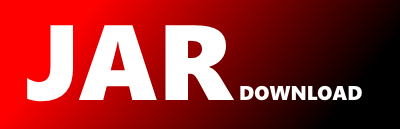
software.amazon.awssdk.services.eventbridge.model.Target Maven / Gradle / Ivy
Show all versions of eventbridge Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.eventbridge.model;
import java.io.Serializable;
import java.util.Arrays;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* Targets are the resources to be invoked when a rule is triggered. For a complete list of services and resources that
* can be set as a target, see PutTargets.
*
*
* If you are setting the event bus of another account as the target, and that account granted permission to your
* account through an organization instead of directly by the account ID, then you must specify a RoleArn
* with proper permissions in the Target
structure. For more information, see Sending
* and Receiving Events Between Amazon Web Services Accounts in the Amazon EventBridge User Guide.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class Target implements SdkPojo, Serializable, ToCopyableBuilder {
private static final SdkField ID_FIELD = SdkField. builder(MarshallingType.STRING).memberName("Id")
.getter(getter(Target::id)).setter(setter(Builder::id))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Id").build()).build();
private static final SdkField ARN_FIELD = SdkField. builder(MarshallingType.STRING).memberName("Arn")
.getter(getter(Target::arn)).setter(setter(Builder::arn))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Arn").build()).build();
private static final SdkField ROLE_ARN_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("RoleArn").getter(getter(Target::roleArn)).setter(setter(Builder::roleArn))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("RoleArn").build()).build();
private static final SdkField INPUT_FIELD = SdkField. builder(MarshallingType.STRING).memberName("Input")
.getter(getter(Target::input)).setter(setter(Builder::input))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Input").build()).build();
private static final SdkField INPUT_PATH_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("InputPath").getter(getter(Target::inputPath)).setter(setter(Builder::inputPath))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("InputPath").build()).build();
private static final SdkField INPUT_TRANSFORMER_FIELD = SdkField
. builder(MarshallingType.SDK_POJO).memberName("InputTransformer")
.getter(getter(Target::inputTransformer)).setter(setter(Builder::inputTransformer))
.constructor(InputTransformer::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("InputTransformer").build()).build();
private static final SdkField KINESIS_PARAMETERS_FIELD = SdkField
. builder(MarshallingType.SDK_POJO).memberName("KinesisParameters")
.getter(getter(Target::kinesisParameters)).setter(setter(Builder::kinesisParameters))
.constructor(KinesisParameters::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("KinesisParameters").build()).build();
private static final SdkField RUN_COMMAND_PARAMETERS_FIELD = SdkField
. builder(MarshallingType.SDK_POJO).memberName("RunCommandParameters")
.getter(getter(Target::runCommandParameters)).setter(setter(Builder::runCommandParameters))
.constructor(RunCommandParameters::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("RunCommandParameters").build())
.build();
private static final SdkField ECS_PARAMETERS_FIELD = SdkField
. builder(MarshallingType.SDK_POJO).memberName("EcsParameters").getter(getter(Target::ecsParameters))
.setter(setter(Builder::ecsParameters)).constructor(EcsParameters::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("EcsParameters").build()).build();
private static final SdkField BATCH_PARAMETERS_FIELD = SdkField
. builder(MarshallingType.SDK_POJO).memberName("BatchParameters")
.getter(getter(Target::batchParameters)).setter(setter(Builder::batchParameters))
.constructor(BatchParameters::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("BatchParameters").build()).build();
private static final SdkField SQS_PARAMETERS_FIELD = SdkField
. builder(MarshallingType.SDK_POJO).memberName("SqsParameters").getter(getter(Target::sqsParameters))
.setter(setter(Builder::sqsParameters)).constructor(SqsParameters::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("SqsParameters").build()).build();
private static final SdkField HTTP_PARAMETERS_FIELD = SdkField
. builder(MarshallingType.SDK_POJO).memberName("HttpParameters")
.getter(getter(Target::httpParameters)).setter(setter(Builder::httpParameters)).constructor(HttpParameters::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("HttpParameters").build()).build();
private static final SdkField REDSHIFT_DATA_PARAMETERS_FIELD = SdkField
. builder(MarshallingType.SDK_POJO).memberName("RedshiftDataParameters")
.getter(getter(Target::redshiftDataParameters)).setter(setter(Builder::redshiftDataParameters))
.constructor(RedshiftDataParameters::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("RedshiftDataParameters").build())
.build();
private static final SdkField SAGE_MAKER_PIPELINE_PARAMETERS_FIELD = SdkField
. builder(MarshallingType.SDK_POJO)
.memberName("SageMakerPipelineParameters")
.getter(getter(Target::sageMakerPipelineParameters))
.setter(setter(Builder::sageMakerPipelineParameters))
.constructor(SageMakerPipelineParameters::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("SageMakerPipelineParameters")
.build()).build();
private static final SdkField DEAD_LETTER_CONFIG_FIELD = SdkField
. builder(MarshallingType.SDK_POJO).memberName("DeadLetterConfig")
.getter(getter(Target::deadLetterConfig)).setter(setter(Builder::deadLetterConfig))
.constructor(DeadLetterConfig::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("DeadLetterConfig").build()).build();
private static final SdkField RETRY_POLICY_FIELD = SdkField. builder(MarshallingType.SDK_POJO)
.memberName("RetryPolicy").getter(getter(Target::retryPolicy)).setter(setter(Builder::retryPolicy))
.constructor(RetryPolicy::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("RetryPolicy").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(ID_FIELD, ARN_FIELD,
ROLE_ARN_FIELD, INPUT_FIELD, INPUT_PATH_FIELD, INPUT_TRANSFORMER_FIELD, KINESIS_PARAMETERS_FIELD,
RUN_COMMAND_PARAMETERS_FIELD, ECS_PARAMETERS_FIELD, BATCH_PARAMETERS_FIELD, SQS_PARAMETERS_FIELD,
HTTP_PARAMETERS_FIELD, REDSHIFT_DATA_PARAMETERS_FIELD, SAGE_MAKER_PIPELINE_PARAMETERS_FIELD,
DEAD_LETTER_CONFIG_FIELD, RETRY_POLICY_FIELD));
private static final long serialVersionUID = 1L;
private final String id;
private final String arn;
private final String roleArn;
private final String input;
private final String inputPath;
private final InputTransformer inputTransformer;
private final KinesisParameters kinesisParameters;
private final RunCommandParameters runCommandParameters;
private final EcsParameters ecsParameters;
private final BatchParameters batchParameters;
private final SqsParameters sqsParameters;
private final HttpParameters httpParameters;
private final RedshiftDataParameters redshiftDataParameters;
private final SageMakerPipelineParameters sageMakerPipelineParameters;
private final DeadLetterConfig deadLetterConfig;
private final RetryPolicy retryPolicy;
private Target(BuilderImpl builder) {
this.id = builder.id;
this.arn = builder.arn;
this.roleArn = builder.roleArn;
this.input = builder.input;
this.inputPath = builder.inputPath;
this.inputTransformer = builder.inputTransformer;
this.kinesisParameters = builder.kinesisParameters;
this.runCommandParameters = builder.runCommandParameters;
this.ecsParameters = builder.ecsParameters;
this.batchParameters = builder.batchParameters;
this.sqsParameters = builder.sqsParameters;
this.httpParameters = builder.httpParameters;
this.redshiftDataParameters = builder.redshiftDataParameters;
this.sageMakerPipelineParameters = builder.sageMakerPipelineParameters;
this.deadLetterConfig = builder.deadLetterConfig;
this.retryPolicy = builder.retryPolicy;
}
/**
*
* The ID of the target within the specified rule. Use this ID to reference the target when updating the rule. We
* recommend using a memorable and unique string.
*
*
* @return The ID of the target within the specified rule. Use this ID to reference the target when updating the
* rule. We recommend using a memorable and unique string.
*/
public final String id() {
return id;
}
/**
*
* The Amazon Resource Name (ARN) of the target.
*
*
* @return The Amazon Resource Name (ARN) of the target.
*/
public final String arn() {
return arn;
}
/**
*
* The Amazon Resource Name (ARN) of the IAM role to be used for this target when the rule is triggered. If one rule
* triggers multiple targets, you can use a different IAM role for each target.
*
*
* @return The Amazon Resource Name (ARN) of the IAM role to be used for this target when the rule is triggered. If
* one rule triggers multiple targets, you can use a different IAM role for each target.
*/
public final String roleArn() {
return roleArn;
}
/**
*
* Valid JSON text passed to the target. In this case, nothing from the event itself is passed to the target. For
* more information, see The JavaScript Object Notation (JSON)
* Data Interchange Format.
*
*
* @return Valid JSON text passed to the target. In this case, nothing from the event itself is passed to the
* target. For more information, see The JavaScript
* Object Notation (JSON) Data Interchange Format.
*/
public final String input() {
return input;
}
/**
*
* The value of the JSONPath that is used for extracting part of the matched event when passing it to the target.
* You may use JSON dot notation or bracket notation. For more information about JSON paths, see JSONPath.
*
*
* @return The value of the JSONPath that is used for extracting part of the matched event when passing it to the
* target. You may use JSON dot notation or bracket notation. For more information about JSON paths, see JSONPath.
*/
public final String inputPath() {
return inputPath;
}
/**
*
* Settings to enable you to provide custom input to a target based on certain event data. You can extract one or
* more key-value pairs from the event and then use that data to send customized input to the target.
*
*
* @return Settings to enable you to provide custom input to a target based on certain event data. You can extract
* one or more key-value pairs from the event and then use that data to send customized input to the target.
*/
public final InputTransformer inputTransformer() {
return inputTransformer;
}
/**
*
* The custom parameter you can use to control the shard assignment, when the target is a Kinesis data stream. If
* you do not include this parameter, the default is to use the eventId
as the partition key.
*
*
* @return The custom parameter you can use to control the shard assignment, when the target is a Kinesis data
* stream. If you do not include this parameter, the default is to use the eventId
as the
* partition key.
*/
public final KinesisParameters kinesisParameters() {
return kinesisParameters;
}
/**
*
* Parameters used when you are using the rule to invoke Amazon EC2 Run Command.
*
*
* @return Parameters used when you are using the rule to invoke Amazon EC2 Run Command.
*/
public final RunCommandParameters runCommandParameters() {
return runCommandParameters;
}
/**
*
* Contains the Amazon ECS task definition and task count to be used, if the event target is an Amazon ECS task. For
* more information about Amazon ECS tasks, see Task Definitions in
* the Amazon EC2 Container Service Developer Guide.
*
*
* @return Contains the Amazon ECS task definition and task count to be used, if the event target is an Amazon ECS
* task. For more information about Amazon ECS tasks, see Task Definitions
* in the Amazon EC2 Container Service Developer Guide.
*/
public final EcsParameters ecsParameters() {
return ecsParameters;
}
/**
*
* If the event target is an Batch job, this contains the job definition, job name, and other parameters. For more
* information, see Jobs in the Batch
* User Guide.
*
*
* @return If the event target is an Batch job, this contains the job definition, job name, and other parameters.
* For more information, see Jobs
* in the Batch User Guide.
*/
public final BatchParameters batchParameters() {
return batchParameters;
}
/**
*
* Contains the message group ID to use when the target is a FIFO queue.
*
*
* If you specify an SQS FIFO queue as a target, the queue must have content-based deduplication enabled.
*
*
* @return Contains the message group ID to use when the target is a FIFO queue.
*
* If you specify an SQS FIFO queue as a target, the queue must have content-based deduplication enabled.
*/
public final SqsParameters sqsParameters() {
return sqsParameters;
}
/**
*
* Contains the HTTP parameters to use when the target is a API Gateway endpoint or EventBridge ApiDestination.
*
*
* If you specify an API Gateway API or EventBridge ApiDestination as a target, you can use this parameter to
* specify headers, path parameters, and query string keys/values as part of your target invoking request. If you're
* using ApiDestinations, the corresponding Connection can also have these values configured. In case of any
* conflicting keys, values from the Connection take precedence.
*
*
* @return Contains the HTTP parameters to use when the target is a API Gateway endpoint or EventBridge
* ApiDestination.
*
* If you specify an API Gateway API or EventBridge ApiDestination as a target, you can use this parameter
* to specify headers, path parameters, and query string keys/values as part of your target invoking
* request. If you're using ApiDestinations, the corresponding Connection can also have these values
* configured. In case of any conflicting keys, values from the Connection take precedence.
*/
public final HttpParameters httpParameters() {
return httpParameters;
}
/**
*
* Contains the Amazon Redshift Data API parameters to use when the target is a Amazon Redshift cluster.
*
*
* If you specify a Amazon Redshift Cluster as a Target, you can use this to specify parameters to invoke the Amazon
* Redshift Data API ExecuteStatement based on EventBridge events.
*
*
* @return Contains the Amazon Redshift Data API parameters to use when the target is a Amazon Redshift cluster.
*
* If you specify a Amazon Redshift Cluster as a Target, you can use this to specify parameters to invoke
* the Amazon Redshift Data API ExecuteStatement based on EventBridge events.
*/
public final RedshiftDataParameters redshiftDataParameters() {
return redshiftDataParameters;
}
/**
*
* Contains the SageMaker Model Building Pipeline parameters to start execution of a SageMaker Model Building
* Pipeline.
*
*
* If you specify a SageMaker Model Building Pipeline as a target, you can use this to specify parameters to start a
* pipeline execution based on EventBridge events.
*
*
* @return Contains the SageMaker Model Building Pipeline parameters to start execution of a SageMaker Model
* Building Pipeline.
*
* If you specify a SageMaker Model Building Pipeline as a target, you can use this to specify parameters to
* start a pipeline execution based on EventBridge events.
*/
public final SageMakerPipelineParameters sageMakerPipelineParameters() {
return sageMakerPipelineParameters;
}
/**
*
* The DeadLetterConfig
that defines the target queue to send dead-letter queue events to.
*
*
* @return The DeadLetterConfig
that defines the target queue to send dead-letter queue events to.
*/
public final DeadLetterConfig deadLetterConfig() {
return deadLetterConfig;
}
/**
*
* The RetryPolicy
object that contains the retry policy configuration to use for the dead-letter
* queue.
*
*
* @return The RetryPolicy
object that contains the retry policy configuration to use for the
* dead-letter queue.
*/
public final RetryPolicy retryPolicy() {
return retryPolicy;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(id());
hashCode = 31 * hashCode + Objects.hashCode(arn());
hashCode = 31 * hashCode + Objects.hashCode(roleArn());
hashCode = 31 * hashCode + Objects.hashCode(input());
hashCode = 31 * hashCode + Objects.hashCode(inputPath());
hashCode = 31 * hashCode + Objects.hashCode(inputTransformer());
hashCode = 31 * hashCode + Objects.hashCode(kinesisParameters());
hashCode = 31 * hashCode + Objects.hashCode(runCommandParameters());
hashCode = 31 * hashCode + Objects.hashCode(ecsParameters());
hashCode = 31 * hashCode + Objects.hashCode(batchParameters());
hashCode = 31 * hashCode + Objects.hashCode(sqsParameters());
hashCode = 31 * hashCode + Objects.hashCode(httpParameters());
hashCode = 31 * hashCode + Objects.hashCode(redshiftDataParameters());
hashCode = 31 * hashCode + Objects.hashCode(sageMakerPipelineParameters());
hashCode = 31 * hashCode + Objects.hashCode(deadLetterConfig());
hashCode = 31 * hashCode + Objects.hashCode(retryPolicy());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof Target)) {
return false;
}
Target other = (Target) obj;
return Objects.equals(id(), other.id()) && Objects.equals(arn(), other.arn())
&& Objects.equals(roleArn(), other.roleArn()) && Objects.equals(input(), other.input())
&& Objects.equals(inputPath(), other.inputPath()) && Objects.equals(inputTransformer(), other.inputTransformer())
&& Objects.equals(kinesisParameters(), other.kinesisParameters())
&& Objects.equals(runCommandParameters(), other.runCommandParameters())
&& Objects.equals(ecsParameters(), other.ecsParameters())
&& Objects.equals(batchParameters(), other.batchParameters())
&& Objects.equals(sqsParameters(), other.sqsParameters())
&& Objects.equals(httpParameters(), other.httpParameters())
&& Objects.equals(redshiftDataParameters(), other.redshiftDataParameters())
&& Objects.equals(sageMakerPipelineParameters(), other.sageMakerPipelineParameters())
&& Objects.equals(deadLetterConfig(), other.deadLetterConfig())
&& Objects.equals(retryPolicy(), other.retryPolicy());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("Target").add("Id", id()).add("Arn", arn()).add("RoleArn", roleArn()).add("Input", input())
.add("InputPath", inputPath()).add("InputTransformer", inputTransformer())
.add("KinesisParameters", kinesisParameters()).add("RunCommandParameters", runCommandParameters())
.add("EcsParameters", ecsParameters()).add("BatchParameters", batchParameters())
.add("SqsParameters", sqsParameters()).add("HttpParameters", httpParameters())
.add("RedshiftDataParameters", redshiftDataParameters())
.add("SageMakerPipelineParameters", sageMakerPipelineParameters()).add("DeadLetterConfig", deadLetterConfig())
.add("RetryPolicy", retryPolicy()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "Id":
return Optional.ofNullable(clazz.cast(id()));
case "Arn":
return Optional.ofNullable(clazz.cast(arn()));
case "RoleArn":
return Optional.ofNullable(clazz.cast(roleArn()));
case "Input":
return Optional.ofNullable(clazz.cast(input()));
case "InputPath":
return Optional.ofNullable(clazz.cast(inputPath()));
case "InputTransformer":
return Optional.ofNullable(clazz.cast(inputTransformer()));
case "KinesisParameters":
return Optional.ofNullable(clazz.cast(kinesisParameters()));
case "RunCommandParameters":
return Optional.ofNullable(clazz.cast(runCommandParameters()));
case "EcsParameters":
return Optional.ofNullable(clazz.cast(ecsParameters()));
case "BatchParameters":
return Optional.ofNullable(clazz.cast(batchParameters()));
case "SqsParameters":
return Optional.ofNullable(clazz.cast(sqsParameters()));
case "HttpParameters":
return Optional.ofNullable(clazz.cast(httpParameters()));
case "RedshiftDataParameters":
return Optional.ofNullable(clazz.cast(redshiftDataParameters()));
case "SageMakerPipelineParameters":
return Optional.ofNullable(clazz.cast(sageMakerPipelineParameters()));
case "DeadLetterConfig":
return Optional.ofNullable(clazz.cast(deadLetterConfig()));
case "RetryPolicy":
return Optional.ofNullable(clazz.cast(retryPolicy()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
private static Function