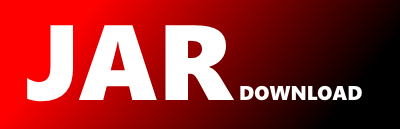
software.amazon.awssdk.services.entityresolution.model.SchemaInputAttribute Maven / Gradle / Ivy
Show all versions of entityresolution Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.entityresolution.model;
import java.io.Serializable;
import java.util.Arrays;
import java.util.Collections;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* An object containing FieldName
, Type
, GroupName
, MatchKey
,
* Hashing
, and SubType
.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class SchemaInputAttribute implements SdkPojo, Serializable,
ToCopyableBuilder {
private static final SdkField FIELD_NAME_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("fieldName").getter(getter(SchemaInputAttribute::fieldName)).setter(setter(Builder::fieldName))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("fieldName").build()).build();
private static final SdkField GROUP_NAME_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("groupName").getter(getter(SchemaInputAttribute::groupName)).setter(setter(Builder::groupName))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("groupName").build()).build();
private static final SdkField HASHED_FIELD = SdkField. builder(MarshallingType.BOOLEAN)
.memberName("hashed").getter(getter(SchemaInputAttribute::hashed)).setter(setter(Builder::hashed))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("hashed").build()).build();
private static final SdkField MATCH_KEY_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("matchKey").getter(getter(SchemaInputAttribute::matchKey)).setter(setter(Builder::matchKey))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("matchKey").build()).build();
private static final SdkField SUB_TYPE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("subType").getter(getter(SchemaInputAttribute::subType)).setter(setter(Builder::subType))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("subType").build()).build();
private static final SdkField TYPE_FIELD = SdkField. builder(MarshallingType.STRING).memberName("type")
.getter(getter(SchemaInputAttribute::typeAsString)).setter(setter(Builder::type))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("type").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(FIELD_NAME_FIELD,
GROUP_NAME_FIELD, HASHED_FIELD, MATCH_KEY_FIELD, SUB_TYPE_FIELD, TYPE_FIELD));
private static final Map> SDK_NAME_TO_FIELD = Collections
.unmodifiableMap(new HashMap>() {
{
put("fieldName", FIELD_NAME_FIELD);
put("groupName", GROUP_NAME_FIELD);
put("hashed", HASHED_FIELD);
put("matchKey", MATCH_KEY_FIELD);
put("subType", SUB_TYPE_FIELD);
put("type", TYPE_FIELD);
}
});
private static final long serialVersionUID = 1L;
private final String fieldName;
private final String groupName;
private final Boolean hashed;
private final String matchKey;
private final String subType;
private final String type;
private SchemaInputAttribute(BuilderImpl builder) {
this.fieldName = builder.fieldName;
this.groupName = builder.groupName;
this.hashed = builder.hashed;
this.matchKey = builder.matchKey;
this.subType = builder.subType;
this.type = builder.type;
}
/**
*
* A string containing the field name.
*
*
* @return A string containing the field name.
*/
public final String fieldName() {
return fieldName;
}
/**
*
* A string that instructs Entity Resolution to combine several columns into a unified column with the identical
* attribute type.
*
*
* For example, when working with columns such as first_name
, middle_name
, and
* last_name
, assigning them a common groupName
will prompt Entity Resolution to
* concatenate them into a single value.
*
*
* @return A string that instructs Entity Resolution to combine several columns into a unified column with the
* identical attribute type.
*
* For example, when working with columns such as first_name
, middle_name
, and
* last_name
, assigning them a common groupName
will prompt Entity Resolution to
* concatenate them into a single value.
*/
public final String groupName() {
return groupName;
}
/**
*
* Indicates if the column values are hashed in the schema input. If the value is set to TRUE
, the
* column values are hashed. If the value is set to FALSE
, the column values are cleartext.
*
*
* @return Indicates if the column values are hashed in the schema input. If the value is set to TRUE
,
* the column values are hashed. If the value is set to FALSE
, the column values are cleartext.
*/
public final Boolean hashed() {
return hashed;
}
/**
*
* A key that allows grouping of multiple input attributes into a unified matching group.
*
*
* For example, consider a scenario where the source table contains various addresses, such as
* business_address
and shipping_address
. By assigning a matchKey
called
* address
to both attributes, Entity Resolution will match records across these fields to create a
* consolidated matching group.
*
*
* If no matchKey
is specified for a column, it won't be utilized for matching purposes but will still
* be included in the output table.
*
*
* @return A key that allows grouping of multiple input attributes into a unified matching group.
*
* For example, consider a scenario where the source table contains various addresses, such as
* business_address
and shipping_address
. By assigning a matchKey
* called address
to both attributes, Entity Resolution will match records across these fields
* to create a consolidated matching group.
*
*
* If no matchKey
is specified for a column, it won't be utilized for matching purposes but
* will still be included in the output table.
*/
public final String matchKey() {
return matchKey;
}
/**
*
* The subtype of the attribute, selected from a list of values.
*
*
* @return The subtype of the attribute, selected from a list of values.
*/
public final String subType() {
return subType;
}
/**
*
* The type of the attribute, selected from a list of values.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #type} will return
* {@link SchemaAttributeType#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #typeAsString}.
*
*
* @return The type of the attribute, selected from a list of values.
* @see SchemaAttributeType
*/
public final SchemaAttributeType type() {
return SchemaAttributeType.fromValue(type);
}
/**
*
* The type of the attribute, selected from a list of values.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #type} will return
* {@link SchemaAttributeType#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #typeAsString}.
*
*
* @return The type of the attribute, selected from a list of values.
* @see SchemaAttributeType
*/
public final String typeAsString() {
return type;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(fieldName());
hashCode = 31 * hashCode + Objects.hashCode(groupName());
hashCode = 31 * hashCode + Objects.hashCode(hashed());
hashCode = 31 * hashCode + Objects.hashCode(matchKey());
hashCode = 31 * hashCode + Objects.hashCode(subType());
hashCode = 31 * hashCode + Objects.hashCode(typeAsString());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof SchemaInputAttribute)) {
return false;
}
SchemaInputAttribute other = (SchemaInputAttribute) obj;
return Objects.equals(fieldName(), other.fieldName()) && Objects.equals(groupName(), other.groupName())
&& Objects.equals(hashed(), other.hashed()) && Objects.equals(matchKey(), other.matchKey())
&& Objects.equals(subType(), other.subType()) && Objects.equals(typeAsString(), other.typeAsString());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("SchemaInputAttribute").add("FieldName", fieldName()).add("GroupName", groupName())
.add("Hashed", hashed()).add("MatchKey", matchKey()).add("SubType", subType()).add("Type", typeAsString())
.build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "fieldName":
return Optional.ofNullable(clazz.cast(fieldName()));
case "groupName":
return Optional.ofNullable(clazz.cast(groupName()));
case "hashed":
return Optional.ofNullable(clazz.cast(hashed()));
case "matchKey":
return Optional.ofNullable(clazz.cast(matchKey()));
case "subType":
return Optional.ofNullable(clazz.cast(subType()));
case "type":
return Optional.ofNullable(clazz.cast(typeAsString()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
@Override
public final Map> sdkFieldNameToField() {
return SDK_NAME_TO_FIELD;
}
private static Function