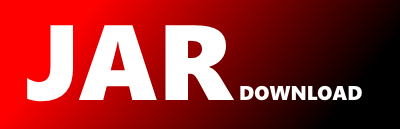
software.amazon.awssdk.services.emr.model.ScalingConstraints Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of emr Show documentation
Show all versions of emr Show documentation
The AWS Java SDK for Amazon EMR module holds the client classes that are used for communicating with
Amazon Elastic MapReduce Service
/*
* Copyright 2014-2019 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.emr.model;
import java.io.Serializable;
import java.util.Arrays;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* The upper and lower EC2 instance limits for an automatic scaling policy. Automatic scaling activities triggered by
* automatic scaling rules will not cause an instance group to grow above or below these limits.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class ScalingConstraints implements SdkPojo, Serializable,
ToCopyableBuilder {
private static final SdkField MIN_CAPACITY_FIELD = SdkField. builder(MarshallingType.INTEGER)
.getter(getter(ScalingConstraints::minCapacity)).setter(setter(Builder::minCapacity))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("MinCapacity").build()).build();
private static final SdkField MAX_CAPACITY_FIELD = SdkField. builder(MarshallingType.INTEGER)
.getter(getter(ScalingConstraints::maxCapacity)).setter(setter(Builder::maxCapacity))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("MaxCapacity").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(MIN_CAPACITY_FIELD,
MAX_CAPACITY_FIELD));
private static final long serialVersionUID = 1L;
private final Integer minCapacity;
private final Integer maxCapacity;
private ScalingConstraints(BuilderImpl builder) {
this.minCapacity = builder.minCapacity;
this.maxCapacity = builder.maxCapacity;
}
/**
*
* The lower boundary of EC2 instances in an instance group below which scaling activities are not allowed to
* shrink. Scale-in activities will not terminate instances below this boundary.
*
*
* @return The lower boundary of EC2 instances in an instance group below which scaling activities are not allowed
* to shrink. Scale-in activities will not terminate instances below this boundary.
*/
public Integer minCapacity() {
return minCapacity;
}
/**
*
* The upper boundary of EC2 instances in an instance group beyond which scaling activities are not allowed to grow.
* Scale-out activities will not add instances beyond this boundary.
*
*
* @return The upper boundary of EC2 instances in an instance group beyond which scaling activities are not allowed
* to grow. Scale-out activities will not add instances beyond this boundary.
*/
public Integer maxCapacity() {
return maxCapacity;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(minCapacity());
hashCode = 31 * hashCode + Objects.hashCode(maxCapacity());
return hashCode;
}
@Override
public boolean equals(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof ScalingConstraints)) {
return false;
}
ScalingConstraints other = (ScalingConstraints) obj;
return Objects.equals(minCapacity(), other.minCapacity()) && Objects.equals(maxCapacity(), other.maxCapacity());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public String toString() {
return ToString.builder("ScalingConstraints").add("MinCapacity", minCapacity()).add("MaxCapacity", maxCapacity()).build();
}
public Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "MinCapacity":
return Optional.ofNullable(clazz.cast(minCapacity()));
case "MaxCapacity":
return Optional.ofNullable(clazz.cast(maxCapacity()));
default:
return Optional.empty();
}
}
@Override
public List> sdkFields() {
return SDK_FIELDS;
}
private static Function
© 2015 - 2025 Weber Informatics LLC | Privacy Policy