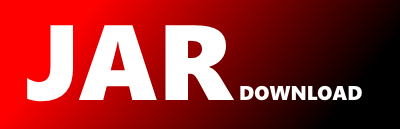
software.amazon.awssdk.services.emr.DefaultEmrAsyncClient Maven / Gradle / Ivy
/*
* Copyright 2014-2019 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.emr;
import java.util.concurrent.CompletableFuture;
import java.util.function.Consumer;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.annotations.SdkInternalApi;
import software.amazon.awssdk.awscore.AwsRequestOverrideConfiguration;
import software.amazon.awssdk.awscore.client.handler.AwsAsyncClientHandler;
import software.amazon.awssdk.awscore.exception.AwsServiceException;
import software.amazon.awssdk.core.ApiName;
import software.amazon.awssdk.core.client.config.SdkClientConfiguration;
import software.amazon.awssdk.core.client.handler.AsyncClientHandler;
import software.amazon.awssdk.core.client.handler.ClientExecutionParams;
import software.amazon.awssdk.core.http.HttpResponseHandler;
import software.amazon.awssdk.core.util.VersionInfo;
import software.amazon.awssdk.protocols.core.ExceptionMetadata;
import software.amazon.awssdk.protocols.json.AwsJsonProtocol;
import software.amazon.awssdk.protocols.json.AwsJsonProtocolFactory;
import software.amazon.awssdk.protocols.json.BaseAwsJsonProtocolFactory;
import software.amazon.awssdk.protocols.json.JsonOperationMetadata;
import software.amazon.awssdk.services.emr.model.AddInstanceFleetRequest;
import software.amazon.awssdk.services.emr.model.AddInstanceFleetResponse;
import software.amazon.awssdk.services.emr.model.AddInstanceGroupsRequest;
import software.amazon.awssdk.services.emr.model.AddInstanceGroupsResponse;
import software.amazon.awssdk.services.emr.model.AddJobFlowStepsRequest;
import software.amazon.awssdk.services.emr.model.AddJobFlowStepsResponse;
import software.amazon.awssdk.services.emr.model.AddTagsRequest;
import software.amazon.awssdk.services.emr.model.AddTagsResponse;
import software.amazon.awssdk.services.emr.model.CancelStepsRequest;
import software.amazon.awssdk.services.emr.model.CancelStepsResponse;
import software.amazon.awssdk.services.emr.model.CreateSecurityConfigurationRequest;
import software.amazon.awssdk.services.emr.model.CreateSecurityConfigurationResponse;
import software.amazon.awssdk.services.emr.model.DeleteSecurityConfigurationRequest;
import software.amazon.awssdk.services.emr.model.DeleteSecurityConfigurationResponse;
import software.amazon.awssdk.services.emr.model.DescribeClusterRequest;
import software.amazon.awssdk.services.emr.model.DescribeClusterResponse;
import software.amazon.awssdk.services.emr.model.DescribeSecurityConfigurationRequest;
import software.amazon.awssdk.services.emr.model.DescribeSecurityConfigurationResponse;
import software.amazon.awssdk.services.emr.model.DescribeStepRequest;
import software.amazon.awssdk.services.emr.model.DescribeStepResponse;
import software.amazon.awssdk.services.emr.model.EmrException;
import software.amazon.awssdk.services.emr.model.EmrRequest;
import software.amazon.awssdk.services.emr.model.InternalServerErrorException;
import software.amazon.awssdk.services.emr.model.InternalServerException;
import software.amazon.awssdk.services.emr.model.InvalidRequestException;
import software.amazon.awssdk.services.emr.model.ListBootstrapActionsRequest;
import software.amazon.awssdk.services.emr.model.ListBootstrapActionsResponse;
import software.amazon.awssdk.services.emr.model.ListClustersRequest;
import software.amazon.awssdk.services.emr.model.ListClustersResponse;
import software.amazon.awssdk.services.emr.model.ListInstanceFleetsRequest;
import software.amazon.awssdk.services.emr.model.ListInstanceFleetsResponse;
import software.amazon.awssdk.services.emr.model.ListInstanceGroupsRequest;
import software.amazon.awssdk.services.emr.model.ListInstanceGroupsResponse;
import software.amazon.awssdk.services.emr.model.ListInstancesRequest;
import software.amazon.awssdk.services.emr.model.ListInstancesResponse;
import software.amazon.awssdk.services.emr.model.ListSecurityConfigurationsRequest;
import software.amazon.awssdk.services.emr.model.ListSecurityConfigurationsResponse;
import software.amazon.awssdk.services.emr.model.ListStepsRequest;
import software.amazon.awssdk.services.emr.model.ListStepsResponse;
import software.amazon.awssdk.services.emr.model.ModifyInstanceFleetRequest;
import software.amazon.awssdk.services.emr.model.ModifyInstanceFleetResponse;
import software.amazon.awssdk.services.emr.model.ModifyInstanceGroupsRequest;
import software.amazon.awssdk.services.emr.model.ModifyInstanceGroupsResponse;
import software.amazon.awssdk.services.emr.model.PutAutoScalingPolicyRequest;
import software.amazon.awssdk.services.emr.model.PutAutoScalingPolicyResponse;
import software.amazon.awssdk.services.emr.model.RemoveAutoScalingPolicyRequest;
import software.amazon.awssdk.services.emr.model.RemoveAutoScalingPolicyResponse;
import software.amazon.awssdk.services.emr.model.RemoveTagsRequest;
import software.amazon.awssdk.services.emr.model.RemoveTagsResponse;
import software.amazon.awssdk.services.emr.model.RunJobFlowRequest;
import software.amazon.awssdk.services.emr.model.RunJobFlowResponse;
import software.amazon.awssdk.services.emr.model.SetTerminationProtectionRequest;
import software.amazon.awssdk.services.emr.model.SetTerminationProtectionResponse;
import software.amazon.awssdk.services.emr.model.SetVisibleToAllUsersRequest;
import software.amazon.awssdk.services.emr.model.SetVisibleToAllUsersResponse;
import software.amazon.awssdk.services.emr.model.TerminateJobFlowsRequest;
import software.amazon.awssdk.services.emr.model.TerminateJobFlowsResponse;
import software.amazon.awssdk.services.emr.paginators.ListBootstrapActionsPublisher;
import software.amazon.awssdk.services.emr.paginators.ListClustersPublisher;
import software.amazon.awssdk.services.emr.paginators.ListInstanceFleetsPublisher;
import software.amazon.awssdk.services.emr.paginators.ListInstanceGroupsPublisher;
import software.amazon.awssdk.services.emr.paginators.ListInstancesPublisher;
import software.amazon.awssdk.services.emr.paginators.ListSecurityConfigurationsPublisher;
import software.amazon.awssdk.services.emr.paginators.ListStepsPublisher;
import software.amazon.awssdk.services.emr.transform.AddInstanceFleetRequestMarshaller;
import software.amazon.awssdk.services.emr.transform.AddInstanceGroupsRequestMarshaller;
import software.amazon.awssdk.services.emr.transform.AddJobFlowStepsRequestMarshaller;
import software.amazon.awssdk.services.emr.transform.AddTagsRequestMarshaller;
import software.amazon.awssdk.services.emr.transform.CancelStepsRequestMarshaller;
import software.amazon.awssdk.services.emr.transform.CreateSecurityConfigurationRequestMarshaller;
import software.amazon.awssdk.services.emr.transform.DeleteSecurityConfigurationRequestMarshaller;
import software.amazon.awssdk.services.emr.transform.DescribeClusterRequestMarshaller;
import software.amazon.awssdk.services.emr.transform.DescribeSecurityConfigurationRequestMarshaller;
import software.amazon.awssdk.services.emr.transform.DescribeStepRequestMarshaller;
import software.amazon.awssdk.services.emr.transform.ListBootstrapActionsRequestMarshaller;
import software.amazon.awssdk.services.emr.transform.ListClustersRequestMarshaller;
import software.amazon.awssdk.services.emr.transform.ListInstanceFleetsRequestMarshaller;
import software.amazon.awssdk.services.emr.transform.ListInstanceGroupsRequestMarshaller;
import software.amazon.awssdk.services.emr.transform.ListInstancesRequestMarshaller;
import software.amazon.awssdk.services.emr.transform.ListSecurityConfigurationsRequestMarshaller;
import software.amazon.awssdk.services.emr.transform.ListStepsRequestMarshaller;
import software.amazon.awssdk.services.emr.transform.ModifyInstanceFleetRequestMarshaller;
import software.amazon.awssdk.services.emr.transform.ModifyInstanceGroupsRequestMarshaller;
import software.amazon.awssdk.services.emr.transform.PutAutoScalingPolicyRequestMarshaller;
import software.amazon.awssdk.services.emr.transform.RemoveAutoScalingPolicyRequestMarshaller;
import software.amazon.awssdk.services.emr.transform.RemoveTagsRequestMarshaller;
import software.amazon.awssdk.services.emr.transform.RunJobFlowRequestMarshaller;
import software.amazon.awssdk.services.emr.transform.SetTerminationProtectionRequestMarshaller;
import software.amazon.awssdk.services.emr.transform.SetVisibleToAllUsersRequestMarshaller;
import software.amazon.awssdk.services.emr.transform.TerminateJobFlowsRequestMarshaller;
import software.amazon.awssdk.utils.CompletableFutureUtils;
/**
* Internal implementation of {@link EmrAsyncClient}.
*
* @see EmrAsyncClient#builder()
*/
@Generated("software.amazon.awssdk:codegen")
@SdkInternalApi
final class DefaultEmrAsyncClient implements EmrAsyncClient {
private static final Logger log = LoggerFactory.getLogger(DefaultEmrAsyncClient.class);
private final AsyncClientHandler clientHandler;
private final AwsJsonProtocolFactory protocolFactory;
private final SdkClientConfiguration clientConfiguration;
protected DefaultEmrAsyncClient(SdkClientConfiguration clientConfiguration) {
this.clientHandler = new AwsAsyncClientHandler(clientConfiguration);
this.clientConfiguration = clientConfiguration;
this.protocolFactory = init(AwsJsonProtocolFactory.builder()).build();
}
@Override
public final String serviceName() {
return SERVICE_NAME;
}
/**
*
* Adds an instance fleet to a running cluster.
*
*
*
* The instance fleet configuration is available only in Amazon EMR versions 4.8.0 and later, excluding 5.0.x.
*
*
*
* @param addInstanceFleetRequest
* @return A Java Future containing the result of the AddInstanceFleet operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InternalServerException This exception occurs when there is an internal failure in the EMR service.
* - InvalidRequestException This exception occurs when there is something wrong with user input.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EmrException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EmrAsyncClient.AddInstanceFleet
* @see AWS API Documentation
*/
@Override
public CompletableFuture addInstanceFleet(AddInstanceFleetRequest addInstanceFleetRequest) {
try {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, AddInstanceFleetResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("AddInstanceFleet")
.withMarshaller(new AddInstanceFleetRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withInput(addInstanceFleetRequest));
return executeFuture;
} catch (Throwable t) {
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Adds one or more instance groups to a running cluster.
*
*
* @param addInstanceGroupsRequest
* Input to an AddInstanceGroups call.
* @return A Java Future containing the result of the AddInstanceGroups operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InternalServerErrorException Indicates that an error occurred while processing the request and that
* the request was not completed.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EmrException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EmrAsyncClient.AddInstanceGroups
* @see AWS API Documentation
*/
@Override
public CompletableFuture addInstanceGroups(AddInstanceGroupsRequest addInstanceGroupsRequest) {
try {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, AddInstanceGroupsResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("AddInstanceGroups")
.withMarshaller(new AddInstanceGroupsRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withInput(addInstanceGroupsRequest));
return executeFuture;
} catch (Throwable t) {
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* AddJobFlowSteps adds new steps to a running cluster. A maximum of 256 steps are allowed in each job flow.
*
*
* If your cluster is long-running (such as a Hive data warehouse) or complex, you may require more than 256 steps
* to process your data. You can bypass the 256-step limitation in various ways, including using SSH to connect to
* the master node and submitting queries directly to the software running on the master node, such as Hive and
* Hadoop. For more information on how to do this, see Add More than 256 Steps to
* a Cluster in the Amazon EMR Management Guide.
*
*
* A step specifies the location of a JAR file stored either on the master node of the cluster or in Amazon S3. Each
* step is performed by the main function of the main class of the JAR file. The main class can be specified either
* in the manifest of the JAR or by using the MainFunction parameter of the step.
*
*
* Amazon EMR executes each step in the order listed. For a step to be considered complete, the main function must
* exit with a zero exit code and all Hadoop jobs started while the step was running must have completed and run
* successfully.
*
*
* You can only add steps to a cluster that is in one of the following states: STARTING, BOOTSTRAPPING, RUNNING, or
* WAITING.
*
*
* @param addJobFlowStepsRequest
* The input argument to the AddJobFlowSteps operation.
* @return A Java Future containing the result of the AddJobFlowSteps operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InternalServerErrorException Indicates that an error occurred while processing the request and that
* the request was not completed.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EmrException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EmrAsyncClient.AddJobFlowSteps
* @see AWS API Documentation
*/
@Override
public CompletableFuture addJobFlowSteps(AddJobFlowStepsRequest addJobFlowStepsRequest) {
try {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, AddJobFlowStepsResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("AddJobFlowSteps")
.withMarshaller(new AddJobFlowStepsRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withInput(addJobFlowStepsRequest));
return executeFuture;
} catch (Throwable t) {
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Adds tags to an Amazon EMR resource. Tags make it easier to associate clusters in various ways, such as grouping
* clusters to track your Amazon EMR resource allocation costs. For more information, see Tag Clusters.
*
*
* @param addTagsRequest
* This input identifies a cluster and a list of tags to attach.
* @return A Java Future containing the result of the AddTags operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InternalServerException This exception occurs when there is an internal failure in the EMR service.
* - InvalidRequestException This exception occurs when there is something wrong with user input.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EmrException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EmrAsyncClient.AddTags
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture addTags(AddTagsRequest addTagsRequest) {
try {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
AddTagsResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams().withOperationName("AddTags")
.withMarshaller(new AddTagsRequestMarshaller(protocolFactory)).withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(addTagsRequest));
return executeFuture;
} catch (Throwable t) {
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Cancels a pending step or steps in a running cluster. Available only in Amazon EMR versions 4.8.0 and later,
* excluding version 5.0.0. A maximum of 256 steps are allowed in each CancelSteps request. CancelSteps is
* idempotent but asynchronous; it does not guarantee a step will be canceled, even if the request is successfully
* submitted. You can only cancel steps that are in a PENDING
state.
*
*
* @param cancelStepsRequest
* The input argument to the CancelSteps operation.
* @return A Java Future containing the result of the CancelSteps operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InternalServerErrorException Indicates that an error occurred while processing the request and that
* the request was not completed.
* - InvalidRequestException This exception occurs when there is something wrong with user input.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EmrException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EmrAsyncClient.CancelSteps
* @see AWS
* API Documentation
*/
@Override
public CompletableFuture cancelSteps(CancelStepsRequest cancelStepsRequest) {
try {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
CancelStepsResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("CancelSteps").withMarshaller(new CancelStepsRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withInput(cancelStepsRequest));
return executeFuture;
} catch (Throwable t) {
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Creates a security configuration, which is stored in the service and can be specified when a cluster is created.
*
*
* @param createSecurityConfigurationRequest
* @return A Java Future containing the result of the CreateSecurityConfiguration operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InternalServerException This exception occurs when there is an internal failure in the EMR service.
* - InvalidRequestException This exception occurs when there is something wrong with user input.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EmrException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EmrAsyncClient.CreateSecurityConfiguration
* @see AWS API Documentation
*/
@Override
public CompletableFuture createSecurityConfiguration(
CreateSecurityConfigurationRequest createSecurityConfigurationRequest) {
try {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, CreateSecurityConfigurationResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("CreateSecurityConfiguration")
.withMarshaller(new CreateSecurityConfigurationRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withInput(createSecurityConfigurationRequest));
return executeFuture;
} catch (Throwable t) {
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Deletes a security configuration.
*
*
* @param deleteSecurityConfigurationRequest
* @return A Java Future containing the result of the DeleteSecurityConfiguration operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InternalServerException This exception occurs when there is an internal failure in the EMR service.
* - InvalidRequestException This exception occurs when there is something wrong with user input.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EmrException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EmrAsyncClient.DeleteSecurityConfiguration
* @see AWS API Documentation
*/
@Override
public CompletableFuture deleteSecurityConfiguration(
DeleteSecurityConfigurationRequest deleteSecurityConfigurationRequest) {
try {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, DeleteSecurityConfigurationResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DeleteSecurityConfiguration")
.withMarshaller(new DeleteSecurityConfigurationRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withInput(deleteSecurityConfigurationRequest));
return executeFuture;
} catch (Throwable t) {
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Provides cluster-level details including status, hardware and software configuration, VPC settings, and so on.
*
*
* @param describeClusterRequest
* This input determines which cluster to describe.
* @return A Java Future containing the result of the DescribeCluster operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InternalServerException This exception occurs when there is an internal failure in the EMR service.
* - InvalidRequestException This exception occurs when there is something wrong with user input.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EmrException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EmrAsyncClient.DescribeCluster
* @see AWS API Documentation
*/
@Override
public CompletableFuture describeCluster(DescribeClusterRequest describeClusterRequest) {
try {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, DescribeClusterResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DescribeCluster")
.withMarshaller(new DescribeClusterRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withInput(describeClusterRequest));
return executeFuture;
} catch (Throwable t) {
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Provides the details of a security configuration by returning the configuration JSON.
*
*
* @param describeSecurityConfigurationRequest
* @return A Java Future containing the result of the DescribeSecurityConfiguration operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InternalServerException This exception occurs when there is an internal failure in the EMR service.
* - InvalidRequestException This exception occurs when there is something wrong with user input.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EmrException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EmrAsyncClient.DescribeSecurityConfiguration
* @see AWS API Documentation
*/
@Override
public CompletableFuture describeSecurityConfiguration(
DescribeSecurityConfigurationRequest describeSecurityConfigurationRequest) {
try {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, DescribeSecurityConfigurationResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DescribeSecurityConfiguration")
.withMarshaller(new DescribeSecurityConfigurationRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withInput(describeSecurityConfigurationRequest));
return executeFuture;
} catch (Throwable t) {
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Provides more detail about the cluster step.
*
*
* @param describeStepRequest
* This input determines which step to describe.
* @return A Java Future containing the result of the DescribeStep operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InternalServerException This exception occurs when there is an internal failure in the EMR service.
* - InvalidRequestException This exception occurs when there is something wrong with user input.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EmrException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EmrAsyncClient.DescribeStep
* @see AWS
* API Documentation
*/
@Override
public CompletableFuture describeStep(DescribeStepRequest describeStepRequest) {
try {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
DescribeStepResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DescribeStep").withMarshaller(new DescribeStepRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withInput(describeStepRequest));
return executeFuture;
} catch (Throwable t) {
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Provides information about the bootstrap actions associated with a cluster.
*
*
* @param listBootstrapActionsRequest
* This input determines which bootstrap actions to retrieve.
* @return A Java Future containing the result of the ListBootstrapActions operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InternalServerException This exception occurs when there is an internal failure in the EMR service.
* - InvalidRequestException This exception occurs when there is something wrong with user input.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EmrException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EmrAsyncClient.ListBootstrapActions
* @see AWS API Documentation
*/
@Override
public CompletableFuture listBootstrapActions(
ListBootstrapActionsRequest listBootstrapActionsRequest) {
try {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, ListBootstrapActionsResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("ListBootstrapActions")
.withMarshaller(new ListBootstrapActionsRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withInput(listBootstrapActionsRequest));
return executeFuture;
} catch (Throwable t) {
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Provides information about the bootstrap actions associated with a cluster.
*
*
*
* This is a variant of
* {@link #listBootstrapActions(software.amazon.awssdk.services.emr.model.ListBootstrapActionsRequest)} operation.
* The return type is a custom publisher that can be subscribed to request a stream of response pages. SDK will
* internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.emr.paginators.ListBootstrapActionsPublisher publisher = client.listBootstrapActionsPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.emr.paginators.ListBootstrapActionsPublisher publisher = client.listBootstrapActionsPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.emr.model.ListBootstrapActionsResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Note: If you prefer to have control on service calls, use the
* {@link #listBootstrapActions(software.amazon.awssdk.services.emr.model.ListBootstrapActionsRequest)}
* operation.
*
*
* @param listBootstrapActionsRequest
* This input determines which bootstrap actions to retrieve.
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InternalServerException This exception occurs when there is an internal failure in the EMR service.
* - InvalidRequestException This exception occurs when there is something wrong with user input.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EmrException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EmrAsyncClient.ListBootstrapActions
* @see AWS API Documentation
*/
public ListBootstrapActionsPublisher listBootstrapActionsPaginator(ListBootstrapActionsRequest listBootstrapActionsRequest) {
return new ListBootstrapActionsPublisher(this, applyPaginatorUserAgent(listBootstrapActionsRequest));
}
/**
*
* Provides the status of all clusters visible to this AWS account. Allows you to filter the list of clusters based
* on certain criteria; for example, filtering by cluster creation date and time or by status. This call returns a
* maximum of 50 clusters per call, but returns a marker to track the paging of the cluster list across multiple
* ListClusters calls.
*
*
* @param listClustersRequest
* This input determines how the ListClusters action filters the list of clusters that it returns.
* @return A Java Future containing the result of the ListClusters operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InternalServerException This exception occurs when there is an internal failure in the EMR service.
* - InvalidRequestException This exception occurs when there is something wrong with user input.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EmrException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EmrAsyncClient.ListClusters
* @see AWS
* API Documentation
*/
@Override
public CompletableFuture listClusters(ListClustersRequest listClustersRequest) {
try {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
ListClustersResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("ListClusters").withMarshaller(new ListClustersRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withInput(listClustersRequest));
return executeFuture;
} catch (Throwable t) {
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Provides the status of all clusters visible to this AWS account. Allows you to filter the list of clusters based
* on certain criteria; for example, filtering by cluster creation date and time or by status. This call returns a
* maximum of 50 clusters per call, but returns a marker to track the paging of the cluster list across multiple
* ListClusters calls.
*
*
*
* This is a variant of {@link #listClusters(software.amazon.awssdk.services.emr.model.ListClustersRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.emr.paginators.ListClustersPublisher publisher = client.listClustersPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.emr.paginators.ListClustersPublisher publisher = client.listClustersPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.emr.model.ListClustersResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Note: If you prefer to have control on service calls, use the
* {@link #listClusters(software.amazon.awssdk.services.emr.model.ListClustersRequest)} operation.
*
*
* @param listClustersRequest
* This input determines how the ListClusters action filters the list of clusters that it returns.
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InternalServerException This exception occurs when there is an internal failure in the EMR service.
* - InvalidRequestException This exception occurs when there is something wrong with user input.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EmrException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EmrAsyncClient.ListClusters
* @see AWS
* API Documentation
*/
public ListClustersPublisher listClustersPaginator(ListClustersRequest listClustersRequest) {
return new ListClustersPublisher(this, applyPaginatorUserAgent(listClustersRequest));
}
/**
*
* Lists all available details about the instance fleets in a cluster.
*
*
*
* The instance fleet configuration is available only in Amazon EMR versions 4.8.0 and later, excluding 5.0.x
* versions.
*
*
*
* @param listInstanceFleetsRequest
* @return A Java Future containing the result of the ListInstanceFleets operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InternalServerException This exception occurs when there is an internal failure in the EMR service.
* - InvalidRequestException This exception occurs when there is something wrong with user input.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EmrException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EmrAsyncClient.ListInstanceFleets
* @see AWS API Documentation
*/
@Override
public CompletableFuture listInstanceFleets(ListInstanceFleetsRequest listInstanceFleetsRequest) {
try {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, ListInstanceFleetsResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("ListInstanceFleets")
.withMarshaller(new ListInstanceFleetsRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withInput(listInstanceFleetsRequest));
return executeFuture;
} catch (Throwable t) {
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Lists all available details about the instance fleets in a cluster.
*
*
*
* The instance fleet configuration is available only in Amazon EMR versions 4.8.0 and later, excluding 5.0.x
* versions.
*
*
*
* This is a variant of
* {@link #listInstanceFleets(software.amazon.awssdk.services.emr.model.ListInstanceFleetsRequest)} operation. The
* return type is a custom publisher that can be subscribed to request a stream of response pages. SDK will
* internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.emr.paginators.ListInstanceFleetsPublisher publisher = client.listInstanceFleetsPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.emr.paginators.ListInstanceFleetsPublisher publisher = client.listInstanceFleetsPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.emr.model.ListInstanceFleetsResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Note: If you prefer to have control on service calls, use the
* {@link #listInstanceFleets(software.amazon.awssdk.services.emr.model.ListInstanceFleetsRequest)} operation.
*
*
* @param listInstanceFleetsRequest
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InternalServerException This exception occurs when there is an internal failure in the EMR service.
* - InvalidRequestException This exception occurs when there is something wrong with user input.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EmrException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EmrAsyncClient.ListInstanceFleets
* @see AWS API Documentation
*/
public ListInstanceFleetsPublisher listInstanceFleetsPaginator(ListInstanceFleetsRequest listInstanceFleetsRequest) {
return new ListInstanceFleetsPublisher(this, applyPaginatorUserAgent(listInstanceFleetsRequest));
}
/**
*
* Provides all available details about the instance groups in a cluster.
*
*
* @param listInstanceGroupsRequest
* This input determines which instance groups to retrieve.
* @return A Java Future containing the result of the ListInstanceGroups operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InternalServerException This exception occurs when there is an internal failure in the EMR service.
* - InvalidRequestException This exception occurs when there is something wrong with user input.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EmrException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EmrAsyncClient.ListInstanceGroups
* @see AWS API Documentation
*/
@Override
public CompletableFuture listInstanceGroups(ListInstanceGroupsRequest listInstanceGroupsRequest) {
try {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, ListInstanceGroupsResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("ListInstanceGroups")
.withMarshaller(new ListInstanceGroupsRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withInput(listInstanceGroupsRequest));
return executeFuture;
} catch (Throwable t) {
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Provides all available details about the instance groups in a cluster.
*
*
*
* This is a variant of
* {@link #listInstanceGroups(software.amazon.awssdk.services.emr.model.ListInstanceGroupsRequest)} operation. The
* return type is a custom publisher that can be subscribed to request a stream of response pages. SDK will
* internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.emr.paginators.ListInstanceGroupsPublisher publisher = client.listInstanceGroupsPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.emr.paginators.ListInstanceGroupsPublisher publisher = client.listInstanceGroupsPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.emr.model.ListInstanceGroupsResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Note: If you prefer to have control on service calls, use the
* {@link #listInstanceGroups(software.amazon.awssdk.services.emr.model.ListInstanceGroupsRequest)} operation.
*
*
* @param listInstanceGroupsRequest
* This input determines which instance groups to retrieve.
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InternalServerException This exception occurs when there is an internal failure in the EMR service.
* - InvalidRequestException This exception occurs when there is something wrong with user input.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EmrException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EmrAsyncClient.ListInstanceGroups
* @see AWS API Documentation
*/
public ListInstanceGroupsPublisher listInstanceGroupsPaginator(ListInstanceGroupsRequest listInstanceGroupsRequest) {
return new ListInstanceGroupsPublisher(this, applyPaginatorUserAgent(listInstanceGroupsRequest));
}
/**
*
* Provides information for all active EC2 instances and EC2 instances terminated in the last 30 days, up to a
* maximum of 2,000. EC2 instances in any of the following states are considered active: AWAITING_FULFILLMENT,
* PROVISIONING, BOOTSTRAPPING, RUNNING.
*
*
* @param listInstancesRequest
* This input determines which instances to list.
* @return A Java Future containing the result of the ListInstances operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InternalServerException This exception occurs when there is an internal failure in the EMR service.
* - InvalidRequestException This exception occurs when there is something wrong with user input.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EmrException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EmrAsyncClient.ListInstances
* @see AWS
* API Documentation
*/
@Override
public CompletableFuture listInstances(ListInstancesRequest listInstancesRequest) {
try {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
ListInstancesResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("ListInstances")
.withMarshaller(new ListInstancesRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withInput(listInstancesRequest));
return executeFuture;
} catch (Throwable t) {
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Provides information for all active EC2 instances and EC2 instances terminated in the last 30 days, up to a
* maximum of 2,000. EC2 instances in any of the following states are considered active: AWAITING_FULFILLMENT,
* PROVISIONING, BOOTSTRAPPING, RUNNING.
*
*
*
* This is a variant of {@link #listInstances(software.amazon.awssdk.services.emr.model.ListInstancesRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.emr.paginators.ListInstancesPublisher publisher = client.listInstancesPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.emr.paginators.ListInstancesPublisher publisher = client.listInstancesPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.emr.model.ListInstancesResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Note: If you prefer to have control on service calls, use the
* {@link #listInstances(software.amazon.awssdk.services.emr.model.ListInstancesRequest)} operation.
*
*
* @param listInstancesRequest
* This input determines which instances to list.
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InternalServerException This exception occurs when there is an internal failure in the EMR service.
* - InvalidRequestException This exception occurs when there is something wrong with user input.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EmrException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EmrAsyncClient.ListInstances
* @see AWS
* API Documentation
*/
public ListInstancesPublisher listInstancesPaginator(ListInstancesRequest listInstancesRequest) {
return new ListInstancesPublisher(this, applyPaginatorUserAgent(listInstancesRequest));
}
/**
*
* Lists all the security configurations visible to this account, providing their creation dates and times, and
* their names. This call returns a maximum of 50 clusters per call, but returns a marker to track the paging of the
* cluster list across multiple ListSecurityConfigurations calls.
*
*
* @param listSecurityConfigurationsRequest
* @return A Java Future containing the result of the ListSecurityConfigurations operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InternalServerException This exception occurs when there is an internal failure in the EMR service.
* - InvalidRequestException This exception occurs when there is something wrong with user input.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EmrException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EmrAsyncClient.ListSecurityConfigurations
* @see AWS API Documentation
*/
@Override
public CompletableFuture listSecurityConfigurations(
ListSecurityConfigurationsRequest listSecurityConfigurationsRequest) {
try {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, ListSecurityConfigurationsResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("ListSecurityConfigurations")
.withMarshaller(new ListSecurityConfigurationsRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withInput(listSecurityConfigurationsRequest));
return executeFuture;
} catch (Throwable t) {
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Lists all the security configurations visible to this account, providing their creation dates and times, and
* their names. This call returns a maximum of 50 clusters per call, but returns a marker to track the paging of the
* cluster list across multiple ListSecurityConfigurations calls.
*
*
*
* This is a variant of
* {@link #listSecurityConfigurations(software.amazon.awssdk.services.emr.model.ListSecurityConfigurationsRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.emr.paginators.ListSecurityConfigurationsPublisher publisher = client.listSecurityConfigurationsPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.emr.paginators.ListSecurityConfigurationsPublisher publisher = client.listSecurityConfigurationsPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.emr.model.ListSecurityConfigurationsResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Note: If you prefer to have control on service calls, use the
* {@link #listSecurityConfigurations(software.amazon.awssdk.services.emr.model.ListSecurityConfigurationsRequest)}
* operation.
*
*
* @param listSecurityConfigurationsRequest
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InternalServerException This exception occurs when there is an internal failure in the EMR service.
* - InvalidRequestException This exception occurs when there is something wrong with user input.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EmrException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EmrAsyncClient.ListSecurityConfigurations
* @see AWS API Documentation
*/
public ListSecurityConfigurationsPublisher listSecurityConfigurationsPaginator(
ListSecurityConfigurationsRequest listSecurityConfigurationsRequest) {
return new ListSecurityConfigurationsPublisher(this, applyPaginatorUserAgent(listSecurityConfigurationsRequest));
}
/**
*
* Provides a list of steps for the cluster in reverse order unless you specify stepIds with the request.
*
*
* @param listStepsRequest
* This input determines which steps to list.
* @return A Java Future containing the result of the ListSteps operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InternalServerException This exception occurs when there is an internal failure in the EMR service.
* - InvalidRequestException This exception occurs when there is something wrong with user input.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EmrException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EmrAsyncClient.ListSteps
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture listSteps(ListStepsRequest listStepsRequest) {
try {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
ListStepsResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams().withOperationName("ListSteps")
.withMarshaller(new ListStepsRequestMarshaller(protocolFactory)).withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(listStepsRequest));
return executeFuture;
} catch (Throwable t) {
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Provides a list of steps for the cluster in reverse order unless you specify stepIds with the request.
*
*
*
* This is a variant of {@link #listSteps(software.amazon.awssdk.services.emr.model.ListStepsRequest)} operation.
* The return type is a custom publisher that can be subscribed to request a stream of response pages. SDK will
* internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.emr.paginators.ListStepsPublisher publisher = client.listStepsPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.emr.paginators.ListStepsPublisher publisher = client.listStepsPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.emr.model.ListStepsResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Note: If you prefer to have control on service calls, use the
* {@link #listSteps(software.amazon.awssdk.services.emr.model.ListStepsRequest)} operation.
*
*
* @param listStepsRequest
* This input determines which steps to list.
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InternalServerException This exception occurs when there is an internal failure in the EMR service.
* - InvalidRequestException This exception occurs when there is something wrong with user input.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EmrException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EmrAsyncClient.ListSteps
* @see AWS API
* Documentation
*/
public ListStepsPublisher listStepsPaginator(ListStepsRequest listStepsRequest) {
return new ListStepsPublisher(this, applyPaginatorUserAgent(listStepsRequest));
}
/**
*
* Modifies the target On-Demand and target Spot capacities for the instance fleet with the specified
* InstanceFleetID within the cluster specified using ClusterID. The call either succeeds or fails atomically.
*
*
*
* The instance fleet configuration is available only in Amazon EMR versions 4.8.0 and later, excluding 5.0.x
* versions.
*
*
*
* @param modifyInstanceFleetRequest
* @return A Java Future containing the result of the ModifyInstanceFleet operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InternalServerException This exception occurs when there is an internal failure in the EMR service.
* - InvalidRequestException This exception occurs when there is something wrong with user input.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EmrException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EmrAsyncClient.ModifyInstanceFleet
* @see AWS API Documentation
*/
@Override
public CompletableFuture modifyInstanceFleet(
ModifyInstanceFleetRequest modifyInstanceFleetRequest) {
try {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, ModifyInstanceFleetResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("ModifyInstanceFleet")
.withMarshaller(new ModifyInstanceFleetRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withInput(modifyInstanceFleetRequest));
return executeFuture;
} catch (Throwable t) {
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* ModifyInstanceGroups modifies the number of nodes and configuration settings of an instance group. The input
* parameters include the new target instance count for the group and the instance group ID. The call will either
* succeed or fail atomically.
*
*
* @param modifyInstanceGroupsRequest
* Change the size of some instance groups.
* @return A Java Future containing the result of the ModifyInstanceGroups operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InternalServerErrorException Indicates that an error occurred while processing the request and that
* the request was not completed.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EmrException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EmrAsyncClient.ModifyInstanceGroups
* @see AWS API Documentation
*/
@Override
public CompletableFuture modifyInstanceGroups(
ModifyInstanceGroupsRequest modifyInstanceGroupsRequest) {
try {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, ModifyInstanceGroupsResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("ModifyInstanceGroups")
.withMarshaller(new ModifyInstanceGroupsRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withInput(modifyInstanceGroupsRequest));
return executeFuture;
} catch (Throwable t) {
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Creates or updates an automatic scaling policy for a core instance group or task instance group in an Amazon EMR
* cluster. The automatic scaling policy defines how an instance group dynamically adds and terminates EC2 instances
* in response to the value of a CloudWatch metric.
*
*
* @param putAutoScalingPolicyRequest
* @return A Java Future containing the result of the PutAutoScalingPolicy operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EmrException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EmrAsyncClient.PutAutoScalingPolicy
* @see AWS API Documentation
*/
@Override
public CompletableFuture putAutoScalingPolicy(
PutAutoScalingPolicyRequest putAutoScalingPolicyRequest) {
try {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, PutAutoScalingPolicyResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("PutAutoScalingPolicy")
.withMarshaller(new PutAutoScalingPolicyRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withInput(putAutoScalingPolicyRequest));
return executeFuture;
} catch (Throwable t) {
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Removes an automatic scaling policy from a specified instance group within an EMR cluster.
*
*
* @param removeAutoScalingPolicyRequest
* @return A Java Future containing the result of the RemoveAutoScalingPolicy operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EmrException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EmrAsyncClient.RemoveAutoScalingPolicy
* @see AWS API Documentation
*/
@Override
public CompletableFuture removeAutoScalingPolicy(
RemoveAutoScalingPolicyRequest removeAutoScalingPolicyRequest) {
try {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, RemoveAutoScalingPolicyResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("RemoveAutoScalingPolicy")
.withMarshaller(new RemoveAutoScalingPolicyRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withInput(removeAutoScalingPolicyRequest));
return executeFuture;
} catch (Throwable t) {
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Removes tags from an Amazon EMR resource. Tags make it easier to associate clusters in various ways, such as
* grouping clusters to track your Amazon EMR resource allocation costs. For more information, see Tag Clusters.
*
*
* The following example removes the stack tag with value Prod from a cluster:
*
*
* @param removeTagsRequest
* This input identifies a cluster and a list of tags to remove.
* @return A Java Future containing the result of the RemoveTags operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InternalServerException This exception occurs when there is an internal failure in the EMR service.
* - InvalidRequestException This exception occurs when there is something wrong with user input.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EmrException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EmrAsyncClient.RemoveTags
* @see AWS
* API Documentation
*/
@Override
public CompletableFuture removeTags(RemoveTagsRequest removeTagsRequest) {
try {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
RemoveTagsResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams().withOperationName("RemoveTags")
.withMarshaller(new RemoveTagsRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withInput(removeTagsRequest));
return executeFuture;
} catch (Throwable t) {
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* RunJobFlow creates and starts running a new cluster (job flow). The cluster runs the steps specified. After the
* steps complete, the cluster stops and the HDFS partition is lost. To prevent loss of data, configure the last
* step of the job flow to store results in Amazon S3. If the JobFlowInstancesConfig
* KeepJobFlowAliveWhenNoSteps
parameter is set to TRUE
, the cluster transitions to the
* WAITING state rather than shutting down after the steps have completed.
*
*
* For additional protection, you can set the JobFlowInstancesConfig TerminationProtected
* parameter to TRUE
to lock the cluster and prevent it from being terminated by API call, user
* intervention, or in the event of a job flow error.
*
*
* A maximum of 256 steps are allowed in each job flow.
*
*
* If your cluster is long-running (such as a Hive data warehouse) or complex, you may require more than 256 steps
* to process your data. You can bypass the 256-step limitation in various ways, including using the SSH shell to
* connect to the master node and submitting queries directly to the software running on the master node, such as
* Hive and Hadoop. For more information on how to do this, see Add More than 256 Steps to
* a Cluster in the Amazon EMR Management Guide.
*
*
* For long running clusters, we recommend that you periodically store your results.
*
*
*
* The instance fleets configuration is available only in Amazon EMR versions 4.8.0 and later, excluding 5.0.x
* versions. The RunJobFlow request can contain InstanceFleets parameters or InstanceGroups parameters, but not
* both.
*
*
*
* @param runJobFlowRequest
* Input to the RunJobFlow operation.
* @return A Java Future containing the result of the RunJobFlow operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InternalServerErrorException Indicates that an error occurred while processing the request and that
* the request was not completed.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EmrException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EmrAsyncClient.RunJobFlow
* @see AWS
* API Documentation
*/
@Override
public CompletableFuture runJobFlow(RunJobFlowRequest runJobFlowRequest) {
try {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
RunJobFlowResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams().withOperationName("RunJobFlow")
.withMarshaller(new RunJobFlowRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withInput(runJobFlowRequest));
return executeFuture;
} catch (Throwable t) {
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* SetTerminationProtection locks a cluster (job flow) so the EC2 instances in the cluster cannot be terminated by
* user intervention, an API call, or in the event of a job-flow error. The cluster still terminates upon successful
* completion of the job flow. Calling SetTerminationProtection
on a cluster is similar to calling the
* Amazon EC2 DisableAPITermination
API on all EC2 instances in a cluster.
*
*
* SetTerminationProtection
is used to prevent accidental termination of a cluster and to ensure that
* in the event of an error, the instances persist so that you can recover any data stored in their ephemeral
* instance storage.
*
*
* To terminate a cluster that has been locked by setting SetTerminationProtection
to true
* , you must first unlock the job flow by a subsequent call to SetTerminationProtection
in which you
* set the value to false
.
*
*
* For more information, seeManaging
* Cluster Termination in the Amazon EMR Management Guide.
*
*
* @param setTerminationProtectionRequest
* The input argument to the TerminationProtection operation.
* @return A Java Future containing the result of the SetTerminationProtection operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InternalServerErrorException Indicates that an error occurred while processing the request and that
* the request was not completed.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EmrException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EmrAsyncClient.SetTerminationProtection
* @see AWS API Documentation
*/
@Override
public CompletableFuture setTerminationProtection(
SetTerminationProtectionRequest setTerminationProtectionRequest) {
try {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, SetTerminationProtectionResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("SetTerminationProtection")
.withMarshaller(new SetTerminationProtectionRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withInput(setTerminationProtectionRequest));
return executeFuture;
} catch (Throwable t) {
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Sets whether all AWS Identity and Access Management (IAM) users under your account can access the specified
* clusters (job flows). This action works on running clusters. You can also set the visibility of a cluster when
* you launch it using the VisibleToAllUsers
parameter of RunJobFlow. The SetVisibleToAllUsers
* action can be called only by an IAM user who created the cluster or the AWS account that owns the cluster.
*
*
* @param setVisibleToAllUsersRequest
* The input to the SetVisibleToAllUsers action.
* @return A Java Future containing the result of the SetVisibleToAllUsers operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InternalServerErrorException Indicates that an error occurred while processing the request and that
* the request was not completed.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EmrException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EmrAsyncClient.SetVisibleToAllUsers
* @see AWS API Documentation
*/
@Override
public CompletableFuture setVisibleToAllUsers(
SetVisibleToAllUsersRequest setVisibleToAllUsersRequest) {
try {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, SetVisibleToAllUsersResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("SetVisibleToAllUsers")
.withMarshaller(new SetVisibleToAllUsersRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withInput(setVisibleToAllUsersRequest));
return executeFuture;
} catch (Throwable t) {
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* TerminateJobFlows shuts a list of clusters (job flows) down. When a job flow is shut down, any step not yet
* completed is canceled and the EC2 instances on which the cluster is running are stopped. Any log files not
* already saved are uploaded to Amazon S3 if a LogUri was specified when the cluster was created.
*
*
* The maximum number of clusters allowed is 10. The call to TerminateJobFlows
is asynchronous.
* Depending on the configuration of the cluster, it may take up to 1-5 minutes for the cluster to completely
* terminate and release allocated resources, such as Amazon EC2 instances.
*
*
* @param terminateJobFlowsRequest
* Input to the TerminateJobFlows operation.
* @return A Java Future containing the result of the TerminateJobFlows operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InternalServerErrorException Indicates that an error occurred while processing the request and that
* the request was not completed.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EmrException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EmrAsyncClient.TerminateJobFlows
* @see AWS API Documentation
*/
@Override
public CompletableFuture terminateJobFlows(TerminateJobFlowsRequest terminateJobFlowsRequest) {
try {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, TerminateJobFlowsResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("TerminateJobFlows")
.withMarshaller(new TerminateJobFlowsRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withInput(terminateJobFlowsRequest));
return executeFuture;
} catch (Throwable t) {
return CompletableFutureUtils.failedFuture(t);
}
}
@Override
public void close() {
clientHandler.close();
}
private > T init(T builder) {
return builder
.clientConfiguration(clientConfiguration)
.defaultServiceExceptionSupplier(EmrException::builder)
.protocol(AwsJsonProtocol.AWS_JSON)
.protocolVersion("1.1")
.registerModeledException(
ExceptionMetadata.builder().errorCode("InvalidRequestException")
.exceptionBuilderSupplier(InvalidRequestException::builder).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("InternalServerException")
.exceptionBuilderSupplier(InternalServerException::builder).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("InternalServerError")
.exceptionBuilderSupplier(InternalServerErrorException::builder).build());
}
private T applyPaginatorUserAgent(T request) {
Consumer userAgentApplier = b -> b.addApiName(ApiName.builder()
.version(VersionInfo.SDK_VERSION).name("PAGINATED").build());
AwsRequestOverrideConfiguration overrideConfiguration = request.overrideConfiguration()
.map(c -> c.toBuilder().applyMutation(userAgentApplier).build())
.orElse((AwsRequestOverrideConfiguration.builder().applyMutation(userAgentApplier).build()));
return (T) request.toBuilder().overrideConfiguration(overrideConfiguration).build();
}
private HttpResponseHandler createErrorResponseHandler(BaseAwsJsonProtocolFactory protocolFactory,
JsonOperationMetadata operationMetadata) {
return protocolFactory.createErrorResponseHandler(operationMetadata);
}
}