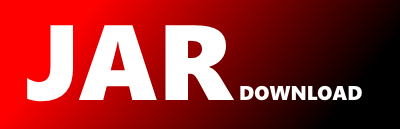
software.amazon.awssdk.services.emrserverless.EmrServerlessAsyncClient Maven / Gradle / Ivy
Show all versions of emrserverless Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.emrserverless;
import java.util.concurrent.CompletableFuture;
import java.util.function.Consumer;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.annotations.SdkPublicApi;
import software.amazon.awssdk.annotations.ThreadSafe;
import software.amazon.awssdk.awscore.AwsClient;
import software.amazon.awssdk.services.emrserverless.model.CancelJobRunRequest;
import software.amazon.awssdk.services.emrserverless.model.CancelJobRunResponse;
import software.amazon.awssdk.services.emrserverless.model.CreateApplicationRequest;
import software.amazon.awssdk.services.emrserverless.model.CreateApplicationResponse;
import software.amazon.awssdk.services.emrserverless.model.DeleteApplicationRequest;
import software.amazon.awssdk.services.emrserverless.model.DeleteApplicationResponse;
import software.amazon.awssdk.services.emrserverless.model.GetApplicationRequest;
import software.amazon.awssdk.services.emrserverless.model.GetApplicationResponse;
import software.amazon.awssdk.services.emrserverless.model.GetDashboardForJobRunRequest;
import software.amazon.awssdk.services.emrserverless.model.GetDashboardForJobRunResponse;
import software.amazon.awssdk.services.emrserverless.model.GetJobRunRequest;
import software.amazon.awssdk.services.emrserverless.model.GetJobRunResponse;
import software.amazon.awssdk.services.emrserverless.model.ListApplicationsRequest;
import software.amazon.awssdk.services.emrserverless.model.ListApplicationsResponse;
import software.amazon.awssdk.services.emrserverless.model.ListJobRunAttemptsRequest;
import software.amazon.awssdk.services.emrserverless.model.ListJobRunAttemptsResponse;
import software.amazon.awssdk.services.emrserverless.model.ListJobRunsRequest;
import software.amazon.awssdk.services.emrserverless.model.ListJobRunsResponse;
import software.amazon.awssdk.services.emrserverless.model.ListTagsForResourceRequest;
import software.amazon.awssdk.services.emrserverless.model.ListTagsForResourceResponse;
import software.amazon.awssdk.services.emrserverless.model.StartApplicationRequest;
import software.amazon.awssdk.services.emrserverless.model.StartApplicationResponse;
import software.amazon.awssdk.services.emrserverless.model.StartJobRunRequest;
import software.amazon.awssdk.services.emrserverless.model.StartJobRunResponse;
import software.amazon.awssdk.services.emrserverless.model.StopApplicationRequest;
import software.amazon.awssdk.services.emrserverless.model.StopApplicationResponse;
import software.amazon.awssdk.services.emrserverless.model.TagResourceRequest;
import software.amazon.awssdk.services.emrserverless.model.TagResourceResponse;
import software.amazon.awssdk.services.emrserverless.model.UntagResourceRequest;
import software.amazon.awssdk.services.emrserverless.model.UntagResourceResponse;
import software.amazon.awssdk.services.emrserverless.model.UpdateApplicationRequest;
import software.amazon.awssdk.services.emrserverless.model.UpdateApplicationResponse;
import software.amazon.awssdk.services.emrserverless.paginators.ListApplicationsPublisher;
import software.amazon.awssdk.services.emrserverless.paginators.ListJobRunAttemptsPublisher;
import software.amazon.awssdk.services.emrserverless.paginators.ListJobRunsPublisher;
/**
* Service client for accessing EMR Serverless asynchronously. This can be created using the static {@link #builder()}
* method.The asynchronous client performs non-blocking I/O when configured with any {@code SdkAsyncHttpClient}
* supported in the SDK. However, full non-blocking is not guaranteed as the async client may perform blocking calls in
* some cases such as credentials retrieval and endpoint discovery as part of the async API call.
*
*
* Amazon EMR Serverless is a new deployment option for Amazon EMR. Amazon EMR Serverless provides a serverless runtime
* environment that simplifies running analytics applications using the latest open source frameworks such as Apache
* Spark and Apache Hive. With Amazon EMR Serverless, you don’t have to configure, optimize, secure, or operate clusters
* to run applications with these frameworks.
*
*
* The API reference to Amazon EMR Serverless is emr-serverless
. The emr-serverless
prefix is
* used in the following scenarios:
*
*
* -
*
* It is the prefix in the CLI commands for Amazon EMR Serverless. For example,
* aws emr-serverless start-job-run
.
*
*
* -
*
* It is the prefix before IAM policy actions for Amazon EMR Serverless. For example,
* "Action": ["emr-serverless:StartJobRun"]
. For more information, see Policy actions for Amazon EMR Serverless.
*
*
* -
*
* It is the prefix used in Amazon EMR Serverless service endpoints. For example,
* emr-serverless.us-east-2.amazonaws.com
.
*
*
*
*/
@Generated("software.amazon.awssdk:codegen")
@SdkPublicApi
@ThreadSafe
public interface EmrServerlessAsyncClient extends AwsClient {
String SERVICE_NAME = "emr-serverless";
/**
* Value for looking up the service's metadata from the
* {@link software.amazon.awssdk.regions.ServiceMetadataProvider}.
*/
String SERVICE_METADATA_ID = "emr-serverless";
/**
*
* Cancels a job run.
*
*
* @param cancelJobRunRequest
* @return A Java Future containing the result of the CancelJobRun operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ValidationException The input fails to satisfy the constraints specified by an Amazon Web Services
* service.
* - ResourceNotFoundException The specified resource was not found.
* - InternalServerException Request processing failed because of an error or failure with the service.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EmrServerlessException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample EmrServerlessAsyncClient.CancelJobRun
* @see AWS
* API Documentation
*/
default CompletableFuture cancelJobRun(CancelJobRunRequest cancelJobRunRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Cancels a job run.
*
*
*
* This is a convenience which creates an instance of the {@link CancelJobRunRequest.Builder} avoiding the need to
* create one manually via {@link CancelJobRunRequest#builder()}
*
*
* @param cancelJobRunRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.emrserverless.model.CancelJobRunRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the CancelJobRun operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ValidationException The input fails to satisfy the constraints specified by an Amazon Web Services
* service.
* - ResourceNotFoundException The specified resource was not found.
* - InternalServerException Request processing failed because of an error or failure with the service.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EmrServerlessException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample EmrServerlessAsyncClient.CancelJobRun
* @see AWS
* API Documentation
*/
default CompletableFuture cancelJobRun(Consumer cancelJobRunRequest) {
return cancelJobRun(CancelJobRunRequest.builder().applyMutation(cancelJobRunRequest).build());
}
/**
*
* Creates an application.
*
*
* @param createApplicationRequest
* @return A Java Future containing the result of the CreateApplication operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ValidationException The input fails to satisfy the constraints specified by an Amazon Web Services
* service.
* - ResourceNotFoundException The specified resource was not found.
* - InternalServerException Request processing failed because of an error or failure with the service.
* - ConflictException The request could not be processed because of conflict in the current state of the
* resource.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EmrServerlessException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample EmrServerlessAsyncClient.CreateApplication
* @see AWS API Documentation
*/
default CompletableFuture createApplication(CreateApplicationRequest createApplicationRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Creates an application.
*
*
*
* This is a convenience which creates an instance of the {@link CreateApplicationRequest.Builder} avoiding the need
* to create one manually via {@link CreateApplicationRequest#builder()}
*
*
* @param createApplicationRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.emrserverless.model.CreateApplicationRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the CreateApplication operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ValidationException The input fails to satisfy the constraints specified by an Amazon Web Services
* service.
* - ResourceNotFoundException The specified resource was not found.
* - InternalServerException Request processing failed because of an error or failure with the service.
* - ConflictException The request could not be processed because of conflict in the current state of the
* resource.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EmrServerlessException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample EmrServerlessAsyncClient.CreateApplication
* @see AWS API Documentation
*/
default CompletableFuture createApplication(
Consumer createApplicationRequest) {
return createApplication(CreateApplicationRequest.builder().applyMutation(createApplicationRequest).build());
}
/**
*
* Deletes an application. An application has to be in a stopped or created state in order to be deleted.
*
*
* @param deleteApplicationRequest
* @return A Java Future containing the result of the DeleteApplication operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ValidationException The input fails to satisfy the constraints specified by an Amazon Web Services
* service.
* - ResourceNotFoundException The specified resource was not found.
* - InternalServerException Request processing failed because of an error or failure with the service.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EmrServerlessException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample EmrServerlessAsyncClient.DeleteApplication
* @see AWS API Documentation
*/
default CompletableFuture deleteApplication(DeleteApplicationRequest deleteApplicationRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Deletes an application. An application has to be in a stopped or created state in order to be deleted.
*
*
*
* This is a convenience which creates an instance of the {@link DeleteApplicationRequest.Builder} avoiding the need
* to create one manually via {@link DeleteApplicationRequest#builder()}
*
*
* @param deleteApplicationRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.emrserverless.model.DeleteApplicationRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the DeleteApplication operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ValidationException The input fails to satisfy the constraints specified by an Amazon Web Services
* service.
* - ResourceNotFoundException The specified resource was not found.
* - InternalServerException Request processing failed because of an error or failure with the service.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EmrServerlessException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample EmrServerlessAsyncClient.DeleteApplication
* @see AWS API Documentation
*/
default CompletableFuture deleteApplication(
Consumer deleteApplicationRequest) {
return deleteApplication(DeleteApplicationRequest.builder().applyMutation(deleteApplicationRequest).build());
}
/**
*
* Displays detailed information about a specified application.
*
*
* @param getApplicationRequest
* @return A Java Future containing the result of the GetApplication operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ValidationException The input fails to satisfy the constraints specified by an Amazon Web Services
* service.
* - ResourceNotFoundException The specified resource was not found.
* - InternalServerException Request processing failed because of an error or failure with the service.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EmrServerlessException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample EmrServerlessAsyncClient.GetApplication
* @see AWS
* API Documentation
*/
default CompletableFuture getApplication(GetApplicationRequest getApplicationRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Displays detailed information about a specified application.
*
*
*
* This is a convenience which creates an instance of the {@link GetApplicationRequest.Builder} avoiding the need to
* create one manually via {@link GetApplicationRequest#builder()}
*
*
* @param getApplicationRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.emrserverless.model.GetApplicationRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the GetApplication operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ValidationException The input fails to satisfy the constraints specified by an Amazon Web Services
* service.
* - ResourceNotFoundException The specified resource was not found.
* - InternalServerException Request processing failed because of an error or failure with the service.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EmrServerlessException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample EmrServerlessAsyncClient.GetApplication
* @see AWS
* API Documentation
*/
default CompletableFuture getApplication(Consumer getApplicationRequest) {
return getApplication(GetApplicationRequest.builder().applyMutation(getApplicationRequest).build());
}
/**
*
* Creates and returns a URL that you can use to access the application UIs for a job run.
*
*
* For jobs in a running state, the application UI is a live user interface such as the Spark or Tez web UI. For
* completed jobs, the application UI is a persistent application user interface such as the Spark History Server or
* persistent Tez UI.
*
*
*
* The URL is valid for one hour after you generate it. To access the application UI after that hour elapses, you
* must invoke the API again to generate a new URL.
*
*
*
* @param getDashboardForJobRunRequest
* @return A Java Future containing the result of the GetDashboardForJobRun operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ValidationException The input fails to satisfy the constraints specified by an Amazon Web Services
* service.
* - ResourceNotFoundException The specified resource was not found.
* - InternalServerException Request processing failed because of an error or failure with the service.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EmrServerlessException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample EmrServerlessAsyncClient.GetDashboardForJobRun
* @see AWS API Documentation
*/
default CompletableFuture getDashboardForJobRun(
GetDashboardForJobRunRequest getDashboardForJobRunRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Creates and returns a URL that you can use to access the application UIs for a job run.
*
*
* For jobs in a running state, the application UI is a live user interface such as the Spark or Tez web UI. For
* completed jobs, the application UI is a persistent application user interface such as the Spark History Server or
* persistent Tez UI.
*
*
*
* The URL is valid for one hour after you generate it. To access the application UI after that hour elapses, you
* must invoke the API again to generate a new URL.
*
*
*
* This is a convenience which creates an instance of the {@link GetDashboardForJobRunRequest.Builder} avoiding the
* need to create one manually via {@link GetDashboardForJobRunRequest#builder()}
*
*
* @param getDashboardForJobRunRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.emrserverless.model.GetDashboardForJobRunRequest.Builder} to create
* a request.
* @return A Java Future containing the result of the GetDashboardForJobRun operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ValidationException The input fails to satisfy the constraints specified by an Amazon Web Services
* service.
* - ResourceNotFoundException The specified resource was not found.
* - InternalServerException Request processing failed because of an error or failure with the service.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EmrServerlessException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample EmrServerlessAsyncClient.GetDashboardForJobRun
* @see AWS API Documentation
*/
default CompletableFuture getDashboardForJobRun(
Consumer getDashboardForJobRunRequest) {
return getDashboardForJobRun(GetDashboardForJobRunRequest.builder().applyMutation(getDashboardForJobRunRequest).build());
}
/**
*
* Displays detailed information about a job run.
*
*
* @param getJobRunRequest
* @return A Java Future containing the result of the GetJobRun operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ValidationException The input fails to satisfy the constraints specified by an Amazon Web Services
* service.
* - ResourceNotFoundException The specified resource was not found.
* - InternalServerException Request processing failed because of an error or failure with the service.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EmrServerlessException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample EmrServerlessAsyncClient.GetJobRun
* @see AWS API
* Documentation
*/
default CompletableFuture getJobRun(GetJobRunRequest getJobRunRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Displays detailed information about a job run.
*
*
*
* This is a convenience which creates an instance of the {@link GetJobRunRequest.Builder} avoiding the need to
* create one manually via {@link GetJobRunRequest#builder()}
*
*
* @param getJobRunRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.emrserverless.model.GetJobRunRequest.Builder} to create a request.
* @return A Java Future containing the result of the GetJobRun operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ValidationException The input fails to satisfy the constraints specified by an Amazon Web Services
* service.
* - ResourceNotFoundException The specified resource was not found.
* - InternalServerException Request processing failed because of an error or failure with the service.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EmrServerlessException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample EmrServerlessAsyncClient.GetJobRun
* @see AWS API
* Documentation
*/
default CompletableFuture getJobRun(Consumer getJobRunRequest) {
return getJobRun(GetJobRunRequest.builder().applyMutation(getJobRunRequest).build());
}
/**
*
* Lists applications based on a set of parameters.
*
*
* @param listApplicationsRequest
* @return A Java Future containing the result of the ListApplications operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ValidationException The input fails to satisfy the constraints specified by an Amazon Web Services
* service.
* - InternalServerException Request processing failed because of an error or failure with the service.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EmrServerlessException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample EmrServerlessAsyncClient.ListApplications
* @see AWS API Documentation
*/
default CompletableFuture listApplications(ListApplicationsRequest listApplicationsRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Lists applications based on a set of parameters.
*
*
*
* This is a convenience which creates an instance of the {@link ListApplicationsRequest.Builder} avoiding the need
* to create one manually via {@link ListApplicationsRequest#builder()}
*
*
* @param listApplicationsRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.emrserverless.model.ListApplicationsRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the ListApplications operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ValidationException The input fails to satisfy the constraints specified by an Amazon Web Services
* service.
* - InternalServerException Request processing failed because of an error or failure with the service.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EmrServerlessException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample EmrServerlessAsyncClient.ListApplications
* @see AWS API Documentation
*/
default CompletableFuture listApplications(
Consumer listApplicationsRequest) {
return listApplications(ListApplicationsRequest.builder().applyMutation(listApplicationsRequest).build());
}
/**
*
* This is a variant of
* {@link #listApplications(software.amazon.awssdk.services.emrserverless.model.ListApplicationsRequest)} operation.
* The return type is a custom publisher that can be subscribed to request a stream of response pages. SDK will
* internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.emrserverless.paginators.ListApplicationsPublisher publisher = client.listApplicationsPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.emrserverless.paginators.ListApplicationsPublisher publisher = client.listApplicationsPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.emrserverless.model.ListApplicationsResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of maxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listApplications(software.amazon.awssdk.services.emrserverless.model.ListApplicationsRequest)}
* operation.
*
*
* @param listApplicationsRequest
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ValidationException The input fails to satisfy the constraints specified by an Amazon Web Services
* service.
* - InternalServerException Request processing failed because of an error or failure with the service.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EmrServerlessException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample EmrServerlessAsyncClient.ListApplications
* @see AWS API Documentation
*/
default ListApplicationsPublisher listApplicationsPaginator(ListApplicationsRequest listApplicationsRequest) {
return new ListApplicationsPublisher(this, listApplicationsRequest);
}
/**
*
* This is a variant of
* {@link #listApplications(software.amazon.awssdk.services.emrserverless.model.ListApplicationsRequest)} operation.
* The return type is a custom publisher that can be subscribed to request a stream of response pages. SDK will
* internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.emrserverless.paginators.ListApplicationsPublisher publisher = client.listApplicationsPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.emrserverless.paginators.ListApplicationsPublisher publisher = client.listApplicationsPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.emrserverless.model.ListApplicationsResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of maxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listApplications(software.amazon.awssdk.services.emrserverless.model.ListApplicationsRequest)}
* operation.
*
*
*
* This is a convenience which creates an instance of the {@link ListApplicationsRequest.Builder} avoiding the need
* to create one manually via {@link ListApplicationsRequest#builder()}
*
*
* @param listApplicationsRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.emrserverless.model.ListApplicationsRequest.Builder} to create a
* request.
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ValidationException The input fails to satisfy the constraints specified by an Amazon Web Services
* service.
* - InternalServerException Request processing failed because of an error or failure with the service.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EmrServerlessException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample EmrServerlessAsyncClient.ListApplications
* @see AWS API Documentation
*/
default ListApplicationsPublisher listApplicationsPaginator(Consumer listApplicationsRequest) {
return listApplicationsPaginator(ListApplicationsRequest.builder().applyMutation(listApplicationsRequest).build());
}
/**
*
* Lists all attempt of a job run.
*
*
* @param listJobRunAttemptsRequest
* @return A Java Future containing the result of the ListJobRunAttempts operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ValidationException The input fails to satisfy the constraints specified by an Amazon Web Services
* service.
* - ResourceNotFoundException The specified resource was not found.
* - InternalServerException Request processing failed because of an error or failure with the service.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EmrServerlessException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample EmrServerlessAsyncClient.ListJobRunAttempts
* @see AWS API Documentation
*/
default CompletableFuture listJobRunAttempts(ListJobRunAttemptsRequest listJobRunAttemptsRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Lists all attempt of a job run.
*
*
*
* This is a convenience which creates an instance of the {@link ListJobRunAttemptsRequest.Builder} avoiding the
* need to create one manually via {@link ListJobRunAttemptsRequest#builder()}
*
*
* @param listJobRunAttemptsRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.emrserverless.model.ListJobRunAttemptsRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the ListJobRunAttempts operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ValidationException The input fails to satisfy the constraints specified by an Amazon Web Services
* service.
* - ResourceNotFoundException The specified resource was not found.
* - InternalServerException Request processing failed because of an error or failure with the service.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EmrServerlessException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample EmrServerlessAsyncClient.ListJobRunAttempts
* @see AWS API Documentation
*/
default CompletableFuture listJobRunAttempts(
Consumer listJobRunAttemptsRequest) {
return listJobRunAttempts(ListJobRunAttemptsRequest.builder().applyMutation(listJobRunAttemptsRequest).build());
}
/**
*
* This is a variant of
* {@link #listJobRunAttempts(software.amazon.awssdk.services.emrserverless.model.ListJobRunAttemptsRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.emrserverless.paginators.ListJobRunAttemptsPublisher publisher = client.listJobRunAttemptsPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.emrserverless.paginators.ListJobRunAttemptsPublisher publisher = client.listJobRunAttemptsPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.emrserverless.model.ListJobRunAttemptsResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of maxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listJobRunAttempts(software.amazon.awssdk.services.emrserverless.model.ListJobRunAttemptsRequest)}
* operation.
*
*
* @param listJobRunAttemptsRequest
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ValidationException The input fails to satisfy the constraints specified by an Amazon Web Services
* service.
* - ResourceNotFoundException The specified resource was not found.
* - InternalServerException Request processing failed because of an error or failure with the service.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EmrServerlessException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample EmrServerlessAsyncClient.ListJobRunAttempts
* @see AWS API Documentation
*/
default ListJobRunAttemptsPublisher listJobRunAttemptsPaginator(ListJobRunAttemptsRequest listJobRunAttemptsRequest) {
return new ListJobRunAttemptsPublisher(this, listJobRunAttemptsRequest);
}
/**
*
* This is a variant of
* {@link #listJobRunAttempts(software.amazon.awssdk.services.emrserverless.model.ListJobRunAttemptsRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.emrserverless.paginators.ListJobRunAttemptsPublisher publisher = client.listJobRunAttemptsPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.emrserverless.paginators.ListJobRunAttemptsPublisher publisher = client.listJobRunAttemptsPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.emrserverless.model.ListJobRunAttemptsResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of maxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listJobRunAttempts(software.amazon.awssdk.services.emrserverless.model.ListJobRunAttemptsRequest)}
* operation.
*
*
*
* This is a convenience which creates an instance of the {@link ListJobRunAttemptsRequest.Builder} avoiding the
* need to create one manually via {@link ListJobRunAttemptsRequest#builder()}
*
*
* @param listJobRunAttemptsRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.emrserverless.model.ListJobRunAttemptsRequest.Builder} to create a
* request.
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ValidationException The input fails to satisfy the constraints specified by an Amazon Web Services
* service.
* - ResourceNotFoundException The specified resource was not found.
* - InternalServerException Request processing failed because of an error or failure with the service.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EmrServerlessException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample EmrServerlessAsyncClient.ListJobRunAttempts
* @see AWS API Documentation
*/
default ListJobRunAttemptsPublisher listJobRunAttemptsPaginator(
Consumer listJobRunAttemptsRequest) {
return listJobRunAttemptsPaginator(ListJobRunAttemptsRequest.builder().applyMutation(listJobRunAttemptsRequest).build());
}
/**
*
* Lists job runs based on a set of parameters.
*
*
* @param listJobRunsRequest
* @return A Java Future containing the result of the ListJobRuns operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ValidationException The input fails to satisfy the constraints specified by an Amazon Web Services
* service.
* - InternalServerException Request processing failed because of an error or failure with the service.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EmrServerlessException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample EmrServerlessAsyncClient.ListJobRuns
* @see AWS
* API Documentation
*/
default CompletableFuture listJobRuns(ListJobRunsRequest listJobRunsRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Lists job runs based on a set of parameters.
*
*
*
* This is a convenience which creates an instance of the {@link ListJobRunsRequest.Builder} avoiding the need to
* create one manually via {@link ListJobRunsRequest#builder()}
*
*
* @param listJobRunsRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.emrserverless.model.ListJobRunsRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the ListJobRuns operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ValidationException The input fails to satisfy the constraints specified by an Amazon Web Services
* service.
* - InternalServerException Request processing failed because of an error or failure with the service.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EmrServerlessException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample EmrServerlessAsyncClient.ListJobRuns
* @see AWS
* API Documentation
*/
default CompletableFuture listJobRuns(Consumer listJobRunsRequest) {
return listJobRuns(ListJobRunsRequest.builder().applyMutation(listJobRunsRequest).build());
}
/**
*
* This is a variant of {@link #listJobRuns(software.amazon.awssdk.services.emrserverless.model.ListJobRunsRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.emrserverless.paginators.ListJobRunsPublisher publisher = client.listJobRunsPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.emrserverless.paginators.ListJobRunsPublisher publisher = client.listJobRunsPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.emrserverless.model.ListJobRunsResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of maxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listJobRuns(software.amazon.awssdk.services.emrserverless.model.ListJobRunsRequest)} operation.
*
*
* @param listJobRunsRequest
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ValidationException The input fails to satisfy the constraints specified by an Amazon Web Services
* service.
* - InternalServerException Request processing failed because of an error or failure with the service.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EmrServerlessException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample EmrServerlessAsyncClient.ListJobRuns
* @see AWS
* API Documentation
*/
default ListJobRunsPublisher listJobRunsPaginator(ListJobRunsRequest listJobRunsRequest) {
return new ListJobRunsPublisher(this, listJobRunsRequest);
}
/**
*
* This is a variant of {@link #listJobRuns(software.amazon.awssdk.services.emrserverless.model.ListJobRunsRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.emrserverless.paginators.ListJobRunsPublisher publisher = client.listJobRunsPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.emrserverless.paginators.ListJobRunsPublisher publisher = client.listJobRunsPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.emrserverless.model.ListJobRunsResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of maxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listJobRuns(software.amazon.awssdk.services.emrserverless.model.ListJobRunsRequest)} operation.
*
*
*
* This is a convenience which creates an instance of the {@link ListJobRunsRequest.Builder} avoiding the need to
* create one manually via {@link ListJobRunsRequest#builder()}
*
*
* @param listJobRunsRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.emrserverless.model.ListJobRunsRequest.Builder} to create a
* request.
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ValidationException The input fails to satisfy the constraints specified by an Amazon Web Services
* service.
* - InternalServerException Request processing failed because of an error or failure with the service.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EmrServerlessException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample EmrServerlessAsyncClient.ListJobRuns
* @see AWS
* API Documentation
*/
default ListJobRunsPublisher listJobRunsPaginator(Consumer listJobRunsRequest) {
return listJobRunsPaginator(ListJobRunsRequest.builder().applyMutation(listJobRunsRequest).build());
}
/**
*
* Lists the tags assigned to the resources.
*
*
* @param listTagsForResourceRequest
* @return A Java Future containing the result of the ListTagsForResource operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ValidationException The input fails to satisfy the constraints specified by an Amazon Web Services
* service.
* - ResourceNotFoundException The specified resource was not found.
* - InternalServerException Request processing failed because of an error or failure with the service.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EmrServerlessException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample EmrServerlessAsyncClient.ListTagsForResource
* @see AWS API Documentation
*/
default CompletableFuture listTagsForResource(
ListTagsForResourceRequest listTagsForResourceRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Lists the tags assigned to the resources.
*
*
*
* This is a convenience which creates an instance of the {@link ListTagsForResourceRequest.Builder} avoiding the
* need to create one manually via {@link ListTagsForResourceRequest#builder()}
*
*
* @param listTagsForResourceRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.emrserverless.model.ListTagsForResourceRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the ListTagsForResource operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ValidationException The input fails to satisfy the constraints specified by an Amazon Web Services
* service.
* - ResourceNotFoundException The specified resource was not found.
* - InternalServerException Request processing failed because of an error or failure with the service.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EmrServerlessException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample EmrServerlessAsyncClient.ListTagsForResource
* @see AWS API Documentation
*/
default CompletableFuture listTagsForResource(
Consumer listTagsForResourceRequest) {
return listTagsForResource(ListTagsForResourceRequest.builder().applyMutation(listTagsForResourceRequest).build());
}
/**
*
* Starts a specified application and initializes initial capacity if configured.
*
*
* @param startApplicationRequest
* @return A Java Future containing the result of the StartApplication operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ValidationException The input fails to satisfy the constraints specified by an Amazon Web Services
* service.
* - ResourceNotFoundException The specified resource was not found.
* - InternalServerException Request processing failed because of an error or failure with the service.
* - ServiceQuotaExceededException The maximum number of resources per account has been reached.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EmrServerlessException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample EmrServerlessAsyncClient.StartApplication
* @see AWS API Documentation
*/
default CompletableFuture startApplication(StartApplicationRequest startApplicationRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Starts a specified application and initializes initial capacity if configured.
*
*
*
* This is a convenience which creates an instance of the {@link StartApplicationRequest.Builder} avoiding the need
* to create one manually via {@link StartApplicationRequest#builder()}
*
*
* @param startApplicationRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.emrserverless.model.StartApplicationRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the StartApplication operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ValidationException The input fails to satisfy the constraints specified by an Amazon Web Services
* service.
* - ResourceNotFoundException The specified resource was not found.
* - InternalServerException Request processing failed because of an error or failure with the service.
* - ServiceQuotaExceededException The maximum number of resources per account has been reached.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EmrServerlessException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample EmrServerlessAsyncClient.StartApplication
* @see AWS API Documentation
*/
default CompletableFuture startApplication(
Consumer startApplicationRequest) {
return startApplication(StartApplicationRequest.builder().applyMutation(startApplicationRequest).build());
}
/**
*
* Starts a job run.
*
*
* @param startJobRunRequest
* @return A Java Future containing the result of the StartJobRun operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ValidationException The input fails to satisfy the constraints specified by an Amazon Web Services
* service.
* - ResourceNotFoundException The specified resource was not found.
* - InternalServerException Request processing failed because of an error or failure with the service.
* - ConflictException The request could not be processed because of conflict in the current state of the
* resource.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EmrServerlessException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample EmrServerlessAsyncClient.StartJobRun
* @see AWS
* API Documentation
*/
default CompletableFuture startJobRun(StartJobRunRequest startJobRunRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Starts a job run.
*
*
*
* This is a convenience which creates an instance of the {@link StartJobRunRequest.Builder} avoiding the need to
* create one manually via {@link StartJobRunRequest#builder()}
*
*
* @param startJobRunRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.emrserverless.model.StartJobRunRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the StartJobRun operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ValidationException The input fails to satisfy the constraints specified by an Amazon Web Services
* service.
* - ResourceNotFoundException The specified resource was not found.
* - InternalServerException Request processing failed because of an error or failure with the service.
* - ConflictException The request could not be processed because of conflict in the current state of the
* resource.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EmrServerlessException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample EmrServerlessAsyncClient.StartJobRun
* @see AWS
* API Documentation
*/
default CompletableFuture startJobRun(Consumer startJobRunRequest) {
return startJobRun(StartJobRunRequest.builder().applyMutation(startJobRunRequest).build());
}
/**
*
* Stops a specified application and releases initial capacity if configured. All scheduled and running jobs must be
* completed or cancelled before stopping an application.
*
*
* @param stopApplicationRequest
* @return A Java Future containing the result of the StopApplication operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ValidationException The input fails to satisfy the constraints specified by an Amazon Web Services
* service.
* - ResourceNotFoundException The specified resource was not found.
* - InternalServerException Request processing failed because of an error or failure with the service.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EmrServerlessException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample EmrServerlessAsyncClient.StopApplication
* @see AWS API Documentation
*/
default CompletableFuture stopApplication(StopApplicationRequest stopApplicationRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Stops a specified application and releases initial capacity if configured. All scheduled and running jobs must be
* completed or cancelled before stopping an application.
*
*
*
* This is a convenience which creates an instance of the {@link StopApplicationRequest.Builder} avoiding the need
* to create one manually via {@link StopApplicationRequest#builder()}
*
*
* @param stopApplicationRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.emrserverless.model.StopApplicationRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the StopApplication operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ValidationException The input fails to satisfy the constraints specified by an Amazon Web Services
* service.
* - ResourceNotFoundException The specified resource was not found.
* - InternalServerException Request processing failed because of an error or failure with the service.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EmrServerlessException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample EmrServerlessAsyncClient.StopApplication
* @see AWS API Documentation
*/
default CompletableFuture stopApplication(
Consumer stopApplicationRequest) {
return stopApplication(StopApplicationRequest.builder().applyMutation(stopApplicationRequest).build());
}
/**
*
* Assigns tags to resources. A tag is a label that you assign to an Amazon Web Services resource. Each tag consists
* of a key and an optional value, both of which you define. Tags enable you to categorize your Amazon Web Services
* resources by attributes such as purpose, owner, or environment. When you have many resources of the same type,
* you can quickly identify a specific resource based on the tags you've assigned to it.
*
*
* @param tagResourceRequest
* @return A Java Future containing the result of the TagResource operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ValidationException The input fails to satisfy the constraints specified by an Amazon Web Services
* service.
* - ResourceNotFoundException The specified resource was not found.
* - InternalServerException Request processing failed because of an error or failure with the service.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EmrServerlessException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample EmrServerlessAsyncClient.TagResource
* @see AWS
* API Documentation
*/
default CompletableFuture tagResource(TagResourceRequest tagResourceRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Assigns tags to resources. A tag is a label that you assign to an Amazon Web Services resource. Each tag consists
* of a key and an optional value, both of which you define. Tags enable you to categorize your Amazon Web Services
* resources by attributes such as purpose, owner, or environment. When you have many resources of the same type,
* you can quickly identify a specific resource based on the tags you've assigned to it.
*
*
*
* This is a convenience which creates an instance of the {@link TagResourceRequest.Builder} avoiding the need to
* create one manually via {@link TagResourceRequest#builder()}
*
*
* @param tagResourceRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.emrserverless.model.TagResourceRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the TagResource operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ValidationException The input fails to satisfy the constraints specified by an Amazon Web Services
* service.
* - ResourceNotFoundException The specified resource was not found.
* - InternalServerException Request processing failed because of an error or failure with the service.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EmrServerlessException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample EmrServerlessAsyncClient.TagResource
* @see AWS
* API Documentation
*/
default CompletableFuture tagResource(Consumer tagResourceRequest) {
return tagResource(TagResourceRequest.builder().applyMutation(tagResourceRequest).build());
}
/**
*
* Removes tags from resources.
*
*
* @param untagResourceRequest
* @return A Java Future containing the result of the UntagResource operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ValidationException The input fails to satisfy the constraints specified by an Amazon Web Services
* service.
* - ResourceNotFoundException The specified resource was not found.
* - InternalServerException Request processing failed because of an error or failure with the service.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EmrServerlessException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample EmrServerlessAsyncClient.UntagResource
* @see AWS
* API Documentation
*/
default CompletableFuture untagResource(UntagResourceRequest untagResourceRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Removes tags from resources.
*
*
*
* This is a convenience which creates an instance of the {@link UntagResourceRequest.Builder} avoiding the need to
* create one manually via {@link UntagResourceRequest#builder()}
*
*
* @param untagResourceRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.emrserverless.model.UntagResourceRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the UntagResource operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ValidationException The input fails to satisfy the constraints specified by an Amazon Web Services
* service.
* - ResourceNotFoundException The specified resource was not found.
* - InternalServerException Request processing failed because of an error or failure with the service.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EmrServerlessException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample EmrServerlessAsyncClient.UntagResource
* @see AWS
* API Documentation
*/
default CompletableFuture untagResource(Consumer untagResourceRequest) {
return untagResource(UntagResourceRequest.builder().applyMutation(untagResourceRequest).build());
}
/**
*
* Updates a specified application. An application has to be in a stopped or created state in order to be updated.
*
*
* @param updateApplicationRequest
* @return A Java Future containing the result of the UpdateApplication operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ValidationException The input fails to satisfy the constraints specified by an Amazon Web Services
* service.
* - ResourceNotFoundException The specified resource was not found.
* - InternalServerException Request processing failed because of an error or failure with the service.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EmrServerlessException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample EmrServerlessAsyncClient.UpdateApplication
* @see AWS API Documentation
*/
default CompletableFuture updateApplication(UpdateApplicationRequest updateApplicationRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Updates a specified application. An application has to be in a stopped or created state in order to be updated.
*
*
*
* This is a convenience which creates an instance of the {@link UpdateApplicationRequest.Builder} avoiding the need
* to create one manually via {@link UpdateApplicationRequest#builder()}
*
*
* @param updateApplicationRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.emrserverless.model.UpdateApplicationRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the UpdateApplication operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ValidationException The input fails to satisfy the constraints specified by an Amazon Web Services
* service.
* - ResourceNotFoundException The specified resource was not found.
* - InternalServerException Request processing failed because of an error or failure with the service.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EmrServerlessException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample EmrServerlessAsyncClient.UpdateApplication
* @see AWS API Documentation
*/
default CompletableFuture updateApplication(
Consumer updateApplicationRequest) {
return updateApplication(UpdateApplicationRequest.builder().applyMutation(updateApplicationRequest).build());
}
@Override
default EmrServerlessServiceClientConfiguration serviceClientConfiguration() {
throw new UnsupportedOperationException();
}
/**
* Create a {@link EmrServerlessAsyncClient} with the region loaded from the
* {@link software.amazon.awssdk.regions.providers.DefaultAwsRegionProviderChain} and credentials loaded from the
* {@link software.amazon.awssdk.auth.credentials.DefaultCredentialsProvider}.
*/
static EmrServerlessAsyncClient create() {
return builder().build();
}
/**
* Create a builder that can be used to configure and create a {@link EmrServerlessAsyncClient}.
*/
static EmrServerlessAsyncClientBuilder builder() {
return new DefaultEmrServerlessAsyncClientBuilder();
}
}