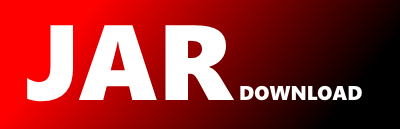
software.amazon.awssdk.services.emr.model.ScalingRule Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of emr Show documentation
Show all versions of emr Show documentation
The AWS Java SDK for Amazon EMR module holds the client classes that are used for communicating with
Amazon Elastic MapReduce Service
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.emr.model;
import java.io.Serializable;
import java.util.Arrays;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* A scale-in or scale-out rule that defines scaling activity, including the CloudWatch metric alarm that triggers
* activity, how EC2 instances are added or removed, and the periodicity of adjustments. The automatic scaling policy
* for an instance group can comprise one or more automatic scaling rules.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class ScalingRule implements SdkPojo, Serializable, ToCopyableBuilder {
private static final SdkField NAME_FIELD = SdkField. builder(MarshallingType.STRING).memberName("Name")
.getter(getter(ScalingRule::name)).setter(setter(Builder::name))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Name").build()).build();
private static final SdkField DESCRIPTION_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("Description").getter(getter(ScalingRule::description)).setter(setter(Builder::description))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Description").build()).build();
private static final SdkField ACTION_FIELD = SdkField. builder(MarshallingType.SDK_POJO)
.memberName("Action").getter(getter(ScalingRule::action)).setter(setter(Builder::action))
.constructor(ScalingAction::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Action").build()).build();
private static final SdkField TRIGGER_FIELD = SdkField. builder(MarshallingType.SDK_POJO)
.memberName("Trigger").getter(getter(ScalingRule::trigger)).setter(setter(Builder::trigger))
.constructor(ScalingTrigger::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Trigger").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(NAME_FIELD, DESCRIPTION_FIELD,
ACTION_FIELD, TRIGGER_FIELD));
private static final long serialVersionUID = 1L;
private final String name;
private final String description;
private final ScalingAction action;
private final ScalingTrigger trigger;
private ScalingRule(BuilderImpl builder) {
this.name = builder.name;
this.description = builder.description;
this.action = builder.action;
this.trigger = builder.trigger;
}
/**
*
* The name used to identify an automatic scaling rule. Rule names must be unique within a scaling policy.
*
*
* @return The name used to identify an automatic scaling rule. Rule names must be unique within a scaling policy.
*/
public final String name() {
return name;
}
/**
*
* A friendly, more verbose description of the automatic scaling rule.
*
*
* @return A friendly, more verbose description of the automatic scaling rule.
*/
public final String description() {
return description;
}
/**
*
* The conditions that trigger an automatic scaling activity.
*
*
* @return The conditions that trigger an automatic scaling activity.
*/
public final ScalingAction action() {
return action;
}
/**
*
* The CloudWatch alarm definition that determines when automatic scaling activity is triggered.
*
*
* @return The CloudWatch alarm definition that determines when automatic scaling activity is triggered.
*/
public final ScalingTrigger trigger() {
return trigger;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(name());
hashCode = 31 * hashCode + Objects.hashCode(description());
hashCode = 31 * hashCode + Objects.hashCode(action());
hashCode = 31 * hashCode + Objects.hashCode(trigger());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof ScalingRule)) {
return false;
}
ScalingRule other = (ScalingRule) obj;
return Objects.equals(name(), other.name()) && Objects.equals(description(), other.description())
&& Objects.equals(action(), other.action()) && Objects.equals(trigger(), other.trigger());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("ScalingRule").add("Name", name()).add("Description", description()).add("Action", action())
.add("Trigger", trigger()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "Name":
return Optional.ofNullable(clazz.cast(name()));
case "Description":
return Optional.ofNullable(clazz.cast(description()));
case "Action":
return Optional.ofNullable(clazz.cast(action()));
case "Trigger":
return Optional.ofNullable(clazz.cast(trigger()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
private static Function
© 2015 - 2025 Weber Informatics LLC | Privacy Policy