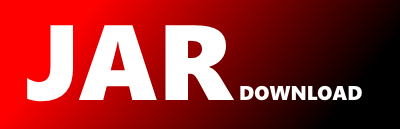
software.amazon.awssdk.services.emr.DefaultEmrClient Maven / Gradle / Ivy
Show all versions of emr Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.emr;
import java.util.Collections;
import java.util.List;
import java.util.function.Consumer;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.annotations.SdkInternalApi;
import software.amazon.awssdk.awscore.client.handler.AwsSyncClientHandler;
import software.amazon.awssdk.awscore.exception.AwsServiceException;
import software.amazon.awssdk.awscore.internal.AwsProtocolMetadata;
import software.amazon.awssdk.awscore.internal.AwsServiceProtocol;
import software.amazon.awssdk.awscore.retry.AwsRetryStrategy;
import software.amazon.awssdk.core.RequestOverrideConfiguration;
import software.amazon.awssdk.core.SdkPlugin;
import software.amazon.awssdk.core.SdkRequest;
import software.amazon.awssdk.core.client.config.ClientOverrideConfiguration;
import software.amazon.awssdk.core.client.config.SdkClientConfiguration;
import software.amazon.awssdk.core.client.config.SdkClientOption;
import software.amazon.awssdk.core.client.handler.ClientExecutionParams;
import software.amazon.awssdk.core.client.handler.SyncClientHandler;
import software.amazon.awssdk.core.exception.SdkClientException;
import software.amazon.awssdk.core.http.HttpResponseHandler;
import software.amazon.awssdk.core.metrics.CoreMetric;
import software.amazon.awssdk.core.retry.RetryMode;
import software.amazon.awssdk.metrics.MetricCollector;
import software.amazon.awssdk.metrics.MetricPublisher;
import software.amazon.awssdk.metrics.NoOpMetricCollector;
import software.amazon.awssdk.protocols.core.ExceptionMetadata;
import software.amazon.awssdk.protocols.json.AwsJsonProtocol;
import software.amazon.awssdk.protocols.json.AwsJsonProtocolFactory;
import software.amazon.awssdk.protocols.json.BaseAwsJsonProtocolFactory;
import software.amazon.awssdk.protocols.json.JsonOperationMetadata;
import software.amazon.awssdk.retries.api.RetryStrategy;
import software.amazon.awssdk.services.emr.internal.EmrServiceClientConfigurationBuilder;
import software.amazon.awssdk.services.emr.model.AddInstanceFleetRequest;
import software.amazon.awssdk.services.emr.model.AddInstanceFleetResponse;
import software.amazon.awssdk.services.emr.model.AddInstanceGroupsRequest;
import software.amazon.awssdk.services.emr.model.AddInstanceGroupsResponse;
import software.amazon.awssdk.services.emr.model.AddJobFlowStepsRequest;
import software.amazon.awssdk.services.emr.model.AddJobFlowStepsResponse;
import software.amazon.awssdk.services.emr.model.AddTagsRequest;
import software.amazon.awssdk.services.emr.model.AddTagsResponse;
import software.amazon.awssdk.services.emr.model.CancelStepsRequest;
import software.amazon.awssdk.services.emr.model.CancelStepsResponse;
import software.amazon.awssdk.services.emr.model.CreateSecurityConfigurationRequest;
import software.amazon.awssdk.services.emr.model.CreateSecurityConfigurationResponse;
import software.amazon.awssdk.services.emr.model.CreateStudioRequest;
import software.amazon.awssdk.services.emr.model.CreateStudioResponse;
import software.amazon.awssdk.services.emr.model.CreateStudioSessionMappingRequest;
import software.amazon.awssdk.services.emr.model.CreateStudioSessionMappingResponse;
import software.amazon.awssdk.services.emr.model.DeleteSecurityConfigurationRequest;
import software.amazon.awssdk.services.emr.model.DeleteSecurityConfigurationResponse;
import software.amazon.awssdk.services.emr.model.DeleteStudioRequest;
import software.amazon.awssdk.services.emr.model.DeleteStudioResponse;
import software.amazon.awssdk.services.emr.model.DeleteStudioSessionMappingRequest;
import software.amazon.awssdk.services.emr.model.DeleteStudioSessionMappingResponse;
import software.amazon.awssdk.services.emr.model.DescribeClusterRequest;
import software.amazon.awssdk.services.emr.model.DescribeClusterResponse;
import software.amazon.awssdk.services.emr.model.DescribeNotebookExecutionRequest;
import software.amazon.awssdk.services.emr.model.DescribeNotebookExecutionResponse;
import software.amazon.awssdk.services.emr.model.DescribeReleaseLabelRequest;
import software.amazon.awssdk.services.emr.model.DescribeReleaseLabelResponse;
import software.amazon.awssdk.services.emr.model.DescribeSecurityConfigurationRequest;
import software.amazon.awssdk.services.emr.model.DescribeSecurityConfigurationResponse;
import software.amazon.awssdk.services.emr.model.DescribeStepRequest;
import software.amazon.awssdk.services.emr.model.DescribeStepResponse;
import software.amazon.awssdk.services.emr.model.DescribeStudioRequest;
import software.amazon.awssdk.services.emr.model.DescribeStudioResponse;
import software.amazon.awssdk.services.emr.model.EmrException;
import software.amazon.awssdk.services.emr.model.GetAutoTerminationPolicyRequest;
import software.amazon.awssdk.services.emr.model.GetAutoTerminationPolicyResponse;
import software.amazon.awssdk.services.emr.model.GetBlockPublicAccessConfigurationRequest;
import software.amazon.awssdk.services.emr.model.GetBlockPublicAccessConfigurationResponse;
import software.amazon.awssdk.services.emr.model.GetClusterSessionCredentialsRequest;
import software.amazon.awssdk.services.emr.model.GetClusterSessionCredentialsResponse;
import software.amazon.awssdk.services.emr.model.GetManagedScalingPolicyRequest;
import software.amazon.awssdk.services.emr.model.GetManagedScalingPolicyResponse;
import software.amazon.awssdk.services.emr.model.GetStudioSessionMappingRequest;
import software.amazon.awssdk.services.emr.model.GetStudioSessionMappingResponse;
import software.amazon.awssdk.services.emr.model.InternalServerErrorException;
import software.amazon.awssdk.services.emr.model.InternalServerException;
import software.amazon.awssdk.services.emr.model.InvalidRequestException;
import software.amazon.awssdk.services.emr.model.ListBootstrapActionsRequest;
import software.amazon.awssdk.services.emr.model.ListBootstrapActionsResponse;
import software.amazon.awssdk.services.emr.model.ListClustersRequest;
import software.amazon.awssdk.services.emr.model.ListClustersResponse;
import software.amazon.awssdk.services.emr.model.ListInstanceFleetsRequest;
import software.amazon.awssdk.services.emr.model.ListInstanceFleetsResponse;
import software.amazon.awssdk.services.emr.model.ListInstanceGroupsRequest;
import software.amazon.awssdk.services.emr.model.ListInstanceGroupsResponse;
import software.amazon.awssdk.services.emr.model.ListInstancesRequest;
import software.amazon.awssdk.services.emr.model.ListInstancesResponse;
import software.amazon.awssdk.services.emr.model.ListNotebookExecutionsRequest;
import software.amazon.awssdk.services.emr.model.ListNotebookExecutionsResponse;
import software.amazon.awssdk.services.emr.model.ListReleaseLabelsRequest;
import software.amazon.awssdk.services.emr.model.ListReleaseLabelsResponse;
import software.amazon.awssdk.services.emr.model.ListSecurityConfigurationsRequest;
import software.amazon.awssdk.services.emr.model.ListSecurityConfigurationsResponse;
import software.amazon.awssdk.services.emr.model.ListStepsRequest;
import software.amazon.awssdk.services.emr.model.ListStepsResponse;
import software.amazon.awssdk.services.emr.model.ListStudioSessionMappingsRequest;
import software.amazon.awssdk.services.emr.model.ListStudioSessionMappingsResponse;
import software.amazon.awssdk.services.emr.model.ListStudiosRequest;
import software.amazon.awssdk.services.emr.model.ListStudiosResponse;
import software.amazon.awssdk.services.emr.model.ListSupportedInstanceTypesRequest;
import software.amazon.awssdk.services.emr.model.ListSupportedInstanceTypesResponse;
import software.amazon.awssdk.services.emr.model.ModifyClusterRequest;
import software.amazon.awssdk.services.emr.model.ModifyClusterResponse;
import software.amazon.awssdk.services.emr.model.ModifyInstanceFleetRequest;
import software.amazon.awssdk.services.emr.model.ModifyInstanceFleetResponse;
import software.amazon.awssdk.services.emr.model.ModifyInstanceGroupsRequest;
import software.amazon.awssdk.services.emr.model.ModifyInstanceGroupsResponse;
import software.amazon.awssdk.services.emr.model.PutAutoScalingPolicyRequest;
import software.amazon.awssdk.services.emr.model.PutAutoScalingPolicyResponse;
import software.amazon.awssdk.services.emr.model.PutAutoTerminationPolicyRequest;
import software.amazon.awssdk.services.emr.model.PutAutoTerminationPolicyResponse;
import software.amazon.awssdk.services.emr.model.PutBlockPublicAccessConfigurationRequest;
import software.amazon.awssdk.services.emr.model.PutBlockPublicAccessConfigurationResponse;
import software.amazon.awssdk.services.emr.model.PutManagedScalingPolicyRequest;
import software.amazon.awssdk.services.emr.model.PutManagedScalingPolicyResponse;
import software.amazon.awssdk.services.emr.model.RemoveAutoScalingPolicyRequest;
import software.amazon.awssdk.services.emr.model.RemoveAutoScalingPolicyResponse;
import software.amazon.awssdk.services.emr.model.RemoveAutoTerminationPolicyRequest;
import software.amazon.awssdk.services.emr.model.RemoveAutoTerminationPolicyResponse;
import software.amazon.awssdk.services.emr.model.RemoveManagedScalingPolicyRequest;
import software.amazon.awssdk.services.emr.model.RemoveManagedScalingPolicyResponse;
import software.amazon.awssdk.services.emr.model.RemoveTagsRequest;
import software.amazon.awssdk.services.emr.model.RemoveTagsResponse;
import software.amazon.awssdk.services.emr.model.RunJobFlowRequest;
import software.amazon.awssdk.services.emr.model.RunJobFlowResponse;
import software.amazon.awssdk.services.emr.model.SetKeepJobFlowAliveWhenNoStepsRequest;
import software.amazon.awssdk.services.emr.model.SetKeepJobFlowAliveWhenNoStepsResponse;
import software.amazon.awssdk.services.emr.model.SetTerminationProtectionRequest;
import software.amazon.awssdk.services.emr.model.SetTerminationProtectionResponse;
import software.amazon.awssdk.services.emr.model.SetUnhealthyNodeReplacementRequest;
import software.amazon.awssdk.services.emr.model.SetUnhealthyNodeReplacementResponse;
import software.amazon.awssdk.services.emr.model.SetVisibleToAllUsersRequest;
import software.amazon.awssdk.services.emr.model.SetVisibleToAllUsersResponse;
import software.amazon.awssdk.services.emr.model.StartNotebookExecutionRequest;
import software.amazon.awssdk.services.emr.model.StartNotebookExecutionResponse;
import software.amazon.awssdk.services.emr.model.StopNotebookExecutionRequest;
import software.amazon.awssdk.services.emr.model.StopNotebookExecutionResponse;
import software.amazon.awssdk.services.emr.model.TerminateJobFlowsRequest;
import software.amazon.awssdk.services.emr.model.TerminateJobFlowsResponse;
import software.amazon.awssdk.services.emr.model.UpdateStudioRequest;
import software.amazon.awssdk.services.emr.model.UpdateStudioResponse;
import software.amazon.awssdk.services.emr.model.UpdateStudioSessionMappingRequest;
import software.amazon.awssdk.services.emr.model.UpdateStudioSessionMappingResponse;
import software.amazon.awssdk.services.emr.transform.AddInstanceFleetRequestMarshaller;
import software.amazon.awssdk.services.emr.transform.AddInstanceGroupsRequestMarshaller;
import software.amazon.awssdk.services.emr.transform.AddJobFlowStepsRequestMarshaller;
import software.amazon.awssdk.services.emr.transform.AddTagsRequestMarshaller;
import software.amazon.awssdk.services.emr.transform.CancelStepsRequestMarshaller;
import software.amazon.awssdk.services.emr.transform.CreateSecurityConfigurationRequestMarshaller;
import software.amazon.awssdk.services.emr.transform.CreateStudioRequestMarshaller;
import software.amazon.awssdk.services.emr.transform.CreateStudioSessionMappingRequestMarshaller;
import software.amazon.awssdk.services.emr.transform.DeleteSecurityConfigurationRequestMarshaller;
import software.amazon.awssdk.services.emr.transform.DeleteStudioRequestMarshaller;
import software.amazon.awssdk.services.emr.transform.DeleteStudioSessionMappingRequestMarshaller;
import software.amazon.awssdk.services.emr.transform.DescribeClusterRequestMarshaller;
import software.amazon.awssdk.services.emr.transform.DescribeNotebookExecutionRequestMarshaller;
import software.amazon.awssdk.services.emr.transform.DescribeReleaseLabelRequestMarshaller;
import software.amazon.awssdk.services.emr.transform.DescribeSecurityConfigurationRequestMarshaller;
import software.amazon.awssdk.services.emr.transform.DescribeStepRequestMarshaller;
import software.amazon.awssdk.services.emr.transform.DescribeStudioRequestMarshaller;
import software.amazon.awssdk.services.emr.transform.GetAutoTerminationPolicyRequestMarshaller;
import software.amazon.awssdk.services.emr.transform.GetBlockPublicAccessConfigurationRequestMarshaller;
import software.amazon.awssdk.services.emr.transform.GetClusterSessionCredentialsRequestMarshaller;
import software.amazon.awssdk.services.emr.transform.GetManagedScalingPolicyRequestMarshaller;
import software.amazon.awssdk.services.emr.transform.GetStudioSessionMappingRequestMarshaller;
import software.amazon.awssdk.services.emr.transform.ListBootstrapActionsRequestMarshaller;
import software.amazon.awssdk.services.emr.transform.ListClustersRequestMarshaller;
import software.amazon.awssdk.services.emr.transform.ListInstanceFleetsRequestMarshaller;
import software.amazon.awssdk.services.emr.transform.ListInstanceGroupsRequestMarshaller;
import software.amazon.awssdk.services.emr.transform.ListInstancesRequestMarshaller;
import software.amazon.awssdk.services.emr.transform.ListNotebookExecutionsRequestMarshaller;
import software.amazon.awssdk.services.emr.transform.ListReleaseLabelsRequestMarshaller;
import software.amazon.awssdk.services.emr.transform.ListSecurityConfigurationsRequestMarshaller;
import software.amazon.awssdk.services.emr.transform.ListStepsRequestMarshaller;
import software.amazon.awssdk.services.emr.transform.ListStudioSessionMappingsRequestMarshaller;
import software.amazon.awssdk.services.emr.transform.ListStudiosRequestMarshaller;
import software.amazon.awssdk.services.emr.transform.ListSupportedInstanceTypesRequestMarshaller;
import software.amazon.awssdk.services.emr.transform.ModifyClusterRequestMarshaller;
import software.amazon.awssdk.services.emr.transform.ModifyInstanceFleetRequestMarshaller;
import software.amazon.awssdk.services.emr.transform.ModifyInstanceGroupsRequestMarshaller;
import software.amazon.awssdk.services.emr.transform.PutAutoScalingPolicyRequestMarshaller;
import software.amazon.awssdk.services.emr.transform.PutAutoTerminationPolicyRequestMarshaller;
import software.amazon.awssdk.services.emr.transform.PutBlockPublicAccessConfigurationRequestMarshaller;
import software.amazon.awssdk.services.emr.transform.PutManagedScalingPolicyRequestMarshaller;
import software.amazon.awssdk.services.emr.transform.RemoveAutoScalingPolicyRequestMarshaller;
import software.amazon.awssdk.services.emr.transform.RemoveAutoTerminationPolicyRequestMarshaller;
import software.amazon.awssdk.services.emr.transform.RemoveManagedScalingPolicyRequestMarshaller;
import software.amazon.awssdk.services.emr.transform.RemoveTagsRequestMarshaller;
import software.amazon.awssdk.services.emr.transform.RunJobFlowRequestMarshaller;
import software.amazon.awssdk.services.emr.transform.SetKeepJobFlowAliveWhenNoStepsRequestMarshaller;
import software.amazon.awssdk.services.emr.transform.SetTerminationProtectionRequestMarshaller;
import software.amazon.awssdk.services.emr.transform.SetUnhealthyNodeReplacementRequestMarshaller;
import software.amazon.awssdk.services.emr.transform.SetVisibleToAllUsersRequestMarshaller;
import software.amazon.awssdk.services.emr.transform.StartNotebookExecutionRequestMarshaller;
import software.amazon.awssdk.services.emr.transform.StopNotebookExecutionRequestMarshaller;
import software.amazon.awssdk.services.emr.transform.TerminateJobFlowsRequestMarshaller;
import software.amazon.awssdk.services.emr.transform.UpdateStudioRequestMarshaller;
import software.amazon.awssdk.services.emr.transform.UpdateStudioSessionMappingRequestMarshaller;
import software.amazon.awssdk.services.emr.waiters.EmrWaiter;
import software.amazon.awssdk.utils.Logger;
/**
* Internal implementation of {@link EmrClient}.
*
* @see EmrClient#builder()
*/
@Generated("software.amazon.awssdk:codegen")
@SdkInternalApi
final class DefaultEmrClient implements EmrClient {
private static final Logger log = Logger.loggerFor(DefaultEmrClient.class);
private static final AwsProtocolMetadata protocolMetadata = AwsProtocolMetadata.builder()
.serviceProtocol(AwsServiceProtocol.AWS_JSON).build();
private final SyncClientHandler clientHandler;
private final AwsJsonProtocolFactory protocolFactory;
private final SdkClientConfiguration clientConfiguration;
protected DefaultEmrClient(SdkClientConfiguration clientConfiguration) {
this.clientHandler = new AwsSyncClientHandler(clientConfiguration);
this.clientConfiguration = clientConfiguration.toBuilder().option(SdkClientOption.SDK_CLIENT, this).build();
this.protocolFactory = init(AwsJsonProtocolFactory.builder()).build();
}
/**
*
* Adds an instance fleet to a running cluster.
*
*
*
* The instance fleet configuration is available only in Amazon EMR releases 4.8.0 and later, excluding 5.0.x.
*
*
*
* @param addInstanceFleetRequest
* @return Result of the AddInstanceFleet operation returned by the service.
* @throws InternalServerException
* This exception occurs when there is an internal failure in the Amazon EMR service.
* @throws InvalidRequestException
* This exception occurs when there is something wrong with user input.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EmrException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EmrClient.AddInstanceFleet
* @see AWS API Documentation
*/
@Override
public AddInstanceFleetResponse addInstanceFleet(AddInstanceFleetRequest addInstanceFleetRequest)
throws InternalServerException, InvalidRequestException, AwsServiceException, SdkClientException, EmrException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
AddInstanceFleetResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(addInstanceFleetRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, addInstanceFleetRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "EMR");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "AddInstanceFleet");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("AddInstanceFleet").withProtocolMetadata(protocolMetadata)
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withInput(addInstanceFleetRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new AddInstanceFleetRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Adds one or more instance groups to a running cluster.
*
*
* @param addInstanceGroupsRequest
* Input to an AddInstanceGroups call.
* @return Result of the AddInstanceGroups operation returned by the service.
* @throws InternalServerErrorException
* Indicates that an error occurred while processing the request and that the request was not completed.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EmrException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EmrClient.AddInstanceGroups
* @see AWS API Documentation
*/
@Override
public AddInstanceGroupsResponse addInstanceGroups(AddInstanceGroupsRequest addInstanceGroupsRequest)
throws InternalServerErrorException, AwsServiceException, SdkClientException, EmrException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
AddInstanceGroupsResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(addInstanceGroupsRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, addInstanceGroupsRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "EMR");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "AddInstanceGroups");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("AddInstanceGroups").withProtocolMetadata(protocolMetadata)
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withInput(addInstanceGroupsRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new AddInstanceGroupsRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* AddJobFlowSteps adds new steps to a running cluster. A maximum of 256 steps are allowed in each job flow.
*
*
* If your cluster is long-running (such as a Hive data warehouse) or complex, you may require more than 256 steps
* to process your data. You can bypass the 256-step limitation in various ways, including using SSH to connect to
* the master node and submitting queries directly to the software running on the master node, such as Hive and
* Hadoop.
*
*
* A step specifies the location of a JAR file stored either on the master node of the cluster or in Amazon S3. Each
* step is performed by the main function of the main class of the JAR file. The main class can be specified either
* in the manifest of the JAR or by using the MainFunction parameter of the step.
*
*
* Amazon EMR executes each step in the order listed. For a step to be considered complete, the main function must
* exit with a zero exit code and all Hadoop jobs started while the step was running must have completed and run
* successfully.
*
*
* You can only add steps to a cluster that is in one of the following states: STARTING, BOOTSTRAPPING, RUNNING, or
* WAITING.
*
*
*
* The string values passed into HadoopJarStep
object cannot exceed a total of 10240 characters.
*
*
*
* @param addJobFlowStepsRequest
* The input argument to the AddJobFlowSteps operation.
* @return Result of the AddJobFlowSteps operation returned by the service.
* @throws InternalServerErrorException
* Indicates that an error occurred while processing the request and that the request was not completed.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EmrException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EmrClient.AddJobFlowSteps
* @see AWS API Documentation
*/
@Override
public AddJobFlowStepsResponse addJobFlowSteps(AddJobFlowStepsRequest addJobFlowStepsRequest)
throws InternalServerErrorException, AwsServiceException, SdkClientException, EmrException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
AddJobFlowStepsResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(addJobFlowStepsRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, addJobFlowStepsRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "EMR");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "AddJobFlowSteps");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("AddJobFlowSteps").withProtocolMetadata(protocolMetadata)
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withInput(addJobFlowStepsRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new AddJobFlowStepsRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Adds tags to an Amazon EMR resource, such as a cluster or an Amazon EMR Studio. Tags make it easier to associate
* resources in various ways, such as grouping clusters to track your Amazon EMR resource allocation costs. For more
* information, see Tag
* Clusters.
*
*
* @param addTagsRequest
* This input identifies an Amazon EMR resource and a list of tags to attach.
* @return Result of the AddTags operation returned by the service.
* @throws InternalServerException
* This exception occurs when there is an internal failure in the Amazon EMR service.
* @throws InvalidRequestException
* This exception occurs when there is something wrong with user input.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EmrException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EmrClient.AddTags
* @see AWS API
* Documentation
*/
@Override
public AddTagsResponse addTags(AddTagsRequest addTagsRequest) throws InternalServerException, InvalidRequestException,
AwsServiceException, SdkClientException, EmrException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
AddTagsResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(addTagsRequest, this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, addTagsRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "EMR");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "AddTags");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("AddTags").withProtocolMetadata(protocolMetadata).withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withRequestConfiguration(clientConfiguration)
.withInput(addTagsRequest).withMetricCollector(apiCallMetricCollector)
.withMarshaller(new AddTagsRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Cancels a pending step or steps in a running cluster. Available only in Amazon EMR versions 4.8.0 and later,
* excluding version 5.0.0. A maximum of 256 steps are allowed in each CancelSteps request. CancelSteps is
* idempotent but asynchronous; it does not guarantee that a step will be canceled, even if the request is
* successfully submitted. When you use Amazon EMR releases 5.28.0 and later, you can cancel steps that are in a
* PENDING
or RUNNING
state. In earlier versions of Amazon EMR, you can only cancel steps
* that are in a PENDING
state.
*
*
* @param cancelStepsRequest
* The input argument to the CancelSteps operation.
* @return Result of the CancelSteps operation returned by the service.
* @throws InternalServerErrorException
* Indicates that an error occurred while processing the request and that the request was not completed.
* @throws InvalidRequestException
* This exception occurs when there is something wrong with user input.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EmrException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EmrClient.CancelSteps
* @see AWS
* API Documentation
*/
@Override
public CancelStepsResponse cancelSteps(CancelStepsRequest cancelStepsRequest) throws InternalServerErrorException,
InvalidRequestException, AwsServiceException, SdkClientException, EmrException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
CancelStepsResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(cancelStepsRequest, this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, cancelStepsRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "EMR");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "CancelSteps");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("CancelSteps").withProtocolMetadata(protocolMetadata).withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withRequestConfiguration(clientConfiguration)
.withInput(cancelStepsRequest).withMetricCollector(apiCallMetricCollector)
.withMarshaller(new CancelStepsRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Creates a security configuration, which is stored in the service and can be specified when a cluster is created.
*
*
* @param createSecurityConfigurationRequest
* @return Result of the CreateSecurityConfiguration operation returned by the service.
* @throws InternalServerException
* This exception occurs when there is an internal failure in the Amazon EMR service.
* @throws InvalidRequestException
* This exception occurs when there is something wrong with user input.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EmrException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EmrClient.CreateSecurityConfiguration
* @see AWS API Documentation
*/
@Override
public CreateSecurityConfigurationResponse createSecurityConfiguration(
CreateSecurityConfigurationRequest createSecurityConfigurationRequest) throws InternalServerException,
InvalidRequestException, AwsServiceException, SdkClientException, EmrException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, CreateSecurityConfigurationResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(createSecurityConfigurationRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, createSecurityConfigurationRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "EMR");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "CreateSecurityConfiguration");
return clientHandler
.execute(new ClientExecutionParams()
.withOperationName("CreateSecurityConfiguration").withProtocolMetadata(protocolMetadata)
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withInput(createSecurityConfigurationRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new CreateSecurityConfigurationRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Creates a new Amazon EMR Studio.
*
*
* @param createStudioRequest
* @return Result of the CreateStudio operation returned by the service.
* @throws InternalServerException
* This exception occurs when there is an internal failure in the Amazon EMR service.
* @throws InvalidRequestException
* This exception occurs when there is something wrong with user input.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EmrException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EmrClient.CreateStudio
* @see AWS
* API Documentation
*/
@Override
public CreateStudioResponse createStudio(CreateStudioRequest createStudioRequest) throws InternalServerException,
InvalidRequestException, AwsServiceException, SdkClientException, EmrException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
CreateStudioResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(createStudioRequest, this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, createStudioRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "EMR");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "CreateStudio");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("CreateStudio").withProtocolMetadata(protocolMetadata)
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withInput(createStudioRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new CreateStudioRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Maps a user or group to the Amazon EMR Studio specified by StudioId
, and applies a session policy to
* refine Studio permissions for that user or group. Use CreateStudioSessionMapping
to assign users to
* a Studio when you use IAM Identity Center authentication. For instructions on how to assign users to a Studio
* when you use IAM authentication, see Assign a user or group to your EMR Studio.
*
*
* @param createStudioSessionMappingRequest
* @return Result of the CreateStudioSessionMapping operation returned by the service.
* @throws InternalServerErrorException
* Indicates that an error occurred while processing the request and that the request was not completed.
* @throws InvalidRequestException
* This exception occurs when there is something wrong with user input.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EmrException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EmrClient.CreateStudioSessionMapping
* @see AWS API Documentation
*/
@Override
public CreateStudioSessionMappingResponse createStudioSessionMapping(
CreateStudioSessionMappingRequest createStudioSessionMappingRequest) throws InternalServerErrorException,
InvalidRequestException, AwsServiceException, SdkClientException, EmrException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, CreateStudioSessionMappingResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(createStudioSessionMappingRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, createStudioSessionMappingRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "EMR");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "CreateStudioSessionMapping");
return clientHandler
.execute(new ClientExecutionParams()
.withOperationName("CreateStudioSessionMapping").withProtocolMetadata(protocolMetadata)
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withInput(createStudioSessionMappingRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new CreateStudioSessionMappingRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Deletes a security configuration.
*
*
* @param deleteSecurityConfigurationRequest
* @return Result of the DeleteSecurityConfiguration operation returned by the service.
* @throws InternalServerException
* This exception occurs when there is an internal failure in the Amazon EMR service.
* @throws InvalidRequestException
* This exception occurs when there is something wrong with user input.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EmrException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EmrClient.DeleteSecurityConfiguration
* @see AWS API Documentation
*/
@Override
public DeleteSecurityConfigurationResponse deleteSecurityConfiguration(
DeleteSecurityConfigurationRequest deleteSecurityConfigurationRequest) throws InternalServerException,
InvalidRequestException, AwsServiceException, SdkClientException, EmrException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, DeleteSecurityConfigurationResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(deleteSecurityConfigurationRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, deleteSecurityConfigurationRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "EMR");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DeleteSecurityConfiguration");
return clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DeleteSecurityConfiguration").withProtocolMetadata(protocolMetadata)
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withInput(deleteSecurityConfigurationRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new DeleteSecurityConfigurationRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Removes an Amazon EMR Studio from the Studio metadata store.
*
*
* @param deleteStudioRequest
* @return Result of the DeleteStudio operation returned by the service.
* @throws InternalServerException
* This exception occurs when there is an internal failure in the Amazon EMR service.
* @throws InvalidRequestException
* This exception occurs when there is something wrong with user input.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EmrException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EmrClient.DeleteStudio
* @see AWS
* API Documentation
*/
@Override
public DeleteStudioResponse deleteStudio(DeleteStudioRequest deleteStudioRequest) throws InternalServerException,
InvalidRequestException, AwsServiceException, SdkClientException, EmrException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
DeleteStudioResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(deleteStudioRequest, this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, deleteStudioRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "EMR");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DeleteStudio");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("DeleteStudio").withProtocolMetadata(protocolMetadata)
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withInput(deleteStudioRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new DeleteStudioRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Removes a user or group from an Amazon EMR Studio.
*
*
* @param deleteStudioSessionMappingRequest
* @return Result of the DeleteStudioSessionMapping operation returned by the service.
* @throws InternalServerErrorException
* Indicates that an error occurred while processing the request and that the request was not completed.
* @throws InvalidRequestException
* This exception occurs when there is something wrong with user input.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EmrException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EmrClient.DeleteStudioSessionMapping
* @see AWS API Documentation
*/
@Override
public DeleteStudioSessionMappingResponse deleteStudioSessionMapping(
DeleteStudioSessionMappingRequest deleteStudioSessionMappingRequest) throws InternalServerErrorException,
InvalidRequestException, AwsServiceException, SdkClientException, EmrException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, DeleteStudioSessionMappingResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(deleteStudioSessionMappingRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, deleteStudioSessionMappingRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "EMR");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DeleteStudioSessionMapping");
return clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DeleteStudioSessionMapping").withProtocolMetadata(protocolMetadata)
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withInput(deleteStudioSessionMappingRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new DeleteStudioSessionMappingRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Provides cluster-level details including status, hardware and software configuration, VPC settings, and so on.
*
*
* @param describeClusterRequest
* This input determines which cluster to describe.
* @return Result of the DescribeCluster operation returned by the service.
* @throws InternalServerException
* This exception occurs when there is an internal failure in the Amazon EMR service.
* @throws InvalidRequestException
* This exception occurs when there is something wrong with user input.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EmrException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EmrClient.DescribeCluster
* @see AWS API Documentation
*/
@Override
public DescribeClusterResponse describeCluster(DescribeClusterRequest describeClusterRequest) throws InternalServerException,
InvalidRequestException, AwsServiceException, SdkClientException, EmrException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
DescribeClusterResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(describeClusterRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, describeClusterRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "EMR");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DescribeCluster");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("DescribeCluster").withProtocolMetadata(protocolMetadata)
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withInput(describeClusterRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new DescribeClusterRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Provides details of a notebook execution.
*
*
* @param describeNotebookExecutionRequest
* @return Result of the DescribeNotebookExecution operation returned by the service.
* @throws InternalServerErrorException
* Indicates that an error occurred while processing the request and that the request was not completed.
* @throws InvalidRequestException
* This exception occurs when there is something wrong with user input.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EmrException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EmrClient.DescribeNotebookExecution
* @see AWS API Documentation
*/
@Override
public DescribeNotebookExecutionResponse describeNotebookExecution(
DescribeNotebookExecutionRequest describeNotebookExecutionRequest) throws InternalServerErrorException,
InvalidRequestException, AwsServiceException, SdkClientException, EmrException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, DescribeNotebookExecutionResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(describeNotebookExecutionRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, describeNotebookExecutionRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "EMR");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DescribeNotebookExecution");
return clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DescribeNotebookExecution").withProtocolMetadata(protocolMetadata)
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withInput(describeNotebookExecutionRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new DescribeNotebookExecutionRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Provides Amazon EMR release label details, such as the releases available the Region where the API request is
* run, and the available applications for a specific Amazon EMR release label. Can also list Amazon EMR releases
* that support a specified version of Spark.
*
*
* @param describeReleaseLabelRequest
* @return Result of the DescribeReleaseLabel operation returned by the service.
* @throws InternalServerException
* This exception occurs when there is an internal failure in the Amazon EMR service.
* @throws InvalidRequestException
* This exception occurs when there is something wrong with user input.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EmrException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EmrClient.DescribeReleaseLabel
* @see AWS API Documentation
*/
@Override
public DescribeReleaseLabelResponse describeReleaseLabel(DescribeReleaseLabelRequest describeReleaseLabelRequest)
throws InternalServerException, InvalidRequestException, AwsServiceException, SdkClientException, EmrException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, DescribeReleaseLabelResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(describeReleaseLabelRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, describeReleaseLabelRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "EMR");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DescribeReleaseLabel");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("DescribeReleaseLabel").withProtocolMetadata(protocolMetadata)
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withInput(describeReleaseLabelRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new DescribeReleaseLabelRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Provides the details of a security configuration by returning the configuration JSON.
*
*
* @param describeSecurityConfigurationRequest
* @return Result of the DescribeSecurityConfiguration operation returned by the service.
* @throws InternalServerException
* This exception occurs when there is an internal failure in the Amazon EMR service.
* @throws InvalidRequestException
* This exception occurs when there is something wrong with user input.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EmrException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EmrClient.DescribeSecurityConfiguration
* @see AWS API Documentation
*/
@Override
public DescribeSecurityConfigurationResponse describeSecurityConfiguration(
DescribeSecurityConfigurationRequest describeSecurityConfigurationRequest) throws InternalServerException,
InvalidRequestException, AwsServiceException, SdkClientException, EmrException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, DescribeSecurityConfigurationResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(describeSecurityConfigurationRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration,
describeSecurityConfigurationRequest.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "EMR");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DescribeSecurityConfiguration");
return clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DescribeSecurityConfiguration").withProtocolMetadata(protocolMetadata)
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withInput(describeSecurityConfigurationRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new DescribeSecurityConfigurationRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Provides more detail about the cluster step.
*
*
* @param describeStepRequest
* This input determines which step to describe.
* @return Result of the DescribeStep operation returned by the service.
* @throws InternalServerException
* This exception occurs when there is an internal failure in the Amazon EMR service.
* @throws InvalidRequestException
* This exception occurs when there is something wrong with user input.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EmrException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EmrClient.DescribeStep
* @see AWS
* API Documentation
*/
@Override
public DescribeStepResponse describeStep(DescribeStepRequest describeStepRequest) throws InternalServerException,
InvalidRequestException, AwsServiceException, SdkClientException, EmrException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
DescribeStepResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(describeStepRequest, this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, describeStepRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "EMR");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DescribeStep");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("DescribeStep").withProtocolMetadata(protocolMetadata)
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withInput(describeStepRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new DescribeStepRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Returns details for the specified Amazon EMR Studio including ID, Name, VPC, Studio access URL, and so on.
*
*
* @param describeStudioRequest
* @return Result of the DescribeStudio operation returned by the service.
* @throws InternalServerException
* This exception occurs when there is an internal failure in the Amazon EMR service.
* @throws InvalidRequestException
* This exception occurs when there is something wrong with user input.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EmrException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EmrClient.DescribeStudio
* @see AWS API Documentation
*/
@Override
public DescribeStudioResponse describeStudio(DescribeStudioRequest describeStudioRequest) throws InternalServerException,
InvalidRequestException, AwsServiceException, SdkClientException, EmrException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
DescribeStudioResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(describeStudioRequest, this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, describeStudioRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "EMR");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DescribeStudio");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("DescribeStudio").withProtocolMetadata(protocolMetadata)
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withInput(describeStudioRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new DescribeStudioRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Returns the auto-termination policy for an Amazon EMR cluster.
*
*
* @param getAutoTerminationPolicyRequest
* @return Result of the GetAutoTerminationPolicy operation returned by the service.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EmrException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EmrClient.GetAutoTerminationPolicy
* @see AWS API Documentation
*/
@Override
public GetAutoTerminationPolicyResponse getAutoTerminationPolicy(
GetAutoTerminationPolicyRequest getAutoTerminationPolicyRequest) throws AwsServiceException, SdkClientException,
EmrException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, GetAutoTerminationPolicyResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(getAutoTerminationPolicyRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, getAutoTerminationPolicyRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "EMR");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "GetAutoTerminationPolicy");
return clientHandler
.execute(new ClientExecutionParams()
.withOperationName("GetAutoTerminationPolicy").withProtocolMetadata(protocolMetadata)
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withInput(getAutoTerminationPolicyRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new GetAutoTerminationPolicyRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Returns the Amazon EMR block public access configuration for your Amazon Web Services account in the current
* Region. For more information see Configure Block
* Public Access for Amazon EMR in the Amazon EMR Management Guide.
*
*
* @param getBlockPublicAccessConfigurationRequest
* @return Result of the GetBlockPublicAccessConfiguration operation returned by the service.
* @throws InternalServerException
* This exception occurs when there is an internal failure in the Amazon EMR service.
* @throws InvalidRequestException
* This exception occurs when there is something wrong with user input.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EmrException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EmrClient.GetBlockPublicAccessConfiguration
* @see AWS API Documentation
*/
@Override
public GetBlockPublicAccessConfigurationResponse getBlockPublicAccessConfiguration(
GetBlockPublicAccessConfigurationRequest getBlockPublicAccessConfigurationRequest) throws InternalServerException,
InvalidRequestException, AwsServiceException, SdkClientException, EmrException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, GetBlockPublicAccessConfigurationResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(getBlockPublicAccessConfigurationRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration,
getBlockPublicAccessConfigurationRequest.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "EMR");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "GetBlockPublicAccessConfiguration");
return clientHandler
.execute(new ClientExecutionParams()
.withOperationName("GetBlockPublicAccessConfiguration").withProtocolMetadata(protocolMetadata)
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withInput(getBlockPublicAccessConfigurationRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new GetBlockPublicAccessConfigurationRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Provides temporary, HTTP basic credentials that are associated with a given runtime IAM role and used by a
* cluster with fine-grained access control activated. You can use these credentials to connect to cluster endpoints
* that support username and password authentication.
*
*
* @param getClusterSessionCredentialsRequest
* @return Result of the GetClusterSessionCredentials operation returned by the service.
* @throws InternalServerErrorException
* Indicates that an error occurred while processing the request and that the request was not completed.
* @throws InvalidRequestException
* This exception occurs when there is something wrong with user input.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EmrException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EmrClient.GetClusterSessionCredentials
* @see AWS API Documentation
*/
@Override
public GetClusterSessionCredentialsResponse getClusterSessionCredentials(
GetClusterSessionCredentialsRequest getClusterSessionCredentialsRequest) throws InternalServerErrorException,
InvalidRequestException, AwsServiceException, SdkClientException, EmrException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, GetClusterSessionCredentialsResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(getClusterSessionCredentialsRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, getClusterSessionCredentialsRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "EMR");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "GetClusterSessionCredentials");
return clientHandler
.execute(new ClientExecutionParams()
.withOperationName("GetClusterSessionCredentials").withProtocolMetadata(protocolMetadata)
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withInput(getClusterSessionCredentialsRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new GetClusterSessionCredentialsRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Fetches the attached managed scaling policy for an Amazon EMR cluster.
*
*
* @param getManagedScalingPolicyRequest
* @return Result of the GetManagedScalingPolicy operation returned by the service.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EmrException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EmrClient.GetManagedScalingPolicy
* @see AWS API Documentation
*/
@Override
public GetManagedScalingPolicyResponse getManagedScalingPolicy(GetManagedScalingPolicyRequest getManagedScalingPolicyRequest)
throws AwsServiceException, SdkClientException, EmrException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, GetManagedScalingPolicyResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(getManagedScalingPolicyRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, getManagedScalingPolicyRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "EMR");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "GetManagedScalingPolicy");
return clientHandler
.execute(new ClientExecutionParams()
.withOperationName("GetManagedScalingPolicy").withProtocolMetadata(protocolMetadata)
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withInput(getManagedScalingPolicyRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new GetManagedScalingPolicyRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Fetches mapping details for the specified Amazon EMR Studio and identity (user or group).
*
*
* @param getStudioSessionMappingRequest
* @return Result of the GetStudioSessionMapping operation returned by the service.
* @throws InternalServerErrorException
* Indicates that an error occurred while processing the request and that the request was not completed.
* @throws InvalidRequestException
* This exception occurs when there is something wrong with user input.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EmrException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EmrClient.GetStudioSessionMapping
* @see AWS API Documentation
*/
@Override
public GetStudioSessionMappingResponse getStudioSessionMapping(GetStudioSessionMappingRequest getStudioSessionMappingRequest)
throws InternalServerErrorException, InvalidRequestException, AwsServiceException, SdkClientException, EmrException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, GetStudioSessionMappingResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(getStudioSessionMappingRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, getStudioSessionMappingRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "EMR");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "GetStudioSessionMapping");
return clientHandler
.execute(new ClientExecutionParams()
.withOperationName("GetStudioSessionMapping").withProtocolMetadata(protocolMetadata)
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withInput(getStudioSessionMappingRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new GetStudioSessionMappingRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Provides information about the bootstrap actions associated with a cluster.
*
*
* @param listBootstrapActionsRequest
* This input determines which bootstrap actions to retrieve.
* @return Result of the ListBootstrapActions operation returned by the service.
* @throws InternalServerException
* This exception occurs when there is an internal failure in the Amazon EMR service.
* @throws InvalidRequestException
* This exception occurs when there is something wrong with user input.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EmrException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EmrClient.ListBootstrapActions
* @see AWS API Documentation
*/
@Override
public ListBootstrapActionsResponse listBootstrapActions(ListBootstrapActionsRequest listBootstrapActionsRequest)
throws InternalServerException, InvalidRequestException, AwsServiceException, SdkClientException, EmrException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, ListBootstrapActionsResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(listBootstrapActionsRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, listBootstrapActionsRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "EMR");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "ListBootstrapActions");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("ListBootstrapActions").withProtocolMetadata(protocolMetadata)
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withInput(listBootstrapActionsRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new ListBootstrapActionsRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Provides the status of all clusters visible to this Amazon Web Services account. Allows you to filter the list of
* clusters based on certain criteria; for example, filtering by cluster creation date and time or by status. This
* call returns a maximum of 50 clusters in unsorted order per call, but returns a marker to track the paging of the
* cluster list across multiple ListClusters calls.
*
*
* @param listClustersRequest
* This input determines how the ListClusters action filters the list of clusters that it returns.
* @return Result of the ListClusters operation returned by the service.
* @throws InternalServerException
* This exception occurs when there is an internal failure in the Amazon EMR service.
* @throws InvalidRequestException
* This exception occurs when there is something wrong with user input.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EmrException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EmrClient.ListClusters
* @see AWS
* API Documentation
*/
@Override
public ListClustersResponse listClusters(ListClustersRequest listClustersRequest) throws InternalServerException,
InvalidRequestException, AwsServiceException, SdkClientException, EmrException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
ListClustersResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(listClustersRequest, this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, listClustersRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "EMR");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "ListClusters");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("ListClusters").withProtocolMetadata(protocolMetadata)
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withInput(listClustersRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new ListClustersRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Lists all available details about the instance fleets in a cluster.
*
*
*
* The instance fleet configuration is available only in Amazon EMR releases 4.8.0 and later, excluding 5.0.x
* versions.
*
*
*
* @param listInstanceFleetsRequest
* @return Result of the ListInstanceFleets operation returned by the service.
* @throws InternalServerException
* This exception occurs when there is an internal failure in the Amazon EMR service.
* @throws InvalidRequestException
* This exception occurs when there is something wrong with user input.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EmrException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EmrClient.ListInstanceFleets
* @see AWS API Documentation
*/
@Override
public ListInstanceFleetsResponse listInstanceFleets(ListInstanceFleetsRequest listInstanceFleetsRequest)
throws InternalServerException, InvalidRequestException, AwsServiceException, SdkClientException, EmrException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, ListInstanceFleetsResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(listInstanceFleetsRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, listInstanceFleetsRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "EMR");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "ListInstanceFleets");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("ListInstanceFleets").withProtocolMetadata(protocolMetadata)
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withInput(listInstanceFleetsRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new ListInstanceFleetsRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Provides all available details about the instance groups in a cluster.
*
*
* @param listInstanceGroupsRequest
* This input determines which instance groups to retrieve.
* @return Result of the ListInstanceGroups operation returned by the service.
* @throws InternalServerException
* This exception occurs when there is an internal failure in the Amazon EMR service.
* @throws InvalidRequestException
* This exception occurs when there is something wrong with user input.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EmrException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EmrClient.ListInstanceGroups
* @see AWS API Documentation
*/
@Override
public ListInstanceGroupsResponse listInstanceGroups(ListInstanceGroupsRequest listInstanceGroupsRequest)
throws InternalServerException, InvalidRequestException, AwsServiceException, SdkClientException, EmrException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, ListInstanceGroupsResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(listInstanceGroupsRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, listInstanceGroupsRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "EMR");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "ListInstanceGroups");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("ListInstanceGroups").withProtocolMetadata(protocolMetadata)
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withInput(listInstanceGroupsRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new ListInstanceGroupsRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Provides information for all active Amazon EC2 instances and Amazon EC2 instances terminated in the last 30 days,
* up to a maximum of 2,000. Amazon EC2 instances in any of the following states are considered active:
* AWAITING_FULFILLMENT, PROVISIONING, BOOTSTRAPPING, RUNNING.
*
*
* @param listInstancesRequest
* This input determines which instances to list.
* @return Result of the ListInstances operation returned by the service.
* @throws InternalServerException
* This exception occurs when there is an internal failure in the Amazon EMR service.
* @throws InvalidRequestException
* This exception occurs when there is something wrong with user input.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EmrException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EmrClient.ListInstances
* @see AWS API Documentation
*/
@Override
public ListInstancesResponse listInstances(ListInstancesRequest listInstancesRequest) throws InternalServerException,
InvalidRequestException, AwsServiceException, SdkClientException, EmrException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
ListInstancesResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(listInstancesRequest, this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, listInstancesRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "EMR");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "ListInstances");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("ListInstances").withProtocolMetadata(protocolMetadata)
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withInput(listInstancesRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new ListInstancesRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Provides summaries of all notebook executions. You can filter the list based on multiple criteria such as status,
* time range, and editor id. Returns a maximum of 50 notebook executions and a marker to track the paging of a
* longer notebook execution list across multiple ListNotebookExecutions
calls.
*
*
* @param listNotebookExecutionsRequest
* @return Result of the ListNotebookExecutions operation returned by the service.
* @throws InternalServerErrorException
* Indicates that an error occurred while processing the request and that the request was not completed.
* @throws InvalidRequestException
* This exception occurs when there is something wrong with user input.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EmrException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EmrClient.ListNotebookExecutions
* @see AWS API Documentation
*/
@Override
public ListNotebookExecutionsResponse listNotebookExecutions(ListNotebookExecutionsRequest listNotebookExecutionsRequest)
throws InternalServerErrorException, InvalidRequestException, AwsServiceException, SdkClientException, EmrException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, ListNotebookExecutionsResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(listNotebookExecutionsRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, listNotebookExecutionsRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "EMR");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "ListNotebookExecutions");
return clientHandler
.execute(new ClientExecutionParams()
.withOperationName("ListNotebookExecutions").withProtocolMetadata(protocolMetadata)
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withInput(listNotebookExecutionsRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new ListNotebookExecutionsRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Retrieves release labels of Amazon EMR services in the Region where the API is called.
*
*
* @param listReleaseLabelsRequest
* @return Result of the ListReleaseLabels operation returned by the service.
* @throws InternalServerException
* This exception occurs when there is an internal failure in the Amazon EMR service.
* @throws InvalidRequestException
* This exception occurs when there is something wrong with user input.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EmrException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EmrClient.ListReleaseLabels
* @see AWS API Documentation
*/
@Override
public ListReleaseLabelsResponse listReleaseLabels(ListReleaseLabelsRequest listReleaseLabelsRequest)
throws InternalServerException, InvalidRequestException, AwsServiceException, SdkClientException, EmrException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
ListReleaseLabelsResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(listReleaseLabelsRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, listReleaseLabelsRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "EMR");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "ListReleaseLabels");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("ListReleaseLabels").withProtocolMetadata(protocolMetadata)
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withInput(listReleaseLabelsRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new ListReleaseLabelsRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Lists all the security configurations visible to this account, providing their creation dates and times, and
* their names. This call returns a maximum of 50 clusters per call, but returns a marker to track the paging of the
* cluster list across multiple ListSecurityConfigurations calls.
*
*
* @param listSecurityConfigurationsRequest
* @return Result of the ListSecurityConfigurations operation returned by the service.
* @throws InternalServerException
* This exception occurs when there is an internal failure in the Amazon EMR service.
* @throws InvalidRequestException
* This exception occurs when there is something wrong with user input.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EmrException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EmrClient.ListSecurityConfigurations
* @see AWS API Documentation
*/
@Override
public ListSecurityConfigurationsResponse listSecurityConfigurations(
ListSecurityConfigurationsRequest listSecurityConfigurationsRequest) throws InternalServerException,
InvalidRequestException, AwsServiceException, SdkClientException, EmrException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, ListSecurityConfigurationsResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(listSecurityConfigurationsRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, listSecurityConfigurationsRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "EMR");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "ListSecurityConfigurations");
return clientHandler
.execute(new ClientExecutionParams()
.withOperationName("ListSecurityConfigurations").withProtocolMetadata(protocolMetadata)
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withInput(listSecurityConfigurationsRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new ListSecurityConfigurationsRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Provides a list of steps for the cluster in reverse order unless you specify stepIds
with the
* request or filter by StepStates
. You can specify a maximum of 10 stepIDs
. The CLI
* automatically paginates results to return a list greater than 50 steps. To return more than 50 steps using the
* CLI, specify a Marker
, which is a pagination token that indicates the next set of steps to retrieve.
*
*
* @param listStepsRequest
* This input determines which steps to list.
* @return Result of the ListSteps operation returned by the service.
* @throws InternalServerException
* This exception occurs when there is an internal failure in the Amazon EMR service.
* @throws InvalidRequestException
* This exception occurs when there is something wrong with user input.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EmrException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EmrClient.ListSteps
* @see AWS
* API Documentation
*/
@Override
public ListStepsResponse listSteps(ListStepsRequest listStepsRequest) throws InternalServerException,
InvalidRequestException, AwsServiceException, SdkClientException, EmrException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
ListStepsResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(listStepsRequest, this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, listStepsRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "EMR");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "ListSteps");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("ListSteps").withProtocolMetadata(protocolMetadata).withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withRequestConfiguration(clientConfiguration)
.withInput(listStepsRequest).withMetricCollector(apiCallMetricCollector)
.withMarshaller(new ListStepsRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Returns a list of all user or group session mappings for the Amazon EMR Studio specified by StudioId
* .
*
*
* @param listStudioSessionMappingsRequest
* @return Result of the ListStudioSessionMappings operation returned by the service.
* @throws InternalServerErrorException
* Indicates that an error occurred while processing the request and that the request was not completed.
* @throws InvalidRequestException
* This exception occurs when there is something wrong with user input.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EmrException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EmrClient.ListStudioSessionMappings
* @see AWS API Documentation
*/
@Override
public ListStudioSessionMappingsResponse listStudioSessionMappings(
ListStudioSessionMappingsRequest listStudioSessionMappingsRequest) throws InternalServerErrorException,
InvalidRequestException, AwsServiceException, SdkClientException, EmrException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, ListStudioSessionMappingsResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(listStudioSessionMappingsRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, listStudioSessionMappingsRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "EMR");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "ListStudioSessionMappings");
return clientHandler
.execute(new ClientExecutionParams()
.withOperationName("ListStudioSessionMappings").withProtocolMetadata(protocolMetadata)
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withInput(listStudioSessionMappingsRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new ListStudioSessionMappingsRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Returns a list of all Amazon EMR Studios associated with the Amazon Web Services account. The list includes
* details such as ID, Studio Access URL, and creation time for each Studio.
*
*
* @param listStudiosRequest
* @return Result of the ListStudios operation returned by the service.
* @throws InternalServerException
* This exception occurs when there is an internal failure in the Amazon EMR service.
* @throws InvalidRequestException
* This exception occurs when there is something wrong with user input.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EmrException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EmrClient.ListStudios
* @see AWS
* API Documentation
*/
@Override
public ListStudiosResponse listStudios(ListStudiosRequest listStudiosRequest) throws InternalServerException,
InvalidRequestException, AwsServiceException, SdkClientException, EmrException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
ListStudiosResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(listStudiosRequest, this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, listStudiosRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "EMR");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "ListStudios");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("ListStudios").withProtocolMetadata(protocolMetadata).withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withRequestConfiguration(clientConfiguration)
.withInput(listStudiosRequest).withMetricCollector(apiCallMetricCollector)
.withMarshaller(new ListStudiosRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* A list of the instance types that Amazon EMR supports. You can filter the list by Amazon Web Services Region and
* Amazon EMR release.
*
*
* @param listSupportedInstanceTypesRequest
* @return Result of the ListSupportedInstanceTypes operation returned by the service.
* @throws InternalServerException
* This exception occurs when there is an internal failure in the Amazon EMR service.
* @throws InvalidRequestException
* This exception occurs when there is something wrong with user input.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EmrException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EmrClient.ListSupportedInstanceTypes
* @see AWS API Documentation
*/
@Override
public ListSupportedInstanceTypesResponse listSupportedInstanceTypes(
ListSupportedInstanceTypesRequest listSupportedInstanceTypesRequest) throws InternalServerException,
InvalidRequestException, AwsServiceException, SdkClientException, EmrException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, ListSupportedInstanceTypesResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(listSupportedInstanceTypesRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, listSupportedInstanceTypesRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "EMR");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "ListSupportedInstanceTypes");
return clientHandler
.execute(new ClientExecutionParams()
.withOperationName("ListSupportedInstanceTypes").withProtocolMetadata(protocolMetadata)
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withInput(listSupportedInstanceTypesRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new ListSupportedInstanceTypesRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Modifies the number of steps that can be executed concurrently for the cluster specified using ClusterID.
*
*
* @param modifyClusterRequest
* @return Result of the ModifyCluster operation returned by the service.
* @throws InternalServerErrorException
* Indicates that an error occurred while processing the request and that the request was not completed.
* @throws InvalidRequestException
* This exception occurs when there is something wrong with user input.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EmrException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EmrClient.ModifyCluster
* @see AWS API Documentation
*/
@Override
public ModifyClusterResponse modifyCluster(ModifyClusterRequest modifyClusterRequest) throws InternalServerErrorException,
InvalidRequestException, AwsServiceException, SdkClientException, EmrException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
ModifyClusterResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(modifyClusterRequest, this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, modifyClusterRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "EMR");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "ModifyCluster");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("ModifyCluster").withProtocolMetadata(protocolMetadata)
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withInput(modifyClusterRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new ModifyClusterRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Modifies the target On-Demand and target Spot capacities for the instance fleet with the specified
* InstanceFleetID within the cluster specified using ClusterID. The call either succeeds or fails atomically.
*
*
*
* The instance fleet configuration is available only in Amazon EMR releases 4.8.0 and later, excluding 5.0.x
* versions.
*
*
*
* @param modifyInstanceFleetRequest
* @return Result of the ModifyInstanceFleet operation returned by the service.
* @throws InternalServerException
* This exception occurs when there is an internal failure in the Amazon EMR service.
* @throws InvalidRequestException
* This exception occurs when there is something wrong with user input.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EmrException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EmrClient.ModifyInstanceFleet
* @see AWS API Documentation
*/
@Override
public ModifyInstanceFleetResponse modifyInstanceFleet(ModifyInstanceFleetRequest modifyInstanceFleetRequest)
throws InternalServerException, InvalidRequestException, AwsServiceException, SdkClientException, EmrException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, ModifyInstanceFleetResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(modifyInstanceFleetRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, modifyInstanceFleetRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "EMR");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "ModifyInstanceFleet");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("ModifyInstanceFleet").withProtocolMetadata(protocolMetadata)
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withInput(modifyInstanceFleetRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new ModifyInstanceFleetRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* ModifyInstanceGroups modifies the number of nodes and configuration settings of an instance group. The input
* parameters include the new target instance count for the group and the instance group ID. The call will either
* succeed or fail atomically.
*
*
* @param modifyInstanceGroupsRequest
* Change the size of some instance groups.
* @return Result of the ModifyInstanceGroups operation returned by the service.
* @throws InternalServerErrorException
* Indicates that an error occurred while processing the request and that the request was not completed.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EmrException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EmrClient.ModifyInstanceGroups
* @see AWS API Documentation
*/
@Override
public ModifyInstanceGroupsResponse modifyInstanceGroups(ModifyInstanceGroupsRequest modifyInstanceGroupsRequest)
throws InternalServerErrorException, AwsServiceException, SdkClientException, EmrException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, ModifyInstanceGroupsResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(modifyInstanceGroupsRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, modifyInstanceGroupsRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "EMR");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "ModifyInstanceGroups");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("ModifyInstanceGroups").withProtocolMetadata(protocolMetadata)
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withInput(modifyInstanceGroupsRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new ModifyInstanceGroupsRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Creates or updates an automatic scaling policy for a core instance group or task instance group in an Amazon EMR
* cluster. The automatic scaling policy defines how an instance group dynamically adds and terminates Amazon EC2
* instances in response to the value of a CloudWatch metric.
*
*
* @param putAutoScalingPolicyRequest
* @return Result of the PutAutoScalingPolicy operation returned by the service.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EmrException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EmrClient.PutAutoScalingPolicy
* @see AWS API Documentation
*/
@Override
public PutAutoScalingPolicyResponse putAutoScalingPolicy(PutAutoScalingPolicyRequest putAutoScalingPolicyRequest)
throws AwsServiceException, SdkClientException, EmrException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, PutAutoScalingPolicyResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(putAutoScalingPolicyRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, putAutoScalingPolicyRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "EMR");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "PutAutoScalingPolicy");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("PutAutoScalingPolicy").withProtocolMetadata(protocolMetadata)
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withInput(putAutoScalingPolicyRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new PutAutoScalingPolicyRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
*
* Auto-termination is supported in Amazon EMR releases 5.30.0 and 6.1.0 and later. For more information, see Using an
* auto-termination policy.
*
*
*
* Creates or updates an auto-termination policy for an Amazon EMR cluster. An auto-termination policy defines the
* amount of idle time in seconds after which a cluster automatically terminates. For alternative cluster
* termination options, see Control cluster
* termination.
*
*
* @param putAutoTerminationPolicyRequest
* @return Result of the PutAutoTerminationPolicy operation returned by the service.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EmrException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EmrClient.PutAutoTerminationPolicy
* @see AWS API Documentation
*/
@Override
public PutAutoTerminationPolicyResponse putAutoTerminationPolicy(
PutAutoTerminationPolicyRequest putAutoTerminationPolicyRequest) throws AwsServiceException, SdkClientException,
EmrException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, PutAutoTerminationPolicyResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(putAutoTerminationPolicyRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, putAutoTerminationPolicyRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "EMR");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "PutAutoTerminationPolicy");
return clientHandler
.execute(new ClientExecutionParams()
.withOperationName("PutAutoTerminationPolicy").withProtocolMetadata(protocolMetadata)
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withInput(putAutoTerminationPolicyRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new PutAutoTerminationPolicyRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Creates or updates an Amazon EMR block public access configuration for your Amazon Web Services account in the
* current Region. For more information see Configure Block
* Public Access for Amazon EMR in the Amazon EMR Management Guide.
*
*
* @param putBlockPublicAccessConfigurationRequest
* @return Result of the PutBlockPublicAccessConfiguration operation returned by the service.
* @throws InternalServerException
* This exception occurs when there is an internal failure in the Amazon EMR service.
* @throws InvalidRequestException
* This exception occurs when there is something wrong with user input.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EmrException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EmrClient.PutBlockPublicAccessConfiguration
* @see AWS API Documentation
*/
@Override
public PutBlockPublicAccessConfigurationResponse putBlockPublicAccessConfiguration(
PutBlockPublicAccessConfigurationRequest putBlockPublicAccessConfigurationRequest) throws InternalServerException,
InvalidRequestException, AwsServiceException, SdkClientException, EmrException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, PutBlockPublicAccessConfigurationResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(putBlockPublicAccessConfigurationRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration,
putBlockPublicAccessConfigurationRequest.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "EMR");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "PutBlockPublicAccessConfiguration");
return clientHandler
.execute(new ClientExecutionParams()
.withOperationName("PutBlockPublicAccessConfiguration").withProtocolMetadata(protocolMetadata)
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withInput(putBlockPublicAccessConfigurationRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new PutBlockPublicAccessConfigurationRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Creates or updates a managed scaling policy for an Amazon EMR cluster. The managed scaling policy defines the
* limits for resources, such as Amazon EC2 instances that can be added or terminated from a cluster. The policy
* only applies to the core and task nodes. The master node cannot be scaled after initial configuration.
*
*
* @param putManagedScalingPolicyRequest
* @return Result of the PutManagedScalingPolicy operation returned by the service.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EmrException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EmrClient.PutManagedScalingPolicy
* @see AWS API Documentation
*/
@Override
public PutManagedScalingPolicyResponse putManagedScalingPolicy(PutManagedScalingPolicyRequest putManagedScalingPolicyRequest)
throws AwsServiceException, SdkClientException, EmrException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, PutManagedScalingPolicyResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(putManagedScalingPolicyRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, putManagedScalingPolicyRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "EMR");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "PutManagedScalingPolicy");
return clientHandler
.execute(new ClientExecutionParams()
.withOperationName("PutManagedScalingPolicy").withProtocolMetadata(protocolMetadata)
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withInput(putManagedScalingPolicyRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new PutManagedScalingPolicyRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Removes an automatic scaling policy from a specified instance group within an Amazon EMR cluster.
*
*
* @param removeAutoScalingPolicyRequest
* @return Result of the RemoveAutoScalingPolicy operation returned by the service.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EmrException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EmrClient.RemoveAutoScalingPolicy
* @see AWS API Documentation
*/
@Override
public RemoveAutoScalingPolicyResponse removeAutoScalingPolicy(RemoveAutoScalingPolicyRequest removeAutoScalingPolicyRequest)
throws AwsServiceException, SdkClientException, EmrException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, RemoveAutoScalingPolicyResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(removeAutoScalingPolicyRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, removeAutoScalingPolicyRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "EMR");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "RemoveAutoScalingPolicy");
return clientHandler
.execute(new ClientExecutionParams()
.withOperationName("RemoveAutoScalingPolicy").withProtocolMetadata(protocolMetadata)
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withInput(removeAutoScalingPolicyRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new RemoveAutoScalingPolicyRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Removes an auto-termination policy from an Amazon EMR cluster.
*
*
* @param removeAutoTerminationPolicyRequest
* @return Result of the RemoveAutoTerminationPolicy operation returned by the service.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EmrException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EmrClient.RemoveAutoTerminationPolicy
* @see AWS API Documentation
*/
@Override
public RemoveAutoTerminationPolicyResponse removeAutoTerminationPolicy(
RemoveAutoTerminationPolicyRequest removeAutoTerminationPolicyRequest) throws AwsServiceException,
SdkClientException, EmrException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, RemoveAutoTerminationPolicyResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(removeAutoTerminationPolicyRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, removeAutoTerminationPolicyRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "EMR");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "RemoveAutoTerminationPolicy");
return clientHandler
.execute(new ClientExecutionParams()
.withOperationName("RemoveAutoTerminationPolicy").withProtocolMetadata(protocolMetadata)
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withInput(removeAutoTerminationPolicyRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new RemoveAutoTerminationPolicyRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Removes a managed scaling policy from a specified Amazon EMR cluster.
*
*
* @param removeManagedScalingPolicyRequest
* @return Result of the RemoveManagedScalingPolicy operation returned by the service.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EmrException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EmrClient.RemoveManagedScalingPolicy
* @see AWS API Documentation
*/
@Override
public RemoveManagedScalingPolicyResponse removeManagedScalingPolicy(
RemoveManagedScalingPolicyRequest removeManagedScalingPolicyRequest) throws AwsServiceException, SdkClientException,
EmrException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, RemoveManagedScalingPolicyResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(removeManagedScalingPolicyRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, removeManagedScalingPolicyRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "EMR");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "RemoveManagedScalingPolicy");
return clientHandler
.execute(new ClientExecutionParams()
.withOperationName("RemoveManagedScalingPolicy").withProtocolMetadata(protocolMetadata)
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withInput(removeManagedScalingPolicyRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new RemoveManagedScalingPolicyRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Removes tags from an Amazon EMR resource, such as a cluster or Amazon EMR Studio. Tags make it easier to
* associate resources in various ways, such as grouping clusters to track your Amazon EMR resource allocation
* costs. For more information, see Tag Clusters.
*
*
* The following example removes the stack tag with value Prod from a cluster:
*
*
* @param removeTagsRequest
* This input identifies an Amazon EMR resource and a list of tags to remove.
* @return Result of the RemoveTags operation returned by the service.
* @throws InternalServerException
* This exception occurs when there is an internal failure in the Amazon EMR service.
* @throws InvalidRequestException
* This exception occurs when there is something wrong with user input.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EmrException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EmrClient.RemoveTags
* @see AWS
* API Documentation
*/
@Override
public RemoveTagsResponse removeTags(RemoveTagsRequest removeTagsRequest) throws InternalServerException,
InvalidRequestException, AwsServiceException, SdkClientException, EmrException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
RemoveTagsResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(removeTagsRequest, this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, removeTagsRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "EMR");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "RemoveTags");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("RemoveTags").withProtocolMetadata(protocolMetadata).withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withRequestConfiguration(clientConfiguration)
.withInput(removeTagsRequest).withMetricCollector(apiCallMetricCollector)
.withMarshaller(new RemoveTagsRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* RunJobFlow creates and starts running a new cluster (job flow). The cluster runs the steps specified. After the
* steps complete, the cluster stops and the HDFS partition is lost. To prevent loss of data, configure the last
* step of the job flow to store results in Amazon S3. If the JobFlowInstancesConfig
* KeepJobFlowAliveWhenNoSteps
parameter is set to TRUE
, the cluster transitions to the
* WAITING state rather than shutting down after the steps have completed.
*
*
* For additional protection, you can set the JobFlowInstancesConfig TerminationProtected
* parameter to TRUE
to lock the cluster and prevent it from being terminated by API call, user
* intervention, or in the event of a job flow error.
*
*
* A maximum of 256 steps are allowed in each job flow.
*
*
* If your cluster is long-running (such as a Hive data warehouse) or complex, you may require more than 256 steps
* to process your data. You can bypass the 256-step limitation in various ways, including using the SSH shell to
* connect to the master node and submitting queries directly to the software running on the master node, such as
* Hive and Hadoop.
*
*
* For long-running clusters, we recommend that you periodically store your results.
*
*
*
* The instance fleets configuration is available only in Amazon EMR releases 4.8.0 and later, excluding 5.0.x
* versions. The RunJobFlow request can contain InstanceFleets parameters or InstanceGroups parameters, but not
* both.
*
*
*
* @param runJobFlowRequest
* Input to the RunJobFlow operation.
* @return Result of the RunJobFlow operation returned by the service.
* @throws InternalServerErrorException
* Indicates that an error occurred while processing the request and that the request was not completed.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EmrException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EmrClient.RunJobFlow
* @see AWS
* API Documentation
*/
@Override
public RunJobFlowResponse runJobFlow(RunJobFlowRequest runJobFlowRequest) throws InternalServerErrorException,
AwsServiceException, SdkClientException, EmrException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
RunJobFlowResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(runJobFlowRequest, this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, runJobFlowRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "EMR");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "RunJobFlow");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("RunJobFlow").withProtocolMetadata(protocolMetadata).withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withRequestConfiguration(clientConfiguration)
.withInput(runJobFlowRequest).withMetricCollector(apiCallMetricCollector)
.withMarshaller(new RunJobFlowRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* You can use the SetKeepJobFlowAliveWhenNoSteps
to configure a cluster (job flow) to terminate after
* the step execution, i.e., all your steps are executed. If you want a transient cluster that shuts down after the
* last of the current executing steps are completed, you can configure SetKeepJobFlowAliveWhenNoSteps
* to false. If you want a long running cluster, configure SetKeepJobFlowAliveWhenNoSteps
to true.
*
*
* For more information, see Managing
* Cluster Termination in the Amazon EMR Management Guide.
*
*
* @param setKeepJobFlowAliveWhenNoStepsRequest
* @return Result of the SetKeepJobFlowAliveWhenNoSteps operation returned by the service.
* @throws InternalServerErrorException
* Indicates that an error occurred while processing the request and that the request was not completed.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EmrException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EmrClient.SetKeepJobFlowAliveWhenNoSteps
* @see AWS API Documentation
*/
@Override
public SetKeepJobFlowAliveWhenNoStepsResponse setKeepJobFlowAliveWhenNoSteps(
SetKeepJobFlowAliveWhenNoStepsRequest setKeepJobFlowAliveWhenNoStepsRequest) throws InternalServerErrorException,
AwsServiceException, SdkClientException, EmrException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, SetKeepJobFlowAliveWhenNoStepsResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(setKeepJobFlowAliveWhenNoStepsRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration,
setKeepJobFlowAliveWhenNoStepsRequest.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "EMR");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "SetKeepJobFlowAliveWhenNoSteps");
return clientHandler
.execute(new ClientExecutionParams()
.withOperationName("SetKeepJobFlowAliveWhenNoSteps").withProtocolMetadata(protocolMetadata)
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withInput(setKeepJobFlowAliveWhenNoStepsRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new SetKeepJobFlowAliveWhenNoStepsRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* SetTerminationProtection locks a cluster (job flow) so the Amazon EC2 instances in the cluster cannot be
* terminated by user intervention, an API call, or in the event of a job-flow error. The cluster still terminates
* upon successful completion of the job flow. Calling SetTerminationProtection
on a cluster is similar
* to calling the Amazon EC2 DisableAPITermination
API on all Amazon EC2 instances in a cluster.
*
*
* SetTerminationProtection
is used to prevent accidental termination of a cluster and to ensure that
* in the event of an error, the instances persist so that you can recover any data stored in their ephemeral
* instance storage.
*
*
* To terminate a cluster that has been locked by setting SetTerminationProtection
to true
* , you must first unlock the job flow by a subsequent call to SetTerminationProtection
in which you
* set the value to false
.
*
*
* For more information, see Managing
* Cluster Termination in the Amazon EMR Management Guide.
*
*
* @param setTerminationProtectionRequest
* The input argument to the TerminationProtection operation.
* @return Result of the SetTerminationProtection operation returned by the service.
* @throws InternalServerErrorException
* Indicates that an error occurred while processing the request and that the request was not completed.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EmrException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EmrClient.SetTerminationProtection
* @see AWS API Documentation
*/
@Override
public SetTerminationProtectionResponse setTerminationProtection(
SetTerminationProtectionRequest setTerminationProtectionRequest) throws InternalServerErrorException,
AwsServiceException, SdkClientException, EmrException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, SetTerminationProtectionResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(setTerminationProtectionRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, setTerminationProtectionRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "EMR");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "SetTerminationProtection");
return clientHandler
.execute(new ClientExecutionParams()
.withOperationName("SetTerminationProtection").withProtocolMetadata(protocolMetadata)
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withInput(setTerminationProtectionRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new SetTerminationProtectionRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Specify whether to enable unhealthy node replacement, which lets Amazon EMR gracefully replace core nodes on a
* cluster if any nodes become unhealthy. For example, a node becomes unhealthy if disk usage is above 90%. If
* unhealthy node replacement is on and TerminationProtected
are off, Amazon EMR immediately terminates
* the unhealthy core nodes. To use unhealthy node replacement and retain unhealthy core nodes, use to turn on
* termination protection. In such cases, Amazon EMR adds the unhealthy nodes to a denylist, reducing job
* interruptions and failures.
*
*
* If unhealthy node replacement is on, Amazon EMR notifies YARN and other applications on the cluster to stop
* scheduling tasks with these nodes, moves the data, and then terminates the nodes.
*
*
* For more information, see graceful node
* replacement in the Amazon EMR Management Guide.
*
*
* @param setUnhealthyNodeReplacementRequest
* @return Result of the SetUnhealthyNodeReplacement operation returned by the service.
* @throws InternalServerErrorException
* Indicates that an error occurred while processing the request and that the request was not completed.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EmrException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EmrClient.SetUnhealthyNodeReplacement
* @see AWS API Documentation
*/
@Override
public SetUnhealthyNodeReplacementResponse setUnhealthyNodeReplacement(
SetUnhealthyNodeReplacementRequest setUnhealthyNodeReplacementRequest) throws InternalServerErrorException,
AwsServiceException, SdkClientException, EmrException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, SetUnhealthyNodeReplacementResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(setUnhealthyNodeReplacementRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, setUnhealthyNodeReplacementRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "EMR");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "SetUnhealthyNodeReplacement");
return clientHandler
.execute(new ClientExecutionParams()
.withOperationName("SetUnhealthyNodeReplacement").withProtocolMetadata(protocolMetadata)
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withInput(setUnhealthyNodeReplacementRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new SetUnhealthyNodeReplacementRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
*
* The SetVisibleToAllUsers parameter is no longer supported. Your cluster may be visible to all users in your
* account. To restrict cluster access using an IAM policy, see Identity and Access
* Management for Amazon EMR.
*
*
*
* Sets the Cluster$VisibleToAllUsers value for an Amazon EMR cluster. When true
, IAM
* principals in the Amazon Web Services account can perform Amazon EMR cluster actions that their IAM policies
* allow. When false
, only the IAM principal that created the cluster and the Amazon Web Services
* account root user can perform Amazon EMR actions on the cluster, regardless of IAM permissions policies attached
* to other IAM principals.
*
*
* This action works on running clusters. When you create a cluster, use the
* RunJobFlowInput$VisibleToAllUsers parameter.
*
*
* For more information, see Understanding the Amazon EMR Cluster VisibleToAllUsers Setting in the Amazon EMR Management Guide.
*
*
* @param setVisibleToAllUsersRequest
* The input to the SetVisibleToAllUsers action.
* @return Result of the SetVisibleToAllUsers operation returned by the service.
* @throws InternalServerErrorException
* Indicates that an error occurred while processing the request and that the request was not completed.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EmrException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EmrClient.SetVisibleToAllUsers
* @see AWS API Documentation
*/
@Override
public SetVisibleToAllUsersResponse setVisibleToAllUsers(SetVisibleToAllUsersRequest setVisibleToAllUsersRequest)
throws InternalServerErrorException, AwsServiceException, SdkClientException, EmrException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, SetVisibleToAllUsersResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(setVisibleToAllUsersRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, setVisibleToAllUsersRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "EMR");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "SetVisibleToAllUsers");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("SetVisibleToAllUsers").withProtocolMetadata(protocolMetadata)
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withInput(setVisibleToAllUsersRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new SetVisibleToAllUsersRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Starts a notebook execution.
*
*
* @param startNotebookExecutionRequest
* @return Result of the StartNotebookExecution operation returned by the service.
* @throws InternalServerException
* This exception occurs when there is an internal failure in the Amazon EMR service.
* @throws InvalidRequestException
* This exception occurs when there is something wrong with user input.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EmrException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EmrClient.StartNotebookExecution
* @see AWS API Documentation
*/
@Override
public StartNotebookExecutionResponse startNotebookExecution(StartNotebookExecutionRequest startNotebookExecutionRequest)
throws InternalServerException, InvalidRequestException, AwsServiceException, SdkClientException, EmrException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, StartNotebookExecutionResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(startNotebookExecutionRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, startNotebookExecutionRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "EMR");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "StartNotebookExecution");
return clientHandler
.execute(new ClientExecutionParams()
.withOperationName("StartNotebookExecution").withProtocolMetadata(protocolMetadata)
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withInput(startNotebookExecutionRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new StartNotebookExecutionRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Stops a notebook execution.
*
*
* @param stopNotebookExecutionRequest
* @return Result of the StopNotebookExecution operation returned by the service.
* @throws InternalServerErrorException
* Indicates that an error occurred while processing the request and that the request was not completed.
* @throws InvalidRequestException
* This exception occurs when there is something wrong with user input.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EmrException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EmrClient.StopNotebookExecution
* @see AWS API Documentation
*/
@Override
public StopNotebookExecutionResponse stopNotebookExecution(StopNotebookExecutionRequest stopNotebookExecutionRequest)
throws InternalServerErrorException, InvalidRequestException, AwsServiceException, SdkClientException, EmrException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, StopNotebookExecutionResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(stopNotebookExecutionRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, stopNotebookExecutionRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "EMR");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "StopNotebookExecution");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("StopNotebookExecution").withProtocolMetadata(protocolMetadata)
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withInput(stopNotebookExecutionRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new StopNotebookExecutionRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* TerminateJobFlows shuts a list of clusters (job flows) down. When a job flow is shut down, any step not yet
* completed is canceled and the Amazon EC2 instances on which the cluster is running are stopped. Any log files not
* already saved are uploaded to Amazon S3 if a LogUri was specified when the cluster was created.
*
*
* The maximum number of clusters allowed is 10. The call to TerminateJobFlows
is asynchronous.
* Depending on the configuration of the cluster, it may take up to 1-5 minutes for the cluster to completely
* terminate and release allocated resources, such as Amazon EC2 instances.
*
*
* @param terminateJobFlowsRequest
* Input to the TerminateJobFlows operation.
* @return Result of the TerminateJobFlows operation returned by the service.
* @throws InternalServerErrorException
* Indicates that an error occurred while processing the request and that the request was not completed.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EmrException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EmrClient.TerminateJobFlows
* @see AWS API Documentation
*/
@Override
public TerminateJobFlowsResponse terminateJobFlows(TerminateJobFlowsRequest terminateJobFlowsRequest)
throws InternalServerErrorException, AwsServiceException, SdkClientException, EmrException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
TerminateJobFlowsResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(terminateJobFlowsRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, terminateJobFlowsRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "EMR");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "TerminateJobFlows");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("TerminateJobFlows").withProtocolMetadata(protocolMetadata)
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withInput(terminateJobFlowsRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new TerminateJobFlowsRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Updates an Amazon EMR Studio configuration, including attributes such as name, description, and subnets.
*
*
* @param updateStudioRequest
* @return Result of the UpdateStudio operation returned by the service.
* @throws InternalServerException
* This exception occurs when there is an internal failure in the Amazon EMR service.
* @throws InvalidRequestException
* This exception occurs when there is something wrong with user input.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EmrException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EmrClient.UpdateStudio
* @see AWS
* API Documentation
*/
@Override
public UpdateStudioResponse updateStudio(UpdateStudioRequest updateStudioRequest) throws InternalServerException,
InvalidRequestException, AwsServiceException, SdkClientException, EmrException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
UpdateStudioResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(updateStudioRequest, this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, updateStudioRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "EMR");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "UpdateStudio");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("UpdateStudio").withProtocolMetadata(protocolMetadata)
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withInput(updateStudioRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new UpdateStudioRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Updates the session policy attached to the user or group for the specified Amazon EMR Studio.
*
*
* @param updateStudioSessionMappingRequest
* @return Result of the UpdateStudioSessionMapping operation returned by the service.
* @throws InternalServerErrorException
* Indicates that an error occurred while processing the request and that the request was not completed.
* @throws InvalidRequestException
* This exception occurs when there is something wrong with user input.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EmrException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EmrClient.UpdateStudioSessionMapping
* @see AWS API Documentation
*/
@Override
public UpdateStudioSessionMappingResponse updateStudioSessionMapping(
UpdateStudioSessionMappingRequest updateStudioSessionMappingRequest) throws InternalServerErrorException,
InvalidRequestException, AwsServiceException, SdkClientException, EmrException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, UpdateStudioSessionMappingResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(updateStudioSessionMappingRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, updateStudioSessionMappingRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "EMR");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "UpdateStudioSessionMapping");
return clientHandler
.execute(new ClientExecutionParams()
.withOperationName("UpdateStudioSessionMapping").withProtocolMetadata(protocolMetadata)
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withInput(updateStudioSessionMappingRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new UpdateStudioSessionMappingRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
* Create an instance of {@link EmrWaiter} using this client.
*
* Waiters created via this method are managed by the SDK and resources will be released when the service client is
* closed.
*
* @return an instance of {@link EmrWaiter}
*/
@Override
public EmrWaiter waiter() {
return EmrWaiter.builder().client(this).build();
}
@Override
public final String serviceName() {
return SERVICE_NAME;
}
private static List resolveMetricPublishers(SdkClientConfiguration clientConfiguration,
RequestOverrideConfiguration requestOverrideConfiguration) {
List publishers = null;
if (requestOverrideConfiguration != null) {
publishers = requestOverrideConfiguration.metricPublishers();
}
if (publishers == null || publishers.isEmpty()) {
publishers = clientConfiguration.option(SdkClientOption.METRIC_PUBLISHERS);
}
if (publishers == null) {
publishers = Collections.emptyList();
}
return publishers;
}
private HttpResponseHandler createErrorResponseHandler(BaseAwsJsonProtocolFactory protocolFactory,
JsonOperationMetadata operationMetadata) {
return protocolFactory.createErrorResponseHandler(operationMetadata);
}
private void updateRetryStrategyClientConfiguration(SdkClientConfiguration.Builder configuration) {
ClientOverrideConfiguration.Builder builder = configuration.asOverrideConfigurationBuilder();
RetryMode retryMode = builder.retryMode();
if (retryMode != null) {
configuration.option(SdkClientOption.RETRY_STRATEGY, AwsRetryStrategy.forRetryMode(retryMode));
} else {
Consumer> configurator = builder.retryStrategyConfigurator();
if (configurator != null) {
RetryStrategy.Builder, ?> defaultBuilder = AwsRetryStrategy.defaultRetryStrategy().toBuilder();
configurator.accept(defaultBuilder);
configuration.option(SdkClientOption.RETRY_STRATEGY, defaultBuilder.build());
} else {
RetryStrategy retryStrategy = builder.retryStrategy();
if (retryStrategy != null) {
configuration.option(SdkClientOption.RETRY_STRATEGY, retryStrategy);
}
}
}
configuration.option(SdkClientOption.CONFIGURED_RETRY_MODE, null);
configuration.option(SdkClientOption.CONFIGURED_RETRY_STRATEGY, null);
configuration.option(SdkClientOption.CONFIGURED_RETRY_CONFIGURATOR, null);
}
private SdkClientConfiguration updateSdkClientConfiguration(SdkRequest request, SdkClientConfiguration clientConfiguration) {
List plugins = request.overrideConfiguration().map(c -> c.plugins()).orElse(Collections.emptyList());
SdkClientConfiguration.Builder configuration = clientConfiguration.toBuilder();
if (plugins.isEmpty()) {
return configuration.build();
}
EmrServiceClientConfigurationBuilder serviceConfigBuilder = new EmrServiceClientConfigurationBuilder(configuration);
for (SdkPlugin plugin : plugins) {
plugin.configureClient(serviceConfigBuilder);
}
updateRetryStrategyClientConfiguration(configuration);
return configuration.build();
}
private > T init(T builder) {
return builder
.clientConfiguration(clientConfiguration)
.defaultServiceExceptionSupplier(EmrException::builder)
.protocol(AwsJsonProtocol.AWS_JSON)
.protocolVersion("1.1")
.registerModeledException(
ExceptionMetadata.builder().errorCode("InvalidRequestException")
.exceptionBuilderSupplier(InvalidRequestException::builder).httpStatusCode(400).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("InternalServerException")
.exceptionBuilderSupplier(InternalServerException::builder).httpStatusCode(500).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("InternalServerError")
.exceptionBuilderSupplier(InternalServerErrorException::builder).httpStatusCode(400).build());
}
@Override
public final EmrServiceClientConfiguration serviceClientConfiguration() {
return new EmrServiceClientConfigurationBuilder(this.clientConfiguration.toBuilder()).build();
}
@Override
public void close() {
clientHandler.close();
}
}