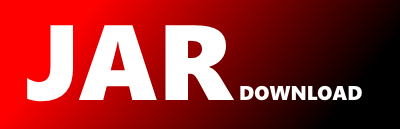
software.amazon.awssdk.services.emr.model.Ec2InstanceAttributes Maven / Gradle / Ivy
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.emr.model;
import java.io.Serializable;
import java.util.Arrays;
import java.util.Collection;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.ListTrait;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.core.util.DefaultSdkAutoConstructList;
import software.amazon.awssdk.core.util.SdkAutoConstructList;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* Provides information about the Amazon EC2 instances in a cluster grouped by category. For example, key name, subnet
* ID, IAM instance profile, and so on.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class Ec2InstanceAttributes implements SdkPojo, Serializable,
ToCopyableBuilder {
private static final SdkField EC2_KEY_NAME_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("Ec2KeyName").getter(getter(Ec2InstanceAttributes::ec2KeyName)).setter(setter(Builder::ec2KeyName))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Ec2KeyName").build()).build();
private static final SdkField EC2_SUBNET_ID_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("Ec2SubnetId").getter(getter(Ec2InstanceAttributes::ec2SubnetId)).setter(setter(Builder::ec2SubnetId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Ec2SubnetId").build()).build();
private static final SdkField> REQUESTED_EC2_SUBNET_IDS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("RequestedEc2SubnetIds")
.getter(getter(Ec2InstanceAttributes::requestedEc2SubnetIds))
.setter(setter(Builder::requestedEc2SubnetIds))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("RequestedEc2SubnetIds").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.STRING)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField EC2_AVAILABILITY_ZONE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("Ec2AvailabilityZone").getter(getter(Ec2InstanceAttributes::ec2AvailabilityZone))
.setter(setter(Builder::ec2AvailabilityZone))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Ec2AvailabilityZone").build())
.build();
private static final SdkField> REQUESTED_EC2_AVAILABILITY_ZONES_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("RequestedEc2AvailabilityZones")
.getter(getter(Ec2InstanceAttributes::requestedEc2AvailabilityZones))
.setter(setter(Builder::requestedEc2AvailabilityZones))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("RequestedEc2AvailabilityZones")
.build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.STRING)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField IAM_INSTANCE_PROFILE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("IamInstanceProfile").getter(getter(Ec2InstanceAttributes::iamInstanceProfile))
.setter(setter(Builder::iamInstanceProfile))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("IamInstanceProfile").build())
.build();
private static final SdkField EMR_MANAGED_MASTER_SECURITY_GROUP_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("EmrManagedMasterSecurityGroup")
.getter(getter(Ec2InstanceAttributes::emrManagedMasterSecurityGroup))
.setter(setter(Builder::emrManagedMasterSecurityGroup))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("EmrManagedMasterSecurityGroup")
.build()).build();
private static final SdkField EMR_MANAGED_SLAVE_SECURITY_GROUP_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("EmrManagedSlaveSecurityGroup")
.getter(getter(Ec2InstanceAttributes::emrManagedSlaveSecurityGroup))
.setter(setter(Builder::emrManagedSlaveSecurityGroup))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("EmrManagedSlaveSecurityGroup")
.build()).build();
private static final SdkField SERVICE_ACCESS_SECURITY_GROUP_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("ServiceAccessSecurityGroup")
.getter(getter(Ec2InstanceAttributes::serviceAccessSecurityGroup))
.setter(setter(Builder::serviceAccessSecurityGroup))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ServiceAccessSecurityGroup").build())
.build();
private static final SdkField> ADDITIONAL_MASTER_SECURITY_GROUPS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("AdditionalMasterSecurityGroups")
.getter(getter(Ec2InstanceAttributes::additionalMasterSecurityGroups))
.setter(setter(Builder::additionalMasterSecurityGroups))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("AdditionalMasterSecurityGroups")
.build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.STRING)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField> ADDITIONAL_SLAVE_SECURITY_GROUPS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("AdditionalSlaveSecurityGroups")
.getter(getter(Ec2InstanceAttributes::additionalSlaveSecurityGroups))
.setter(setter(Builder::additionalSlaveSecurityGroups))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("AdditionalSlaveSecurityGroups")
.build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.STRING)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(EC2_KEY_NAME_FIELD,
EC2_SUBNET_ID_FIELD, REQUESTED_EC2_SUBNET_IDS_FIELD, EC2_AVAILABILITY_ZONE_FIELD,
REQUESTED_EC2_AVAILABILITY_ZONES_FIELD, IAM_INSTANCE_PROFILE_FIELD, EMR_MANAGED_MASTER_SECURITY_GROUP_FIELD,
EMR_MANAGED_SLAVE_SECURITY_GROUP_FIELD, SERVICE_ACCESS_SECURITY_GROUP_FIELD, ADDITIONAL_MASTER_SECURITY_GROUPS_FIELD,
ADDITIONAL_SLAVE_SECURITY_GROUPS_FIELD));
private static final long serialVersionUID = 1L;
private final String ec2KeyName;
private final String ec2SubnetId;
private final List requestedEc2SubnetIds;
private final String ec2AvailabilityZone;
private final List requestedEc2AvailabilityZones;
private final String iamInstanceProfile;
private final String emrManagedMasterSecurityGroup;
private final String emrManagedSlaveSecurityGroup;
private final String serviceAccessSecurityGroup;
private final List additionalMasterSecurityGroups;
private final List additionalSlaveSecurityGroups;
private Ec2InstanceAttributes(BuilderImpl builder) {
this.ec2KeyName = builder.ec2KeyName;
this.ec2SubnetId = builder.ec2SubnetId;
this.requestedEc2SubnetIds = builder.requestedEc2SubnetIds;
this.ec2AvailabilityZone = builder.ec2AvailabilityZone;
this.requestedEc2AvailabilityZones = builder.requestedEc2AvailabilityZones;
this.iamInstanceProfile = builder.iamInstanceProfile;
this.emrManagedMasterSecurityGroup = builder.emrManagedMasterSecurityGroup;
this.emrManagedSlaveSecurityGroup = builder.emrManagedSlaveSecurityGroup;
this.serviceAccessSecurityGroup = builder.serviceAccessSecurityGroup;
this.additionalMasterSecurityGroups = builder.additionalMasterSecurityGroups;
this.additionalSlaveSecurityGroups = builder.additionalSlaveSecurityGroups;
}
/**
*
* The name of the Amazon EC2 key pair to use when connecting with SSH into the master node as a user named
* "hadoop".
*
*
* @return The name of the Amazon EC2 key pair to use when connecting with SSH into the master node as a user named
* "hadoop".
*/
public final String ec2KeyName() {
return ec2KeyName;
}
/**
*
* Set this parameter to the identifier of the Amazon VPC subnet where you want the cluster to launch. If you do not
* specify this value, and your account supports EC2-Classic, the cluster launches in EC2-Classic.
*
*
* @return Set this parameter to the identifier of the Amazon VPC subnet where you want the cluster to launch. If
* you do not specify this value, and your account supports EC2-Classic, the cluster launches in
* EC2-Classic.
*/
public final String ec2SubnetId() {
return ec2SubnetId;
}
/**
* For responses, this returns true if the service returned a value for the RequestedEc2SubnetIds property. This
* DOES NOT check that the value is non-empty (for which, you should check the {@code isEmpty()} method on the
* property). This is useful because the SDK will never return a null collection or map, but you may need to
* differentiate between the service returning nothing (or null) and the service returning an empty collection or
* map. For requests, this returns true if a value for the property was specified in the request builder, and false
* if a value was not specified.
*/
public final boolean hasRequestedEc2SubnetIds() {
return requestedEc2SubnetIds != null && !(requestedEc2SubnetIds instanceof SdkAutoConstructList);
}
/**
*
* Applies to clusters configured with the instance fleets option. Specifies the unique identifier of one or more
* Amazon EC2 subnets in which to launch Amazon EC2 cluster instances. Subnets must exist within the same VPC.
* Amazon EMR chooses the Amazon EC2 subnet with the best fit from among the list of
* RequestedEc2SubnetIds
, and then launches all cluster instances within that Subnet. If this value is
* not specified, and the account and Region support EC2-Classic networks, the cluster launches instances in the
* EC2-Classic network and uses RequestedEc2AvailabilityZones
instead of this setting. If EC2-Classic
* is not supported, and no Subnet is specified, Amazon EMR chooses the subnet for you.
* RequestedEc2SubnetIDs
and RequestedEc2AvailabilityZones
cannot be specified together.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasRequestedEc2SubnetIds} method.
*
*
* @return Applies to clusters configured with the instance fleets option. Specifies the unique identifier of one or
* more Amazon EC2 subnets in which to launch Amazon EC2 cluster instances. Subnets must exist within the
* same VPC. Amazon EMR chooses the Amazon EC2 subnet with the best fit from among the list of
* RequestedEc2SubnetIds
, and then launches all cluster instances within that Subnet. If this
* value is not specified, and the account and Region support EC2-Classic networks, the cluster launches
* instances in the EC2-Classic network and uses RequestedEc2AvailabilityZones
instead of this
* setting. If EC2-Classic is not supported, and no Subnet is specified, Amazon EMR chooses the subnet for
* you. RequestedEc2SubnetIDs
and RequestedEc2AvailabilityZones
cannot be
* specified together.
*/
public final List requestedEc2SubnetIds() {
return requestedEc2SubnetIds;
}
/**
*
* The Availability Zone in which the cluster will run.
*
*
* @return The Availability Zone in which the cluster will run.
*/
public final String ec2AvailabilityZone() {
return ec2AvailabilityZone;
}
/**
* For responses, this returns true if the service returned a value for the RequestedEc2AvailabilityZones property.
* This DOES NOT check that the value is non-empty (for which, you should check the {@code isEmpty()} method on the
* property). This is useful because the SDK will never return a null collection or map, but you may need to
* differentiate between the service returning nothing (or null) and the service returning an empty collection or
* map. For requests, this returns true if a value for the property was specified in the request builder, and false
* if a value was not specified.
*/
public final boolean hasRequestedEc2AvailabilityZones() {
return requestedEc2AvailabilityZones != null && !(requestedEc2AvailabilityZones instanceof SdkAutoConstructList);
}
/**
*
* Applies to clusters configured with the instance fleets option. Specifies one or more Availability Zones in which
* to launch Amazon EC2 cluster instances when the EC2-Classic network configuration is supported. Amazon EMR
* chooses the Availability Zone with the best fit from among the list of RequestedEc2AvailabilityZones
* , and then launches all cluster instances within that Availability Zone. If you do not specify this value, Amazon
* EMR chooses the Availability Zone for you. RequestedEc2SubnetIDs
and
* RequestedEc2AvailabilityZones
cannot be specified together.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasRequestedEc2AvailabilityZones} method.
*
*
* @return Applies to clusters configured with the instance fleets option. Specifies one or more Availability Zones
* in which to launch Amazon EC2 cluster instances when the EC2-Classic network configuration is supported.
* Amazon EMR chooses the Availability Zone with the best fit from among the list of
* RequestedEc2AvailabilityZones
, and then launches all cluster instances within that
* Availability Zone. If you do not specify this value, Amazon EMR chooses the Availability Zone for you.
* RequestedEc2SubnetIDs
and RequestedEc2AvailabilityZones
cannot be specified
* together.
*/
public final List requestedEc2AvailabilityZones() {
return requestedEc2AvailabilityZones;
}
/**
*
* The IAM role that was specified when the cluster was launched. The Amazon EC2 instances of the cluster assume
* this role.
*
*
* @return The IAM role that was specified when the cluster was launched. The Amazon EC2 instances of the cluster
* assume this role.
*/
public final String iamInstanceProfile() {
return iamInstanceProfile;
}
/**
*
* The identifier of the Amazon EC2 security group for the master node.
*
*
* @return The identifier of the Amazon EC2 security group for the master node.
*/
public final String emrManagedMasterSecurityGroup() {
return emrManagedMasterSecurityGroup;
}
/**
*
* The identifier of the Amazon EC2 security group for the core and task nodes.
*
*
* @return The identifier of the Amazon EC2 security group for the core and task nodes.
*/
public final String emrManagedSlaveSecurityGroup() {
return emrManagedSlaveSecurityGroup;
}
/**
*
* The identifier of the Amazon EC2 security group for the Amazon EMR service to access clusters in VPC private
* subnets.
*
*
* @return The identifier of the Amazon EC2 security group for the Amazon EMR service to access clusters in VPC
* private subnets.
*/
public final String serviceAccessSecurityGroup() {
return serviceAccessSecurityGroup;
}
/**
* For responses, this returns true if the service returned a value for the AdditionalMasterSecurityGroups property.
* This DOES NOT check that the value is non-empty (for which, you should check the {@code isEmpty()} method on the
* property). This is useful because the SDK will never return a null collection or map, but you may need to
* differentiate between the service returning nothing (or null) and the service returning an empty collection or
* map. For requests, this returns true if a value for the property was specified in the request builder, and false
* if a value was not specified.
*/
public final boolean hasAdditionalMasterSecurityGroups() {
return additionalMasterSecurityGroups != null && !(additionalMasterSecurityGroups instanceof SdkAutoConstructList);
}
/**
*
* A list of additional Amazon EC2 security group IDs for the master node.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasAdditionalMasterSecurityGroups} method.
*
*
* @return A list of additional Amazon EC2 security group IDs for the master node.
*/
public final List additionalMasterSecurityGroups() {
return additionalMasterSecurityGroups;
}
/**
* For responses, this returns true if the service returned a value for the AdditionalSlaveSecurityGroups property.
* This DOES NOT check that the value is non-empty (for which, you should check the {@code isEmpty()} method on the
* property). This is useful because the SDK will never return a null collection or map, but you may need to
* differentiate between the service returning nothing (or null) and the service returning an empty collection or
* map. For requests, this returns true if a value for the property was specified in the request builder, and false
* if a value was not specified.
*/
public final boolean hasAdditionalSlaveSecurityGroups() {
return additionalSlaveSecurityGroups != null && !(additionalSlaveSecurityGroups instanceof SdkAutoConstructList);
}
/**
*
* A list of additional Amazon EC2 security group IDs for the core and task nodes.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasAdditionalSlaveSecurityGroups} method.
*
*
* @return A list of additional Amazon EC2 security group IDs for the core and task nodes.
*/
public final List additionalSlaveSecurityGroups() {
return additionalSlaveSecurityGroups;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(ec2KeyName());
hashCode = 31 * hashCode + Objects.hashCode(ec2SubnetId());
hashCode = 31 * hashCode + Objects.hashCode(hasRequestedEc2SubnetIds() ? requestedEc2SubnetIds() : null);
hashCode = 31 * hashCode + Objects.hashCode(ec2AvailabilityZone());
hashCode = 31 * hashCode + Objects.hashCode(hasRequestedEc2AvailabilityZones() ? requestedEc2AvailabilityZones() : null);
hashCode = 31 * hashCode + Objects.hashCode(iamInstanceProfile());
hashCode = 31 * hashCode + Objects.hashCode(emrManagedMasterSecurityGroup());
hashCode = 31 * hashCode + Objects.hashCode(emrManagedSlaveSecurityGroup());
hashCode = 31 * hashCode + Objects.hashCode(serviceAccessSecurityGroup());
hashCode = 31 * hashCode
+ Objects.hashCode(hasAdditionalMasterSecurityGroups() ? additionalMasterSecurityGroups() : null);
hashCode = 31 * hashCode + Objects.hashCode(hasAdditionalSlaveSecurityGroups() ? additionalSlaveSecurityGroups() : null);
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof Ec2InstanceAttributes)) {
return false;
}
Ec2InstanceAttributes other = (Ec2InstanceAttributes) obj;
return Objects.equals(ec2KeyName(), other.ec2KeyName()) && Objects.equals(ec2SubnetId(), other.ec2SubnetId())
&& hasRequestedEc2SubnetIds() == other.hasRequestedEc2SubnetIds()
&& Objects.equals(requestedEc2SubnetIds(), other.requestedEc2SubnetIds())
&& Objects.equals(ec2AvailabilityZone(), other.ec2AvailabilityZone())
&& hasRequestedEc2AvailabilityZones() == other.hasRequestedEc2AvailabilityZones()
&& Objects.equals(requestedEc2AvailabilityZones(), other.requestedEc2AvailabilityZones())
&& Objects.equals(iamInstanceProfile(), other.iamInstanceProfile())
&& Objects.equals(emrManagedMasterSecurityGroup(), other.emrManagedMasterSecurityGroup())
&& Objects.equals(emrManagedSlaveSecurityGroup(), other.emrManagedSlaveSecurityGroup())
&& Objects.equals(serviceAccessSecurityGroup(), other.serviceAccessSecurityGroup())
&& hasAdditionalMasterSecurityGroups() == other.hasAdditionalMasterSecurityGroups()
&& Objects.equals(additionalMasterSecurityGroups(), other.additionalMasterSecurityGroups())
&& hasAdditionalSlaveSecurityGroups() == other.hasAdditionalSlaveSecurityGroups()
&& Objects.equals(additionalSlaveSecurityGroups(), other.additionalSlaveSecurityGroups());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString
.builder("Ec2InstanceAttributes")
.add("Ec2KeyName", ec2KeyName())
.add("Ec2SubnetId", ec2SubnetId())
.add("RequestedEc2SubnetIds", hasRequestedEc2SubnetIds() ? requestedEc2SubnetIds() : null)
.add("Ec2AvailabilityZone", ec2AvailabilityZone())
.add("RequestedEc2AvailabilityZones", hasRequestedEc2AvailabilityZones() ? requestedEc2AvailabilityZones() : null)
.add("IamInstanceProfile", iamInstanceProfile())
.add("EmrManagedMasterSecurityGroup", emrManagedMasterSecurityGroup())
.add("EmrManagedSlaveSecurityGroup", emrManagedSlaveSecurityGroup())
.add("ServiceAccessSecurityGroup", serviceAccessSecurityGroup())
.add("AdditionalMasterSecurityGroups",
hasAdditionalMasterSecurityGroups() ? additionalMasterSecurityGroups() : null)
.add("AdditionalSlaveSecurityGroups", hasAdditionalSlaveSecurityGroups() ? additionalSlaveSecurityGroups() : null)
.build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "Ec2KeyName":
return Optional.ofNullable(clazz.cast(ec2KeyName()));
case "Ec2SubnetId":
return Optional.ofNullable(clazz.cast(ec2SubnetId()));
case "RequestedEc2SubnetIds":
return Optional.ofNullable(clazz.cast(requestedEc2SubnetIds()));
case "Ec2AvailabilityZone":
return Optional.ofNullable(clazz.cast(ec2AvailabilityZone()));
case "RequestedEc2AvailabilityZones":
return Optional.ofNullable(clazz.cast(requestedEc2AvailabilityZones()));
case "IamInstanceProfile":
return Optional.ofNullable(clazz.cast(iamInstanceProfile()));
case "EmrManagedMasterSecurityGroup":
return Optional.ofNullable(clazz.cast(emrManagedMasterSecurityGroup()));
case "EmrManagedSlaveSecurityGroup":
return Optional.ofNullable(clazz.cast(emrManagedSlaveSecurityGroup()));
case "ServiceAccessSecurityGroup":
return Optional.ofNullable(clazz.cast(serviceAccessSecurityGroup()));
case "AdditionalMasterSecurityGroups":
return Optional.ofNullable(clazz.cast(additionalMasterSecurityGroups()));
case "AdditionalSlaveSecurityGroups":
return Optional.ofNullable(clazz.cast(additionalSlaveSecurityGroups()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
private static Function