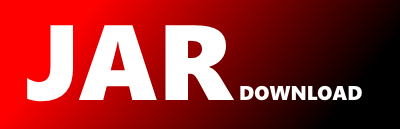
software.amazon.awssdk.services.eventbridge.model.Target Maven / Gradle / Ivy
Show all versions of eventbridge Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.eventbridge.model;
import java.io.Serializable;
import java.util.Arrays;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* Targets are the resources to be invoked when a rule is triggered. For a complete list of services and resources that
* can be set as a target, see PutTargets.
*
*
* If you are setting the event bus of another account as the target, and that account granted permission to your
* account through an organization instead of directly by the account ID, then you must specify a RoleArn
* with proper permissions in the Target
structure. For more information, see Sending
* and Receiving Events Between AWS Accounts in the Amazon EventBridge User Guide.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class Target implements SdkPojo, Serializable, ToCopyableBuilder {
private static final SdkField ID_FIELD = SdkField. builder(MarshallingType.STRING).getter(getter(Target::id))
.setter(setter(Builder::id))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Id").build()).build();
private static final SdkField ARN_FIELD = SdkField. builder(MarshallingType.STRING)
.getter(getter(Target::arn)).setter(setter(Builder::arn))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Arn").build()).build();
private static final SdkField ROLE_ARN_FIELD = SdkField. builder(MarshallingType.STRING)
.getter(getter(Target::roleArn)).setter(setter(Builder::roleArn))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("RoleArn").build()).build();
private static final SdkField INPUT_FIELD = SdkField. builder(MarshallingType.STRING)
.getter(getter(Target::input)).setter(setter(Builder::input))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Input").build()).build();
private static final SdkField INPUT_PATH_FIELD = SdkField. builder(MarshallingType.STRING)
.getter(getter(Target::inputPath)).setter(setter(Builder::inputPath))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("InputPath").build()).build();
private static final SdkField INPUT_TRANSFORMER_FIELD = SdkField
. builder(MarshallingType.SDK_POJO).getter(getter(Target::inputTransformer))
.setter(setter(Builder::inputTransformer)).constructor(InputTransformer::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("InputTransformer").build()).build();
private static final SdkField KINESIS_PARAMETERS_FIELD = SdkField
. builder(MarshallingType.SDK_POJO).getter(getter(Target::kinesisParameters))
.setter(setter(Builder::kinesisParameters)).constructor(KinesisParameters::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("KinesisParameters").build()).build();
private static final SdkField RUN_COMMAND_PARAMETERS_FIELD = SdkField
. builder(MarshallingType.SDK_POJO).getter(getter(Target::runCommandParameters))
.setter(setter(Builder::runCommandParameters)).constructor(RunCommandParameters::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("RunCommandParameters").build())
.build();
private static final SdkField ECS_PARAMETERS_FIELD = SdkField
. builder(MarshallingType.SDK_POJO).getter(getter(Target::ecsParameters))
.setter(setter(Builder::ecsParameters)).constructor(EcsParameters::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("EcsParameters").build()).build();
private static final SdkField BATCH_PARAMETERS_FIELD = SdkField
. builder(MarshallingType.SDK_POJO).getter(getter(Target::batchParameters))
.setter(setter(Builder::batchParameters)).constructor(BatchParameters::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("BatchParameters").build()).build();
private static final SdkField SQS_PARAMETERS_FIELD = SdkField
. builder(MarshallingType.SDK_POJO).getter(getter(Target::sqsParameters))
.setter(setter(Builder::sqsParameters)).constructor(SqsParameters::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("SqsParameters").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(ID_FIELD, ARN_FIELD,
ROLE_ARN_FIELD, INPUT_FIELD, INPUT_PATH_FIELD, INPUT_TRANSFORMER_FIELD, KINESIS_PARAMETERS_FIELD,
RUN_COMMAND_PARAMETERS_FIELD, ECS_PARAMETERS_FIELD, BATCH_PARAMETERS_FIELD, SQS_PARAMETERS_FIELD));
private static final long serialVersionUID = 1L;
private final String id;
private final String arn;
private final String roleArn;
private final String input;
private final String inputPath;
private final InputTransformer inputTransformer;
private final KinesisParameters kinesisParameters;
private final RunCommandParameters runCommandParameters;
private final EcsParameters ecsParameters;
private final BatchParameters batchParameters;
private final SqsParameters sqsParameters;
private Target(BuilderImpl builder) {
this.id = builder.id;
this.arn = builder.arn;
this.roleArn = builder.roleArn;
this.input = builder.input;
this.inputPath = builder.inputPath;
this.inputTransformer = builder.inputTransformer;
this.kinesisParameters = builder.kinesisParameters;
this.runCommandParameters = builder.runCommandParameters;
this.ecsParameters = builder.ecsParameters;
this.batchParameters = builder.batchParameters;
this.sqsParameters = builder.sqsParameters;
}
/**
*
* The ID of the target.
*
*
* @return The ID of the target.
*/
public String id() {
return id;
}
/**
*
* The Amazon Resource Name (ARN) of the target.
*
*
* @return The Amazon Resource Name (ARN) of the target.
*/
public String arn() {
return arn;
}
/**
*
* The Amazon Resource Name (ARN) of the IAM role to be used for this target when the rule is triggered. If one rule
* triggers multiple targets, you can use a different IAM role for each target.
*
*
* @return The Amazon Resource Name (ARN) of the IAM role to be used for this target when the rule is triggered. If
* one rule triggers multiple targets, you can use a different IAM role for each target.
*/
public String roleArn() {
return roleArn;
}
/**
*
* Valid JSON text passed to the target. In this case, nothing from the event itself is passed to the target. For
* more information, see The JavaScript Object Notation (JSON)
* Data Interchange Format.
*
*
* @return Valid JSON text passed to the target. In this case, nothing from the event itself is passed to the
* target. For more information, see The JavaScript
* Object Notation (JSON) Data Interchange Format.
*/
public String input() {
return input;
}
/**
*
* The value of the JSONPath that is used for extracting part of the matched event when passing it to the target.
* You must use JSON dot notation, not bracket notation. For more information about JSON paths, see JSONPath.
*
*
* @return The value of the JSONPath that is used for extracting part of the matched event when passing it to the
* target. You must use JSON dot notation, not bracket notation. For more information about JSON paths, see
* JSONPath.
*/
public String inputPath() {
return inputPath;
}
/**
*
* Settings to enable you to provide custom input to a target based on certain event data. You can extract one or
* more key-value pairs from the event and then use that data to send customized input to the target.
*
*
* @return Settings to enable you to provide custom input to a target based on certain event data. You can extract
* one or more key-value pairs from the event and then use that data to send customized input to the target.
*/
public InputTransformer inputTransformer() {
return inputTransformer;
}
/**
*
* The custom parameter you can use to control the shard assignment, when the target is a Kinesis data stream. If
* you do not include this parameter, the default is to use the eventId
as the partition key.
*
*
* @return The custom parameter you can use to control the shard assignment, when the target is a Kinesis data
* stream. If you do not include this parameter, the default is to use the eventId
as the
* partition key.
*/
public KinesisParameters kinesisParameters() {
return kinesisParameters;
}
/**
*
* Parameters used when you are using the rule to invoke Amazon EC2 Run Command.
*
*
* @return Parameters used when you are using the rule to invoke Amazon EC2 Run Command.
*/
public RunCommandParameters runCommandParameters() {
return runCommandParameters;
}
/**
*
* Contains the Amazon ECS task definition and task count to be used, if the event target is an Amazon ECS task. For
* more information about Amazon ECS tasks, see Task Definitions in
* the Amazon EC2 Container Service Developer Guide.
*
*
* @return Contains the Amazon ECS task definition and task count to be used, if the event target is an Amazon ECS
* task. For more information about Amazon ECS tasks, see Task Definitions
* in the Amazon EC2 Container Service Developer Guide.
*/
public EcsParameters ecsParameters() {
return ecsParameters;
}
/**
*
* If the event target is an AWS Batch job, this contains the job definition, job name, and other parameters. For
* more information, see Jobs in the
* AWS Batch User Guide.
*
*
* @return If the event target is an AWS Batch job, this contains the job definition, job name, and other
* parameters. For more information, see Jobs in the AWS Batch User
* Guide.
*/
public BatchParameters batchParameters() {
return batchParameters;
}
/**
*
* Contains the message group ID to use when the target is a FIFO queue.
*
*
* If you specify an SQS FIFO queue as a target, the queue must have content-based deduplication enabled.
*
*
* @return Contains the message group ID to use when the target is a FIFO queue.
*
* If you specify an SQS FIFO queue as a target, the queue must have content-based deduplication enabled.
*/
public SqsParameters sqsParameters() {
return sqsParameters;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(id());
hashCode = 31 * hashCode + Objects.hashCode(arn());
hashCode = 31 * hashCode + Objects.hashCode(roleArn());
hashCode = 31 * hashCode + Objects.hashCode(input());
hashCode = 31 * hashCode + Objects.hashCode(inputPath());
hashCode = 31 * hashCode + Objects.hashCode(inputTransformer());
hashCode = 31 * hashCode + Objects.hashCode(kinesisParameters());
hashCode = 31 * hashCode + Objects.hashCode(runCommandParameters());
hashCode = 31 * hashCode + Objects.hashCode(ecsParameters());
hashCode = 31 * hashCode + Objects.hashCode(batchParameters());
hashCode = 31 * hashCode + Objects.hashCode(sqsParameters());
return hashCode;
}
@Override
public boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof Target)) {
return false;
}
Target other = (Target) obj;
return Objects.equals(id(), other.id()) && Objects.equals(arn(), other.arn())
&& Objects.equals(roleArn(), other.roleArn()) && Objects.equals(input(), other.input())
&& Objects.equals(inputPath(), other.inputPath()) && Objects.equals(inputTransformer(), other.inputTransformer())
&& Objects.equals(kinesisParameters(), other.kinesisParameters())
&& Objects.equals(runCommandParameters(), other.runCommandParameters())
&& Objects.equals(ecsParameters(), other.ecsParameters())
&& Objects.equals(batchParameters(), other.batchParameters())
&& Objects.equals(sqsParameters(), other.sqsParameters());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public String toString() {
return ToString.builder("Target").add("Id", id()).add("Arn", arn()).add("RoleArn", roleArn()).add("Input", input())
.add("InputPath", inputPath()).add("InputTransformer", inputTransformer())
.add("KinesisParameters", kinesisParameters()).add("RunCommandParameters", runCommandParameters())
.add("EcsParameters", ecsParameters()).add("BatchParameters", batchParameters())
.add("SqsParameters", sqsParameters()).build();
}
public Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "Id":
return Optional.ofNullable(clazz.cast(id()));
case "Arn":
return Optional.ofNullable(clazz.cast(arn()));
case "RoleArn":
return Optional.ofNullable(clazz.cast(roleArn()));
case "Input":
return Optional.ofNullable(clazz.cast(input()));
case "InputPath":
return Optional.ofNullable(clazz.cast(inputPath()));
case "InputTransformer":
return Optional.ofNullable(clazz.cast(inputTransformer()));
case "KinesisParameters":
return Optional.ofNullable(clazz.cast(kinesisParameters()));
case "RunCommandParameters":
return Optional.ofNullable(clazz.cast(runCommandParameters()));
case "EcsParameters":
return Optional.ofNullable(clazz.cast(ecsParameters()));
case "BatchParameters":
return Optional.ofNullable(clazz.cast(batchParameters()));
case "SqsParameters":
return Optional.ofNullable(clazz.cast(sqsParameters()));
default:
return Optional.empty();
}
}
@Override
public List> sdkFields() {
return SDK_FIELDS;
}
private static Function
*
* If you specify an SQS FIFO queue as a target, the queue must have content-based deduplication enabled.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder sqsParameters(SqsParameters sqsParameters);
/**
*
* Contains the message group ID to use when the target is a FIFO queue.
*
*
* If you specify an SQS FIFO queue as a target, the queue must have content-based deduplication enabled.
*
* This is a convenience that creates an instance of the {@link SqsParameters.Builder} avoiding the need to
* create one manually via {@link SqsParameters#builder()}.
*
* When the {@link Consumer} completes, {@link SqsParameters.Builder#build()} is called immediately and its
* result is passed to {@link #sqsParameters(SqsParameters)}.
*
* @param sqsParameters
* a consumer that will call methods on {@link SqsParameters.Builder}
* @return Returns a reference to this object so that method calls can be chained together.
* @see #sqsParameters(SqsParameters)
*/
default Builder sqsParameters(Consumer sqsParameters) {
return sqsParameters(SqsParameters.builder().applyMutation(sqsParameters).build());
}
}
static final class BuilderImpl implements Builder {
private String id;
private String arn;
private String roleArn;
private String input;
private String inputPath;
private InputTransformer inputTransformer;
private KinesisParameters kinesisParameters;
private RunCommandParameters runCommandParameters;
private EcsParameters ecsParameters;
private BatchParameters batchParameters;
private SqsParameters sqsParameters;
private BuilderImpl() {
}
private BuilderImpl(Target model) {
id(model.id);
arn(model.arn);
roleArn(model.roleArn);
input(model.input);
inputPath(model.inputPath);
inputTransformer(model.inputTransformer);
kinesisParameters(model.kinesisParameters);
runCommandParameters(model.runCommandParameters);
ecsParameters(model.ecsParameters);
batchParameters(model.batchParameters);
sqsParameters(model.sqsParameters);
}
public final String getId() {
return id;
}
@Override
public final Builder id(String id) {
this.id = id;
return this;
}
public final void setId(String id) {
this.id = id;
}
public final String getArn() {
return arn;
}
@Override
public final Builder arn(String arn) {
this.arn = arn;
return this;
}
public final void setArn(String arn) {
this.arn = arn;
}
public final String getRoleArn() {
return roleArn;
}
@Override
public final Builder roleArn(String roleArn) {
this.roleArn = roleArn;
return this;
}
public final void setRoleArn(String roleArn) {
this.roleArn = roleArn;
}
public final String getInput() {
return input;
}
@Override
public final Builder input(String input) {
this.input = input;
return this;
}
public final void setInput(String input) {
this.input = input;
}
public final String getInputPath() {
return inputPath;
}
@Override
public final Builder inputPath(String inputPath) {
this.inputPath = inputPath;
return this;
}
public final void setInputPath(String inputPath) {
this.inputPath = inputPath;
}
public final InputTransformer.Builder getInputTransformer() {
return inputTransformer != null ? inputTransformer.toBuilder() : null;
}
@Override
public final Builder inputTransformer(InputTransformer inputTransformer) {
this.inputTransformer = inputTransformer;
return this;
}
public final void setInputTransformer(InputTransformer.BuilderImpl inputTransformer) {
this.inputTransformer = inputTransformer != null ? inputTransformer.build() : null;
}
public final KinesisParameters.Builder getKinesisParameters() {
return kinesisParameters != null ? kinesisParameters.toBuilder() : null;
}
@Override
public final Builder kinesisParameters(KinesisParameters kinesisParameters) {
this.kinesisParameters = kinesisParameters;
return this;
}
public final void setKinesisParameters(KinesisParameters.BuilderImpl kinesisParameters) {
this.kinesisParameters = kinesisParameters != null ? kinesisParameters.build() : null;
}
public final RunCommandParameters.Builder getRunCommandParameters() {
return runCommandParameters != null ? runCommandParameters.toBuilder() : null;
}
@Override
public final Builder runCommandParameters(RunCommandParameters runCommandParameters) {
this.runCommandParameters = runCommandParameters;
return this;
}
public final void setRunCommandParameters(RunCommandParameters.BuilderImpl runCommandParameters) {
this.runCommandParameters = runCommandParameters != null ? runCommandParameters.build() : null;
}
public final EcsParameters.Builder getEcsParameters() {
return ecsParameters != null ? ecsParameters.toBuilder() : null;
}
@Override
public final Builder ecsParameters(EcsParameters ecsParameters) {
this.ecsParameters = ecsParameters;
return this;
}
public final void setEcsParameters(EcsParameters.BuilderImpl ecsParameters) {
this.ecsParameters = ecsParameters != null ? ecsParameters.build() : null;
}
public final BatchParameters.Builder getBatchParameters() {
return batchParameters != null ? batchParameters.toBuilder() : null;
}
@Override
public final Builder batchParameters(BatchParameters batchParameters) {
this.batchParameters = batchParameters;
return this;
}
public final void setBatchParameters(BatchParameters.BuilderImpl batchParameters) {
this.batchParameters = batchParameters != null ? batchParameters.build() : null;
}
public final SqsParameters.Builder getSqsParameters() {
return sqsParameters != null ? sqsParameters.toBuilder() : null;
}
@Override
public final Builder sqsParameters(SqsParameters sqsParameters) {
this.sqsParameters = sqsParameters;
return this;
}
public final void setSqsParameters(SqsParameters.BuilderImpl sqsParameters) {
this.sqsParameters = sqsParameters != null ? sqsParameters.build() : null;
}
@Override
public Target build() {
return new Target(this);
}
@Override
public List> sdkFields() {
return SDK_FIELDS;
}
}
}