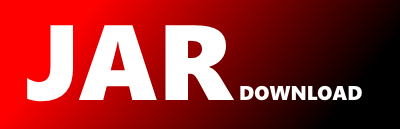
software.amazon.awssdk.services.emr.model.CloudWatchAlarmDefinition Maven / Gradle / Ivy
Show all versions of emr Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.emr.model;
import java.io.Serializable;
import java.util.Arrays;
import java.util.Collection;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import java.util.stream.Collectors;
import java.util.stream.Stream;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.ListTrait;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.core.util.DefaultSdkAutoConstructList;
import software.amazon.awssdk.core.util.SdkAutoConstructList;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* The definition of a CloudWatch metric alarm, which determines when an automatic scaling activity is triggered. When
* the defined alarm conditions are satisfied, scaling activity begins.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class CloudWatchAlarmDefinition implements SdkPojo, Serializable,
ToCopyableBuilder {
private static final SdkField COMPARISON_OPERATOR_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("ComparisonOperator").getter(getter(CloudWatchAlarmDefinition::comparisonOperatorAsString))
.setter(setter(Builder::comparisonOperator))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ComparisonOperator").build())
.build();
private static final SdkField EVALUATION_PERIODS_FIELD = SdkField. builder(MarshallingType.INTEGER)
.memberName("EvaluationPeriods").getter(getter(CloudWatchAlarmDefinition::evaluationPeriods))
.setter(setter(Builder::evaluationPeriods))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("EvaluationPeriods").build()).build();
private static final SdkField METRIC_NAME_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("MetricName").getter(getter(CloudWatchAlarmDefinition::metricName)).setter(setter(Builder::metricName))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("MetricName").build()).build();
private static final SdkField NAMESPACE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("Namespace").getter(getter(CloudWatchAlarmDefinition::namespace)).setter(setter(Builder::namespace))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Namespace").build()).build();
private static final SdkField PERIOD_FIELD = SdkField. builder(MarshallingType.INTEGER)
.memberName("Period").getter(getter(CloudWatchAlarmDefinition::period)).setter(setter(Builder::period))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Period").build()).build();
private static final SdkField STATISTIC_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("Statistic").getter(getter(CloudWatchAlarmDefinition::statisticAsString))
.setter(setter(Builder::statistic))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Statistic").build()).build();
private static final SdkField THRESHOLD_FIELD = SdkField. builder(MarshallingType.DOUBLE)
.memberName("Threshold").getter(getter(CloudWatchAlarmDefinition::threshold)).setter(setter(Builder::threshold))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Threshold").build()).build();
private static final SdkField UNIT_FIELD = SdkField. builder(MarshallingType.STRING).memberName("Unit")
.getter(getter(CloudWatchAlarmDefinition::unitAsString)).setter(setter(Builder::unit))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Unit").build()).build();
private static final SdkField> DIMENSIONS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("Dimensions")
.getter(getter(CloudWatchAlarmDefinition::dimensions))
.setter(setter(Builder::dimensions))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Dimensions").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(MetricDimension::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(COMPARISON_OPERATOR_FIELD,
EVALUATION_PERIODS_FIELD, METRIC_NAME_FIELD, NAMESPACE_FIELD, PERIOD_FIELD, STATISTIC_FIELD, THRESHOLD_FIELD,
UNIT_FIELD, DIMENSIONS_FIELD));
private static final long serialVersionUID = 1L;
private final String comparisonOperator;
private final Integer evaluationPeriods;
private final String metricName;
private final String namespace;
private final Integer period;
private final String statistic;
private final Double threshold;
private final String unit;
private final List dimensions;
private CloudWatchAlarmDefinition(BuilderImpl builder) {
this.comparisonOperator = builder.comparisonOperator;
this.evaluationPeriods = builder.evaluationPeriods;
this.metricName = builder.metricName;
this.namespace = builder.namespace;
this.period = builder.period;
this.statistic = builder.statistic;
this.threshold = builder.threshold;
this.unit = builder.unit;
this.dimensions = builder.dimensions;
}
/**
*
* Determines how the metric specified by MetricName
is compared to the value specified by
* Threshold
.
*
*
* If the service returns an enum value that is not available in the current SDK version,
* {@link #comparisonOperator} will return {@link ComparisonOperator#UNKNOWN_TO_SDK_VERSION}. The raw value returned
* by the service is available from {@link #comparisonOperatorAsString}.
*
*
* @return Determines how the metric specified by MetricName
is compared to the value specified by
* Threshold
.
* @see ComparisonOperator
*/
public final ComparisonOperator comparisonOperator() {
return ComparisonOperator.fromValue(comparisonOperator);
}
/**
*
* Determines how the metric specified by MetricName
is compared to the value specified by
* Threshold
.
*
*
* If the service returns an enum value that is not available in the current SDK version,
* {@link #comparisonOperator} will return {@link ComparisonOperator#UNKNOWN_TO_SDK_VERSION}. The raw value returned
* by the service is available from {@link #comparisonOperatorAsString}.
*
*
* @return Determines how the metric specified by MetricName
is compared to the value specified by
* Threshold
.
* @see ComparisonOperator
*/
public final String comparisonOperatorAsString() {
return comparisonOperator;
}
/**
*
* The number of periods, in five-minute increments, during which the alarm condition must exist before the alarm
* triggers automatic scaling activity. The default value is 1
.
*
*
* @return The number of periods, in five-minute increments, during which the alarm condition must exist before the
* alarm triggers automatic scaling activity. The default value is 1
.
*/
public final Integer evaluationPeriods() {
return evaluationPeriods;
}
/**
*
* The name of the CloudWatch metric that is watched to determine an alarm condition.
*
*
* @return The name of the CloudWatch metric that is watched to determine an alarm condition.
*/
public final String metricName() {
return metricName;
}
/**
*
* The namespace for the CloudWatch metric. The default is AWS/ElasticMapReduce
.
*
*
* @return The namespace for the CloudWatch metric. The default is AWS/ElasticMapReduce
.
*/
public final String namespace() {
return namespace;
}
/**
*
* The period, in seconds, over which the statistic is applied. CloudWatch metrics for Amazon EMR are emitted every
* five minutes (300 seconds), so if you specify a CloudWatch metric, specify 300
.
*
*
* @return The period, in seconds, over which the statistic is applied. CloudWatch metrics for Amazon EMR are
* emitted every five minutes (300 seconds), so if you specify a CloudWatch metric, specify 300
* .
*/
public final Integer period() {
return period;
}
/**
*
* The statistic to apply to the metric associated with the alarm. The default is AVERAGE
.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #statistic} will
* return {@link Statistic#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #statisticAsString}.
*
*
* @return The statistic to apply to the metric associated with the alarm. The default is AVERAGE
.
* @see Statistic
*/
public final Statistic statistic() {
return Statistic.fromValue(statistic);
}
/**
*
* The statistic to apply to the metric associated with the alarm. The default is AVERAGE
.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #statistic} will
* return {@link Statistic#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #statisticAsString}.
*
*
* @return The statistic to apply to the metric associated with the alarm. The default is AVERAGE
.
* @see Statistic
*/
public final String statisticAsString() {
return statistic;
}
/**
*
* The value against which the specified statistic is compared.
*
*
* @return The value against which the specified statistic is compared.
*/
public final Double threshold() {
return threshold;
}
/**
*
* The unit of measure associated with the CloudWatch metric being watched. The value specified for
* Unit
must correspond to the units specified in the CloudWatch metric.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #unit} will return
* {@link Unit#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #unitAsString}.
*
*
* @return The unit of measure associated with the CloudWatch metric being watched. The value specified for
* Unit
must correspond to the units specified in the CloudWatch metric.
* @see Unit
*/
public final Unit unit() {
return Unit.fromValue(unit);
}
/**
*
* The unit of measure associated with the CloudWatch metric being watched. The value specified for
* Unit
must correspond to the units specified in the CloudWatch metric.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #unit} will return
* {@link Unit#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #unitAsString}.
*
*
* @return The unit of measure associated with the CloudWatch metric being watched. The value specified for
* Unit
must correspond to the units specified in the CloudWatch metric.
* @see Unit
*/
public final String unitAsString() {
return unit;
}
/**
* For responses, this returns true if the service returned a value for the Dimensions property. This DOES NOT check
* that the value is non-empty (for which, you should check the {@code isEmpty()} method on the property). This is
* useful because the SDK will never return a null collection or map, but you may need to differentiate between the
* service returning nothing (or null) and the service returning an empty collection or map. For requests, this
* returns true if a value for the property was specified in the request builder, and false if a value was not
* specified.
*/
public final boolean hasDimensions() {
return dimensions != null && !(dimensions instanceof SdkAutoConstructList);
}
/**
*
* A CloudWatch metric dimension.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasDimensions} method.
*
*
* @return A CloudWatch metric dimension.
*/
public final List dimensions() {
return dimensions;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(comparisonOperatorAsString());
hashCode = 31 * hashCode + Objects.hashCode(evaluationPeriods());
hashCode = 31 * hashCode + Objects.hashCode(metricName());
hashCode = 31 * hashCode + Objects.hashCode(namespace());
hashCode = 31 * hashCode + Objects.hashCode(period());
hashCode = 31 * hashCode + Objects.hashCode(statisticAsString());
hashCode = 31 * hashCode + Objects.hashCode(threshold());
hashCode = 31 * hashCode + Objects.hashCode(unitAsString());
hashCode = 31 * hashCode + Objects.hashCode(hasDimensions() ? dimensions() : null);
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof CloudWatchAlarmDefinition)) {
return false;
}
CloudWatchAlarmDefinition other = (CloudWatchAlarmDefinition) obj;
return Objects.equals(comparisonOperatorAsString(), other.comparisonOperatorAsString())
&& Objects.equals(evaluationPeriods(), other.evaluationPeriods())
&& Objects.equals(metricName(), other.metricName()) && Objects.equals(namespace(), other.namespace())
&& Objects.equals(period(), other.period()) && Objects.equals(statisticAsString(), other.statisticAsString())
&& Objects.equals(threshold(), other.threshold()) && Objects.equals(unitAsString(), other.unitAsString())
&& hasDimensions() == other.hasDimensions() && Objects.equals(dimensions(), other.dimensions());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("CloudWatchAlarmDefinition").add("ComparisonOperator", comparisonOperatorAsString())
.add("EvaluationPeriods", evaluationPeriods()).add("MetricName", metricName()).add("Namespace", namespace())
.add("Period", period()).add("Statistic", statisticAsString()).add("Threshold", threshold())
.add("Unit", unitAsString()).add("Dimensions", hasDimensions() ? dimensions() : null).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "ComparisonOperator":
return Optional.ofNullable(clazz.cast(comparisonOperatorAsString()));
case "EvaluationPeriods":
return Optional.ofNullable(clazz.cast(evaluationPeriods()));
case "MetricName":
return Optional.ofNullable(clazz.cast(metricName()));
case "Namespace":
return Optional.ofNullable(clazz.cast(namespace()));
case "Period":
return Optional.ofNullable(clazz.cast(period()));
case "Statistic":
return Optional.ofNullable(clazz.cast(statisticAsString()));
case "Threshold":
return Optional.ofNullable(clazz.cast(threshold()));
case "Unit":
return Optional.ofNullable(clazz.cast(unitAsString()));
case "Dimensions":
return Optional.ofNullable(clazz.cast(dimensions()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
private static Function