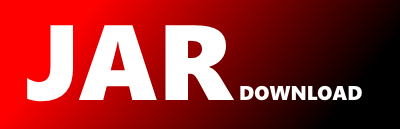
software.amazon.awssdk.services.emr.model.NotebookExecutionSummary Maven / Gradle / Ivy
Show all versions of emr Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.emr.model;
import java.io.Serializable;
import java.time.Instant;
import java.util.Arrays;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* Details for a notebook execution. The details include information such as the unique ID and status of the notebook
* execution.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class NotebookExecutionSummary implements SdkPojo, Serializable,
ToCopyableBuilder {
private static final SdkField NOTEBOOK_EXECUTION_ID_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("NotebookExecutionId").getter(getter(NotebookExecutionSummary::notebookExecutionId))
.setter(setter(Builder::notebookExecutionId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("NotebookExecutionId").build())
.build();
private static final SdkField EDITOR_ID_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("EditorId").getter(getter(NotebookExecutionSummary::editorId)).setter(setter(Builder::editorId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("EditorId").build()).build();
private static final SdkField NOTEBOOK_EXECUTION_NAME_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("NotebookExecutionName").getter(getter(NotebookExecutionSummary::notebookExecutionName))
.setter(setter(Builder::notebookExecutionName))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("NotebookExecutionName").build())
.build();
private static final SdkField STATUS_FIELD = SdkField. builder(MarshallingType.STRING).memberName("Status")
.getter(getter(NotebookExecutionSummary::statusAsString)).setter(setter(Builder::status))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Status").build()).build();
private static final SdkField START_TIME_FIELD = SdkField. builder(MarshallingType.INSTANT)
.memberName("StartTime").getter(getter(NotebookExecutionSummary::startTime)).setter(setter(Builder::startTime))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("StartTime").build()).build();
private static final SdkField END_TIME_FIELD = SdkField. builder(MarshallingType.INSTANT)
.memberName("EndTime").getter(getter(NotebookExecutionSummary::endTime)).setter(setter(Builder::endTime))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("EndTime").build()).build();
private static final SdkField NOTEBOOK_S3_LOCATION_FIELD = SdkField
. builder(MarshallingType.SDK_POJO).memberName("NotebookS3Location")
.getter(getter(NotebookExecutionSummary::notebookS3Location)).setter(setter(Builder::notebookS3Location))
.constructor(NotebookS3LocationForOutput::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("NotebookS3Location").build())
.build();
private static final SdkField EXECUTION_ENGINE_ID_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("ExecutionEngineId").getter(getter(NotebookExecutionSummary::executionEngineId))
.setter(setter(Builder::executionEngineId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ExecutionEngineId").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(NOTEBOOK_EXECUTION_ID_FIELD,
EDITOR_ID_FIELD, NOTEBOOK_EXECUTION_NAME_FIELD, STATUS_FIELD, START_TIME_FIELD, END_TIME_FIELD,
NOTEBOOK_S3_LOCATION_FIELD, EXECUTION_ENGINE_ID_FIELD));
private static final long serialVersionUID = 1L;
private final String notebookExecutionId;
private final String editorId;
private final String notebookExecutionName;
private final String status;
private final Instant startTime;
private final Instant endTime;
private final NotebookS3LocationForOutput notebookS3Location;
private final String executionEngineId;
private NotebookExecutionSummary(BuilderImpl builder) {
this.notebookExecutionId = builder.notebookExecutionId;
this.editorId = builder.editorId;
this.notebookExecutionName = builder.notebookExecutionName;
this.status = builder.status;
this.startTime = builder.startTime;
this.endTime = builder.endTime;
this.notebookS3Location = builder.notebookS3Location;
this.executionEngineId = builder.executionEngineId;
}
/**
*
* The unique identifier of the notebook execution.
*
*
* @return The unique identifier of the notebook execution.
*/
public final String notebookExecutionId() {
return notebookExecutionId;
}
/**
*
* The unique identifier of the editor associated with the notebook execution.
*
*
* @return The unique identifier of the editor associated with the notebook execution.
*/
public final String editorId() {
return editorId;
}
/**
*
* The name of the notebook execution.
*
*
* @return The name of the notebook execution.
*/
public final String notebookExecutionName() {
return notebookExecutionName;
}
/**
*
* The status of the notebook execution.
*
*
* -
*
* START_PENDING
indicates that the cluster has received the execution request but execution has not
* begun.
*
*
* -
*
* STARTING
indicates that the execution is starting on the cluster.
*
*
* -
*
* RUNNING
indicates that the execution is being processed by the cluster.
*
*
* -
*
* FINISHING
indicates that execution processing is in the final stages.
*
*
* -
*
* FINISHED
indicates that the execution has completed without error.
*
*
* -
*
* FAILING
indicates that the execution is failing and will not finish successfully.
*
*
* -
*
* FAILED
indicates that the execution failed.
*
*
* -
*
* STOP_PENDING
indicates that the cluster has received a StopNotebookExecution
request
* and the stop is pending.
*
*
* -
*
* STOPPING
indicates that the cluster is in the process of stopping the execution as a result of a
* StopNotebookExecution
request.
*
*
* -
*
* STOPPED
indicates that the execution stopped because of a StopNotebookExecution
* request.
*
*
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #status} will
* return {@link NotebookExecutionStatus#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available
* from {@link #statusAsString}.
*
*
* @return The status of the notebook execution.
*
* -
*
* START_PENDING
indicates that the cluster has received the execution request but execution
* has not begun.
*
*
* -
*
* STARTING
indicates that the execution is starting on the cluster.
*
*
* -
*
* RUNNING
indicates that the execution is being processed by the cluster.
*
*
* -
*
* FINISHING
indicates that execution processing is in the final stages.
*
*
* -
*
* FINISHED
indicates that the execution has completed without error.
*
*
* -
*
* FAILING
indicates that the execution is failing and will not finish successfully.
*
*
* -
*
* FAILED
indicates that the execution failed.
*
*
* -
*
* STOP_PENDING
indicates that the cluster has received a StopNotebookExecution
* request and the stop is pending.
*
*
* -
*
* STOPPING
indicates that the cluster is in the process of stopping the execution as a result
* of a StopNotebookExecution
request.
*
*
* -
*
* STOPPED
indicates that the execution stopped because of a StopNotebookExecution
* request.
*
*
* @see NotebookExecutionStatus
*/
public final NotebookExecutionStatus status() {
return NotebookExecutionStatus.fromValue(status);
}
/**
*
* The status of the notebook execution.
*
*
* -
*
* START_PENDING
indicates that the cluster has received the execution request but execution has not
* begun.
*
*
* -
*
* STARTING
indicates that the execution is starting on the cluster.
*
*
* -
*
* RUNNING
indicates that the execution is being processed by the cluster.
*
*
* -
*
* FINISHING
indicates that execution processing is in the final stages.
*
*
* -
*
* FINISHED
indicates that the execution has completed without error.
*
*
* -
*
* FAILING
indicates that the execution is failing and will not finish successfully.
*
*
* -
*
* FAILED
indicates that the execution failed.
*
*
* -
*
* STOP_PENDING
indicates that the cluster has received a StopNotebookExecution
request
* and the stop is pending.
*
*
* -
*
* STOPPING
indicates that the cluster is in the process of stopping the execution as a result of a
* StopNotebookExecution
request.
*
*
* -
*
* STOPPED
indicates that the execution stopped because of a StopNotebookExecution
* request.
*
*
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #status} will
* return {@link NotebookExecutionStatus#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available
* from {@link #statusAsString}.
*
*
* @return The status of the notebook execution.
*
* -
*
* START_PENDING
indicates that the cluster has received the execution request but execution
* has not begun.
*
*
* -
*
* STARTING
indicates that the execution is starting on the cluster.
*
*
* -
*
* RUNNING
indicates that the execution is being processed by the cluster.
*
*
* -
*
* FINISHING
indicates that execution processing is in the final stages.
*
*
* -
*
* FINISHED
indicates that the execution has completed without error.
*
*
* -
*
* FAILING
indicates that the execution is failing and will not finish successfully.
*
*
* -
*
* FAILED
indicates that the execution failed.
*
*
* -
*
* STOP_PENDING
indicates that the cluster has received a StopNotebookExecution
* request and the stop is pending.
*
*
* -
*
* STOPPING
indicates that the cluster is in the process of stopping the execution as a result
* of a StopNotebookExecution
request.
*
*
* -
*
* STOPPED
indicates that the execution stopped because of a StopNotebookExecution
* request.
*
*
* @see NotebookExecutionStatus
*/
public final String statusAsString() {
return status;
}
/**
*
* The timestamp when notebook execution started.
*
*
* @return The timestamp when notebook execution started.
*/
public final Instant startTime() {
return startTime;
}
/**
*
* The timestamp when notebook execution started.
*
*
* @return The timestamp when notebook execution started.
*/
public final Instant endTime() {
return endTime;
}
/**
*
* The Amazon S3 location that stores the notebook execution input.
*
*
* @return The Amazon S3 location that stores the notebook execution input.
*/
public final NotebookS3LocationForOutput notebookS3Location() {
return notebookS3Location;
}
/**
*
* The unique ID of the execution engine for the notebook execution.
*
*
* @return The unique ID of the execution engine for the notebook execution.
*/
public final String executionEngineId() {
return executionEngineId;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(notebookExecutionId());
hashCode = 31 * hashCode + Objects.hashCode(editorId());
hashCode = 31 * hashCode + Objects.hashCode(notebookExecutionName());
hashCode = 31 * hashCode + Objects.hashCode(statusAsString());
hashCode = 31 * hashCode + Objects.hashCode(startTime());
hashCode = 31 * hashCode + Objects.hashCode(endTime());
hashCode = 31 * hashCode + Objects.hashCode(notebookS3Location());
hashCode = 31 * hashCode + Objects.hashCode(executionEngineId());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof NotebookExecutionSummary)) {
return false;
}
NotebookExecutionSummary other = (NotebookExecutionSummary) obj;
return Objects.equals(notebookExecutionId(), other.notebookExecutionId()) && Objects.equals(editorId(), other.editorId())
&& Objects.equals(notebookExecutionName(), other.notebookExecutionName())
&& Objects.equals(statusAsString(), other.statusAsString()) && Objects.equals(startTime(), other.startTime())
&& Objects.equals(endTime(), other.endTime()) && Objects.equals(notebookS3Location(), other.notebookS3Location())
&& Objects.equals(executionEngineId(), other.executionEngineId());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("NotebookExecutionSummary").add("NotebookExecutionId", notebookExecutionId())
.add("EditorId", editorId()).add("NotebookExecutionName", notebookExecutionName())
.add("Status", statusAsString()).add("StartTime", startTime()).add("EndTime", endTime())
.add("NotebookS3Location", notebookS3Location()).add("ExecutionEngineId", executionEngineId()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "NotebookExecutionId":
return Optional.ofNullable(clazz.cast(notebookExecutionId()));
case "EditorId":
return Optional.ofNullable(clazz.cast(editorId()));
case "NotebookExecutionName":
return Optional.ofNullable(clazz.cast(notebookExecutionName()));
case "Status":
return Optional.ofNullable(clazz.cast(statusAsString()));
case "StartTime":
return Optional.ofNullable(clazz.cast(startTime()));
case "EndTime":
return Optional.ofNullable(clazz.cast(endTime()));
case "NotebookS3Location":
return Optional.ofNullable(clazz.cast(notebookS3Location()));
case "ExecutionEngineId":
return Optional.ofNullable(clazz.cast(executionEngineId()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
private static Function