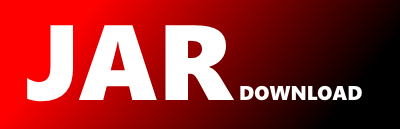
software.amazon.awssdk.services.emr.DefaultEmrAsyncClient Maven / Gradle / Ivy
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.emr;
import java.util.Collections;
import java.util.List;
import java.util.concurrent.CompletableFuture;
import java.util.concurrent.ScheduledExecutorService;
import java.util.function.Consumer;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.annotations.SdkInternalApi;
import software.amazon.awssdk.awscore.AwsRequestOverrideConfiguration;
import software.amazon.awssdk.awscore.client.handler.AwsAsyncClientHandler;
import software.amazon.awssdk.awscore.exception.AwsServiceException;
import software.amazon.awssdk.core.ApiName;
import software.amazon.awssdk.core.RequestOverrideConfiguration;
import software.amazon.awssdk.core.client.config.SdkClientConfiguration;
import software.amazon.awssdk.core.client.config.SdkClientOption;
import software.amazon.awssdk.core.client.handler.AsyncClientHandler;
import software.amazon.awssdk.core.client.handler.ClientExecutionParams;
import software.amazon.awssdk.core.http.HttpResponseHandler;
import software.amazon.awssdk.core.metrics.CoreMetric;
import software.amazon.awssdk.core.util.VersionInfo;
import software.amazon.awssdk.metrics.MetricCollector;
import software.amazon.awssdk.metrics.MetricPublisher;
import software.amazon.awssdk.metrics.NoOpMetricCollector;
import software.amazon.awssdk.protocols.core.ExceptionMetadata;
import software.amazon.awssdk.protocols.json.AwsJsonProtocol;
import software.amazon.awssdk.protocols.json.AwsJsonProtocolFactory;
import software.amazon.awssdk.protocols.json.BaseAwsJsonProtocolFactory;
import software.amazon.awssdk.protocols.json.JsonOperationMetadata;
import software.amazon.awssdk.services.emr.model.AddInstanceFleetRequest;
import software.amazon.awssdk.services.emr.model.AddInstanceFleetResponse;
import software.amazon.awssdk.services.emr.model.AddInstanceGroupsRequest;
import software.amazon.awssdk.services.emr.model.AddInstanceGroupsResponse;
import software.amazon.awssdk.services.emr.model.AddJobFlowStepsRequest;
import software.amazon.awssdk.services.emr.model.AddJobFlowStepsResponse;
import software.amazon.awssdk.services.emr.model.AddTagsRequest;
import software.amazon.awssdk.services.emr.model.AddTagsResponse;
import software.amazon.awssdk.services.emr.model.CancelStepsRequest;
import software.amazon.awssdk.services.emr.model.CancelStepsResponse;
import software.amazon.awssdk.services.emr.model.CreateSecurityConfigurationRequest;
import software.amazon.awssdk.services.emr.model.CreateSecurityConfigurationResponse;
import software.amazon.awssdk.services.emr.model.CreateStudioRequest;
import software.amazon.awssdk.services.emr.model.CreateStudioResponse;
import software.amazon.awssdk.services.emr.model.CreateStudioSessionMappingRequest;
import software.amazon.awssdk.services.emr.model.CreateStudioSessionMappingResponse;
import software.amazon.awssdk.services.emr.model.DeleteSecurityConfigurationRequest;
import software.amazon.awssdk.services.emr.model.DeleteSecurityConfigurationResponse;
import software.amazon.awssdk.services.emr.model.DeleteStudioRequest;
import software.amazon.awssdk.services.emr.model.DeleteStudioResponse;
import software.amazon.awssdk.services.emr.model.DeleteStudioSessionMappingRequest;
import software.amazon.awssdk.services.emr.model.DeleteStudioSessionMappingResponse;
import software.amazon.awssdk.services.emr.model.DescribeClusterRequest;
import software.amazon.awssdk.services.emr.model.DescribeClusterResponse;
import software.amazon.awssdk.services.emr.model.DescribeNotebookExecutionRequest;
import software.amazon.awssdk.services.emr.model.DescribeNotebookExecutionResponse;
import software.amazon.awssdk.services.emr.model.DescribeReleaseLabelRequest;
import software.amazon.awssdk.services.emr.model.DescribeReleaseLabelResponse;
import software.amazon.awssdk.services.emr.model.DescribeSecurityConfigurationRequest;
import software.amazon.awssdk.services.emr.model.DescribeSecurityConfigurationResponse;
import software.amazon.awssdk.services.emr.model.DescribeStepRequest;
import software.amazon.awssdk.services.emr.model.DescribeStepResponse;
import software.amazon.awssdk.services.emr.model.DescribeStudioRequest;
import software.amazon.awssdk.services.emr.model.DescribeStudioResponse;
import software.amazon.awssdk.services.emr.model.EmrException;
import software.amazon.awssdk.services.emr.model.EmrRequest;
import software.amazon.awssdk.services.emr.model.GetAutoTerminationPolicyRequest;
import software.amazon.awssdk.services.emr.model.GetAutoTerminationPolicyResponse;
import software.amazon.awssdk.services.emr.model.GetBlockPublicAccessConfigurationRequest;
import software.amazon.awssdk.services.emr.model.GetBlockPublicAccessConfigurationResponse;
import software.amazon.awssdk.services.emr.model.GetManagedScalingPolicyRequest;
import software.amazon.awssdk.services.emr.model.GetManagedScalingPolicyResponse;
import software.amazon.awssdk.services.emr.model.GetStudioSessionMappingRequest;
import software.amazon.awssdk.services.emr.model.GetStudioSessionMappingResponse;
import software.amazon.awssdk.services.emr.model.InternalServerErrorException;
import software.amazon.awssdk.services.emr.model.InternalServerException;
import software.amazon.awssdk.services.emr.model.InvalidRequestException;
import software.amazon.awssdk.services.emr.model.ListBootstrapActionsRequest;
import software.amazon.awssdk.services.emr.model.ListBootstrapActionsResponse;
import software.amazon.awssdk.services.emr.model.ListClustersRequest;
import software.amazon.awssdk.services.emr.model.ListClustersResponse;
import software.amazon.awssdk.services.emr.model.ListInstanceFleetsRequest;
import software.amazon.awssdk.services.emr.model.ListInstanceFleetsResponse;
import software.amazon.awssdk.services.emr.model.ListInstanceGroupsRequest;
import software.amazon.awssdk.services.emr.model.ListInstanceGroupsResponse;
import software.amazon.awssdk.services.emr.model.ListInstancesRequest;
import software.amazon.awssdk.services.emr.model.ListInstancesResponse;
import software.amazon.awssdk.services.emr.model.ListNotebookExecutionsRequest;
import software.amazon.awssdk.services.emr.model.ListNotebookExecutionsResponse;
import software.amazon.awssdk.services.emr.model.ListReleaseLabelsRequest;
import software.amazon.awssdk.services.emr.model.ListReleaseLabelsResponse;
import software.amazon.awssdk.services.emr.model.ListSecurityConfigurationsRequest;
import software.amazon.awssdk.services.emr.model.ListSecurityConfigurationsResponse;
import software.amazon.awssdk.services.emr.model.ListStepsRequest;
import software.amazon.awssdk.services.emr.model.ListStepsResponse;
import software.amazon.awssdk.services.emr.model.ListStudioSessionMappingsRequest;
import software.amazon.awssdk.services.emr.model.ListStudioSessionMappingsResponse;
import software.amazon.awssdk.services.emr.model.ListStudiosRequest;
import software.amazon.awssdk.services.emr.model.ListStudiosResponse;
import software.amazon.awssdk.services.emr.model.ModifyClusterRequest;
import software.amazon.awssdk.services.emr.model.ModifyClusterResponse;
import software.amazon.awssdk.services.emr.model.ModifyInstanceFleetRequest;
import software.amazon.awssdk.services.emr.model.ModifyInstanceFleetResponse;
import software.amazon.awssdk.services.emr.model.ModifyInstanceGroupsRequest;
import software.amazon.awssdk.services.emr.model.ModifyInstanceGroupsResponse;
import software.amazon.awssdk.services.emr.model.PutAutoScalingPolicyRequest;
import software.amazon.awssdk.services.emr.model.PutAutoScalingPolicyResponse;
import software.amazon.awssdk.services.emr.model.PutAutoTerminationPolicyRequest;
import software.amazon.awssdk.services.emr.model.PutAutoTerminationPolicyResponse;
import software.amazon.awssdk.services.emr.model.PutBlockPublicAccessConfigurationRequest;
import software.amazon.awssdk.services.emr.model.PutBlockPublicAccessConfigurationResponse;
import software.amazon.awssdk.services.emr.model.PutManagedScalingPolicyRequest;
import software.amazon.awssdk.services.emr.model.PutManagedScalingPolicyResponse;
import software.amazon.awssdk.services.emr.model.RemoveAutoScalingPolicyRequest;
import software.amazon.awssdk.services.emr.model.RemoveAutoScalingPolicyResponse;
import software.amazon.awssdk.services.emr.model.RemoveAutoTerminationPolicyRequest;
import software.amazon.awssdk.services.emr.model.RemoveAutoTerminationPolicyResponse;
import software.amazon.awssdk.services.emr.model.RemoveManagedScalingPolicyRequest;
import software.amazon.awssdk.services.emr.model.RemoveManagedScalingPolicyResponse;
import software.amazon.awssdk.services.emr.model.RemoveTagsRequest;
import software.amazon.awssdk.services.emr.model.RemoveTagsResponse;
import software.amazon.awssdk.services.emr.model.RunJobFlowRequest;
import software.amazon.awssdk.services.emr.model.RunJobFlowResponse;
import software.amazon.awssdk.services.emr.model.SetTerminationProtectionRequest;
import software.amazon.awssdk.services.emr.model.SetTerminationProtectionResponse;
import software.amazon.awssdk.services.emr.model.SetVisibleToAllUsersRequest;
import software.amazon.awssdk.services.emr.model.SetVisibleToAllUsersResponse;
import software.amazon.awssdk.services.emr.model.StartNotebookExecutionRequest;
import software.amazon.awssdk.services.emr.model.StartNotebookExecutionResponse;
import software.amazon.awssdk.services.emr.model.StopNotebookExecutionRequest;
import software.amazon.awssdk.services.emr.model.StopNotebookExecutionResponse;
import software.amazon.awssdk.services.emr.model.TerminateJobFlowsRequest;
import software.amazon.awssdk.services.emr.model.TerminateJobFlowsResponse;
import software.amazon.awssdk.services.emr.model.UpdateStudioRequest;
import software.amazon.awssdk.services.emr.model.UpdateStudioResponse;
import software.amazon.awssdk.services.emr.model.UpdateStudioSessionMappingRequest;
import software.amazon.awssdk.services.emr.model.UpdateStudioSessionMappingResponse;
import software.amazon.awssdk.services.emr.paginators.ListBootstrapActionsPublisher;
import software.amazon.awssdk.services.emr.paginators.ListClustersPublisher;
import software.amazon.awssdk.services.emr.paginators.ListInstanceFleetsPublisher;
import software.amazon.awssdk.services.emr.paginators.ListInstanceGroupsPublisher;
import software.amazon.awssdk.services.emr.paginators.ListInstancesPublisher;
import software.amazon.awssdk.services.emr.paginators.ListNotebookExecutionsPublisher;
import software.amazon.awssdk.services.emr.paginators.ListReleaseLabelsPublisher;
import software.amazon.awssdk.services.emr.paginators.ListSecurityConfigurationsPublisher;
import software.amazon.awssdk.services.emr.paginators.ListStepsPublisher;
import software.amazon.awssdk.services.emr.paginators.ListStudioSessionMappingsPublisher;
import software.amazon.awssdk.services.emr.paginators.ListStudiosPublisher;
import software.amazon.awssdk.services.emr.transform.AddInstanceFleetRequestMarshaller;
import software.amazon.awssdk.services.emr.transform.AddInstanceGroupsRequestMarshaller;
import software.amazon.awssdk.services.emr.transform.AddJobFlowStepsRequestMarshaller;
import software.amazon.awssdk.services.emr.transform.AddTagsRequestMarshaller;
import software.amazon.awssdk.services.emr.transform.CancelStepsRequestMarshaller;
import software.amazon.awssdk.services.emr.transform.CreateSecurityConfigurationRequestMarshaller;
import software.amazon.awssdk.services.emr.transform.CreateStudioRequestMarshaller;
import software.amazon.awssdk.services.emr.transform.CreateStudioSessionMappingRequestMarshaller;
import software.amazon.awssdk.services.emr.transform.DeleteSecurityConfigurationRequestMarshaller;
import software.amazon.awssdk.services.emr.transform.DeleteStudioRequestMarshaller;
import software.amazon.awssdk.services.emr.transform.DeleteStudioSessionMappingRequestMarshaller;
import software.amazon.awssdk.services.emr.transform.DescribeClusterRequestMarshaller;
import software.amazon.awssdk.services.emr.transform.DescribeNotebookExecutionRequestMarshaller;
import software.amazon.awssdk.services.emr.transform.DescribeReleaseLabelRequestMarshaller;
import software.amazon.awssdk.services.emr.transform.DescribeSecurityConfigurationRequestMarshaller;
import software.amazon.awssdk.services.emr.transform.DescribeStepRequestMarshaller;
import software.amazon.awssdk.services.emr.transform.DescribeStudioRequestMarshaller;
import software.amazon.awssdk.services.emr.transform.GetAutoTerminationPolicyRequestMarshaller;
import software.amazon.awssdk.services.emr.transform.GetBlockPublicAccessConfigurationRequestMarshaller;
import software.amazon.awssdk.services.emr.transform.GetManagedScalingPolicyRequestMarshaller;
import software.amazon.awssdk.services.emr.transform.GetStudioSessionMappingRequestMarshaller;
import software.amazon.awssdk.services.emr.transform.ListBootstrapActionsRequestMarshaller;
import software.amazon.awssdk.services.emr.transform.ListClustersRequestMarshaller;
import software.amazon.awssdk.services.emr.transform.ListInstanceFleetsRequestMarshaller;
import software.amazon.awssdk.services.emr.transform.ListInstanceGroupsRequestMarshaller;
import software.amazon.awssdk.services.emr.transform.ListInstancesRequestMarshaller;
import software.amazon.awssdk.services.emr.transform.ListNotebookExecutionsRequestMarshaller;
import software.amazon.awssdk.services.emr.transform.ListReleaseLabelsRequestMarshaller;
import software.amazon.awssdk.services.emr.transform.ListSecurityConfigurationsRequestMarshaller;
import software.amazon.awssdk.services.emr.transform.ListStepsRequestMarshaller;
import software.amazon.awssdk.services.emr.transform.ListStudioSessionMappingsRequestMarshaller;
import software.amazon.awssdk.services.emr.transform.ListStudiosRequestMarshaller;
import software.amazon.awssdk.services.emr.transform.ModifyClusterRequestMarshaller;
import software.amazon.awssdk.services.emr.transform.ModifyInstanceFleetRequestMarshaller;
import software.amazon.awssdk.services.emr.transform.ModifyInstanceGroupsRequestMarshaller;
import software.amazon.awssdk.services.emr.transform.PutAutoScalingPolicyRequestMarshaller;
import software.amazon.awssdk.services.emr.transform.PutAutoTerminationPolicyRequestMarshaller;
import software.amazon.awssdk.services.emr.transform.PutBlockPublicAccessConfigurationRequestMarshaller;
import software.amazon.awssdk.services.emr.transform.PutManagedScalingPolicyRequestMarshaller;
import software.amazon.awssdk.services.emr.transform.RemoveAutoScalingPolicyRequestMarshaller;
import software.amazon.awssdk.services.emr.transform.RemoveAutoTerminationPolicyRequestMarshaller;
import software.amazon.awssdk.services.emr.transform.RemoveManagedScalingPolicyRequestMarshaller;
import software.amazon.awssdk.services.emr.transform.RemoveTagsRequestMarshaller;
import software.amazon.awssdk.services.emr.transform.RunJobFlowRequestMarshaller;
import software.amazon.awssdk.services.emr.transform.SetTerminationProtectionRequestMarshaller;
import software.amazon.awssdk.services.emr.transform.SetVisibleToAllUsersRequestMarshaller;
import software.amazon.awssdk.services.emr.transform.StartNotebookExecutionRequestMarshaller;
import software.amazon.awssdk.services.emr.transform.StopNotebookExecutionRequestMarshaller;
import software.amazon.awssdk.services.emr.transform.TerminateJobFlowsRequestMarshaller;
import software.amazon.awssdk.services.emr.transform.UpdateStudioRequestMarshaller;
import software.amazon.awssdk.services.emr.transform.UpdateStudioSessionMappingRequestMarshaller;
import software.amazon.awssdk.services.emr.waiters.EmrAsyncWaiter;
import software.amazon.awssdk.utils.CompletableFutureUtils;
/**
* Internal implementation of {@link EmrAsyncClient}.
*
* @see EmrAsyncClient#builder()
*/
@Generated("software.amazon.awssdk:codegen")
@SdkInternalApi
final class DefaultEmrAsyncClient implements EmrAsyncClient {
private static final Logger log = LoggerFactory.getLogger(DefaultEmrAsyncClient.class);
private final AsyncClientHandler clientHandler;
private final AwsJsonProtocolFactory protocolFactory;
private final SdkClientConfiguration clientConfiguration;
private final ScheduledExecutorService executorService;
protected DefaultEmrAsyncClient(SdkClientConfiguration clientConfiguration) {
this.clientHandler = new AwsAsyncClientHandler(clientConfiguration);
this.clientConfiguration = clientConfiguration;
this.protocolFactory = init(AwsJsonProtocolFactory.builder()).build();
this.executorService = clientConfiguration.option(SdkClientOption.SCHEDULED_EXECUTOR_SERVICE);
}
@Override
public final String serviceName() {
return SERVICE_NAME;
}
/**
*
* Adds an instance fleet to a running cluster.
*
*
*
* The instance fleet configuration is available only in Amazon EMR versions 4.8.0 and later, excluding 5.0.x.
*
*
*
* @param addInstanceFleetRequest
* @return A Java Future containing the result of the AddInstanceFleet operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InternalServerException This exception occurs when there is an internal failure in the Amazon EMR
* service.
* - InvalidRequestException This exception occurs when there is something wrong with user input.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EmrException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EmrAsyncClient.AddInstanceFleet
* @see AWS API Documentation
*/
@Override
public CompletableFuture addInstanceFleet(AddInstanceFleetRequest addInstanceFleetRequest) {
List metricPublishers = resolveMetricPublishers(clientConfiguration, addInstanceFleetRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "EMR");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "AddInstanceFleet");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, AddInstanceFleetResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("AddInstanceFleet")
.withMarshaller(new AddInstanceFleetRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withMetricCollector(apiCallMetricCollector).withInput(addInstanceFleetRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Adds one or more instance groups to a running cluster.
*
*
* @param addInstanceGroupsRequest
* Input to an AddInstanceGroups call.
* @return A Java Future containing the result of the AddInstanceGroups operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InternalServerErrorException Indicates that an error occurred while processing the request and that
* the request was not completed.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EmrException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EmrAsyncClient.AddInstanceGroups
* @see AWS API Documentation
*/
@Override
public CompletableFuture addInstanceGroups(AddInstanceGroupsRequest addInstanceGroupsRequest) {
List metricPublishers = resolveMetricPublishers(clientConfiguration, addInstanceGroupsRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "EMR");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "AddInstanceGroups");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, AddInstanceGroupsResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("AddInstanceGroups")
.withMarshaller(new AddInstanceGroupsRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withMetricCollector(apiCallMetricCollector).withInput(addInstanceGroupsRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* AddJobFlowSteps adds new steps to a running cluster. A maximum of 256 steps are allowed in each job flow.
*
*
* If your cluster is long-running (such as a Hive data warehouse) or complex, you may require more than 256 steps
* to process your data. You can bypass the 256-step limitation in various ways, including using SSH to connect to
* the master node and submitting queries directly to the software running on the master node, such as Hive and
* Hadoop. For more information on how to do this, see Add More than 256 Steps to
* a Cluster in the Amazon EMR Management Guide.
*
*
* A step specifies the location of a JAR file stored either on the master node of the cluster or in Amazon S3. Each
* step is performed by the main function of the main class of the JAR file. The main class can be specified either
* in the manifest of the JAR or by using the MainFunction parameter of the step.
*
*
* Amazon EMR executes each step in the order listed. For a step to be considered complete, the main function must
* exit with a zero exit code and all Hadoop jobs started while the step was running must have completed and run
* successfully.
*
*
* You can only add steps to a cluster that is in one of the following states: STARTING, BOOTSTRAPPING, RUNNING, or
* WAITING.
*
*
*
* The string values passed into HadoopJarStep
object cannot exceed a total of 10240 characters.
*
*
*
* @param addJobFlowStepsRequest
* The input argument to the AddJobFlowSteps operation.
* @return A Java Future containing the result of the AddJobFlowSteps operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InternalServerErrorException Indicates that an error occurred while processing the request and that
* the request was not completed.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EmrException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EmrAsyncClient.AddJobFlowSteps
* @see AWS API Documentation
*/
@Override
public CompletableFuture addJobFlowSteps(AddJobFlowStepsRequest addJobFlowStepsRequest) {
List metricPublishers = resolveMetricPublishers(clientConfiguration, addJobFlowStepsRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "EMR");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "AddJobFlowSteps");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, AddJobFlowStepsResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("AddJobFlowSteps")
.withMarshaller(new AddJobFlowStepsRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withMetricCollector(apiCallMetricCollector).withInput(addJobFlowStepsRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Adds tags to an Amazon EMR resource, such as a cluster or an Amazon EMR Studio. Tags make it easier to associate
* resources in various ways, such as grouping clusters to track your Amazon EMR resource allocation costs. For more
* information, see Tag
* Clusters.
*
*
* @param addTagsRequest
* This input identifies an Amazon EMR resource and a list of tags to attach.
* @return A Java Future containing the result of the AddTags operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InternalServerException This exception occurs when there is an internal failure in the Amazon EMR
* service.
* - InvalidRequestException This exception occurs when there is something wrong with user input.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EmrException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EmrAsyncClient.AddTags
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture addTags(AddTagsRequest addTagsRequest) {
List metricPublishers = resolveMetricPublishers(clientConfiguration, addTagsRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "EMR");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "AddTags");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
AddTagsResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams().withOperationName("AddTags")
.withMarshaller(new AddTagsRequestMarshaller(protocolFactory)).withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withMetricCollector(apiCallMetricCollector)
.withInput(addTagsRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Cancels a pending step or steps in a running cluster. Available only in Amazon EMR versions 4.8.0 and later,
* excluding version 5.0.0. A maximum of 256 steps are allowed in each CancelSteps request. CancelSteps is
* idempotent but asynchronous; it does not guarantee that a step will be canceled, even if the request is
* successfully submitted. When you use Amazon EMR versions 5.28.0 and later, you can cancel steps that are in a
* PENDING
or RUNNING
state. In earlier versions of Amazon EMR, you can only cancel steps
* that are in a PENDING
state.
*
*
* @param cancelStepsRequest
* The input argument to the CancelSteps operation.
* @return A Java Future containing the result of the CancelSteps operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InternalServerErrorException Indicates that an error occurred while processing the request and that
* the request was not completed.
* - InvalidRequestException This exception occurs when there is something wrong with user input.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EmrException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EmrAsyncClient.CancelSteps
* @see AWS
* API Documentation
*/
@Override
public CompletableFuture cancelSteps(CancelStepsRequest cancelStepsRequest) {
List metricPublishers = resolveMetricPublishers(clientConfiguration, cancelStepsRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "EMR");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "CancelSteps");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
CancelStepsResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("CancelSteps").withMarshaller(new CancelStepsRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withMetricCollector(apiCallMetricCollector).withInput(cancelStepsRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Creates a security configuration, which is stored in the service and can be specified when a cluster is created.
*
*
* @param createSecurityConfigurationRequest
* @return A Java Future containing the result of the CreateSecurityConfiguration operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InternalServerException This exception occurs when there is an internal failure in the Amazon EMR
* service.
* - InvalidRequestException This exception occurs when there is something wrong with user input.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EmrException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EmrAsyncClient.CreateSecurityConfiguration
* @see AWS API Documentation
*/
@Override
public CompletableFuture createSecurityConfiguration(
CreateSecurityConfigurationRequest createSecurityConfigurationRequest) {
List metricPublishers = resolveMetricPublishers(clientConfiguration, createSecurityConfigurationRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "EMR");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "CreateSecurityConfiguration");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, CreateSecurityConfigurationResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("CreateSecurityConfiguration")
.withMarshaller(new CreateSecurityConfigurationRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withMetricCollector(apiCallMetricCollector).withInput(createSecurityConfigurationRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Creates a new Amazon EMR Studio.
*
*
* @param createStudioRequest
* @return A Java Future containing the result of the CreateStudio operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InternalServerException This exception occurs when there is an internal failure in the Amazon EMR
* service.
* - InvalidRequestException This exception occurs when there is something wrong with user input.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EmrException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EmrAsyncClient.CreateStudio
* @see AWS
* API Documentation
*/
@Override
public CompletableFuture createStudio(CreateStudioRequest createStudioRequest) {
List metricPublishers = resolveMetricPublishers(clientConfiguration, createStudioRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "EMR");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "CreateStudio");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
CreateStudioResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("CreateStudio").withMarshaller(new CreateStudioRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withMetricCollector(apiCallMetricCollector).withInput(createStudioRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Maps a user or group to the Amazon EMR Studio specified by StudioId
, and applies a session policy to
* refine Studio permissions for that user or group. Use CreateStudioSessionMapping
to assign users to
* a Studio when you use Amazon Web Services SSO authentication. For instructions on how to assign users to a Studio
* when you use IAM authentication, see Assign a user or group to your EMR Studio.
*
*
* @param createStudioSessionMappingRequest
* @return A Java Future containing the result of the CreateStudioSessionMapping operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InternalServerErrorException Indicates that an error occurred while processing the request and that
* the request was not completed.
* - InvalidRequestException This exception occurs when there is something wrong with user input.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EmrException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EmrAsyncClient.CreateStudioSessionMapping
* @see AWS API Documentation
*/
@Override
public CompletableFuture createStudioSessionMapping(
CreateStudioSessionMappingRequest createStudioSessionMappingRequest) {
List metricPublishers = resolveMetricPublishers(clientConfiguration, createStudioSessionMappingRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "EMR");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "CreateStudioSessionMapping");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, CreateStudioSessionMappingResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("CreateStudioSessionMapping")
.withMarshaller(new CreateStudioSessionMappingRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withMetricCollector(apiCallMetricCollector).withInput(createStudioSessionMappingRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Deletes a security configuration.
*
*
* @param deleteSecurityConfigurationRequest
* @return A Java Future containing the result of the DeleteSecurityConfiguration operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InternalServerException This exception occurs when there is an internal failure in the Amazon EMR
* service.
* - InvalidRequestException This exception occurs when there is something wrong with user input.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EmrException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EmrAsyncClient.DeleteSecurityConfiguration
* @see AWS API Documentation
*/
@Override
public CompletableFuture deleteSecurityConfiguration(
DeleteSecurityConfigurationRequest deleteSecurityConfigurationRequest) {
List metricPublishers = resolveMetricPublishers(clientConfiguration, deleteSecurityConfigurationRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "EMR");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DeleteSecurityConfiguration");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, DeleteSecurityConfigurationResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DeleteSecurityConfiguration")
.withMarshaller(new DeleteSecurityConfigurationRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withMetricCollector(apiCallMetricCollector).withInput(deleteSecurityConfigurationRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Removes an Amazon EMR Studio from the Studio metadata store.
*
*
* @param deleteStudioRequest
* @return A Java Future containing the result of the DeleteStudio operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InternalServerException This exception occurs when there is an internal failure in the Amazon EMR
* service.
* - InvalidRequestException This exception occurs when there is something wrong with user input.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EmrException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EmrAsyncClient.DeleteStudio
* @see AWS
* API Documentation
*/
@Override
public CompletableFuture deleteStudio(DeleteStudioRequest deleteStudioRequest) {
List metricPublishers = resolveMetricPublishers(clientConfiguration, deleteStudioRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "EMR");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DeleteStudio");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
DeleteStudioResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DeleteStudio").withMarshaller(new DeleteStudioRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withMetricCollector(apiCallMetricCollector).withInput(deleteStudioRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Removes a user or group from an Amazon EMR Studio.
*
*
* @param deleteStudioSessionMappingRequest
* @return A Java Future containing the result of the DeleteStudioSessionMapping operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InternalServerErrorException Indicates that an error occurred while processing the request and that
* the request was not completed.
* - InvalidRequestException This exception occurs when there is something wrong with user input.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EmrException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EmrAsyncClient.DeleteStudioSessionMapping
* @see AWS API Documentation
*/
@Override
public CompletableFuture deleteStudioSessionMapping(
DeleteStudioSessionMappingRequest deleteStudioSessionMappingRequest) {
List metricPublishers = resolveMetricPublishers(clientConfiguration, deleteStudioSessionMappingRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "EMR");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DeleteStudioSessionMapping");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, DeleteStudioSessionMappingResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DeleteStudioSessionMapping")
.withMarshaller(new DeleteStudioSessionMappingRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withMetricCollector(apiCallMetricCollector).withInput(deleteStudioSessionMappingRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Provides cluster-level details including status, hardware and software configuration, VPC settings, and so on.
*
*
* @param describeClusterRequest
* This input determines which cluster to describe.
* @return A Java Future containing the result of the DescribeCluster operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InternalServerException This exception occurs when there is an internal failure in the Amazon EMR
* service.
* - InvalidRequestException This exception occurs when there is something wrong with user input.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EmrException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EmrAsyncClient.DescribeCluster
* @see AWS API Documentation
*/
@Override
public CompletableFuture describeCluster(DescribeClusterRequest describeClusterRequest) {
List metricPublishers = resolveMetricPublishers(clientConfiguration, describeClusterRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "EMR");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DescribeCluster");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, DescribeClusterResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DescribeCluster")
.withMarshaller(new DescribeClusterRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withMetricCollector(apiCallMetricCollector).withInput(describeClusterRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Provides details of a notebook execution.
*
*
* @param describeNotebookExecutionRequest
* @return A Java Future containing the result of the DescribeNotebookExecution operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InternalServerErrorException Indicates that an error occurred while processing the request and that
* the request was not completed.
* - InvalidRequestException This exception occurs when there is something wrong with user input.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EmrException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EmrAsyncClient.DescribeNotebookExecution
* @see AWS API Documentation
*/
@Override
public CompletableFuture describeNotebookExecution(
DescribeNotebookExecutionRequest describeNotebookExecutionRequest) {
List metricPublishers = resolveMetricPublishers(clientConfiguration, describeNotebookExecutionRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "EMR");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DescribeNotebookExecution");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, DescribeNotebookExecutionResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DescribeNotebookExecution")
.withMarshaller(new DescribeNotebookExecutionRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withMetricCollector(apiCallMetricCollector).withInput(describeNotebookExecutionRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Provides EMR release label details, such as releases available the region where the API request is run, and the
* available applications for a specific EMR release label. Can also list EMR release versions that support a
* specified version of Spark.
*
*
* @param describeReleaseLabelRequest
* @return A Java Future containing the result of the DescribeReleaseLabel operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InternalServerException This exception occurs when there is an internal failure in the Amazon EMR
* service.
* - InvalidRequestException This exception occurs when there is something wrong with user input.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EmrException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EmrAsyncClient.DescribeReleaseLabel
* @see AWS API Documentation
*/
@Override
public CompletableFuture describeReleaseLabel(
DescribeReleaseLabelRequest describeReleaseLabelRequest) {
List metricPublishers = resolveMetricPublishers(clientConfiguration, describeReleaseLabelRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "EMR");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DescribeReleaseLabel");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, DescribeReleaseLabelResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DescribeReleaseLabel")
.withMarshaller(new DescribeReleaseLabelRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withMetricCollector(apiCallMetricCollector).withInput(describeReleaseLabelRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Provides the details of a security configuration by returning the configuration JSON.
*
*
* @param describeSecurityConfigurationRequest
* @return A Java Future containing the result of the DescribeSecurityConfiguration operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InternalServerException This exception occurs when there is an internal failure in the Amazon EMR
* service.
* - InvalidRequestException This exception occurs when there is something wrong with user input.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EmrException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EmrAsyncClient.DescribeSecurityConfiguration
* @see AWS API Documentation
*/
@Override
public CompletableFuture describeSecurityConfiguration(
DescribeSecurityConfigurationRequest describeSecurityConfigurationRequest) {
List metricPublishers = resolveMetricPublishers(clientConfiguration,
describeSecurityConfigurationRequest.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "EMR");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DescribeSecurityConfiguration");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, DescribeSecurityConfigurationResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DescribeSecurityConfiguration")
.withMarshaller(new DescribeSecurityConfigurationRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withMetricCollector(apiCallMetricCollector).withInput(describeSecurityConfigurationRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Provides more detail about the cluster step.
*
*
* @param describeStepRequest
* This input determines which step to describe.
* @return A Java Future containing the result of the DescribeStep operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InternalServerException This exception occurs when there is an internal failure in the Amazon EMR
* service.
* - InvalidRequestException This exception occurs when there is something wrong with user input.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EmrException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EmrAsyncClient.DescribeStep
* @see AWS
* API Documentation
*/
@Override
public CompletableFuture describeStep(DescribeStepRequest describeStepRequest) {
List metricPublishers = resolveMetricPublishers(clientConfiguration, describeStepRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "EMR");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DescribeStep");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
DescribeStepResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DescribeStep").withMarshaller(new DescribeStepRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withMetricCollector(apiCallMetricCollector).withInput(describeStepRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Returns details for the specified Amazon EMR Studio including ID, Name, VPC, Studio access URL, and so on.
*
*
* @param describeStudioRequest
* @return A Java Future containing the result of the DescribeStudio operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InternalServerException This exception occurs when there is an internal failure in the Amazon EMR
* service.
* - InvalidRequestException This exception occurs when there is something wrong with user input.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EmrException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EmrAsyncClient.DescribeStudio
* @see AWS API Documentation
*/
@Override
public CompletableFuture describeStudio(DescribeStudioRequest describeStudioRequest) {
List metricPublishers = resolveMetricPublishers(clientConfiguration, describeStudioRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "EMR");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DescribeStudio");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, DescribeStudioResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DescribeStudio")
.withMarshaller(new DescribeStudioRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withMetricCollector(apiCallMetricCollector).withInput(describeStudioRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Returns the auto-termination policy for an Amazon EMR cluster.
*
*
* @param getAutoTerminationPolicyRequest
* @return A Java Future containing the result of the GetAutoTerminationPolicy operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EmrException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EmrAsyncClient.GetAutoTerminationPolicy
* @see AWS API Documentation
*/
@Override
public CompletableFuture getAutoTerminationPolicy(
GetAutoTerminationPolicyRequest getAutoTerminationPolicyRequest) {
List metricPublishers = resolveMetricPublishers(clientConfiguration, getAutoTerminationPolicyRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "EMR");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "GetAutoTerminationPolicy");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, GetAutoTerminationPolicyResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("GetAutoTerminationPolicy")
.withMarshaller(new GetAutoTerminationPolicyRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withMetricCollector(apiCallMetricCollector).withInput(getAutoTerminationPolicyRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Returns the Amazon EMR block public access configuration for your Amazon Web Services account in the current
* Region. For more information see Configure Block
* Public Access for Amazon EMR in the Amazon EMR Management Guide.
*
*
* @param getBlockPublicAccessConfigurationRequest
* @return A Java Future containing the result of the GetBlockPublicAccessConfiguration operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InternalServerException This exception occurs when there is an internal failure in the Amazon EMR
* service.
* - InvalidRequestException This exception occurs when there is something wrong with user input.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EmrException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EmrAsyncClient.GetBlockPublicAccessConfiguration
* @see AWS API Documentation
*/
@Override
public CompletableFuture getBlockPublicAccessConfiguration(
GetBlockPublicAccessConfigurationRequest getBlockPublicAccessConfigurationRequest) {
List metricPublishers = resolveMetricPublishers(clientConfiguration,
getBlockPublicAccessConfigurationRequest.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "EMR");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "GetBlockPublicAccessConfiguration");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory
.createResponseHandler(operationMetadata, GetBlockPublicAccessConfigurationResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("GetBlockPublicAccessConfiguration")
.withMarshaller(new GetBlockPublicAccessConfigurationRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withMetricCollector(apiCallMetricCollector).withInput(getBlockPublicAccessConfigurationRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Fetches the attached managed scaling policy for an Amazon EMR cluster.
*
*
* @param getManagedScalingPolicyRequest
* @return A Java Future containing the result of the GetManagedScalingPolicy operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EmrException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EmrAsyncClient.GetManagedScalingPolicy
* @see AWS API Documentation
*/
@Override
public CompletableFuture getManagedScalingPolicy(
GetManagedScalingPolicyRequest getManagedScalingPolicyRequest) {
List metricPublishers = resolveMetricPublishers(clientConfiguration, getManagedScalingPolicyRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "EMR");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "GetManagedScalingPolicy");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, GetManagedScalingPolicyResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("GetManagedScalingPolicy")
.withMarshaller(new GetManagedScalingPolicyRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withMetricCollector(apiCallMetricCollector).withInput(getManagedScalingPolicyRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Fetches mapping details for the specified Amazon EMR Studio and identity (user or group).
*
*
* @param getStudioSessionMappingRequest
* @return A Java Future containing the result of the GetStudioSessionMapping operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InternalServerErrorException Indicates that an error occurred while processing the request and that
* the request was not completed.
* - InvalidRequestException This exception occurs when there is something wrong with user input.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EmrException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EmrAsyncClient.GetStudioSessionMapping
* @see AWS API Documentation
*/
@Override
public CompletableFuture getStudioSessionMapping(
GetStudioSessionMappingRequest getStudioSessionMappingRequest) {
List metricPublishers = resolveMetricPublishers(clientConfiguration, getStudioSessionMappingRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "EMR");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "GetStudioSessionMapping");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, GetStudioSessionMappingResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("GetStudioSessionMapping")
.withMarshaller(new GetStudioSessionMappingRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withMetricCollector(apiCallMetricCollector).withInput(getStudioSessionMappingRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Provides information about the bootstrap actions associated with a cluster.
*
*
* @param listBootstrapActionsRequest
* This input determines which bootstrap actions to retrieve.
* @return A Java Future containing the result of the ListBootstrapActions operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InternalServerException This exception occurs when there is an internal failure in the Amazon EMR
* service.
* - InvalidRequestException This exception occurs when there is something wrong with user input.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EmrException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EmrAsyncClient.ListBootstrapActions
* @see AWS API Documentation
*/
@Override
public CompletableFuture listBootstrapActions(
ListBootstrapActionsRequest listBootstrapActionsRequest) {
List metricPublishers = resolveMetricPublishers(clientConfiguration, listBootstrapActionsRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "EMR");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "ListBootstrapActions");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, ListBootstrapActionsResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("ListBootstrapActions")
.withMarshaller(new ListBootstrapActionsRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withMetricCollector(apiCallMetricCollector).withInput(listBootstrapActionsRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Provides information about the bootstrap actions associated with a cluster.
*
*
*
* This is a variant of
* {@link #listBootstrapActions(software.amazon.awssdk.services.emr.model.ListBootstrapActionsRequest)} operation.
* The return type is a custom publisher that can be subscribed to request a stream of response pages. SDK will
* internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.emr.paginators.ListBootstrapActionsPublisher publisher = client.listBootstrapActionsPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.emr.paginators.ListBootstrapActionsPublisher publisher = client.listBootstrapActionsPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.emr.model.ListBootstrapActionsResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of null won't limit the number of results you get with the paginator. It
* only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listBootstrapActions(software.amazon.awssdk.services.emr.model.ListBootstrapActionsRequest)}
* operation.
*
*
* @param listBootstrapActionsRequest
* This input determines which bootstrap actions to retrieve.
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InternalServerException This exception occurs when there is an internal failure in the Amazon EMR
* service.
* - InvalidRequestException This exception occurs when there is something wrong with user input.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EmrException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EmrAsyncClient.ListBootstrapActions
* @see AWS API Documentation
*/
public ListBootstrapActionsPublisher listBootstrapActionsPaginator(ListBootstrapActionsRequest listBootstrapActionsRequest) {
return new ListBootstrapActionsPublisher(this, applyPaginatorUserAgent(listBootstrapActionsRequest));
}
/**
*
* Provides the status of all clusters visible to this Amazon Web Services account. Allows you to filter the list of
* clusters based on certain criteria; for example, filtering by cluster creation date and time or by status. This
* call returns a maximum of 50 clusters in unsorted order per call, but returns a marker to track the paging of the
* cluster list across multiple ListClusters calls.
*
*
* @param listClustersRequest
* This input determines how the ListClusters action filters the list of clusters that it returns.
* @return A Java Future containing the result of the ListClusters operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InternalServerException This exception occurs when there is an internal failure in the Amazon EMR
* service.
* - InvalidRequestException This exception occurs when there is something wrong with user input.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EmrException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EmrAsyncClient.ListClusters
* @see AWS
* API Documentation
*/
@Override
public CompletableFuture listClusters(ListClustersRequest listClustersRequest) {
List metricPublishers = resolveMetricPublishers(clientConfiguration, listClustersRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "EMR");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "ListClusters");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
ListClustersResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("ListClusters").withMarshaller(new ListClustersRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withMetricCollector(apiCallMetricCollector).withInput(listClustersRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Provides the status of all clusters visible to this Amazon Web Services account. Allows you to filter the list of
* clusters based on certain criteria; for example, filtering by cluster creation date and time or by status. This
* call returns a maximum of 50 clusters in unsorted order per call, but returns a marker to track the paging of the
* cluster list across multiple ListClusters calls.
*
*
*
* This is a variant of {@link #listClusters(software.amazon.awssdk.services.emr.model.ListClustersRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.emr.paginators.ListClustersPublisher publisher = client.listClustersPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.emr.paginators.ListClustersPublisher publisher = client.listClustersPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.emr.model.ListClustersResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of null won't limit the number of results you get with the paginator. It
* only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listClusters(software.amazon.awssdk.services.emr.model.ListClustersRequest)} operation.
*
*
* @param listClustersRequest
* This input determines how the ListClusters action filters the list of clusters that it returns.
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InternalServerException This exception occurs when there is an internal failure in the Amazon EMR
* service.
* - InvalidRequestException This exception occurs when there is something wrong with user input.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EmrException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EmrAsyncClient.ListClusters
* @see AWS
* API Documentation
*/
public ListClustersPublisher listClustersPaginator(ListClustersRequest listClustersRequest) {
return new ListClustersPublisher(this, applyPaginatorUserAgent(listClustersRequest));
}
/**
*
* Lists all available details about the instance fleets in a cluster.
*
*
*
* The instance fleet configuration is available only in Amazon EMR versions 4.8.0 and later, excluding 5.0.x
* versions.
*
*
*
* @param listInstanceFleetsRequest
* @return A Java Future containing the result of the ListInstanceFleets operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InternalServerException This exception occurs when there is an internal failure in the Amazon EMR
* service.
* - InvalidRequestException This exception occurs when there is something wrong with user input.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EmrException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EmrAsyncClient.ListInstanceFleets
* @see AWS API Documentation
*/
@Override
public CompletableFuture listInstanceFleets(ListInstanceFleetsRequest listInstanceFleetsRequest) {
List metricPublishers = resolveMetricPublishers(clientConfiguration, listInstanceFleetsRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "EMR");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "ListInstanceFleets");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, ListInstanceFleetsResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("ListInstanceFleets")
.withMarshaller(new ListInstanceFleetsRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withMetricCollector(apiCallMetricCollector).withInput(listInstanceFleetsRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Lists all available details about the instance fleets in a cluster.
*
*
*
* The instance fleet configuration is available only in Amazon EMR versions 4.8.0 and later, excluding 5.0.x
* versions.
*
*
*
* This is a variant of
* {@link #listInstanceFleets(software.amazon.awssdk.services.emr.model.ListInstanceFleetsRequest)} operation. The
* return type is a custom publisher that can be subscribed to request a stream of response pages. SDK will
* internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.emr.paginators.ListInstanceFleetsPublisher publisher = client.listInstanceFleetsPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.emr.paginators.ListInstanceFleetsPublisher publisher = client.listInstanceFleetsPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.emr.model.ListInstanceFleetsResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of null won't limit the number of results you get with the paginator. It
* only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listInstanceFleets(software.amazon.awssdk.services.emr.model.ListInstanceFleetsRequest)} operation.
*
*
* @param listInstanceFleetsRequest
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InternalServerException This exception occurs when there is an internal failure in the Amazon EMR
* service.
* - InvalidRequestException This exception occurs when there is something wrong with user input.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EmrException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EmrAsyncClient.ListInstanceFleets
* @see AWS API Documentation
*/
public ListInstanceFleetsPublisher listInstanceFleetsPaginator(ListInstanceFleetsRequest listInstanceFleetsRequest) {
return new ListInstanceFleetsPublisher(this, applyPaginatorUserAgent(listInstanceFleetsRequest));
}
/**
*
* Provides all available details about the instance groups in a cluster.
*
*
* @param listInstanceGroupsRequest
* This input determines which instance groups to retrieve.
* @return A Java Future containing the result of the ListInstanceGroups operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InternalServerException This exception occurs when there is an internal failure in the Amazon EMR
* service.
* - InvalidRequestException This exception occurs when there is something wrong with user input.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EmrException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EmrAsyncClient.ListInstanceGroups
* @see AWS API Documentation
*/
@Override
public CompletableFuture listInstanceGroups(ListInstanceGroupsRequest listInstanceGroupsRequest) {
List metricPublishers = resolveMetricPublishers(clientConfiguration, listInstanceGroupsRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "EMR");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "ListInstanceGroups");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, ListInstanceGroupsResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("ListInstanceGroups")
.withMarshaller(new ListInstanceGroupsRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withMetricCollector(apiCallMetricCollector).withInput(listInstanceGroupsRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Provides all available details about the instance groups in a cluster.
*
*
*
* This is a variant of
* {@link #listInstanceGroups(software.amazon.awssdk.services.emr.model.ListInstanceGroupsRequest)} operation. The
* return type is a custom publisher that can be subscribed to request a stream of response pages. SDK will
* internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.emr.paginators.ListInstanceGroupsPublisher publisher = client.listInstanceGroupsPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.emr.paginators.ListInstanceGroupsPublisher publisher = client.listInstanceGroupsPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.emr.model.ListInstanceGroupsResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of null won't limit the number of results you get with the paginator. It
* only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listInstanceGroups(software.amazon.awssdk.services.emr.model.ListInstanceGroupsRequest)} operation.
*
*
* @param listInstanceGroupsRequest
* This input determines which instance groups to retrieve.
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InternalServerException This exception occurs when there is an internal failure in the Amazon EMR
* service.
* - InvalidRequestException This exception occurs when there is something wrong with user input.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EmrException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EmrAsyncClient.ListInstanceGroups
* @see AWS API Documentation
*/
public ListInstanceGroupsPublisher listInstanceGroupsPaginator(ListInstanceGroupsRequest listInstanceGroupsRequest) {
return new ListInstanceGroupsPublisher(this, applyPaginatorUserAgent(listInstanceGroupsRequest));
}
/**
*
* Provides information for all active EC2 instances and EC2 instances terminated in the last 30 days, up to a
* maximum of 2,000. EC2 instances in any of the following states are considered active: AWAITING_FULFILLMENT,
* PROVISIONING, BOOTSTRAPPING, RUNNING.
*
*
* @param listInstancesRequest
* This input determines which instances to list.
* @return A Java Future containing the result of the ListInstances operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InternalServerException This exception occurs when there is an internal failure in the Amazon EMR
* service.
* - InvalidRequestException This exception occurs when there is something wrong with user input.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EmrException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EmrAsyncClient.ListInstances
* @see AWS API Documentation
*/
@Override
public CompletableFuture listInstances(ListInstancesRequest listInstancesRequest) {
List metricPublishers = resolveMetricPublishers(clientConfiguration, listInstancesRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "EMR");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "ListInstances");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
ListInstancesResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("ListInstances")
.withMarshaller(new ListInstancesRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withMetricCollector(apiCallMetricCollector).withInput(listInstancesRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Provides information for all active EC2 instances and EC2 instances terminated in the last 30 days, up to a
* maximum of 2,000. EC2 instances in any of the following states are considered active: AWAITING_FULFILLMENT,
* PROVISIONING, BOOTSTRAPPING, RUNNING.
*
*
*
* This is a variant of {@link #listInstances(software.amazon.awssdk.services.emr.model.ListInstancesRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.emr.paginators.ListInstancesPublisher publisher = client.listInstancesPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.emr.paginators.ListInstancesPublisher publisher = client.listInstancesPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.emr.model.ListInstancesResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of null won't limit the number of results you get with the paginator. It
* only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listInstances(software.amazon.awssdk.services.emr.model.ListInstancesRequest)} operation.
*
*
* @param listInstancesRequest
* This input determines which instances to list.
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InternalServerException This exception occurs when there is an internal failure in the Amazon EMR
* service.
* - InvalidRequestException This exception occurs when there is something wrong with user input.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EmrException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EmrAsyncClient.ListInstances
* @see AWS API Documentation
*/
public ListInstancesPublisher listInstancesPaginator(ListInstancesRequest listInstancesRequest) {
return new ListInstancesPublisher(this, applyPaginatorUserAgent(listInstancesRequest));
}
/**
*
* Provides summaries of all notebook executions. You can filter the list based on multiple criteria such as status,
* time range, and editor id. Returns a maximum of 50 notebook executions and a marker to track the paging of a
* longer notebook execution list across multiple ListNotebookExecution
calls.
*
*
* @param listNotebookExecutionsRequest
* @return A Java Future containing the result of the ListNotebookExecutions operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InternalServerErrorException Indicates that an error occurred while processing the request and that
* the request was not completed.
* - InvalidRequestException This exception occurs when there is something wrong with user input.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EmrException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EmrAsyncClient.ListNotebookExecutions
* @see AWS API Documentation
*/
@Override
public CompletableFuture listNotebookExecutions(
ListNotebookExecutionsRequest listNotebookExecutionsRequest) {
List metricPublishers = resolveMetricPublishers(clientConfiguration, listNotebookExecutionsRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "EMR");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "ListNotebookExecutions");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, ListNotebookExecutionsResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("ListNotebookExecutions")
.withMarshaller(new ListNotebookExecutionsRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withMetricCollector(apiCallMetricCollector).withInput(listNotebookExecutionsRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Provides summaries of all notebook executions. You can filter the list based on multiple criteria such as status,
* time range, and editor id. Returns a maximum of 50 notebook executions and a marker to track the paging of a
* longer notebook execution list across multiple ListNotebookExecution
calls.
*
*
*
* This is a variant of
* {@link #listNotebookExecutions(software.amazon.awssdk.services.emr.model.ListNotebookExecutionsRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.emr.paginators.ListNotebookExecutionsPublisher publisher = client.listNotebookExecutionsPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.emr.paginators.ListNotebookExecutionsPublisher publisher = client.listNotebookExecutionsPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.emr.model.ListNotebookExecutionsResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of null won't limit the number of results you get with the paginator. It
* only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listNotebookExecutions(software.amazon.awssdk.services.emr.model.ListNotebookExecutionsRequest)}
* operation.
*
*
* @param listNotebookExecutionsRequest
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InternalServerErrorException Indicates that an error occurred while processing the request and that
* the request was not completed.
* - InvalidRequestException This exception occurs when there is something wrong with user input.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EmrException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EmrAsyncClient.ListNotebookExecutions
* @see AWS API Documentation
*/
public ListNotebookExecutionsPublisher listNotebookExecutionsPaginator(
ListNotebookExecutionsRequest listNotebookExecutionsRequest) {
return new ListNotebookExecutionsPublisher(this, applyPaginatorUserAgent(listNotebookExecutionsRequest));
}
/**
*
* Retrieves release labels of EMR services in the region where the API is called.
*
*
* @param listReleaseLabelsRequest
* @return A Java Future containing the result of the ListReleaseLabels operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InternalServerException This exception occurs when there is an internal failure in the Amazon EMR
* service.
* - InvalidRequestException This exception occurs when there is something wrong with user input.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EmrException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EmrAsyncClient.ListReleaseLabels
* @see AWS API Documentation
*/
@Override
public CompletableFuture listReleaseLabels(ListReleaseLabelsRequest listReleaseLabelsRequest) {
List metricPublishers = resolveMetricPublishers(clientConfiguration, listReleaseLabelsRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "EMR");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "ListReleaseLabels");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, ListReleaseLabelsResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("ListReleaseLabels")
.withMarshaller(new ListReleaseLabelsRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withMetricCollector(apiCallMetricCollector).withInput(listReleaseLabelsRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Retrieves release labels of EMR services in the region where the API is called.
*
*
*
* This is a variant of
* {@link #listReleaseLabels(software.amazon.awssdk.services.emr.model.ListReleaseLabelsRequest)} operation. The
* return type is a custom publisher that can be subscribed to request a stream of response pages. SDK will
* internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.emr.paginators.ListReleaseLabelsPublisher publisher = client.listReleaseLabelsPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.emr.paginators.ListReleaseLabelsPublisher publisher = client.listReleaseLabelsPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.emr.model.ListReleaseLabelsResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listReleaseLabels(software.amazon.awssdk.services.emr.model.ListReleaseLabelsRequest)} operation.
*
*
* @param listReleaseLabelsRequest
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InternalServerException This exception occurs when there is an internal failure in the Amazon EMR
* service.
* - InvalidRequestException This exception occurs when there is something wrong with user input.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EmrException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EmrAsyncClient.ListReleaseLabels
* @see AWS API Documentation
*/
public ListReleaseLabelsPublisher listReleaseLabelsPaginator(ListReleaseLabelsRequest listReleaseLabelsRequest) {
return new ListReleaseLabelsPublisher(this, applyPaginatorUserAgent(listReleaseLabelsRequest));
}
/**
*
* Lists all the security configurations visible to this account, providing their creation dates and times, and
* their names. This call returns a maximum of 50 clusters per call, but returns a marker to track the paging of the
* cluster list across multiple ListSecurityConfigurations calls.
*
*
* @param listSecurityConfigurationsRequest
* @return A Java Future containing the result of the ListSecurityConfigurations operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InternalServerException This exception occurs when there is an internal failure in the Amazon EMR
* service.
* - InvalidRequestException This exception occurs when there is something wrong with user input.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EmrException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EmrAsyncClient.ListSecurityConfigurations
* @see AWS API Documentation
*/
@Override
public CompletableFuture listSecurityConfigurations(
ListSecurityConfigurationsRequest listSecurityConfigurationsRequest) {
List metricPublishers = resolveMetricPublishers(clientConfiguration, listSecurityConfigurationsRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "EMR");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "ListSecurityConfigurations");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, ListSecurityConfigurationsResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("ListSecurityConfigurations")
.withMarshaller(new ListSecurityConfigurationsRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withMetricCollector(apiCallMetricCollector).withInput(listSecurityConfigurationsRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Lists all the security configurations visible to this account, providing their creation dates and times, and
* their names. This call returns a maximum of 50 clusters per call, but returns a marker to track the paging of the
* cluster list across multiple ListSecurityConfigurations calls.
*
*
*
* This is a variant of
* {@link #listSecurityConfigurations(software.amazon.awssdk.services.emr.model.ListSecurityConfigurationsRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.emr.paginators.ListSecurityConfigurationsPublisher publisher = client.listSecurityConfigurationsPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.emr.paginators.ListSecurityConfigurationsPublisher publisher = client.listSecurityConfigurationsPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.emr.model.ListSecurityConfigurationsResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of null won't limit the number of results you get with the paginator. It
* only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listSecurityConfigurations(software.amazon.awssdk.services.emr.model.ListSecurityConfigurationsRequest)}
* operation.
*
*
* @param listSecurityConfigurationsRequest
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InternalServerException This exception occurs when there is an internal failure in the Amazon EMR
* service.
* - InvalidRequestException This exception occurs when there is something wrong with user input.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EmrException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EmrAsyncClient.ListSecurityConfigurations
* @see AWS API Documentation
*/
public ListSecurityConfigurationsPublisher listSecurityConfigurationsPaginator(
ListSecurityConfigurationsRequest listSecurityConfigurationsRequest) {
return new ListSecurityConfigurationsPublisher(this, applyPaginatorUserAgent(listSecurityConfigurationsRequest));
}
/**
*
* Provides a list of steps for the cluster in reverse order unless you specify stepIds
with the
* request or filter by StepStates
. You can specify a maximum of 10 stepIDs
. The CLI
* automatically paginates results to return a list greater than 50 steps. To return more than 50 steps using the
* CLI, specify a Marker
, which is a pagination token that indicates the next set of steps to retrieve.
*
*
* @param listStepsRequest
* This input determines which steps to list.
* @return A Java Future containing the result of the ListSteps operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InternalServerException This exception occurs when there is an internal failure in the Amazon EMR
* service.
* - InvalidRequestException This exception occurs when there is something wrong with user input.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EmrException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EmrAsyncClient.ListSteps
* @see AWS
* API Documentation
*/
@Override
public CompletableFuture listSteps(ListStepsRequest listStepsRequest) {
List metricPublishers = resolveMetricPublishers(clientConfiguration, listStepsRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "EMR");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "ListSteps");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
ListStepsResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams().withOperationName("ListSteps")
.withMarshaller(new ListStepsRequestMarshaller(protocolFactory)).withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withMetricCollector(apiCallMetricCollector)
.withInput(listStepsRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Provides a list of steps for the cluster in reverse order unless you specify stepIds
with the
* request or filter by StepStates
. You can specify a maximum of 10 stepIDs
. The CLI
* automatically paginates results to return a list greater than 50 steps. To return more than 50 steps using the
* CLI, specify a Marker
, which is a pagination token that indicates the next set of steps to retrieve.
*
*
*
* This is a variant of {@link #listSteps(software.amazon.awssdk.services.emr.model.ListStepsRequest)} operation.
* The return type is a custom publisher that can be subscribed to request a stream of response pages. SDK will
* internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.emr.paginators.ListStepsPublisher publisher = client.listStepsPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.emr.paginators.ListStepsPublisher publisher = client.listStepsPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.emr.model.ListStepsResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of null won't limit the number of results you get with the paginator. It
* only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listSteps(software.amazon.awssdk.services.emr.model.ListStepsRequest)} operation.
*
*
* @param listStepsRequest
* This input determines which steps to list.
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InternalServerException This exception occurs when there is an internal failure in the Amazon EMR
* service.
* - InvalidRequestException This exception occurs when there is something wrong with user input.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EmrException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EmrAsyncClient.ListSteps
* @see AWS
* API Documentation
*/
public ListStepsPublisher listStepsPaginator(ListStepsRequest listStepsRequest) {
return new ListStepsPublisher(this, applyPaginatorUserAgent(listStepsRequest));
}
/**
*
* Returns a list of all user or group session mappings for the Amazon EMR Studio specified by StudioId
* .
*
*
* @param listStudioSessionMappingsRequest
* @return A Java Future containing the result of the ListStudioSessionMappings operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InternalServerErrorException Indicates that an error occurred while processing the request and that
* the request was not completed.
* - InvalidRequestException This exception occurs when there is something wrong with user input.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EmrException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EmrAsyncClient.ListStudioSessionMappings
* @see AWS API Documentation
*/
@Override
public CompletableFuture listStudioSessionMappings(
ListStudioSessionMappingsRequest listStudioSessionMappingsRequest) {
List metricPublishers = resolveMetricPublishers(clientConfiguration, listStudioSessionMappingsRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "EMR");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "ListStudioSessionMappings");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, ListStudioSessionMappingsResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("ListStudioSessionMappings")
.withMarshaller(new ListStudioSessionMappingsRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withMetricCollector(apiCallMetricCollector).withInput(listStudioSessionMappingsRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Returns a list of all user or group session mappings for the Amazon EMR Studio specified by StudioId
* .
*
*
*
* This is a variant of
* {@link #listStudioSessionMappings(software.amazon.awssdk.services.emr.model.ListStudioSessionMappingsRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.emr.paginators.ListStudioSessionMappingsPublisher publisher = client.listStudioSessionMappingsPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.emr.paginators.ListStudioSessionMappingsPublisher publisher = client.listStudioSessionMappingsPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.emr.model.ListStudioSessionMappingsResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of null won't limit the number of results you get with the paginator. It
* only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listStudioSessionMappings(software.amazon.awssdk.services.emr.model.ListStudioSessionMappingsRequest)}
* operation.
*
*
* @param listStudioSessionMappingsRequest
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InternalServerErrorException Indicates that an error occurred while processing the request and that
* the request was not completed.
* - InvalidRequestException This exception occurs when there is something wrong with user input.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EmrException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EmrAsyncClient.ListStudioSessionMappings
* @see AWS API Documentation
*/
public ListStudioSessionMappingsPublisher listStudioSessionMappingsPaginator(
ListStudioSessionMappingsRequest listStudioSessionMappingsRequest) {
return new ListStudioSessionMappingsPublisher(this, applyPaginatorUserAgent(listStudioSessionMappingsRequest));
}
/**
*
* Returns a list of all Amazon EMR Studios associated with the Amazon Web Services account. The list includes
* details such as ID, Studio Access URL, and creation time for each Studio.
*
*
* @param listStudiosRequest
* @return A Java Future containing the result of the ListStudios operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InternalServerException This exception occurs when there is an internal failure in the Amazon EMR
* service.
* - InvalidRequestException This exception occurs when there is something wrong with user input.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EmrException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EmrAsyncClient.ListStudios
* @see AWS
* API Documentation
*/
@Override
public CompletableFuture listStudios(ListStudiosRequest listStudiosRequest) {
List metricPublishers = resolveMetricPublishers(clientConfiguration, listStudiosRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "EMR");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "ListStudios");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
ListStudiosResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("ListStudios").withMarshaller(new ListStudiosRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withMetricCollector(apiCallMetricCollector).withInput(listStudiosRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Returns a list of all Amazon EMR Studios associated with the Amazon Web Services account. The list includes
* details such as ID, Studio Access URL, and creation time for each Studio.
*
*
*
* This is a variant of {@link #listStudios(software.amazon.awssdk.services.emr.model.ListStudiosRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.emr.paginators.ListStudiosPublisher publisher = client.listStudiosPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.emr.paginators.ListStudiosPublisher publisher = client.listStudiosPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.emr.model.ListStudiosResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of null won't limit the number of results you get with the paginator. It
* only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listStudios(software.amazon.awssdk.services.emr.model.ListStudiosRequest)} operation.
*
*
* @param listStudiosRequest
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InternalServerException This exception occurs when there is an internal failure in the Amazon EMR
* service.
* - InvalidRequestException This exception occurs when there is something wrong with user input.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EmrException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EmrAsyncClient.ListStudios
* @see AWS
* API Documentation
*/
public ListStudiosPublisher listStudiosPaginator(ListStudiosRequest listStudiosRequest) {
return new ListStudiosPublisher(this, applyPaginatorUserAgent(listStudiosRequest));
}
/**
*
* Modifies the number of steps that can be executed concurrently for the cluster specified using ClusterID.
*
*
* @param modifyClusterRequest
* @return A Java Future containing the result of the ModifyCluster operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InternalServerErrorException Indicates that an error occurred while processing the request and that
* the request was not completed.
* - InvalidRequestException This exception occurs when there is something wrong with user input.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EmrException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EmrAsyncClient.ModifyCluster
* @see AWS API Documentation
*/
@Override
public CompletableFuture modifyCluster(ModifyClusterRequest modifyClusterRequest) {
List metricPublishers = resolveMetricPublishers(clientConfiguration, modifyClusterRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "EMR");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "ModifyCluster");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
ModifyClusterResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("ModifyCluster")
.withMarshaller(new ModifyClusterRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withMetricCollector(apiCallMetricCollector).withInput(modifyClusterRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Modifies the target On-Demand and target Spot capacities for the instance fleet with the specified
* InstanceFleetID within the cluster specified using ClusterID. The call either succeeds or fails atomically.
*
*
*
* The instance fleet configuration is available only in Amazon EMR versions 4.8.0 and later, excluding 5.0.x
* versions.
*
*
*
* @param modifyInstanceFleetRequest
* @return A Java Future containing the result of the ModifyInstanceFleet operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InternalServerException This exception occurs when there is an internal failure in the Amazon EMR
* service.
* - InvalidRequestException This exception occurs when there is something wrong with user input.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EmrException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EmrAsyncClient.ModifyInstanceFleet
* @see AWS API Documentation
*/
@Override
public CompletableFuture modifyInstanceFleet(
ModifyInstanceFleetRequest modifyInstanceFleetRequest) {
List metricPublishers = resolveMetricPublishers(clientConfiguration, modifyInstanceFleetRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "EMR");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "ModifyInstanceFleet");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, ModifyInstanceFleetResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("ModifyInstanceFleet")
.withMarshaller(new ModifyInstanceFleetRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withMetricCollector(apiCallMetricCollector).withInput(modifyInstanceFleetRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* ModifyInstanceGroups modifies the number of nodes and configuration settings of an instance group. The input
* parameters include the new target instance count for the group and the instance group ID. The call will either
* succeed or fail atomically.
*
*
* @param modifyInstanceGroupsRequest
* Change the size of some instance groups.
* @return A Java Future containing the result of the ModifyInstanceGroups operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InternalServerErrorException Indicates that an error occurred while processing the request and that
* the request was not completed.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EmrException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EmrAsyncClient.ModifyInstanceGroups
* @see AWS API Documentation
*/
@Override
public CompletableFuture modifyInstanceGroups(
ModifyInstanceGroupsRequest modifyInstanceGroupsRequest) {
List metricPublishers = resolveMetricPublishers(clientConfiguration, modifyInstanceGroupsRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "EMR");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "ModifyInstanceGroups");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, ModifyInstanceGroupsResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("ModifyInstanceGroups")
.withMarshaller(new ModifyInstanceGroupsRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withMetricCollector(apiCallMetricCollector).withInput(modifyInstanceGroupsRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Creates or updates an automatic scaling policy for a core instance group or task instance group in an Amazon EMR
* cluster. The automatic scaling policy defines how an instance group dynamically adds and terminates EC2 instances
* in response to the value of a CloudWatch metric.
*
*
* @param putAutoScalingPolicyRequest
* @return A Java Future containing the result of the PutAutoScalingPolicy operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EmrException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EmrAsyncClient.PutAutoScalingPolicy
* @see AWS API Documentation
*/
@Override
public CompletableFuture putAutoScalingPolicy(
PutAutoScalingPolicyRequest putAutoScalingPolicyRequest) {
List metricPublishers = resolveMetricPublishers(clientConfiguration, putAutoScalingPolicyRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "EMR");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "PutAutoScalingPolicy");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, PutAutoScalingPolicyResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams