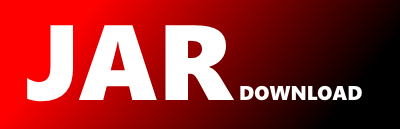
software.amazon.awssdk.services.eventbridge.model.PutPermissionRequest Maven / Gradle / Ivy
Show all versions of eventbridge Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.eventbridge.model;
import java.util.Arrays;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.awscore.AwsRequestOverrideConfiguration;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*/
@Generated("software.amazon.awssdk:codegen")
public final class PutPermissionRequest extends EventBridgeRequest implements
ToCopyableBuilder {
private static final SdkField EVENT_BUS_NAME_FIELD = SdkField. builder(MarshallingType.STRING)
.getter(getter(PutPermissionRequest::eventBusName)).setter(setter(Builder::eventBusName))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("EventBusName").build()).build();
private static final SdkField ACTION_FIELD = SdkField. builder(MarshallingType.STRING)
.getter(getter(PutPermissionRequest::action)).setter(setter(Builder::action))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Action").build()).build();
private static final SdkField PRINCIPAL_FIELD = SdkField. builder(MarshallingType.STRING)
.getter(getter(PutPermissionRequest::principal)).setter(setter(Builder::principal))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Principal").build()).build();
private static final SdkField STATEMENT_ID_FIELD = SdkField. builder(MarshallingType.STRING)
.getter(getter(PutPermissionRequest::statementId)).setter(setter(Builder::statementId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("StatementId").build()).build();
private static final SdkField CONDITION_FIELD = SdkField. builder(MarshallingType.SDK_POJO)
.getter(getter(PutPermissionRequest::condition)).setter(setter(Builder::condition)).constructor(Condition::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Condition").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(EVENT_BUS_NAME_FIELD,
ACTION_FIELD, PRINCIPAL_FIELD, STATEMENT_ID_FIELD, CONDITION_FIELD));
private final String eventBusName;
private final String action;
private final String principal;
private final String statementId;
private final Condition condition;
private PutPermissionRequest(BuilderImpl builder) {
super(builder);
this.eventBusName = builder.eventBusName;
this.action = builder.action;
this.principal = builder.principal;
this.statementId = builder.statementId;
this.condition = builder.condition;
}
/**
*
* The event bus associated with the rule. If you omit this, the default event bus is used.
*
*
* @return The event bus associated with the rule. If you omit this, the default event bus is used.
*/
public String eventBusName() {
return eventBusName;
}
/**
*
* The action that you are enabling the other account to perform. Currently, this must be
* events:PutEvents
.
*
*
* @return The action that you are enabling the other account to perform. Currently, this must be
* events:PutEvents
.
*/
public String action() {
return action;
}
/**
*
* The 12-digit AWS account ID that you are permitting to put events to your default event bus. Specify "*" to
* permit any account to put events to your default event bus.
*
*
* If you specify "*" without specifying Condition
, avoid creating rules that may match undesirable
* events. To create more secure rules, make sure that the event pattern for each rule contains an
* account
field with a specific account ID from which to receive events. Rules with an account field
* do not match any events sent from other accounts.
*
*
* @return The 12-digit AWS account ID that you are permitting to put events to your default event bus. Specify "*"
* to permit any account to put events to your default event bus.
*
* If you specify "*" without specifying Condition
, avoid creating rules that may match
* undesirable events. To create more secure rules, make sure that the event pattern for each rule contains
* an account
field with a specific account ID from which to receive events. Rules with an
* account field do not match any events sent from other accounts.
*/
public String principal() {
return principal;
}
/**
*
* An identifier string for the external account that you are granting permissions to. If you later want to revoke
* the permission for this external account, specify this StatementId
when you run
* RemovePermission.
*
*
* @return An identifier string for the external account that you are granting permissions to. If you later want to
* revoke the permission for this external account, specify this StatementId
when you run
* RemovePermission.
*/
public String statementId() {
return statementId;
}
/**
*
* This parameter enables you to limit the permission to accounts that fulfill a certain condition, such as being a
* member of a certain AWS organization. For more information about AWS Organizations, see What Is AWS
* Organizations in the AWS Organizations User Guide.
*
*
* If you specify Condition
with an AWS organization ID, and specify "*" as the value for
* Principal
, you grant permission to all the accounts in the named organization.
*
*
* The Condition
is a JSON string which must contain Type
, Key
, and
* Value
fields.
*
*
* @return This parameter enables you to limit the permission to accounts that fulfill a certain condition, such as
* being a member of a certain AWS organization. For more information about AWS Organizations, see What Is AWS
* Organizations in the AWS Organizations User Guide.
*
* If you specify Condition
with an AWS organization ID, and specify "*" as the value for
* Principal
, you grant permission to all the accounts in the named organization.
*
*
* The Condition
is a JSON string which must contain Type
, Key
, and
* Value
fields.
*/
public Condition condition() {
return condition;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + super.hashCode();
hashCode = 31 * hashCode + Objects.hashCode(eventBusName());
hashCode = 31 * hashCode + Objects.hashCode(action());
hashCode = 31 * hashCode + Objects.hashCode(principal());
hashCode = 31 * hashCode + Objects.hashCode(statementId());
hashCode = 31 * hashCode + Objects.hashCode(condition());
return hashCode;
}
@Override
public boolean equals(Object obj) {
return super.equals(obj) && equalsBySdkFields(obj);
}
@Override
public boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof PutPermissionRequest)) {
return false;
}
PutPermissionRequest other = (PutPermissionRequest) obj;
return Objects.equals(eventBusName(), other.eventBusName()) && Objects.equals(action(), other.action())
&& Objects.equals(principal(), other.principal()) && Objects.equals(statementId(), other.statementId())
&& Objects.equals(condition(), other.condition());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public String toString() {
return ToString.builder("PutPermissionRequest").add("EventBusName", eventBusName()).add("Action", action())
.add("Principal", principal()).add("StatementId", statementId()).add("Condition", condition()).build();
}
public Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "EventBusName":
return Optional.ofNullable(clazz.cast(eventBusName()));
case "Action":
return Optional.ofNullable(clazz.cast(action()));
case "Principal":
return Optional.ofNullable(clazz.cast(principal()));
case "StatementId":
return Optional.ofNullable(clazz.cast(statementId()));
case "Condition":
return Optional.ofNullable(clazz.cast(condition()));
default:
return Optional.empty();
}
}
@Override
public List> sdkFields() {
return SDK_FIELDS;
}
private static Function
*
* If you specify "*" without specifying Condition
, avoid creating rules that may match
* undesirable events. To create more secure rules, make sure that the event pattern for each rule
* contains an account
field with a specific account ID from which to receive events. Rules
* with an account field do not match any events sent from other accounts.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder principal(String principal);
/**
*
* An identifier string for the external account that you are granting permissions to. If you later want to
* revoke the permission for this external account, specify this StatementId
when you run
* RemovePermission.
*
*
* @param statementId
* An identifier string for the external account that you are granting permissions to. If you later want
* to revoke the permission for this external account, specify this StatementId
when you run
* RemovePermission.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder statementId(String statementId);
/**
*
* This parameter enables you to limit the permission to accounts that fulfill a certain condition, such as
* being a member of a certain AWS organization. For more information about AWS Organizations, see What Is AWS
* Organizations in the AWS Organizations User Guide.
*
*
* If you specify Condition
with an AWS organization ID, and specify "*" as the value for
* Principal
, you grant permission to all the accounts in the named organization.
*
*
* The Condition
is a JSON string which must contain Type
, Key
, and
* Value
fields.
*
*
* @param condition
* This parameter enables you to limit the permission to accounts that fulfill a certain condition, such
* as being a member of a certain AWS organization. For more information about AWS Organizations, see What Is AWS
* Organizations in the AWS Organizations User Guide.
*
* If you specify Condition
with an AWS organization ID, and specify "*" as the value for
* Principal
, you grant permission to all the accounts in the named organization.
*
*
* The Condition
is a JSON string which must contain Type
, Key
,
* and Value
fields.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder condition(Condition condition);
/**
*
* This parameter enables you to limit the permission to accounts that fulfill a certain condition, such as
* being a member of a certain AWS organization. For more information about AWS Organizations, see What Is AWS
* Organizations in the AWS Organizations User Guide.
*
*
* If you specify Condition
with an AWS organization ID, and specify "*" as the value for
* Principal
, you grant permission to all the accounts in the named organization.
*
*
* The Condition
is a JSON string which must contain Type
, Key
, and
* Value
fields.
*
* This is a convenience that creates an instance of the {@link Condition.Builder} avoiding the need to create
* one manually via {@link Condition#builder()}.
*
* When the {@link Consumer} completes, {@link Condition.Builder#build()} is called immediately and its result
* is passed to {@link #condition(Condition)}.
*
* @param condition
* a consumer that will call methods on {@link Condition.Builder}
* @return Returns a reference to this object so that method calls can be chained together.
* @see #condition(Condition)
*/
default Builder condition(Consumer condition) {
return condition(Condition.builder().applyMutation(condition).build());
}
@Override
Builder overrideConfiguration(AwsRequestOverrideConfiguration overrideConfiguration);
@Override
Builder overrideConfiguration(Consumer builderConsumer);
}
static final class BuilderImpl extends EventBridgeRequest.BuilderImpl implements Builder {
private String eventBusName;
private String action;
private String principal;
private String statementId;
private Condition condition;
private BuilderImpl() {
}
private BuilderImpl(PutPermissionRequest model) {
super(model);
eventBusName(model.eventBusName);
action(model.action);
principal(model.principal);
statementId(model.statementId);
condition(model.condition);
}
public final String getEventBusName() {
return eventBusName;
}
@Override
public final Builder eventBusName(String eventBusName) {
this.eventBusName = eventBusName;
return this;
}
public final void setEventBusName(String eventBusName) {
this.eventBusName = eventBusName;
}
public final String getAction() {
return action;
}
@Override
public final Builder action(String action) {
this.action = action;
return this;
}
public final void setAction(String action) {
this.action = action;
}
public final String getPrincipal() {
return principal;
}
@Override
public final Builder principal(String principal) {
this.principal = principal;
return this;
}
public final void setPrincipal(String principal) {
this.principal = principal;
}
public final String getStatementId() {
return statementId;
}
@Override
public final Builder statementId(String statementId) {
this.statementId = statementId;
return this;
}
public final void setStatementId(String statementId) {
this.statementId = statementId;
}
public final Condition.Builder getCondition() {
return condition != null ? condition.toBuilder() : null;
}
@Override
public final Builder condition(Condition condition) {
this.condition = condition;
return this;
}
public final void setCondition(Condition.BuilderImpl condition) {
this.condition = condition != null ? condition.build() : null;
}
@Override
public Builder overrideConfiguration(AwsRequestOverrideConfiguration overrideConfiguration) {
super.overrideConfiguration(overrideConfiguration);
return this;
}
@Override
public Builder overrideConfiguration(Consumer builderConsumer) {
super.overrideConfiguration(builderConsumer);
return this;
}
@Override
public PutPermissionRequest build() {
return new PutPermissionRequest(this);
}
@Override
public List> sdkFields() {
return SDK_FIELDS;
}
}
}