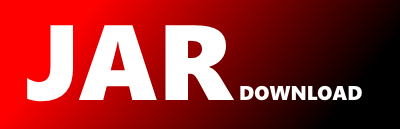
software.amazon.awssdk.services.entityresolution.model.UpdateMatchingWorkflowResponse Maven / Gradle / Ivy
Show all versions of entityresolution Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.entityresolution.model;
import java.util.Arrays;
import java.util.Collection;
import java.util.Collections;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import java.util.stream.Collectors;
import java.util.stream.Stream;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.ListTrait;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.core.util.DefaultSdkAutoConstructList;
import software.amazon.awssdk.core.util.SdkAutoConstructList;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*/
@Generated("software.amazon.awssdk:codegen")
public final class UpdateMatchingWorkflowResponse extends EntityResolutionResponse implements
ToCopyableBuilder {
private static final SdkField DESCRIPTION_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("description").getter(getter(UpdateMatchingWorkflowResponse::description))
.setter(setter(Builder::description))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("description").build()).build();
private static final SdkField INCREMENTAL_RUN_CONFIG_FIELD = SdkField
. builder(MarshallingType.SDK_POJO).memberName("incrementalRunConfig")
.getter(getter(UpdateMatchingWorkflowResponse::incrementalRunConfig)).setter(setter(Builder::incrementalRunConfig))
.constructor(IncrementalRunConfig::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("incrementalRunConfig").build())
.build();
private static final SdkField> INPUT_SOURCE_CONFIG_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("inputSourceConfig")
.getter(getter(UpdateMatchingWorkflowResponse::inputSourceConfig))
.setter(setter(Builder::inputSourceConfig))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("inputSourceConfig").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(InputSource::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField> OUTPUT_SOURCE_CONFIG_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("outputSourceConfig")
.getter(getter(UpdateMatchingWorkflowResponse::outputSourceConfig))
.setter(setter(Builder::outputSourceConfig))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("outputSourceConfig").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(OutputSource::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField RESOLUTION_TECHNIQUES_FIELD = SdkField
. builder(MarshallingType.SDK_POJO).memberName("resolutionTechniques")
.getter(getter(UpdateMatchingWorkflowResponse::resolutionTechniques)).setter(setter(Builder::resolutionTechniques))
.constructor(ResolutionTechniques::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("resolutionTechniques").build())
.build();
private static final SdkField ROLE_ARN_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("roleArn").getter(getter(UpdateMatchingWorkflowResponse::roleArn)).setter(setter(Builder::roleArn))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("roleArn").build()).build();
private static final SdkField WORKFLOW_NAME_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("workflowName").getter(getter(UpdateMatchingWorkflowResponse::workflowName))
.setter(setter(Builder::workflowName))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("workflowName").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(DESCRIPTION_FIELD,
INCREMENTAL_RUN_CONFIG_FIELD, INPUT_SOURCE_CONFIG_FIELD, OUTPUT_SOURCE_CONFIG_FIELD, RESOLUTION_TECHNIQUES_FIELD,
ROLE_ARN_FIELD, WORKFLOW_NAME_FIELD));
private static final Map> SDK_NAME_TO_FIELD = memberNameToFieldInitializer();
private final String description;
private final IncrementalRunConfig incrementalRunConfig;
private final List inputSourceConfig;
private final List outputSourceConfig;
private final ResolutionTechniques resolutionTechniques;
private final String roleArn;
private final String workflowName;
private UpdateMatchingWorkflowResponse(BuilderImpl builder) {
super(builder);
this.description = builder.description;
this.incrementalRunConfig = builder.incrementalRunConfig;
this.inputSourceConfig = builder.inputSourceConfig;
this.outputSourceConfig = builder.outputSourceConfig;
this.resolutionTechniques = builder.resolutionTechniques;
this.roleArn = builder.roleArn;
this.workflowName = builder.workflowName;
}
/**
*
* A description of the workflow.
*
*
* @return A description of the workflow.
*/
public final String description() {
return description;
}
/**
*
* An object which defines an incremental run type and has only incrementalRunType
as a field.
*
*
* @return An object which defines an incremental run type and has only incrementalRunType
as a field.
*/
public final IncrementalRunConfig incrementalRunConfig() {
return incrementalRunConfig;
}
/**
* For responses, this returns true if the service returned a value for the InputSourceConfig property. This DOES
* NOT check that the value is non-empty (for which, you should check the {@code isEmpty()} method on the property).
* This is useful because the SDK will never return a null collection or map, but you may need to differentiate
* between the service returning nothing (or null) and the service returning an empty collection or map. For
* requests, this returns true if a value for the property was specified in the request builder, and false if a
* value was not specified.
*/
public final boolean hasInputSourceConfig() {
return inputSourceConfig != null && !(inputSourceConfig instanceof SdkAutoConstructList);
}
/**
*
* A list of InputSource
objects, which have the fields InputSourceARN
and
* SchemaName
.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasInputSourceConfig} method.
*
*
* @return A list of InputSource
objects, which have the fields InputSourceARN
and
* SchemaName
.
*/
public final List inputSourceConfig() {
return inputSourceConfig;
}
/**
* For responses, this returns true if the service returned a value for the OutputSourceConfig property. This DOES
* NOT check that the value is non-empty (for which, you should check the {@code isEmpty()} method on the property).
* This is useful because the SDK will never return a null collection or map, but you may need to differentiate
* between the service returning nothing (or null) and the service returning an empty collection or map. For
* requests, this returns true if a value for the property was specified in the request builder, and false if a
* value was not specified.
*/
public final boolean hasOutputSourceConfig() {
return outputSourceConfig != null && !(outputSourceConfig instanceof SdkAutoConstructList);
}
/**
*
* A list of OutputSource
objects, each of which contains fields OutputS3Path
,
* ApplyNormalization
, and Output
.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasOutputSourceConfig} method.
*
*
* @return A list of OutputSource
objects, each of which contains fields OutputS3Path
,
* ApplyNormalization
, and Output
.
*/
public final List outputSourceConfig() {
return outputSourceConfig;
}
/**
*
* An object which defines the resolutionType
and the ruleBasedProperties
*
*
* @return An object which defines the resolutionType
and the ruleBasedProperties
*/
public final ResolutionTechniques resolutionTechniques() {
return resolutionTechniques;
}
/**
*
* The Amazon Resource Name (ARN) of the IAM role. Entity Resolution assumes this role to create resources on your
* behalf as part of workflow execution.
*
*
* @return The Amazon Resource Name (ARN) of the IAM role. Entity Resolution assumes this role to create resources
* on your behalf as part of workflow execution.
*/
public final String roleArn() {
return roleArn;
}
/**
*
* The name of the workflow.
*
*
* @return The name of the workflow.
*/
public final String workflowName() {
return workflowName;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + super.hashCode();
hashCode = 31 * hashCode + Objects.hashCode(description());
hashCode = 31 * hashCode + Objects.hashCode(incrementalRunConfig());
hashCode = 31 * hashCode + Objects.hashCode(hasInputSourceConfig() ? inputSourceConfig() : null);
hashCode = 31 * hashCode + Objects.hashCode(hasOutputSourceConfig() ? outputSourceConfig() : null);
hashCode = 31 * hashCode + Objects.hashCode(resolutionTechniques());
hashCode = 31 * hashCode + Objects.hashCode(roleArn());
hashCode = 31 * hashCode + Objects.hashCode(workflowName());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return super.equals(obj) && equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof UpdateMatchingWorkflowResponse)) {
return false;
}
UpdateMatchingWorkflowResponse other = (UpdateMatchingWorkflowResponse) obj;
return Objects.equals(description(), other.description())
&& Objects.equals(incrementalRunConfig(), other.incrementalRunConfig())
&& hasInputSourceConfig() == other.hasInputSourceConfig()
&& Objects.equals(inputSourceConfig(), other.inputSourceConfig())
&& hasOutputSourceConfig() == other.hasOutputSourceConfig()
&& Objects.equals(outputSourceConfig(), other.outputSourceConfig())
&& Objects.equals(resolutionTechniques(), other.resolutionTechniques())
&& Objects.equals(roleArn(), other.roleArn()) && Objects.equals(workflowName(), other.workflowName());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("UpdateMatchingWorkflowResponse").add("Description", description())
.add("IncrementalRunConfig", incrementalRunConfig())
.add("InputSourceConfig", hasInputSourceConfig() ? inputSourceConfig() : null)
.add("OutputSourceConfig", hasOutputSourceConfig() ? outputSourceConfig() : null)
.add("ResolutionTechniques", resolutionTechniques()).add("RoleArn", roleArn())
.add("WorkflowName", workflowName()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "description":
return Optional.ofNullable(clazz.cast(description()));
case "incrementalRunConfig":
return Optional.ofNullable(clazz.cast(incrementalRunConfig()));
case "inputSourceConfig":
return Optional.ofNullable(clazz.cast(inputSourceConfig()));
case "outputSourceConfig":
return Optional.ofNullable(clazz.cast(outputSourceConfig()));
case "resolutionTechniques":
return Optional.ofNullable(clazz.cast(resolutionTechniques()));
case "roleArn":
return Optional.ofNullable(clazz.cast(roleArn()));
case "workflowName":
return Optional.ofNullable(clazz.cast(workflowName()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
@Override
public final Map> sdkFieldNameToField() {
return SDK_NAME_TO_FIELD;
}
private static Map> memberNameToFieldInitializer() {
Map> map = new HashMap<>();
map.put("description", DESCRIPTION_FIELD);
map.put("incrementalRunConfig", INCREMENTAL_RUN_CONFIG_FIELD);
map.put("inputSourceConfig", INPUT_SOURCE_CONFIG_FIELD);
map.put("outputSourceConfig", OUTPUT_SOURCE_CONFIG_FIELD);
map.put("resolutionTechniques", RESOLUTION_TECHNIQUES_FIELD);
map.put("roleArn", ROLE_ARN_FIELD);
map.put("workflowName", WORKFLOW_NAME_FIELD);
return Collections.unmodifiableMap(map);
}
private static Function