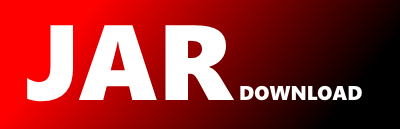
software.amazon.awssdk.services.entityresolution.model.GetProviderServiceResponse Maven / Gradle / Ivy
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.entityresolution.model;
import java.util.Arrays;
import java.util.Collections;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.document.Document;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*/
@Generated("software.amazon.awssdk:codegen")
public final class GetProviderServiceResponse extends EntityResolutionResponse implements
ToCopyableBuilder {
private static final SdkField ANONYMIZED_OUTPUT_FIELD = SdkField. builder(MarshallingType.BOOLEAN)
.memberName("anonymizedOutput").getter(getter(GetProviderServiceResponse::anonymizedOutput))
.setter(setter(Builder::anonymizedOutput))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("anonymizedOutput").build()).build();
private static final SdkField PROVIDER_COMPONENT_SCHEMA_FIELD = SdkField
. builder(MarshallingType.SDK_POJO).memberName("providerComponentSchema")
.getter(getter(GetProviderServiceResponse::providerComponentSchema)).setter(setter(Builder::providerComponentSchema))
.constructor(ProviderComponentSchema::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("providerComponentSchema").build())
.build();
private static final SdkField PROVIDER_CONFIGURATION_DEFINITION_FIELD = SdkField
. builder(MarshallingType.DOCUMENT)
.memberName("providerConfigurationDefinition")
.getter(getter(GetProviderServiceResponse::providerConfigurationDefinition))
.setter(setter(Builder::providerConfigurationDefinition))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("providerConfigurationDefinition")
.build()).build();
private static final SdkField PROVIDER_ENDPOINT_CONFIGURATION_FIELD = SdkField
. builder(MarshallingType.SDK_POJO)
.memberName("providerEndpointConfiguration")
.getter(getter(GetProviderServiceResponse::providerEndpointConfiguration))
.setter(setter(Builder::providerEndpointConfiguration))
.constructor(ProviderEndpointConfiguration::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("providerEndpointConfiguration")
.build()).build();
private static final SdkField PROVIDER_ENTITY_OUTPUT_DEFINITION_FIELD = SdkField
. builder(MarshallingType.DOCUMENT)
.memberName("providerEntityOutputDefinition")
.getter(getter(GetProviderServiceResponse::providerEntityOutputDefinition))
.setter(setter(Builder::providerEntityOutputDefinition))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("providerEntityOutputDefinition")
.build()).build();
private static final SdkField PROVIDER_ID_NAME_SPACE_CONFIGURATION_FIELD = SdkField
. builder(MarshallingType.SDK_POJO)
.memberName("providerIdNameSpaceConfiguration")
.getter(getter(GetProviderServiceResponse::providerIdNameSpaceConfiguration))
.setter(setter(Builder::providerIdNameSpaceConfiguration))
.constructor(ProviderIdNameSpaceConfiguration::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("providerIdNameSpaceConfiguration")
.build()).build();
private static final SdkField PROVIDER_INTERMEDIATE_DATA_ACCESS_CONFIGURATION_FIELD = SdkField
. builder(MarshallingType.SDK_POJO)
.memberName("providerIntermediateDataAccessConfiguration")
.getter(getter(GetProviderServiceResponse::providerIntermediateDataAccessConfiguration))
.setter(setter(Builder::providerIntermediateDataAccessConfiguration))
.constructor(ProviderIntermediateDataAccessConfiguration::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("providerIntermediateDataAccessConfiguration").build()).build();
private static final SdkField PROVIDER_JOB_CONFIGURATION_FIELD = SdkField
. builder(MarshallingType.DOCUMENT).memberName("providerJobConfiguration")
.getter(getter(GetProviderServiceResponse::providerJobConfiguration))
.setter(setter(Builder::providerJobConfiguration))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("providerJobConfiguration").build())
.build();
private static final SdkField PROVIDER_NAME_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("providerName").getter(getter(GetProviderServiceResponse::providerName))
.setter(setter(Builder::providerName))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("providerName").build()).build();
private static final SdkField PROVIDER_SERVICE_ARN_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("providerServiceArn").getter(getter(GetProviderServiceResponse::providerServiceArn))
.setter(setter(Builder::providerServiceArn))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("providerServiceArn").build())
.build();
private static final SdkField PROVIDER_SERVICE_DISPLAY_NAME_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("providerServiceDisplayName")
.getter(getter(GetProviderServiceResponse::providerServiceDisplayName))
.setter(setter(Builder::providerServiceDisplayName))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("providerServiceDisplayName").build())
.build();
private static final SdkField PROVIDER_SERVICE_NAME_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("providerServiceName").getter(getter(GetProviderServiceResponse::providerServiceName))
.setter(setter(Builder::providerServiceName))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("providerServiceName").build())
.build();
private static final SdkField PROVIDER_SERVICE_TYPE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("providerServiceType").getter(getter(GetProviderServiceResponse::providerServiceTypeAsString))
.setter(setter(Builder::providerServiceType))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("providerServiceType").build())
.build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(ANONYMIZED_OUTPUT_FIELD,
PROVIDER_COMPONENT_SCHEMA_FIELD, PROVIDER_CONFIGURATION_DEFINITION_FIELD, PROVIDER_ENDPOINT_CONFIGURATION_FIELD,
PROVIDER_ENTITY_OUTPUT_DEFINITION_FIELD, PROVIDER_ID_NAME_SPACE_CONFIGURATION_FIELD,
PROVIDER_INTERMEDIATE_DATA_ACCESS_CONFIGURATION_FIELD, PROVIDER_JOB_CONFIGURATION_FIELD, PROVIDER_NAME_FIELD,
PROVIDER_SERVICE_ARN_FIELD, PROVIDER_SERVICE_DISPLAY_NAME_FIELD, PROVIDER_SERVICE_NAME_FIELD,
PROVIDER_SERVICE_TYPE_FIELD));
private static final Map> SDK_NAME_TO_FIELD = memberNameToFieldInitializer();
private final Boolean anonymizedOutput;
private final ProviderComponentSchema providerComponentSchema;
private final Document providerConfigurationDefinition;
private final ProviderEndpointConfiguration providerEndpointConfiguration;
private final Document providerEntityOutputDefinition;
private final ProviderIdNameSpaceConfiguration providerIdNameSpaceConfiguration;
private final ProviderIntermediateDataAccessConfiguration providerIntermediateDataAccessConfiguration;
private final Document providerJobConfiguration;
private final String providerName;
private final String providerServiceArn;
private final String providerServiceDisplayName;
private final String providerServiceName;
private final String providerServiceType;
private GetProviderServiceResponse(BuilderImpl builder) {
super(builder);
this.anonymizedOutput = builder.anonymizedOutput;
this.providerComponentSchema = builder.providerComponentSchema;
this.providerConfigurationDefinition = builder.providerConfigurationDefinition;
this.providerEndpointConfiguration = builder.providerEndpointConfiguration;
this.providerEntityOutputDefinition = builder.providerEntityOutputDefinition;
this.providerIdNameSpaceConfiguration = builder.providerIdNameSpaceConfiguration;
this.providerIntermediateDataAccessConfiguration = builder.providerIntermediateDataAccessConfiguration;
this.providerJobConfiguration = builder.providerJobConfiguration;
this.providerName = builder.providerName;
this.providerServiceArn = builder.providerServiceArn;
this.providerServiceDisplayName = builder.providerServiceDisplayName;
this.providerServiceName = builder.providerServiceName;
this.providerServiceType = builder.providerServiceType;
}
/**
*
* Specifies whether output data from the provider is anonymized. A value of TRUE
means the output will
* be anonymized and you can't relate the data that comes back from the provider to the identifying input. A value
* of FALSE
means the output won't be anonymized and you can relate the data that comes back from the
* provider to your source data.
*
*
* @return Specifies whether output data from the provider is anonymized. A value of TRUE
means the
* output will be anonymized and you can't relate the data that comes back from the provider to the
* identifying input. A value of FALSE
means the output won't be anonymized and you can relate
* the data that comes back from the provider to your source data.
*/
public final Boolean anonymizedOutput() {
return anonymizedOutput;
}
/**
*
* Input schema for the provider service.
*
*
* @return Input schema for the provider service.
*/
public final ProviderComponentSchema providerComponentSchema() {
return providerComponentSchema;
}
/**
*
* The definition of the provider configuration.
*
*
* @return The definition of the provider configuration.
*/
public final Document providerConfigurationDefinition() {
return providerConfigurationDefinition;
}
/**
*
* The required configuration fields to use with the provider service.
*
*
* @return The required configuration fields to use with the provider service.
*/
public final ProviderEndpointConfiguration providerEndpointConfiguration() {
return providerEndpointConfiguration;
}
/**
*
* The definition of the provider entity output.
*
*
* @return The definition of the provider entity output.
*/
public final Document providerEntityOutputDefinition() {
return providerEntityOutputDefinition;
}
/**
*
* The provider configuration required for different ID namespace types.
*
*
* @return The provider configuration required for different ID namespace types.
*/
public final ProviderIdNameSpaceConfiguration providerIdNameSpaceConfiguration() {
return providerIdNameSpaceConfiguration;
}
/**
*
* The Amazon Web Services accounts and the S3 permissions that are required by some providers to create an S3
* bucket for intermediate data storage.
*
*
* @return The Amazon Web Services accounts and the S3 permissions that are required by some providers to create an
* S3 bucket for intermediate data storage.
*/
public final ProviderIntermediateDataAccessConfiguration providerIntermediateDataAccessConfiguration() {
return providerIntermediateDataAccessConfiguration;
}
/**
*
* Provider service job configurations.
*
*
* @return Provider service job configurations.
*/
public final Document providerJobConfiguration() {
return providerJobConfiguration;
}
/**
*
* The name of the provider. This name is typically the company name.
*
*
* @return The name of the provider. This name is typically the company name.
*/
public final String providerName() {
return providerName;
}
/**
*
* The ARN (Amazon Resource Name) that Entity Resolution generated for the provider service.
*
*
* @return The ARN (Amazon Resource Name) that Entity Resolution generated for the provider service.
*/
public final String providerServiceArn() {
return providerServiceArn;
}
/**
*
* The display name of the provider service.
*
*
* @return The display name of the provider service.
*/
public final String providerServiceDisplayName() {
return providerServiceDisplayName;
}
/**
*
* The name of the product that the provider service provides.
*
*
* @return The name of the product that the provider service provides.
*/
public final String providerServiceName() {
return providerServiceName;
}
/**
*
* The type of provider service.
*
*
* If the service returns an enum value that is not available in the current SDK version,
* {@link #providerServiceType} will return {@link ServiceType#UNKNOWN_TO_SDK_VERSION}. The raw value returned by
* the service is available from {@link #providerServiceTypeAsString}.
*
*
* @return The type of provider service.
* @see ServiceType
*/
public final ServiceType providerServiceType() {
return ServiceType.fromValue(providerServiceType);
}
/**
*
* The type of provider service.
*
*
* If the service returns an enum value that is not available in the current SDK version,
* {@link #providerServiceType} will return {@link ServiceType#UNKNOWN_TO_SDK_VERSION}. The raw value returned by
* the service is available from {@link #providerServiceTypeAsString}.
*
*
* @return The type of provider service.
* @see ServiceType
*/
public final String providerServiceTypeAsString() {
return providerServiceType;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + super.hashCode();
hashCode = 31 * hashCode + Objects.hashCode(anonymizedOutput());
hashCode = 31 * hashCode + Objects.hashCode(providerComponentSchema());
hashCode = 31 * hashCode + Objects.hashCode(providerConfigurationDefinition());
hashCode = 31 * hashCode + Objects.hashCode(providerEndpointConfiguration());
hashCode = 31 * hashCode + Objects.hashCode(providerEntityOutputDefinition());
hashCode = 31 * hashCode + Objects.hashCode(providerIdNameSpaceConfiguration());
hashCode = 31 * hashCode + Objects.hashCode(providerIntermediateDataAccessConfiguration());
hashCode = 31 * hashCode + Objects.hashCode(providerJobConfiguration());
hashCode = 31 * hashCode + Objects.hashCode(providerName());
hashCode = 31 * hashCode + Objects.hashCode(providerServiceArn());
hashCode = 31 * hashCode + Objects.hashCode(providerServiceDisplayName());
hashCode = 31 * hashCode + Objects.hashCode(providerServiceName());
hashCode = 31 * hashCode + Objects.hashCode(providerServiceTypeAsString());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return super.equals(obj) && equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof GetProviderServiceResponse)) {
return false;
}
GetProviderServiceResponse other = (GetProviderServiceResponse) obj;
return Objects.equals(anonymizedOutput(), other.anonymizedOutput())
&& Objects.equals(providerComponentSchema(), other.providerComponentSchema())
&& Objects.equals(providerConfigurationDefinition(), other.providerConfigurationDefinition())
&& Objects.equals(providerEndpointConfiguration(), other.providerEndpointConfiguration())
&& Objects.equals(providerEntityOutputDefinition(), other.providerEntityOutputDefinition())
&& Objects.equals(providerIdNameSpaceConfiguration(), other.providerIdNameSpaceConfiguration())
&& Objects.equals(providerIntermediateDataAccessConfiguration(),
other.providerIntermediateDataAccessConfiguration())
&& Objects.equals(providerJobConfiguration(), other.providerJobConfiguration())
&& Objects.equals(providerName(), other.providerName())
&& Objects.equals(providerServiceArn(), other.providerServiceArn())
&& Objects.equals(providerServiceDisplayName(), other.providerServiceDisplayName())
&& Objects.equals(providerServiceName(), other.providerServiceName())
&& Objects.equals(providerServiceTypeAsString(), other.providerServiceTypeAsString());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("GetProviderServiceResponse").add("AnonymizedOutput", anonymizedOutput())
.add("ProviderComponentSchema", providerComponentSchema())
.add("ProviderConfigurationDefinition", providerConfigurationDefinition())
.add("ProviderEndpointConfiguration", providerEndpointConfiguration())
.add("ProviderEntityOutputDefinition", providerEntityOutputDefinition())
.add("ProviderIdNameSpaceConfiguration", providerIdNameSpaceConfiguration())
.add("ProviderIntermediateDataAccessConfiguration", providerIntermediateDataAccessConfiguration())
.add("ProviderJobConfiguration", providerJobConfiguration()).add("ProviderName", providerName())
.add("ProviderServiceArn", providerServiceArn()).add("ProviderServiceDisplayName", providerServiceDisplayName())
.add("ProviderServiceName", providerServiceName()).add("ProviderServiceType", providerServiceTypeAsString())
.build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "anonymizedOutput":
return Optional.ofNullable(clazz.cast(anonymizedOutput()));
case "providerComponentSchema":
return Optional.ofNullable(clazz.cast(providerComponentSchema()));
case "providerConfigurationDefinition":
return Optional.ofNullable(clazz.cast(providerConfigurationDefinition()));
case "providerEndpointConfiguration":
return Optional.ofNullable(clazz.cast(providerEndpointConfiguration()));
case "providerEntityOutputDefinition":
return Optional.ofNullable(clazz.cast(providerEntityOutputDefinition()));
case "providerIdNameSpaceConfiguration":
return Optional.ofNullable(clazz.cast(providerIdNameSpaceConfiguration()));
case "providerIntermediateDataAccessConfiguration":
return Optional.ofNullable(clazz.cast(providerIntermediateDataAccessConfiguration()));
case "providerJobConfiguration":
return Optional.ofNullable(clazz.cast(providerJobConfiguration()));
case "providerName":
return Optional.ofNullable(clazz.cast(providerName()));
case "providerServiceArn":
return Optional.ofNullable(clazz.cast(providerServiceArn()));
case "providerServiceDisplayName":
return Optional.ofNullable(clazz.cast(providerServiceDisplayName()));
case "providerServiceName":
return Optional.ofNullable(clazz.cast(providerServiceName()));
case "providerServiceType":
return Optional.ofNullable(clazz.cast(providerServiceTypeAsString()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
@Override
public final Map> sdkFieldNameToField() {
return SDK_NAME_TO_FIELD;
}
private static Map> memberNameToFieldInitializer() {
Map> map = new HashMap<>();
map.put("anonymizedOutput", ANONYMIZED_OUTPUT_FIELD);
map.put("providerComponentSchema", PROVIDER_COMPONENT_SCHEMA_FIELD);
map.put("providerConfigurationDefinition", PROVIDER_CONFIGURATION_DEFINITION_FIELD);
map.put("providerEndpointConfiguration", PROVIDER_ENDPOINT_CONFIGURATION_FIELD);
map.put("providerEntityOutputDefinition", PROVIDER_ENTITY_OUTPUT_DEFINITION_FIELD);
map.put("providerIdNameSpaceConfiguration", PROVIDER_ID_NAME_SPACE_CONFIGURATION_FIELD);
map.put("providerIntermediateDataAccessConfiguration", PROVIDER_INTERMEDIATE_DATA_ACCESS_CONFIGURATION_FIELD);
map.put("providerJobConfiguration", PROVIDER_JOB_CONFIGURATION_FIELD);
map.put("providerName", PROVIDER_NAME_FIELD);
map.put("providerServiceArn", PROVIDER_SERVICE_ARN_FIELD);
map.put("providerServiceDisplayName", PROVIDER_SERVICE_DISPLAY_NAME_FIELD);
map.put("providerServiceName", PROVIDER_SERVICE_NAME_FIELD);
map.put("providerServiceType", PROVIDER_SERVICE_TYPE_FIELD);
return Collections.unmodifiableMap(map);
}
private static Function