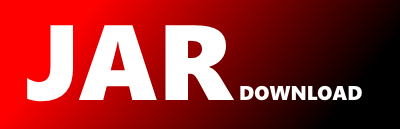
software.amazon.awssdk.services.finspacedata.model.UpdateUserRequest Maven / Gradle / Ivy
Show all versions of finspacedata Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.finspacedata.model;
import java.util.Arrays;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.awscore.AwsRequestOverrideConfiguration;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.DefaultValueTrait;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*/
@Generated("software.amazon.awssdk:codegen")
public final class UpdateUserRequest extends FinspaceDataRequest implements
ToCopyableBuilder {
private static final SdkField USER_ID_FIELD = SdkField. builder(MarshallingType.STRING).memberName("userId")
.getter(getter(UpdateUserRequest::userId)).setter(setter(Builder::userId))
.traits(LocationTrait.builder().location(MarshallLocation.PATH).locationName("userId").build()).build();
private static final SdkField TYPE_FIELD = SdkField. builder(MarshallingType.STRING).memberName("type")
.getter(getter(UpdateUserRequest::typeAsString)).setter(setter(Builder::type))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("type").build()).build();
private static final SdkField FIRST_NAME_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("firstName").getter(getter(UpdateUserRequest::firstName)).setter(setter(Builder::firstName))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("firstName").build()).build();
private static final SdkField LAST_NAME_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("lastName").getter(getter(UpdateUserRequest::lastName)).setter(setter(Builder::lastName))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("lastName").build()).build();
private static final SdkField API_ACCESS_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("apiAccess").getter(getter(UpdateUserRequest::apiAccessAsString)).setter(setter(Builder::apiAccess))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("apiAccess").build()).build();
private static final SdkField API_ACCESS_PRINCIPAL_ARN_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("apiAccessPrincipalArn").getter(getter(UpdateUserRequest::apiAccessPrincipalArn))
.setter(setter(Builder::apiAccessPrincipalArn))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("apiAccessPrincipalArn").build())
.build();
private static final SdkField CLIENT_TOKEN_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("clientToken")
.getter(getter(UpdateUserRequest::clientToken))
.setter(setter(Builder::clientToken))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("clientToken").build(),
DefaultValueTrait.idempotencyToken()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(USER_ID_FIELD, TYPE_FIELD,
FIRST_NAME_FIELD, LAST_NAME_FIELD, API_ACCESS_FIELD, API_ACCESS_PRINCIPAL_ARN_FIELD, CLIENT_TOKEN_FIELD));
private final String userId;
private final String type;
private final String firstName;
private final String lastName;
private final String apiAccess;
private final String apiAccessPrincipalArn;
private final String clientToken;
private UpdateUserRequest(BuilderImpl builder) {
super(builder);
this.userId = builder.userId;
this.type = builder.type;
this.firstName = builder.firstName;
this.lastName = builder.lastName;
this.apiAccess = builder.apiAccess;
this.apiAccessPrincipalArn = builder.apiAccessPrincipalArn;
this.clientToken = builder.clientToken;
}
/**
*
* The unique identifier for the user account to update.
*
*
* @return The unique identifier for the user account to update.
*/
public final String userId() {
return userId;
}
/**
*
* The option to indicate the type of user.
*
*
* -
*
* SUPER_USER
– A user with permission to all the functionality and data in FinSpace.
*
*
* -
*
* APP_USER
– A user with specific permissions in FinSpace. The users are assigned permissions by
* adding them to a permissions group.
*
*
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #type} will return
* {@link UserType#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #typeAsString}.
*
*
* @return The option to indicate the type of user.
*
* -
*
* SUPER_USER
– A user with permission to all the functionality and data in FinSpace.
*
*
* -
*
* APP_USER
– A user with specific permissions in FinSpace. The users are assigned permissions
* by adding them to a permissions group.
*
*
* @see UserType
*/
public final UserType type() {
return UserType.fromValue(type);
}
/**
*
* The option to indicate the type of user.
*
*
* -
*
* SUPER_USER
– A user with permission to all the functionality and data in FinSpace.
*
*
* -
*
* APP_USER
– A user with specific permissions in FinSpace. The users are assigned permissions by
* adding them to a permissions group.
*
*
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #type} will return
* {@link UserType#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #typeAsString}.
*
*
* @return The option to indicate the type of user.
*
* -
*
* SUPER_USER
– A user with permission to all the functionality and data in FinSpace.
*
*
* -
*
* APP_USER
– A user with specific permissions in FinSpace. The users are assigned permissions
* by adding them to a permissions group.
*
*
* @see UserType
*/
public final String typeAsString() {
return type;
}
/**
*
* The first name of the user.
*
*
* @return The first name of the user.
*/
public final String firstName() {
return firstName;
}
/**
*
* The last name of the user.
*
*
* @return The last name of the user.
*/
public final String lastName() {
return lastName;
}
/**
*
* The option to indicate whether the user can use the GetProgrammaticAccessCredentials
API to obtain
* credentials that can then be used to access other FinSpace Data API operations.
*
*
* -
*
* ENABLED
– The user has permissions to use the APIs.
*
*
* -
*
* DISABLED
– The user does not have permissions to use any APIs.
*
*
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #apiAccess} will
* return {@link ApiAccess#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #apiAccessAsString}.
*
*
* @return The option to indicate whether the user can use the GetProgrammaticAccessCredentials
API to
* obtain credentials that can then be used to access other FinSpace Data API operations.
*
* -
*
* ENABLED
– The user has permissions to use the APIs.
*
*
* -
*
* DISABLED
– The user does not have permissions to use any APIs.
*
*
* @see ApiAccess
*/
public final ApiAccess apiAccess() {
return ApiAccess.fromValue(apiAccess);
}
/**
*
* The option to indicate whether the user can use the GetProgrammaticAccessCredentials
API to obtain
* credentials that can then be used to access other FinSpace Data API operations.
*
*
* -
*
* ENABLED
– The user has permissions to use the APIs.
*
*
* -
*
* DISABLED
– The user does not have permissions to use any APIs.
*
*
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #apiAccess} will
* return {@link ApiAccess#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #apiAccessAsString}.
*
*
* @return The option to indicate whether the user can use the GetProgrammaticAccessCredentials
API to
* obtain credentials that can then be used to access other FinSpace Data API operations.
*
* -
*
* ENABLED
– The user has permissions to use the APIs.
*
*
* -
*
* DISABLED
– The user does not have permissions to use any APIs.
*
*
* @see ApiAccess
*/
public final String apiAccessAsString() {
return apiAccess;
}
/**
*
* The ARN identifier of an AWS user or role that is allowed to call the
* GetProgrammaticAccessCredentials
API to obtain a credentials token for a specific FinSpace user.
* This must be an IAM role within your FinSpace account.
*
*
* @return The ARN identifier of an AWS user or role that is allowed to call the
* GetProgrammaticAccessCredentials
API to obtain a credentials token for a specific FinSpace
* user. This must be an IAM role within your FinSpace account.
*/
public final String apiAccessPrincipalArn() {
return apiAccessPrincipalArn;
}
/**
*
* A token that ensures idempotency. This token expires in 10 minutes.
*
*
* @return A token that ensures idempotency. This token expires in 10 minutes.
*/
public final String clientToken() {
return clientToken;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + super.hashCode();
hashCode = 31 * hashCode + Objects.hashCode(userId());
hashCode = 31 * hashCode + Objects.hashCode(typeAsString());
hashCode = 31 * hashCode + Objects.hashCode(firstName());
hashCode = 31 * hashCode + Objects.hashCode(lastName());
hashCode = 31 * hashCode + Objects.hashCode(apiAccessAsString());
hashCode = 31 * hashCode + Objects.hashCode(apiAccessPrincipalArn());
hashCode = 31 * hashCode + Objects.hashCode(clientToken());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return super.equals(obj) && equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof UpdateUserRequest)) {
return false;
}
UpdateUserRequest other = (UpdateUserRequest) obj;
return Objects.equals(userId(), other.userId()) && Objects.equals(typeAsString(), other.typeAsString())
&& Objects.equals(firstName(), other.firstName()) && Objects.equals(lastName(), other.lastName())
&& Objects.equals(apiAccessAsString(), other.apiAccessAsString())
&& Objects.equals(apiAccessPrincipalArn(), other.apiAccessPrincipalArn())
&& Objects.equals(clientToken(), other.clientToken());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("UpdateUserRequest").add("UserId", userId()).add("Type", typeAsString())
.add("FirstName", firstName() == null ? null : "*** Sensitive Data Redacted ***")
.add("LastName", lastName() == null ? null : "*** Sensitive Data Redacted ***")
.add("ApiAccess", apiAccessAsString()).add("ApiAccessPrincipalArn", apiAccessPrincipalArn())
.add("ClientToken", clientToken()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "userId":
return Optional.ofNullable(clazz.cast(userId()));
case "type":
return Optional.ofNullable(clazz.cast(typeAsString()));
case "firstName":
return Optional.ofNullable(clazz.cast(firstName()));
case "lastName":
return Optional.ofNullable(clazz.cast(lastName()));
case "apiAccess":
return Optional.ofNullable(clazz.cast(apiAccessAsString()));
case "apiAccessPrincipalArn":
return Optional.ofNullable(clazz.cast(apiAccessPrincipalArn()));
case "clientToken":
return Optional.ofNullable(clazz.cast(clientToken()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
private static Function