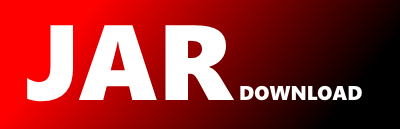
software.amazon.awssdk.services.eventbridge.DefaultEventBridgeAsyncClient Maven / Gradle / Ivy
Show all versions of eventbridge Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.eventbridge;
import java.util.Collections;
import java.util.List;
import java.util.concurrent.CompletableFuture;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.annotations.SdkInternalApi;
import software.amazon.awssdk.awscore.client.handler.AwsAsyncClientHandler;
import software.amazon.awssdk.awscore.exception.AwsServiceException;
import software.amazon.awssdk.awscore.internal.AwsProtocolMetadata;
import software.amazon.awssdk.awscore.internal.AwsServiceProtocol;
import software.amazon.awssdk.core.RequestOverrideConfiguration;
import software.amazon.awssdk.core.SdkPlugin;
import software.amazon.awssdk.core.SdkRequest;
import software.amazon.awssdk.core.client.config.SdkClientConfiguration;
import software.amazon.awssdk.core.client.config.SdkClientOption;
import software.amazon.awssdk.core.client.handler.AsyncClientHandler;
import software.amazon.awssdk.core.client.handler.ClientExecutionParams;
import software.amazon.awssdk.core.http.HttpResponseHandler;
import software.amazon.awssdk.core.metrics.CoreMetric;
import software.amazon.awssdk.metrics.MetricCollector;
import software.amazon.awssdk.metrics.MetricPublisher;
import software.amazon.awssdk.metrics.NoOpMetricCollector;
import software.amazon.awssdk.protocols.core.ExceptionMetadata;
import software.amazon.awssdk.protocols.json.AwsJsonProtocol;
import software.amazon.awssdk.protocols.json.AwsJsonProtocolFactory;
import software.amazon.awssdk.protocols.json.BaseAwsJsonProtocolFactory;
import software.amazon.awssdk.protocols.json.JsonOperationMetadata;
import software.amazon.awssdk.services.eventbridge.internal.EventBridgeServiceClientConfigurationBuilder;
import software.amazon.awssdk.services.eventbridge.model.ActivateEventSourceRequest;
import software.amazon.awssdk.services.eventbridge.model.ActivateEventSourceResponse;
import software.amazon.awssdk.services.eventbridge.model.CancelReplayRequest;
import software.amazon.awssdk.services.eventbridge.model.CancelReplayResponse;
import software.amazon.awssdk.services.eventbridge.model.ConcurrentModificationException;
import software.amazon.awssdk.services.eventbridge.model.CreateApiDestinationRequest;
import software.amazon.awssdk.services.eventbridge.model.CreateApiDestinationResponse;
import software.amazon.awssdk.services.eventbridge.model.CreateArchiveRequest;
import software.amazon.awssdk.services.eventbridge.model.CreateArchiveResponse;
import software.amazon.awssdk.services.eventbridge.model.CreateConnectionRequest;
import software.amazon.awssdk.services.eventbridge.model.CreateConnectionResponse;
import software.amazon.awssdk.services.eventbridge.model.CreateEndpointRequest;
import software.amazon.awssdk.services.eventbridge.model.CreateEndpointResponse;
import software.amazon.awssdk.services.eventbridge.model.CreateEventBusRequest;
import software.amazon.awssdk.services.eventbridge.model.CreateEventBusResponse;
import software.amazon.awssdk.services.eventbridge.model.CreatePartnerEventSourceRequest;
import software.amazon.awssdk.services.eventbridge.model.CreatePartnerEventSourceResponse;
import software.amazon.awssdk.services.eventbridge.model.DeactivateEventSourceRequest;
import software.amazon.awssdk.services.eventbridge.model.DeactivateEventSourceResponse;
import software.amazon.awssdk.services.eventbridge.model.DeauthorizeConnectionRequest;
import software.amazon.awssdk.services.eventbridge.model.DeauthorizeConnectionResponse;
import software.amazon.awssdk.services.eventbridge.model.DeleteApiDestinationRequest;
import software.amazon.awssdk.services.eventbridge.model.DeleteApiDestinationResponse;
import software.amazon.awssdk.services.eventbridge.model.DeleteArchiveRequest;
import software.amazon.awssdk.services.eventbridge.model.DeleteArchiveResponse;
import software.amazon.awssdk.services.eventbridge.model.DeleteConnectionRequest;
import software.amazon.awssdk.services.eventbridge.model.DeleteConnectionResponse;
import software.amazon.awssdk.services.eventbridge.model.DeleteEndpointRequest;
import software.amazon.awssdk.services.eventbridge.model.DeleteEndpointResponse;
import software.amazon.awssdk.services.eventbridge.model.DeleteEventBusRequest;
import software.amazon.awssdk.services.eventbridge.model.DeleteEventBusResponse;
import software.amazon.awssdk.services.eventbridge.model.DeletePartnerEventSourceRequest;
import software.amazon.awssdk.services.eventbridge.model.DeletePartnerEventSourceResponse;
import software.amazon.awssdk.services.eventbridge.model.DeleteRuleRequest;
import software.amazon.awssdk.services.eventbridge.model.DeleteRuleResponse;
import software.amazon.awssdk.services.eventbridge.model.DescribeApiDestinationRequest;
import software.amazon.awssdk.services.eventbridge.model.DescribeApiDestinationResponse;
import software.amazon.awssdk.services.eventbridge.model.DescribeArchiveRequest;
import software.amazon.awssdk.services.eventbridge.model.DescribeArchiveResponse;
import software.amazon.awssdk.services.eventbridge.model.DescribeConnectionRequest;
import software.amazon.awssdk.services.eventbridge.model.DescribeConnectionResponse;
import software.amazon.awssdk.services.eventbridge.model.DescribeEndpointRequest;
import software.amazon.awssdk.services.eventbridge.model.DescribeEndpointResponse;
import software.amazon.awssdk.services.eventbridge.model.DescribeEventBusRequest;
import software.amazon.awssdk.services.eventbridge.model.DescribeEventBusResponse;
import software.amazon.awssdk.services.eventbridge.model.DescribeEventSourceRequest;
import software.amazon.awssdk.services.eventbridge.model.DescribeEventSourceResponse;
import software.amazon.awssdk.services.eventbridge.model.DescribePartnerEventSourceRequest;
import software.amazon.awssdk.services.eventbridge.model.DescribePartnerEventSourceResponse;
import software.amazon.awssdk.services.eventbridge.model.DescribeReplayRequest;
import software.amazon.awssdk.services.eventbridge.model.DescribeReplayResponse;
import software.amazon.awssdk.services.eventbridge.model.DescribeRuleRequest;
import software.amazon.awssdk.services.eventbridge.model.DescribeRuleResponse;
import software.amazon.awssdk.services.eventbridge.model.DisableRuleRequest;
import software.amazon.awssdk.services.eventbridge.model.DisableRuleResponse;
import software.amazon.awssdk.services.eventbridge.model.EnableRuleRequest;
import software.amazon.awssdk.services.eventbridge.model.EnableRuleResponse;
import software.amazon.awssdk.services.eventbridge.model.EventBridgeException;
import software.amazon.awssdk.services.eventbridge.model.IllegalStatusException;
import software.amazon.awssdk.services.eventbridge.model.InternalException;
import software.amazon.awssdk.services.eventbridge.model.InvalidEventPatternException;
import software.amazon.awssdk.services.eventbridge.model.InvalidStateException;
import software.amazon.awssdk.services.eventbridge.model.LimitExceededException;
import software.amazon.awssdk.services.eventbridge.model.ListApiDestinationsRequest;
import software.amazon.awssdk.services.eventbridge.model.ListApiDestinationsResponse;
import software.amazon.awssdk.services.eventbridge.model.ListArchivesRequest;
import software.amazon.awssdk.services.eventbridge.model.ListArchivesResponse;
import software.amazon.awssdk.services.eventbridge.model.ListConnectionsRequest;
import software.amazon.awssdk.services.eventbridge.model.ListConnectionsResponse;
import software.amazon.awssdk.services.eventbridge.model.ListEndpointsRequest;
import software.amazon.awssdk.services.eventbridge.model.ListEndpointsResponse;
import software.amazon.awssdk.services.eventbridge.model.ListEventBusesRequest;
import software.amazon.awssdk.services.eventbridge.model.ListEventBusesResponse;
import software.amazon.awssdk.services.eventbridge.model.ListEventSourcesRequest;
import software.amazon.awssdk.services.eventbridge.model.ListEventSourcesResponse;
import software.amazon.awssdk.services.eventbridge.model.ListPartnerEventSourceAccountsRequest;
import software.amazon.awssdk.services.eventbridge.model.ListPartnerEventSourceAccountsResponse;
import software.amazon.awssdk.services.eventbridge.model.ListPartnerEventSourcesRequest;
import software.amazon.awssdk.services.eventbridge.model.ListPartnerEventSourcesResponse;
import software.amazon.awssdk.services.eventbridge.model.ListReplaysRequest;
import software.amazon.awssdk.services.eventbridge.model.ListReplaysResponse;
import software.amazon.awssdk.services.eventbridge.model.ListRuleNamesByTargetRequest;
import software.amazon.awssdk.services.eventbridge.model.ListRuleNamesByTargetResponse;
import software.amazon.awssdk.services.eventbridge.model.ListRulesRequest;
import software.amazon.awssdk.services.eventbridge.model.ListRulesResponse;
import software.amazon.awssdk.services.eventbridge.model.ListTagsForResourceRequest;
import software.amazon.awssdk.services.eventbridge.model.ListTagsForResourceResponse;
import software.amazon.awssdk.services.eventbridge.model.ListTargetsByRuleRequest;
import software.amazon.awssdk.services.eventbridge.model.ListTargetsByRuleResponse;
import software.amazon.awssdk.services.eventbridge.model.ManagedRuleException;
import software.amazon.awssdk.services.eventbridge.model.OperationDisabledException;
import software.amazon.awssdk.services.eventbridge.model.PolicyLengthExceededException;
import software.amazon.awssdk.services.eventbridge.model.PutEventsRequest;
import software.amazon.awssdk.services.eventbridge.model.PutEventsResponse;
import software.amazon.awssdk.services.eventbridge.model.PutPartnerEventsRequest;
import software.amazon.awssdk.services.eventbridge.model.PutPartnerEventsResponse;
import software.amazon.awssdk.services.eventbridge.model.PutPermissionRequest;
import software.amazon.awssdk.services.eventbridge.model.PutPermissionResponse;
import software.amazon.awssdk.services.eventbridge.model.PutRuleRequest;
import software.amazon.awssdk.services.eventbridge.model.PutRuleResponse;
import software.amazon.awssdk.services.eventbridge.model.PutTargetsRequest;
import software.amazon.awssdk.services.eventbridge.model.PutTargetsResponse;
import software.amazon.awssdk.services.eventbridge.model.RemovePermissionRequest;
import software.amazon.awssdk.services.eventbridge.model.RemovePermissionResponse;
import software.amazon.awssdk.services.eventbridge.model.RemoveTargetsRequest;
import software.amazon.awssdk.services.eventbridge.model.RemoveTargetsResponse;
import software.amazon.awssdk.services.eventbridge.model.ResourceAlreadyExistsException;
import software.amazon.awssdk.services.eventbridge.model.ResourceNotFoundException;
import software.amazon.awssdk.services.eventbridge.model.StartReplayRequest;
import software.amazon.awssdk.services.eventbridge.model.StartReplayResponse;
import software.amazon.awssdk.services.eventbridge.model.TagResourceRequest;
import software.amazon.awssdk.services.eventbridge.model.TagResourceResponse;
import software.amazon.awssdk.services.eventbridge.model.TestEventPatternRequest;
import software.amazon.awssdk.services.eventbridge.model.TestEventPatternResponse;
import software.amazon.awssdk.services.eventbridge.model.UntagResourceRequest;
import software.amazon.awssdk.services.eventbridge.model.UntagResourceResponse;
import software.amazon.awssdk.services.eventbridge.model.UpdateApiDestinationRequest;
import software.amazon.awssdk.services.eventbridge.model.UpdateApiDestinationResponse;
import software.amazon.awssdk.services.eventbridge.model.UpdateArchiveRequest;
import software.amazon.awssdk.services.eventbridge.model.UpdateArchiveResponse;
import software.amazon.awssdk.services.eventbridge.model.UpdateConnectionRequest;
import software.amazon.awssdk.services.eventbridge.model.UpdateConnectionResponse;
import software.amazon.awssdk.services.eventbridge.model.UpdateEndpointRequest;
import software.amazon.awssdk.services.eventbridge.model.UpdateEndpointResponse;
import software.amazon.awssdk.services.eventbridge.transform.ActivateEventSourceRequestMarshaller;
import software.amazon.awssdk.services.eventbridge.transform.CancelReplayRequestMarshaller;
import software.amazon.awssdk.services.eventbridge.transform.CreateApiDestinationRequestMarshaller;
import software.amazon.awssdk.services.eventbridge.transform.CreateArchiveRequestMarshaller;
import software.amazon.awssdk.services.eventbridge.transform.CreateConnectionRequestMarshaller;
import software.amazon.awssdk.services.eventbridge.transform.CreateEndpointRequestMarshaller;
import software.amazon.awssdk.services.eventbridge.transform.CreateEventBusRequestMarshaller;
import software.amazon.awssdk.services.eventbridge.transform.CreatePartnerEventSourceRequestMarshaller;
import software.amazon.awssdk.services.eventbridge.transform.DeactivateEventSourceRequestMarshaller;
import software.amazon.awssdk.services.eventbridge.transform.DeauthorizeConnectionRequestMarshaller;
import software.amazon.awssdk.services.eventbridge.transform.DeleteApiDestinationRequestMarshaller;
import software.amazon.awssdk.services.eventbridge.transform.DeleteArchiveRequestMarshaller;
import software.amazon.awssdk.services.eventbridge.transform.DeleteConnectionRequestMarshaller;
import software.amazon.awssdk.services.eventbridge.transform.DeleteEndpointRequestMarshaller;
import software.amazon.awssdk.services.eventbridge.transform.DeleteEventBusRequestMarshaller;
import software.amazon.awssdk.services.eventbridge.transform.DeletePartnerEventSourceRequestMarshaller;
import software.amazon.awssdk.services.eventbridge.transform.DeleteRuleRequestMarshaller;
import software.amazon.awssdk.services.eventbridge.transform.DescribeApiDestinationRequestMarshaller;
import software.amazon.awssdk.services.eventbridge.transform.DescribeArchiveRequestMarshaller;
import software.amazon.awssdk.services.eventbridge.transform.DescribeConnectionRequestMarshaller;
import software.amazon.awssdk.services.eventbridge.transform.DescribeEndpointRequestMarshaller;
import software.amazon.awssdk.services.eventbridge.transform.DescribeEventBusRequestMarshaller;
import software.amazon.awssdk.services.eventbridge.transform.DescribeEventSourceRequestMarshaller;
import software.amazon.awssdk.services.eventbridge.transform.DescribePartnerEventSourceRequestMarshaller;
import software.amazon.awssdk.services.eventbridge.transform.DescribeReplayRequestMarshaller;
import software.amazon.awssdk.services.eventbridge.transform.DescribeRuleRequestMarshaller;
import software.amazon.awssdk.services.eventbridge.transform.DisableRuleRequestMarshaller;
import software.amazon.awssdk.services.eventbridge.transform.EnableRuleRequestMarshaller;
import software.amazon.awssdk.services.eventbridge.transform.ListApiDestinationsRequestMarshaller;
import software.amazon.awssdk.services.eventbridge.transform.ListArchivesRequestMarshaller;
import software.amazon.awssdk.services.eventbridge.transform.ListConnectionsRequestMarshaller;
import software.amazon.awssdk.services.eventbridge.transform.ListEndpointsRequestMarshaller;
import software.amazon.awssdk.services.eventbridge.transform.ListEventBusesRequestMarshaller;
import software.amazon.awssdk.services.eventbridge.transform.ListEventSourcesRequestMarshaller;
import software.amazon.awssdk.services.eventbridge.transform.ListPartnerEventSourceAccountsRequestMarshaller;
import software.amazon.awssdk.services.eventbridge.transform.ListPartnerEventSourcesRequestMarshaller;
import software.amazon.awssdk.services.eventbridge.transform.ListReplaysRequestMarshaller;
import software.amazon.awssdk.services.eventbridge.transform.ListRuleNamesByTargetRequestMarshaller;
import software.amazon.awssdk.services.eventbridge.transform.ListRulesRequestMarshaller;
import software.amazon.awssdk.services.eventbridge.transform.ListTagsForResourceRequestMarshaller;
import software.amazon.awssdk.services.eventbridge.transform.ListTargetsByRuleRequestMarshaller;
import software.amazon.awssdk.services.eventbridge.transform.PutEventsRequestMarshaller;
import software.amazon.awssdk.services.eventbridge.transform.PutPartnerEventsRequestMarshaller;
import software.amazon.awssdk.services.eventbridge.transform.PutPermissionRequestMarshaller;
import software.amazon.awssdk.services.eventbridge.transform.PutRuleRequestMarshaller;
import software.amazon.awssdk.services.eventbridge.transform.PutTargetsRequestMarshaller;
import software.amazon.awssdk.services.eventbridge.transform.RemovePermissionRequestMarshaller;
import software.amazon.awssdk.services.eventbridge.transform.RemoveTargetsRequestMarshaller;
import software.amazon.awssdk.services.eventbridge.transform.StartReplayRequestMarshaller;
import software.amazon.awssdk.services.eventbridge.transform.TagResourceRequestMarshaller;
import software.amazon.awssdk.services.eventbridge.transform.TestEventPatternRequestMarshaller;
import software.amazon.awssdk.services.eventbridge.transform.UntagResourceRequestMarshaller;
import software.amazon.awssdk.services.eventbridge.transform.UpdateApiDestinationRequestMarshaller;
import software.amazon.awssdk.services.eventbridge.transform.UpdateArchiveRequestMarshaller;
import software.amazon.awssdk.services.eventbridge.transform.UpdateConnectionRequestMarshaller;
import software.amazon.awssdk.services.eventbridge.transform.UpdateEndpointRequestMarshaller;
import software.amazon.awssdk.utils.CompletableFutureUtils;
/**
* Internal implementation of {@link EventBridgeAsyncClient}.
*
* @see EventBridgeAsyncClient#builder()
*/
@Generated("software.amazon.awssdk:codegen")
@SdkInternalApi
final class DefaultEventBridgeAsyncClient implements EventBridgeAsyncClient {
private static final Logger log = LoggerFactory.getLogger(DefaultEventBridgeAsyncClient.class);
private static final AwsProtocolMetadata protocolMetadata = AwsProtocolMetadata.builder()
.serviceProtocol(AwsServiceProtocol.AWS_JSON).build();
private final AsyncClientHandler clientHandler;
private final AwsJsonProtocolFactory protocolFactory;
private final SdkClientConfiguration clientConfiguration;
protected DefaultEventBridgeAsyncClient(SdkClientConfiguration clientConfiguration) {
this.clientHandler = new AwsAsyncClientHandler(clientConfiguration);
this.clientConfiguration = clientConfiguration;
this.protocolFactory = init(AwsJsonProtocolFactory.builder()).build();
}
/**
*
* Activates a partner event source that has been deactivated. Once activated, your matching event bus will start
* receiving events from the event source.
*
*
* @param activateEventSourceRequest
* @return A Java Future containing the result of the ActivateEventSource operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ResourceNotFoundException An entity that you specified does not exist.
* - ConcurrentModificationException There is concurrent modification on a rule, target, archive, or
* replay.
* - InvalidStateException The specified state is not a valid state for an event source.
* - InternalException This exception occurs due to unexpected causes.
* - OperationDisabledException The operation you are attempting is not available in this region.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EventBridgeException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample EventBridgeAsyncClient.ActivateEventSource
* @see AWS API Documentation
*/
@Override
public CompletableFuture activateEventSource(
ActivateEventSourceRequest activateEventSourceRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(activateEventSourceRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, activateEventSourceRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "EventBridge");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "ActivateEventSource");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, ActivateEventSourceResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("ActivateEventSource").withProtocolMetadata(protocolMetadata)
.withMarshaller(new ActivateEventSourceRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(activateEventSourceRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Cancels the specified replay.
*
*
* @param cancelReplayRequest
* @return A Java Future containing the result of the CancelReplay operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ResourceNotFoundException An entity that you specified does not exist.
* - ConcurrentModificationException There is concurrent modification on a rule, target, archive, or
* replay.
* - IllegalStatusException An error occurred because a replay can be canceled only when the state is
* Running or Starting.
* - InternalException This exception occurs due to unexpected causes.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EventBridgeException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample EventBridgeAsyncClient.CancelReplay
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture cancelReplay(CancelReplayRequest cancelReplayRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(cancelReplayRequest, this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, cancelReplayRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "EventBridge");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "CancelReplay");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
CancelReplayResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("CancelReplay").withProtocolMetadata(protocolMetadata)
.withMarshaller(new CancelReplayRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(cancelReplayRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Creates an API destination, which is an HTTP invocation endpoint configured as a target for events.
*
*
* API destinations do not support private destinations, such as interface VPC endpoints.
*
*
* For more information, see API destinations in
* the EventBridge User Guide.
*
*
* @param createApiDestinationRequest
* @return A Java Future containing the result of the CreateApiDestination operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ResourceAlreadyExistsException The resource you are trying to create already exists.
* - ResourceNotFoundException An entity that you specified does not exist.
* - LimitExceededException The request failed because it attempted to create resource beyond the allowed
* service quota.
* - InternalException This exception occurs due to unexpected causes.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EventBridgeException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample EventBridgeAsyncClient.CreateApiDestination
* @see AWS API Documentation
*/
@Override
public CompletableFuture createApiDestination(
CreateApiDestinationRequest createApiDestinationRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(createApiDestinationRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, createApiDestinationRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "EventBridge");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "CreateApiDestination");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, CreateApiDestinationResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("CreateApiDestination").withProtocolMetadata(protocolMetadata)
.withMarshaller(new CreateApiDestinationRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(createApiDestinationRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Creates an archive of events with the specified settings. When you create an archive, incoming events might not
* immediately start being sent to the archive. Allow a short period of time for changes to take effect. If you do
* not specify a pattern to filter events sent to the archive, all events are sent to the archive except replayed
* events. Replayed events are not sent to an archive.
*
*
* @param createArchiveRequest
* @return A Java Future containing the result of the CreateArchive operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ConcurrentModificationException There is concurrent modification on a rule, target, archive, or
* replay.
* - ResourceAlreadyExistsException The resource you are trying to create already exists.
* - ResourceNotFoundException An entity that you specified does not exist.
* - InternalException This exception occurs due to unexpected causes.
* - LimitExceededException The request failed because it attempted to create resource beyond the allowed
* service quota.
* - InvalidEventPatternException The event pattern is not valid.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EventBridgeException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample EventBridgeAsyncClient.CreateArchive
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture createArchive(CreateArchiveRequest createArchiveRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(createArchiveRequest, this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, createArchiveRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "EventBridge");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "CreateArchive");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
CreateArchiveResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("CreateArchive").withProtocolMetadata(protocolMetadata)
.withMarshaller(new CreateArchiveRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(createArchiveRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Creates a connection. A connection defines the authorization type and credentials to use for authorization with
* an API destination HTTP endpoint.
*
*
* @param createConnectionRequest
* @return A Java Future containing the result of the CreateConnection operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ResourceAlreadyExistsException The resource you are trying to create already exists.
* - LimitExceededException The request failed because it attempted to create resource beyond the allowed
* service quota.
* - InternalException This exception occurs due to unexpected causes.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EventBridgeException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample EventBridgeAsyncClient.CreateConnection
* @see AWS
* API Documentation
*/
@Override
public CompletableFuture createConnection(CreateConnectionRequest createConnectionRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(createConnectionRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, createConnectionRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "EventBridge");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "CreateConnection");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, CreateConnectionResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("CreateConnection").withProtocolMetadata(protocolMetadata)
.withMarshaller(new CreateConnectionRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(createConnectionRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Creates a global endpoint. Global endpoints improve your application's availability by making it regional-fault
* tolerant. To do this, you define a primary and secondary Region with event buses in each Region. You also create
* a Amazon Route 53 health check that will tell EventBridge to route events to the secondary Region when an
* "unhealthy" state is encountered and events will be routed back to the primary Region when the health check
* reports a "healthy" state.
*
*
* @param createEndpointRequest
* @return A Java Future containing the result of the CreateEndpoint operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ResourceAlreadyExistsException The resource you are trying to create already exists.
* - LimitExceededException The request failed because it attempted to create resource beyond the allowed
* service quota.
* - InternalException This exception occurs due to unexpected causes.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EventBridgeException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample EventBridgeAsyncClient.CreateEndpoint
* @see AWS
* API Documentation
*/
@Override
public CompletableFuture createEndpoint(CreateEndpointRequest createEndpointRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(createEndpointRequest, this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, createEndpointRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "EventBridge");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "CreateEndpoint");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, CreateEndpointResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("CreateEndpoint").withProtocolMetadata(protocolMetadata)
.withMarshaller(new CreateEndpointRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(createEndpointRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Creates a new event bus within your account. This can be a custom event bus which you can use to receive events
* from your custom applications and services, or it can be a partner event bus which can be matched to a partner
* event source.
*
*
* @param createEventBusRequest
* @return A Java Future containing the result of the CreateEventBus operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ResourceAlreadyExistsException The resource you are trying to create already exists.
* - ResourceNotFoundException An entity that you specified does not exist.
* - InvalidStateException The specified state is not a valid state for an event source.
* - InternalException This exception occurs due to unexpected causes.
* - ConcurrentModificationException There is concurrent modification on a rule, target, archive, or
* replay.
* - LimitExceededException The request failed because it attempted to create resource beyond the allowed
* service quota.
* - OperationDisabledException The operation you are attempting is not available in this region.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EventBridgeException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample EventBridgeAsyncClient.CreateEventBus
* @see AWS
* API Documentation
*/
@Override
public CompletableFuture createEventBus(CreateEventBusRequest createEventBusRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(createEventBusRequest, this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, createEventBusRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "EventBridge");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "CreateEventBus");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, CreateEventBusResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("CreateEventBus").withProtocolMetadata(protocolMetadata)
.withMarshaller(new CreateEventBusRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(createEventBusRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Called by an SaaS partner to create a partner event source. This operation is not used by Amazon Web Services
* customers.
*
*
* Each partner event source can be used by one Amazon Web Services account to create a matching partner event bus
* in that Amazon Web Services account. A SaaS partner must create one partner event source for each Amazon Web
* Services account that wants to receive those event types.
*
*
* A partner event source creates events based on resources within the SaaS partner's service or application.
*
*
* An Amazon Web Services account that creates a partner event bus that matches the partner event source can use
* that event bus to receive events from the partner, and then process them using Amazon Web Services Events rules
* and targets.
*
*
* Partner event source names follow this format:
*
*
* partner_name/event_namespace/event_name
*
*
* -
*
* partner_name is determined during partner registration, and identifies the partner to Amazon Web Services
* customers.
*
*
* -
*
* event_namespace is determined by the partner, and is a way for the partner to categorize their events.
*
*
* -
*
* event_name is determined by the partner, and should uniquely identify an event-generating resource within
* the partner system.
*
*
* The event_name must be unique across all Amazon Web Services customers. This is because the event source
* is a shared resource between the partner and customer accounts, and each partner event source unique in the
* partner account.
*
*
*
*
* The combination of event_namespace and event_name should help Amazon Web Services customers decide
* whether to create an event bus to receive these events.
*
*
* @param createPartnerEventSourceRequest
* @return A Java Future containing the result of the CreatePartnerEventSource operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ResourceAlreadyExistsException The resource you are trying to create already exists.
* - InternalException This exception occurs due to unexpected causes.
* - ConcurrentModificationException There is concurrent modification on a rule, target, archive, or
* replay.
* - LimitExceededException The request failed because it attempted to create resource beyond the allowed
* service quota.
* - OperationDisabledException The operation you are attempting is not available in this region.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EventBridgeException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample EventBridgeAsyncClient.CreatePartnerEventSource
* @see AWS API Documentation
*/
@Override
public CompletableFuture createPartnerEventSource(
CreatePartnerEventSourceRequest createPartnerEventSourceRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(createPartnerEventSourceRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, createPartnerEventSourceRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "EventBridge");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "CreatePartnerEventSource");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, CreatePartnerEventSourceResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("CreatePartnerEventSource").withProtocolMetadata(protocolMetadata)
.withMarshaller(new CreatePartnerEventSourceRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(createPartnerEventSourceRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* You can use this operation to temporarily stop receiving events from the specified partner event source. The
* matching event bus is not deleted.
*
*
* When you deactivate a partner event source, the source goes into PENDING state. If it remains in PENDING state
* for more than two weeks, it is deleted.
*
*
* To activate a deactivated partner event source, use ActivateEventSource.
*
*
* @param deactivateEventSourceRequest
* @return A Java Future containing the result of the DeactivateEventSource operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ResourceNotFoundException An entity that you specified does not exist.
* - ConcurrentModificationException There is concurrent modification on a rule, target, archive, or
* replay.
* - InvalidStateException The specified state is not a valid state for an event source.
* - InternalException This exception occurs due to unexpected causes.
* - OperationDisabledException The operation you are attempting is not available in this region.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EventBridgeException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample EventBridgeAsyncClient.DeactivateEventSource
* @see AWS API Documentation
*/
@Override
public CompletableFuture deactivateEventSource(
DeactivateEventSourceRequest deactivateEventSourceRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(deactivateEventSourceRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, deactivateEventSourceRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "EventBridge");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DeactivateEventSource");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, DeactivateEventSourceResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DeactivateEventSource").withProtocolMetadata(protocolMetadata)
.withMarshaller(new DeactivateEventSourceRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(deactivateEventSourceRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Removes all authorization parameters from the connection. This lets you remove the secret from the connection so
* you can reuse it without having to create a new connection.
*
*
* @param deauthorizeConnectionRequest
* @return A Java Future containing the result of the DeauthorizeConnection operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ConcurrentModificationException There is concurrent modification on a rule, target, archive, or
* replay.
* - ResourceNotFoundException An entity that you specified does not exist.
* - InternalException This exception occurs due to unexpected causes.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EventBridgeException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample EventBridgeAsyncClient.DeauthorizeConnection
* @see AWS API Documentation
*/
@Override
public CompletableFuture deauthorizeConnection(
DeauthorizeConnectionRequest deauthorizeConnectionRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(deauthorizeConnectionRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, deauthorizeConnectionRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "EventBridge");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DeauthorizeConnection");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, DeauthorizeConnectionResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DeauthorizeConnection").withProtocolMetadata(protocolMetadata)
.withMarshaller(new DeauthorizeConnectionRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(deauthorizeConnectionRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Deletes the specified API destination.
*
*
* @param deleteApiDestinationRequest
* @return A Java Future containing the result of the DeleteApiDestination operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ConcurrentModificationException There is concurrent modification on a rule, target, archive, or
* replay.
* - ResourceNotFoundException An entity that you specified does not exist.
* - InternalException This exception occurs due to unexpected causes.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EventBridgeException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample EventBridgeAsyncClient.DeleteApiDestination
* @see AWS API Documentation
*/
@Override
public CompletableFuture deleteApiDestination(
DeleteApiDestinationRequest deleteApiDestinationRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(deleteApiDestinationRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, deleteApiDestinationRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "EventBridge");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DeleteApiDestination");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, DeleteApiDestinationResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DeleteApiDestination").withProtocolMetadata(protocolMetadata)
.withMarshaller(new DeleteApiDestinationRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(deleteApiDestinationRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Deletes the specified archive.
*
*
* @param deleteArchiveRequest
* @return A Java Future containing the result of the DeleteArchive operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ConcurrentModificationException There is concurrent modification on a rule, target, archive, or
* replay.
* - ResourceNotFoundException An entity that you specified does not exist.
* - InternalException This exception occurs due to unexpected causes.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EventBridgeException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample EventBridgeAsyncClient.DeleteArchive
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture deleteArchive(DeleteArchiveRequest deleteArchiveRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(deleteArchiveRequest, this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, deleteArchiveRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "EventBridge");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DeleteArchive");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
DeleteArchiveResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DeleteArchive").withProtocolMetadata(protocolMetadata)
.withMarshaller(new DeleteArchiveRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(deleteArchiveRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Deletes a connection.
*
*
* @param deleteConnectionRequest
* @return A Java Future containing the result of the DeleteConnection operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ConcurrentModificationException There is concurrent modification on a rule, target, archive, or
* replay.
* - ResourceNotFoundException An entity that you specified does not exist.
* - InternalException This exception occurs due to unexpected causes.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EventBridgeException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample EventBridgeAsyncClient.DeleteConnection
* @see AWS
* API Documentation
*/
@Override
public CompletableFuture deleteConnection(DeleteConnectionRequest deleteConnectionRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(deleteConnectionRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, deleteConnectionRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "EventBridge");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DeleteConnection");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, DeleteConnectionResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DeleteConnection").withProtocolMetadata(protocolMetadata)
.withMarshaller(new DeleteConnectionRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(deleteConnectionRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Delete an existing global endpoint. For more information about global endpoints, see Making applications
* Regional-fault tolerant with global endpoints and event replication in the Amazon EventBridge User
* Guide.
*
*
* @param deleteEndpointRequest
* @return A Java Future containing the result of the DeleteEndpoint operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ConcurrentModificationException There is concurrent modification on a rule, target, archive, or
* replay.
* - ResourceNotFoundException An entity that you specified does not exist.
* - InternalException This exception occurs due to unexpected causes.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EventBridgeException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample EventBridgeAsyncClient.DeleteEndpoint
* @see AWS
* API Documentation
*/
@Override
public CompletableFuture deleteEndpoint(DeleteEndpointRequest deleteEndpointRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(deleteEndpointRequest, this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, deleteEndpointRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "EventBridge");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DeleteEndpoint");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, DeleteEndpointResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DeleteEndpoint").withProtocolMetadata(protocolMetadata)
.withMarshaller(new DeleteEndpointRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(deleteEndpointRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Deletes the specified custom event bus or partner event bus. All rules associated with this event bus need to be
* deleted. You can't delete your account's default event bus.
*
*
* @param deleteEventBusRequest
* @return A Java Future containing the result of the DeleteEventBus operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InternalException This exception occurs due to unexpected causes.
* - ConcurrentModificationException There is concurrent modification on a rule, target, archive, or
* replay.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EventBridgeException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample EventBridgeAsyncClient.DeleteEventBus
* @see AWS
* API Documentation
*/
@Override
public CompletableFuture deleteEventBus(DeleteEventBusRequest deleteEventBusRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(deleteEventBusRequest, this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, deleteEventBusRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "EventBridge");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DeleteEventBus");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, DeleteEventBusResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DeleteEventBus").withProtocolMetadata(protocolMetadata)
.withMarshaller(new DeleteEventBusRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(deleteEventBusRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* This operation is used by SaaS partners to delete a partner event source. This operation is not used by Amazon
* Web Services customers.
*
*
* When you delete an event source, the status of the corresponding partner event bus in the Amazon Web Services
* customer account becomes DELETED.
*
*
*
* @param deletePartnerEventSourceRequest
* @return A Java Future containing the result of the DeletePartnerEventSource operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InternalException This exception occurs due to unexpected causes.
-
* ConcurrentModificationException There is concurrent modification on a rule, target, archive, or replay.
*
- OperationDisabledException The operation you are attempting is not available in this region.
*
- SdkException Base class for all exceptions that can be thrown by the SDK (both service and
* client). Can be used for catch all scenarios.
- SdkClientException If any client side error occurs
* such as an IO related failure, failure to get credentials, etc.
- EventBridgeException Base class
* for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
*
* @sample EventBridgeAsyncClient.DeletePartnerEventSource
* @see AWS API Documentation
*/
@Override
public CompletableFuture deletePartnerEventSource(
DeletePartnerEventSourceRequest deletePartnerEventSourceRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(deletePartnerEventSourceRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, deletePartnerEventSourceRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "EventBridge");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DeletePartnerEventSource");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, DeletePartnerEventSourceResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DeletePartnerEventSource").withProtocolMetadata(protocolMetadata)
.withMarshaller(new DeletePartnerEventSourceRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(deletePartnerEventSourceRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Deletes the specified rule.
*
*
* Before you can delete the rule, you must remove all targets, using RemoveTargets.
*
*
* When you delete a rule, incoming events might continue to match to the deleted rule. Allow a short period of time
* for changes to take effect.
*
*
* If you call delete rule multiple times for the same rule, all calls will succeed. When you call delete rule for a
* non-existent custom eventbus, ResourceNotFoundException
is returned.
*
*
* Managed rules are rules created and managed by another Amazon Web Services service on your behalf. These rules
* are created by those other Amazon Web Services services to support functionality in those services. You can
* delete these rules using the Force
option, but you should do so only if you are sure the other
* service is not still using that rule.
*
*
* @param deleteRuleRequest
* @return A Java Future containing the result of the DeleteRule operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ConcurrentModificationException There is concurrent modification on a rule, target, archive, or
* replay.
* - ManagedRuleException This rule was created by an Amazon Web Services service on behalf of your
* account. It is managed by that service. If you see this error in response to
DeleteRule
or
* RemoveTargets
, you can use the Force
parameter in those calls to delete the
* rule or remove targets from the rule. You cannot modify these managed rules by using
* DisableRule
, EnableRule
, PutTargets
, PutRule
,
* TagResource
, or UntagResource
.
* - InternalException This exception occurs due to unexpected causes.
* - ResourceNotFoundException An entity that you specified does not exist.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EventBridgeException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample EventBridgeAsyncClient.DeleteRule
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture deleteRule(DeleteRuleRequest deleteRuleRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(deleteRuleRequest, this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, deleteRuleRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "EventBridge");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DeleteRule");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
DeleteRuleResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams().withOperationName("DeleteRule")
.withProtocolMetadata(protocolMetadata)
.withMarshaller(new DeleteRuleRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(deleteRuleRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Retrieves details about an API destination.
*
*
* @param describeApiDestinationRequest
* @return A Java Future containing the result of the DescribeApiDestination operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ResourceNotFoundException An entity that you specified does not exist.
* - InternalException This exception occurs due to unexpected causes.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EventBridgeException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample EventBridgeAsyncClient.DescribeApiDestination
* @see AWS API Documentation
*/
@Override
public CompletableFuture describeApiDestination(
DescribeApiDestinationRequest describeApiDestinationRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(describeApiDestinationRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, describeApiDestinationRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "EventBridge");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DescribeApiDestination");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, DescribeApiDestinationResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DescribeApiDestination").withProtocolMetadata(protocolMetadata)
.withMarshaller(new DescribeApiDestinationRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(describeApiDestinationRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Retrieves details about an archive.
*
*
* @param describeArchiveRequest
* @return A Java Future containing the result of the DescribeArchive operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ResourceAlreadyExistsException The resource you are trying to create already exists.
* - ResourceNotFoundException An entity that you specified does not exist.
* - InternalException This exception occurs due to unexpected causes.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EventBridgeException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample EventBridgeAsyncClient.DescribeArchive
* @see AWS
* API Documentation
*/
@Override
public CompletableFuture describeArchive(DescribeArchiveRequest describeArchiveRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(describeArchiveRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, describeArchiveRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "EventBridge");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DescribeArchive");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, DescribeArchiveResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DescribeArchive").withProtocolMetadata(protocolMetadata)
.withMarshaller(new DescribeArchiveRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(describeArchiveRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Retrieves details about a connection.
*
*
* @param describeConnectionRequest
* @return A Java Future containing the result of the DescribeConnection operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ResourceNotFoundException An entity that you specified does not exist.
* - InternalException This exception occurs due to unexpected causes.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EventBridgeException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample EventBridgeAsyncClient.DescribeConnection
* @see AWS API Documentation
*/
@Override
public CompletableFuture describeConnection(DescribeConnectionRequest describeConnectionRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(describeConnectionRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, describeConnectionRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "EventBridge");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DescribeConnection");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, DescribeConnectionResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DescribeConnection").withProtocolMetadata(protocolMetadata)
.withMarshaller(new DescribeConnectionRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(describeConnectionRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Get the information about an existing global endpoint. For more information about global endpoints, see Making applications
* Regional-fault tolerant with global endpoints and event replication in the Amazon EventBridge User
* Guide.
*
*
* @param describeEndpointRequest
* @return A Java Future containing the result of the DescribeEndpoint operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ResourceNotFoundException An entity that you specified does not exist.
* - InternalException This exception occurs due to unexpected causes.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EventBridgeException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample EventBridgeAsyncClient.DescribeEndpoint
* @see AWS
* API Documentation
*/
@Override
public CompletableFuture describeEndpoint(DescribeEndpointRequest describeEndpointRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(describeEndpointRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, describeEndpointRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "EventBridge");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DescribeEndpoint");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, DescribeEndpointResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DescribeEndpoint").withProtocolMetadata(protocolMetadata)
.withMarshaller(new DescribeEndpointRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(describeEndpointRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Displays details about an event bus in your account. This can include the external Amazon Web Services accounts
* that are permitted to write events to your default event bus, and the associated policy. For custom event buses
* and partner event buses, it displays the name, ARN, policy, state, and creation time.
*
*
* To enable your account to receive events from other accounts on its default event bus, use PutPermission.
*
*
* For more information about partner event buses, see CreateEventBus.
*
*
* @param describeEventBusRequest
* @return A Java Future containing the result of the DescribeEventBus operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ResourceNotFoundException An entity that you specified does not exist.
* - InternalException This exception occurs due to unexpected causes.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EventBridgeException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample EventBridgeAsyncClient.DescribeEventBus
* @see AWS
* API Documentation
*/
@Override
public CompletableFuture describeEventBus(DescribeEventBusRequest describeEventBusRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(describeEventBusRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, describeEventBusRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "EventBridge");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DescribeEventBus");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, DescribeEventBusResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DescribeEventBus").withProtocolMetadata(protocolMetadata)
.withMarshaller(new DescribeEventBusRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(describeEventBusRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* This operation lists details about a partner event source that is shared with your account.
*
*
* @param describeEventSourceRequest
* @return A Java Future containing the result of the DescribeEventSource operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ResourceNotFoundException An entity that you specified does not exist.
* - InternalException This exception occurs due to unexpected causes.
* - OperationDisabledException The operation you are attempting is not available in this region.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EventBridgeException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample EventBridgeAsyncClient.DescribeEventSource
* @see AWS API Documentation
*/
@Override
public CompletableFuture describeEventSource(
DescribeEventSourceRequest describeEventSourceRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(describeEventSourceRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, describeEventSourceRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "EventBridge");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DescribeEventSource");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, DescribeEventSourceResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DescribeEventSource").withProtocolMetadata(protocolMetadata)
.withMarshaller(new DescribeEventSourceRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(describeEventSourceRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* An SaaS partner can use this operation to list details about a partner event source that they have created.
* Amazon Web Services customers do not use this operation. Instead, Amazon Web Services customers can use DescribeEventSource to see details about a partner event source that is shared with them.
*
*
* @param describePartnerEventSourceRequest
* @return A Java Future containing the result of the DescribePartnerEventSource operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ResourceNotFoundException An entity that you specified does not exist.
* - InternalException This exception occurs due to unexpected causes.
* - OperationDisabledException The operation you are attempting is not available in this region.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EventBridgeException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample EventBridgeAsyncClient.DescribePartnerEventSource
* @see AWS API Documentation
*/
@Override
public CompletableFuture describePartnerEventSource(
DescribePartnerEventSourceRequest describePartnerEventSourceRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(describePartnerEventSourceRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, describePartnerEventSourceRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "EventBridge");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DescribePartnerEventSource");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, DescribePartnerEventSourceResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DescribePartnerEventSource").withProtocolMetadata(protocolMetadata)
.withMarshaller(new DescribePartnerEventSourceRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(describePartnerEventSourceRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Retrieves details about a replay. Use DescribeReplay
to determine the progress of a running replay.
* A replay processes events to replay based on the time in the event, and replays them using 1 minute intervals. If
* you use StartReplay
and specify an EventStartTime
and an EventEndTime
that
* covers a 20 minute time range, the events are replayed from the first minute of that 20 minute range first. Then
* the events from the second minute are replayed. You can use DescribeReplay
to determine the progress
* of a replay. The value returned for EventLastReplayedTime
indicates the time within the specified
* time range associated with the last event replayed.
*
*
* @param describeReplayRequest
* @return A Java Future containing the result of the DescribeReplay operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ResourceNotFoundException An entity that you specified does not exist.
* - InternalException This exception occurs due to unexpected causes.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EventBridgeException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample EventBridgeAsyncClient.DescribeReplay
* @see AWS
* API Documentation
*/
@Override
public CompletableFuture describeReplay(DescribeReplayRequest describeReplayRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(describeReplayRequest, this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, describeReplayRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "EventBridge");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DescribeReplay");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, DescribeReplayResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DescribeReplay").withProtocolMetadata(protocolMetadata)
.withMarshaller(new DescribeReplayRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(describeReplayRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Describes the specified rule.
*
*
* DescribeRule does not list the targets of a rule. To see the targets associated with a rule, use ListTargetsByRule.
*
*
* @param describeRuleRequest
* @return A Java Future containing the result of the DescribeRule operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ResourceNotFoundException An entity that you specified does not exist.
* - InternalException This exception occurs due to unexpected causes.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EventBridgeException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample EventBridgeAsyncClient.DescribeRule
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture describeRule(DescribeRuleRequest describeRuleRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(describeRuleRequest, this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, describeRuleRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "EventBridge");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DescribeRule");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
DescribeRuleResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DescribeRule").withProtocolMetadata(protocolMetadata)
.withMarshaller(new DescribeRuleRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(describeRuleRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Disables the specified rule. A disabled rule won't match any events, and won't self-trigger if it has a schedule
* expression.
*
*
* When you disable a rule, incoming events might continue to match to the disabled rule. Allow a short period of
* time for changes to take effect.
*
*
* @param disableRuleRequest
* @return A Java Future containing the result of the DisableRule operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ResourceNotFoundException An entity that you specified does not exist.
* - ConcurrentModificationException There is concurrent modification on a rule, target, archive, or
* replay.
* - ManagedRuleException This rule was created by an Amazon Web Services service on behalf of your
* account. It is managed by that service. If you see this error in response to
DeleteRule
or
* RemoveTargets
, you can use the Force
parameter in those calls to delete the
* rule or remove targets from the rule. You cannot modify these managed rules by using
* DisableRule
, EnableRule
, PutTargets
, PutRule
,
* TagResource
, or UntagResource
.
* - InternalException This exception occurs due to unexpected causes.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EventBridgeException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample EventBridgeAsyncClient.DisableRule
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture disableRule(DisableRuleRequest disableRuleRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(disableRuleRequest, this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, disableRuleRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "EventBridge");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DisableRule");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
DisableRuleResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DisableRule").withProtocolMetadata(protocolMetadata)
.withMarshaller(new DisableRuleRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(disableRuleRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Enables the specified rule. If the rule does not exist, the operation fails.
*
*
* When you enable a rule, incoming events might not immediately start matching to a newly enabled rule. Allow a
* short period of time for changes to take effect.
*
*
* @param enableRuleRequest
* @return A Java Future containing the result of the EnableRule operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ResourceNotFoundException An entity that you specified does not exist.
* - ConcurrentModificationException There is concurrent modification on a rule, target, archive, or
* replay.
* - ManagedRuleException This rule was created by an Amazon Web Services service on behalf of your
* account. It is managed by that service. If you see this error in response to
DeleteRule
or
* RemoveTargets
, you can use the Force
parameter in those calls to delete the
* rule or remove targets from the rule. You cannot modify these managed rules by using
* DisableRule
, EnableRule
, PutTargets
, PutRule
,
* TagResource
, or UntagResource
.
* - InternalException This exception occurs due to unexpected causes.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EventBridgeException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample EventBridgeAsyncClient.EnableRule
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture enableRule(EnableRuleRequest enableRuleRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(enableRuleRequest, this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, enableRuleRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "EventBridge");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "EnableRule");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
EnableRuleResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams().withOperationName("EnableRule")
.withProtocolMetadata(protocolMetadata)
.withMarshaller(new EnableRuleRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(enableRuleRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Retrieves a list of API destination in the account in the current Region.
*
*
* @param listApiDestinationsRequest
* @return A Java Future containing the result of the ListApiDestinations operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InternalException This exception occurs due to unexpected causes.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EventBridgeException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample EventBridgeAsyncClient.ListApiDestinations
* @see AWS API Documentation
*/
@Override
public CompletableFuture listApiDestinations(
ListApiDestinationsRequest listApiDestinationsRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(listApiDestinationsRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, listApiDestinationsRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "EventBridge");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "ListApiDestinations");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, ListApiDestinationsResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("ListApiDestinations").withProtocolMetadata(protocolMetadata)
.withMarshaller(new ListApiDestinationsRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(listApiDestinationsRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Lists your archives. You can either list all the archives or you can provide a prefix to match to the archive
* names. Filter parameters are exclusive.
*
*
* @param listArchivesRequest
* @return A Java Future containing the result of the ListArchives operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ResourceNotFoundException An entity that you specified does not exist.
* - InternalException This exception occurs due to unexpected causes.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EventBridgeException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample EventBridgeAsyncClient.ListArchives
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture listArchives(ListArchivesRequest listArchivesRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(listArchivesRequest, this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, listArchivesRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "EventBridge");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "ListArchives");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
ListArchivesResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("ListArchives").withProtocolMetadata(protocolMetadata)
.withMarshaller(new ListArchivesRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(listArchivesRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Retrieves a list of connections from the account.
*
*
* @param listConnectionsRequest
* @return A Java Future containing the result of the ListConnections operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InternalException This exception occurs due to unexpected causes.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EventBridgeException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample EventBridgeAsyncClient.ListConnections
* @see AWS
* API Documentation
*/
@Override
public CompletableFuture listConnections(ListConnectionsRequest listConnectionsRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(listConnectionsRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, listConnectionsRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "EventBridge");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "ListConnections");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, ListConnectionsResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("ListConnections").withProtocolMetadata(protocolMetadata)
.withMarshaller(new ListConnectionsRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(listConnectionsRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* List the global endpoints associated with this account. For more information about global endpoints, see Making applications
* Regional-fault tolerant with global endpoints and event replication in the Amazon EventBridge User
* Guide.
*
*
* @param listEndpointsRequest
* @return A Java Future containing the result of the ListEndpoints operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InternalException This exception occurs due to unexpected causes.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EventBridgeException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample EventBridgeAsyncClient.ListEndpoints
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture listEndpoints(ListEndpointsRequest listEndpointsRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(listEndpointsRequest, this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, listEndpointsRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "EventBridge");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "ListEndpoints");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
ListEndpointsResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("ListEndpoints").withProtocolMetadata(protocolMetadata)
.withMarshaller(new ListEndpointsRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(listEndpointsRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Lists all the event buses in your account, including the default event bus, custom event buses, and partner event
* buses.
*
*
* @param listEventBusesRequest
* @return A Java Future containing the result of the ListEventBuses operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InternalException This exception occurs due to unexpected causes.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EventBridgeException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample EventBridgeAsyncClient.ListEventBuses
* @see AWS
* API Documentation
*/
@Override
public CompletableFuture listEventBuses(ListEventBusesRequest listEventBusesRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(listEventBusesRequest, this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, listEventBusesRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "EventBridge");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "ListEventBuses");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, ListEventBusesResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("ListEventBuses").withProtocolMetadata(protocolMetadata)
.withMarshaller(new ListEventBusesRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(listEventBusesRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* You can use this to see all the partner event sources that have been shared with your Amazon Web Services
* account. For more information about partner event sources, see CreateEventBus.
*
*
* @param listEventSourcesRequest
* @return A Java Future containing the result of the ListEventSources operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InternalException This exception occurs due to unexpected causes.
* - OperationDisabledException The operation you are attempting is not available in this region.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EventBridgeException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample EventBridgeAsyncClient.ListEventSources
* @see AWS
* API Documentation
*/
@Override
public CompletableFuture listEventSources(ListEventSourcesRequest listEventSourcesRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(listEventSourcesRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, listEventSourcesRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "EventBridge");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "ListEventSources");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, ListEventSourcesResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("ListEventSources").withProtocolMetadata(protocolMetadata)
.withMarshaller(new ListEventSourcesRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(listEventSourcesRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* An SaaS partner can use this operation to display the Amazon Web Services account ID that a particular partner
* event source name is associated with. This operation is not used by Amazon Web Services customers.
*
*
* @param listPartnerEventSourceAccountsRequest
* @return A Java Future containing the result of the ListPartnerEventSourceAccounts operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ResourceNotFoundException An entity that you specified does not exist.
* - InternalException This exception occurs due to unexpected causes.
* - OperationDisabledException The operation you are attempting is not available in this region.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EventBridgeException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample EventBridgeAsyncClient.ListPartnerEventSourceAccounts
* @see AWS API Documentation
*/
@Override
public CompletableFuture listPartnerEventSourceAccounts(
ListPartnerEventSourceAccountsRequest listPartnerEventSourceAccountsRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(listPartnerEventSourceAccountsRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration,
listPartnerEventSourceAccountsRequest.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "EventBridge");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "ListPartnerEventSourceAccounts");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, ListPartnerEventSourceAccountsResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("ListPartnerEventSourceAccounts").withProtocolMetadata(protocolMetadata)
.withMarshaller(new ListPartnerEventSourceAccountsRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(listPartnerEventSourceAccountsRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* An SaaS partner can use this operation to list all the partner event source names that they have created. This
* operation is not used by Amazon Web Services customers.
*
*
* @param listPartnerEventSourcesRequest
* @return A Java Future containing the result of the ListPartnerEventSources operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InternalException This exception occurs due to unexpected causes.
* - OperationDisabledException The operation you are attempting is not available in this region.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EventBridgeException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample EventBridgeAsyncClient.ListPartnerEventSources
* @see AWS API Documentation
*/
@Override
public CompletableFuture listPartnerEventSources(
ListPartnerEventSourcesRequest listPartnerEventSourcesRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(listPartnerEventSourcesRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, listPartnerEventSourcesRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "EventBridge");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "ListPartnerEventSources");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, ListPartnerEventSourcesResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams