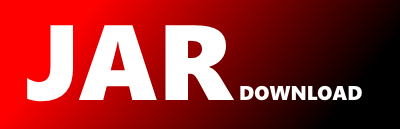
software.amazon.awssdk.services.entityresolution.DefaultEntityResolutionClient Maven / Gradle / Ivy
Show all versions of entityresolution Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.entityresolution;
import java.util.Collections;
import java.util.List;
import java.util.function.Consumer;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.annotations.SdkInternalApi;
import software.amazon.awssdk.awscore.client.handler.AwsSyncClientHandler;
import software.amazon.awssdk.awscore.exception.AwsServiceException;
import software.amazon.awssdk.awscore.internal.AwsProtocolMetadata;
import software.amazon.awssdk.awscore.internal.AwsServiceProtocol;
import software.amazon.awssdk.awscore.retry.AwsRetryStrategy;
import software.amazon.awssdk.core.RequestOverrideConfiguration;
import software.amazon.awssdk.core.SdkPlugin;
import software.amazon.awssdk.core.SdkRequest;
import software.amazon.awssdk.core.client.config.ClientOverrideConfiguration;
import software.amazon.awssdk.core.client.config.SdkClientConfiguration;
import software.amazon.awssdk.core.client.config.SdkClientOption;
import software.amazon.awssdk.core.client.handler.ClientExecutionParams;
import software.amazon.awssdk.core.client.handler.SyncClientHandler;
import software.amazon.awssdk.core.exception.SdkClientException;
import software.amazon.awssdk.core.http.HttpResponseHandler;
import software.amazon.awssdk.core.metrics.CoreMetric;
import software.amazon.awssdk.core.retry.RetryMode;
import software.amazon.awssdk.metrics.MetricCollector;
import software.amazon.awssdk.metrics.MetricPublisher;
import software.amazon.awssdk.metrics.NoOpMetricCollector;
import software.amazon.awssdk.protocols.core.ExceptionMetadata;
import software.amazon.awssdk.protocols.json.AwsJsonProtocol;
import software.amazon.awssdk.protocols.json.AwsJsonProtocolFactory;
import software.amazon.awssdk.protocols.json.BaseAwsJsonProtocolFactory;
import software.amazon.awssdk.protocols.json.JsonOperationMetadata;
import software.amazon.awssdk.retries.api.RetryStrategy;
import software.amazon.awssdk.services.entityresolution.internal.EntityResolutionServiceClientConfigurationBuilder;
import software.amazon.awssdk.services.entityresolution.model.AccessDeniedException;
import software.amazon.awssdk.services.entityresolution.model.AddPolicyStatementRequest;
import software.amazon.awssdk.services.entityresolution.model.AddPolicyStatementResponse;
import software.amazon.awssdk.services.entityresolution.model.BatchDeleteUniqueIdRequest;
import software.amazon.awssdk.services.entityresolution.model.BatchDeleteUniqueIdResponse;
import software.amazon.awssdk.services.entityresolution.model.ConflictException;
import software.amazon.awssdk.services.entityresolution.model.CreateIdMappingWorkflowRequest;
import software.amazon.awssdk.services.entityresolution.model.CreateIdMappingWorkflowResponse;
import software.amazon.awssdk.services.entityresolution.model.CreateIdNamespaceRequest;
import software.amazon.awssdk.services.entityresolution.model.CreateIdNamespaceResponse;
import software.amazon.awssdk.services.entityresolution.model.CreateMatchingWorkflowRequest;
import software.amazon.awssdk.services.entityresolution.model.CreateMatchingWorkflowResponse;
import software.amazon.awssdk.services.entityresolution.model.CreateSchemaMappingRequest;
import software.amazon.awssdk.services.entityresolution.model.CreateSchemaMappingResponse;
import software.amazon.awssdk.services.entityresolution.model.DeleteIdMappingWorkflowRequest;
import software.amazon.awssdk.services.entityresolution.model.DeleteIdMappingWorkflowResponse;
import software.amazon.awssdk.services.entityresolution.model.DeleteIdNamespaceRequest;
import software.amazon.awssdk.services.entityresolution.model.DeleteIdNamespaceResponse;
import software.amazon.awssdk.services.entityresolution.model.DeleteMatchingWorkflowRequest;
import software.amazon.awssdk.services.entityresolution.model.DeleteMatchingWorkflowResponse;
import software.amazon.awssdk.services.entityresolution.model.DeletePolicyStatementRequest;
import software.amazon.awssdk.services.entityresolution.model.DeletePolicyStatementResponse;
import software.amazon.awssdk.services.entityresolution.model.DeleteSchemaMappingRequest;
import software.amazon.awssdk.services.entityresolution.model.DeleteSchemaMappingResponse;
import software.amazon.awssdk.services.entityresolution.model.EntityResolutionException;
import software.amazon.awssdk.services.entityresolution.model.ExceedsLimitException;
import software.amazon.awssdk.services.entityresolution.model.GetIdMappingJobRequest;
import software.amazon.awssdk.services.entityresolution.model.GetIdMappingJobResponse;
import software.amazon.awssdk.services.entityresolution.model.GetIdMappingWorkflowRequest;
import software.amazon.awssdk.services.entityresolution.model.GetIdMappingWorkflowResponse;
import software.amazon.awssdk.services.entityresolution.model.GetIdNamespaceRequest;
import software.amazon.awssdk.services.entityresolution.model.GetIdNamespaceResponse;
import software.amazon.awssdk.services.entityresolution.model.GetMatchIdRequest;
import software.amazon.awssdk.services.entityresolution.model.GetMatchIdResponse;
import software.amazon.awssdk.services.entityresolution.model.GetMatchingJobRequest;
import software.amazon.awssdk.services.entityresolution.model.GetMatchingJobResponse;
import software.amazon.awssdk.services.entityresolution.model.GetMatchingWorkflowRequest;
import software.amazon.awssdk.services.entityresolution.model.GetMatchingWorkflowResponse;
import software.amazon.awssdk.services.entityresolution.model.GetPolicyRequest;
import software.amazon.awssdk.services.entityresolution.model.GetPolicyResponse;
import software.amazon.awssdk.services.entityresolution.model.GetProviderServiceRequest;
import software.amazon.awssdk.services.entityresolution.model.GetProviderServiceResponse;
import software.amazon.awssdk.services.entityresolution.model.GetSchemaMappingRequest;
import software.amazon.awssdk.services.entityresolution.model.GetSchemaMappingResponse;
import software.amazon.awssdk.services.entityresolution.model.InternalServerException;
import software.amazon.awssdk.services.entityresolution.model.ListIdMappingJobsRequest;
import software.amazon.awssdk.services.entityresolution.model.ListIdMappingJobsResponse;
import software.amazon.awssdk.services.entityresolution.model.ListIdMappingWorkflowsRequest;
import software.amazon.awssdk.services.entityresolution.model.ListIdMappingWorkflowsResponse;
import software.amazon.awssdk.services.entityresolution.model.ListIdNamespacesRequest;
import software.amazon.awssdk.services.entityresolution.model.ListIdNamespacesResponse;
import software.amazon.awssdk.services.entityresolution.model.ListMatchingJobsRequest;
import software.amazon.awssdk.services.entityresolution.model.ListMatchingJobsResponse;
import software.amazon.awssdk.services.entityresolution.model.ListMatchingWorkflowsRequest;
import software.amazon.awssdk.services.entityresolution.model.ListMatchingWorkflowsResponse;
import software.amazon.awssdk.services.entityresolution.model.ListProviderServicesRequest;
import software.amazon.awssdk.services.entityresolution.model.ListProviderServicesResponse;
import software.amazon.awssdk.services.entityresolution.model.ListSchemaMappingsRequest;
import software.amazon.awssdk.services.entityresolution.model.ListSchemaMappingsResponse;
import software.amazon.awssdk.services.entityresolution.model.ListTagsForResourceRequest;
import software.amazon.awssdk.services.entityresolution.model.ListTagsForResourceResponse;
import software.amazon.awssdk.services.entityresolution.model.PutPolicyRequest;
import software.amazon.awssdk.services.entityresolution.model.PutPolicyResponse;
import software.amazon.awssdk.services.entityresolution.model.ResourceNotFoundException;
import software.amazon.awssdk.services.entityresolution.model.StartIdMappingJobRequest;
import software.amazon.awssdk.services.entityresolution.model.StartIdMappingJobResponse;
import software.amazon.awssdk.services.entityresolution.model.StartMatchingJobRequest;
import software.amazon.awssdk.services.entityresolution.model.StartMatchingJobResponse;
import software.amazon.awssdk.services.entityresolution.model.TagResourceRequest;
import software.amazon.awssdk.services.entityresolution.model.TagResourceResponse;
import software.amazon.awssdk.services.entityresolution.model.ThrottlingException;
import software.amazon.awssdk.services.entityresolution.model.UntagResourceRequest;
import software.amazon.awssdk.services.entityresolution.model.UntagResourceResponse;
import software.amazon.awssdk.services.entityresolution.model.UpdateIdMappingWorkflowRequest;
import software.amazon.awssdk.services.entityresolution.model.UpdateIdMappingWorkflowResponse;
import software.amazon.awssdk.services.entityresolution.model.UpdateIdNamespaceRequest;
import software.amazon.awssdk.services.entityresolution.model.UpdateIdNamespaceResponse;
import software.amazon.awssdk.services.entityresolution.model.UpdateMatchingWorkflowRequest;
import software.amazon.awssdk.services.entityresolution.model.UpdateMatchingWorkflowResponse;
import software.amazon.awssdk.services.entityresolution.model.UpdateSchemaMappingRequest;
import software.amazon.awssdk.services.entityresolution.model.UpdateSchemaMappingResponse;
import software.amazon.awssdk.services.entityresolution.model.ValidationException;
import software.amazon.awssdk.services.entityresolution.transform.AddPolicyStatementRequestMarshaller;
import software.amazon.awssdk.services.entityresolution.transform.BatchDeleteUniqueIdRequestMarshaller;
import software.amazon.awssdk.services.entityresolution.transform.CreateIdMappingWorkflowRequestMarshaller;
import software.amazon.awssdk.services.entityresolution.transform.CreateIdNamespaceRequestMarshaller;
import software.amazon.awssdk.services.entityresolution.transform.CreateMatchingWorkflowRequestMarshaller;
import software.amazon.awssdk.services.entityresolution.transform.CreateSchemaMappingRequestMarshaller;
import software.amazon.awssdk.services.entityresolution.transform.DeleteIdMappingWorkflowRequestMarshaller;
import software.amazon.awssdk.services.entityresolution.transform.DeleteIdNamespaceRequestMarshaller;
import software.amazon.awssdk.services.entityresolution.transform.DeleteMatchingWorkflowRequestMarshaller;
import software.amazon.awssdk.services.entityresolution.transform.DeletePolicyStatementRequestMarshaller;
import software.amazon.awssdk.services.entityresolution.transform.DeleteSchemaMappingRequestMarshaller;
import software.amazon.awssdk.services.entityresolution.transform.GetIdMappingJobRequestMarshaller;
import software.amazon.awssdk.services.entityresolution.transform.GetIdMappingWorkflowRequestMarshaller;
import software.amazon.awssdk.services.entityresolution.transform.GetIdNamespaceRequestMarshaller;
import software.amazon.awssdk.services.entityresolution.transform.GetMatchIdRequestMarshaller;
import software.amazon.awssdk.services.entityresolution.transform.GetMatchingJobRequestMarshaller;
import software.amazon.awssdk.services.entityresolution.transform.GetMatchingWorkflowRequestMarshaller;
import software.amazon.awssdk.services.entityresolution.transform.GetPolicyRequestMarshaller;
import software.amazon.awssdk.services.entityresolution.transform.GetProviderServiceRequestMarshaller;
import software.amazon.awssdk.services.entityresolution.transform.GetSchemaMappingRequestMarshaller;
import software.amazon.awssdk.services.entityresolution.transform.ListIdMappingJobsRequestMarshaller;
import software.amazon.awssdk.services.entityresolution.transform.ListIdMappingWorkflowsRequestMarshaller;
import software.amazon.awssdk.services.entityresolution.transform.ListIdNamespacesRequestMarshaller;
import software.amazon.awssdk.services.entityresolution.transform.ListMatchingJobsRequestMarshaller;
import software.amazon.awssdk.services.entityresolution.transform.ListMatchingWorkflowsRequestMarshaller;
import software.amazon.awssdk.services.entityresolution.transform.ListProviderServicesRequestMarshaller;
import software.amazon.awssdk.services.entityresolution.transform.ListSchemaMappingsRequestMarshaller;
import software.amazon.awssdk.services.entityresolution.transform.ListTagsForResourceRequestMarshaller;
import software.amazon.awssdk.services.entityresolution.transform.PutPolicyRequestMarshaller;
import software.amazon.awssdk.services.entityresolution.transform.StartIdMappingJobRequestMarshaller;
import software.amazon.awssdk.services.entityresolution.transform.StartMatchingJobRequestMarshaller;
import software.amazon.awssdk.services.entityresolution.transform.TagResourceRequestMarshaller;
import software.amazon.awssdk.services.entityresolution.transform.UntagResourceRequestMarshaller;
import software.amazon.awssdk.services.entityresolution.transform.UpdateIdMappingWorkflowRequestMarshaller;
import software.amazon.awssdk.services.entityresolution.transform.UpdateIdNamespaceRequestMarshaller;
import software.amazon.awssdk.services.entityresolution.transform.UpdateMatchingWorkflowRequestMarshaller;
import software.amazon.awssdk.services.entityresolution.transform.UpdateSchemaMappingRequestMarshaller;
import software.amazon.awssdk.utils.Logger;
/**
* Internal implementation of {@link EntityResolutionClient}.
*
* @see EntityResolutionClient#builder()
*/
@Generated("software.amazon.awssdk:codegen")
@SdkInternalApi
final class DefaultEntityResolutionClient implements EntityResolutionClient {
private static final Logger log = Logger.loggerFor(DefaultEntityResolutionClient.class);
private static final AwsProtocolMetadata protocolMetadata = AwsProtocolMetadata.builder()
.serviceProtocol(AwsServiceProtocol.REST_JSON).build();
private final SyncClientHandler clientHandler;
private final AwsJsonProtocolFactory protocolFactory;
private final SdkClientConfiguration clientConfiguration;
protected DefaultEntityResolutionClient(SdkClientConfiguration clientConfiguration) {
this.clientHandler = new AwsSyncClientHandler(clientConfiguration);
this.clientConfiguration = clientConfiguration.toBuilder().option(SdkClientOption.SDK_CLIENT, this).build();
this.protocolFactory = init(AwsJsonProtocolFactory.builder()).build();
}
/**
*
* Adds a policy statement object. To retrieve a list of existing policy statements, use the GetPolicy
* API.
*
*
* @param addPolicyStatementRequest
* @return Result of the AddPolicyStatement operation returned by the service.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws InternalServerException
* This exception occurs when there is an internal failure in the Entity Resolution service.
* @throws ResourceNotFoundException
* The resource could not be found.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ConflictException
* The request could not be processed because of conflict in the current state of the resource. Example:
* Workflow already exists, Schema already exists, Workflow is currently running, etc.
* @throws ValidationException
* The input fails to satisfy the constraints specified by Entity Resolution.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EntityResolutionException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EntityResolutionClient.AddPolicyStatement
* @see AWS API Documentation
*/
@Override
public AddPolicyStatementResponse addPolicyStatement(AddPolicyStatementRequest addPolicyStatementRequest)
throws ThrottlingException, InternalServerException, ResourceNotFoundException, AccessDeniedException,
ConflictException, ValidationException, AwsServiceException, SdkClientException, EntityResolutionException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, AddPolicyStatementResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(addPolicyStatementRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, addPolicyStatementRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "EntityResolution");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "AddPolicyStatement");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("AddPolicyStatement").withProtocolMetadata(protocolMetadata)
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withInput(addPolicyStatementRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new AddPolicyStatementRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Deletes multiple unique IDs in a matching workflow.
*
*
* @param batchDeleteUniqueIdRequest
* @return Result of the BatchDeleteUniqueId operation returned by the service.
* @throws InternalServerException
* This exception occurs when there is an internal failure in the Entity Resolution service.
* @throws ResourceNotFoundException
* The resource could not be found.
* @throws ValidationException
* The input fails to satisfy the constraints specified by Entity Resolution.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EntityResolutionException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EntityResolutionClient.BatchDeleteUniqueId
* @see AWS API Documentation
*/
@Override
public BatchDeleteUniqueIdResponse batchDeleteUniqueId(BatchDeleteUniqueIdRequest batchDeleteUniqueIdRequest)
throws InternalServerException, ResourceNotFoundException, ValidationException, AwsServiceException,
SdkClientException, EntityResolutionException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, BatchDeleteUniqueIdResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(batchDeleteUniqueIdRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, batchDeleteUniqueIdRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "EntityResolution");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "BatchDeleteUniqueId");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("BatchDeleteUniqueId").withProtocolMetadata(protocolMetadata)
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withInput(batchDeleteUniqueIdRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new BatchDeleteUniqueIdRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Creates an IdMappingWorkflow
object which stores the configuration of the data processing job to be
* run. Each IdMappingWorkflow
must have a unique workflow name. To modify an existing workflow, use
* the UpdateIdMappingWorkflow
API.
*
*
* @param createIdMappingWorkflowRequest
* @return Result of the CreateIdMappingWorkflow operation returned by the service.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws InternalServerException
* This exception occurs when there is an internal failure in the Entity Resolution service.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ExceedsLimitException
* The request was rejected because it attempted to create resources beyond the current Entity Resolution
* account limits. The error message describes the limit exceeded.
* @throws ConflictException
* The request could not be processed because of conflict in the current state of the resource. Example:
* Workflow already exists, Schema already exists, Workflow is currently running, etc.
* @throws ValidationException
* The input fails to satisfy the constraints specified by Entity Resolution.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EntityResolutionException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EntityResolutionClient.CreateIdMappingWorkflow
* @see AWS API Documentation
*/
@Override
public CreateIdMappingWorkflowResponse createIdMappingWorkflow(CreateIdMappingWorkflowRequest createIdMappingWorkflowRequest)
throws ThrottlingException, InternalServerException, AccessDeniedException, ExceedsLimitException, ConflictException,
ValidationException, AwsServiceException, SdkClientException, EntityResolutionException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, CreateIdMappingWorkflowResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(createIdMappingWorkflowRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, createIdMappingWorkflowRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "EntityResolution");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "CreateIdMappingWorkflow");
return clientHandler
.execute(new ClientExecutionParams()
.withOperationName("CreateIdMappingWorkflow").withProtocolMetadata(protocolMetadata)
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withInput(createIdMappingWorkflowRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new CreateIdMappingWorkflowRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Creates an ID namespace object which will help customers provide metadata explaining their dataset and how to use
* it. Each ID namespace must have a unique name. To modify an existing ID namespace, use the
* UpdateIdNamespace
API.
*
*
* @param createIdNamespaceRequest
* @return Result of the CreateIdNamespace operation returned by the service.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws InternalServerException
* This exception occurs when there is an internal failure in the Entity Resolution service.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ExceedsLimitException
* The request was rejected because it attempted to create resources beyond the current Entity Resolution
* account limits. The error message describes the limit exceeded.
* @throws ConflictException
* The request could not be processed because of conflict in the current state of the resource. Example:
* Workflow already exists, Schema already exists, Workflow is currently running, etc.
* @throws ValidationException
* The input fails to satisfy the constraints specified by Entity Resolution.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EntityResolutionException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EntityResolutionClient.CreateIdNamespace
* @see AWS API Documentation
*/
@Override
public CreateIdNamespaceResponse createIdNamespace(CreateIdNamespaceRequest createIdNamespaceRequest)
throws ThrottlingException, InternalServerException, AccessDeniedException, ExceedsLimitException, ConflictException,
ValidationException, AwsServiceException, SdkClientException, EntityResolutionException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
CreateIdNamespaceResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(createIdNamespaceRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, createIdNamespaceRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "EntityResolution");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "CreateIdNamespace");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("CreateIdNamespace").withProtocolMetadata(protocolMetadata)
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withInput(createIdNamespaceRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new CreateIdNamespaceRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Creates a MatchingWorkflow
object which stores the configuration of the data processing job to be
* run. It is important to note that there should not be a pre-existing MatchingWorkflow
with the same
* name. To modify an existing workflow, utilize the UpdateMatchingWorkflow
API.
*
*
* @param createMatchingWorkflowRequest
* @return Result of the CreateMatchingWorkflow operation returned by the service.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws InternalServerException
* This exception occurs when there is an internal failure in the Entity Resolution service.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ExceedsLimitException
* The request was rejected because it attempted to create resources beyond the current Entity Resolution
* account limits. The error message describes the limit exceeded.
* @throws ConflictException
* The request could not be processed because of conflict in the current state of the resource. Example:
* Workflow already exists, Schema already exists, Workflow is currently running, etc.
* @throws ValidationException
* The input fails to satisfy the constraints specified by Entity Resolution.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EntityResolutionException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EntityResolutionClient.CreateMatchingWorkflow
* @see AWS API Documentation
*/
@Override
public CreateMatchingWorkflowResponse createMatchingWorkflow(CreateMatchingWorkflowRequest createMatchingWorkflowRequest)
throws ThrottlingException, InternalServerException, AccessDeniedException, ExceedsLimitException, ConflictException,
ValidationException, AwsServiceException, SdkClientException, EntityResolutionException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, CreateMatchingWorkflowResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(createMatchingWorkflowRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, createMatchingWorkflowRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "EntityResolution");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "CreateMatchingWorkflow");
return clientHandler
.execute(new ClientExecutionParams()
.withOperationName("CreateMatchingWorkflow").withProtocolMetadata(protocolMetadata)
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withInput(createMatchingWorkflowRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new CreateMatchingWorkflowRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Creates a schema mapping, which defines the schema of the input customer records table. The
* SchemaMapping
also provides Entity Resolution with some metadata about the table, such as the
* attribute types of the columns and which columns to match on.
*
*
* @param createSchemaMappingRequest
* @return Result of the CreateSchemaMapping operation returned by the service.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws InternalServerException
* This exception occurs when there is an internal failure in the Entity Resolution service.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ExceedsLimitException
* The request was rejected because it attempted to create resources beyond the current Entity Resolution
* account limits. The error message describes the limit exceeded.
* @throws ConflictException
* The request could not be processed because of conflict in the current state of the resource. Example:
* Workflow already exists, Schema already exists, Workflow is currently running, etc.
* @throws ValidationException
* The input fails to satisfy the constraints specified by Entity Resolution.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EntityResolutionException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EntityResolutionClient.CreateSchemaMapping
* @see AWS API Documentation
*/
@Override
public CreateSchemaMappingResponse createSchemaMapping(CreateSchemaMappingRequest createSchemaMappingRequest)
throws ThrottlingException, InternalServerException, AccessDeniedException, ExceedsLimitException, ConflictException,
ValidationException, AwsServiceException, SdkClientException, EntityResolutionException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, CreateSchemaMappingResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(createSchemaMappingRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, createSchemaMappingRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "EntityResolution");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "CreateSchemaMapping");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("CreateSchemaMapping").withProtocolMetadata(protocolMetadata)
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withInput(createSchemaMappingRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new CreateSchemaMappingRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Deletes the IdMappingWorkflow
with a given name. This operation will succeed even if a workflow with
* the given name does not exist.
*
*
* @param deleteIdMappingWorkflowRequest
* @return Result of the DeleteIdMappingWorkflow operation returned by the service.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws InternalServerException
* This exception occurs when there is an internal failure in the Entity Resolution service.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ConflictException
* The request could not be processed because of conflict in the current state of the resource. Example:
* Workflow already exists, Schema already exists, Workflow is currently running, etc.
* @throws ValidationException
* The input fails to satisfy the constraints specified by Entity Resolution.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EntityResolutionException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EntityResolutionClient.DeleteIdMappingWorkflow
* @see AWS API Documentation
*/
@Override
public DeleteIdMappingWorkflowResponse deleteIdMappingWorkflow(DeleteIdMappingWorkflowRequest deleteIdMappingWorkflowRequest)
throws ThrottlingException, InternalServerException, AccessDeniedException, ConflictException, ValidationException,
AwsServiceException, SdkClientException, EntityResolutionException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, DeleteIdMappingWorkflowResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(deleteIdMappingWorkflowRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, deleteIdMappingWorkflowRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "EntityResolution");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DeleteIdMappingWorkflow");
return clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DeleteIdMappingWorkflow").withProtocolMetadata(protocolMetadata)
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withInput(deleteIdMappingWorkflowRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new DeleteIdMappingWorkflowRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Deletes the IdNamespace
with a given name.
*
*
* @param deleteIdNamespaceRequest
* @return Result of the DeleteIdNamespace operation returned by the service.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws InternalServerException
* This exception occurs when there is an internal failure in the Entity Resolution service.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ValidationException
* The input fails to satisfy the constraints specified by Entity Resolution.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EntityResolutionException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EntityResolutionClient.DeleteIdNamespace
* @see AWS API Documentation
*/
@Override
public DeleteIdNamespaceResponse deleteIdNamespace(DeleteIdNamespaceRequest deleteIdNamespaceRequest)
throws ThrottlingException, InternalServerException, AccessDeniedException, ValidationException, AwsServiceException,
SdkClientException, EntityResolutionException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
DeleteIdNamespaceResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(deleteIdNamespaceRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, deleteIdNamespaceRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "EntityResolution");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DeleteIdNamespace");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("DeleteIdNamespace").withProtocolMetadata(protocolMetadata)
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withInput(deleteIdNamespaceRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new DeleteIdNamespaceRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Deletes the MatchingWorkflow
with a given name. This operation will succeed even if a workflow with
* the given name does not exist.
*
*
* @param deleteMatchingWorkflowRequest
* @return Result of the DeleteMatchingWorkflow operation returned by the service.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws InternalServerException
* This exception occurs when there is an internal failure in the Entity Resolution service.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ConflictException
* The request could not be processed because of conflict in the current state of the resource. Example:
* Workflow already exists, Schema already exists, Workflow is currently running, etc.
* @throws ValidationException
* The input fails to satisfy the constraints specified by Entity Resolution.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EntityResolutionException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EntityResolutionClient.DeleteMatchingWorkflow
* @see AWS API Documentation
*/
@Override
public DeleteMatchingWorkflowResponse deleteMatchingWorkflow(DeleteMatchingWorkflowRequest deleteMatchingWorkflowRequest)
throws ThrottlingException, InternalServerException, AccessDeniedException, ConflictException, ValidationException,
AwsServiceException, SdkClientException, EntityResolutionException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, DeleteMatchingWorkflowResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(deleteMatchingWorkflowRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, deleteMatchingWorkflowRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "EntityResolution");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DeleteMatchingWorkflow");
return clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DeleteMatchingWorkflow").withProtocolMetadata(protocolMetadata)
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withInput(deleteMatchingWorkflowRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new DeleteMatchingWorkflowRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Deletes the policy statement.
*
*
* @param deletePolicyStatementRequest
* @return Result of the DeletePolicyStatement operation returned by the service.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws InternalServerException
* This exception occurs when there is an internal failure in the Entity Resolution service.
* @throws ResourceNotFoundException
* The resource could not be found.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ConflictException
* The request could not be processed because of conflict in the current state of the resource. Example:
* Workflow already exists, Schema already exists, Workflow is currently running, etc.
* @throws ValidationException
* The input fails to satisfy the constraints specified by Entity Resolution.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EntityResolutionException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EntityResolutionClient.DeletePolicyStatement
* @see AWS API Documentation
*/
@Override
public DeletePolicyStatementResponse deletePolicyStatement(DeletePolicyStatementRequest deletePolicyStatementRequest)
throws ThrottlingException, InternalServerException, ResourceNotFoundException, AccessDeniedException,
ConflictException, ValidationException, AwsServiceException, SdkClientException, EntityResolutionException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, DeletePolicyStatementResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(deletePolicyStatementRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, deletePolicyStatementRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "EntityResolution");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DeletePolicyStatement");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("DeletePolicyStatement").withProtocolMetadata(protocolMetadata)
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withInput(deletePolicyStatementRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new DeletePolicyStatementRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Deletes the SchemaMapping
with a given name. This operation will succeed even if a schema with the
* given name does not exist. This operation will fail if there is a MatchingWorkflow
object that
* references the SchemaMapping
in the workflow's InputSourceConfig
.
*
*
* @param deleteSchemaMappingRequest
* @return Result of the DeleteSchemaMapping operation returned by the service.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws InternalServerException
* This exception occurs when there is an internal failure in the Entity Resolution service.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ConflictException
* The request could not be processed because of conflict in the current state of the resource. Example:
* Workflow already exists, Schema already exists, Workflow is currently running, etc.
* @throws ValidationException
* The input fails to satisfy the constraints specified by Entity Resolution.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EntityResolutionException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EntityResolutionClient.DeleteSchemaMapping
* @see AWS API Documentation
*/
@Override
public DeleteSchemaMappingResponse deleteSchemaMapping(DeleteSchemaMappingRequest deleteSchemaMappingRequest)
throws ThrottlingException, InternalServerException, AccessDeniedException, ConflictException, ValidationException,
AwsServiceException, SdkClientException, EntityResolutionException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, DeleteSchemaMappingResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(deleteSchemaMappingRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, deleteSchemaMappingRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "EntityResolution");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DeleteSchemaMapping");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("DeleteSchemaMapping").withProtocolMetadata(protocolMetadata)
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withInput(deleteSchemaMappingRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new DeleteSchemaMappingRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Gets the status, metrics, and errors (if there are any) that are associated with a job.
*
*
* @param getIdMappingJobRequest
* @return Result of the GetIdMappingJob operation returned by the service.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws InternalServerException
* This exception occurs when there is an internal failure in the Entity Resolution service.
* @throws ResourceNotFoundException
* The resource could not be found.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ValidationException
* The input fails to satisfy the constraints specified by Entity Resolution.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EntityResolutionException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EntityResolutionClient.GetIdMappingJob
* @see AWS API Documentation
*/
@Override
public GetIdMappingJobResponse getIdMappingJob(GetIdMappingJobRequest getIdMappingJobRequest) throws ThrottlingException,
InternalServerException, ResourceNotFoundException, AccessDeniedException, ValidationException, AwsServiceException,
SdkClientException, EntityResolutionException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
GetIdMappingJobResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(getIdMappingJobRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, getIdMappingJobRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "EntityResolution");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "GetIdMappingJob");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("GetIdMappingJob").withProtocolMetadata(protocolMetadata)
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withInput(getIdMappingJobRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new GetIdMappingJobRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Returns the IdMappingWorkflow
with a given name, if it exists.
*
*
* @param getIdMappingWorkflowRequest
* @return Result of the GetIdMappingWorkflow operation returned by the service.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws InternalServerException
* This exception occurs when there is an internal failure in the Entity Resolution service.
* @throws ResourceNotFoundException
* The resource could not be found.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ValidationException
* The input fails to satisfy the constraints specified by Entity Resolution.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EntityResolutionException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EntityResolutionClient.GetIdMappingWorkflow
* @see AWS API Documentation
*/
@Override
public GetIdMappingWorkflowResponse getIdMappingWorkflow(GetIdMappingWorkflowRequest getIdMappingWorkflowRequest)
throws ThrottlingException, InternalServerException, ResourceNotFoundException, AccessDeniedException,
ValidationException, AwsServiceException, SdkClientException, EntityResolutionException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, GetIdMappingWorkflowResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(getIdMappingWorkflowRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, getIdMappingWorkflowRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "EntityResolution");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "GetIdMappingWorkflow");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("GetIdMappingWorkflow").withProtocolMetadata(protocolMetadata)
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withInput(getIdMappingWorkflowRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new GetIdMappingWorkflowRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Returns the IdNamespace
with a given name, if it exists.
*
*
* @param getIdNamespaceRequest
* @return Result of the GetIdNamespace operation returned by the service.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws InternalServerException
* This exception occurs when there is an internal failure in the Entity Resolution service.
* @throws ResourceNotFoundException
* The resource could not be found.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ValidationException
* The input fails to satisfy the constraints specified by Entity Resolution.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EntityResolutionException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EntityResolutionClient.GetIdNamespace
* @see AWS API Documentation
*/
@Override
public GetIdNamespaceResponse getIdNamespace(GetIdNamespaceRequest getIdNamespaceRequest) throws ThrottlingException,
InternalServerException, ResourceNotFoundException, AccessDeniedException, ValidationException, AwsServiceException,
SdkClientException, EntityResolutionException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
GetIdNamespaceResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(getIdNamespaceRequest, this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, getIdNamespaceRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "EntityResolution");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "GetIdNamespace");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("GetIdNamespace").withProtocolMetadata(protocolMetadata)
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withInput(getIdNamespaceRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new GetIdNamespaceRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Returns the corresponding Match ID of a customer record if the record has been processed.
*
*
* @param getMatchIdRequest
* @return Result of the GetMatchId operation returned by the service.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws InternalServerException
* This exception occurs when there is an internal failure in the Entity Resolution service.
* @throws ResourceNotFoundException
* The resource could not be found.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ValidationException
* The input fails to satisfy the constraints specified by Entity Resolution.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EntityResolutionException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EntityResolutionClient.GetMatchId
* @see AWS
* API Documentation
*/
@Override
public GetMatchIdResponse getMatchId(GetMatchIdRequest getMatchIdRequest) throws ThrottlingException,
InternalServerException, ResourceNotFoundException, AccessDeniedException, ValidationException, AwsServiceException,
SdkClientException, EntityResolutionException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
GetMatchIdResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(getMatchIdRequest, this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, getMatchIdRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "EntityResolution");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "GetMatchId");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("GetMatchId").withProtocolMetadata(protocolMetadata).withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withRequestConfiguration(clientConfiguration)
.withInput(getMatchIdRequest).withMetricCollector(apiCallMetricCollector)
.withMarshaller(new GetMatchIdRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Gets the status, metrics, and errors (if there are any) that are associated with a job.
*
*
* @param getMatchingJobRequest
* @return Result of the GetMatchingJob operation returned by the service.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws InternalServerException
* This exception occurs when there is an internal failure in the Entity Resolution service.
* @throws ResourceNotFoundException
* The resource could not be found.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ValidationException
* The input fails to satisfy the constraints specified by Entity Resolution.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EntityResolutionException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EntityResolutionClient.GetMatchingJob
* @see AWS API Documentation
*/
@Override
public GetMatchingJobResponse getMatchingJob(GetMatchingJobRequest getMatchingJobRequest) throws ThrottlingException,
InternalServerException, ResourceNotFoundException, AccessDeniedException, ValidationException, AwsServiceException,
SdkClientException, EntityResolutionException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
GetMatchingJobResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(getMatchingJobRequest, this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, getMatchingJobRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "EntityResolution");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "GetMatchingJob");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("GetMatchingJob").withProtocolMetadata(protocolMetadata)
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withInput(getMatchingJobRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new GetMatchingJobRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Returns the MatchingWorkflow
with a given name, if it exists.
*
*
* @param getMatchingWorkflowRequest
* @return Result of the GetMatchingWorkflow operation returned by the service.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws InternalServerException
* This exception occurs when there is an internal failure in the Entity Resolution service.
* @throws ResourceNotFoundException
* The resource could not be found.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ValidationException
* The input fails to satisfy the constraints specified by Entity Resolution.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EntityResolutionException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EntityResolutionClient.GetMatchingWorkflow
* @see AWS API Documentation
*/
@Override
public GetMatchingWorkflowResponse getMatchingWorkflow(GetMatchingWorkflowRequest getMatchingWorkflowRequest)
throws ThrottlingException, InternalServerException, ResourceNotFoundException, AccessDeniedException,
ValidationException, AwsServiceException, SdkClientException, EntityResolutionException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, GetMatchingWorkflowResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(getMatchingWorkflowRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, getMatchingWorkflowRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "EntityResolution");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "GetMatchingWorkflow");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("GetMatchingWorkflow").withProtocolMetadata(protocolMetadata)
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withInput(getMatchingWorkflowRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new GetMatchingWorkflowRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Returns the resource-based policy.
*
*
* @param getPolicyRequest
* @return Result of the GetPolicy operation returned by the service.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws InternalServerException
* This exception occurs when there is an internal failure in the Entity Resolution service.
* @throws ResourceNotFoundException
* The resource could not be found.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ValidationException
* The input fails to satisfy the constraints specified by Entity Resolution.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EntityResolutionException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EntityResolutionClient.GetPolicy
* @see AWS
* API Documentation
*/
@Override
public GetPolicyResponse getPolicy(GetPolicyRequest getPolicyRequest) throws ThrottlingException, InternalServerException,
ResourceNotFoundException, AccessDeniedException, ValidationException, AwsServiceException, SdkClientException,
EntityResolutionException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
GetPolicyResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(getPolicyRequest, this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, getPolicyRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "EntityResolution");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "GetPolicy");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("GetPolicy").withProtocolMetadata(protocolMetadata).withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withRequestConfiguration(clientConfiguration)
.withInput(getPolicyRequest).withMetricCollector(apiCallMetricCollector)
.withMarshaller(new GetPolicyRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Returns the ProviderService
of a given name.
*
*
* @param getProviderServiceRequest
* @return Result of the GetProviderService operation returned by the service.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws InternalServerException
* This exception occurs when there is an internal failure in the Entity Resolution service.
* @throws ResourceNotFoundException
* The resource could not be found.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ValidationException
* The input fails to satisfy the constraints specified by Entity Resolution.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EntityResolutionException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EntityResolutionClient.GetProviderService
* @see AWS API Documentation
*/
@Override
public GetProviderServiceResponse getProviderService(GetProviderServiceRequest getProviderServiceRequest)
throws ThrottlingException, InternalServerException, ResourceNotFoundException, AccessDeniedException,
ValidationException, AwsServiceException, SdkClientException, EntityResolutionException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, GetProviderServiceResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(getProviderServiceRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, getProviderServiceRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "EntityResolution");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "GetProviderService");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("GetProviderService").withProtocolMetadata(protocolMetadata)
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withInput(getProviderServiceRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new GetProviderServiceRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Returns the SchemaMapping of a given name.
*
*
* @param getSchemaMappingRequest
* @return Result of the GetSchemaMapping operation returned by the service.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws InternalServerException
* This exception occurs when there is an internal failure in the Entity Resolution service.
* @throws ResourceNotFoundException
* The resource could not be found.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ValidationException
* The input fails to satisfy the constraints specified by Entity Resolution.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EntityResolutionException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EntityResolutionClient.GetSchemaMapping
* @see AWS API Documentation
*/
@Override
public GetSchemaMappingResponse getSchemaMapping(GetSchemaMappingRequest getSchemaMappingRequest) throws ThrottlingException,
InternalServerException, ResourceNotFoundException, AccessDeniedException, ValidationException, AwsServiceException,
SdkClientException, EntityResolutionException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
GetSchemaMappingResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(getSchemaMappingRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, getSchemaMappingRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "EntityResolution");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "GetSchemaMapping");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("GetSchemaMapping").withProtocolMetadata(protocolMetadata)
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withInput(getSchemaMappingRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new GetSchemaMappingRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Lists all ID mapping jobs for a given workflow.
*
*
* @param listIdMappingJobsRequest
* @return Result of the ListIdMappingJobs operation returned by the service.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws InternalServerException
* This exception occurs when there is an internal failure in the Entity Resolution service.
* @throws ResourceNotFoundException
* The resource could not be found.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ValidationException
* The input fails to satisfy the constraints specified by Entity Resolution.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EntityResolutionException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EntityResolutionClient.ListIdMappingJobs
* @see AWS API Documentation
*/
@Override
public ListIdMappingJobsResponse listIdMappingJobs(ListIdMappingJobsRequest listIdMappingJobsRequest)
throws ThrottlingException, InternalServerException, ResourceNotFoundException, AccessDeniedException,
ValidationException, AwsServiceException, SdkClientException, EntityResolutionException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
ListIdMappingJobsResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(listIdMappingJobsRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, listIdMappingJobsRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "EntityResolution");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "ListIdMappingJobs");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("ListIdMappingJobs").withProtocolMetadata(protocolMetadata)
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withInput(listIdMappingJobsRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new ListIdMappingJobsRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Returns a list of all the IdMappingWorkflows
that have been created for an Amazon Web Services
* account.
*
*
* @param listIdMappingWorkflowsRequest
* @return Result of the ListIdMappingWorkflows operation returned by the service.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws InternalServerException
* This exception occurs when there is an internal failure in the Entity Resolution service.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ValidationException
* The input fails to satisfy the constraints specified by Entity Resolution.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EntityResolutionException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EntityResolutionClient.ListIdMappingWorkflows
* @see AWS API Documentation
*/
@Override
public ListIdMappingWorkflowsResponse listIdMappingWorkflows(ListIdMappingWorkflowsRequest listIdMappingWorkflowsRequest)
throws ThrottlingException, InternalServerException, AccessDeniedException, ValidationException, AwsServiceException,
SdkClientException, EntityResolutionException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, ListIdMappingWorkflowsResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(listIdMappingWorkflowsRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, listIdMappingWorkflowsRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "EntityResolution");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "ListIdMappingWorkflows");
return clientHandler
.execute(new ClientExecutionParams()
.withOperationName("ListIdMappingWorkflows").withProtocolMetadata(protocolMetadata)
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withInput(listIdMappingWorkflowsRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new ListIdMappingWorkflowsRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Returns a list of all ID namespaces.
*
*
* @param listIdNamespacesRequest
* @return Result of the ListIdNamespaces operation returned by the service.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws InternalServerException
* This exception occurs when there is an internal failure in the Entity Resolution service.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ValidationException
* The input fails to satisfy the constraints specified by Entity Resolution.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EntityResolutionException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EntityResolutionClient.ListIdNamespaces
* @see AWS API Documentation
*/
@Override
public ListIdNamespacesResponse listIdNamespaces(ListIdNamespacesRequest listIdNamespacesRequest) throws ThrottlingException,
InternalServerException, AccessDeniedException, ValidationException, AwsServiceException, SdkClientException,
EntityResolutionException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
ListIdNamespacesResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(listIdNamespacesRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, listIdNamespacesRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "EntityResolution");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "ListIdNamespaces");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("ListIdNamespaces").withProtocolMetadata(protocolMetadata)
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withInput(listIdNamespacesRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new ListIdNamespacesRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Lists all jobs for a given workflow.
*
*
* @param listMatchingJobsRequest
* @return Result of the ListMatchingJobs operation returned by the service.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws InternalServerException
* This exception occurs when there is an internal failure in the Entity Resolution service.
* @throws ResourceNotFoundException
* The resource could not be found.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ValidationException
* The input fails to satisfy the constraints specified by Entity Resolution.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EntityResolutionException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EntityResolutionClient.ListMatchingJobs
* @see AWS API Documentation
*/
@Override
public ListMatchingJobsResponse listMatchingJobs(ListMatchingJobsRequest listMatchingJobsRequest) throws ThrottlingException,
InternalServerException, ResourceNotFoundException, AccessDeniedException, ValidationException, AwsServiceException,
SdkClientException, EntityResolutionException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
ListMatchingJobsResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(listMatchingJobsRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, listMatchingJobsRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "EntityResolution");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "ListMatchingJobs");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("ListMatchingJobs").withProtocolMetadata(protocolMetadata)
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withInput(listMatchingJobsRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new ListMatchingJobsRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Returns a list of all the MatchingWorkflows
that have been created for an Amazon Web Services
* account.
*
*
* @param listMatchingWorkflowsRequest
* @return Result of the ListMatchingWorkflows operation returned by the service.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws InternalServerException
* This exception occurs when there is an internal failure in the Entity Resolution service.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ValidationException
* The input fails to satisfy the constraints specified by Entity Resolution.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EntityResolutionException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EntityResolutionClient.ListMatchingWorkflows
* @see AWS API Documentation
*/
@Override
public ListMatchingWorkflowsResponse listMatchingWorkflows(ListMatchingWorkflowsRequest listMatchingWorkflowsRequest)
throws ThrottlingException, InternalServerException, AccessDeniedException, ValidationException, AwsServiceException,
SdkClientException, EntityResolutionException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, ListMatchingWorkflowsResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(listMatchingWorkflowsRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, listMatchingWorkflowsRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "EntityResolution");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "ListMatchingWorkflows");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("ListMatchingWorkflows").withProtocolMetadata(protocolMetadata)
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withInput(listMatchingWorkflowsRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new ListMatchingWorkflowsRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Returns a list of all the ProviderServices
that are available in this Amazon Web Services Region.
*
*
* @param listProviderServicesRequest
* @return Result of the ListProviderServices operation returned by the service.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws InternalServerException
* This exception occurs when there is an internal failure in the Entity Resolution service.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ValidationException
* The input fails to satisfy the constraints specified by Entity Resolution.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EntityResolutionException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EntityResolutionClient.ListProviderServices
* @see AWS API Documentation
*/
@Override
public ListProviderServicesResponse listProviderServices(ListProviderServicesRequest listProviderServicesRequest)
throws ThrottlingException, InternalServerException, AccessDeniedException, ValidationException, AwsServiceException,
SdkClientException, EntityResolutionException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, ListProviderServicesResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(listProviderServicesRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, listProviderServicesRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "EntityResolution");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "ListProviderServices");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("ListProviderServices").withProtocolMetadata(protocolMetadata)
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withInput(listProviderServicesRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new ListProviderServicesRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Returns a list of all the SchemaMappings
that have been created for an Amazon Web Services account.
*
*
* @param listSchemaMappingsRequest
* @return Result of the ListSchemaMappings operation returned by the service.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws InternalServerException
* This exception occurs when there is an internal failure in the Entity Resolution service.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ValidationException
* The input fails to satisfy the constraints specified by Entity Resolution.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EntityResolutionException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EntityResolutionClient.ListSchemaMappings
* @see AWS API Documentation
*/
@Override
public ListSchemaMappingsResponse listSchemaMappings(ListSchemaMappingsRequest listSchemaMappingsRequest)
throws ThrottlingException, InternalServerException, AccessDeniedException, ValidationException, AwsServiceException,
SdkClientException, EntityResolutionException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, ListSchemaMappingsResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(listSchemaMappingsRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, listSchemaMappingsRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "EntityResolution");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "ListSchemaMappings");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("ListSchemaMappings").withProtocolMetadata(protocolMetadata)
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withInput(listSchemaMappingsRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new ListSchemaMappingsRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Displays the tags associated with an Entity Resolution resource. In Entity Resolution, SchemaMapping
* , and MatchingWorkflow
can be tagged.
*
*
* @param listTagsForResourceRequest
* @return Result of the ListTagsForResource operation returned by the service.
* @throws InternalServerException
* This exception occurs when there is an internal failure in the Entity Resolution service.
* @throws ResourceNotFoundException
* The resource could not be found.
* @throws ValidationException
* The input fails to satisfy the constraints specified by Entity Resolution.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EntityResolutionException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EntityResolutionClient.ListTagsForResource
* @see AWS API Documentation
*/
@Override
public ListTagsForResourceResponse listTagsForResource(ListTagsForResourceRequest listTagsForResourceRequest)
throws InternalServerException, ResourceNotFoundException, ValidationException, AwsServiceException,
SdkClientException, EntityResolutionException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, ListTagsForResourceResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(listTagsForResourceRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, listTagsForResourceRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "EntityResolution");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "ListTagsForResource");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("ListTagsForResource").withProtocolMetadata(protocolMetadata)
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withInput(listTagsForResourceRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new ListTagsForResourceRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Updates the resource-based policy.
*
*
* @param putPolicyRequest
* @return Result of the PutPolicy operation returned by the service.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws InternalServerException
* This exception occurs when there is an internal failure in the Entity Resolution service.
* @throws ResourceNotFoundException
* The resource could not be found.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ConflictException
* The request could not be processed because of conflict in the current state of the resource. Example:
* Workflow already exists, Schema already exists, Workflow is currently running, etc.
* @throws ValidationException
* The input fails to satisfy the constraints specified by Entity Resolution.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EntityResolutionException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EntityResolutionClient.PutPolicy
* @see AWS
* API Documentation
*/
@Override
public PutPolicyResponse putPolicy(PutPolicyRequest putPolicyRequest) throws ThrottlingException, InternalServerException,
ResourceNotFoundException, AccessDeniedException, ConflictException, ValidationException, AwsServiceException,
SdkClientException, EntityResolutionException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
PutPolicyResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(putPolicyRequest, this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, putPolicyRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "EntityResolution");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "PutPolicy");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("PutPolicy").withProtocolMetadata(protocolMetadata).withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withRequestConfiguration(clientConfiguration)
.withInput(putPolicyRequest).withMetricCollector(apiCallMetricCollector)
.withMarshaller(new PutPolicyRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Starts the IdMappingJob
of a workflow. The workflow must have previously been created using the
* CreateIdMappingWorkflow
endpoint.
*
*
* @param startIdMappingJobRequest
* @return Result of the StartIdMappingJob operation returned by the service.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws InternalServerException
* This exception occurs when there is an internal failure in the Entity Resolution service.
* @throws ResourceNotFoundException
* The resource could not be found.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ExceedsLimitException
* The request was rejected because it attempted to create resources beyond the current Entity Resolution
* account limits. The error message describes the limit exceeded.
* @throws ConflictException
* The request could not be processed because of conflict in the current state of the resource. Example:
* Workflow already exists, Schema already exists, Workflow is currently running, etc.
* @throws ValidationException
* The input fails to satisfy the constraints specified by Entity Resolution.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EntityResolutionException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EntityResolutionClient.StartIdMappingJob
* @see AWS API Documentation
*/
@Override
public StartIdMappingJobResponse startIdMappingJob(StartIdMappingJobRequest startIdMappingJobRequest)
throws ThrottlingException, InternalServerException, ResourceNotFoundException, AccessDeniedException,
ExceedsLimitException, ConflictException, ValidationException, AwsServiceException, SdkClientException,
EntityResolutionException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
StartIdMappingJobResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(startIdMappingJobRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, startIdMappingJobRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "EntityResolution");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "StartIdMappingJob");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("StartIdMappingJob").withProtocolMetadata(protocolMetadata)
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withInput(startIdMappingJobRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new StartIdMappingJobRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Starts the MatchingJob
of a workflow. The workflow must have previously been created using the
* CreateMatchingWorkflow
endpoint.
*
*
* @param startMatchingJobRequest
* @return Result of the StartMatchingJob operation returned by the service.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws InternalServerException
* This exception occurs when there is an internal failure in the Entity Resolution service.
* @throws ResourceNotFoundException
* The resource could not be found.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ExceedsLimitException
* The request was rejected because it attempted to create resources beyond the current Entity Resolution
* account limits. The error message describes the limit exceeded.
* @throws ConflictException
* The request could not be processed because of conflict in the current state of the resource. Example:
* Workflow already exists, Schema already exists, Workflow is currently running, etc.
* @throws ValidationException
* The input fails to satisfy the constraints specified by Entity Resolution.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EntityResolutionException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EntityResolutionClient.StartMatchingJob
* @see AWS API Documentation
*/
@Override
public StartMatchingJobResponse startMatchingJob(StartMatchingJobRequest startMatchingJobRequest) throws ThrottlingException,
InternalServerException, ResourceNotFoundException, AccessDeniedException, ExceedsLimitException, ConflictException,
ValidationException, AwsServiceException, SdkClientException, EntityResolutionException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
StartMatchingJobResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(startMatchingJobRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, startMatchingJobRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "EntityResolution");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "StartMatchingJob");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("StartMatchingJob").withProtocolMetadata(protocolMetadata)
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withInput(startMatchingJobRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new StartMatchingJobRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Assigns one or more tags (key-value pairs) to the specified Entity Resolution resource. Tags can help you
* organize and categorize your resources. You can also use them to scope user permissions by granting a user
* permission to access or change only resources with certain tag values. In Entity Resolution,
* SchemaMapping
and MatchingWorkflow
can be tagged. Tags don't have any semantic meaning
* to Amazon Web Services and are interpreted strictly as strings of characters. You can use the
* TagResource
action with a resource that already has tags. If you specify a new tag key, this tag is
* appended to the list of tags associated with the resource. If you specify a tag key that is already associated
* with the resource, the new tag value that you specify replaces the previous value for that tag.
*
*
* @param tagResourceRequest
* @return Result of the TagResource operation returned by the service.
* @throws InternalServerException
* This exception occurs when there is an internal failure in the Entity Resolution service.
* @throws ResourceNotFoundException
* The resource could not be found.
* @throws ValidationException
* The input fails to satisfy the constraints specified by Entity Resolution.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EntityResolutionException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EntityResolutionClient.TagResource
* @see AWS
* API Documentation
*/
@Override
public TagResourceResponse tagResource(TagResourceRequest tagResourceRequest) throws InternalServerException,
ResourceNotFoundException, ValidationException, AwsServiceException, SdkClientException, EntityResolutionException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
TagResourceResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(tagResourceRequest, this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, tagResourceRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "EntityResolution");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "TagResource");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("TagResource").withProtocolMetadata(protocolMetadata).withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withRequestConfiguration(clientConfiguration)
.withInput(tagResourceRequest).withMetricCollector(apiCallMetricCollector)
.withMarshaller(new TagResourceRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Removes one or more tags from the specified Entity Resolution resource. In Entity Resolution,
* SchemaMapping
, and MatchingWorkflow
can be tagged.
*
*
* @param untagResourceRequest
* @return Result of the UntagResource operation returned by the service.
* @throws InternalServerException
* This exception occurs when there is an internal failure in the Entity Resolution service.
* @throws ResourceNotFoundException
* The resource could not be found.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EntityResolutionException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EntityResolutionClient.UntagResource
* @see AWS API Documentation
*/
@Override
public UntagResourceResponse untagResource(UntagResourceRequest untagResourceRequest) throws InternalServerException,
ResourceNotFoundException, AwsServiceException, SdkClientException, EntityResolutionException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
UntagResourceResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(untagResourceRequest, this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, untagResourceRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "EntityResolution");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "UntagResource");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("UntagResource").withProtocolMetadata(protocolMetadata)
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withInput(untagResourceRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new UntagResourceRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Updates an existing IdMappingWorkflow
. This method is identical to
* CreateIdMappingWorkflow
, except it uses an HTTP PUT
request instead of a
* POST
request, and the IdMappingWorkflow
must already exist for the method to succeed.
*
*
* @param updateIdMappingWorkflowRequest
* @return Result of the UpdateIdMappingWorkflow operation returned by the service.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws InternalServerException
* This exception occurs when there is an internal failure in the Entity Resolution service.
* @throws ResourceNotFoundException
* The resource could not be found.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ValidationException
* The input fails to satisfy the constraints specified by Entity Resolution.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EntityResolutionException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EntityResolutionClient.UpdateIdMappingWorkflow
* @see AWS API Documentation
*/
@Override
public UpdateIdMappingWorkflowResponse updateIdMappingWorkflow(UpdateIdMappingWorkflowRequest updateIdMappingWorkflowRequest)
throws ThrottlingException, InternalServerException, ResourceNotFoundException, AccessDeniedException,
ValidationException, AwsServiceException, SdkClientException, EntityResolutionException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, UpdateIdMappingWorkflowResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(updateIdMappingWorkflowRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, updateIdMappingWorkflowRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "EntityResolution");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "UpdateIdMappingWorkflow");
return clientHandler
.execute(new ClientExecutionParams()
.withOperationName("UpdateIdMappingWorkflow").withProtocolMetadata(protocolMetadata)
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withInput(updateIdMappingWorkflowRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new UpdateIdMappingWorkflowRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Updates an existing ID namespace.
*
*
* @param updateIdNamespaceRequest
* @return Result of the UpdateIdNamespace operation returned by the service.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws InternalServerException
* This exception occurs when there is an internal failure in the Entity Resolution service.
* @throws ResourceNotFoundException
* The resource could not be found.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ValidationException
* The input fails to satisfy the constraints specified by Entity Resolution.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EntityResolutionException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EntityResolutionClient.UpdateIdNamespace
* @see AWS API Documentation
*/
@Override
public UpdateIdNamespaceResponse updateIdNamespace(UpdateIdNamespaceRequest updateIdNamespaceRequest)
throws ThrottlingException, InternalServerException, ResourceNotFoundException, AccessDeniedException,
ValidationException, AwsServiceException, SdkClientException, EntityResolutionException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
UpdateIdNamespaceResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(updateIdNamespaceRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, updateIdNamespaceRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "EntityResolution");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "UpdateIdNamespace");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("UpdateIdNamespace").withProtocolMetadata(protocolMetadata)
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withInput(updateIdNamespaceRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new UpdateIdNamespaceRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Updates an existing MatchingWorkflow
. This method is identical to
* CreateMatchingWorkflow
, except it uses an HTTP PUT
request instead of a
* POST
request, and the MatchingWorkflow
must already exist for the method to succeed.
*
*
* @param updateMatchingWorkflowRequest
* @return Result of the UpdateMatchingWorkflow operation returned by the service.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws InternalServerException
* This exception occurs when there is an internal failure in the Entity Resolution service.
* @throws ResourceNotFoundException
* The resource could not be found.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ValidationException
* The input fails to satisfy the constraints specified by Entity Resolution.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EntityResolutionException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EntityResolutionClient.UpdateMatchingWorkflow
* @see AWS API Documentation
*/
@Override
public UpdateMatchingWorkflowResponse updateMatchingWorkflow(UpdateMatchingWorkflowRequest updateMatchingWorkflowRequest)
throws ThrottlingException, InternalServerException, ResourceNotFoundException, AccessDeniedException,
ValidationException, AwsServiceException, SdkClientException, EntityResolutionException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, UpdateMatchingWorkflowResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(updateMatchingWorkflowRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, updateMatchingWorkflowRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "EntityResolution");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "UpdateMatchingWorkflow");
return clientHandler
.execute(new ClientExecutionParams()
.withOperationName("UpdateMatchingWorkflow").withProtocolMetadata(protocolMetadata)
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withInput(updateMatchingWorkflowRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new UpdateMatchingWorkflowRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Updates a schema mapping.
*
*
*
* A schema is immutable if it is being used by a workflow. Therefore, you can't update a schema mapping if it's
* associated with a workflow.
*
*
*
* @param updateSchemaMappingRequest
* @return Result of the UpdateSchemaMapping operation returned by the service.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws InternalServerException
* This exception occurs when there is an internal failure in the Entity Resolution service.
* @throws ResourceNotFoundException
* The resource could not be found.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ConflictException
* The request could not be processed because of conflict in the current state of the resource. Example:
* Workflow already exists, Schema already exists, Workflow is currently running, etc.
* @throws ValidationException
* The input fails to satisfy the constraints specified by Entity Resolution.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws EntityResolutionException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample EntityResolutionClient.UpdateSchemaMapping
* @see AWS API Documentation
*/
@Override
public UpdateSchemaMappingResponse updateSchemaMapping(UpdateSchemaMappingRequest updateSchemaMappingRequest)
throws ThrottlingException, InternalServerException, ResourceNotFoundException, AccessDeniedException,
ConflictException, ValidationException, AwsServiceException, SdkClientException, EntityResolutionException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, UpdateSchemaMappingResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(updateSchemaMappingRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, updateSchemaMappingRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "EntityResolution");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "UpdateSchemaMapping");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("UpdateSchemaMapping").withProtocolMetadata(protocolMetadata)
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withInput(updateSchemaMappingRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new UpdateSchemaMappingRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
@Override
public final String serviceName() {
return SERVICE_NAME;
}
private static List resolveMetricPublishers(SdkClientConfiguration clientConfiguration,
RequestOverrideConfiguration requestOverrideConfiguration) {
List publishers = null;
if (requestOverrideConfiguration != null) {
publishers = requestOverrideConfiguration.metricPublishers();
}
if (publishers == null || publishers.isEmpty()) {
publishers = clientConfiguration.option(SdkClientOption.METRIC_PUBLISHERS);
}
if (publishers == null) {
publishers = Collections.emptyList();
}
return publishers;
}
private HttpResponseHandler createErrorResponseHandler(BaseAwsJsonProtocolFactory protocolFactory,
JsonOperationMetadata operationMetadata) {
return protocolFactory.createErrorResponseHandler(operationMetadata);
}
private void updateRetryStrategyClientConfiguration(SdkClientConfiguration.Builder configuration) {
ClientOverrideConfiguration.Builder builder = configuration.asOverrideConfigurationBuilder();
RetryMode retryMode = builder.retryMode();
if (retryMode != null) {
configuration.option(SdkClientOption.RETRY_STRATEGY, AwsRetryStrategy.forRetryMode(retryMode));
} else {
Consumer> configurator = builder.retryStrategyConfigurator();
if (configurator != null) {
RetryStrategy.Builder, ?> defaultBuilder = AwsRetryStrategy.defaultRetryStrategy().toBuilder();
configurator.accept(defaultBuilder);
configuration.option(SdkClientOption.RETRY_STRATEGY, defaultBuilder.build());
} else {
RetryStrategy retryStrategy = builder.retryStrategy();
if (retryStrategy != null) {
configuration.option(SdkClientOption.RETRY_STRATEGY, retryStrategy);
}
}
}
configuration.option(SdkClientOption.CONFIGURED_RETRY_MODE, null);
configuration.option(SdkClientOption.CONFIGURED_RETRY_STRATEGY, null);
configuration.option(SdkClientOption.CONFIGURED_RETRY_CONFIGURATOR, null);
}
private SdkClientConfiguration updateSdkClientConfiguration(SdkRequest request, SdkClientConfiguration clientConfiguration) {
List plugins = request.overrideConfiguration().map(c -> c.plugins()).orElse(Collections.emptyList());
SdkClientConfiguration.Builder configuration = clientConfiguration.toBuilder();
if (plugins.isEmpty()) {
return configuration.build();
}
EntityResolutionServiceClientConfigurationBuilder serviceConfigBuilder = new EntityResolutionServiceClientConfigurationBuilder(
configuration);
for (SdkPlugin plugin : plugins) {
plugin.configureClient(serviceConfigBuilder);
}
updateRetryStrategyClientConfiguration(configuration);
return configuration.build();
}
private > T init(T builder) {
return builder
.clientConfiguration(clientConfiguration)
.defaultServiceExceptionSupplier(EntityResolutionException::builder)
.protocol(AwsJsonProtocol.REST_JSON)
.protocolVersion("1.1")
.registerModeledException(
ExceptionMetadata.builder().errorCode("ThrottlingException")
.exceptionBuilderSupplier(ThrottlingException::builder).httpStatusCode(429).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("InternalServerException")
.exceptionBuilderSupplier(InternalServerException::builder).httpStatusCode(500).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("AccessDeniedException")
.exceptionBuilderSupplier(AccessDeniedException::builder).httpStatusCode(403).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("ConflictException")
.exceptionBuilderSupplier(ConflictException::builder).httpStatusCode(400).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("ResourceNotFoundException")
.exceptionBuilderSupplier(ResourceNotFoundException::builder).httpStatusCode(404).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("ValidationException")
.exceptionBuilderSupplier(ValidationException::builder).httpStatusCode(400).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("ExceedsLimitException")
.exceptionBuilderSupplier(ExceedsLimitException::builder).httpStatusCode(402).build());
}
@Override
public final EntityResolutionServiceClientConfiguration serviceClientConfiguration() {
return new EntityResolutionServiceClientConfigurationBuilder(this.clientConfiguration.toBuilder()).build();
}
@Override
public void close() {
clientHandler.close();
}
}