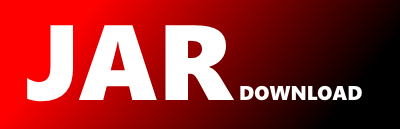
software.amazon.awssdk.services.finspacedata.model.GetDatasetResponse Maven / Gradle / Ivy
Show all versions of finspacedata Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.finspacedata.model;
import java.util.Arrays;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
* Response for the GetDataset operation
*/
@Generated("software.amazon.awssdk:codegen")
public final class GetDatasetResponse extends FinspaceDataResponse implements
ToCopyableBuilder {
private static final SdkField DATASET_ID_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("datasetId").getter(getter(GetDatasetResponse::datasetId)).setter(setter(Builder::datasetId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("datasetId").build()).build();
private static final SdkField DATASET_ARN_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("datasetArn").getter(getter(GetDatasetResponse::datasetArn)).setter(setter(Builder::datasetArn))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("datasetArn").build()).build();
private static final SdkField DATASET_TITLE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("datasetTitle").getter(getter(GetDatasetResponse::datasetTitle)).setter(setter(Builder::datasetTitle))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("datasetTitle").build()).build();
private static final SdkField KIND_FIELD = SdkField. builder(MarshallingType.STRING).memberName("kind")
.getter(getter(GetDatasetResponse::kindAsString)).setter(setter(Builder::kind))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("kind").build()).build();
private static final SdkField DATASET_DESCRIPTION_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("datasetDescription").getter(getter(GetDatasetResponse::datasetDescription))
.setter(setter(Builder::datasetDescription))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("datasetDescription").build())
.build();
private static final SdkField CREATE_TIME_FIELD = SdkField. builder(MarshallingType.LONG)
.memberName("createTime").getter(getter(GetDatasetResponse::createTime)).setter(setter(Builder::createTime))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("createTime").build()).build();
private static final SdkField LAST_MODIFIED_TIME_FIELD = SdkField. builder(MarshallingType.LONG)
.memberName("lastModifiedTime").getter(getter(GetDatasetResponse::lastModifiedTime))
.setter(setter(Builder::lastModifiedTime))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("lastModifiedTime").build()).build();
private static final SdkField SCHEMA_DEFINITION_FIELD = SdkField. builder(MarshallingType.SDK_POJO)
.memberName("schemaDefinition").getter(getter(GetDatasetResponse::schemaDefinition))
.setter(setter(Builder::schemaDefinition)).constructor(SchemaUnion::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("schemaDefinition").build()).build();
private static final SdkField ALIAS_FIELD = SdkField. builder(MarshallingType.STRING).memberName("alias")
.getter(getter(GetDatasetResponse::alias)).setter(setter(Builder::alias))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("alias").build()).build();
private static final SdkField STATUS_FIELD = SdkField. builder(MarshallingType.STRING).memberName("status")
.getter(getter(GetDatasetResponse::statusAsString)).setter(setter(Builder::status))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("status").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(DATASET_ID_FIELD,
DATASET_ARN_FIELD, DATASET_TITLE_FIELD, KIND_FIELD, DATASET_DESCRIPTION_FIELD, CREATE_TIME_FIELD,
LAST_MODIFIED_TIME_FIELD, SCHEMA_DEFINITION_FIELD, ALIAS_FIELD, STATUS_FIELD));
private final String datasetId;
private final String datasetArn;
private final String datasetTitle;
private final String kind;
private final String datasetDescription;
private final Long createTime;
private final Long lastModifiedTime;
private final SchemaUnion schemaDefinition;
private final String alias;
private final String status;
private GetDatasetResponse(BuilderImpl builder) {
super(builder);
this.datasetId = builder.datasetId;
this.datasetArn = builder.datasetArn;
this.datasetTitle = builder.datasetTitle;
this.kind = builder.kind;
this.datasetDescription = builder.datasetDescription;
this.createTime = builder.createTime;
this.lastModifiedTime = builder.lastModifiedTime;
this.schemaDefinition = builder.schemaDefinition;
this.alias = builder.alias;
this.status = builder.status;
}
/**
*
* The unique identifier for a Dataset.
*
*
* @return The unique identifier for a Dataset.
*/
public final String datasetId() {
return datasetId;
}
/**
*
* The ARN identifier of the Dataset.
*
*
* @return The ARN identifier of the Dataset.
*/
public final String datasetArn() {
return datasetArn;
}
/**
*
* Display title for a Dataset.
*
*
* @return Display title for a Dataset.
*/
public final String datasetTitle() {
return datasetTitle;
}
/**
*
* The format in which Dataset data is structured.
*
*
* -
*
* TABULAR
– Data is structured in a tabular format.
*
*
* -
*
* NON_TABULAR
– Data is structured in a non-tabular format.
*
*
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #kind} will return
* {@link DatasetKind#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #kindAsString}.
*
*
* @return The format in which Dataset data is structured.
*
* -
*
* TABULAR
– Data is structured in a tabular format.
*
*
* -
*
* NON_TABULAR
– Data is structured in a non-tabular format.
*
*
* @see DatasetKind
*/
public final DatasetKind kind() {
return DatasetKind.fromValue(kind);
}
/**
*
* The format in which Dataset data is structured.
*
*
* -
*
* TABULAR
– Data is structured in a tabular format.
*
*
* -
*
* NON_TABULAR
– Data is structured in a non-tabular format.
*
*
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #kind} will return
* {@link DatasetKind#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #kindAsString}.
*
*
* @return The format in which Dataset data is structured.
*
* -
*
* TABULAR
– Data is structured in a tabular format.
*
*
* -
*
* NON_TABULAR
– Data is structured in a non-tabular format.
*
*
* @see DatasetKind
*/
public final String kindAsString() {
return kind;
}
/**
*
* A description of the Dataset.
*
*
* @return A description of the Dataset.
*/
public final String datasetDescription() {
return datasetDescription;
}
/**
*
* The timestamp at which the Dataset was created in FinSpace. The value is determined as epoch time in
* milliseconds. For example, the value for Monday, November 1, 2021 12:00:00 PM UTC is specified as 1635768000000.
*
*
* @return The timestamp at which the Dataset was created in FinSpace. The value is determined as epoch time in
* milliseconds. For example, the value for Monday, November 1, 2021 12:00:00 PM UTC is specified as
* 1635768000000.
*/
public final Long createTime() {
return createTime;
}
/**
*
* The last time that the Dataset was modified. The value is determined as epoch time in milliseconds. For example,
* the value for Monday, November 1, 2021 12:00:00 PM UTC is specified as 1635768000000.
*
*
* @return The last time that the Dataset was modified. The value is determined as epoch time in milliseconds. For
* example, the value for Monday, November 1, 2021 12:00:00 PM UTC is specified as 1635768000000.
*/
public final Long lastModifiedTime() {
return lastModifiedTime;
}
/**
*
* Definition for a schema on a tabular Dataset.
*
*
* @return Definition for a schema on a tabular Dataset.
*/
public final SchemaUnion schemaDefinition() {
return schemaDefinition;
}
/**
*
* The unique resource identifier for a Dataset.
*
*
* @return The unique resource identifier for a Dataset.
*/
public final String alias() {
return alias;
}
/**
*
* Status of the Dataset creation.
*
*
* -
*
* PENDING
– Dataset is pending creation.
*
*
* -
*
* FAILED
– Dataset creation has failed.
*
*
* -
*
* SUCCESS
– Dataset creation has succeeded.
*
*
* -
*
* RUNNING
– Dataset creation is running.
*
*
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #status} will
* return {@link DatasetStatus#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #statusAsString}.
*
*
* @return Status of the Dataset creation.
*
* -
*
* PENDING
– Dataset is pending creation.
*
*
* -
*
* FAILED
– Dataset creation has failed.
*
*
* -
*
* SUCCESS
– Dataset creation has succeeded.
*
*
* -
*
* RUNNING
– Dataset creation is running.
*
*
* @see DatasetStatus
*/
public final DatasetStatus status() {
return DatasetStatus.fromValue(status);
}
/**
*
* Status of the Dataset creation.
*
*
* -
*
* PENDING
– Dataset is pending creation.
*
*
* -
*
* FAILED
– Dataset creation has failed.
*
*
* -
*
* SUCCESS
– Dataset creation has succeeded.
*
*
* -
*
* RUNNING
– Dataset creation is running.
*
*
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #status} will
* return {@link DatasetStatus#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #statusAsString}.
*
*
* @return Status of the Dataset creation.
*
* -
*
* PENDING
– Dataset is pending creation.
*
*
* -
*
* FAILED
– Dataset creation has failed.
*
*
* -
*
* SUCCESS
– Dataset creation has succeeded.
*
*
* -
*
* RUNNING
– Dataset creation is running.
*
*
* @see DatasetStatus
*/
public final String statusAsString() {
return status;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + super.hashCode();
hashCode = 31 * hashCode + Objects.hashCode(datasetId());
hashCode = 31 * hashCode + Objects.hashCode(datasetArn());
hashCode = 31 * hashCode + Objects.hashCode(datasetTitle());
hashCode = 31 * hashCode + Objects.hashCode(kindAsString());
hashCode = 31 * hashCode + Objects.hashCode(datasetDescription());
hashCode = 31 * hashCode + Objects.hashCode(createTime());
hashCode = 31 * hashCode + Objects.hashCode(lastModifiedTime());
hashCode = 31 * hashCode + Objects.hashCode(schemaDefinition());
hashCode = 31 * hashCode + Objects.hashCode(alias());
hashCode = 31 * hashCode + Objects.hashCode(statusAsString());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return super.equals(obj) && equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof GetDatasetResponse)) {
return false;
}
GetDatasetResponse other = (GetDatasetResponse) obj;
return Objects.equals(datasetId(), other.datasetId()) && Objects.equals(datasetArn(), other.datasetArn())
&& Objects.equals(datasetTitle(), other.datasetTitle()) && Objects.equals(kindAsString(), other.kindAsString())
&& Objects.equals(datasetDescription(), other.datasetDescription())
&& Objects.equals(createTime(), other.createTime())
&& Objects.equals(lastModifiedTime(), other.lastModifiedTime())
&& Objects.equals(schemaDefinition(), other.schemaDefinition()) && Objects.equals(alias(), other.alias())
&& Objects.equals(statusAsString(), other.statusAsString());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("GetDatasetResponse").add("DatasetId", datasetId()).add("DatasetArn", datasetArn())
.add("DatasetTitle", datasetTitle()).add("Kind", kindAsString()).add("DatasetDescription", datasetDescription())
.add("CreateTime", createTime()).add("LastModifiedTime", lastModifiedTime())
.add("SchemaDefinition", schemaDefinition()).add("Alias", alias()).add("Status", statusAsString()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "datasetId":
return Optional.ofNullable(clazz.cast(datasetId()));
case "datasetArn":
return Optional.ofNullable(clazz.cast(datasetArn()));
case "datasetTitle":
return Optional.ofNullable(clazz.cast(datasetTitle()));
case "kind":
return Optional.ofNullable(clazz.cast(kindAsString()));
case "datasetDescription":
return Optional.ofNullable(clazz.cast(datasetDescription()));
case "createTime":
return Optional.ofNullable(clazz.cast(createTime()));
case "lastModifiedTime":
return Optional.ofNullable(clazz.cast(lastModifiedTime()));
case "schemaDefinition":
return Optional.ofNullable(clazz.cast(schemaDefinition()));
case "alias":
return Optional.ofNullable(clazz.cast(alias()));
case "status":
return Optional.ofNullable(clazz.cast(statusAsString()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
private static Function