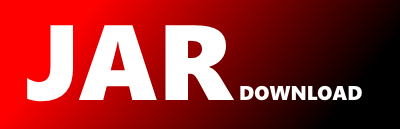
software.amazon.awssdk.services.emrserverless.model.JobRunSummary Maven / Gradle / Ivy
Show all versions of emrserverless Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.emrserverless.model;
import java.io.Serializable;
import java.time.Instant;
import java.util.Arrays;
import java.util.Collections;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* The summary of attributes associated with a job run.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class JobRunSummary implements SdkPojo, Serializable, ToCopyableBuilder {
private static final SdkField APPLICATION_ID_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("applicationId").getter(getter(JobRunSummary::applicationId)).setter(setter(Builder::applicationId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("applicationId").build()).build();
private static final SdkField ID_FIELD = SdkField. builder(MarshallingType.STRING).memberName("id")
.getter(getter(JobRunSummary::id)).setter(setter(Builder::id))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("id").build()).build();
private static final SdkField NAME_FIELD = SdkField. builder(MarshallingType.STRING).memberName("name")
.getter(getter(JobRunSummary::name)).setter(setter(Builder::name))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("name").build()).build();
private static final SdkField MODE_FIELD = SdkField. builder(MarshallingType.STRING).memberName("mode")
.getter(getter(JobRunSummary::modeAsString)).setter(setter(Builder::mode))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("mode").build()).build();
private static final SdkField ARN_FIELD = SdkField. builder(MarshallingType.STRING).memberName("arn")
.getter(getter(JobRunSummary::arn)).setter(setter(Builder::arn))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("arn").build()).build();
private static final SdkField CREATED_BY_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("createdBy").getter(getter(JobRunSummary::createdBy)).setter(setter(Builder::createdBy))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("createdBy").build()).build();
private static final SdkField CREATED_AT_FIELD = SdkField. builder(MarshallingType.INSTANT)
.memberName("createdAt").getter(getter(JobRunSummary::createdAt)).setter(setter(Builder::createdAt))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("createdAt").build()).build();
private static final SdkField UPDATED_AT_FIELD = SdkField. builder(MarshallingType.INSTANT)
.memberName("updatedAt").getter(getter(JobRunSummary::updatedAt)).setter(setter(Builder::updatedAt))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("updatedAt").build()).build();
private static final SdkField EXECUTION_ROLE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("executionRole").getter(getter(JobRunSummary::executionRole)).setter(setter(Builder::executionRole))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("executionRole").build()).build();
private static final SdkField STATE_FIELD = SdkField. builder(MarshallingType.STRING).memberName("state")
.getter(getter(JobRunSummary::stateAsString)).setter(setter(Builder::state))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("state").build()).build();
private static final SdkField STATE_DETAILS_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("stateDetails").getter(getter(JobRunSummary::stateDetails)).setter(setter(Builder::stateDetails))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("stateDetails").build()).build();
private static final SdkField RELEASE_LABEL_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("releaseLabel").getter(getter(JobRunSummary::releaseLabel)).setter(setter(Builder::releaseLabel))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("releaseLabel").build()).build();
private static final SdkField TYPE_FIELD = SdkField. builder(MarshallingType.STRING).memberName("type")
.getter(getter(JobRunSummary::type)).setter(setter(Builder::type))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("type").build()).build();
private static final SdkField ATTEMPT_FIELD = SdkField. builder(MarshallingType.INTEGER)
.memberName("attempt").getter(getter(JobRunSummary::attempt)).setter(setter(Builder::attempt))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("attempt").build()).build();
private static final SdkField ATTEMPT_CREATED_AT_FIELD = SdkField. builder(MarshallingType.INSTANT)
.memberName("attemptCreatedAt").getter(getter(JobRunSummary::attemptCreatedAt))
.setter(setter(Builder::attemptCreatedAt))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("attemptCreatedAt").build()).build();
private static final SdkField ATTEMPT_UPDATED_AT_FIELD = SdkField. builder(MarshallingType.INSTANT)
.memberName("attemptUpdatedAt").getter(getter(JobRunSummary::attemptUpdatedAt))
.setter(setter(Builder::attemptUpdatedAt))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("attemptUpdatedAt").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(APPLICATION_ID_FIELD,
ID_FIELD, NAME_FIELD, MODE_FIELD, ARN_FIELD, CREATED_BY_FIELD, CREATED_AT_FIELD, UPDATED_AT_FIELD,
EXECUTION_ROLE_FIELD, STATE_FIELD, STATE_DETAILS_FIELD, RELEASE_LABEL_FIELD, TYPE_FIELD, ATTEMPT_FIELD,
ATTEMPT_CREATED_AT_FIELD, ATTEMPT_UPDATED_AT_FIELD));
private static final Map> SDK_NAME_TO_FIELD = Collections
.unmodifiableMap(new HashMap>() {
{
put("applicationId", APPLICATION_ID_FIELD);
put("id", ID_FIELD);
put("name", NAME_FIELD);
put("mode", MODE_FIELD);
put("arn", ARN_FIELD);
put("createdBy", CREATED_BY_FIELD);
put("createdAt", CREATED_AT_FIELD);
put("updatedAt", UPDATED_AT_FIELD);
put("executionRole", EXECUTION_ROLE_FIELD);
put("state", STATE_FIELD);
put("stateDetails", STATE_DETAILS_FIELD);
put("releaseLabel", RELEASE_LABEL_FIELD);
put("type", TYPE_FIELD);
put("attempt", ATTEMPT_FIELD);
put("attemptCreatedAt", ATTEMPT_CREATED_AT_FIELD);
put("attemptUpdatedAt", ATTEMPT_UPDATED_AT_FIELD);
}
});
private static final long serialVersionUID = 1L;
private final String applicationId;
private final String id;
private final String name;
private final String mode;
private final String arn;
private final String createdBy;
private final Instant createdAt;
private final Instant updatedAt;
private final String executionRole;
private final String state;
private final String stateDetails;
private final String releaseLabel;
private final String type;
private final Integer attempt;
private final Instant attemptCreatedAt;
private final Instant attemptUpdatedAt;
private JobRunSummary(BuilderImpl builder) {
this.applicationId = builder.applicationId;
this.id = builder.id;
this.name = builder.name;
this.mode = builder.mode;
this.arn = builder.arn;
this.createdBy = builder.createdBy;
this.createdAt = builder.createdAt;
this.updatedAt = builder.updatedAt;
this.executionRole = builder.executionRole;
this.state = builder.state;
this.stateDetails = builder.stateDetails;
this.releaseLabel = builder.releaseLabel;
this.type = builder.type;
this.attempt = builder.attempt;
this.attemptCreatedAt = builder.attemptCreatedAt;
this.attemptUpdatedAt = builder.attemptUpdatedAt;
}
/**
*
* The ID of the application the job is running on.
*
*
* @return The ID of the application the job is running on.
*/
public final String applicationId() {
return applicationId;
}
/**
*
* The ID of the job run.
*
*
* @return The ID of the job run.
*/
public final String id() {
return id;
}
/**
*
* The optional job run name. This doesn't have to be unique.
*
*
* @return The optional job run name. This doesn't have to be unique.
*/
public final String name() {
return name;
}
/**
*
* The mode of the job run.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #mode} will return
* {@link JobRunMode#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #modeAsString}.
*
*
* @return The mode of the job run.
* @see JobRunMode
*/
public final JobRunMode mode() {
return JobRunMode.fromValue(mode);
}
/**
*
* The mode of the job run.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #mode} will return
* {@link JobRunMode#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #modeAsString}.
*
*
* @return The mode of the job run.
* @see JobRunMode
*/
public final String modeAsString() {
return mode;
}
/**
*
* The ARN of the job run.
*
*
* @return The ARN of the job run.
*/
public final String arn() {
return arn;
}
/**
*
* The user who created the job run.
*
*
* @return The user who created the job run.
*/
public final String createdBy() {
return createdBy;
}
/**
*
* The date and time when the job run was created.
*
*
* @return The date and time when the job run was created.
*/
public final Instant createdAt() {
return createdAt;
}
/**
*
* The date and time when the job run was last updated.
*
*
* @return The date and time when the job run was last updated.
*/
public final Instant updatedAt() {
return updatedAt;
}
/**
*
* The execution role ARN of the job run.
*
*
* @return The execution role ARN of the job run.
*/
public final String executionRole() {
return executionRole;
}
/**
*
* The state of the job run.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #state} will return
* {@link JobRunState#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #stateAsString}.
*
*
* @return The state of the job run.
* @see JobRunState
*/
public final JobRunState state() {
return JobRunState.fromValue(state);
}
/**
*
* The state of the job run.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #state} will return
* {@link JobRunState#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #stateAsString}.
*
*
* @return The state of the job run.
* @see JobRunState
*/
public final String stateAsString() {
return state;
}
/**
*
* The state details of the job run.
*
*
* @return The state details of the job run.
*/
public final String stateDetails() {
return stateDetails;
}
/**
*
* The Amazon EMR release associated with the application your job is running on.
*
*
* @return The Amazon EMR release associated with the application your job is running on.
*/
public final String releaseLabel() {
return releaseLabel;
}
/**
*
* The type of job run, such as Spark or Hive.
*
*
* @return The type of job run, such as Spark or Hive.
*/
public final String type() {
return type;
}
/**
*
* The attempt number of the job run execution.
*
*
* @return The attempt number of the job run execution.
*/
public final Integer attempt() {
return attempt;
}
/**
*
* The date and time of when the job run attempt was created.
*
*
* @return The date and time of when the job run attempt was created.
*/
public final Instant attemptCreatedAt() {
return attemptCreatedAt;
}
/**
*
* The date and time of when the job run attempt was last updated.
*
*
* @return The date and time of when the job run attempt was last updated.
*/
public final Instant attemptUpdatedAt() {
return attemptUpdatedAt;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(applicationId());
hashCode = 31 * hashCode + Objects.hashCode(id());
hashCode = 31 * hashCode + Objects.hashCode(name());
hashCode = 31 * hashCode + Objects.hashCode(modeAsString());
hashCode = 31 * hashCode + Objects.hashCode(arn());
hashCode = 31 * hashCode + Objects.hashCode(createdBy());
hashCode = 31 * hashCode + Objects.hashCode(createdAt());
hashCode = 31 * hashCode + Objects.hashCode(updatedAt());
hashCode = 31 * hashCode + Objects.hashCode(executionRole());
hashCode = 31 * hashCode + Objects.hashCode(stateAsString());
hashCode = 31 * hashCode + Objects.hashCode(stateDetails());
hashCode = 31 * hashCode + Objects.hashCode(releaseLabel());
hashCode = 31 * hashCode + Objects.hashCode(type());
hashCode = 31 * hashCode + Objects.hashCode(attempt());
hashCode = 31 * hashCode + Objects.hashCode(attemptCreatedAt());
hashCode = 31 * hashCode + Objects.hashCode(attemptUpdatedAt());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof JobRunSummary)) {
return false;
}
JobRunSummary other = (JobRunSummary) obj;
return Objects.equals(applicationId(), other.applicationId()) && Objects.equals(id(), other.id())
&& Objects.equals(name(), other.name()) && Objects.equals(modeAsString(), other.modeAsString())
&& Objects.equals(arn(), other.arn()) && Objects.equals(createdBy(), other.createdBy())
&& Objects.equals(createdAt(), other.createdAt()) && Objects.equals(updatedAt(), other.updatedAt())
&& Objects.equals(executionRole(), other.executionRole())
&& Objects.equals(stateAsString(), other.stateAsString()) && Objects.equals(stateDetails(), other.stateDetails())
&& Objects.equals(releaseLabel(), other.releaseLabel()) && Objects.equals(type(), other.type())
&& Objects.equals(attempt(), other.attempt()) && Objects.equals(attemptCreatedAt(), other.attemptCreatedAt())
&& Objects.equals(attemptUpdatedAt(), other.attemptUpdatedAt());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("JobRunSummary").add("ApplicationId", applicationId()).add("Id", id()).add("Name", name())
.add("Mode", modeAsString()).add("Arn", arn()).add("CreatedBy", createdBy()).add("CreatedAt", createdAt())
.add("UpdatedAt", updatedAt()).add("ExecutionRole", executionRole()).add("State", stateAsString())
.add("StateDetails", stateDetails()).add("ReleaseLabel", releaseLabel()).add("Type", type())
.add("Attempt", attempt()).add("AttemptCreatedAt", attemptCreatedAt())
.add("AttemptUpdatedAt", attemptUpdatedAt()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "applicationId":
return Optional.ofNullable(clazz.cast(applicationId()));
case "id":
return Optional.ofNullable(clazz.cast(id()));
case "name":
return Optional.ofNullable(clazz.cast(name()));
case "mode":
return Optional.ofNullable(clazz.cast(modeAsString()));
case "arn":
return Optional.ofNullable(clazz.cast(arn()));
case "createdBy":
return Optional.ofNullable(clazz.cast(createdBy()));
case "createdAt":
return Optional.ofNullable(clazz.cast(createdAt()));
case "updatedAt":
return Optional.ofNullable(clazz.cast(updatedAt()));
case "executionRole":
return Optional.ofNullable(clazz.cast(executionRole()));
case "state":
return Optional.ofNullable(clazz.cast(stateAsString()));
case "stateDetails":
return Optional.ofNullable(clazz.cast(stateDetails()));
case "releaseLabel":
return Optional.ofNullable(clazz.cast(releaseLabel()));
case "type":
return Optional.ofNullable(clazz.cast(type()));
case "attempt":
return Optional.ofNullable(clazz.cast(attempt()));
case "attemptCreatedAt":
return Optional.ofNullable(clazz.cast(attemptCreatedAt()));
case "attemptUpdatedAt":
return Optional.ofNullable(clazz.cast(attemptUpdatedAt()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
@Override
public final Map> sdkFieldNameToField() {
return SDK_NAME_TO_FIELD;
}
private static Function