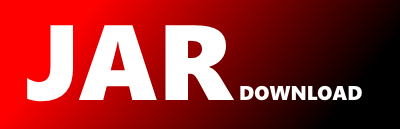
software.amazon.awssdk.services.emr.model.SimpleScalingPolicyConfiguration Maven / Gradle / Ivy
Show all versions of emr Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.emr.model;
import java.io.Serializable;
import java.util.Arrays;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* An automatic scaling configuration, which describes how the policy adds or removes instances, the cooldown period,
* and the number of EC2 instances that will be added each time the CloudWatch metric alarm condition is satisfied.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class SimpleScalingPolicyConfiguration implements SdkPojo, Serializable,
ToCopyableBuilder {
private static final SdkField ADJUSTMENT_TYPE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("AdjustmentType").getter(getter(SimpleScalingPolicyConfiguration::adjustmentTypeAsString))
.setter(setter(Builder::adjustmentType))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("AdjustmentType").build()).build();
private static final SdkField SCALING_ADJUSTMENT_FIELD = SdkField. builder(MarshallingType.INTEGER)
.memberName("ScalingAdjustment").getter(getter(SimpleScalingPolicyConfiguration::scalingAdjustment))
.setter(setter(Builder::scalingAdjustment))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ScalingAdjustment").build()).build();
private static final SdkField COOL_DOWN_FIELD = SdkField. builder(MarshallingType.INTEGER)
.memberName("CoolDown").getter(getter(SimpleScalingPolicyConfiguration::coolDown)).setter(setter(Builder::coolDown))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("CoolDown").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(ADJUSTMENT_TYPE_FIELD,
SCALING_ADJUSTMENT_FIELD, COOL_DOWN_FIELD));
private static final long serialVersionUID = 1L;
private final String adjustmentType;
private final Integer scalingAdjustment;
private final Integer coolDown;
private SimpleScalingPolicyConfiguration(BuilderImpl builder) {
this.adjustmentType = builder.adjustmentType;
this.scalingAdjustment = builder.scalingAdjustment;
this.coolDown = builder.coolDown;
}
/**
*
* The way in which EC2 instances are added (if ScalingAdjustment
is a positive number) or terminated
* (if ScalingAdjustment
is a negative number) each time the scaling activity is triggered.
* CHANGE_IN_CAPACITY
is the default. CHANGE_IN_CAPACITY
indicates that the EC2 instance
* count increments or decrements by ScalingAdjustment
, which should be expressed as an integer.
* PERCENT_CHANGE_IN_CAPACITY
indicates the instance count increments or decrements by the percentage
* specified by ScalingAdjustment
, which should be expressed as an integer. For example, 20 indicates
* an increase in 20% increments of cluster capacity. EXACT_CAPACITY
indicates the scaling activity
* results in an instance group with the number of EC2 instances specified by ScalingAdjustment
, which
* should be expressed as a positive integer.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #adjustmentType}
* will return {@link AdjustmentType#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available
* from {@link #adjustmentTypeAsString}.
*
*
* @return The way in which EC2 instances are added (if ScalingAdjustment
is a positive number) or
* terminated (if ScalingAdjustment
is a negative number) each time the scaling activity is
* triggered. CHANGE_IN_CAPACITY
is the default. CHANGE_IN_CAPACITY
indicates that
* the EC2 instance count increments or decrements by ScalingAdjustment
, which should be
* expressed as an integer. PERCENT_CHANGE_IN_CAPACITY
indicates the instance count increments
* or decrements by the percentage specified by ScalingAdjustment
, which should be expressed as
* an integer. For example, 20 indicates an increase in 20% increments of cluster capacity.
* EXACT_CAPACITY
indicates the scaling activity results in an instance group with the number
* of EC2 instances specified by ScalingAdjustment
, which should be expressed as a positive
* integer.
* @see AdjustmentType
*/
public final AdjustmentType adjustmentType() {
return AdjustmentType.fromValue(adjustmentType);
}
/**
*
* The way in which EC2 instances are added (if ScalingAdjustment
is a positive number) or terminated
* (if ScalingAdjustment
is a negative number) each time the scaling activity is triggered.
* CHANGE_IN_CAPACITY
is the default. CHANGE_IN_CAPACITY
indicates that the EC2 instance
* count increments or decrements by ScalingAdjustment
, which should be expressed as an integer.
* PERCENT_CHANGE_IN_CAPACITY
indicates the instance count increments or decrements by the percentage
* specified by ScalingAdjustment
, which should be expressed as an integer. For example, 20 indicates
* an increase in 20% increments of cluster capacity. EXACT_CAPACITY
indicates the scaling activity
* results in an instance group with the number of EC2 instances specified by ScalingAdjustment
, which
* should be expressed as a positive integer.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #adjustmentType}
* will return {@link AdjustmentType#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available
* from {@link #adjustmentTypeAsString}.
*
*
* @return The way in which EC2 instances are added (if ScalingAdjustment
is a positive number) or
* terminated (if ScalingAdjustment
is a negative number) each time the scaling activity is
* triggered. CHANGE_IN_CAPACITY
is the default. CHANGE_IN_CAPACITY
indicates that
* the EC2 instance count increments or decrements by ScalingAdjustment
, which should be
* expressed as an integer. PERCENT_CHANGE_IN_CAPACITY
indicates the instance count increments
* or decrements by the percentage specified by ScalingAdjustment
, which should be expressed as
* an integer. For example, 20 indicates an increase in 20% increments of cluster capacity.
* EXACT_CAPACITY
indicates the scaling activity results in an instance group with the number
* of EC2 instances specified by ScalingAdjustment
, which should be expressed as a positive
* integer.
* @see AdjustmentType
*/
public final String adjustmentTypeAsString() {
return adjustmentType;
}
/**
*
* The amount by which to scale in or scale out, based on the specified AdjustmentType
. A positive
* value adds to the instance group's EC2 instance count while a negative number removes instances. If
* AdjustmentType
is set to EXACT_CAPACITY
, the number should only be a positive integer.
* If AdjustmentType
is set to PERCENT_CHANGE_IN_CAPACITY
, the value should express the
* percentage as an integer. For example, -20 indicates a decrease in 20% increments of cluster capacity.
*
*
* @return The amount by which to scale in or scale out, based on the specified AdjustmentType
. A
* positive value adds to the instance group's EC2 instance count while a negative number removes instances.
* If AdjustmentType
is set to EXACT_CAPACITY
, the number should only be a
* positive integer. If AdjustmentType
is set to PERCENT_CHANGE_IN_CAPACITY
, the
* value should express the percentage as an integer. For example, -20 indicates a decrease in 20%
* increments of cluster capacity.
*/
public final Integer scalingAdjustment() {
return scalingAdjustment;
}
/**
*
* The amount of time, in seconds, after a scaling activity completes before any further trigger-related scaling
* activities can start. The default value is 0.
*
*
* @return The amount of time, in seconds, after a scaling activity completes before any further trigger-related
* scaling activities can start. The default value is 0.
*/
public final Integer coolDown() {
return coolDown;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(adjustmentTypeAsString());
hashCode = 31 * hashCode + Objects.hashCode(scalingAdjustment());
hashCode = 31 * hashCode + Objects.hashCode(coolDown());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof SimpleScalingPolicyConfiguration)) {
return false;
}
SimpleScalingPolicyConfiguration other = (SimpleScalingPolicyConfiguration) obj;
return Objects.equals(adjustmentTypeAsString(), other.adjustmentTypeAsString())
&& Objects.equals(scalingAdjustment(), other.scalingAdjustment()) && Objects.equals(coolDown(), other.coolDown());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("SimpleScalingPolicyConfiguration").add("AdjustmentType", adjustmentTypeAsString())
.add("ScalingAdjustment", scalingAdjustment()).add("CoolDown", coolDown()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "AdjustmentType":
return Optional.ofNullable(clazz.cast(adjustmentTypeAsString()));
case "ScalingAdjustment":
return Optional.ofNullable(clazz.cast(scalingAdjustment()));
case "CoolDown":
return Optional.ofNullable(clazz.cast(coolDown()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
private static Function