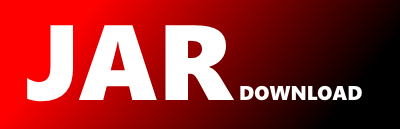
software.amazon.awssdk.services.emr.model.RunJobFlowRequest Maven / Gradle / Ivy
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.emr.model;
import java.beans.Transient;
import java.util.Arrays;
import java.util.Collection;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import java.util.stream.Collectors;
import java.util.stream.Stream;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.awscore.AwsRequestOverrideConfiguration;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.ListTrait;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.core.util.DefaultSdkAutoConstructList;
import software.amazon.awssdk.core.util.SdkAutoConstructList;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* Input to the RunJobFlow operation.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class RunJobFlowRequest extends EmrRequest implements
ToCopyableBuilder {
private static final SdkField NAME_FIELD = SdkField. builder(MarshallingType.STRING).memberName("Name")
.getter(getter(RunJobFlowRequest::name)).setter(setter(Builder::name))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Name").build()).build();
private static final SdkField LOG_URI_FIELD = SdkField. builder(MarshallingType.STRING).memberName("LogUri")
.getter(getter(RunJobFlowRequest::logUri)).setter(setter(Builder::logUri))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("LogUri").build()).build();
private static final SdkField LOG_ENCRYPTION_KMS_KEY_ID_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("LogEncryptionKmsKeyId").getter(getter(RunJobFlowRequest::logEncryptionKmsKeyId))
.setter(setter(Builder::logEncryptionKmsKeyId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("LogEncryptionKmsKeyId").build())
.build();
private static final SdkField ADDITIONAL_INFO_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("AdditionalInfo").getter(getter(RunJobFlowRequest::additionalInfo))
.setter(setter(Builder::additionalInfo))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("AdditionalInfo").build()).build();
private static final SdkField AMI_VERSION_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("AmiVersion").getter(getter(RunJobFlowRequest::amiVersion)).setter(setter(Builder::amiVersion))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("AmiVersion").build()).build();
private static final SdkField RELEASE_LABEL_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("ReleaseLabel").getter(getter(RunJobFlowRequest::releaseLabel)).setter(setter(Builder::releaseLabel))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ReleaseLabel").build()).build();
private static final SdkField INSTANCES_FIELD = SdkField
. builder(MarshallingType.SDK_POJO).memberName("Instances")
.getter(getter(RunJobFlowRequest::instances)).setter(setter(Builder::instances))
.constructor(JobFlowInstancesConfig::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Instances").build()).build();
private static final SdkField> STEPS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("Steps")
.getter(getter(RunJobFlowRequest::steps))
.setter(setter(Builder::steps))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Steps").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(StepConfig::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField> BOOTSTRAP_ACTIONS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("BootstrapActions")
.getter(getter(RunJobFlowRequest::bootstrapActions))
.setter(setter(Builder::bootstrapActions))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("BootstrapActions").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(BootstrapActionConfig::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField> SUPPORTED_PRODUCTS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("SupportedProducts")
.getter(getter(RunJobFlowRequest::supportedProducts))
.setter(setter(Builder::supportedProducts))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("SupportedProducts").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.STRING)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField> NEW_SUPPORTED_PRODUCTS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("NewSupportedProducts")
.getter(getter(RunJobFlowRequest::newSupportedProducts))
.setter(setter(Builder::newSupportedProducts))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("NewSupportedProducts").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(SupportedProductConfig::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField> APPLICATIONS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("Applications")
.getter(getter(RunJobFlowRequest::applications))
.setter(setter(Builder::applications))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Applications").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(Application::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField> CONFIGURATIONS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("Configurations")
.getter(getter(RunJobFlowRequest::configurations))
.setter(setter(Builder::configurations))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Configurations").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(Configuration::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField VISIBLE_TO_ALL_USERS_FIELD = SdkField. builder(MarshallingType.BOOLEAN)
.memberName("VisibleToAllUsers").getter(getter(RunJobFlowRequest::visibleToAllUsers))
.setter(setter(Builder::visibleToAllUsers))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("VisibleToAllUsers").build()).build();
private static final SdkField JOB_FLOW_ROLE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("JobFlowRole").getter(getter(RunJobFlowRequest::jobFlowRole)).setter(setter(Builder::jobFlowRole))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("JobFlowRole").build()).build();
private static final SdkField SERVICE_ROLE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("ServiceRole").getter(getter(RunJobFlowRequest::serviceRole)).setter(setter(Builder::serviceRole))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ServiceRole").build()).build();
private static final SdkField> TAGS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("Tags")
.getter(getter(RunJobFlowRequest::tags))
.setter(setter(Builder::tags))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Tags").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(Tag::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField SECURITY_CONFIGURATION_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("SecurityConfiguration").getter(getter(RunJobFlowRequest::securityConfiguration))
.setter(setter(Builder::securityConfiguration))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("SecurityConfiguration").build())
.build();
private static final SdkField AUTO_SCALING_ROLE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("AutoScalingRole").getter(getter(RunJobFlowRequest::autoScalingRole))
.setter(setter(Builder::autoScalingRole))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("AutoScalingRole").build()).build();
private static final SdkField SCALE_DOWN_BEHAVIOR_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("ScaleDownBehavior").getter(getter(RunJobFlowRequest::scaleDownBehaviorAsString))
.setter(setter(Builder::scaleDownBehavior))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ScaleDownBehavior").build()).build();
private static final SdkField CUSTOM_AMI_ID_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("CustomAmiId").getter(getter(RunJobFlowRequest::customAmiId)).setter(setter(Builder::customAmiId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("CustomAmiId").build()).build();
private static final SdkField EBS_ROOT_VOLUME_SIZE_FIELD = SdkField. builder(MarshallingType.INTEGER)
.memberName("EbsRootVolumeSize").getter(getter(RunJobFlowRequest::ebsRootVolumeSize))
.setter(setter(Builder::ebsRootVolumeSize))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("EbsRootVolumeSize").build()).build();
private static final SdkField REPO_UPGRADE_ON_BOOT_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("RepoUpgradeOnBoot").getter(getter(RunJobFlowRequest::repoUpgradeOnBootAsString))
.setter(setter(Builder::repoUpgradeOnBoot))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("RepoUpgradeOnBoot").build()).build();
private static final SdkField KERBEROS_ATTRIBUTES_FIELD = SdkField
. builder(MarshallingType.SDK_POJO).memberName("KerberosAttributes")
.getter(getter(RunJobFlowRequest::kerberosAttributes)).setter(setter(Builder::kerberosAttributes))
.constructor(KerberosAttributes::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("KerberosAttributes").build())
.build();
private static final SdkField STEP_CONCURRENCY_LEVEL_FIELD = SdkField. builder(MarshallingType.INTEGER)
.memberName("StepConcurrencyLevel").getter(getter(RunJobFlowRequest::stepConcurrencyLevel))
.setter(setter(Builder::stepConcurrencyLevel))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("StepConcurrencyLevel").build())
.build();
private static final SdkField MANAGED_SCALING_POLICY_FIELD = SdkField
. builder(MarshallingType.SDK_POJO).memberName("ManagedScalingPolicy")
.getter(getter(RunJobFlowRequest::managedScalingPolicy)).setter(setter(Builder::managedScalingPolicy))
.constructor(ManagedScalingPolicy::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ManagedScalingPolicy").build())
.build();
private static final SdkField> PLACEMENT_GROUP_CONFIGS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("PlacementGroupConfigs")
.getter(getter(RunJobFlowRequest::placementGroupConfigs))
.setter(setter(Builder::placementGroupConfigs))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("PlacementGroupConfigs").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(PlacementGroupConfig::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField AUTO_TERMINATION_POLICY_FIELD = SdkField
. builder(MarshallingType.SDK_POJO).memberName("AutoTerminationPolicy")
.getter(getter(RunJobFlowRequest::autoTerminationPolicy)).setter(setter(Builder::autoTerminationPolicy))
.constructor(AutoTerminationPolicy::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("AutoTerminationPolicy").build())
.build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(NAME_FIELD, LOG_URI_FIELD,
LOG_ENCRYPTION_KMS_KEY_ID_FIELD, ADDITIONAL_INFO_FIELD, AMI_VERSION_FIELD, RELEASE_LABEL_FIELD, INSTANCES_FIELD,
STEPS_FIELD, BOOTSTRAP_ACTIONS_FIELD, SUPPORTED_PRODUCTS_FIELD, NEW_SUPPORTED_PRODUCTS_FIELD, APPLICATIONS_FIELD,
CONFIGURATIONS_FIELD, VISIBLE_TO_ALL_USERS_FIELD, JOB_FLOW_ROLE_FIELD, SERVICE_ROLE_FIELD, TAGS_FIELD,
SECURITY_CONFIGURATION_FIELD, AUTO_SCALING_ROLE_FIELD, SCALE_DOWN_BEHAVIOR_FIELD, CUSTOM_AMI_ID_FIELD,
EBS_ROOT_VOLUME_SIZE_FIELD, REPO_UPGRADE_ON_BOOT_FIELD, KERBEROS_ATTRIBUTES_FIELD, STEP_CONCURRENCY_LEVEL_FIELD,
MANAGED_SCALING_POLICY_FIELD, PLACEMENT_GROUP_CONFIGS_FIELD, AUTO_TERMINATION_POLICY_FIELD));
private final String name;
private final String logUri;
private final String logEncryptionKmsKeyId;
private final String additionalInfo;
private final String amiVersion;
private final String releaseLabel;
private final JobFlowInstancesConfig instances;
private final List steps;
private final List bootstrapActions;
private final List supportedProducts;
private final List newSupportedProducts;
private final List applications;
private final List configurations;
private final Boolean visibleToAllUsers;
private final String jobFlowRole;
private final String serviceRole;
private final List tags;
private final String securityConfiguration;
private final String autoScalingRole;
private final String scaleDownBehavior;
private final String customAmiId;
private final Integer ebsRootVolumeSize;
private final String repoUpgradeOnBoot;
private final KerberosAttributes kerberosAttributes;
private final Integer stepConcurrencyLevel;
private final ManagedScalingPolicy managedScalingPolicy;
private final List placementGroupConfigs;
private final AutoTerminationPolicy autoTerminationPolicy;
private RunJobFlowRequest(BuilderImpl builder) {
super(builder);
this.name = builder.name;
this.logUri = builder.logUri;
this.logEncryptionKmsKeyId = builder.logEncryptionKmsKeyId;
this.additionalInfo = builder.additionalInfo;
this.amiVersion = builder.amiVersion;
this.releaseLabel = builder.releaseLabel;
this.instances = builder.instances;
this.steps = builder.steps;
this.bootstrapActions = builder.bootstrapActions;
this.supportedProducts = builder.supportedProducts;
this.newSupportedProducts = builder.newSupportedProducts;
this.applications = builder.applications;
this.configurations = builder.configurations;
this.visibleToAllUsers = builder.visibleToAllUsers;
this.jobFlowRole = builder.jobFlowRole;
this.serviceRole = builder.serviceRole;
this.tags = builder.tags;
this.securityConfiguration = builder.securityConfiguration;
this.autoScalingRole = builder.autoScalingRole;
this.scaleDownBehavior = builder.scaleDownBehavior;
this.customAmiId = builder.customAmiId;
this.ebsRootVolumeSize = builder.ebsRootVolumeSize;
this.repoUpgradeOnBoot = builder.repoUpgradeOnBoot;
this.kerberosAttributes = builder.kerberosAttributes;
this.stepConcurrencyLevel = builder.stepConcurrencyLevel;
this.managedScalingPolicy = builder.managedScalingPolicy;
this.placementGroupConfigs = builder.placementGroupConfigs;
this.autoTerminationPolicy = builder.autoTerminationPolicy;
}
/**
*
* The name of the job flow.
*
*
* @return The name of the job flow.
*/
public final String name() {
return name;
}
/**
*
* The location in Amazon S3 to write the log files of the job flow. If a value is not provided, logs are not
* created.
*
*
* @return The location in Amazon S3 to write the log files of the job flow. If a value is not provided, logs are
* not created.
*/
public final String logUri() {
return logUri;
}
/**
*
* The KMS key used for encrypting log files. If a value is not provided, the logs remain encrypted by AES-256. This
* attribute is only available with Amazon EMR version 5.30.0 and later, excluding Amazon EMR 6.0.0.
*
*
* @return The KMS key used for encrypting log files. If a value is not provided, the logs remain encrypted by
* AES-256. This attribute is only available with Amazon EMR version 5.30.0 and later, excluding Amazon EMR
* 6.0.0.
*/
public final String logEncryptionKmsKeyId() {
return logEncryptionKmsKeyId;
}
/**
*
* A JSON string for selecting additional features.
*
*
* @return A JSON string for selecting additional features.
*/
public final String additionalInfo() {
return additionalInfo;
}
/**
*
* Applies only to Amazon EMR AMI versions 3.x and 2.x. For Amazon EMR releases 4.0 and later,
* ReleaseLabel
is used. To specify a custom AMI, use CustomAmiID
.
*
*
* @return Applies only to Amazon EMR AMI versions 3.x and 2.x. For Amazon EMR releases 4.0 and later,
* ReleaseLabel
is used. To specify a custom AMI, use CustomAmiID
.
*/
public final String amiVersion() {
return amiVersion;
}
/**
*
* The Amazon EMR release label, which determines the version of open-source application packages installed on the
* cluster. Release labels are in the form emr-x.x.x
, where x.x.x is an Amazon EMR release version such
* as emr-5.14.0
. For more information about Amazon EMR release versions and included application
* versions and features, see https://docs.aws.amazon.
* com/emr/latest/ReleaseGuide/. The release label applies only to Amazon EMR releases version 4.0 and later.
* Earlier versions use AmiVersion
.
*
*
* @return The Amazon EMR release label, which determines the version of open-source application packages installed
* on the cluster. Release labels are in the form emr-x.x.x
, where x.x.x is an Amazon EMR
* release version such as emr-5.14.0
. For more information about Amazon EMR release versions
* and included application versions and features, see https://docs.aws.amazon.com/emr/latest/ReleaseGuide/. The release label applies only to Amazon EMR
* releases version 4.0 and later. Earlier versions use AmiVersion
.
*/
public final String releaseLabel() {
return releaseLabel;
}
/**
*
* A specification of the number and type of Amazon EC2 instances.
*
*
* @return A specification of the number and type of Amazon EC2 instances.
*/
public final JobFlowInstancesConfig instances() {
return instances;
}
/**
* For responses, this returns true if the service returned a value for the Steps property. This DOES NOT check that
* the value is non-empty (for which, you should check the {@code isEmpty()} method on the property). This is useful
* because the SDK will never return a null collection or map, but you may need to differentiate between the service
* returning nothing (or null) and the service returning an empty collection or map. For requests, this returns true
* if a value for the property was specified in the request builder, and false if a value was not specified.
*/
public final boolean hasSteps() {
return steps != null && !(steps instanceof SdkAutoConstructList);
}
/**
*
* A list of steps to run.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasSteps} method.
*
*
* @return A list of steps to run.
*/
public final List steps() {
return steps;
}
/**
* For responses, this returns true if the service returned a value for the BootstrapActions property. This DOES NOT
* check that the value is non-empty (for which, you should check the {@code isEmpty()} method on the property).
* This is useful because the SDK will never return a null collection or map, but you may need to differentiate
* between the service returning nothing (or null) and the service returning an empty collection or map. For
* requests, this returns true if a value for the property was specified in the request builder, and false if a
* value was not specified.
*/
public final boolean hasBootstrapActions() {
return bootstrapActions != null && !(bootstrapActions instanceof SdkAutoConstructList);
}
/**
*
* A list of bootstrap actions to run before Hadoop starts on the cluster nodes.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasBootstrapActions} method.
*
*
* @return A list of bootstrap actions to run before Hadoop starts on the cluster nodes.
*/
public final List bootstrapActions() {
return bootstrapActions;
}
/**
* For responses, this returns true if the service returned a value for the SupportedProducts property. This DOES
* NOT check that the value is non-empty (for which, you should check the {@code isEmpty()} method on the property).
* This is useful because the SDK will never return a null collection or map, but you may need to differentiate
* between the service returning nothing (or null) and the service returning an empty collection or map. For
* requests, this returns true if a value for the property was specified in the request builder, and false if a
* value was not specified.
*/
public final boolean hasSupportedProducts() {
return supportedProducts != null && !(supportedProducts instanceof SdkAutoConstructList);
}
/**
*
*
* For Amazon EMR releases 3.x and 2.x. For Amazon EMR releases 4.x and later, use Applications.
*
*
*
* A list of strings that indicates third-party software to use. For more information, see the Amazon EMR Developer Guide. Currently
* supported values are:
*
*
* -
*
* "mapr-m3" - launch the job flow using MapR M3 Edition.
*
*
* -
*
* "mapr-m5" - launch the job flow using MapR M5 Edition.
*
*
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasSupportedProducts} method.
*
*
* @return
* For Amazon EMR releases 3.x and 2.x. For Amazon EMR releases 4.x and later, use Applications.
*
*
*
* A list of strings that indicates third-party software to use. For more information, see the Amazon EMR Developer Guide.
* Currently supported values are:
*
*
* -
*
* "mapr-m3" - launch the job flow using MapR M3 Edition.
*
*
* -
*
* "mapr-m5" - launch the job flow using MapR M5 Edition.
*
*
*/
public final List supportedProducts() {
return supportedProducts;
}
/**
* For responses, this returns true if the service returned a value for the NewSupportedProducts property. This DOES
* NOT check that the value is non-empty (for which, you should check the {@code isEmpty()} method on the property).
* This is useful because the SDK will never return a null collection or map, but you may need to differentiate
* between the service returning nothing (or null) and the service returning an empty collection or map. For
* requests, this returns true if a value for the property was specified in the request builder, and false if a
* value was not specified.
*/
public final boolean hasNewSupportedProducts() {
return newSupportedProducts != null && !(newSupportedProducts instanceof SdkAutoConstructList);
}
/**
*
*
* For Amazon EMR releases 3.x and 2.x. For Amazon EMR releases 4.x and later, use Applications.
*
*
*
* A list of strings that indicates third-party software to use with the job flow that accepts a user argument list.
* EMR accepts and forwards the argument list to the corresponding installation script as bootstrap action
* arguments. For more information, see "Launch a Job Flow on the MapR Distribution for Hadoop" in the Amazon EMR Developer Guide. Supported
* values are:
*
*
* -
*
* "mapr-m3" - launch the cluster using MapR M3 Edition.
*
*
* -
*
* "mapr-m5" - launch the cluster using MapR M5 Edition.
*
*
* -
*
* "mapr" with the user arguments specifying "--edition,m3" or "--edition,m5" - launch the job flow using MapR M3 or
* M5 Edition respectively.
*
*
* -
*
* "mapr-m7" - launch the cluster using MapR M7 Edition.
*
*
* -
*
* "hunk" - launch the cluster with the Hunk Big Data Analytics Platform.
*
*
* -
*
* "hue"- launch the cluster with Hue installed.
*
*
* -
*
* "spark" - launch the cluster with Apache Spark installed.
*
*
* -
*
* "ganglia" - launch the cluster with the Ganglia Monitoring System installed.
*
*
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasNewSupportedProducts} method.
*
*
* @return
* For Amazon EMR releases 3.x and 2.x. For Amazon EMR releases 4.x and later, use Applications.
*
*
*
* A list of strings that indicates third-party software to use with the job flow that accepts a user
* argument list. EMR accepts and forwards the argument list to the corresponding installation script as
* bootstrap action arguments. For more information, see
* "Launch a Job Flow on the MapR Distribution for Hadoop" in the Amazon EMR Developer Guide.
* Supported values are:
*
*
* -
*
* "mapr-m3" - launch the cluster using MapR M3 Edition.
*
*
* -
*
* "mapr-m5" - launch the cluster using MapR M5 Edition.
*
*
* -
*
* "mapr" with the user arguments specifying "--edition,m3" or "--edition,m5" - launch the job flow using
* MapR M3 or M5 Edition respectively.
*
*
* -
*
* "mapr-m7" - launch the cluster using MapR M7 Edition.
*
*
* -
*
* "hunk" - launch the cluster with the Hunk Big Data Analytics Platform.
*
*
* -
*
* "hue"- launch the cluster with Hue installed.
*
*
* -
*
* "spark" - launch the cluster with Apache Spark installed.
*
*
* -
*
* "ganglia" - launch the cluster with the Ganglia Monitoring System installed.
*
*
*/
public final List newSupportedProducts() {
return newSupportedProducts;
}
/**
* For responses, this returns true if the service returned a value for the Applications property. This DOES NOT
* check that the value is non-empty (for which, you should check the {@code isEmpty()} method on the property).
* This is useful because the SDK will never return a null collection or map, but you may need to differentiate
* between the service returning nothing (or null) and the service returning an empty collection or map. For
* requests, this returns true if a value for the property was specified in the request builder, and false if a
* value was not specified.
*/
public final boolean hasApplications() {
return applications != null && !(applications instanceof SdkAutoConstructList);
}
/**
*
* Applies to Amazon EMR releases 4.0 and later. A case-insensitive list of applications for Amazon EMR to install
* and configure when launching the cluster. For a list of applications available for each Amazon EMR release
* version, see the Amazon EMR Release Guide.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasApplications} method.
*
*
* @return Applies to Amazon EMR releases 4.0 and later. A case-insensitive list of applications for Amazon EMR to
* install and configure when launching the cluster. For a list of applications available for each Amazon
* EMR release version, see the Amazon EMR
* Release Guide.
*/
public final List applications() {
return applications;
}
/**
* For responses, this returns true if the service returned a value for the Configurations property. This DOES NOT
* check that the value is non-empty (for which, you should check the {@code isEmpty()} method on the property).
* This is useful because the SDK will never return a null collection or map, but you may need to differentiate
* between the service returning nothing (or null) and the service returning an empty collection or map. For
* requests, this returns true if a value for the property was specified in the request builder, and false if a
* value was not specified.
*/
public final boolean hasConfigurations() {
return configurations != null && !(configurations instanceof SdkAutoConstructList);
}
/**
*
* For Amazon EMR releases 4.0 and later. The list of configurations supplied for the EMR cluster you are creating.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasConfigurations} method.
*
*
* @return For Amazon EMR releases 4.0 and later. The list of configurations supplied for the EMR cluster you are
* creating.
*/
public final List configurations() {
return configurations;
}
/**
*
* Set this value to true
so that IAM principals in the Amazon Web Services account associated with the
* cluster can perform EMR actions on the cluster that their IAM policies allow. This value defaults to
* true
for clusters created using the EMR API or the CLI create-cluster command.
*
*
* When set to false
, only the IAM principal that created the cluster and the Amazon Web Services
* account root user can perform EMR actions for the cluster, regardless of the IAM permissions policies attached to
* other IAM principals. For more information, see Understanding the EMR Cluster VisibleToAllUsers Setting in the Amazon EMRManagement Guide.
*
*
* @return Set this value to true
so that IAM principals in the Amazon Web Services account associated
* with the cluster can perform EMR actions on the cluster that their IAM policies allow. This value
* defaults to true
for clusters created using the EMR API or the CLI create-cluster
* command.
*
* When set to false
, only the IAM principal that created the cluster and the Amazon Web
* Services account root user can perform EMR actions for the cluster, regardless of the IAM permissions
* policies attached to other IAM principals. For more information, see Understanding the EMR Cluster VisibleToAllUsers Setting in the Amazon EMRManagement Guide.
*/
public final Boolean visibleToAllUsers() {
return visibleToAllUsers;
}
/**
*
* Also called instance profile and EC2 role. An IAM role for an EMR cluster. The EC2 instances of the cluster
* assume this role. The default role is EMR_EC2_DefaultRole
. In order to use the default role, you
* must have already created it using the CLI or console.
*
*
* @return Also called instance profile and EC2 role. An IAM role for an EMR cluster. The EC2 instances of the
* cluster assume this role. The default role is EMR_EC2_DefaultRole
. In order to use the
* default role, you must have already created it using the CLI or console.
*/
public final String jobFlowRole() {
return jobFlowRole;
}
/**
*
* The IAM role that Amazon EMR assumes in order to access Amazon Web Services resources on your behalf.
*
*
* @return The IAM role that Amazon EMR assumes in order to access Amazon Web Services resources on your behalf.
*/
public final String serviceRole() {
return serviceRole;
}
/**
* For responses, this returns true if the service returned a value for the Tags property. This DOES NOT check that
* the value is non-empty (for which, you should check the {@code isEmpty()} method on the property). This is useful
* because the SDK will never return a null collection or map, but you may need to differentiate between the service
* returning nothing (or null) and the service returning an empty collection or map. For requests, this returns true
* if a value for the property was specified in the request builder, and false if a value was not specified.
*/
public final boolean hasTags() {
return tags != null && !(tags instanceof SdkAutoConstructList);
}
/**
*
* A list of tags to associate with a cluster and propagate to Amazon EC2 instances.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasTags} method.
*
*
* @return A list of tags to associate with a cluster and propagate to Amazon EC2 instances.
*/
public final List tags() {
return tags;
}
/**
*
* The name of a security configuration to apply to the cluster.
*
*
* @return The name of a security configuration to apply to the cluster.
*/
public final String securityConfiguration() {
return securityConfiguration;
}
/**
*
* An IAM role for automatic scaling policies. The default role is EMR_AutoScaling_DefaultRole
. The IAM
* role provides permissions that the automatic scaling feature requires to launch and terminate EC2 instances in an
* instance group.
*
*
* @return An IAM role for automatic scaling policies. The default role is EMR_AutoScaling_DefaultRole
.
* The IAM role provides permissions that the automatic scaling feature requires to launch and terminate EC2
* instances in an instance group.
*/
public final String autoScalingRole() {
return autoScalingRole;
}
/**
*
* Specifies the way that individual Amazon EC2 instances terminate when an automatic scale-in activity occurs or an
* instance group is resized. TERMINATE_AT_INSTANCE_HOUR
indicates that Amazon EMR terminates nodes at
* the instance-hour boundary, regardless of when the request to terminate the instance was submitted. This option
* is only available with Amazon EMR 5.1.0 and later and is the default for clusters created using that version.
* TERMINATE_AT_TASK_COMPLETION
indicates that Amazon EMR adds nodes to a deny list and drains tasks
* from nodes before terminating the Amazon EC2 instances, regardless of the instance-hour boundary. With either
* behavior, Amazon EMR removes the least active nodes first and blocks instance termination if it could lead to
* HDFS corruption. TERMINATE_AT_TASK_COMPLETION
available only in Amazon EMR version 4.1.0 and later,
* and is the default for versions of Amazon EMR earlier than 5.1.0.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #scaleDownBehavior}
* will return {@link ScaleDownBehavior#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available
* from {@link #scaleDownBehaviorAsString}.
*
*
* @return Specifies the way that individual Amazon EC2 instances terminate when an automatic scale-in activity
* occurs or an instance group is resized. TERMINATE_AT_INSTANCE_HOUR
indicates that Amazon EMR
* terminates nodes at the instance-hour boundary, regardless of when the request to terminate the instance
* was submitted. This option is only available with Amazon EMR 5.1.0 and later and is the default for
* clusters created using that version. TERMINATE_AT_TASK_COMPLETION
indicates that Amazon EMR
* adds nodes to a deny list and drains tasks from nodes before terminating the Amazon EC2 instances,
* regardless of the instance-hour boundary. With either behavior, Amazon EMR removes the least active nodes
* first and blocks instance termination if it could lead to HDFS corruption.
* TERMINATE_AT_TASK_COMPLETION
available only in Amazon EMR version 4.1.0 and later, and is
* the default for versions of Amazon EMR earlier than 5.1.0.
* @see ScaleDownBehavior
*/
public final ScaleDownBehavior scaleDownBehavior() {
return ScaleDownBehavior.fromValue(scaleDownBehavior);
}
/**
*
* Specifies the way that individual Amazon EC2 instances terminate when an automatic scale-in activity occurs or an
* instance group is resized. TERMINATE_AT_INSTANCE_HOUR
indicates that Amazon EMR terminates nodes at
* the instance-hour boundary, regardless of when the request to terminate the instance was submitted. This option
* is only available with Amazon EMR 5.1.0 and later and is the default for clusters created using that version.
* TERMINATE_AT_TASK_COMPLETION
indicates that Amazon EMR adds nodes to a deny list and drains tasks
* from nodes before terminating the Amazon EC2 instances, regardless of the instance-hour boundary. With either
* behavior, Amazon EMR removes the least active nodes first and blocks instance termination if it could lead to
* HDFS corruption. TERMINATE_AT_TASK_COMPLETION
available only in Amazon EMR version 4.1.0 and later,
* and is the default for versions of Amazon EMR earlier than 5.1.0.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #scaleDownBehavior}
* will return {@link ScaleDownBehavior#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available
* from {@link #scaleDownBehaviorAsString}.
*
*
* @return Specifies the way that individual Amazon EC2 instances terminate when an automatic scale-in activity
* occurs or an instance group is resized. TERMINATE_AT_INSTANCE_HOUR
indicates that Amazon EMR
* terminates nodes at the instance-hour boundary, regardless of when the request to terminate the instance
* was submitted. This option is only available with Amazon EMR 5.1.0 and later and is the default for
* clusters created using that version. TERMINATE_AT_TASK_COMPLETION
indicates that Amazon EMR
* adds nodes to a deny list and drains tasks from nodes before terminating the Amazon EC2 instances,
* regardless of the instance-hour boundary. With either behavior, Amazon EMR removes the least active nodes
* first and blocks instance termination if it could lead to HDFS corruption.
* TERMINATE_AT_TASK_COMPLETION
available only in Amazon EMR version 4.1.0 and later, and is
* the default for versions of Amazon EMR earlier than 5.1.0.
* @see ScaleDownBehavior
*/
public final String scaleDownBehaviorAsString() {
return scaleDownBehavior;
}
/**
*
* Available only in Amazon EMR version 5.7.0 and later. The ID of a custom Amazon EBS-backed Linux AMI. If
* specified, Amazon EMR uses this AMI when it launches cluster EC2 instances. For more information about custom
* AMIs in Amazon EMR, see Using a Custom AMI in the
* Amazon EMR Management Guide. If omitted, the cluster uses the base Linux AMI for the
* ReleaseLabel
specified. For Amazon EMR versions 2.x and 3.x, use AmiVersion
instead.
*
*
* For information about creating a custom AMI, see Creating an Amazon EBS-Backed
* Linux AMI in the Amazon Elastic Compute Cloud User Guide for Linux Instances. For information about
* finding an AMI ID, see Finding
* a Linux AMI.
*
*
* @return Available only in Amazon EMR version 5.7.0 and later. The ID of a custom Amazon EBS-backed Linux AMI. If
* specified, Amazon EMR uses this AMI when it launches cluster EC2 instances. For more information about
* custom AMIs in Amazon EMR, see Using a Custom AMI
* in the Amazon EMR Management Guide. If omitted, the cluster uses the base Linux AMI for the
* ReleaseLabel
specified. For Amazon EMR versions 2.x and 3.x, use AmiVersion
* instead.
*
* For information about creating a custom AMI, see Creating an Amazon
* EBS-Backed Linux AMI in the Amazon Elastic Compute Cloud User Guide for Linux Instances. For
* information about finding an AMI ID, see Finding a Linux AMI.
*/
public final String customAmiId() {
return customAmiId;
}
/**
*
* The size, in GiB, of the Amazon EBS root device volume of the Linux AMI that is used for each EC2 instance.
* Available in Amazon EMR version 4.x and later.
*
*
* @return The size, in GiB, of the Amazon EBS root device volume of the Linux AMI that is used for each EC2
* instance. Available in Amazon EMR version 4.x and later.
*/
public final Integer ebsRootVolumeSize() {
return ebsRootVolumeSize;
}
/**
*
* Applies only when CustomAmiID
is used. Specifies which updates from the Amazon Linux AMI package
* repositories to apply automatically when the instance boots using the AMI. If omitted, the default is
* SECURITY
, which indicates that only security updates are applied. If NONE
is specified,
* no updates are applied, and all updates must be applied manually.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #repoUpgradeOnBoot}
* will return {@link RepoUpgradeOnBoot#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available
* from {@link #repoUpgradeOnBootAsString}.
*
*
* @return Applies only when CustomAmiID
is used. Specifies which updates from the Amazon Linux AMI
* package repositories to apply automatically when the instance boots using the AMI. If omitted, the
* default is SECURITY
, which indicates that only security updates are applied. If
* NONE
is specified, no updates are applied, and all updates must be applied manually.
* @see RepoUpgradeOnBoot
*/
public final RepoUpgradeOnBoot repoUpgradeOnBoot() {
return RepoUpgradeOnBoot.fromValue(repoUpgradeOnBoot);
}
/**
*
* Applies only when CustomAmiID
is used. Specifies which updates from the Amazon Linux AMI package
* repositories to apply automatically when the instance boots using the AMI. If omitted, the default is
* SECURITY
, which indicates that only security updates are applied. If NONE
is specified,
* no updates are applied, and all updates must be applied manually.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #repoUpgradeOnBoot}
* will return {@link RepoUpgradeOnBoot#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available
* from {@link #repoUpgradeOnBootAsString}.
*
*
* @return Applies only when CustomAmiID
is used. Specifies which updates from the Amazon Linux AMI
* package repositories to apply automatically when the instance boots using the AMI. If omitted, the
* default is SECURITY
, which indicates that only security updates are applied. If
* NONE
is specified, no updates are applied, and all updates must be applied manually.
* @see RepoUpgradeOnBoot
*/
public final String repoUpgradeOnBootAsString() {
return repoUpgradeOnBoot;
}
/**
*
* Attributes for Kerberos configuration when Kerberos authentication is enabled using a security configuration. For
* more information see Use
* Kerberos Authentication in the Amazon EMR Management Guide.
*
*
* @return Attributes for Kerberos configuration when Kerberos authentication is enabled using a security
* configuration. For more information see Use Kerberos
* Authentication in the Amazon EMR Management Guide.
*/
public final KerberosAttributes kerberosAttributes() {
return kerberosAttributes;
}
/**
*
* Specifies the number of steps that can be executed concurrently. The default value is 1
. The maximum
* value is 256
.
*
*
* @return Specifies the number of steps that can be executed concurrently. The default value is 1
. The
* maximum value is 256
.
*/
public final Integer stepConcurrencyLevel() {
return stepConcurrencyLevel;
}
/**
*
* The specified managed scaling policy for an Amazon EMR cluster.
*
*
* @return The specified managed scaling policy for an Amazon EMR cluster.
*/
public final ManagedScalingPolicy managedScalingPolicy() {
return managedScalingPolicy;
}
/**
* For responses, this returns true if the service returned a value for the PlacementGroupConfigs property. This
* DOES NOT check that the value is non-empty (for which, you should check the {@code isEmpty()} method on the
* property). This is useful because the SDK will never return a null collection or map, but you may need to
* differentiate between the service returning nothing (or null) and the service returning an empty collection or
* map. For requests, this returns true if a value for the property was specified in the request builder, and false
* if a value was not specified.
*/
public final boolean hasPlacementGroupConfigs() {
return placementGroupConfigs != null && !(placementGroupConfigs instanceof SdkAutoConstructList);
}
/**
*
* The specified placement group configuration for an Amazon EMR cluster.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasPlacementGroupConfigs} method.
*
*
* @return The specified placement group configuration for an Amazon EMR cluster.
*/
public final List placementGroupConfigs() {
return placementGroupConfigs;
}
/**
* Returns the value of the AutoTerminationPolicy property for this object.
*
* @return The value of the AutoTerminationPolicy property for this object.
*/
public final AutoTerminationPolicy autoTerminationPolicy() {
return autoTerminationPolicy;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + super.hashCode();
hashCode = 31 * hashCode + Objects.hashCode(name());
hashCode = 31 * hashCode + Objects.hashCode(logUri());
hashCode = 31 * hashCode + Objects.hashCode(logEncryptionKmsKeyId());
hashCode = 31 * hashCode + Objects.hashCode(additionalInfo());
hashCode = 31 * hashCode + Objects.hashCode(amiVersion());
hashCode = 31 * hashCode + Objects.hashCode(releaseLabel());
hashCode = 31 * hashCode + Objects.hashCode(instances());
hashCode = 31 * hashCode + Objects.hashCode(hasSteps() ? steps() : null);
hashCode = 31 * hashCode + Objects.hashCode(hasBootstrapActions() ? bootstrapActions() : null);
hashCode = 31 * hashCode + Objects.hashCode(hasSupportedProducts() ? supportedProducts() : null);
hashCode = 31 * hashCode + Objects.hashCode(hasNewSupportedProducts() ? newSupportedProducts() : null);
hashCode = 31 * hashCode + Objects.hashCode(hasApplications() ? applications() : null);
hashCode = 31 * hashCode + Objects.hashCode(hasConfigurations() ? configurations() : null);
hashCode = 31 * hashCode + Objects.hashCode(visibleToAllUsers());
hashCode = 31 * hashCode + Objects.hashCode(jobFlowRole());
hashCode = 31 * hashCode + Objects.hashCode(serviceRole());
hashCode = 31 * hashCode + Objects.hashCode(hasTags() ? tags() : null);
hashCode = 31 * hashCode + Objects.hashCode(securityConfiguration());
hashCode = 31 * hashCode + Objects.hashCode(autoScalingRole());
hashCode = 31 * hashCode + Objects.hashCode(scaleDownBehaviorAsString());
hashCode = 31 * hashCode + Objects.hashCode(customAmiId());
hashCode = 31 * hashCode + Objects.hashCode(ebsRootVolumeSize());
hashCode = 31 * hashCode + Objects.hashCode(repoUpgradeOnBootAsString());
hashCode = 31 * hashCode + Objects.hashCode(kerberosAttributes());
hashCode = 31 * hashCode + Objects.hashCode(stepConcurrencyLevel());
hashCode = 31 * hashCode + Objects.hashCode(managedScalingPolicy());
hashCode = 31 * hashCode + Objects.hashCode(hasPlacementGroupConfigs() ? placementGroupConfigs() : null);
hashCode = 31 * hashCode + Objects.hashCode(autoTerminationPolicy());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return super.equals(obj) && equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof RunJobFlowRequest)) {
return false;
}
RunJobFlowRequest other = (RunJobFlowRequest) obj;
return Objects.equals(name(), other.name()) && Objects.equals(logUri(), other.logUri())
&& Objects.equals(logEncryptionKmsKeyId(), other.logEncryptionKmsKeyId())
&& Objects.equals(additionalInfo(), other.additionalInfo()) && Objects.equals(amiVersion(), other.amiVersion())
&& Objects.equals(releaseLabel(), other.releaseLabel()) && Objects.equals(instances(), other.instances())
&& hasSteps() == other.hasSteps() && Objects.equals(steps(), other.steps())
&& hasBootstrapActions() == other.hasBootstrapActions()
&& Objects.equals(bootstrapActions(), other.bootstrapActions())
&& hasSupportedProducts() == other.hasSupportedProducts()
&& Objects.equals(supportedProducts(), other.supportedProducts())
&& hasNewSupportedProducts() == other.hasNewSupportedProducts()
&& Objects.equals(newSupportedProducts(), other.newSupportedProducts())
&& hasApplications() == other.hasApplications() && Objects.equals(applications(), other.applications())
&& hasConfigurations() == other.hasConfigurations() && Objects.equals(configurations(), other.configurations())
&& Objects.equals(visibleToAllUsers(), other.visibleToAllUsers())
&& Objects.equals(jobFlowRole(), other.jobFlowRole()) && Objects.equals(serviceRole(), other.serviceRole())
&& hasTags() == other.hasTags() && Objects.equals(tags(), other.tags())
&& Objects.equals(securityConfiguration(), other.securityConfiguration())
&& Objects.equals(autoScalingRole(), other.autoScalingRole())
&& Objects.equals(scaleDownBehaviorAsString(), other.scaleDownBehaviorAsString())
&& Objects.equals(customAmiId(), other.customAmiId())
&& Objects.equals(ebsRootVolumeSize(), other.ebsRootVolumeSize())
&& Objects.equals(repoUpgradeOnBootAsString(), other.repoUpgradeOnBootAsString())
&& Objects.equals(kerberosAttributes(), other.kerberosAttributes())
&& Objects.equals(stepConcurrencyLevel(), other.stepConcurrencyLevel())
&& Objects.equals(managedScalingPolicy(), other.managedScalingPolicy())
&& hasPlacementGroupConfigs() == other.hasPlacementGroupConfigs()
&& Objects.equals(placementGroupConfigs(), other.placementGroupConfigs())
&& Objects.equals(autoTerminationPolicy(), other.autoTerminationPolicy());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("RunJobFlowRequest").add("Name", name()).add("LogUri", logUri())
.add("LogEncryptionKmsKeyId", logEncryptionKmsKeyId()).add("AdditionalInfo", additionalInfo())
.add("AmiVersion", amiVersion()).add("ReleaseLabel", releaseLabel()).add("Instances", instances())
.add("Steps", hasSteps() ? steps() : null)
.add("BootstrapActions", hasBootstrapActions() ? bootstrapActions() : null)
.add("SupportedProducts", hasSupportedProducts() ? supportedProducts() : null)
.add("NewSupportedProducts", hasNewSupportedProducts() ? newSupportedProducts() : null)
.add("Applications", hasApplications() ? applications() : null)
.add("Configurations", hasConfigurations() ? configurations() : null)
.add("VisibleToAllUsers", visibleToAllUsers()).add("JobFlowRole", jobFlowRole())
.add("ServiceRole", serviceRole()).add("Tags", hasTags() ? tags() : null)
.add("SecurityConfiguration", securityConfiguration()).add("AutoScalingRole", autoScalingRole())
.add("ScaleDownBehavior", scaleDownBehaviorAsString()).add("CustomAmiId", customAmiId())
.add("EbsRootVolumeSize", ebsRootVolumeSize()).add("RepoUpgradeOnBoot", repoUpgradeOnBootAsString())
.add("KerberosAttributes", kerberosAttributes()).add("StepConcurrencyLevel", stepConcurrencyLevel())
.add("ManagedScalingPolicy", managedScalingPolicy())
.add("PlacementGroupConfigs", hasPlacementGroupConfigs() ? placementGroupConfigs() : null)
.add("AutoTerminationPolicy", autoTerminationPolicy()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "Name":
return Optional.ofNullable(clazz.cast(name()));
case "LogUri":
return Optional.ofNullable(clazz.cast(logUri()));
case "LogEncryptionKmsKeyId":
return Optional.ofNullable(clazz.cast(logEncryptionKmsKeyId()));
case "AdditionalInfo":
return Optional.ofNullable(clazz.cast(additionalInfo()));
case "AmiVersion":
return Optional.ofNullable(clazz.cast(amiVersion()));
case "ReleaseLabel":
return Optional.ofNullable(clazz.cast(releaseLabel()));
case "Instances":
return Optional.ofNullable(clazz.cast(instances()));
case "Steps":
return Optional.ofNullable(clazz.cast(steps()));
case "BootstrapActions":
return Optional.ofNullable(clazz.cast(bootstrapActions()));
case "SupportedProducts":
return Optional.ofNullable(clazz.cast(supportedProducts()));
case "NewSupportedProducts":
return Optional.ofNullable(clazz.cast(newSupportedProducts()));
case "Applications":
return Optional.ofNullable(clazz.cast(applications()));
case "Configurations":
return Optional.ofNullable(clazz.cast(configurations()));
case "VisibleToAllUsers":
return Optional.ofNullable(clazz.cast(visibleToAllUsers()));
case "JobFlowRole":
return Optional.ofNullable(clazz.cast(jobFlowRole()));
case "ServiceRole":
return Optional.ofNullable(clazz.cast(serviceRole()));
case "Tags":
return Optional.ofNullable(clazz.cast(tags()));
case "SecurityConfiguration":
return Optional.ofNullable(clazz.cast(securityConfiguration()));
case "AutoScalingRole":
return Optional.ofNullable(clazz.cast(autoScalingRole()));
case "ScaleDownBehavior":
return Optional.ofNullable(clazz.cast(scaleDownBehaviorAsString()));
case "CustomAmiId":
return Optional.ofNullable(clazz.cast(customAmiId()));
case "EbsRootVolumeSize":
return Optional.ofNullable(clazz.cast(ebsRootVolumeSize()));
case "RepoUpgradeOnBoot":
return Optional.ofNullable(clazz.cast(repoUpgradeOnBootAsString()));
case "KerberosAttributes":
return Optional.ofNullable(clazz.cast(kerberosAttributes()));
case "StepConcurrencyLevel":
return Optional.ofNullable(clazz.cast(stepConcurrencyLevel()));
case "ManagedScalingPolicy":
return Optional.ofNullable(clazz.cast(managedScalingPolicy()));
case "PlacementGroupConfigs":
return Optional.ofNullable(clazz.cast(placementGroupConfigs()));
case "AutoTerminationPolicy":
return Optional.ofNullable(clazz.cast(autoTerminationPolicy()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
private static Function