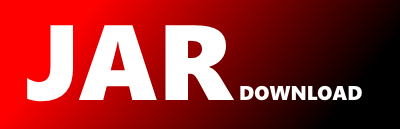
software.amazon.awssdk.services.eventbridge.DefaultEventBridgeAsyncClient Maven / Gradle / Ivy
Show all versions of eventbridge Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.eventbridge;
import java.util.Collections;
import java.util.List;
import java.util.concurrent.CompletableFuture;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.annotations.SdkInternalApi;
import software.amazon.awssdk.awscore.AwsRequestOverrideConfiguration;
import software.amazon.awssdk.awscore.client.handler.AwsAsyncClientHandler;
import software.amazon.awssdk.awscore.exception.AwsServiceException;
import software.amazon.awssdk.core.RequestOverrideConfiguration;
import software.amazon.awssdk.core.client.config.SdkClientConfiguration;
import software.amazon.awssdk.core.client.config.SdkClientOption;
import software.amazon.awssdk.core.client.handler.AsyncClientHandler;
import software.amazon.awssdk.core.client.handler.ClientExecutionParams;
import software.amazon.awssdk.core.http.HttpResponseHandler;
import software.amazon.awssdk.core.metrics.CoreMetric;
import software.amazon.awssdk.metrics.MetricCollector;
import software.amazon.awssdk.metrics.MetricPublisher;
import software.amazon.awssdk.metrics.NoOpMetricCollector;
import software.amazon.awssdk.protocols.core.ExceptionMetadata;
import software.amazon.awssdk.protocols.json.AwsJsonProtocol;
import software.amazon.awssdk.protocols.json.AwsJsonProtocolFactory;
import software.amazon.awssdk.protocols.json.BaseAwsJsonProtocolFactory;
import software.amazon.awssdk.protocols.json.JsonOperationMetadata;
import software.amazon.awssdk.services.eventbridge.model.ActivateEventSourceRequest;
import software.amazon.awssdk.services.eventbridge.model.ActivateEventSourceResponse;
import software.amazon.awssdk.services.eventbridge.model.CancelReplayRequest;
import software.amazon.awssdk.services.eventbridge.model.CancelReplayResponse;
import software.amazon.awssdk.services.eventbridge.model.ConcurrentModificationException;
import software.amazon.awssdk.services.eventbridge.model.CreateArchiveRequest;
import software.amazon.awssdk.services.eventbridge.model.CreateArchiveResponse;
import software.amazon.awssdk.services.eventbridge.model.CreateEventBusRequest;
import software.amazon.awssdk.services.eventbridge.model.CreateEventBusResponse;
import software.amazon.awssdk.services.eventbridge.model.CreatePartnerEventSourceRequest;
import software.amazon.awssdk.services.eventbridge.model.CreatePartnerEventSourceResponse;
import software.amazon.awssdk.services.eventbridge.model.DeactivateEventSourceRequest;
import software.amazon.awssdk.services.eventbridge.model.DeactivateEventSourceResponse;
import software.amazon.awssdk.services.eventbridge.model.DeleteArchiveRequest;
import software.amazon.awssdk.services.eventbridge.model.DeleteArchiveResponse;
import software.amazon.awssdk.services.eventbridge.model.DeleteEventBusRequest;
import software.amazon.awssdk.services.eventbridge.model.DeleteEventBusResponse;
import software.amazon.awssdk.services.eventbridge.model.DeletePartnerEventSourceRequest;
import software.amazon.awssdk.services.eventbridge.model.DeletePartnerEventSourceResponse;
import software.amazon.awssdk.services.eventbridge.model.DeleteRuleRequest;
import software.amazon.awssdk.services.eventbridge.model.DeleteRuleResponse;
import software.amazon.awssdk.services.eventbridge.model.DescribeArchiveRequest;
import software.amazon.awssdk.services.eventbridge.model.DescribeArchiveResponse;
import software.amazon.awssdk.services.eventbridge.model.DescribeEventBusRequest;
import software.amazon.awssdk.services.eventbridge.model.DescribeEventBusResponse;
import software.amazon.awssdk.services.eventbridge.model.DescribeEventSourceRequest;
import software.amazon.awssdk.services.eventbridge.model.DescribeEventSourceResponse;
import software.amazon.awssdk.services.eventbridge.model.DescribePartnerEventSourceRequest;
import software.amazon.awssdk.services.eventbridge.model.DescribePartnerEventSourceResponse;
import software.amazon.awssdk.services.eventbridge.model.DescribeReplayRequest;
import software.amazon.awssdk.services.eventbridge.model.DescribeReplayResponse;
import software.amazon.awssdk.services.eventbridge.model.DescribeRuleRequest;
import software.amazon.awssdk.services.eventbridge.model.DescribeRuleResponse;
import software.amazon.awssdk.services.eventbridge.model.DisableRuleRequest;
import software.amazon.awssdk.services.eventbridge.model.DisableRuleResponse;
import software.amazon.awssdk.services.eventbridge.model.EnableRuleRequest;
import software.amazon.awssdk.services.eventbridge.model.EnableRuleResponse;
import software.amazon.awssdk.services.eventbridge.model.EventBridgeException;
import software.amazon.awssdk.services.eventbridge.model.IllegalStatusException;
import software.amazon.awssdk.services.eventbridge.model.InternalException;
import software.amazon.awssdk.services.eventbridge.model.InvalidEventPatternException;
import software.amazon.awssdk.services.eventbridge.model.InvalidStateException;
import software.amazon.awssdk.services.eventbridge.model.LimitExceededException;
import software.amazon.awssdk.services.eventbridge.model.ListArchivesRequest;
import software.amazon.awssdk.services.eventbridge.model.ListArchivesResponse;
import software.amazon.awssdk.services.eventbridge.model.ListEventBusesRequest;
import software.amazon.awssdk.services.eventbridge.model.ListEventBusesResponse;
import software.amazon.awssdk.services.eventbridge.model.ListEventSourcesRequest;
import software.amazon.awssdk.services.eventbridge.model.ListEventSourcesResponse;
import software.amazon.awssdk.services.eventbridge.model.ListPartnerEventSourceAccountsRequest;
import software.amazon.awssdk.services.eventbridge.model.ListPartnerEventSourceAccountsResponse;
import software.amazon.awssdk.services.eventbridge.model.ListPartnerEventSourcesRequest;
import software.amazon.awssdk.services.eventbridge.model.ListPartnerEventSourcesResponse;
import software.amazon.awssdk.services.eventbridge.model.ListReplaysRequest;
import software.amazon.awssdk.services.eventbridge.model.ListReplaysResponse;
import software.amazon.awssdk.services.eventbridge.model.ListRuleNamesByTargetRequest;
import software.amazon.awssdk.services.eventbridge.model.ListRuleNamesByTargetResponse;
import software.amazon.awssdk.services.eventbridge.model.ListRulesRequest;
import software.amazon.awssdk.services.eventbridge.model.ListRulesResponse;
import software.amazon.awssdk.services.eventbridge.model.ListTagsForResourceRequest;
import software.amazon.awssdk.services.eventbridge.model.ListTagsForResourceResponse;
import software.amazon.awssdk.services.eventbridge.model.ListTargetsByRuleRequest;
import software.amazon.awssdk.services.eventbridge.model.ListTargetsByRuleResponse;
import software.amazon.awssdk.services.eventbridge.model.ManagedRuleException;
import software.amazon.awssdk.services.eventbridge.model.OperationDisabledException;
import software.amazon.awssdk.services.eventbridge.model.PolicyLengthExceededException;
import software.amazon.awssdk.services.eventbridge.model.PutEventsRequest;
import software.amazon.awssdk.services.eventbridge.model.PutEventsResponse;
import software.amazon.awssdk.services.eventbridge.model.PutPartnerEventsRequest;
import software.amazon.awssdk.services.eventbridge.model.PutPartnerEventsResponse;
import software.amazon.awssdk.services.eventbridge.model.PutPermissionRequest;
import software.amazon.awssdk.services.eventbridge.model.PutPermissionResponse;
import software.amazon.awssdk.services.eventbridge.model.PutRuleRequest;
import software.amazon.awssdk.services.eventbridge.model.PutRuleResponse;
import software.amazon.awssdk.services.eventbridge.model.PutTargetsRequest;
import software.amazon.awssdk.services.eventbridge.model.PutTargetsResponse;
import software.amazon.awssdk.services.eventbridge.model.RemovePermissionRequest;
import software.amazon.awssdk.services.eventbridge.model.RemovePermissionResponse;
import software.amazon.awssdk.services.eventbridge.model.RemoveTargetsRequest;
import software.amazon.awssdk.services.eventbridge.model.RemoveTargetsResponse;
import software.amazon.awssdk.services.eventbridge.model.ResourceAlreadyExistsException;
import software.amazon.awssdk.services.eventbridge.model.ResourceNotFoundException;
import software.amazon.awssdk.services.eventbridge.model.StartReplayRequest;
import software.amazon.awssdk.services.eventbridge.model.StartReplayResponse;
import software.amazon.awssdk.services.eventbridge.model.TagResourceRequest;
import software.amazon.awssdk.services.eventbridge.model.TagResourceResponse;
import software.amazon.awssdk.services.eventbridge.model.TestEventPatternRequest;
import software.amazon.awssdk.services.eventbridge.model.TestEventPatternResponse;
import software.amazon.awssdk.services.eventbridge.model.UntagResourceRequest;
import software.amazon.awssdk.services.eventbridge.model.UntagResourceResponse;
import software.amazon.awssdk.services.eventbridge.model.UpdateArchiveRequest;
import software.amazon.awssdk.services.eventbridge.model.UpdateArchiveResponse;
import software.amazon.awssdk.services.eventbridge.transform.ActivateEventSourceRequestMarshaller;
import software.amazon.awssdk.services.eventbridge.transform.CancelReplayRequestMarshaller;
import software.amazon.awssdk.services.eventbridge.transform.CreateArchiveRequestMarshaller;
import software.amazon.awssdk.services.eventbridge.transform.CreateEventBusRequestMarshaller;
import software.amazon.awssdk.services.eventbridge.transform.CreatePartnerEventSourceRequestMarshaller;
import software.amazon.awssdk.services.eventbridge.transform.DeactivateEventSourceRequestMarshaller;
import software.amazon.awssdk.services.eventbridge.transform.DeleteArchiveRequestMarshaller;
import software.amazon.awssdk.services.eventbridge.transform.DeleteEventBusRequestMarshaller;
import software.amazon.awssdk.services.eventbridge.transform.DeletePartnerEventSourceRequestMarshaller;
import software.amazon.awssdk.services.eventbridge.transform.DeleteRuleRequestMarshaller;
import software.amazon.awssdk.services.eventbridge.transform.DescribeArchiveRequestMarshaller;
import software.amazon.awssdk.services.eventbridge.transform.DescribeEventBusRequestMarshaller;
import software.amazon.awssdk.services.eventbridge.transform.DescribeEventSourceRequestMarshaller;
import software.amazon.awssdk.services.eventbridge.transform.DescribePartnerEventSourceRequestMarshaller;
import software.amazon.awssdk.services.eventbridge.transform.DescribeReplayRequestMarshaller;
import software.amazon.awssdk.services.eventbridge.transform.DescribeRuleRequestMarshaller;
import software.amazon.awssdk.services.eventbridge.transform.DisableRuleRequestMarshaller;
import software.amazon.awssdk.services.eventbridge.transform.EnableRuleRequestMarshaller;
import software.amazon.awssdk.services.eventbridge.transform.ListArchivesRequestMarshaller;
import software.amazon.awssdk.services.eventbridge.transform.ListEventBusesRequestMarshaller;
import software.amazon.awssdk.services.eventbridge.transform.ListEventSourcesRequestMarshaller;
import software.amazon.awssdk.services.eventbridge.transform.ListPartnerEventSourceAccountsRequestMarshaller;
import software.amazon.awssdk.services.eventbridge.transform.ListPartnerEventSourcesRequestMarshaller;
import software.amazon.awssdk.services.eventbridge.transform.ListReplaysRequestMarshaller;
import software.amazon.awssdk.services.eventbridge.transform.ListRuleNamesByTargetRequestMarshaller;
import software.amazon.awssdk.services.eventbridge.transform.ListRulesRequestMarshaller;
import software.amazon.awssdk.services.eventbridge.transform.ListTagsForResourceRequestMarshaller;
import software.amazon.awssdk.services.eventbridge.transform.ListTargetsByRuleRequestMarshaller;
import software.amazon.awssdk.services.eventbridge.transform.PutEventsRequestMarshaller;
import software.amazon.awssdk.services.eventbridge.transform.PutPartnerEventsRequestMarshaller;
import software.amazon.awssdk.services.eventbridge.transform.PutPermissionRequestMarshaller;
import software.amazon.awssdk.services.eventbridge.transform.PutRuleRequestMarshaller;
import software.amazon.awssdk.services.eventbridge.transform.PutTargetsRequestMarshaller;
import software.amazon.awssdk.services.eventbridge.transform.RemovePermissionRequestMarshaller;
import software.amazon.awssdk.services.eventbridge.transform.RemoveTargetsRequestMarshaller;
import software.amazon.awssdk.services.eventbridge.transform.StartReplayRequestMarshaller;
import software.amazon.awssdk.services.eventbridge.transform.TagResourceRequestMarshaller;
import software.amazon.awssdk.services.eventbridge.transform.TestEventPatternRequestMarshaller;
import software.amazon.awssdk.services.eventbridge.transform.UntagResourceRequestMarshaller;
import software.amazon.awssdk.services.eventbridge.transform.UpdateArchiveRequestMarshaller;
import software.amazon.awssdk.utils.CompletableFutureUtils;
/**
* Internal implementation of {@link EventBridgeAsyncClient}.
*
* @see EventBridgeAsyncClient#builder()
*/
@Generated("software.amazon.awssdk:codegen")
@SdkInternalApi
final class DefaultEventBridgeAsyncClient implements EventBridgeAsyncClient {
private static final Logger log = LoggerFactory.getLogger(DefaultEventBridgeAsyncClient.class);
private final AsyncClientHandler clientHandler;
private final AwsJsonProtocolFactory protocolFactory;
private final SdkClientConfiguration clientConfiguration;
protected DefaultEventBridgeAsyncClient(SdkClientConfiguration clientConfiguration) {
this.clientHandler = new AwsAsyncClientHandler(clientConfiguration);
this.clientConfiguration = clientConfiguration;
this.protocolFactory = init(AwsJsonProtocolFactory.builder()).build();
}
@Override
public final String serviceName() {
return SERVICE_NAME;
}
/**
*
* Activates a partner event source that has been deactivated. Once activated, your matching event bus will start
* receiving events from the event source.
*
*
* @param activateEventSourceRequest
* @return A Java Future containing the result of the ActivateEventSource operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ResourceNotFoundException An entity that you specified does not exist.
* - ConcurrentModificationException There is concurrent modification on a rule, target, archive, or
* replay.
* - InvalidStateException The specified state is not a valid state for an event source.
* - InternalException This exception occurs due to unexpected causes.
* - OperationDisabledException The operation you are attempting is not available in this region.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EventBridgeException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample EventBridgeAsyncClient.ActivateEventSource
* @see AWS API Documentation
*/
@Override
public CompletableFuture activateEventSource(
ActivateEventSourceRequest activateEventSourceRequest) {
List metricPublishers = resolveMetricPublishers(clientConfiguration, activateEventSourceRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "EventBridge");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "ActivateEventSource");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, ActivateEventSourceResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("ActivateEventSource")
.withMarshaller(new ActivateEventSourceRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withMetricCollector(apiCallMetricCollector).withInput(activateEventSourceRequest));
AwsRequestOverrideConfiguration requestOverrideConfig = activateEventSourceRequest.overrideConfiguration().orElse(
null);
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Cancels the specified replay.
*
*
* @param cancelReplayRequest
* @return A Java Future containing the result of the CancelReplay operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ResourceNotFoundException An entity that you specified does not exist.
* - ConcurrentModificationException There is concurrent modification on a rule, target, archive, or
* replay.
* - IllegalStatusException An error occurred because a replay can be canceled only when the state is
* Running or Starting.
* - InternalException This exception occurs due to unexpected causes.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EventBridgeException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample EventBridgeAsyncClient.CancelReplay
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture cancelReplay(CancelReplayRequest cancelReplayRequest) {
List metricPublishers = resolveMetricPublishers(clientConfiguration, cancelReplayRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "EventBridge");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "CancelReplay");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
CancelReplayResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("CancelReplay").withMarshaller(new CancelReplayRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withMetricCollector(apiCallMetricCollector).withInput(cancelReplayRequest));
AwsRequestOverrideConfiguration requestOverrideConfig = cancelReplayRequest.overrideConfiguration().orElse(null);
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Creates an archive of events with the specified settings. When you create an archive, incoming events might not
* immediately start being sent to the archive. Allow a short period of time for changes to take effect. If you do
* not specify a pattern to filter events sent to the archive, all events are sent to the archive except replayed
* events. Replayed events are not sent to an archive.
*
*
* @param createArchiveRequest
* @return A Java Future containing the result of the CreateArchive operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ConcurrentModificationException There is concurrent modification on a rule, target, archive, or
* replay.
* - ResourceAlreadyExistsException The resource you are trying to create already exists.
* - ResourceNotFoundException An entity that you specified does not exist.
* - InternalException This exception occurs due to unexpected causes.
* - LimitExceededException The request failed because it attempted to create resource beyond the allowed
* service quota.
* - InvalidEventPatternException The event pattern is not valid.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EventBridgeException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample EventBridgeAsyncClient.CreateArchive
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture createArchive(CreateArchiveRequest createArchiveRequest) {
List metricPublishers = resolveMetricPublishers(clientConfiguration, createArchiveRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "EventBridge");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "CreateArchive");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
CreateArchiveResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("CreateArchive")
.withMarshaller(new CreateArchiveRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withMetricCollector(apiCallMetricCollector).withInput(createArchiveRequest));
AwsRequestOverrideConfiguration requestOverrideConfig = createArchiveRequest.overrideConfiguration().orElse(null);
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Creates a new event bus within your account. This can be a custom event bus which you can use to receive events
* from your custom applications and services, or it can be a partner event bus which can be matched to a partner
* event source.
*
*
* @param createEventBusRequest
* @return A Java Future containing the result of the CreateEventBus operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ResourceAlreadyExistsException The resource you are trying to create already exists.
* - ResourceNotFoundException An entity that you specified does not exist.
* - InvalidStateException The specified state is not a valid state for an event source.
* - InternalException This exception occurs due to unexpected causes.
* - ConcurrentModificationException There is concurrent modification on a rule, target, archive, or
* replay.
* - LimitExceededException The request failed because it attempted to create resource beyond the allowed
* service quota.
* - OperationDisabledException The operation you are attempting is not available in this region.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EventBridgeException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample EventBridgeAsyncClient.CreateEventBus
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture createEventBus(CreateEventBusRequest createEventBusRequest) {
List metricPublishers = resolveMetricPublishers(clientConfiguration, createEventBusRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "EventBridge");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "CreateEventBus");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, CreateEventBusResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("CreateEventBus")
.withMarshaller(new CreateEventBusRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withMetricCollector(apiCallMetricCollector).withInput(createEventBusRequest));
AwsRequestOverrideConfiguration requestOverrideConfig = createEventBusRequest.overrideConfiguration().orElse(null);
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Called by an SaaS partner to create a partner event source. This operation is not used by AWS customers.
*
*
* Each partner event source can be used by one AWS account to create a matching partner event bus in that AWS
* account. A SaaS partner must create one partner event source for each AWS account that wants to receive those
* event types.
*
*
* A partner event source creates events based on resources within the SaaS partner's service or application.
*
*
* An AWS account that creates a partner event bus that matches the partner event source can use that event bus to
* receive events from the partner, and then process them using AWS Events rules and targets.
*
*
* Partner event source names follow this format:
*
*
* partner_name/event_namespace/event_name
*
*
* partner_name is determined during partner registration and identifies the partner to AWS customers.
* event_namespace is determined by the partner and is a way for the partner to categorize their events.
* event_name is determined by the partner, and should uniquely identify an event-generating resource within
* the partner system. The combination of event_namespace and event_name should help AWS customers
* decide whether to create an event bus to receive these events.
*
*
* @param createPartnerEventSourceRequest
* @return A Java Future containing the result of the CreatePartnerEventSource operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ResourceAlreadyExistsException The resource you are trying to create already exists.
* - InternalException This exception occurs due to unexpected causes.
* - ConcurrentModificationException There is concurrent modification on a rule, target, archive, or
* replay.
* - LimitExceededException The request failed because it attempted to create resource beyond the allowed
* service quota.
* - OperationDisabledException The operation you are attempting is not available in this region.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EventBridgeException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample EventBridgeAsyncClient.CreatePartnerEventSource
* @see AWS API Documentation
*/
@Override
public CompletableFuture createPartnerEventSource(
CreatePartnerEventSourceRequest createPartnerEventSourceRequest) {
List metricPublishers = resolveMetricPublishers(clientConfiguration, createPartnerEventSourceRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "EventBridge");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "CreatePartnerEventSource");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, CreatePartnerEventSourceResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("CreatePartnerEventSource")
.withMarshaller(new CreatePartnerEventSourceRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withMetricCollector(apiCallMetricCollector).withInput(createPartnerEventSourceRequest));
AwsRequestOverrideConfiguration requestOverrideConfig = createPartnerEventSourceRequest.overrideConfiguration()
.orElse(null);
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* You can use this operation to temporarily stop receiving events from the specified partner event source. The
* matching event bus is not deleted.
*
*
* When you deactivate a partner event source, the source goes into PENDING state. If it remains in PENDING state
* for more than two weeks, it is deleted.
*
*
* To activate a deactivated partner event source, use ActivateEventSource.
*
*
* @param deactivateEventSourceRequest
* @return A Java Future containing the result of the DeactivateEventSource operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ResourceNotFoundException An entity that you specified does not exist.
* - ConcurrentModificationException There is concurrent modification on a rule, target, archive, or
* replay.
* - InvalidStateException The specified state is not a valid state for an event source.
* - InternalException This exception occurs due to unexpected causes.
* - OperationDisabledException The operation you are attempting is not available in this region.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EventBridgeException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample EventBridgeAsyncClient.DeactivateEventSource
* @see AWS API Documentation
*/
@Override
public CompletableFuture deactivateEventSource(
DeactivateEventSourceRequest deactivateEventSourceRequest) {
List metricPublishers = resolveMetricPublishers(clientConfiguration, deactivateEventSourceRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "EventBridge");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DeactivateEventSource");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, DeactivateEventSourceResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DeactivateEventSource")
.withMarshaller(new DeactivateEventSourceRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withMetricCollector(apiCallMetricCollector).withInput(deactivateEventSourceRequest));
AwsRequestOverrideConfiguration requestOverrideConfig = deactivateEventSourceRequest.overrideConfiguration().orElse(
null);
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Deletes the specified archive.
*
*
* @param deleteArchiveRequest
* @return A Java Future containing the result of the DeleteArchive operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ConcurrentModificationException There is concurrent modification on a rule, target, archive, or
* replay.
* - ResourceNotFoundException An entity that you specified does not exist.
* - InternalException This exception occurs due to unexpected causes.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EventBridgeException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample EventBridgeAsyncClient.DeleteArchive
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture deleteArchive(DeleteArchiveRequest deleteArchiveRequest) {
List metricPublishers = resolveMetricPublishers(clientConfiguration, deleteArchiveRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "EventBridge");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DeleteArchive");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
DeleteArchiveResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DeleteArchive")
.withMarshaller(new DeleteArchiveRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withMetricCollector(apiCallMetricCollector).withInput(deleteArchiveRequest));
AwsRequestOverrideConfiguration requestOverrideConfig = deleteArchiveRequest.overrideConfiguration().orElse(null);
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Deletes the specified custom event bus or partner event bus. All rules associated with this event bus need to be
* deleted. You can't delete your account's default event bus.
*
*
* @param deleteEventBusRequest
* @return A Java Future containing the result of the DeleteEventBus operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InternalException This exception occurs due to unexpected causes.
* - ConcurrentModificationException There is concurrent modification on a rule, target, archive, or
* replay.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EventBridgeException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample EventBridgeAsyncClient.DeleteEventBus
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture deleteEventBus(DeleteEventBusRequest deleteEventBusRequest) {
List metricPublishers = resolveMetricPublishers(clientConfiguration, deleteEventBusRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "EventBridge");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DeleteEventBus");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, DeleteEventBusResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DeleteEventBus")
.withMarshaller(new DeleteEventBusRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withMetricCollector(apiCallMetricCollector).withInput(deleteEventBusRequest));
AwsRequestOverrideConfiguration requestOverrideConfig = deleteEventBusRequest.overrideConfiguration().orElse(null);
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* This operation is used by SaaS partners to delete a partner event source. This operation is not used by AWS
* customers.
*
*
* When you delete an event source, the status of the corresponding partner event bus in the AWS customer account
* becomes DELETED.
*
*
*
* @param deletePartnerEventSourceRequest
* @return A Java Future containing the result of the DeletePartnerEventSource operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InternalException This exception occurs due to unexpected causes.
-
* ConcurrentModificationException There is concurrent modification on a rule, target, archive, or replay.
*
- OperationDisabledException The operation you are attempting is not available in this region.
*
- SdkException Base class for all exceptions that can be thrown by the SDK (both service and
* client). Can be used for catch all scenarios.
- SdkClientException If any client side error occurs
* such as an IO related failure, failure to get credentials, etc.
- EventBridgeException Base class
* for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
*
* @sample EventBridgeAsyncClient.DeletePartnerEventSource
* @see AWS API Documentation
*/
@Override
public CompletableFuture deletePartnerEventSource(
DeletePartnerEventSourceRequest deletePartnerEventSourceRequest) {
List metricPublishers = resolveMetricPublishers(clientConfiguration, deletePartnerEventSourceRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "EventBridge");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DeletePartnerEventSource");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, DeletePartnerEventSourceResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DeletePartnerEventSource")
.withMarshaller(new DeletePartnerEventSourceRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withMetricCollector(apiCallMetricCollector).withInput(deletePartnerEventSourceRequest));
AwsRequestOverrideConfiguration requestOverrideConfig = deletePartnerEventSourceRequest.overrideConfiguration()
.orElse(null);
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Deletes the specified rule.
*
*
* Before you can delete the rule, you must remove all targets, using RemoveTargets.
*
*
* When you delete a rule, incoming events might continue to match to the deleted rule. Allow a short period of time
* for changes to take effect.
*
*
* Managed rules are rules created and managed by another AWS service on your behalf. These rules are created by
* those other AWS services to support functionality in those services. You can delete these rules using the
* Force
option, but you should do so only if you are sure the other service is not still using that
* rule.
*
*
* @param deleteRuleRequest
* @return A Java Future containing the result of the DeleteRule operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ConcurrentModificationException There is concurrent modification on a rule, target, archive, or
* replay.
* - ManagedRuleException This rule was created by an AWS service on behalf of your account. It is managed
* by that service. If you see this error in response to
DeleteRule
or
* RemoveTargets
, you can use the Force
parameter in those calls to delete the
* rule or remove targets from the rule. You cannot modify these managed rules by using
* DisableRule
, EnableRule
, PutTargets
, PutRule
,
* TagResource
, or UntagResource
.
* - InternalException This exception occurs due to unexpected causes.
* - ResourceNotFoundException An entity that you specified does not exist.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EventBridgeException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample EventBridgeAsyncClient.DeleteRule
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture deleteRule(DeleteRuleRequest deleteRuleRequest) {
List metricPublishers = resolveMetricPublishers(clientConfiguration, deleteRuleRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "EventBridge");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DeleteRule");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
DeleteRuleResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams().withOperationName("DeleteRule")
.withMarshaller(new DeleteRuleRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withMetricCollector(apiCallMetricCollector).withInput(deleteRuleRequest));
AwsRequestOverrideConfiguration requestOverrideConfig = deleteRuleRequest.overrideConfiguration().orElse(null);
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Retrieves details about an archive.
*
*
* @param describeArchiveRequest
* @return A Java Future containing the result of the DescribeArchive operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ResourceAlreadyExistsException The resource you are trying to create already exists.
* - ResourceNotFoundException An entity that you specified does not exist.
* - InternalException This exception occurs due to unexpected causes.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EventBridgeException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample EventBridgeAsyncClient.DescribeArchive
* @see AWS
* API Documentation
*/
@Override
public CompletableFuture describeArchive(DescribeArchiveRequest describeArchiveRequest) {
List metricPublishers = resolveMetricPublishers(clientConfiguration, describeArchiveRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "EventBridge");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DescribeArchive");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, DescribeArchiveResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DescribeArchive")
.withMarshaller(new DescribeArchiveRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withMetricCollector(apiCallMetricCollector).withInput(describeArchiveRequest));
AwsRequestOverrideConfiguration requestOverrideConfig = describeArchiveRequest.overrideConfiguration().orElse(null);
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Displays details about an event bus in your account. This can include the external AWS accounts that are
* permitted to write events to your default event bus, and the associated policy. For custom event buses and
* partner event buses, it displays the name, ARN, policy, state, and creation time.
*
*
* To enable your account to receive events from other accounts on its default event bus, use PutPermission.
*
*
* For more information about partner event buses, see CreateEventBus.
*
*
* @param describeEventBusRequest
* @return A Java Future containing the result of the DescribeEventBus operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ResourceNotFoundException An entity that you specified does not exist.
* - InternalException This exception occurs due to unexpected causes.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EventBridgeException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample EventBridgeAsyncClient.DescribeEventBus
* @see AWS
* API Documentation
*/
@Override
public CompletableFuture describeEventBus(DescribeEventBusRequest describeEventBusRequest) {
List metricPublishers = resolveMetricPublishers(clientConfiguration, describeEventBusRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "EventBridge");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DescribeEventBus");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, DescribeEventBusResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DescribeEventBus")
.withMarshaller(new DescribeEventBusRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withMetricCollector(apiCallMetricCollector).withInput(describeEventBusRequest));
AwsRequestOverrideConfiguration requestOverrideConfig = describeEventBusRequest.overrideConfiguration().orElse(null);
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* This operation lists details about a partner event source that is shared with your account.
*
*
* @param describeEventSourceRequest
* @return A Java Future containing the result of the DescribeEventSource operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ResourceNotFoundException An entity that you specified does not exist.
* - InternalException This exception occurs due to unexpected causes.
* - OperationDisabledException The operation you are attempting is not available in this region.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EventBridgeException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample EventBridgeAsyncClient.DescribeEventSource
* @see AWS API Documentation
*/
@Override
public CompletableFuture describeEventSource(
DescribeEventSourceRequest describeEventSourceRequest) {
List metricPublishers = resolveMetricPublishers(clientConfiguration, describeEventSourceRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "EventBridge");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DescribeEventSource");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, DescribeEventSourceResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DescribeEventSource")
.withMarshaller(new DescribeEventSourceRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withMetricCollector(apiCallMetricCollector).withInput(describeEventSourceRequest));
AwsRequestOverrideConfiguration requestOverrideConfig = describeEventSourceRequest.overrideConfiguration().orElse(
null);
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* An SaaS partner can use this operation to list details about a partner event source that they have created. AWS
* customers do not use this operation. Instead, AWS customers can use DescribeEventSource to see details
* about a partner event source that is shared with them.
*
*
* @param describePartnerEventSourceRequest
* @return A Java Future containing the result of the DescribePartnerEventSource operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ResourceNotFoundException An entity that you specified does not exist.
* - InternalException This exception occurs due to unexpected causes.
* - OperationDisabledException The operation you are attempting is not available in this region.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EventBridgeException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample EventBridgeAsyncClient.DescribePartnerEventSource
* @see AWS API Documentation
*/
@Override
public CompletableFuture describePartnerEventSource(
DescribePartnerEventSourceRequest describePartnerEventSourceRequest) {
List metricPublishers = resolveMetricPublishers(clientConfiguration, describePartnerEventSourceRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "EventBridge");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DescribePartnerEventSource");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, DescribePartnerEventSourceResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DescribePartnerEventSource")
.withMarshaller(new DescribePartnerEventSourceRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withMetricCollector(apiCallMetricCollector).withInput(describePartnerEventSourceRequest));
AwsRequestOverrideConfiguration requestOverrideConfig = describePartnerEventSourceRequest.overrideConfiguration()
.orElse(null);
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Retrieves details about a replay. Use DescribeReplay
to determine the progress of a running replay.
* A replay processes events to replay based on the time in the event, and replays them using 1 minute intervals. If
* you use StartReplay
and specify an EventStartTime
and an EventEndTime
that
* covers a 20 minute time range, the events are replayed from the first minute of that 20 minute range first. Then
* the events from the second minute are replayed. You can use DescribeReplay
to determine the progress
* of a replay. The value returned for EventLastReplayedTime
indicates the time within the specified
* time range associated with the last event replayed.
*
*
* @param describeReplayRequest
* @return A Java Future containing the result of the DescribeReplay operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ResourceNotFoundException An entity that you specified does not exist.
* - InternalException This exception occurs due to unexpected causes.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EventBridgeException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample EventBridgeAsyncClient.DescribeReplay
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture describeReplay(DescribeReplayRequest describeReplayRequest) {
List metricPublishers = resolveMetricPublishers(clientConfiguration, describeReplayRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "EventBridge");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DescribeReplay");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, DescribeReplayResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DescribeReplay")
.withMarshaller(new DescribeReplayRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withMetricCollector(apiCallMetricCollector).withInput(describeReplayRequest));
AwsRequestOverrideConfiguration requestOverrideConfig = describeReplayRequest.overrideConfiguration().orElse(null);
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Describes the specified rule.
*
*
* DescribeRule does not list the targets of a rule. To see the targets associated with a rule, use
* ListTargetsByRule.
*
*
* @param describeRuleRequest
* @return A Java Future containing the result of the DescribeRule operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ResourceNotFoundException An entity that you specified does not exist.
* - InternalException This exception occurs due to unexpected causes.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EventBridgeException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample EventBridgeAsyncClient.DescribeRule
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture describeRule(DescribeRuleRequest describeRuleRequest) {
List metricPublishers = resolveMetricPublishers(clientConfiguration, describeRuleRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "EventBridge");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DescribeRule");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
DescribeRuleResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DescribeRule").withMarshaller(new DescribeRuleRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withMetricCollector(apiCallMetricCollector).withInput(describeRuleRequest));
AwsRequestOverrideConfiguration requestOverrideConfig = describeRuleRequest.overrideConfiguration().orElse(null);
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Disables the specified rule. A disabled rule won't match any events, and won't self-trigger if it has a schedule
* expression.
*
*
* When you disable a rule, incoming events might continue to match to the disabled rule. Allow a short period of
* time for changes to take effect.
*
*
* @param disableRuleRequest
* @return A Java Future containing the result of the DisableRule operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ResourceNotFoundException An entity that you specified does not exist.
* - ConcurrentModificationException There is concurrent modification on a rule, target, archive, or
* replay.
* - ManagedRuleException This rule was created by an AWS service on behalf of your account. It is managed
* by that service. If you see this error in response to
DeleteRule
or
* RemoveTargets
, you can use the Force
parameter in those calls to delete the
* rule or remove targets from the rule. You cannot modify these managed rules by using
* DisableRule
, EnableRule
, PutTargets
, PutRule
,
* TagResource
, or UntagResource
.
* - InternalException This exception occurs due to unexpected causes.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EventBridgeException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample EventBridgeAsyncClient.DisableRule
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture disableRule(DisableRuleRequest disableRuleRequest) {
List metricPublishers = resolveMetricPublishers(clientConfiguration, disableRuleRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "EventBridge");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DisableRule");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
DisableRuleResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DisableRule").withMarshaller(new DisableRuleRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withMetricCollector(apiCallMetricCollector).withInput(disableRuleRequest));
AwsRequestOverrideConfiguration requestOverrideConfig = disableRuleRequest.overrideConfiguration().orElse(null);
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Enables the specified rule. If the rule does not exist, the operation fails.
*
*
* When you enable a rule, incoming events might not immediately start matching to a newly enabled rule. Allow a
* short period of time for changes to take effect.
*
*
* @param enableRuleRequest
* @return A Java Future containing the result of the EnableRule operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ResourceNotFoundException An entity that you specified does not exist.
* - ConcurrentModificationException There is concurrent modification on a rule, target, archive, or
* replay.
* - ManagedRuleException This rule was created by an AWS service on behalf of your account. It is managed
* by that service. If you see this error in response to
DeleteRule
or
* RemoveTargets
, you can use the Force
parameter in those calls to delete the
* rule or remove targets from the rule. You cannot modify these managed rules by using
* DisableRule
, EnableRule
, PutTargets
, PutRule
,
* TagResource
, or UntagResource
.
* - InternalException This exception occurs due to unexpected causes.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EventBridgeException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample EventBridgeAsyncClient.EnableRule
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture enableRule(EnableRuleRequest enableRuleRequest) {
List metricPublishers = resolveMetricPublishers(clientConfiguration, enableRuleRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "EventBridge");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "EnableRule");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
EnableRuleResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams().withOperationName("EnableRule")
.withMarshaller(new EnableRuleRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withMetricCollector(apiCallMetricCollector).withInput(enableRuleRequest));
AwsRequestOverrideConfiguration requestOverrideConfig = enableRuleRequest.overrideConfiguration().orElse(null);
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Lists your archives. You can either list all the archives or you can provide a prefix to match to the archive
* names. Filter parameters are exclusive.
*
*
* @param listArchivesRequest
* @return A Java Future containing the result of the ListArchives operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ResourceNotFoundException An entity that you specified does not exist.
* - InternalException This exception occurs due to unexpected causes.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EventBridgeException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample EventBridgeAsyncClient.ListArchives
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture listArchives(ListArchivesRequest listArchivesRequest) {
List metricPublishers = resolveMetricPublishers(clientConfiguration, listArchivesRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "EventBridge");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "ListArchives");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
ListArchivesResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("ListArchives").withMarshaller(new ListArchivesRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withMetricCollector(apiCallMetricCollector).withInput(listArchivesRequest));
AwsRequestOverrideConfiguration requestOverrideConfig = listArchivesRequest.overrideConfiguration().orElse(null);
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Lists all the event buses in your account, including the default event bus, custom event buses, and partner event
* buses.
*
*
* @param listEventBusesRequest
* @return A Java Future containing the result of the ListEventBuses operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InternalException This exception occurs due to unexpected causes.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EventBridgeException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample EventBridgeAsyncClient.ListEventBuses
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture listEventBuses(ListEventBusesRequest listEventBusesRequest) {
List metricPublishers = resolveMetricPublishers(clientConfiguration, listEventBusesRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "EventBridge");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "ListEventBuses");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, ListEventBusesResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("ListEventBuses")
.withMarshaller(new ListEventBusesRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withMetricCollector(apiCallMetricCollector).withInput(listEventBusesRequest));
AwsRequestOverrideConfiguration requestOverrideConfig = listEventBusesRequest.overrideConfiguration().orElse(null);
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* You can use this to see all the partner event sources that have been shared with your AWS account. For more
* information about partner event sources, see CreateEventBus.
*
*
* @param listEventSourcesRequest
* @return A Java Future containing the result of the ListEventSources operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InternalException This exception occurs due to unexpected causes.
* - OperationDisabledException The operation you are attempting is not available in this region.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EventBridgeException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample EventBridgeAsyncClient.ListEventSources
* @see AWS
* API Documentation
*/
@Override
public CompletableFuture listEventSources(ListEventSourcesRequest listEventSourcesRequest) {
List metricPublishers = resolveMetricPublishers(clientConfiguration, listEventSourcesRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "EventBridge");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "ListEventSources");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, ListEventSourcesResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("ListEventSources")
.withMarshaller(new ListEventSourcesRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withMetricCollector(apiCallMetricCollector).withInput(listEventSourcesRequest));
AwsRequestOverrideConfiguration requestOverrideConfig = listEventSourcesRequest.overrideConfiguration().orElse(null);
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* An SaaS partner can use this operation to display the AWS account ID that a particular partner event source name
* is associated with. This operation is not used by AWS customers.
*
*
* @param listPartnerEventSourceAccountsRequest
* @return A Java Future containing the result of the ListPartnerEventSourceAccounts operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ResourceNotFoundException An entity that you specified does not exist.
* - InternalException This exception occurs due to unexpected causes.
* - OperationDisabledException The operation you are attempting is not available in this region.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EventBridgeException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample EventBridgeAsyncClient.ListPartnerEventSourceAccounts
* @see AWS API Documentation
*/
@Override
public CompletableFuture listPartnerEventSourceAccounts(
ListPartnerEventSourceAccountsRequest listPartnerEventSourceAccountsRequest) {
List metricPublishers = resolveMetricPublishers(clientConfiguration,
listPartnerEventSourceAccountsRequest.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "EventBridge");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "ListPartnerEventSourceAccounts");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, ListPartnerEventSourceAccountsResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("ListPartnerEventSourceAccounts")
.withMarshaller(new ListPartnerEventSourceAccountsRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withMetricCollector(apiCallMetricCollector).withInput(listPartnerEventSourceAccountsRequest));
AwsRequestOverrideConfiguration requestOverrideConfig = listPartnerEventSourceAccountsRequest.overrideConfiguration()
.orElse(null);
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* An SaaS partner can use this operation to list all the partner event source names that they have created. This
* operation is not used by AWS customers.
*
*
* @param listPartnerEventSourcesRequest
* @return A Java Future containing the result of the ListPartnerEventSources operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InternalException This exception occurs due to unexpected causes.
* - OperationDisabledException The operation you are attempting is not available in this region.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EventBridgeException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample EventBridgeAsyncClient.ListPartnerEventSources
* @see AWS API Documentation
*/
@Override
public CompletableFuture listPartnerEventSources(
ListPartnerEventSourcesRequest listPartnerEventSourcesRequest) {
List metricPublishers = resolveMetricPublishers(clientConfiguration, listPartnerEventSourcesRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "EventBridge");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "ListPartnerEventSources");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, ListPartnerEventSourcesResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("ListPartnerEventSources")
.withMarshaller(new ListPartnerEventSourcesRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withMetricCollector(apiCallMetricCollector).withInput(listPartnerEventSourcesRequest));
AwsRequestOverrideConfiguration requestOverrideConfig = listPartnerEventSourcesRequest.overrideConfiguration()
.orElse(null);
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Lists your replays. You can either list all the replays or you can provide a prefix to match to the replay names.
* Filter parameters are exclusive.
*
*
* @param listReplaysRequest
* @return A Java Future containing the result of the ListReplays operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InternalException This exception occurs due to unexpected causes.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EventBridgeException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample EventBridgeAsyncClient.ListReplays
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture listReplays(ListReplaysRequest listReplaysRequest) {
List metricPublishers = resolveMetricPublishers(clientConfiguration, listReplaysRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "EventBridge");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "ListReplays");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
ListReplaysResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("ListReplays").withMarshaller(new ListReplaysRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withMetricCollector(apiCallMetricCollector).withInput(listReplaysRequest));
AwsRequestOverrideConfiguration requestOverrideConfig = listReplaysRequest.overrideConfiguration().orElse(null);
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Lists the rules for the specified target. You can see which of the rules in Amazon EventBridge can invoke a
* specific target in your account.
*
*
* @param listRuleNamesByTargetRequest
* @return A Java Future containing the result of the ListRuleNamesByTarget operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InternalException This exception occurs due to unexpected causes.
* - ResourceNotFoundException An entity that you specified does not exist.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EventBridgeException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample EventBridgeAsyncClient.ListRuleNamesByTarget
* @see AWS API Documentation
*/
@Override
public CompletableFuture listRuleNamesByTarget(
ListRuleNamesByTargetRequest listRuleNamesByTargetRequest) {
List metricPublishers = resolveMetricPublishers(clientConfiguration, listRuleNamesByTargetRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "EventBridge");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "ListRuleNamesByTarget");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, ListRuleNamesByTargetResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("ListRuleNamesByTarget")
.withMarshaller(new ListRuleNamesByTargetRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withMetricCollector(apiCallMetricCollector).withInput(listRuleNamesByTargetRequest));
AwsRequestOverrideConfiguration requestOverrideConfig = listRuleNamesByTargetRequest.overrideConfiguration().orElse(
null);
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Lists your Amazon EventBridge rules. You can either list all the rules or you can provide a prefix to match to
* the rule names.
*
*
* ListRules does not list the targets of a rule. To see the targets associated with a rule, use
* ListTargetsByRule.
*
*
* @param listRulesRequest
* @return A Java Future containing the result of the ListRules operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InternalException This exception occurs due to unexpected causes.
* - ResourceNotFoundException An entity that you specified does not exist.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EventBridgeException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample EventBridgeAsyncClient.ListRules
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture listRules(ListRulesRequest listRulesRequest) {
List metricPublishers = resolveMetricPublishers(clientConfiguration, listRulesRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "EventBridge");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "ListRules");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
ListRulesResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams().withOperationName("ListRules")
.withMarshaller(new ListRulesRequestMarshaller(protocolFactory)).withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withMetricCollector(apiCallMetricCollector)
.withInput(listRulesRequest));
AwsRequestOverrideConfiguration requestOverrideConfig = listRulesRequest.overrideConfiguration().orElse(null);
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Displays the tags associated with an EventBridge resource. In EventBridge, rules and event buses can be tagged.
*
*
* @param listTagsForResourceRequest
* @return A Java Future containing the result of the ListTagsForResource operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ResourceNotFoundException An entity that you specified does not exist.
* - InternalException This exception occurs due to unexpected causes.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EventBridgeException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample EventBridgeAsyncClient.ListTagsForResource
* @see AWS API Documentation
*/
@Override
public CompletableFuture listTagsForResource(
ListTagsForResourceRequest listTagsForResourceRequest) {
List metricPublishers = resolveMetricPublishers(clientConfiguration, listTagsForResourceRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "EventBridge");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "ListTagsForResource");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, ListTagsForResourceResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("ListTagsForResource")
.withMarshaller(new ListTagsForResourceRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withMetricCollector(apiCallMetricCollector).withInput(listTagsForResourceRequest));
AwsRequestOverrideConfiguration requestOverrideConfig = listTagsForResourceRequest.overrideConfiguration().orElse(
null);
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Lists the targets assigned to the specified rule.
*
*
* @param listTargetsByRuleRequest
* @return A Java Future containing the result of the ListTargetsByRule operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ResourceNotFoundException An entity that you specified does not exist.
* - InternalException This exception occurs due to unexpected causes.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EventBridgeException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample EventBridgeAsyncClient.ListTargetsByRule
* @see AWS
* API Documentation
*/
@Override
public CompletableFuture listTargetsByRule(ListTargetsByRuleRequest listTargetsByRuleRequest) {
List metricPublishers = resolveMetricPublishers(clientConfiguration, listTargetsByRuleRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "EventBridge");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "ListTargetsByRule");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, ListTargetsByRuleResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("ListTargetsByRule")
.withMarshaller(new ListTargetsByRuleRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withMetricCollector(apiCallMetricCollector).withInput(listTargetsByRuleRequest));
AwsRequestOverrideConfiguration requestOverrideConfig = listTargetsByRuleRequest.overrideConfiguration().orElse(null);
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Sends custom events to Amazon EventBridge so that they can be matched to rules.
*
*
* @param putEventsRequest
* @return A Java Future containing the result of the PutEvents operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InternalException This exception occurs due to unexpected causes.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EventBridgeException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample EventBridgeAsyncClient.PutEvents
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture putEvents(PutEventsRequest putEventsRequest) {
List metricPublishers = resolveMetricPublishers(clientConfiguration, putEventsRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "EventBridge");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "PutEvents");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
PutEventsResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams().withOperationName("PutEvents")
.withMarshaller(new PutEventsRequestMarshaller(protocolFactory)).withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withMetricCollector(apiCallMetricCollector)
.withInput(putEventsRequest));
AwsRequestOverrideConfiguration requestOverrideConfig = putEventsRequest.overrideConfiguration().orElse(null);
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* This is used by SaaS partners to write events to a customer's partner event bus. AWS customers do not use this
* operation.
*
*
* @param putPartnerEventsRequest
* @return A Java Future containing the result of the PutPartnerEvents operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InternalException This exception occurs due to unexpected causes.
* - OperationDisabledException The operation you are attempting is not available in this region.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EventBridgeException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample EventBridgeAsyncClient.PutPartnerEvents
* @see AWS
* API Documentation
*/
@Override
public CompletableFuture putPartnerEvents(PutPartnerEventsRequest putPartnerEventsRequest) {
List metricPublishers = resolveMetricPublishers(clientConfiguration, putPartnerEventsRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "EventBridge");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "PutPartnerEvents");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, PutPartnerEventsResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("PutPartnerEvents")
.withMarshaller(new PutPartnerEventsRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withMetricCollector(apiCallMetricCollector).withInput(putPartnerEventsRequest));
AwsRequestOverrideConfiguration requestOverrideConfig = putPartnerEventsRequest.overrideConfiguration().orElse(null);
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Running PutPermission
permits the specified AWS account or AWS organization to put events to the
* specified event bus. Amazon EventBridge (CloudWatch Events) rules in your account are triggered by these
* events arriving to an event bus in your account.
*
*
* For another account to send events to your account, that external account must have an EventBridge rule with your
* account's event bus as a target.
*
*
* To enable multiple AWS accounts to put events to your event bus, run PutPermission
once for each of
* these accounts. Or, if all the accounts are members of the same AWS organization, you can run
* PutPermission
once specifying Principal
as "*" and specifying the AWS organization ID
* in Condition
, to grant permissions to all accounts in that organization.
*
*
* If you grant permissions using an organization, then accounts in that organization must specify a
* RoleArn
with proper permissions when they use PutTarget
to add your account's event bus
* as a target. For more information, see Sending and Receiving Events Between AWS Accounts in the Amazon EventBridge User Guide.
*
*
* The permission policy on the default event bus cannot exceed 10 KB in size.
*
*
* @param putPermissionRequest
* @return A Java Future containing the result of the PutPermission operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ResourceNotFoundException An entity that you specified does not exist.
* - PolicyLengthExceededException The event bus policy is too long. For more information, see the limits.
*
* - InternalException This exception occurs due to unexpected causes.
* - ConcurrentModificationException There is concurrent modification on a rule, target, archive, or
* replay.
* - OperationDisabledException The operation you are attempting is not available in this region.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EventBridgeException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample EventBridgeAsyncClient.PutPermission
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture putPermission(PutPermissionRequest putPermissionRequest) {
List metricPublishers = resolveMetricPublishers(clientConfiguration, putPermissionRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "EventBridge");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "PutPermission");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
PutPermissionResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("PutPermission")
.withMarshaller(new PutPermissionRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withMetricCollector(apiCallMetricCollector).withInput(putPermissionRequest));
AwsRequestOverrideConfiguration requestOverrideConfig = putPermissionRequest.overrideConfiguration().orElse(null);
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Creates or updates the specified rule. Rules are enabled by default, or based on value of the state. You can
* disable a rule using DisableRule.
*
*
* A single rule watches for events from a single event bus. Events generated by AWS services go to your account's
* default event bus. Events generated by SaaS partner services or applications go to the matching partner event
* bus. If you have custom applications or services, you can specify whether their events go to your default event
* bus or a custom event bus that you have created. For more information, see CreateEventBus.
*
*
* If you are updating an existing rule, the rule is replaced with what you specify in this PutRule
* command. If you omit arguments in PutRule
, the old values for those arguments are not kept. Instead,
* they are replaced with null values.
*
*
* When you create or update a rule, incoming events might not immediately start matching to new or updated rules.
* Allow a short period of time for changes to take effect.
*
*
* A rule must contain at least an EventPattern or ScheduleExpression. Rules with EventPatterns are triggered when a
* matching event is observed. Rules with ScheduleExpressions self-trigger based on the given schedule. A rule can
* have both an EventPattern and a ScheduleExpression, in which case the rule triggers on matching events as well as
* on a schedule.
*
*
* When you initially create a rule, you can optionally assign one or more tags to the rule. Tags can help you
* organize and categorize your resources. You can also use them to scope user permissions, by granting a user
* permission to access or change only rules with certain tag values. To use the PutRule
operation and
* assign tags, you must have both the events:PutRule
and events:TagResource
permissions.
*
*
* If you are updating an existing rule, any tags you specify in the PutRule
operation are ignored. To
* update the tags of an existing rule, use TagResource and UntagResource.
*
*
* Most services in AWS treat : or / as the same character in Amazon Resource Names (ARNs). However, EventBridge
* uses an exact match in event patterns and rules. Be sure to use the correct ARN characters when creating event
* patterns so that they match the ARN syntax in the event you want to match.
*
*
* In EventBridge, it is possible to create rules that lead to infinite loops, where a rule is fired repeatedly. For
* example, a rule might detect that ACLs have changed on an S3 bucket, and trigger software to change them to the
* desired state. If the rule is not written carefully, the subsequent change to the ACLs fires the rule again,
* creating an infinite loop.
*
*
* To prevent this, write the rules so that the triggered actions do not re-fire the same rule. For example, your
* rule could fire only if ACLs are found to be in a bad state, instead of after any change.
*
*
* An infinite loop can quickly cause higher than expected charges. We recommend that you use budgeting, which
* alerts you when charges exceed your specified limit. For more information, see Managing Your
* Costs with Budgets.
*
*
* @param putRuleRequest
* @return A Java Future containing the result of the PutRule operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InvalidEventPatternException The event pattern is not valid.
* - LimitExceededException The request failed because it attempted to create resource beyond the allowed
* service quota.
* - ConcurrentModificationException There is concurrent modification on a rule, target, archive, or
* replay.
* - ManagedRuleException This rule was created by an AWS service on behalf of your account. It is managed
* by that service. If you see this error in response to
DeleteRule
or
* RemoveTargets
, you can use the Force
parameter in those calls to delete the
* rule or remove targets from the rule. You cannot modify these managed rules by using
* DisableRule
, EnableRule
, PutTargets
, PutRule
,
* TagResource
, or UntagResource
.
* - InternalException This exception occurs due to unexpected causes.
* - ResourceNotFoundException An entity that you specified does not exist.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EventBridgeException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample EventBridgeAsyncClient.PutRule
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture putRule(PutRuleRequest putRuleRequest) {
List metricPublishers = resolveMetricPublishers(clientConfiguration, putRuleRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "EventBridge");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "PutRule");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
PutRuleResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams().withOperationName("PutRule")
.withMarshaller(new PutRuleRequestMarshaller(protocolFactory)).withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withMetricCollector(apiCallMetricCollector)
.withInput(putRuleRequest));
AwsRequestOverrideConfiguration requestOverrideConfig = putRuleRequest.overrideConfiguration().orElse(null);
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Adds the specified targets to the specified rule, or updates the targets if they are already associated with the
* rule.
*
*
* Targets are the resources that are invoked when a rule is triggered.
*
*
* You can configure the following as targets for Events:
*
*
* -
*
* EC2 instances
*
*
* -
*
* SSM Run Command
*
*
* -
*
* SSM Automation
*
*
* -
*
* AWS Lambda functions
*
*
* -
*
* Data streams in Amazon Kinesis Data Streams
*
*
* -
*
* Data delivery streams in Amazon Kinesis Data Firehose
*
*
* -
*
* Amazon ECS tasks
*
*
* -
*
* AWS Step Functions state machines
*
*
* -
*
* AWS Batch jobs
*
*
* -
*
* AWS CodeBuild projects
*
*
* -
*
* Pipelines in AWS CodePipeline
*
*
* -
*
* Amazon Inspector assessment templates
*
*
* -
*
* Amazon SNS topics
*
*
* -
*
* Amazon SQS queues, including FIFO queues
*
*
* -
*
* The default event bus of another AWS account
*
*
* -
*
* Amazon API Gateway REST APIs
*
*
* -
*
* Redshift Clusters to invoke Data API ExecuteStatement on
*
*
*
*
* Creating rules with built-in targets is supported only in the AWS Management Console. The built-in targets are
* EC2 CreateSnapshot API call
, EC2 RebootInstances API call
,
* EC2 StopInstances API call
, and EC2 TerminateInstances API call
.
*
*
* For some target types, PutTargets
provides target-specific parameters. If the target is a Kinesis
* data stream, you can optionally specify which shard the event goes to by using the KinesisParameters
* argument. To invoke a command on multiple EC2 instances with one rule, you can use the
* RunCommandParameters
field.
*
*
* To be able to make API calls against the resources that you own, Amazon EventBridge (CloudWatch Events) needs the
* appropriate permissions. For AWS Lambda and Amazon SNS resources, EventBridge relies on resource-based policies.
* For EC2 instances, Kinesis data streams, AWS Step Functions state machines and API Gateway REST APIs, EventBridge
* relies on IAM roles that you specify in the RoleARN
argument in PutTargets
. For more
* information, see Authentication and Access Control in the Amazon EventBridge User Guide.
*
*
* If another AWS account is in the same region and has granted you permission (using PutPermission
),
* you can send events to that account. Set that account's event bus as a target of the rules in your account. To
* send the matched events to the other account, specify that account's event bus as the Arn
value when
* you run PutTargets
. If your account sends events to another account, your account is charged for
* each sent event. Each event sent to another account is charged as a custom event. The account receiving the event
* is not charged. For more information, see Amazon
* EventBridge (CloudWatch Events) Pricing.
*
*
*
* Input
, InputPath
, and InputTransformer
are not available with
* PutTarget
if the target is an event bus of a different AWS account.
*
*
*
* If you are setting the event bus of another account as the target, and that account granted permission to your
* account through an organization instead of directly by the account ID, then you must specify a
* RoleArn
with proper permissions in the Target
structure. For more information, see
* Sending and Receiving Events Between AWS Accounts in the Amazon EventBridge User Guide.
*
*
* For more information about enabling cross-account events, see PutPermission.
*
*
* Input, InputPath, and InputTransformer are mutually exclusive and optional parameters of a
* target. When a rule is triggered due to a matched event:
*
*
* -
*
* If none of the following arguments are specified for a target, then the entire event is passed to the target in
* JSON format (unless the target is Amazon EC2 Run Command or Amazon ECS task, in which case nothing from the event
* is passed to the target).
*
*
* -
*
* If Input is specified in the form of valid JSON, then the matched event is overridden with this constant.
*
*
* -
*
* If InputPath is specified in the form of JSONPath (for example, $.detail
), then only the
* part of the event specified in the path is passed to the target (for example, only the detail part of the event
* is passed).
*
*
* -
*
* If InputTransformer is specified, then one or more specified JSONPaths are extracted from the event and
* used as values in a template that you specify as the input to the target.
*
*
*
*
* When you specify InputPath
or InputTransformer
, you must use JSON dot notation, not
* bracket notation.
*
*
* When you add targets to a rule and the associated rule triggers soon after, new or updated targets might not be
* immediately invoked. Allow a short period of time for changes to take effect.
*
*
* This action can partially fail if too many requests are made at the same time. If that happens,
* FailedEntryCount
is non-zero in the response and each entry in FailedEntries
provides
* the ID of the failed target and the error code.
*
*
* @param putTargetsRequest
* @return A Java Future containing the result of the PutTargets operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ResourceNotFoundException An entity that you specified does not exist.
* - ConcurrentModificationException There is concurrent modification on a rule, target, archive, or
* replay.
* - LimitExceededException The request failed because it attempted to create resource beyond the allowed
* service quota.
* - ManagedRuleException This rule was created by an AWS service on behalf of your account. It is managed
* by that service. If you see this error in response to
DeleteRule
or
* RemoveTargets
, you can use the Force
parameter in those calls to delete the
* rule or remove targets from the rule. You cannot modify these managed rules by using
* DisableRule
, EnableRule
, PutTargets
, PutRule
,
* TagResource
, or UntagResource
.
* - InternalException This exception occurs due to unexpected causes.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EventBridgeException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample EventBridgeAsyncClient.PutTargets
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture putTargets(PutTargetsRequest putTargetsRequest) {
List metricPublishers = resolveMetricPublishers(clientConfiguration, putTargetsRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "EventBridge");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "PutTargets");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
PutTargetsResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams().withOperationName("PutTargets")
.withMarshaller(new PutTargetsRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withMetricCollector(apiCallMetricCollector).withInput(putTargetsRequest));
AwsRequestOverrideConfiguration requestOverrideConfig = putTargetsRequest.overrideConfiguration().orElse(null);
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Revokes the permission of another AWS account to be able to put events to the specified event bus. Specify the
* account to revoke by the StatementId
value that you associated with the account when you granted it
* permission with PutPermission
. You can find the StatementId
by using
* DescribeEventBus.
*
*
* @param removePermissionRequest
* @return A Java Future containing the result of the RemovePermission operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ResourceNotFoundException An entity that you specified does not exist.
* - InternalException This exception occurs due to unexpected causes.
* - ConcurrentModificationException There is concurrent modification on a rule, target, archive, or
* replay.
* - OperationDisabledException The operation you are attempting is not available in this region.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EventBridgeException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample EventBridgeAsyncClient.RemovePermission
* @see AWS
* API Documentation
*/
@Override
public CompletableFuture removePermission(RemovePermissionRequest removePermissionRequest) {
List metricPublishers = resolveMetricPublishers(clientConfiguration, removePermissionRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "EventBridge");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "RemovePermission");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, RemovePermissionResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("RemovePermission")
.withMarshaller(new RemovePermissionRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withMetricCollector(apiCallMetricCollector).withInput(removePermissionRequest));
AwsRequestOverrideConfiguration requestOverrideConfig = removePermissionRequest.overrideConfiguration().orElse(null);
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Removes the specified targets from the specified rule. When the rule is triggered, those targets are no longer be
* invoked.
*
*
* When you remove a target, when the associated rule triggers, removed targets might continue to be invoked. Allow
* a short period of time for changes to take effect.
*
*
* This action can partially fail if too many requests are made at the same time. If that happens,
* FailedEntryCount
is non-zero in the response and each entry in FailedEntries
provides
* the ID of the failed target and the error code.
*
*
* @param removeTargetsRequest
* @return A Java Future containing the result of the RemoveTargets operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ResourceNotFoundException An entity that you specified does not exist.
* - ConcurrentModificationException There is concurrent modification on a rule, target, archive, or
* replay.
* - ManagedRuleException This rule was created by an AWS service on behalf of your account. It is managed
* by that service. If you see this error in response to
DeleteRule
or
* RemoveTargets
, you can use the Force
parameter in those calls to delete the
* rule or remove targets from the rule. You cannot modify these managed rules by using
* DisableRule
, EnableRule
, PutTargets
, PutRule
,
* TagResource
, or UntagResource
.
* - InternalException This exception occurs due to unexpected causes.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EventBridgeException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample EventBridgeAsyncClient.RemoveTargets
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture removeTargets(RemoveTargetsRequest removeTargetsRequest) {
List metricPublishers = resolveMetricPublishers(clientConfiguration, removeTargetsRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "EventBridge");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "RemoveTargets");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
RemoveTargetsResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("RemoveTargets")
.withMarshaller(new RemoveTargetsRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withMetricCollector(apiCallMetricCollector).withInput(removeTargetsRequest));
AwsRequestOverrideConfiguration requestOverrideConfig = removeTargetsRequest.overrideConfiguration().orElse(null);
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Starts the specified replay. Events are not necessarily replayed in the exact same order that they were added to
* the archive. A replay processes events to replay based on the time in the event, and replays them using 1 minute
* intervals. If you specify an EventStartTime
and an EventEndTime
that covers a 20 minute
* time range, the events are replayed from the first minute of that 20 minute range first. Then the events from the
* second minute are replayed. You can use DescribeReplay
to determine the progress of a replay. The
* value returned for EventLastReplayedTime
indicates the time within the specified time range
* associated with the last event replayed.
*
*
* @param startReplayRequest
* @return A Java Future containing the result of the StartReplay operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ResourceNotFoundException An entity that you specified does not exist.
* - ResourceAlreadyExistsException The resource you are trying to create already exists.
* - InvalidEventPatternException The event pattern is not valid.
* - LimitExceededException The request failed because it attempted to create resource beyond the allowed
* service quota.
* - InternalException This exception occurs due to unexpected causes.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EventBridgeException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample EventBridgeAsyncClient.StartReplay
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture startReplay(StartReplayRequest startReplayRequest) {
List metricPublishers = resolveMetricPublishers(clientConfiguration, startReplayRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "EventBridge");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "StartReplay");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
StartReplayResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams