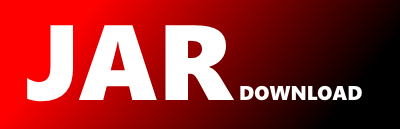
software.amazon.awssdk.services.emr.model.VolumeSpecification Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of emr Show documentation
Show all versions of emr Show documentation
The AWS Java SDK for Amazon EMR module holds the client classes that are used for communicating with
Amazon Elastic MapReduce Service
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.emr.model;
import java.io.Serializable;
import java.util.Arrays;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* EBS volume specifications such as volume type, IOPS, size (GiB) and throughput (MiB/s) that are requested for the EBS
* volume attached to an EC2 instance in the cluster.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class VolumeSpecification implements SdkPojo, Serializable,
ToCopyableBuilder {
private static final SdkField VOLUME_TYPE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("VolumeType").getter(getter(VolumeSpecification::volumeType)).setter(setter(Builder::volumeType))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("VolumeType").build()).build();
private static final SdkField IOPS_FIELD = SdkField. builder(MarshallingType.INTEGER).memberName("Iops")
.getter(getter(VolumeSpecification::iops)).setter(setter(Builder::iops))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Iops").build()).build();
private static final SdkField SIZE_IN_GB_FIELD = SdkField. builder(MarshallingType.INTEGER)
.memberName("SizeInGB").getter(getter(VolumeSpecification::sizeInGB)).setter(setter(Builder::sizeInGB))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("SizeInGB").build()).build();
private static final SdkField THROUGHPUT_FIELD = SdkField. builder(MarshallingType.INTEGER)
.memberName("Throughput").getter(getter(VolumeSpecification::throughput)).setter(setter(Builder::throughput))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Throughput").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(VOLUME_TYPE_FIELD, IOPS_FIELD,
SIZE_IN_GB_FIELD, THROUGHPUT_FIELD));
private static final long serialVersionUID = 1L;
private final String volumeType;
private final Integer iops;
private final Integer sizeInGB;
private final Integer throughput;
private VolumeSpecification(BuilderImpl builder) {
this.volumeType = builder.volumeType;
this.iops = builder.iops;
this.sizeInGB = builder.sizeInGB;
this.throughput = builder.throughput;
}
/**
*
* The volume type. Volume types supported are gp2, io1, and standard.
*
*
* @return The volume type. Volume types supported are gp2, io1, and standard.
*/
public final String volumeType() {
return volumeType;
}
/**
*
* The number of I/O operations per second (IOPS) that the volume supports.
*
*
* @return The number of I/O operations per second (IOPS) that the volume supports.
*/
public final Integer iops() {
return iops;
}
/**
*
* The volume size, in gibibytes (GiB). This can be a number from 1 - 1024. If the volume type is EBS-optimized, the
* minimum value is 10.
*
*
* @return The volume size, in gibibytes (GiB). This can be a number from 1 - 1024. If the volume type is
* EBS-optimized, the minimum value is 10.
*/
public final Integer sizeInGB() {
return sizeInGB;
}
/**
*
* The throughput, in mebibyte per second (MiB/s). This optional parameter can be a number from 125 - 1000 and is
* valid only for gp3 volumes.
*
*
* @return The throughput, in mebibyte per second (MiB/s). This optional parameter can be a number from 125 - 1000
* and is valid only for gp3 volumes.
*/
public final Integer throughput() {
return throughput;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(volumeType());
hashCode = 31 * hashCode + Objects.hashCode(iops());
hashCode = 31 * hashCode + Objects.hashCode(sizeInGB());
hashCode = 31 * hashCode + Objects.hashCode(throughput());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof VolumeSpecification)) {
return false;
}
VolumeSpecification other = (VolumeSpecification) obj;
return Objects.equals(volumeType(), other.volumeType()) && Objects.equals(iops(), other.iops())
&& Objects.equals(sizeInGB(), other.sizeInGB()) && Objects.equals(throughput(), other.throughput());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("VolumeSpecification").add("VolumeType", volumeType()).add("Iops", iops())
.add("SizeInGB", sizeInGB()).add("Throughput", throughput()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "VolumeType":
return Optional.ofNullable(clazz.cast(volumeType()));
case "Iops":
return Optional.ofNullable(clazz.cast(iops()));
case "SizeInGB":
return Optional.ofNullable(clazz.cast(sizeInGB()));
case "Throughput":
return Optional.ofNullable(clazz.cast(throughput()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
private static Function
© 2015 - 2025 Weber Informatics LLC | Privacy Policy