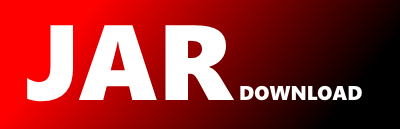
software.amazon.awssdk.services.emr.model.InstanceGroup Maven / Gradle / Ivy
Show all versions of emr Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.emr.model;
import java.io.Serializable;
import java.util.Arrays;
import java.util.Collection;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import java.util.stream.Collectors;
import java.util.stream.Stream;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.ListTrait;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.core.util.DefaultSdkAutoConstructList;
import software.amazon.awssdk.core.util.SdkAutoConstructList;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* This entity represents an instance group, which is a group of instances that have common purpose. For example, CORE
* instance group is used for HDFS.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class InstanceGroup implements SdkPojo, Serializable, ToCopyableBuilder {
private static final SdkField ID_FIELD = SdkField. builder(MarshallingType.STRING).memberName("Id")
.getter(getter(InstanceGroup::id)).setter(setter(Builder::id))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Id").build()).build();
private static final SdkField NAME_FIELD = SdkField. builder(MarshallingType.STRING).memberName("Name")
.getter(getter(InstanceGroup::name)).setter(setter(Builder::name))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Name").build()).build();
private static final SdkField MARKET_FIELD = SdkField. builder(MarshallingType.STRING).memberName("Market")
.getter(getter(InstanceGroup::marketAsString)).setter(setter(Builder::market))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Market").build()).build();
private static final SdkField INSTANCE_GROUP_TYPE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("InstanceGroupType").getter(getter(InstanceGroup::instanceGroupTypeAsString))
.setter(setter(Builder::instanceGroupType))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("InstanceGroupType").build()).build();
private static final SdkField BID_PRICE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("BidPrice").getter(getter(InstanceGroup::bidPrice)).setter(setter(Builder::bidPrice))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("BidPrice").build()).build();
private static final SdkField INSTANCE_TYPE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("InstanceType").getter(getter(InstanceGroup::instanceType)).setter(setter(Builder::instanceType))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("InstanceType").build()).build();
private static final SdkField REQUESTED_INSTANCE_COUNT_FIELD = SdkField. builder(MarshallingType.INTEGER)
.memberName("RequestedInstanceCount").getter(getter(InstanceGroup::requestedInstanceCount))
.setter(setter(Builder::requestedInstanceCount))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("RequestedInstanceCount").build())
.build();
private static final SdkField RUNNING_INSTANCE_COUNT_FIELD = SdkField. builder(MarshallingType.INTEGER)
.memberName("RunningInstanceCount").getter(getter(InstanceGroup::runningInstanceCount))
.setter(setter(Builder::runningInstanceCount))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("RunningInstanceCount").build())
.build();
private static final SdkField STATUS_FIELD = SdkField
. builder(MarshallingType.SDK_POJO).memberName("Status").getter(getter(InstanceGroup::status))
.setter(setter(Builder::status)).constructor(InstanceGroupStatus::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Status").build()).build();
private static final SdkField> CONFIGURATIONS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("Configurations")
.getter(getter(InstanceGroup::configurations))
.setter(setter(Builder::configurations))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Configurations").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(Configuration::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField CONFIGURATIONS_VERSION_FIELD = SdkField. builder(MarshallingType.LONG)
.memberName("ConfigurationsVersion").getter(getter(InstanceGroup::configurationsVersion))
.setter(setter(Builder::configurationsVersion))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ConfigurationsVersion").build())
.build();
private static final SdkField> LAST_SUCCESSFULLY_APPLIED_CONFIGURATIONS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("LastSuccessfullyAppliedConfigurations")
.getter(getter(InstanceGroup::lastSuccessfullyAppliedConfigurations))
.setter(setter(Builder::lastSuccessfullyAppliedConfigurations))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("LastSuccessfullyAppliedConfigurations").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(Configuration::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField LAST_SUCCESSFULLY_APPLIED_CONFIGURATIONS_VERSION_FIELD = SdkField
. builder(MarshallingType.LONG)
.memberName("LastSuccessfullyAppliedConfigurationsVersion")
.getter(getter(InstanceGroup::lastSuccessfullyAppliedConfigurationsVersion))
.setter(setter(Builder::lastSuccessfullyAppliedConfigurationsVersion))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("LastSuccessfullyAppliedConfigurationsVersion").build()).build();
private static final SdkField> EBS_BLOCK_DEVICES_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("EbsBlockDevices")
.getter(getter(InstanceGroup::ebsBlockDevices))
.setter(setter(Builder::ebsBlockDevices))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("EbsBlockDevices").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(EbsBlockDevice::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField EBS_OPTIMIZED_FIELD = SdkField. builder(MarshallingType.BOOLEAN)
.memberName("EbsOptimized").getter(getter(InstanceGroup::ebsOptimized)).setter(setter(Builder::ebsOptimized))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("EbsOptimized").build()).build();
private static final SdkField SHRINK_POLICY_FIELD = SdkField. builder(MarshallingType.SDK_POJO)
.memberName("ShrinkPolicy").getter(getter(InstanceGroup::shrinkPolicy)).setter(setter(Builder::shrinkPolicy))
.constructor(ShrinkPolicy::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ShrinkPolicy").build()).build();
private static final SdkField AUTO_SCALING_POLICY_FIELD = SdkField
. builder(MarshallingType.SDK_POJO).memberName("AutoScalingPolicy")
.getter(getter(InstanceGroup::autoScalingPolicy)).setter(setter(Builder::autoScalingPolicy))
.constructor(AutoScalingPolicyDescription::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("AutoScalingPolicy").build()).build();
private static final SdkField CUSTOM_AMI_ID_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("CustomAmiId").getter(getter(InstanceGroup::customAmiId)).setter(setter(Builder::customAmiId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("CustomAmiId").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(ID_FIELD, NAME_FIELD,
MARKET_FIELD, INSTANCE_GROUP_TYPE_FIELD, BID_PRICE_FIELD, INSTANCE_TYPE_FIELD, REQUESTED_INSTANCE_COUNT_FIELD,
RUNNING_INSTANCE_COUNT_FIELD, STATUS_FIELD, CONFIGURATIONS_FIELD, CONFIGURATIONS_VERSION_FIELD,
LAST_SUCCESSFULLY_APPLIED_CONFIGURATIONS_FIELD, LAST_SUCCESSFULLY_APPLIED_CONFIGURATIONS_VERSION_FIELD,
EBS_BLOCK_DEVICES_FIELD, EBS_OPTIMIZED_FIELD, SHRINK_POLICY_FIELD, AUTO_SCALING_POLICY_FIELD, CUSTOM_AMI_ID_FIELD));
private static final long serialVersionUID = 1L;
private final String id;
private final String name;
private final String market;
private final String instanceGroupType;
private final String bidPrice;
private final String instanceType;
private final Integer requestedInstanceCount;
private final Integer runningInstanceCount;
private final InstanceGroupStatus status;
private final List configurations;
private final Long configurationsVersion;
private final List lastSuccessfullyAppliedConfigurations;
private final Long lastSuccessfullyAppliedConfigurationsVersion;
private final List ebsBlockDevices;
private final Boolean ebsOptimized;
private final ShrinkPolicy shrinkPolicy;
private final AutoScalingPolicyDescription autoScalingPolicy;
private final String customAmiId;
private InstanceGroup(BuilderImpl builder) {
this.id = builder.id;
this.name = builder.name;
this.market = builder.market;
this.instanceGroupType = builder.instanceGroupType;
this.bidPrice = builder.bidPrice;
this.instanceType = builder.instanceType;
this.requestedInstanceCount = builder.requestedInstanceCount;
this.runningInstanceCount = builder.runningInstanceCount;
this.status = builder.status;
this.configurations = builder.configurations;
this.configurationsVersion = builder.configurationsVersion;
this.lastSuccessfullyAppliedConfigurations = builder.lastSuccessfullyAppliedConfigurations;
this.lastSuccessfullyAppliedConfigurationsVersion = builder.lastSuccessfullyAppliedConfigurationsVersion;
this.ebsBlockDevices = builder.ebsBlockDevices;
this.ebsOptimized = builder.ebsOptimized;
this.shrinkPolicy = builder.shrinkPolicy;
this.autoScalingPolicy = builder.autoScalingPolicy;
this.customAmiId = builder.customAmiId;
}
/**
*
* The identifier of the instance group.
*
*
* @return The identifier of the instance group.
*/
public final String id() {
return id;
}
/**
*
* The name of the instance group.
*
*
* @return The name of the instance group.
*/
public final String name() {
return name;
}
/**
*
* The marketplace to provision instances for this group. Valid values are ON_DEMAND or SPOT.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #market} will
* return {@link MarketType#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #marketAsString}.
*
*
* @return The marketplace to provision instances for this group. Valid values are ON_DEMAND or SPOT.
* @see MarketType
*/
public final MarketType market() {
return MarketType.fromValue(market);
}
/**
*
* The marketplace to provision instances for this group. Valid values are ON_DEMAND or SPOT.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #market} will
* return {@link MarketType#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #marketAsString}.
*
*
* @return The marketplace to provision instances for this group. Valid values are ON_DEMAND or SPOT.
* @see MarketType
*/
public final String marketAsString() {
return market;
}
/**
*
* The type of the instance group. Valid values are MASTER, CORE or TASK.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #instanceGroupType}
* will return {@link InstanceGroupType#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available
* from {@link #instanceGroupTypeAsString}.
*
*
* @return The type of the instance group. Valid values are MASTER, CORE or TASK.
* @see InstanceGroupType
*/
public final InstanceGroupType instanceGroupType() {
return InstanceGroupType.fromValue(instanceGroupType);
}
/**
*
* The type of the instance group. Valid values are MASTER, CORE or TASK.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #instanceGroupType}
* will return {@link InstanceGroupType#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available
* from {@link #instanceGroupTypeAsString}.
*
*
* @return The type of the instance group. Valid values are MASTER, CORE or TASK.
* @see InstanceGroupType
*/
public final String instanceGroupTypeAsString() {
return instanceGroupType;
}
/**
*
* If specified, indicates that the instance group uses Spot Instances. This is the maximum price you are willing to
* pay for Spot Instances. Specify OnDemandPrice
to set the amount equal to the On-Demand price, or
* specify an amount in USD.
*
*
* @return If specified, indicates that the instance group uses Spot Instances. This is the maximum price you are
* willing to pay for Spot Instances. Specify OnDemandPrice
to set the amount equal to the
* On-Demand price, or specify an amount in USD.
*/
public final String bidPrice() {
return bidPrice;
}
/**
*
* The Amazon EC2 instance type for all instances in the instance group.
*
*
* @return The Amazon EC2 instance type for all instances in the instance group.
*/
public final String instanceType() {
return instanceType;
}
/**
*
* The target number of instances for the instance group.
*
*
* @return The target number of instances for the instance group.
*/
public final Integer requestedInstanceCount() {
return requestedInstanceCount;
}
/**
*
* The number of instances currently running in this instance group.
*
*
* @return The number of instances currently running in this instance group.
*/
public final Integer runningInstanceCount() {
return runningInstanceCount;
}
/**
*
* The current status of the instance group.
*
*
* @return The current status of the instance group.
*/
public final InstanceGroupStatus status() {
return status;
}
/**
* For responses, this returns true if the service returned a value for the Configurations property. This DOES NOT
* check that the value is non-empty (for which, you should check the {@code isEmpty()} method on the property).
* This is useful because the SDK will never return a null collection or map, but you may need to differentiate
* between the service returning nothing (or null) and the service returning an empty collection or map. For
* requests, this returns true if a value for the property was specified in the request builder, and false if a
* value was not specified.
*/
public final boolean hasConfigurations() {
return configurations != null && !(configurations instanceof SdkAutoConstructList);
}
/**
*
*
* Amazon EMR releases 4.x or later.
*
*
*
* The list of configurations supplied for an Amazon EMR cluster instance group. You can specify a separate
* configuration for each instance group (master, core, and task).
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasConfigurations} method.
*
*
* @return
* Amazon EMR releases 4.x or later.
*
*
*
* The list of configurations supplied for an Amazon EMR cluster instance group. You can specify a separate
* configuration for each instance group (master, core, and task).
*/
public final List configurations() {
return configurations;
}
/**
*
* The version number of the requested configuration specification for this instance group.
*
*
* @return The version number of the requested configuration specification for this instance group.
*/
public final Long configurationsVersion() {
return configurationsVersion;
}
/**
* For responses, this returns true if the service returned a value for the LastSuccessfullyAppliedConfigurations
* property. This DOES NOT check that the value is non-empty (for which, you should check the {@code isEmpty()}
* method on the property). This is useful because the SDK will never return a null collection or map, but you may
* need to differentiate between the service returning nothing (or null) and the service returning an empty
* collection or map. For requests, this returns true if a value for the property was specified in the request
* builder, and false if a value was not specified.
*/
public final boolean hasLastSuccessfullyAppliedConfigurations() {
return lastSuccessfullyAppliedConfigurations != null
&& !(lastSuccessfullyAppliedConfigurations instanceof SdkAutoConstructList);
}
/**
*
* A list of configurations that were successfully applied for an instance group last time.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasLastSuccessfullyAppliedConfigurations}
* method.
*
*
* @return A list of configurations that were successfully applied for an instance group last time.
*/
public final List lastSuccessfullyAppliedConfigurations() {
return lastSuccessfullyAppliedConfigurations;
}
/**
*
* The version number of a configuration specification that was successfully applied for an instance group last
* time.
*
*
* @return The version number of a configuration specification that was successfully applied for an instance group
* last time.
*/
public final Long lastSuccessfullyAppliedConfigurationsVersion() {
return lastSuccessfullyAppliedConfigurationsVersion;
}
/**
* For responses, this returns true if the service returned a value for the EbsBlockDevices property. This DOES NOT
* check that the value is non-empty (for which, you should check the {@code isEmpty()} method on the property).
* This is useful because the SDK will never return a null collection or map, but you may need to differentiate
* between the service returning nothing (or null) and the service returning an empty collection or map. For
* requests, this returns true if a value for the property was specified in the request builder, and false if a
* value was not specified.
*/
public final boolean hasEbsBlockDevices() {
return ebsBlockDevices != null && !(ebsBlockDevices instanceof SdkAutoConstructList);
}
/**
*
* The EBS block devices that are mapped to this instance group.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasEbsBlockDevices} method.
*
*
* @return The EBS block devices that are mapped to this instance group.
*/
public final List ebsBlockDevices() {
return ebsBlockDevices;
}
/**
*
* If the instance group is EBS-optimized. An Amazon EBS-optimized instance uses an optimized configuration stack
* and provides additional, dedicated capacity for Amazon EBS I/O.
*
*
* @return If the instance group is EBS-optimized. An Amazon EBS-optimized instance uses an optimized configuration
* stack and provides additional, dedicated capacity for Amazon EBS I/O.
*/
public final Boolean ebsOptimized() {
return ebsOptimized;
}
/**
*
* Policy for customizing shrink operations.
*
*
* @return Policy for customizing shrink operations.
*/
public final ShrinkPolicy shrinkPolicy() {
return shrinkPolicy;
}
/**
*
* An automatic scaling policy for a core instance group or task instance group in an Amazon EMR cluster. The
* automatic scaling policy defines how an instance group dynamically adds and terminates Amazon EC2 instances in
* response to the value of a CloudWatch metric. See PutAutoScalingPolicy.
*
*
* @return An automatic scaling policy for a core instance group or task instance group in an Amazon EMR cluster.
* The automatic scaling policy defines how an instance group dynamically adds and terminates Amazon EC2
* instances in response to the value of a CloudWatch metric. See PutAutoScalingPolicy.
*/
public final AutoScalingPolicyDescription autoScalingPolicy() {
return autoScalingPolicy;
}
/**
*
* The custom AMI ID to use for the provisioned instance group.
*
*
* @return The custom AMI ID to use for the provisioned instance group.
*/
public final String customAmiId() {
return customAmiId;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(id());
hashCode = 31 * hashCode + Objects.hashCode(name());
hashCode = 31 * hashCode + Objects.hashCode(marketAsString());
hashCode = 31 * hashCode + Objects.hashCode(instanceGroupTypeAsString());
hashCode = 31 * hashCode + Objects.hashCode(bidPrice());
hashCode = 31 * hashCode + Objects.hashCode(instanceType());
hashCode = 31 * hashCode + Objects.hashCode(requestedInstanceCount());
hashCode = 31 * hashCode + Objects.hashCode(runningInstanceCount());
hashCode = 31 * hashCode + Objects.hashCode(status());
hashCode = 31 * hashCode + Objects.hashCode(hasConfigurations() ? configurations() : null);
hashCode = 31 * hashCode + Objects.hashCode(configurationsVersion());
hashCode = 31 * hashCode
+ Objects.hashCode(hasLastSuccessfullyAppliedConfigurations() ? lastSuccessfullyAppliedConfigurations() : null);
hashCode = 31 * hashCode + Objects.hashCode(lastSuccessfullyAppliedConfigurationsVersion());
hashCode = 31 * hashCode + Objects.hashCode(hasEbsBlockDevices() ? ebsBlockDevices() : null);
hashCode = 31 * hashCode + Objects.hashCode(ebsOptimized());
hashCode = 31 * hashCode + Objects.hashCode(shrinkPolicy());
hashCode = 31 * hashCode + Objects.hashCode(autoScalingPolicy());
hashCode = 31 * hashCode + Objects.hashCode(customAmiId());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof InstanceGroup)) {
return false;
}
InstanceGroup other = (InstanceGroup) obj;
return Objects.equals(id(), other.id())
&& Objects.equals(name(), other.name())
&& Objects.equals(marketAsString(), other.marketAsString())
&& Objects.equals(instanceGroupTypeAsString(), other.instanceGroupTypeAsString())
&& Objects.equals(bidPrice(), other.bidPrice())
&& Objects.equals(instanceType(), other.instanceType())
&& Objects.equals(requestedInstanceCount(), other.requestedInstanceCount())
&& Objects.equals(runningInstanceCount(), other.runningInstanceCount())
&& Objects.equals(status(), other.status())
&& hasConfigurations() == other.hasConfigurations()
&& Objects.equals(configurations(), other.configurations())
&& Objects.equals(configurationsVersion(), other.configurationsVersion())
&& hasLastSuccessfullyAppliedConfigurations() == other.hasLastSuccessfullyAppliedConfigurations()
&& Objects.equals(lastSuccessfullyAppliedConfigurations(), other.lastSuccessfullyAppliedConfigurations())
&& Objects.equals(lastSuccessfullyAppliedConfigurationsVersion(),
other.lastSuccessfullyAppliedConfigurationsVersion())
&& hasEbsBlockDevices() == other.hasEbsBlockDevices()
&& Objects.equals(ebsBlockDevices(), other.ebsBlockDevices())
&& Objects.equals(ebsOptimized(), other.ebsOptimized()) && Objects.equals(shrinkPolicy(), other.shrinkPolicy())
&& Objects.equals(autoScalingPolicy(), other.autoScalingPolicy())
&& Objects.equals(customAmiId(), other.customAmiId());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString
.builder("InstanceGroup")
.add("Id", id())
.add("Name", name())
.add("Market", marketAsString())
.add("InstanceGroupType", instanceGroupTypeAsString())
.add("BidPrice", bidPrice())
.add("InstanceType", instanceType())
.add("RequestedInstanceCount", requestedInstanceCount())
.add("RunningInstanceCount", runningInstanceCount())
.add("Status", status())
.add("Configurations", hasConfigurations() ? configurations() : null)
.add("ConfigurationsVersion", configurationsVersion())
.add("LastSuccessfullyAppliedConfigurations",
hasLastSuccessfullyAppliedConfigurations() ? lastSuccessfullyAppliedConfigurations() : null)
.add("LastSuccessfullyAppliedConfigurationsVersion", lastSuccessfullyAppliedConfigurationsVersion())
.add("EbsBlockDevices", hasEbsBlockDevices() ? ebsBlockDevices() : null).add("EbsOptimized", ebsOptimized())
.add("ShrinkPolicy", shrinkPolicy()).add("AutoScalingPolicy", autoScalingPolicy())
.add("CustomAmiId", customAmiId()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "Id":
return Optional.ofNullable(clazz.cast(id()));
case "Name":
return Optional.ofNullable(clazz.cast(name()));
case "Market":
return Optional.ofNullable(clazz.cast(marketAsString()));
case "InstanceGroupType":
return Optional.ofNullable(clazz.cast(instanceGroupTypeAsString()));
case "BidPrice":
return Optional.ofNullable(clazz.cast(bidPrice()));
case "InstanceType":
return Optional.ofNullable(clazz.cast(instanceType()));
case "RequestedInstanceCount":
return Optional.ofNullable(clazz.cast(requestedInstanceCount()));
case "RunningInstanceCount":
return Optional.ofNullable(clazz.cast(runningInstanceCount()));
case "Status":
return Optional.ofNullable(clazz.cast(status()));
case "Configurations":
return Optional.ofNullable(clazz.cast(configurations()));
case "ConfigurationsVersion":
return Optional.ofNullable(clazz.cast(configurationsVersion()));
case "LastSuccessfullyAppliedConfigurations":
return Optional.ofNullable(clazz.cast(lastSuccessfullyAppliedConfigurations()));
case "LastSuccessfullyAppliedConfigurationsVersion":
return Optional.ofNullable(clazz.cast(lastSuccessfullyAppliedConfigurationsVersion()));
case "EbsBlockDevices":
return Optional.ofNullable(clazz.cast(ebsBlockDevices()));
case "EbsOptimized":
return Optional.ofNullable(clazz.cast(ebsOptimized()));
case "ShrinkPolicy":
return Optional.ofNullable(clazz.cast(shrinkPolicy()));
case "AutoScalingPolicy":
return Optional.ofNullable(clazz.cast(autoScalingPolicy()));
case "CustomAmiId":
return Optional.ofNullable(clazz.cast(customAmiId()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
private static Function