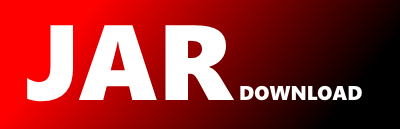
software.amazon.awssdk.services.entityresolution.model.IdMappingJobMetrics Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of entityresolution Show documentation
Show all versions of entityresolution Show documentation
The AWS Java SDK for Entity Resolution module holds the client classes that are used for
communicating with Entity Resolution.
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.entityresolution.model;
import java.io.Serializable;
import java.util.Arrays;
import java.util.Collections;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* An object containing InputRecords
, RecordsNotProcessed
, TotalRecordsProcessed
,
* TotalMappedRecords
, TotalMappedSourceRecords
, and TotalMappedTargetRecords
.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class IdMappingJobMetrics implements SdkPojo, Serializable,
ToCopyableBuilder {
private static final SdkField INPUT_RECORDS_FIELD = SdkField. builder(MarshallingType.INTEGER)
.memberName("inputRecords").getter(getter(IdMappingJobMetrics::inputRecords)).setter(setter(Builder::inputRecords))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("inputRecords").build()).build();
private static final SdkField RECORDS_NOT_PROCESSED_FIELD = SdkField. builder(MarshallingType.INTEGER)
.memberName("recordsNotProcessed").getter(getter(IdMappingJobMetrics::recordsNotProcessed))
.setter(setter(Builder::recordsNotProcessed))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("recordsNotProcessed").build())
.build();
private static final SdkField TOTAL_MAPPED_RECORDS_FIELD = SdkField. builder(MarshallingType.INTEGER)
.memberName("totalMappedRecords").getter(getter(IdMappingJobMetrics::totalMappedRecords))
.setter(setter(Builder::totalMappedRecords))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("totalMappedRecords").build())
.build();
private static final SdkField TOTAL_MAPPED_SOURCE_RECORDS_FIELD = SdkField
. builder(MarshallingType.INTEGER).memberName("totalMappedSourceRecords")
.getter(getter(IdMappingJobMetrics::totalMappedSourceRecords)).setter(setter(Builder::totalMappedSourceRecords))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("totalMappedSourceRecords").build())
.build();
private static final SdkField TOTAL_MAPPED_TARGET_RECORDS_FIELD = SdkField
. builder(MarshallingType.INTEGER).memberName("totalMappedTargetRecords")
.getter(getter(IdMappingJobMetrics::totalMappedTargetRecords)).setter(setter(Builder::totalMappedTargetRecords))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("totalMappedTargetRecords").build())
.build();
private static final SdkField TOTAL_RECORDS_PROCESSED_FIELD = SdkField. builder(MarshallingType.INTEGER)
.memberName("totalRecordsProcessed").getter(getter(IdMappingJobMetrics::totalRecordsProcessed))
.setter(setter(Builder::totalRecordsProcessed))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("totalRecordsProcessed").build())
.build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(INPUT_RECORDS_FIELD,
RECORDS_NOT_PROCESSED_FIELD, TOTAL_MAPPED_RECORDS_FIELD, TOTAL_MAPPED_SOURCE_RECORDS_FIELD,
TOTAL_MAPPED_TARGET_RECORDS_FIELD, TOTAL_RECORDS_PROCESSED_FIELD));
private static final Map> SDK_NAME_TO_FIELD = memberNameToFieldInitializer();
private static final long serialVersionUID = 1L;
private final Integer inputRecords;
private final Integer recordsNotProcessed;
private final Integer totalMappedRecords;
private final Integer totalMappedSourceRecords;
private final Integer totalMappedTargetRecords;
private final Integer totalRecordsProcessed;
private IdMappingJobMetrics(BuilderImpl builder) {
this.inputRecords = builder.inputRecords;
this.recordsNotProcessed = builder.recordsNotProcessed;
this.totalMappedRecords = builder.totalMappedRecords;
this.totalMappedSourceRecords = builder.totalMappedSourceRecords;
this.totalMappedTargetRecords = builder.totalMappedTargetRecords;
this.totalRecordsProcessed = builder.totalRecordsProcessed;
}
/**
*
* The total number of records that were input for processing.
*
*
* @return The total number of records that were input for processing.
*/
public final Integer inputRecords() {
return inputRecords;
}
/**
*
* The total number of records that did not get processed.
*
*
* @return The total number of records that did not get processed.
*/
public final Integer recordsNotProcessed() {
return recordsNotProcessed;
}
/**
*
* The total number of records that were mapped.
*
*
* @return The total number of records that were mapped.
*/
public final Integer totalMappedRecords() {
return totalMappedRecords;
}
/**
*
* The total number of mapped source records.
*
*
* @return The total number of mapped source records.
*/
public final Integer totalMappedSourceRecords() {
return totalMappedSourceRecords;
}
/**
*
* The total number of distinct mapped target records.
*
*
* @return The total number of distinct mapped target records.
*/
public final Integer totalMappedTargetRecords() {
return totalMappedTargetRecords;
}
/**
*
* The total number of records that were processed.
*
*
* @return The total number of records that were processed.
*/
public final Integer totalRecordsProcessed() {
return totalRecordsProcessed;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(inputRecords());
hashCode = 31 * hashCode + Objects.hashCode(recordsNotProcessed());
hashCode = 31 * hashCode + Objects.hashCode(totalMappedRecords());
hashCode = 31 * hashCode + Objects.hashCode(totalMappedSourceRecords());
hashCode = 31 * hashCode + Objects.hashCode(totalMappedTargetRecords());
hashCode = 31 * hashCode + Objects.hashCode(totalRecordsProcessed());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof IdMappingJobMetrics)) {
return false;
}
IdMappingJobMetrics other = (IdMappingJobMetrics) obj;
return Objects.equals(inputRecords(), other.inputRecords())
&& Objects.equals(recordsNotProcessed(), other.recordsNotProcessed())
&& Objects.equals(totalMappedRecords(), other.totalMappedRecords())
&& Objects.equals(totalMappedSourceRecords(), other.totalMappedSourceRecords())
&& Objects.equals(totalMappedTargetRecords(), other.totalMappedTargetRecords())
&& Objects.equals(totalRecordsProcessed(), other.totalRecordsProcessed());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("IdMappingJobMetrics").add("InputRecords", inputRecords())
.add("RecordsNotProcessed", recordsNotProcessed()).add("TotalMappedRecords", totalMappedRecords())
.add("TotalMappedSourceRecords", totalMappedSourceRecords())
.add("TotalMappedTargetRecords", totalMappedTargetRecords())
.add("TotalRecordsProcessed", totalRecordsProcessed()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "inputRecords":
return Optional.ofNullable(clazz.cast(inputRecords()));
case "recordsNotProcessed":
return Optional.ofNullable(clazz.cast(recordsNotProcessed()));
case "totalMappedRecords":
return Optional.ofNullable(clazz.cast(totalMappedRecords()));
case "totalMappedSourceRecords":
return Optional.ofNullable(clazz.cast(totalMappedSourceRecords()));
case "totalMappedTargetRecords":
return Optional.ofNullable(clazz.cast(totalMappedTargetRecords()));
case "totalRecordsProcessed":
return Optional.ofNullable(clazz.cast(totalRecordsProcessed()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
@Override
public final Map> sdkFieldNameToField() {
return SDK_NAME_TO_FIELD;
}
private static Map> memberNameToFieldInitializer() {
Map> map = new HashMap<>();
map.put("inputRecords", INPUT_RECORDS_FIELD);
map.put("recordsNotProcessed", RECORDS_NOT_PROCESSED_FIELD);
map.put("totalMappedRecords", TOTAL_MAPPED_RECORDS_FIELD);
map.put("totalMappedSourceRecords", TOTAL_MAPPED_SOURCE_RECORDS_FIELD);
map.put("totalMappedTargetRecords", TOTAL_MAPPED_TARGET_RECORDS_FIELD);
map.put("totalRecordsProcessed", TOTAL_RECORDS_PROCESSED_FIELD);
return Collections.unmodifiableMap(map);
}
private static Function
© 2015 - 2025 Weber Informatics LLC | Privacy Policy