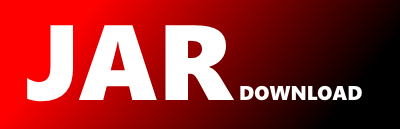
software.amazon.awssdk.services.eventbridge.model.EcsParameters Maven / Gradle / Ivy
Show all versions of eventbridge Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.eventbridge.model;
import java.io.Serializable;
import java.util.Arrays;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* The custom parameters to be used when the target is an Amazon ECS task.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class EcsParameters implements SdkPojo, Serializable, ToCopyableBuilder {
private static final SdkField TASK_DEFINITION_ARN_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("TaskDefinitionArn").getter(getter(EcsParameters::taskDefinitionArn))
.setter(setter(Builder::taskDefinitionArn))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("TaskDefinitionArn").build()).build();
private static final SdkField TASK_COUNT_FIELD = SdkField. builder(MarshallingType.INTEGER)
.memberName("TaskCount").getter(getter(EcsParameters::taskCount)).setter(setter(Builder::taskCount))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("TaskCount").build()).build();
private static final SdkField LAUNCH_TYPE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("LaunchType").getter(getter(EcsParameters::launchTypeAsString)).setter(setter(Builder::launchType))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("LaunchType").build()).build();
private static final SdkField NETWORK_CONFIGURATION_FIELD = SdkField
. builder(MarshallingType.SDK_POJO).memberName("NetworkConfiguration")
.getter(getter(EcsParameters::networkConfiguration)).setter(setter(Builder::networkConfiguration))
.constructor(NetworkConfiguration::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("NetworkConfiguration").build())
.build();
private static final SdkField PLATFORM_VERSION_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("PlatformVersion").getter(getter(EcsParameters::platformVersion))
.setter(setter(Builder::platformVersion))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("PlatformVersion").build()).build();
private static final SdkField GROUP_FIELD = SdkField. builder(MarshallingType.STRING).memberName("Group")
.getter(getter(EcsParameters::group)).setter(setter(Builder::group))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Group").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(TASK_DEFINITION_ARN_FIELD,
TASK_COUNT_FIELD, LAUNCH_TYPE_FIELD, NETWORK_CONFIGURATION_FIELD, PLATFORM_VERSION_FIELD, GROUP_FIELD));
private static final long serialVersionUID = 1L;
private final String taskDefinitionArn;
private final Integer taskCount;
private final String launchType;
private final NetworkConfiguration networkConfiguration;
private final String platformVersion;
private final String group;
private EcsParameters(BuilderImpl builder) {
this.taskDefinitionArn = builder.taskDefinitionArn;
this.taskCount = builder.taskCount;
this.launchType = builder.launchType;
this.networkConfiguration = builder.networkConfiguration;
this.platformVersion = builder.platformVersion;
this.group = builder.group;
}
/**
*
* The ARN of the task definition to use if the event target is an Amazon ECS task.
*
*
* @return The ARN of the task definition to use if the event target is an Amazon ECS task.
*/
public final String taskDefinitionArn() {
return taskDefinitionArn;
}
/**
*
* The number of tasks to create based on TaskDefinition
. The default is 1.
*
*
* @return The number of tasks to create based on TaskDefinition
. The default is 1.
*/
public final Integer taskCount() {
return taskCount;
}
/**
*
* Specifies the launch type on which your task is running. The launch type that you specify here must match one of
* the launch type (compatibilities) of the target task. The FARGATE
value is supported only in the
* Regions where AWS Fargate with Amazon ECS is supported. For more information, see AWS Fargate on Amazon ECS
* in the Amazon Elastic Container Service Developer Guide.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #launchType} will
* return {@link LaunchType#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #launchTypeAsString}.
*
*
* @return Specifies the launch type on which your task is running. The launch type that you specify here must match
* one of the launch type (compatibilities) of the target task. The FARGATE
value is supported
* only in the Regions where AWS Fargate with Amazon ECS is supported. For more information, see AWS Fargate on Amazon
* ECS in the Amazon Elastic Container Service Developer Guide.
* @see LaunchType
*/
public final LaunchType launchType() {
return LaunchType.fromValue(launchType);
}
/**
*
* Specifies the launch type on which your task is running. The launch type that you specify here must match one of
* the launch type (compatibilities) of the target task. The FARGATE
value is supported only in the
* Regions where AWS Fargate with Amazon ECS is supported. For more information, see AWS Fargate on Amazon ECS
* in the Amazon Elastic Container Service Developer Guide.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #launchType} will
* return {@link LaunchType#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #launchTypeAsString}.
*
*
* @return Specifies the launch type on which your task is running. The launch type that you specify here must match
* one of the launch type (compatibilities) of the target task. The FARGATE
value is supported
* only in the Regions where AWS Fargate with Amazon ECS is supported. For more information, see AWS Fargate on Amazon
* ECS in the Amazon Elastic Container Service Developer Guide.
* @see LaunchType
*/
public final String launchTypeAsString() {
return launchType;
}
/**
*
* Use this structure if the ECS task uses the awsvpc
network mode. This structure specifies the VPC
* subnets and security groups associated with the task, and whether a public IP address is to be used. This
* structure is required if LaunchType
is FARGATE
because the awsvpc
mode is
* required for Fargate tasks.
*
*
* If you specify NetworkConfiguration
when the target ECS task does not use the awsvpc
* network mode, the task fails.
*
*
* @return Use this structure if the ECS task uses the awsvpc
network mode. This structure specifies
* the VPC subnets and security groups associated with the task, and whether a public IP address is to be
* used. This structure is required if LaunchType
is FARGATE
because the
* awsvpc
mode is required for Fargate tasks.
*
* If you specify NetworkConfiguration
when the target ECS task does not use the
* awsvpc
network mode, the task fails.
*/
public final NetworkConfiguration networkConfiguration() {
return networkConfiguration;
}
/**
*
* Specifies the platform version for the task. Specify only the numeric portion of the platform version, such as
* 1.1.0
.
*
*
* This structure is used only if LaunchType
is FARGATE
. For more information about valid
* platform versions, see AWS Fargate Platform
* Versions in the Amazon Elastic Container Service Developer Guide.
*
*
* @return Specifies the platform version for the task. Specify only the numeric portion of the platform version,
* such as 1.1.0
.
*
* This structure is used only if LaunchType
is FARGATE
. For more information
* about valid platform versions, see AWS Fargate
* Platform Versions in the Amazon Elastic Container Service Developer Guide.
*/
public final String platformVersion() {
return platformVersion;
}
/**
*
* Specifies an ECS task group for the task. The maximum length is 255 characters.
*
*
* @return Specifies an ECS task group for the task. The maximum length is 255 characters.
*/
public final String group() {
return group;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(taskDefinitionArn());
hashCode = 31 * hashCode + Objects.hashCode(taskCount());
hashCode = 31 * hashCode + Objects.hashCode(launchTypeAsString());
hashCode = 31 * hashCode + Objects.hashCode(networkConfiguration());
hashCode = 31 * hashCode + Objects.hashCode(platformVersion());
hashCode = 31 * hashCode + Objects.hashCode(group());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof EcsParameters)) {
return false;
}
EcsParameters other = (EcsParameters) obj;
return Objects.equals(taskDefinitionArn(), other.taskDefinitionArn()) && Objects.equals(taskCount(), other.taskCount())
&& Objects.equals(launchTypeAsString(), other.launchTypeAsString())
&& Objects.equals(networkConfiguration(), other.networkConfiguration())
&& Objects.equals(platformVersion(), other.platformVersion()) && Objects.equals(group(), other.group());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("EcsParameters").add("TaskDefinitionArn", taskDefinitionArn()).add("TaskCount", taskCount())
.add("LaunchType", launchTypeAsString()).add("NetworkConfiguration", networkConfiguration())
.add("PlatformVersion", platformVersion()).add("Group", group()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "TaskDefinitionArn":
return Optional.ofNullable(clazz.cast(taskDefinitionArn()));
case "TaskCount":
return Optional.ofNullable(clazz.cast(taskCount()));
case "LaunchType":
return Optional.ofNullable(clazz.cast(launchTypeAsString()));
case "NetworkConfiguration":
return Optional.ofNullable(clazz.cast(networkConfiguration()));
case "PlatformVersion":
return Optional.ofNullable(clazz.cast(platformVersion()));
case "Group":
return Optional.ofNullable(clazz.cast(group()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
private static Function