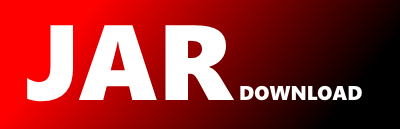
software.amazon.awssdk.services.emr.model.DeleteStudioSessionMappingRequest Maven / Gradle / Ivy
Show all versions of emr Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.emr.model;
import java.util.Arrays;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.awscore.AwsRequestOverrideConfiguration;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*/
@Generated("software.amazon.awssdk:codegen")
public final class DeleteStudioSessionMappingRequest extends EmrRequest implements
ToCopyableBuilder {
private static final SdkField STUDIO_ID_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("StudioId").getter(getter(DeleteStudioSessionMappingRequest::studioId)).setter(setter(Builder::studioId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("StudioId").build()).build();
private static final SdkField IDENTITY_ID_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("IdentityId").getter(getter(DeleteStudioSessionMappingRequest::identityId))
.setter(setter(Builder::identityId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("IdentityId").build()).build();
private static final SdkField IDENTITY_NAME_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("IdentityName").getter(getter(DeleteStudioSessionMappingRequest::identityName))
.setter(setter(Builder::identityName))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("IdentityName").build()).build();
private static final SdkField IDENTITY_TYPE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("IdentityType").getter(getter(DeleteStudioSessionMappingRequest::identityTypeAsString))
.setter(setter(Builder::identityType))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("IdentityType").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(STUDIO_ID_FIELD,
IDENTITY_ID_FIELD, IDENTITY_NAME_FIELD, IDENTITY_TYPE_FIELD));
private final String studioId;
private final String identityId;
private final String identityName;
private final String identityType;
private DeleteStudioSessionMappingRequest(BuilderImpl builder) {
super(builder);
this.studioId = builder.studioId;
this.identityId = builder.identityId;
this.identityName = builder.identityName;
this.identityType = builder.identityType;
}
/**
*
* The ID of the Amazon EMR Studio.
*
*
* @return The ID of the Amazon EMR Studio.
*/
public final String studioId() {
return studioId;
}
/**
*
* The globally unique identifier (GUID) of the user or group to remove from the Amazon EMR Studio. For more
* information, see UserId and GroupId in the IAM Identity Center Identity Store API Reference. Either IdentityName
or
* IdentityId
must be specified.
*
*
* @return The globally unique identifier (GUID) of the user or group to remove from the Amazon EMR Studio. For more
* information, see UserId and GroupId in the IAM Identity Center Identity Store API Reference. Either
* IdentityName
or IdentityId
must be specified.
*/
public final String identityId() {
return identityId;
}
/**
*
* The name of the user name or group to remove from the Amazon EMR Studio. For more information, see UserName and DisplayName in the IAM Identity Center Store API Reference. Either IdentityName
or
* IdentityId
must be specified.
*
*
* @return The name of the user name or group to remove from the Amazon EMR Studio. For more information, see UserName and DisplayName in the IAM Identity Center Store API Reference. Either IdentityName
* or IdentityId
must be specified.
*/
public final String identityName() {
return identityName;
}
/**
*
* Specifies whether the identity to delete from the Amazon EMR Studio is a user or a group.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #identityType} will
* return {@link IdentityType#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #identityTypeAsString}.
*
*
* @return Specifies whether the identity to delete from the Amazon EMR Studio is a user or a group.
* @see IdentityType
*/
public final IdentityType identityType() {
return IdentityType.fromValue(identityType);
}
/**
*
* Specifies whether the identity to delete from the Amazon EMR Studio is a user or a group.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #identityType} will
* return {@link IdentityType#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #identityTypeAsString}.
*
*
* @return Specifies whether the identity to delete from the Amazon EMR Studio is a user or a group.
* @see IdentityType
*/
public final String identityTypeAsString() {
return identityType;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + super.hashCode();
hashCode = 31 * hashCode + Objects.hashCode(studioId());
hashCode = 31 * hashCode + Objects.hashCode(identityId());
hashCode = 31 * hashCode + Objects.hashCode(identityName());
hashCode = 31 * hashCode + Objects.hashCode(identityTypeAsString());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return super.equals(obj) && equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof DeleteStudioSessionMappingRequest)) {
return false;
}
DeleteStudioSessionMappingRequest other = (DeleteStudioSessionMappingRequest) obj;
return Objects.equals(studioId(), other.studioId()) && Objects.equals(identityId(), other.identityId())
&& Objects.equals(identityName(), other.identityName())
&& Objects.equals(identityTypeAsString(), other.identityTypeAsString());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("DeleteStudioSessionMappingRequest").add("StudioId", studioId()).add("IdentityId", identityId())
.add("IdentityName", identityName()).add("IdentityType", identityTypeAsString()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "StudioId":
return Optional.ofNullable(clazz.cast(studioId()));
case "IdentityId":
return Optional.ofNullable(clazz.cast(identityId()));
case "IdentityName":
return Optional.ofNullable(clazz.cast(identityName()));
case "IdentityType":
return Optional.ofNullable(clazz.cast(identityTypeAsString()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
private static Function