 * hashCode + Objects.hashCode(container());
hashCode = 31 * hashCode + Objects.hashCode(encryption());
hashCode = 31 * hashCode + Objects.hashCode(timeSpan());
hashCode = 31 * hashCode + Objects.hashCode(inputCaptions());
hashCode = 31 * hashCode + Objects.hashCode(detectedProperties());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof JobInput)) {
return false;
}
JobInput other = (JobInput) obj;
return Objects.equals(key(), other.key()) && Objects.equals(frameRate(), other.frameRate())
&& Objects.equals(resolution(), other.resolution()) && Objects.equals(aspectRatio(), other.aspectRatio())
&& Objects.equals(interlaced(), other.interlaced()) && Objects.equals(container(), other.container())
&& Objects.equals(encryption(), other.encryption()) && Objects.equals(timeSpan(), other.timeSpan())
&& Objects.equals(inputCaptions(), other.inputCaptions())
&& Objects.equals(detectedProperties(), other.detectedProperties());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("JobInput").add("Key", key()).add("FrameRate", frameRate()).add("Resolution", resolution())
.add("AspectRatio", aspectRatio()).add("Interlaced", interlaced()).add("Container", container())
.add("Encryption", encryption()).add("TimeSpan", timeSpan()).add("InputCaptions", inputCaptions())
.add("DetectedProperties", detectedProperties()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "Key":
return Optional.ofNullable(clazz.cast(key()));
case "FrameRate":
return Optional.ofNullable(clazz.cast(frameRate()));
case "Resolution":
return Optional.ofNullable(clazz.cast(resolution()));
case "AspectRatio":
return Optional.ofNullable(clazz.cast(aspectRatio()));
case "Interlaced":
return Optional.ofNullable(clazz.cast(interlaced()));
case "Container":
return Optional.ofNullable(clazz.cast(container()));
case "Encryption":
return Optional.ofNullable(clazz.cast(encryption()));
case "TimeSpan":
return Optional.ofNullable(clazz.cast(timeSpan()));
case "InputCaptions":
return Optional.ofNullable(clazz.cast(inputCaptions()));
case "DetectedProperties":
return Optional.ofNullable(clazz.cast(detectedProperties()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
private static Function