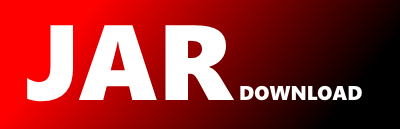
software.amazon.awssdk.services.emr.model.ComputeLimits Maven / Gradle / Ivy
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.emr.model;
import java.io.Serializable;
import java.util.Arrays;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* The Amazon EC2 unit limits for a managed scaling policy. The managed scaling activity of a cluster can not be above
* or below these limits. The limit only applies to the core and task nodes. The master node cannot be scaled after
* initial configuration.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class ComputeLimits implements SdkPojo, Serializable, ToCopyableBuilder {
private static final SdkField UNIT_TYPE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("UnitType").getter(getter(ComputeLimits::unitTypeAsString)).setter(setter(Builder::unitType))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("UnitType").build()).build();
private static final SdkField MINIMUM_CAPACITY_UNITS_FIELD = SdkField. builder(MarshallingType.INTEGER)
.memberName("MinimumCapacityUnits").getter(getter(ComputeLimits::minimumCapacityUnits))
.setter(setter(Builder::minimumCapacityUnits))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("MinimumCapacityUnits").build())
.build();
private static final SdkField MAXIMUM_CAPACITY_UNITS_FIELD = SdkField. builder(MarshallingType.INTEGER)
.memberName("MaximumCapacityUnits").getter(getter(ComputeLimits::maximumCapacityUnits))
.setter(setter(Builder::maximumCapacityUnits))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("MaximumCapacityUnits").build())
.build();
private static final SdkField MAXIMUM_ON_DEMAND_CAPACITY_UNITS_FIELD = SdkField
. builder(MarshallingType.INTEGER)
.memberName("MaximumOnDemandCapacityUnits")
.getter(getter(ComputeLimits::maximumOnDemandCapacityUnits))
.setter(setter(Builder::maximumOnDemandCapacityUnits))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("MaximumOnDemandCapacityUnits")
.build()).build();
private static final SdkField MAXIMUM_CORE_CAPACITY_UNITS_FIELD = SdkField
. builder(MarshallingType.INTEGER).memberName("MaximumCoreCapacityUnits")
.getter(getter(ComputeLimits::maximumCoreCapacityUnits)).setter(setter(Builder::maximumCoreCapacityUnits))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("MaximumCoreCapacityUnits").build())
.build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(UNIT_TYPE_FIELD,
MINIMUM_CAPACITY_UNITS_FIELD, MAXIMUM_CAPACITY_UNITS_FIELD, MAXIMUM_ON_DEMAND_CAPACITY_UNITS_FIELD,
MAXIMUM_CORE_CAPACITY_UNITS_FIELD));
private static final long serialVersionUID = 1L;
private final String unitType;
private final Integer minimumCapacityUnits;
private final Integer maximumCapacityUnits;
private final Integer maximumOnDemandCapacityUnits;
private final Integer maximumCoreCapacityUnits;
private ComputeLimits(BuilderImpl builder) {
this.unitType = builder.unitType;
this.minimumCapacityUnits = builder.minimumCapacityUnits;
this.maximumCapacityUnits = builder.maximumCapacityUnits;
this.maximumOnDemandCapacityUnits = builder.maximumOnDemandCapacityUnits;
this.maximumCoreCapacityUnits = builder.maximumCoreCapacityUnits;
}
/**
*
* The unit type used for specifying a managed scaling policy.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #unitType} will
* return {@link ComputeLimitsUnitType#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available
* from {@link #unitTypeAsString}.
*
*
* @return The unit type used for specifying a managed scaling policy.
* @see ComputeLimitsUnitType
*/
public final ComputeLimitsUnitType unitType() {
return ComputeLimitsUnitType.fromValue(unitType);
}
/**
*
* The unit type used for specifying a managed scaling policy.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #unitType} will
* return {@link ComputeLimitsUnitType#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available
* from {@link #unitTypeAsString}.
*
*
* @return The unit type used for specifying a managed scaling policy.
* @see ComputeLimitsUnitType
*/
public final String unitTypeAsString() {
return unitType;
}
/**
*
* The lower boundary of Amazon EC2 units. It is measured through vCPU cores or instances for instance groups and
* measured through units for instance fleets. Managed scaling activities are not allowed beyond this boundary. The
* limit only applies to the core and task nodes. The master node cannot be scaled after initial configuration.
*
*
* @return The lower boundary of Amazon EC2 units. It is measured through vCPU cores or instances for instance
* groups and measured through units for instance fleets. Managed scaling activities are not allowed beyond
* this boundary. The limit only applies to the core and task nodes. The master node cannot be scaled after
* initial configuration.
*/
public final Integer minimumCapacityUnits() {
return minimumCapacityUnits;
}
/**
*
* The upper boundary of Amazon EC2 units. It is measured through vCPU cores or instances for instance groups and
* measured through units for instance fleets. Managed scaling activities are not allowed beyond this boundary. The
* limit only applies to the core and task nodes. The master node cannot be scaled after initial configuration.
*
*
* @return The upper boundary of Amazon EC2 units. It is measured through vCPU cores or instances for instance
* groups and measured through units for instance fleets. Managed scaling activities are not allowed beyond
* this boundary. The limit only applies to the core and task nodes. The master node cannot be scaled after
* initial configuration.
*/
public final Integer maximumCapacityUnits() {
return maximumCapacityUnits;
}
/**
*
* The upper boundary of On-Demand Amazon EC2 units. It is measured through vCPU cores or instances for instance
* groups and measured through units for instance fleets. The On-Demand units are not allowed to scale beyond this
* boundary. The parameter is used to split capacity allocation between On-Demand and Spot Instances.
*
*
* @return The upper boundary of On-Demand Amazon EC2 units. It is measured through vCPU cores or instances for
* instance groups and measured through units for instance fleets. The On-Demand units are not allowed to
* scale beyond this boundary. The parameter is used to split capacity allocation between On-Demand and Spot
* Instances.
*/
public final Integer maximumOnDemandCapacityUnits() {
return maximumOnDemandCapacityUnits;
}
/**
*
* The upper boundary of Amazon EC2 units for core node type in a cluster. It is measured through vCPU cores or
* instances for instance groups and measured through units for instance fleets. The core units are not allowed to
* scale beyond this boundary. The parameter is used to split capacity allocation between core and task nodes.
*
*
* @return The upper boundary of Amazon EC2 units for core node type in a cluster. It is measured through vCPU cores
* or instances for instance groups and measured through units for instance fleets. The core units are not
* allowed to scale beyond this boundary. The parameter is used to split capacity allocation between core
* and task nodes.
*/
public final Integer maximumCoreCapacityUnits() {
return maximumCoreCapacityUnits;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(unitTypeAsString());
hashCode = 31 * hashCode + Objects.hashCode(minimumCapacityUnits());
hashCode = 31 * hashCode + Objects.hashCode(maximumCapacityUnits());
hashCode = 31 * hashCode + Objects.hashCode(maximumOnDemandCapacityUnits());
hashCode = 31 * hashCode + Objects.hashCode(maximumCoreCapacityUnits());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof ComputeLimits)) {
return false;
}
ComputeLimits other = (ComputeLimits) obj;
return Objects.equals(unitTypeAsString(), other.unitTypeAsString())
&& Objects.equals(minimumCapacityUnits(), other.minimumCapacityUnits())
&& Objects.equals(maximumCapacityUnits(), other.maximumCapacityUnits())
&& Objects.equals(maximumOnDemandCapacityUnits(), other.maximumOnDemandCapacityUnits())
&& Objects.equals(maximumCoreCapacityUnits(), other.maximumCoreCapacityUnits());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("ComputeLimits").add("UnitType", unitTypeAsString())
.add("MinimumCapacityUnits", minimumCapacityUnits()).add("MaximumCapacityUnits", maximumCapacityUnits())
.add("MaximumOnDemandCapacityUnits", maximumOnDemandCapacityUnits())
.add("MaximumCoreCapacityUnits", maximumCoreCapacityUnits()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "UnitType":
return Optional.ofNullable(clazz.cast(unitTypeAsString()));
case "MinimumCapacityUnits":
return Optional.ofNullable(clazz.cast(minimumCapacityUnits()));
case "MaximumCapacityUnits":
return Optional.ofNullable(clazz.cast(maximumCapacityUnits()));
case "MaximumOnDemandCapacityUnits":
return Optional.ofNullable(clazz.cast(maximumOnDemandCapacityUnits()));
case "MaximumCoreCapacityUnits":
return Optional.ofNullable(clazz.cast(maximumCoreCapacityUnits()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
private static Function