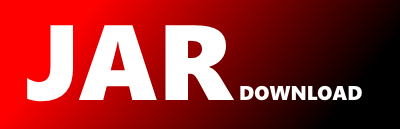
software.amazon.awssdk.services.emr.model.Cluster Maven / Gradle / Ivy
Show all versions of emr Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.emr.model;
import java.io.Serializable;
import java.util.Arrays;
import java.util.Collection;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import java.util.stream.Collectors;
import java.util.stream.Stream;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.ListTrait;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.core.util.DefaultSdkAutoConstructList;
import software.amazon.awssdk.core.util.SdkAutoConstructList;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* The detailed description of the cluster.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class Cluster implements SdkPojo, Serializable, ToCopyableBuilder {
private static final SdkField ID_FIELD = SdkField. builder(MarshallingType.STRING).memberName("Id")
.getter(getter(Cluster::id)).setter(setter(Builder::id))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Id").build()).build();
private static final SdkField NAME_FIELD = SdkField. builder(MarshallingType.STRING).memberName("Name")
.getter(getter(Cluster::name)).setter(setter(Builder::name))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Name").build()).build();
private static final SdkField STATUS_FIELD = SdkField. builder(MarshallingType.SDK_POJO)
.memberName("Status").getter(getter(Cluster::status)).setter(setter(Builder::status))
.constructor(ClusterStatus::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Status").build()).build();
private static final SdkField EC2_INSTANCE_ATTRIBUTES_FIELD = SdkField
. builder(MarshallingType.SDK_POJO).memberName("Ec2InstanceAttributes")
.getter(getter(Cluster::ec2InstanceAttributes)).setter(setter(Builder::ec2InstanceAttributes))
.constructor(Ec2InstanceAttributes::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Ec2InstanceAttributes").build())
.build();
private static final SdkField INSTANCE_COLLECTION_TYPE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("InstanceCollectionType").getter(getter(Cluster::instanceCollectionTypeAsString))
.setter(setter(Builder::instanceCollectionType))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("InstanceCollectionType").build())
.build();
private static final SdkField LOG_URI_FIELD = SdkField. builder(MarshallingType.STRING).memberName("LogUri")
.getter(getter(Cluster::logUri)).setter(setter(Builder::logUri))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("LogUri").build()).build();
private static final SdkField LOG_ENCRYPTION_KMS_KEY_ID_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("LogEncryptionKmsKeyId").getter(getter(Cluster::logEncryptionKmsKeyId))
.setter(setter(Builder::logEncryptionKmsKeyId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("LogEncryptionKmsKeyId").build())
.build();
private static final SdkField REQUESTED_AMI_VERSION_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("RequestedAmiVersion").getter(getter(Cluster::requestedAmiVersion))
.setter(setter(Builder::requestedAmiVersion))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("RequestedAmiVersion").build())
.build();
private static final SdkField RUNNING_AMI_VERSION_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("RunningAmiVersion").getter(getter(Cluster::runningAmiVersion))
.setter(setter(Builder::runningAmiVersion))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("RunningAmiVersion").build()).build();
private static final SdkField RELEASE_LABEL_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("ReleaseLabel").getter(getter(Cluster::releaseLabel)).setter(setter(Builder::releaseLabel))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ReleaseLabel").build()).build();
private static final SdkField AUTO_TERMINATE_FIELD = SdkField. builder(MarshallingType.BOOLEAN)
.memberName("AutoTerminate").getter(getter(Cluster::autoTerminate)).setter(setter(Builder::autoTerminate))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("AutoTerminate").build()).build();
private static final SdkField TERMINATION_PROTECTED_FIELD = SdkField. builder(MarshallingType.BOOLEAN)
.memberName("TerminationProtected").getter(getter(Cluster::terminationProtected))
.setter(setter(Builder::terminationProtected))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("TerminationProtected").build())
.build();
private static final SdkField UNHEALTHY_NODE_REPLACEMENT_FIELD = SdkField. builder(MarshallingType.BOOLEAN)
.memberName("UnhealthyNodeReplacement").getter(getter(Cluster::unhealthyNodeReplacement))
.setter(setter(Builder::unhealthyNodeReplacement))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("UnhealthyNodeReplacement").build())
.build();
private static final SdkField VISIBLE_TO_ALL_USERS_FIELD = SdkField. builder(MarshallingType.BOOLEAN)
.memberName("VisibleToAllUsers").getter(getter(Cluster::visibleToAllUsers))
.setter(setter(Builder::visibleToAllUsers))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("VisibleToAllUsers").build()).build();
private static final SdkField> APPLICATIONS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("Applications")
.getter(getter(Cluster::applications))
.setter(setter(Builder::applications))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Applications").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(Application::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField> TAGS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("Tags")
.getter(getter(Cluster::tags))
.setter(setter(Builder::tags))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Tags").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(Tag::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField SERVICE_ROLE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("ServiceRole").getter(getter(Cluster::serviceRole)).setter(setter(Builder::serviceRole))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ServiceRole").build()).build();
private static final SdkField NORMALIZED_INSTANCE_HOURS_FIELD = SdkField. builder(MarshallingType.INTEGER)
.memberName("NormalizedInstanceHours").getter(getter(Cluster::normalizedInstanceHours))
.setter(setter(Builder::normalizedInstanceHours))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("NormalizedInstanceHours").build())
.build();
private static final SdkField MASTER_PUBLIC_DNS_NAME_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("MasterPublicDnsName").getter(getter(Cluster::masterPublicDnsName))
.setter(setter(Builder::masterPublicDnsName))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("MasterPublicDnsName").build())
.build();
private static final SdkField> CONFIGURATIONS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("Configurations")
.getter(getter(Cluster::configurations))
.setter(setter(Builder::configurations))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Configurations").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(Configuration::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField SECURITY_CONFIGURATION_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("SecurityConfiguration").getter(getter(Cluster::securityConfiguration))
.setter(setter(Builder::securityConfiguration))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("SecurityConfiguration").build())
.build();
private static final SdkField AUTO_SCALING_ROLE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("AutoScalingRole").getter(getter(Cluster::autoScalingRole)).setter(setter(Builder::autoScalingRole))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("AutoScalingRole").build()).build();
private static final SdkField SCALE_DOWN_BEHAVIOR_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("ScaleDownBehavior").getter(getter(Cluster::scaleDownBehaviorAsString))
.setter(setter(Builder::scaleDownBehavior))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ScaleDownBehavior").build()).build();
private static final SdkField CUSTOM_AMI_ID_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("CustomAmiId").getter(getter(Cluster::customAmiId)).setter(setter(Builder::customAmiId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("CustomAmiId").build()).build();
private static final SdkField EBS_ROOT_VOLUME_SIZE_FIELD = SdkField. builder(MarshallingType.INTEGER)
.memberName("EbsRootVolumeSize").getter(getter(Cluster::ebsRootVolumeSize))
.setter(setter(Builder::ebsRootVolumeSize))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("EbsRootVolumeSize").build()).build();
private static final SdkField REPO_UPGRADE_ON_BOOT_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("RepoUpgradeOnBoot").getter(getter(Cluster::repoUpgradeOnBootAsString))
.setter(setter(Builder::repoUpgradeOnBoot))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("RepoUpgradeOnBoot").build()).build();
private static final SdkField KERBEROS_ATTRIBUTES_FIELD = SdkField
. builder(MarshallingType.SDK_POJO).memberName("KerberosAttributes")
.getter(getter(Cluster::kerberosAttributes)).setter(setter(Builder::kerberosAttributes))
.constructor(KerberosAttributes::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("KerberosAttributes").build())
.build();
private static final SdkField CLUSTER_ARN_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("ClusterArn").getter(getter(Cluster::clusterArn)).setter(setter(Builder::clusterArn))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ClusterArn").build()).build();
private static final SdkField OUTPOST_ARN_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("OutpostArn").getter(getter(Cluster::outpostArn)).setter(setter(Builder::outpostArn))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("OutpostArn").build()).build();
private static final SdkField STEP_CONCURRENCY_LEVEL_FIELD = SdkField. builder(MarshallingType.INTEGER)
.memberName("StepConcurrencyLevel").getter(getter(Cluster::stepConcurrencyLevel))
.setter(setter(Builder::stepConcurrencyLevel))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("StepConcurrencyLevel").build())
.build();
private static final SdkField> PLACEMENT_GROUPS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("PlacementGroups")
.getter(getter(Cluster::placementGroups))
.setter(setter(Builder::placementGroups))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("PlacementGroups").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(PlacementGroupConfig::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField OS_RELEASE_LABEL_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("OSReleaseLabel").getter(getter(Cluster::osReleaseLabel)).setter(setter(Builder::osReleaseLabel))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("OSReleaseLabel").build()).build();
private static final SdkField EBS_ROOT_VOLUME_IOPS_FIELD = SdkField. builder(MarshallingType.INTEGER)
.memberName("EbsRootVolumeIops").getter(getter(Cluster::ebsRootVolumeIops))
.setter(setter(Builder::ebsRootVolumeIops))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("EbsRootVolumeIops").build()).build();
private static final SdkField EBS_ROOT_VOLUME_THROUGHPUT_FIELD = SdkField. builder(MarshallingType.INTEGER)
.memberName("EbsRootVolumeThroughput").getter(getter(Cluster::ebsRootVolumeThroughput))
.setter(setter(Builder::ebsRootVolumeThroughput))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("EbsRootVolumeThroughput").build())
.build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(ID_FIELD, NAME_FIELD,
STATUS_FIELD, EC2_INSTANCE_ATTRIBUTES_FIELD, INSTANCE_COLLECTION_TYPE_FIELD, LOG_URI_FIELD,
LOG_ENCRYPTION_KMS_KEY_ID_FIELD, REQUESTED_AMI_VERSION_FIELD, RUNNING_AMI_VERSION_FIELD, RELEASE_LABEL_FIELD,
AUTO_TERMINATE_FIELD, TERMINATION_PROTECTED_FIELD, UNHEALTHY_NODE_REPLACEMENT_FIELD, VISIBLE_TO_ALL_USERS_FIELD,
APPLICATIONS_FIELD, TAGS_FIELD, SERVICE_ROLE_FIELD, NORMALIZED_INSTANCE_HOURS_FIELD, MASTER_PUBLIC_DNS_NAME_FIELD,
CONFIGURATIONS_FIELD, SECURITY_CONFIGURATION_FIELD, AUTO_SCALING_ROLE_FIELD, SCALE_DOWN_BEHAVIOR_FIELD,
CUSTOM_AMI_ID_FIELD, EBS_ROOT_VOLUME_SIZE_FIELD, REPO_UPGRADE_ON_BOOT_FIELD, KERBEROS_ATTRIBUTES_FIELD,
CLUSTER_ARN_FIELD, OUTPOST_ARN_FIELD, STEP_CONCURRENCY_LEVEL_FIELD, PLACEMENT_GROUPS_FIELD, OS_RELEASE_LABEL_FIELD,
EBS_ROOT_VOLUME_IOPS_FIELD, EBS_ROOT_VOLUME_THROUGHPUT_FIELD));
private static final long serialVersionUID = 1L;
private final String id;
private final String name;
private final ClusterStatus status;
private final Ec2InstanceAttributes ec2InstanceAttributes;
private final String instanceCollectionType;
private final String logUri;
private final String logEncryptionKmsKeyId;
private final String requestedAmiVersion;
private final String runningAmiVersion;
private final String releaseLabel;
private final Boolean autoTerminate;
private final Boolean terminationProtected;
private final Boolean unhealthyNodeReplacement;
private final Boolean visibleToAllUsers;
private final List applications;
private final List tags;
private final String serviceRole;
private final Integer normalizedInstanceHours;
private final String masterPublicDnsName;
private final List configurations;
private final String securityConfiguration;
private final String autoScalingRole;
private final String scaleDownBehavior;
private final String customAmiId;
private final Integer ebsRootVolumeSize;
private final String repoUpgradeOnBoot;
private final KerberosAttributes kerberosAttributes;
private final String clusterArn;
private final String outpostArn;
private final Integer stepConcurrencyLevel;
private final List placementGroups;
private final String osReleaseLabel;
private final Integer ebsRootVolumeIops;
private final Integer ebsRootVolumeThroughput;
private Cluster(BuilderImpl builder) {
this.id = builder.id;
this.name = builder.name;
this.status = builder.status;
this.ec2InstanceAttributes = builder.ec2InstanceAttributes;
this.instanceCollectionType = builder.instanceCollectionType;
this.logUri = builder.logUri;
this.logEncryptionKmsKeyId = builder.logEncryptionKmsKeyId;
this.requestedAmiVersion = builder.requestedAmiVersion;
this.runningAmiVersion = builder.runningAmiVersion;
this.releaseLabel = builder.releaseLabel;
this.autoTerminate = builder.autoTerminate;
this.terminationProtected = builder.terminationProtected;
this.unhealthyNodeReplacement = builder.unhealthyNodeReplacement;
this.visibleToAllUsers = builder.visibleToAllUsers;
this.applications = builder.applications;
this.tags = builder.tags;
this.serviceRole = builder.serviceRole;
this.normalizedInstanceHours = builder.normalizedInstanceHours;
this.masterPublicDnsName = builder.masterPublicDnsName;
this.configurations = builder.configurations;
this.securityConfiguration = builder.securityConfiguration;
this.autoScalingRole = builder.autoScalingRole;
this.scaleDownBehavior = builder.scaleDownBehavior;
this.customAmiId = builder.customAmiId;
this.ebsRootVolumeSize = builder.ebsRootVolumeSize;
this.repoUpgradeOnBoot = builder.repoUpgradeOnBoot;
this.kerberosAttributes = builder.kerberosAttributes;
this.clusterArn = builder.clusterArn;
this.outpostArn = builder.outpostArn;
this.stepConcurrencyLevel = builder.stepConcurrencyLevel;
this.placementGroups = builder.placementGroups;
this.osReleaseLabel = builder.osReleaseLabel;
this.ebsRootVolumeIops = builder.ebsRootVolumeIops;
this.ebsRootVolumeThroughput = builder.ebsRootVolumeThroughput;
}
/**
*
* The unique identifier for the cluster.
*
*
* @return The unique identifier for the cluster.
*/
public final String id() {
return id;
}
/**
*
* The name of the cluster. This parameter can't contain the characters <, >, $, |, or ` (backtick).
*
*
* @return The name of the cluster. This parameter can't contain the characters <, >, $, |, or ` (backtick).
*/
public final String name() {
return name;
}
/**
*
* The current status details about the cluster.
*
*
* @return The current status details about the cluster.
*/
public final ClusterStatus status() {
return status;
}
/**
*
* Provides information about the Amazon EC2 instances in a cluster grouped by category. For example, key name,
* subnet ID, IAM instance profile, and so on.
*
*
* @return Provides information about the Amazon EC2 instances in a cluster grouped by category. For example, key
* name, subnet ID, IAM instance profile, and so on.
*/
public final Ec2InstanceAttributes ec2InstanceAttributes() {
return ec2InstanceAttributes;
}
/**
*
*
* The instance fleet configuration is available only in Amazon EMR releases 4.8.0 and later, excluding 5.0.x
* versions.
*
*
*
* The instance group configuration of the cluster. A value of INSTANCE_GROUP
indicates a uniform
* instance group configuration. A value of INSTANCE_FLEET
indicates an instance fleets configuration.
*
*
* If the service returns an enum value that is not available in the current SDK version,
* {@link #instanceCollectionType} will return {@link InstanceCollectionType#UNKNOWN_TO_SDK_VERSION}. The raw value
* returned by the service is available from {@link #instanceCollectionTypeAsString}.
*
*
* @return
* The instance fleet configuration is available only in Amazon EMR releases 4.8.0 and later, excluding
* 5.0.x versions.
*
*
*
* The instance group configuration of the cluster. A value of INSTANCE_GROUP
indicates a
* uniform instance group configuration. A value of INSTANCE_FLEET
indicates an instance fleets
* configuration.
* @see InstanceCollectionType
*/
public final InstanceCollectionType instanceCollectionType() {
return InstanceCollectionType.fromValue(instanceCollectionType);
}
/**
*
*
* The instance fleet configuration is available only in Amazon EMR releases 4.8.0 and later, excluding 5.0.x
* versions.
*
*
*
* The instance group configuration of the cluster. A value of INSTANCE_GROUP
indicates a uniform
* instance group configuration. A value of INSTANCE_FLEET
indicates an instance fleets configuration.
*
*
* If the service returns an enum value that is not available in the current SDK version,
* {@link #instanceCollectionType} will return {@link InstanceCollectionType#UNKNOWN_TO_SDK_VERSION}. The raw value
* returned by the service is available from {@link #instanceCollectionTypeAsString}.
*
*
* @return
* The instance fleet configuration is available only in Amazon EMR releases 4.8.0 and later, excluding
* 5.0.x versions.
*
*
*
* The instance group configuration of the cluster. A value of INSTANCE_GROUP
indicates a
* uniform instance group configuration. A value of INSTANCE_FLEET
indicates an instance fleets
* configuration.
* @see InstanceCollectionType
*/
public final String instanceCollectionTypeAsString() {
return instanceCollectionType;
}
/**
*
* The path to the Amazon S3 location where logs for this cluster are stored.
*
*
* @return The path to the Amazon S3 location where logs for this cluster are stored.
*/
public final String logUri() {
return logUri;
}
/**
*
* The KMS key used for encrypting log files. This attribute is only available with Amazon EMR 5.30.0 and later,
* excluding Amazon EMR 6.0.0.
*
*
* @return The KMS key used for encrypting log files. This attribute is only available with Amazon EMR 5.30.0 and
* later, excluding Amazon EMR 6.0.0.
*/
public final String logEncryptionKmsKeyId() {
return logEncryptionKmsKeyId;
}
/**
*
* The AMI version requested for this cluster.
*
*
* @return The AMI version requested for this cluster.
*/
public final String requestedAmiVersion() {
return requestedAmiVersion;
}
/**
*
* The AMI version running on this cluster.
*
*
* @return The AMI version running on this cluster.
*/
public final String runningAmiVersion() {
return runningAmiVersion;
}
/**
*
* The Amazon EMR release label, which determines the version of open-source application packages installed on the
* cluster. Release labels are in the form emr-x.x.x
, where x.x.x is an Amazon EMR release version such
* as emr-5.14.0
. For more information about Amazon EMR release versions and included application
* versions and features, see https://docs.aws.amazon.
* com/emr/latest/ReleaseGuide/. The release label applies only to Amazon EMR releases version 4.0 and later.
* Earlier versions use AmiVersion
.
*
*
* @return The Amazon EMR release label, which determines the version of open-source application packages installed
* on the cluster. Release labels are in the form emr-x.x.x
, where x.x.x is an Amazon EMR
* release version such as emr-5.14.0
. For more information about Amazon EMR release versions
* and included application versions and features, see https://docs.aws.amazon.com/emr/latest/ReleaseGuide/. The release label applies only to Amazon EMR
* releases version 4.0 and later. Earlier versions use AmiVersion
.
*/
public final String releaseLabel() {
return releaseLabel;
}
/**
*
* Specifies whether the cluster should terminate after completing all steps.
*
*
* @return Specifies whether the cluster should terminate after completing all steps.
*/
public final Boolean autoTerminate() {
return autoTerminate;
}
/**
*
* Indicates whether Amazon EMR will lock the cluster to prevent the Amazon EC2 instances from being terminated by
* an API call or user intervention, or in the event of a cluster error.
*
*
* @return Indicates whether Amazon EMR will lock the cluster to prevent the Amazon EC2 instances from being
* terminated by an API call or user intervention, or in the event of a cluster error.
*/
public final Boolean terminationProtected() {
return terminationProtected;
}
/**
*
* Indicates whether Amazon EMR should gracefully replace Amazon EC2 core instances that have degraded within the
* cluster.
*
*
* @return Indicates whether Amazon EMR should gracefully replace Amazon EC2 core instances that have degraded
* within the cluster.
*/
public final Boolean unhealthyNodeReplacement() {
return unhealthyNodeReplacement;
}
/**
*
* Indicates whether the cluster is visible to IAM principals in the Amazon Web Services account associated with the
* cluster. When true
, IAM principals in the Amazon Web Services account can perform Amazon EMR cluster
* actions on the cluster that their IAM policies allow. When false
, only the IAM principal that
* created the cluster and the Amazon Web Services account root user can perform Amazon EMR actions, regardless of
* IAM permissions policies attached to other IAM principals.
*
*
* The default value is true
if a value is not provided when creating a cluster using the Amazon EMR
* API RunJobFlow command, the CLI create-cluster command, or
* the Amazon Web Services Management Console.
*
*
* @return Indicates whether the cluster is visible to IAM principals in the Amazon Web Services account associated
* with the cluster. When true
, IAM principals in the Amazon Web Services account can perform
* Amazon EMR cluster actions on the cluster that their IAM policies allow. When false
, only
* the IAM principal that created the cluster and the Amazon Web Services account root user can perform
* Amazon EMR actions, regardless of IAM permissions policies attached to other IAM principals.
*
* The default value is true
if a value is not provided when creating a cluster using the
* Amazon EMR API RunJobFlow command, the CLI create-cluster
* command, or the Amazon Web Services Management Console.
*/
public final Boolean visibleToAllUsers() {
return visibleToAllUsers;
}
/**
* For responses, this returns true if the service returned a value for the Applications property. This DOES NOT
* check that the value is non-empty (for which, you should check the {@code isEmpty()} method on the property).
* This is useful because the SDK will never return a null collection or map, but you may need to differentiate
* between the service returning nothing (or null) and the service returning an empty collection or map. For
* requests, this returns true if a value for the property was specified in the request builder, and false if a
* value was not specified.
*/
public final boolean hasApplications() {
return applications != null && !(applications instanceof SdkAutoConstructList);
}
/**
*
* The applications installed on this cluster.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasApplications} method.
*
*
* @return The applications installed on this cluster.
*/
public final List applications() {
return applications;
}
/**
* For responses, this returns true if the service returned a value for the Tags property. This DOES NOT check that
* the value is non-empty (for which, you should check the {@code isEmpty()} method on the property). This is useful
* because the SDK will never return a null collection or map, but you may need to differentiate between the service
* returning nothing (or null) and the service returning an empty collection or map. For requests, this returns true
* if a value for the property was specified in the request builder, and false if a value was not specified.
*/
public final boolean hasTags() {
return tags != null && !(tags instanceof SdkAutoConstructList);
}
/**
*
* A list of tags associated with a cluster.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasTags} method.
*
*
* @return A list of tags associated with a cluster.
*/
public final List tags() {
return tags;
}
/**
*
* The IAM role that Amazon EMR assumes in order to access Amazon Web Services resources on your behalf.
*
*
* @return The IAM role that Amazon EMR assumes in order to access Amazon Web Services resources on your behalf.
*/
public final String serviceRole() {
return serviceRole;
}
/**
*
* An approximation of the cost of the cluster, represented in m1.small/hours. This value is incremented one time
* for every hour an m1.small instance runs. Larger instances are weighted more, so an Amazon EC2 instance that is
* roughly four times more expensive would result in the normalized instance hours being incremented by four. This
* result is only an approximation and does not reflect the actual billing rate.
*
*
* @return An approximation of the cost of the cluster, represented in m1.small/hours. This value is incremented one
* time for every hour an m1.small instance runs. Larger instances are weighted more, so an Amazon EC2
* instance that is roughly four times more expensive would result in the normalized instance hours being
* incremented by four. This result is only an approximation and does not reflect the actual billing rate.
*/
public final Integer normalizedInstanceHours() {
return normalizedInstanceHours;
}
/**
*
* The DNS name of the master node. If the cluster is on a private subnet, this is the private DNS name. On a public
* subnet, this is the public DNS name.
*
*
* @return The DNS name of the master node. If the cluster is on a private subnet, this is the private DNS name. On
* a public subnet, this is the public DNS name.
*/
public final String masterPublicDnsName() {
return masterPublicDnsName;
}
/**
* For responses, this returns true if the service returned a value for the Configurations property. This DOES NOT
* check that the value is non-empty (for which, you should check the {@code isEmpty()} method on the property).
* This is useful because the SDK will never return a null collection or map, but you may need to differentiate
* between the service returning nothing (or null) and the service returning an empty collection or map. For
* requests, this returns true if a value for the property was specified in the request builder, and false if a
* value was not specified.
*/
public final boolean hasConfigurations() {
return configurations != null && !(configurations instanceof SdkAutoConstructList);
}
/**
*
* Applies only to Amazon EMR releases 4.x and later. The list of configurations that are supplied to the Amazon EMR
* cluster.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasConfigurations} method.
*
*
* @return Applies only to Amazon EMR releases 4.x and later. The list of configurations that are supplied to the
* Amazon EMR cluster.
*/
public final List configurations() {
return configurations;
}
/**
*
* The name of the security configuration applied to the cluster.
*
*
* @return The name of the security configuration applied to the cluster.
*/
public final String securityConfiguration() {
return securityConfiguration;
}
/**
*
* An IAM role for automatic scaling policies. The default role is EMR_AutoScaling_DefaultRole
. The IAM
* role provides permissions that the automatic scaling feature requires to launch and terminate Amazon EC2
* instances in an instance group.
*
*
* @return An IAM role for automatic scaling policies. The default role is EMR_AutoScaling_DefaultRole
.
* The IAM role provides permissions that the automatic scaling feature requires to launch and terminate
* Amazon EC2 instances in an instance group.
*/
public final String autoScalingRole() {
return autoScalingRole;
}
/**
*
* The way that individual Amazon EC2 instances terminate when an automatic scale-in activity occurs or an instance
* group is resized. TERMINATE_AT_INSTANCE_HOUR
indicates that Amazon EMR terminates nodes at the
* instance-hour boundary, regardless of when the request to terminate the instance was submitted. This option is
* only available with Amazon EMR 5.1.0 and later and is the default for clusters created using that version.
* TERMINATE_AT_TASK_COMPLETION
indicates that Amazon EMR adds nodes to a deny list and drains tasks
* from nodes before terminating the Amazon EC2 instances, regardless of the instance-hour boundary. With either
* behavior, Amazon EMR removes the least active nodes first and blocks instance termination if it could lead to
* HDFS corruption. TERMINATE_AT_TASK_COMPLETION
is available only in Amazon EMR releases 4.1.0 and
* later, and is the default for versions of Amazon EMR earlier than 5.1.0.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #scaleDownBehavior}
* will return {@link ScaleDownBehavior#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available
* from {@link #scaleDownBehaviorAsString}.
*
*
* @return The way that individual Amazon EC2 instances terminate when an automatic scale-in activity occurs or an
* instance group is resized. TERMINATE_AT_INSTANCE_HOUR
indicates that Amazon EMR terminates
* nodes at the instance-hour boundary, regardless of when the request to terminate the instance was
* submitted. This option is only available with Amazon EMR 5.1.0 and later and is the default for clusters
* created using that version. TERMINATE_AT_TASK_COMPLETION
indicates that Amazon EMR adds
* nodes to a deny list and drains tasks from nodes before terminating the Amazon EC2 instances, regardless
* of the instance-hour boundary. With either behavior, Amazon EMR removes the least active nodes first and
* blocks instance termination if it could lead to HDFS corruption.
* TERMINATE_AT_TASK_COMPLETION
is available only in Amazon EMR releases 4.1.0 and later, and
* is the default for versions of Amazon EMR earlier than 5.1.0.
* @see ScaleDownBehavior
*/
public final ScaleDownBehavior scaleDownBehavior() {
return ScaleDownBehavior.fromValue(scaleDownBehavior);
}
/**
*
* The way that individual Amazon EC2 instances terminate when an automatic scale-in activity occurs or an instance
* group is resized. TERMINATE_AT_INSTANCE_HOUR
indicates that Amazon EMR terminates nodes at the
* instance-hour boundary, regardless of when the request to terminate the instance was submitted. This option is
* only available with Amazon EMR 5.1.0 and later and is the default for clusters created using that version.
* TERMINATE_AT_TASK_COMPLETION
indicates that Amazon EMR adds nodes to a deny list and drains tasks
* from nodes before terminating the Amazon EC2 instances, regardless of the instance-hour boundary. With either
* behavior, Amazon EMR removes the least active nodes first and blocks instance termination if it could lead to
* HDFS corruption. TERMINATE_AT_TASK_COMPLETION
is available only in Amazon EMR releases 4.1.0 and
* later, and is the default for versions of Amazon EMR earlier than 5.1.0.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #scaleDownBehavior}
* will return {@link ScaleDownBehavior#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available
* from {@link #scaleDownBehaviorAsString}.
*
*
* @return The way that individual Amazon EC2 instances terminate when an automatic scale-in activity occurs or an
* instance group is resized. TERMINATE_AT_INSTANCE_HOUR
indicates that Amazon EMR terminates
* nodes at the instance-hour boundary, regardless of when the request to terminate the instance was
* submitted. This option is only available with Amazon EMR 5.1.0 and later and is the default for clusters
* created using that version. TERMINATE_AT_TASK_COMPLETION
indicates that Amazon EMR adds
* nodes to a deny list and drains tasks from nodes before terminating the Amazon EC2 instances, regardless
* of the instance-hour boundary. With either behavior, Amazon EMR removes the least active nodes first and
* blocks instance termination if it could lead to HDFS corruption.
* TERMINATE_AT_TASK_COMPLETION
is available only in Amazon EMR releases 4.1.0 and later, and
* is the default for versions of Amazon EMR earlier than 5.1.0.
* @see ScaleDownBehavior
*/
public final String scaleDownBehaviorAsString() {
return scaleDownBehavior;
}
/**
*
* Available only in Amazon EMR releases 5.7.0 and later. The ID of a custom Amazon EBS-backed Linux AMI if the
* cluster uses a custom AMI.
*
*
* @return Available only in Amazon EMR releases 5.7.0 and later. The ID of a custom Amazon EBS-backed Linux AMI if
* the cluster uses a custom AMI.
*/
public final String customAmiId() {
return customAmiId;
}
/**
*
* The size, in GiB, of the Amazon EBS root device volume of the Linux AMI that is used for each Amazon EC2
* instance. Available in Amazon EMR releases 4.x and later.
*
*
* @return The size, in GiB, of the Amazon EBS root device volume of the Linux AMI that is used for each Amazon EC2
* instance. Available in Amazon EMR releases 4.x and later.
*/
public final Integer ebsRootVolumeSize() {
return ebsRootVolumeSize;
}
/**
*
* Applies only when CustomAmiID
is used. Specifies the type of updates that the Amazon Linux AMI
* package repositories apply when an instance boots using the AMI.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #repoUpgradeOnBoot}
* will return {@link RepoUpgradeOnBoot#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available
* from {@link #repoUpgradeOnBootAsString}.
*
*
* @return Applies only when CustomAmiID
is used. Specifies the type of updates that the Amazon Linux
* AMI package repositories apply when an instance boots using the AMI.
* @see RepoUpgradeOnBoot
*/
public final RepoUpgradeOnBoot repoUpgradeOnBoot() {
return RepoUpgradeOnBoot.fromValue(repoUpgradeOnBoot);
}
/**
*
* Applies only when CustomAmiID
is used. Specifies the type of updates that the Amazon Linux AMI
* package repositories apply when an instance boots using the AMI.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #repoUpgradeOnBoot}
* will return {@link RepoUpgradeOnBoot#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available
* from {@link #repoUpgradeOnBootAsString}.
*
*
* @return Applies only when CustomAmiID
is used. Specifies the type of updates that the Amazon Linux
* AMI package repositories apply when an instance boots using the AMI.
* @see RepoUpgradeOnBoot
*/
public final String repoUpgradeOnBootAsString() {
return repoUpgradeOnBoot;
}
/**
*
* Attributes for Kerberos configuration when Kerberos authentication is enabled using a security configuration. For
* more information see Use
* Kerberos Authentication in the Amazon EMR Management Guide.
*
*
* @return Attributes for Kerberos configuration when Kerberos authentication is enabled using a security
* configuration. For more information see Use Kerberos
* Authentication in the Amazon EMR Management Guide.
*/
public final KerberosAttributes kerberosAttributes() {
return kerberosAttributes;
}
/**
*
* The Amazon Resource Name of the cluster.
*
*
* @return The Amazon Resource Name of the cluster.
*/
public final String clusterArn() {
return clusterArn;
}
/**
*
* The Amazon Resource Name (ARN) of the Outpost where the cluster is launched.
*
*
* @return The Amazon Resource Name (ARN) of the Outpost where the cluster is launched.
*/
public final String outpostArn() {
return outpostArn;
}
/**
*
* Specifies the number of steps that can be executed concurrently.
*
*
* @return Specifies the number of steps that can be executed concurrently.
*/
public final Integer stepConcurrencyLevel() {
return stepConcurrencyLevel;
}
/**
* For responses, this returns true if the service returned a value for the PlacementGroups property. This DOES NOT
* check that the value is non-empty (for which, you should check the {@code isEmpty()} method on the property).
* This is useful because the SDK will never return a null collection or map, but you may need to differentiate
* between the service returning nothing (or null) and the service returning an empty collection or map. For
* requests, this returns true if a value for the property was specified in the request builder, and false if a
* value was not specified.
*/
public final boolean hasPlacementGroups() {
return placementGroups != null && !(placementGroups instanceof SdkAutoConstructList);
}
/**
*
* Placement group configured for an Amazon EMR cluster.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasPlacementGroups} method.
*
*
* @return Placement group configured for an Amazon EMR cluster.
*/
public final List placementGroups() {
return placementGroups;
}
/**
*
* The Amazon Linux release specified in a cluster launch RunJobFlow request. If no Amazon Linux release was
* specified, the default Amazon Linux release is shown in the response.
*
*
* @return The Amazon Linux release specified in a cluster launch RunJobFlow request. If no Amazon Linux release was
* specified, the default Amazon Linux release is shown in the response.
*/
public final String osReleaseLabel() {
return osReleaseLabel;
}
/**
*
* The IOPS, of the Amazon EBS root device volume of the Linux AMI that is used for each Amazon EC2 instance.
* Available in Amazon EMR releases 6.15.0 and later.
*
*
* @return The IOPS, of the Amazon EBS root device volume of the Linux AMI that is used for each Amazon EC2
* instance. Available in Amazon EMR releases 6.15.0 and later.
*/
public final Integer ebsRootVolumeIops() {
return ebsRootVolumeIops;
}
/**
*
* The throughput, in MiB/s, of the Amazon EBS root device volume of the Linux AMI that is used for each Amazon EC2
* instance. Available in Amazon EMR releases 6.15.0 and later.
*
*
* @return The throughput, in MiB/s, of the Amazon EBS root device volume of the Linux AMI that is used for each
* Amazon EC2 instance. Available in Amazon EMR releases 6.15.0 and later.
*/
public final Integer ebsRootVolumeThroughput() {
return ebsRootVolumeThroughput;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(id());
hashCode = 31 * hashCode + Objects.hashCode(name());
hashCode = 31 * hashCode + Objects.hashCode(status());
hashCode = 31 * hashCode + Objects.hashCode(ec2InstanceAttributes());
hashCode = 31 * hashCode + Objects.hashCode(instanceCollectionTypeAsString());
hashCode = 31 * hashCode + Objects.hashCode(logUri());
hashCode = 31 * hashCode + Objects.hashCode(logEncryptionKmsKeyId());
hashCode = 31 * hashCode + Objects.hashCode(requestedAmiVersion());
hashCode = 31 * hashCode + Objects.hashCode(runningAmiVersion());
hashCode = 31 * hashCode + Objects.hashCode(releaseLabel());
hashCode = 31 * hashCode + Objects.hashCode(autoTerminate());
hashCode = 31 * hashCode + Objects.hashCode(terminationProtected());
hashCode = 31 * hashCode + Objects.hashCode(unhealthyNodeReplacement());
hashCode = 31 * hashCode + Objects.hashCode(visibleToAllUsers());
hashCode = 31 * hashCode + Objects.hashCode(hasApplications() ? applications() : null);
hashCode = 31 * hashCode + Objects.hashCode(hasTags() ? tags() : null);
hashCode = 31 * hashCode + Objects.hashCode(serviceRole());
hashCode = 31 * hashCode + Objects.hashCode(normalizedInstanceHours());
hashCode = 31 * hashCode + Objects.hashCode(masterPublicDnsName());
hashCode = 31 * hashCode + Objects.hashCode(hasConfigurations() ? configurations() : null);
hashCode = 31 * hashCode + Objects.hashCode(securityConfiguration());
hashCode = 31 * hashCode + Objects.hashCode(autoScalingRole());
hashCode = 31 * hashCode + Objects.hashCode(scaleDownBehaviorAsString());
hashCode = 31 * hashCode + Objects.hashCode(customAmiId());
hashCode = 31 * hashCode + Objects.hashCode(ebsRootVolumeSize());
hashCode = 31 * hashCode + Objects.hashCode(repoUpgradeOnBootAsString());
hashCode = 31 * hashCode + Objects.hashCode(kerberosAttributes());
hashCode = 31 * hashCode + Objects.hashCode(clusterArn());
hashCode = 31 * hashCode + Objects.hashCode(outpostArn());
hashCode = 31 * hashCode + Objects.hashCode(stepConcurrencyLevel());
hashCode = 31 * hashCode + Objects.hashCode(hasPlacementGroups() ? placementGroups() : null);
hashCode = 31 * hashCode + Objects.hashCode(osReleaseLabel());
hashCode = 31 * hashCode + Objects.hashCode(ebsRootVolumeIops());
hashCode = 31 * hashCode + Objects.hashCode(ebsRootVolumeThroughput());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof Cluster)) {
return false;
}
Cluster other = (Cluster) obj;
return Objects.equals(id(), other.id()) && Objects.equals(name(), other.name())
&& Objects.equals(status(), other.status())
&& Objects.equals(ec2InstanceAttributes(), other.ec2InstanceAttributes())
&& Objects.equals(instanceCollectionTypeAsString(), other.instanceCollectionTypeAsString())
&& Objects.equals(logUri(), other.logUri())
&& Objects.equals(logEncryptionKmsKeyId(), other.logEncryptionKmsKeyId())
&& Objects.equals(requestedAmiVersion(), other.requestedAmiVersion())
&& Objects.equals(runningAmiVersion(), other.runningAmiVersion())
&& Objects.equals(releaseLabel(), other.releaseLabel()) && Objects.equals(autoTerminate(), other.autoTerminate())
&& Objects.equals(terminationProtected(), other.terminationProtected())
&& Objects.equals(unhealthyNodeReplacement(), other.unhealthyNodeReplacement())
&& Objects.equals(visibleToAllUsers(), other.visibleToAllUsers()) && hasApplications() == other.hasApplications()
&& Objects.equals(applications(), other.applications()) && hasTags() == other.hasTags()
&& Objects.equals(tags(), other.tags()) && Objects.equals(serviceRole(), other.serviceRole())
&& Objects.equals(normalizedInstanceHours(), other.normalizedInstanceHours())
&& Objects.equals(masterPublicDnsName(), other.masterPublicDnsName())
&& hasConfigurations() == other.hasConfigurations() && Objects.equals(configurations(), other.configurations())
&& Objects.equals(securityConfiguration(), other.securityConfiguration())
&& Objects.equals(autoScalingRole(), other.autoScalingRole())
&& Objects.equals(scaleDownBehaviorAsString(), other.scaleDownBehaviorAsString())
&& Objects.equals(customAmiId(), other.customAmiId())
&& Objects.equals(ebsRootVolumeSize(), other.ebsRootVolumeSize())
&& Objects.equals(repoUpgradeOnBootAsString(), other.repoUpgradeOnBootAsString())
&& Objects.equals(kerberosAttributes(), other.kerberosAttributes())
&& Objects.equals(clusterArn(), other.clusterArn()) && Objects.equals(outpostArn(), other.outpostArn())
&& Objects.equals(stepConcurrencyLevel(), other.stepConcurrencyLevel())
&& hasPlacementGroups() == other.hasPlacementGroups()
&& Objects.equals(placementGroups(), other.placementGroups())
&& Objects.equals(osReleaseLabel(), other.osReleaseLabel())
&& Objects.equals(ebsRootVolumeIops(), other.ebsRootVolumeIops())
&& Objects.equals(ebsRootVolumeThroughput(), other.ebsRootVolumeThroughput());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("Cluster").add("Id", id()).add("Name", name()).add("Status", status())
.add("Ec2InstanceAttributes", ec2InstanceAttributes())
.add("InstanceCollectionType", instanceCollectionTypeAsString()).add("LogUri", logUri())
.add("LogEncryptionKmsKeyId", logEncryptionKmsKeyId()).add("RequestedAmiVersion", requestedAmiVersion())
.add("RunningAmiVersion", runningAmiVersion()).add("ReleaseLabel", releaseLabel())
.add("AutoTerminate", autoTerminate()).add("TerminationProtected", terminationProtected())
.add("UnhealthyNodeReplacement", unhealthyNodeReplacement()).add("VisibleToAllUsers", visibleToAllUsers())
.add("Applications", hasApplications() ? applications() : null).add("Tags", hasTags() ? tags() : null)
.add("ServiceRole", serviceRole()).add("NormalizedInstanceHours", normalizedInstanceHours())
.add("MasterPublicDnsName", masterPublicDnsName())
.add("Configurations", hasConfigurations() ? configurations() : null)
.add("SecurityConfiguration", securityConfiguration()).add("AutoScalingRole", autoScalingRole())
.add("ScaleDownBehavior", scaleDownBehaviorAsString()).add("CustomAmiId", customAmiId())
.add("EbsRootVolumeSize", ebsRootVolumeSize()).add("RepoUpgradeOnBoot", repoUpgradeOnBootAsString())
.add("KerberosAttributes", kerberosAttributes()).add("ClusterArn", clusterArn()).add("OutpostArn", outpostArn())
.add("StepConcurrencyLevel", stepConcurrencyLevel())
.add("PlacementGroups", hasPlacementGroups() ? placementGroups() : null).add("OSReleaseLabel", osReleaseLabel())
.add("EbsRootVolumeIops", ebsRootVolumeIops()).add("EbsRootVolumeThroughput", ebsRootVolumeThroughput()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "Id":
return Optional.ofNullable(clazz.cast(id()));
case "Name":
return Optional.ofNullable(clazz.cast(name()));
case "Status":
return Optional.ofNullable(clazz.cast(status()));
case "Ec2InstanceAttributes":
return Optional.ofNullable(clazz.cast(ec2InstanceAttributes()));
case "InstanceCollectionType":
return Optional.ofNullable(clazz.cast(instanceCollectionTypeAsString()));
case "LogUri":
return Optional.ofNullable(clazz.cast(logUri()));
case "LogEncryptionKmsKeyId":
return Optional.ofNullable(clazz.cast(logEncryptionKmsKeyId()));
case "RequestedAmiVersion":
return Optional.ofNullable(clazz.cast(requestedAmiVersion()));
case "RunningAmiVersion":
return Optional.ofNullable(clazz.cast(runningAmiVersion()));
case "ReleaseLabel":
return Optional.ofNullable(clazz.cast(releaseLabel()));
case "AutoTerminate":
return Optional.ofNullable(clazz.cast(autoTerminate()));
case "TerminationProtected":
return Optional.ofNullable(clazz.cast(terminationProtected()));
case "UnhealthyNodeReplacement":
return Optional.ofNullable(clazz.cast(unhealthyNodeReplacement()));
case "VisibleToAllUsers":
return Optional.ofNullable(clazz.cast(visibleToAllUsers()));
case "Applications":
return Optional.ofNullable(clazz.cast(applications()));
case "Tags":
return Optional.ofNullable(clazz.cast(tags()));
case "ServiceRole":
return Optional.ofNullable(clazz.cast(serviceRole()));
case "NormalizedInstanceHours":
return Optional.ofNullable(clazz.cast(normalizedInstanceHours()));
case "MasterPublicDnsName":
return Optional.ofNullable(clazz.cast(masterPublicDnsName()));
case "Configurations":
return Optional.ofNullable(clazz.cast(configurations()));
case "SecurityConfiguration":
return Optional.ofNullable(clazz.cast(securityConfiguration()));
case "AutoScalingRole":
return Optional.ofNullable(clazz.cast(autoScalingRole()));
case "ScaleDownBehavior":
return Optional.ofNullable(clazz.cast(scaleDownBehaviorAsString()));
case "CustomAmiId":
return Optional.ofNullable(clazz.cast(customAmiId()));
case "EbsRootVolumeSize":
return Optional.ofNullable(clazz.cast(ebsRootVolumeSize()));
case "RepoUpgradeOnBoot":
return Optional.ofNullable(clazz.cast(repoUpgradeOnBootAsString()));
case "KerberosAttributes":
return Optional.ofNullable(clazz.cast(kerberosAttributes()));
case "ClusterArn":
return Optional.ofNullable(clazz.cast(clusterArn()));
case "OutpostArn":
return Optional.ofNullable(clazz.cast(outpostArn()));
case "StepConcurrencyLevel":
return Optional.ofNullable(clazz.cast(stepConcurrencyLevel()));
case "PlacementGroups":
return Optional.ofNullable(clazz.cast(placementGroups()));
case "OSReleaseLabel":
return Optional.ofNullable(clazz.cast(osReleaseLabel()));
case "EbsRootVolumeIops":
return Optional.ofNullable(clazz.cast(ebsRootVolumeIops()));
case "EbsRootVolumeThroughput":
return Optional.ofNullable(clazz.cast(ebsRootVolumeThroughput()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
private static Function