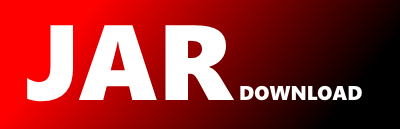
software.amazon.awssdk.services.emr.model.SpotProvisioningSpecification Maven / Gradle / Ivy
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.emr.model;
import java.io.Serializable;
import java.util.Arrays;
import java.util.Collections;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* The launch specification for Spot Instances in the instance fleet, which determines the defined duration,
* provisioning timeout behavior, and allocation strategy.
*
*
*
* The instance fleet configuration is available only in Amazon EMR releases 4.8.0 and later, excluding 5.0.x versions.
* Spot Instance allocation strategy is available in Amazon EMR releases 5.12.1 and later.
*
*
*
* Spot Instances with a defined duration (also known as Spot blocks) are no longer available to new customers from July
* 1, 2021. For customers who have previously used the feature, we will continue to support Spot Instances with a
* defined duration until December 31, 2022.
*
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class SpotProvisioningSpecification implements SdkPojo, Serializable,
ToCopyableBuilder {
private static final SdkField TIMEOUT_DURATION_MINUTES_FIELD = SdkField. builder(MarshallingType.INTEGER)
.memberName("TimeoutDurationMinutes").getter(getter(SpotProvisioningSpecification::timeoutDurationMinutes))
.setter(setter(Builder::timeoutDurationMinutes))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("TimeoutDurationMinutes").build())
.build();
private static final SdkField TIMEOUT_ACTION_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("TimeoutAction").getter(getter(SpotProvisioningSpecification::timeoutActionAsString))
.setter(setter(Builder::timeoutAction))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("TimeoutAction").build()).build();
private static final SdkField BLOCK_DURATION_MINUTES_FIELD = SdkField. builder(MarshallingType.INTEGER)
.memberName("BlockDurationMinutes").getter(getter(SpotProvisioningSpecification::blockDurationMinutes))
.setter(setter(Builder::blockDurationMinutes))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("BlockDurationMinutes").build())
.build();
private static final SdkField ALLOCATION_STRATEGY_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("AllocationStrategy").getter(getter(SpotProvisioningSpecification::allocationStrategyAsString))
.setter(setter(Builder::allocationStrategy))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("AllocationStrategy").build())
.build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(
TIMEOUT_DURATION_MINUTES_FIELD, TIMEOUT_ACTION_FIELD, BLOCK_DURATION_MINUTES_FIELD, ALLOCATION_STRATEGY_FIELD));
private static final Map> SDK_NAME_TO_FIELD = Collections
.unmodifiableMap(new HashMap>() {
{
put("TimeoutDurationMinutes", TIMEOUT_DURATION_MINUTES_FIELD);
put("TimeoutAction", TIMEOUT_ACTION_FIELD);
put("BlockDurationMinutes", BLOCK_DURATION_MINUTES_FIELD);
put("AllocationStrategy", ALLOCATION_STRATEGY_FIELD);
}
});
private static final long serialVersionUID = 1L;
private final Integer timeoutDurationMinutes;
private final String timeoutAction;
private final Integer blockDurationMinutes;
private final String allocationStrategy;
private SpotProvisioningSpecification(BuilderImpl builder) {
this.timeoutDurationMinutes = builder.timeoutDurationMinutes;
this.timeoutAction = builder.timeoutAction;
this.blockDurationMinutes = builder.blockDurationMinutes;
this.allocationStrategy = builder.allocationStrategy;
}
/**
*
* The Spot provisioning timeout period in minutes. If Spot Instances are not provisioned within this time period,
* the TimeOutAction
is taken. Minimum value is 5 and maximum value is 1440. The timeout applies only
* during initial provisioning, when the cluster is first created.
*
*
* @return The Spot provisioning timeout period in minutes. If Spot Instances are not provisioned within this time
* period, the TimeOutAction
is taken. Minimum value is 5 and maximum value is 1440. The
* timeout applies only during initial provisioning, when the cluster is first created.
*/
public final Integer timeoutDurationMinutes() {
return timeoutDurationMinutes;
}
/**
*
* The action to take when TargetSpotCapacity
has not been fulfilled when the
* TimeoutDurationMinutes
has expired; that is, when all Spot Instances could not be provisioned within
* the Spot provisioning timeout. Valid values are TERMINATE_CLUSTER
and
* SWITCH_TO_ON_DEMAND
. SWITCH_TO_ON_DEMAND specifies that if no Spot Instances are available,
* On-Demand Instances should be provisioned to fulfill any remaining Spot capacity.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #timeoutAction}
* will return {@link SpotProvisioningTimeoutAction#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service
* is available from {@link #timeoutActionAsString}.
*
*
* @return The action to take when TargetSpotCapacity
has not been fulfilled when the
* TimeoutDurationMinutes
has expired; that is, when all Spot Instances could not be
* provisioned within the Spot provisioning timeout. Valid values are TERMINATE_CLUSTER
and
* SWITCH_TO_ON_DEMAND
. SWITCH_TO_ON_DEMAND specifies that if no Spot Instances are available,
* On-Demand Instances should be provisioned to fulfill any remaining Spot capacity.
* @see SpotProvisioningTimeoutAction
*/
public final SpotProvisioningTimeoutAction timeoutAction() {
return SpotProvisioningTimeoutAction.fromValue(timeoutAction);
}
/**
*
* The action to take when TargetSpotCapacity
has not been fulfilled when the
* TimeoutDurationMinutes
has expired; that is, when all Spot Instances could not be provisioned within
* the Spot provisioning timeout. Valid values are TERMINATE_CLUSTER
and
* SWITCH_TO_ON_DEMAND
. SWITCH_TO_ON_DEMAND specifies that if no Spot Instances are available,
* On-Demand Instances should be provisioned to fulfill any remaining Spot capacity.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #timeoutAction}
* will return {@link SpotProvisioningTimeoutAction#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service
* is available from {@link #timeoutActionAsString}.
*
*
* @return The action to take when TargetSpotCapacity
has not been fulfilled when the
* TimeoutDurationMinutes
has expired; that is, when all Spot Instances could not be
* provisioned within the Spot provisioning timeout. Valid values are TERMINATE_CLUSTER
and
* SWITCH_TO_ON_DEMAND
. SWITCH_TO_ON_DEMAND specifies that if no Spot Instances are available,
* On-Demand Instances should be provisioned to fulfill any remaining Spot capacity.
* @see SpotProvisioningTimeoutAction
*/
public final String timeoutActionAsString() {
return timeoutAction;
}
/**
*
* The defined duration for Spot Instances (also known as Spot blocks) in minutes. When specified, the Spot Instance
* does not terminate before the defined duration expires, and defined duration pricing for Spot Instances applies.
* Valid values are 60, 120, 180, 240, 300, or 360. The duration period starts as soon as a Spot Instance receives
* its instance ID. At the end of the duration, Amazon EC2 marks the Spot Instance for termination and provides a
* Spot Instance termination notice, which gives the instance a two-minute warning before it terminates.
*
*
*
* Spot Instances with a defined duration (also known as Spot blocks) are no longer available to new customers from
* July 1, 2021. For customers who have previously used the feature, we will continue to support Spot Instances with
* a defined duration until December 31, 2022.
*
*
*
* @return The defined duration for Spot Instances (also known as Spot blocks) in minutes. When specified, the Spot
* Instance does not terminate before the defined duration expires, and defined duration pricing for Spot
* Instances applies. Valid values are 60, 120, 180, 240, 300, or 360. The duration period starts as soon as
* a Spot Instance receives its instance ID. At the end of the duration, Amazon EC2 marks the Spot Instance
* for termination and provides a Spot Instance termination notice, which gives the instance a two-minute
* warning before it terminates.
*
* Spot Instances with a defined duration (also known as Spot blocks) are no longer available to new
* customers from July 1, 2021. For customers who have previously used the feature, we will continue to
* support Spot Instances with a defined duration until December 31, 2022.
*
*/
public final Integer blockDurationMinutes() {
return blockDurationMinutes;
}
/**
*
* Specifies one of the following strategies to launch Spot Instance fleets: capacity-optimized
,
* price-capacity-optimized
, lowest-price
, or diversified
, and
* capacity-optimized-prioritized
. For more information on the provisioning strategies, see Allocation
* strategies for Spot Instances in the Amazon EC2 User Guide for Linux Instances.
*
*
*
* When you launch a Spot Instance fleet with the old console, it automatically launches with the
* capacity-optimized
strategy. You can't change the allocation strategy from the old console.
*
*
*
* If the service returns an enum value that is not available in the current SDK version,
* {@link #allocationStrategy} will return {@link SpotProvisioningAllocationStrategy#UNKNOWN_TO_SDK_VERSION}. The
* raw value returned by the service is available from {@link #allocationStrategyAsString}.
*
*
* @return Specifies one of the following strategies to launch Spot Instance fleets: capacity-optimized
* , price-capacity-optimized
, lowest-price
, or diversified
, and
* capacity-optimized-prioritized
. For more information on the provisioning strategies, see Allocation
* strategies for Spot Instances in the Amazon EC2 User Guide for Linux Instances.
*
* When you launch a Spot Instance fleet with the old console, it automatically launches with the
* capacity-optimized
strategy. You can't change the allocation strategy from the old console.
*
* @see SpotProvisioningAllocationStrategy
*/
public final SpotProvisioningAllocationStrategy allocationStrategy() {
return SpotProvisioningAllocationStrategy.fromValue(allocationStrategy);
}
/**
*
* Specifies one of the following strategies to launch Spot Instance fleets: capacity-optimized
,
* price-capacity-optimized
, lowest-price
, or diversified
, and
* capacity-optimized-prioritized
. For more information on the provisioning strategies, see Allocation
* strategies for Spot Instances in the Amazon EC2 User Guide for Linux Instances.
*
*
*
* When you launch a Spot Instance fleet with the old console, it automatically launches with the
* capacity-optimized
strategy. You can't change the allocation strategy from the old console.
*
*
*
* If the service returns an enum value that is not available in the current SDK version,
* {@link #allocationStrategy} will return {@link SpotProvisioningAllocationStrategy#UNKNOWN_TO_SDK_VERSION}. The
* raw value returned by the service is available from {@link #allocationStrategyAsString}.
*
*
* @return Specifies one of the following strategies to launch Spot Instance fleets: capacity-optimized
* , price-capacity-optimized
, lowest-price
, or diversified
, and
* capacity-optimized-prioritized
. For more information on the provisioning strategies, see Allocation
* strategies for Spot Instances in the Amazon EC2 User Guide for Linux Instances.
*
* When you launch a Spot Instance fleet with the old console, it automatically launches with the
* capacity-optimized
strategy. You can't change the allocation strategy from the old console.
*
* @see SpotProvisioningAllocationStrategy
*/
public final String allocationStrategyAsString() {
return allocationStrategy;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(timeoutDurationMinutes());
hashCode = 31 * hashCode + Objects.hashCode(timeoutActionAsString());
hashCode = 31 * hashCode + Objects.hashCode(blockDurationMinutes());
hashCode = 31 * hashCode + Objects.hashCode(allocationStrategyAsString());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof SpotProvisioningSpecification)) {
return false;
}
SpotProvisioningSpecification other = (SpotProvisioningSpecification) obj;
return Objects.equals(timeoutDurationMinutes(), other.timeoutDurationMinutes())
&& Objects.equals(timeoutActionAsString(), other.timeoutActionAsString())
&& Objects.equals(blockDurationMinutes(), other.blockDurationMinutes())
&& Objects.equals(allocationStrategyAsString(), other.allocationStrategyAsString());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("SpotProvisioningSpecification").add("TimeoutDurationMinutes", timeoutDurationMinutes())
.add("TimeoutAction", timeoutActionAsString()).add("BlockDurationMinutes", blockDurationMinutes())
.add("AllocationStrategy", allocationStrategyAsString()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "TimeoutDurationMinutes":
return Optional.ofNullable(clazz.cast(timeoutDurationMinutes()));
case "TimeoutAction":
return Optional.ofNullable(clazz.cast(timeoutActionAsString()));
case "BlockDurationMinutes":
return Optional.ofNullable(clazz.cast(blockDurationMinutes()));
case "AllocationStrategy":
return Optional.ofNullable(clazz.cast(allocationStrategyAsString()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
@Override
public final Map> sdkFieldNameToField() {
return SDK_NAME_TO_FIELD;
}
private static Function