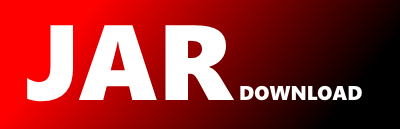
software.amazon.awssdk.services.eventbridge.model.UpdateConnectionOAuthRequestParameters Maven / Gradle / Ivy
Show all versions of eventbridge Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.eventbridge.model;
import java.io.Serializable;
import java.util.Arrays;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* Contains the OAuth request parameters to use for the connection.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class UpdateConnectionOAuthRequestParameters implements SdkPojo, Serializable,
ToCopyableBuilder {
private static final SdkField CLIENT_PARAMETERS_FIELD = SdkField
. builder(MarshallingType.SDK_POJO).memberName("ClientParameters")
.getter(getter(UpdateConnectionOAuthRequestParameters::clientParameters)).setter(setter(Builder::clientParameters))
.constructor(UpdateConnectionOAuthClientRequestParameters::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ClientParameters").build()).build();
private static final SdkField AUTHORIZATION_ENDPOINT_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("AuthorizationEndpoint").getter(getter(UpdateConnectionOAuthRequestParameters::authorizationEndpoint))
.setter(setter(Builder::authorizationEndpoint))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("AuthorizationEndpoint").build())
.build();
private static final SdkField HTTP_METHOD_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("HttpMethod").getter(getter(UpdateConnectionOAuthRequestParameters::httpMethodAsString))
.setter(setter(Builder::httpMethod))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("HttpMethod").build()).build();
private static final SdkField O_AUTH_HTTP_PARAMETERS_FIELD = SdkField
. builder(MarshallingType.SDK_POJO).memberName("OAuthHttpParameters")
.getter(getter(UpdateConnectionOAuthRequestParameters::oAuthHttpParameters))
.setter(setter(Builder::oAuthHttpParameters)).constructor(ConnectionHttpParameters::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("OAuthHttpParameters").build())
.build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(CLIENT_PARAMETERS_FIELD,
AUTHORIZATION_ENDPOINT_FIELD, HTTP_METHOD_FIELD, O_AUTH_HTTP_PARAMETERS_FIELD));
private static final long serialVersionUID = 1L;
private final UpdateConnectionOAuthClientRequestParameters clientParameters;
private final String authorizationEndpoint;
private final String httpMethod;
private final ConnectionHttpParameters oAuthHttpParameters;
private UpdateConnectionOAuthRequestParameters(BuilderImpl builder) {
this.clientParameters = builder.clientParameters;
this.authorizationEndpoint = builder.authorizationEndpoint;
this.httpMethod = builder.httpMethod;
this.oAuthHttpParameters = builder.oAuthHttpParameters;
}
/**
*
* A UpdateConnectionOAuthClientRequestParameters
object that contains the client parameters to use for
* the connection when OAuth is specified as the authorization type.
*
*
* @return A UpdateConnectionOAuthClientRequestParameters
object that contains the client parameters to
* use for the connection when OAuth is specified as the authorization type.
*/
public final UpdateConnectionOAuthClientRequestParameters clientParameters() {
return clientParameters;
}
/**
*
* The URL to the authorization endpoint when OAuth is specified as the authorization type.
*
*
* @return The URL to the authorization endpoint when OAuth is specified as the authorization type.
*/
public final String authorizationEndpoint() {
return authorizationEndpoint;
}
/**
*
* The method used to connect to the HTTP endpoint.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #httpMethod} will
* return {@link ConnectionOAuthHttpMethod#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is
* available from {@link #httpMethodAsString}.
*
*
* @return The method used to connect to the HTTP endpoint.
* @see ConnectionOAuthHttpMethod
*/
public final ConnectionOAuthHttpMethod httpMethod() {
return ConnectionOAuthHttpMethod.fromValue(httpMethod);
}
/**
*
* The method used to connect to the HTTP endpoint.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #httpMethod} will
* return {@link ConnectionOAuthHttpMethod#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is
* available from {@link #httpMethodAsString}.
*
*
* @return The method used to connect to the HTTP endpoint.
* @see ConnectionOAuthHttpMethod
*/
public final String httpMethodAsString() {
return httpMethod;
}
/**
*
* The additional HTTP parameters used for the OAuth authorization request.
*
*
* @return The additional HTTP parameters used for the OAuth authorization request.
*/
public final ConnectionHttpParameters oAuthHttpParameters() {
return oAuthHttpParameters;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(clientParameters());
hashCode = 31 * hashCode + Objects.hashCode(authorizationEndpoint());
hashCode = 31 * hashCode + Objects.hashCode(httpMethodAsString());
hashCode = 31 * hashCode + Objects.hashCode(oAuthHttpParameters());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof UpdateConnectionOAuthRequestParameters)) {
return false;
}
UpdateConnectionOAuthRequestParameters other = (UpdateConnectionOAuthRequestParameters) obj;
return Objects.equals(clientParameters(), other.clientParameters())
&& Objects.equals(authorizationEndpoint(), other.authorizationEndpoint())
&& Objects.equals(httpMethodAsString(), other.httpMethodAsString())
&& Objects.equals(oAuthHttpParameters(), other.oAuthHttpParameters());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("UpdateConnectionOAuthRequestParameters").add("ClientParameters", clientParameters())
.add("AuthorizationEndpoint", authorizationEndpoint()).add("HttpMethod", httpMethodAsString())
.add("OAuthHttpParameters", oAuthHttpParameters()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "ClientParameters":
return Optional.ofNullable(clazz.cast(clientParameters()));
case "AuthorizationEndpoint":
return Optional.ofNullable(clazz.cast(authorizationEndpoint()));
case "HttpMethod":
return Optional.ofNullable(clazz.cast(httpMethodAsString()));
case "OAuthHttpParameters":
return Optional.ofNullable(clazz.cast(oAuthHttpParameters()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
private static Function