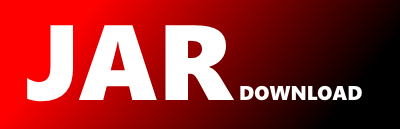
software.amazon.awssdk.services.finspacedata.FinspaceDataAsyncClient Maven / Gradle / Ivy
Show all versions of finspacedata Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.finspacedata;
import java.util.concurrent.CompletableFuture;
import java.util.function.Consumer;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.annotations.SdkPublicApi;
import software.amazon.awssdk.annotations.ThreadSafe;
import software.amazon.awssdk.core.SdkClient;
import software.amazon.awssdk.services.finspacedata.model.AssociateUserToPermissionGroupRequest;
import software.amazon.awssdk.services.finspacedata.model.AssociateUserToPermissionGroupResponse;
import software.amazon.awssdk.services.finspacedata.model.CreateChangesetRequest;
import software.amazon.awssdk.services.finspacedata.model.CreateChangesetResponse;
import software.amazon.awssdk.services.finspacedata.model.CreateDataViewRequest;
import software.amazon.awssdk.services.finspacedata.model.CreateDataViewResponse;
import software.amazon.awssdk.services.finspacedata.model.CreateDatasetRequest;
import software.amazon.awssdk.services.finspacedata.model.CreateDatasetResponse;
import software.amazon.awssdk.services.finspacedata.model.CreatePermissionGroupRequest;
import software.amazon.awssdk.services.finspacedata.model.CreatePermissionGroupResponse;
import software.amazon.awssdk.services.finspacedata.model.CreateUserRequest;
import software.amazon.awssdk.services.finspacedata.model.CreateUserResponse;
import software.amazon.awssdk.services.finspacedata.model.DeleteDatasetRequest;
import software.amazon.awssdk.services.finspacedata.model.DeleteDatasetResponse;
import software.amazon.awssdk.services.finspacedata.model.DeletePermissionGroupRequest;
import software.amazon.awssdk.services.finspacedata.model.DeletePermissionGroupResponse;
import software.amazon.awssdk.services.finspacedata.model.DisableUserRequest;
import software.amazon.awssdk.services.finspacedata.model.DisableUserResponse;
import software.amazon.awssdk.services.finspacedata.model.DisassociateUserFromPermissionGroupRequest;
import software.amazon.awssdk.services.finspacedata.model.DisassociateUserFromPermissionGroupResponse;
import software.amazon.awssdk.services.finspacedata.model.EnableUserRequest;
import software.amazon.awssdk.services.finspacedata.model.EnableUserResponse;
import software.amazon.awssdk.services.finspacedata.model.GetChangesetRequest;
import software.amazon.awssdk.services.finspacedata.model.GetChangesetResponse;
import software.amazon.awssdk.services.finspacedata.model.GetDataViewRequest;
import software.amazon.awssdk.services.finspacedata.model.GetDataViewResponse;
import software.amazon.awssdk.services.finspacedata.model.GetDatasetRequest;
import software.amazon.awssdk.services.finspacedata.model.GetDatasetResponse;
import software.amazon.awssdk.services.finspacedata.model.GetExternalDataViewAccessDetailsRequest;
import software.amazon.awssdk.services.finspacedata.model.GetExternalDataViewAccessDetailsResponse;
import software.amazon.awssdk.services.finspacedata.model.GetPermissionGroupRequest;
import software.amazon.awssdk.services.finspacedata.model.GetPermissionGroupResponse;
import software.amazon.awssdk.services.finspacedata.model.GetProgrammaticAccessCredentialsRequest;
import software.amazon.awssdk.services.finspacedata.model.GetProgrammaticAccessCredentialsResponse;
import software.amazon.awssdk.services.finspacedata.model.GetUserRequest;
import software.amazon.awssdk.services.finspacedata.model.GetUserResponse;
import software.amazon.awssdk.services.finspacedata.model.GetWorkingLocationRequest;
import software.amazon.awssdk.services.finspacedata.model.GetWorkingLocationResponse;
import software.amazon.awssdk.services.finspacedata.model.ListChangesetsRequest;
import software.amazon.awssdk.services.finspacedata.model.ListChangesetsResponse;
import software.amazon.awssdk.services.finspacedata.model.ListDataViewsRequest;
import software.amazon.awssdk.services.finspacedata.model.ListDataViewsResponse;
import software.amazon.awssdk.services.finspacedata.model.ListDatasetsRequest;
import software.amazon.awssdk.services.finspacedata.model.ListDatasetsResponse;
import software.amazon.awssdk.services.finspacedata.model.ListPermissionGroupsByUserRequest;
import software.amazon.awssdk.services.finspacedata.model.ListPermissionGroupsByUserResponse;
import software.amazon.awssdk.services.finspacedata.model.ListPermissionGroupsRequest;
import software.amazon.awssdk.services.finspacedata.model.ListPermissionGroupsResponse;
import software.amazon.awssdk.services.finspacedata.model.ListUsersByPermissionGroupRequest;
import software.amazon.awssdk.services.finspacedata.model.ListUsersByPermissionGroupResponse;
import software.amazon.awssdk.services.finspacedata.model.ListUsersRequest;
import software.amazon.awssdk.services.finspacedata.model.ListUsersResponse;
import software.amazon.awssdk.services.finspacedata.model.ResetUserPasswordRequest;
import software.amazon.awssdk.services.finspacedata.model.ResetUserPasswordResponse;
import software.amazon.awssdk.services.finspacedata.model.UpdateChangesetRequest;
import software.amazon.awssdk.services.finspacedata.model.UpdateChangesetResponse;
import software.amazon.awssdk.services.finspacedata.model.UpdateDatasetRequest;
import software.amazon.awssdk.services.finspacedata.model.UpdateDatasetResponse;
import software.amazon.awssdk.services.finspacedata.model.UpdatePermissionGroupRequest;
import software.amazon.awssdk.services.finspacedata.model.UpdatePermissionGroupResponse;
import software.amazon.awssdk.services.finspacedata.model.UpdateUserRequest;
import software.amazon.awssdk.services.finspacedata.model.UpdateUserResponse;
import software.amazon.awssdk.services.finspacedata.paginators.ListChangesetsPublisher;
import software.amazon.awssdk.services.finspacedata.paginators.ListDataViewsPublisher;
import software.amazon.awssdk.services.finspacedata.paginators.ListDatasetsPublisher;
import software.amazon.awssdk.services.finspacedata.paginators.ListPermissionGroupsPublisher;
import software.amazon.awssdk.services.finspacedata.paginators.ListUsersPublisher;
/**
* Service client for accessing FinSpace Data asynchronously. This can be created using the static {@link #builder()}
* method.
*
*
* The FinSpace APIs let you take actions inside the FinSpace.
*
*/
@Generated("software.amazon.awssdk:codegen")
@SdkPublicApi
@ThreadSafe
public interface FinspaceDataAsyncClient extends SdkClient {
String SERVICE_NAME = "finspace-api";
/**
* Value for looking up the service's metadata from the
* {@link software.amazon.awssdk.regions.ServiceMetadataProvider}.
*/
String SERVICE_METADATA_ID = "finspace-api";
/**
*
* Adds a user account to a permission group to grant permissions for actions a user can perform in FinSpace.
*
*
* @param associateUserToPermissionGroupRequest
* @return A Java Future containing the result of the AssociateUserToPermissionGroup operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InternalServerException The request processing has failed because of an unknown error, exception or
* failure.
* - ValidationException The input fails to satisfy the constraints specified by an AWS service.
* - ThrottlingException The request was denied due to request throttling.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ResourceNotFoundException One or more resources can't be found.
* - ConflictException The request conflicts with an existing resource.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - FinspaceDataException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample FinspaceDataAsyncClient.AssociateUserToPermissionGroup
* @see AWS API Documentation
*/
default CompletableFuture associateUserToPermissionGroup(
AssociateUserToPermissionGroupRequest associateUserToPermissionGroupRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Adds a user account to a permission group to grant permissions for actions a user can perform in FinSpace.
*
*
*
* This is a convenience which creates an instance of the {@link AssociateUserToPermissionGroupRequest.Builder}
* avoiding the need to create one manually via {@link AssociateUserToPermissionGroupRequest#builder()}
*
*
* @param associateUserToPermissionGroupRequest
* A {@link Consumer} that will call methods on {@link AssociateUserToPermissionGroupRequest.Builder} to
* create a request.
* @return A Java Future containing the result of the AssociateUserToPermissionGroup operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InternalServerException The request processing has failed because of an unknown error, exception or
* failure.
* - ValidationException The input fails to satisfy the constraints specified by an AWS service.
* - ThrottlingException The request was denied due to request throttling.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ResourceNotFoundException One or more resources can't be found.
* - ConflictException The request conflicts with an existing resource.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - FinspaceDataException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample FinspaceDataAsyncClient.AssociateUserToPermissionGroup
* @see AWS API Documentation
*/
default CompletableFuture associateUserToPermissionGroup(
Consumer associateUserToPermissionGroupRequest) {
return associateUserToPermissionGroup(AssociateUserToPermissionGroupRequest.builder()
.applyMutation(associateUserToPermissionGroupRequest).build());
}
/**
*
* Creates a new Changeset in a FinSpace Dataset.
*
*
* @param createChangesetRequest
* The request for a CreateChangeset operation.
* @return A Java Future containing the result of the CreateChangeset operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ResourceNotFoundException One or more resources can't be found.
* - InternalServerException The request processing has failed because of an unknown error, exception or
* failure.
* - ValidationException The input fails to satisfy the constraints specified by an AWS service.
* - ThrottlingException The request was denied due to request throttling.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - LimitExceededException A limit has exceeded.
* - ConflictException The request conflicts with an existing resource.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - FinspaceDataException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample FinspaceDataAsyncClient.CreateChangeset
* @see AWS API
* Documentation
*/
default CompletableFuture createChangeset(CreateChangesetRequest createChangesetRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Creates a new Changeset in a FinSpace Dataset.
*
*
*
* This is a convenience which creates an instance of the {@link CreateChangesetRequest.Builder} avoiding the need
* to create one manually via {@link CreateChangesetRequest#builder()}
*
*
* @param createChangesetRequest
* A {@link Consumer} that will call methods on {@link CreateChangesetRequest.Builder} to create a request.
* The request for a CreateChangeset operation.
* @return A Java Future containing the result of the CreateChangeset operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ResourceNotFoundException One or more resources can't be found.
* - InternalServerException The request processing has failed because of an unknown error, exception or
* failure.
* - ValidationException The input fails to satisfy the constraints specified by an AWS service.
* - ThrottlingException The request was denied due to request throttling.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - LimitExceededException A limit has exceeded.
* - ConflictException The request conflicts with an existing resource.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - FinspaceDataException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample FinspaceDataAsyncClient.CreateChangeset
* @see AWS API
* Documentation
*/
default CompletableFuture createChangeset(
Consumer createChangesetRequest) {
return createChangeset(CreateChangesetRequest.builder().applyMutation(createChangesetRequest).build());
}
/**
*
* Creates a Dataview for a Dataset.
*
*
* @param createDataViewRequest
* Request for creating a data view.
* @return A Java Future containing the result of the CreateDataView operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InternalServerException The request processing has failed because of an unknown error, exception or
* failure.
* - ThrottlingException The request was denied due to request throttling.
* - ValidationException The input fails to satisfy the constraints specified by an AWS service.
* - LimitExceededException A limit has exceeded.
* - ConflictException The request conflicts with an existing resource.
* - ResourceNotFoundException One or more resources can't be found.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - FinspaceDataException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample FinspaceDataAsyncClient.CreateDataView
* @see AWS API
* Documentation
*/
default CompletableFuture createDataView(CreateDataViewRequest createDataViewRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Creates a Dataview for a Dataset.
*
*
*
* This is a convenience which creates an instance of the {@link CreateDataViewRequest.Builder} avoiding the need to
* create one manually via {@link CreateDataViewRequest#builder()}
*
*
* @param createDataViewRequest
* A {@link Consumer} that will call methods on {@link CreateDataViewRequest.Builder} to create a request.
* Request for creating a data view.
* @return A Java Future containing the result of the CreateDataView operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InternalServerException The request processing has failed because of an unknown error, exception or
* failure.
* - ThrottlingException The request was denied due to request throttling.
* - ValidationException The input fails to satisfy the constraints specified by an AWS service.
* - LimitExceededException A limit has exceeded.
* - ConflictException The request conflicts with an existing resource.
* - ResourceNotFoundException One or more resources can't be found.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - FinspaceDataException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample FinspaceDataAsyncClient.CreateDataView
* @see AWS API
* Documentation
*/
default CompletableFuture createDataView(Consumer createDataViewRequest) {
return createDataView(CreateDataViewRequest.builder().applyMutation(createDataViewRequest).build());
}
/**
*
* Creates a new FinSpace Dataset.
*
*
* @param createDatasetRequest
* The request for a CreateDataset operation
* @return A Java Future containing the result of the CreateDataset operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InternalServerException The request processing has failed because of an unknown error, exception or
* failure.
* - ValidationException The input fails to satisfy the constraints specified by an AWS service.
* - ThrottlingException The request was denied due to request throttling.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - LimitExceededException A limit has exceeded.
* - ConflictException The request conflicts with an existing resource.
* - ResourceNotFoundException One or more resources can't be found.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - FinspaceDataException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample FinspaceDataAsyncClient.CreateDataset
* @see AWS API
* Documentation
*/
default CompletableFuture createDataset(CreateDatasetRequest createDatasetRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Creates a new FinSpace Dataset.
*
*
*
* This is a convenience which creates an instance of the {@link CreateDatasetRequest.Builder} avoiding the need to
* create one manually via {@link CreateDatasetRequest#builder()}
*
*
* @param createDatasetRequest
* A {@link Consumer} that will call methods on {@link CreateDatasetRequest.Builder} to create a request. The
* request for a CreateDataset operation
* @return A Java Future containing the result of the CreateDataset operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InternalServerException The request processing has failed because of an unknown error, exception or
* failure.
* - ValidationException The input fails to satisfy the constraints specified by an AWS service.
* - ThrottlingException The request was denied due to request throttling.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - LimitExceededException A limit has exceeded.
* - ConflictException The request conflicts with an existing resource.
* - ResourceNotFoundException One or more resources can't be found.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - FinspaceDataException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample FinspaceDataAsyncClient.CreateDataset
* @see AWS API
* Documentation
*/
default CompletableFuture createDataset(Consumer createDatasetRequest) {
return createDataset(CreateDatasetRequest.builder().applyMutation(createDatasetRequest).build());
}
/**
*
* Creates a group of permissions for various actions that a user can perform in FinSpace.
*
*
* @param createPermissionGroupRequest
* @return A Java Future containing the result of the CreatePermissionGroup operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InternalServerException The request processing has failed because of an unknown error, exception or
* failure.
* - ValidationException The input fails to satisfy the constraints specified by an AWS service.
* - ThrottlingException The request was denied due to request throttling.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - LimitExceededException A limit has exceeded.
* - ConflictException The request conflicts with an existing resource.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - FinspaceDataException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample FinspaceDataAsyncClient.CreatePermissionGroup
* @see AWS API Documentation
*/
default CompletableFuture createPermissionGroup(
CreatePermissionGroupRequest createPermissionGroupRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Creates a group of permissions for various actions that a user can perform in FinSpace.
*
*
*
* This is a convenience which creates an instance of the {@link CreatePermissionGroupRequest.Builder} avoiding the
* need to create one manually via {@link CreatePermissionGroupRequest#builder()}
*
*
* @param createPermissionGroupRequest
* A {@link Consumer} that will call methods on {@link CreatePermissionGroupRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the CreatePermissionGroup operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InternalServerException The request processing has failed because of an unknown error, exception or
* failure.
* - ValidationException The input fails to satisfy the constraints specified by an AWS service.
* - ThrottlingException The request was denied due to request throttling.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - LimitExceededException A limit has exceeded.
* - ConflictException The request conflicts with an existing resource.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - FinspaceDataException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample FinspaceDataAsyncClient.CreatePermissionGroup
* @see AWS API Documentation
*/
default CompletableFuture createPermissionGroup(
Consumer createPermissionGroupRequest) {
return createPermissionGroup(CreatePermissionGroupRequest.builder().applyMutation(createPermissionGroupRequest).build());
}
/**
*
* Creates a new user in FinSpace.
*
*
* @param createUserRequest
* @return A Java Future containing the result of the CreateUser operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InternalServerException The request processing has failed because of an unknown error, exception or
* failure.
* - ValidationException The input fails to satisfy the constraints specified by an AWS service.
* - ThrottlingException The request was denied due to request throttling.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - LimitExceededException A limit has exceeded.
* - ConflictException The request conflicts with an existing resource.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - FinspaceDataException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample FinspaceDataAsyncClient.CreateUser
* @see AWS API
* Documentation
*/
default CompletableFuture createUser(CreateUserRequest createUserRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Creates a new user in FinSpace.
*
*
*
* This is a convenience which creates an instance of the {@link CreateUserRequest.Builder} avoiding the need to
* create one manually via {@link CreateUserRequest#builder()}
*
*
* @param createUserRequest
* A {@link Consumer} that will call methods on {@link CreateUserRequest.Builder} to create a request.
* @return A Java Future containing the result of the CreateUser operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InternalServerException The request processing has failed because of an unknown error, exception or
* failure.
* - ValidationException The input fails to satisfy the constraints specified by an AWS service.
* - ThrottlingException The request was denied due to request throttling.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - LimitExceededException A limit has exceeded.
* - ConflictException The request conflicts with an existing resource.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - FinspaceDataException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample FinspaceDataAsyncClient.CreateUser
* @see AWS API
* Documentation
*/
default CompletableFuture createUser(Consumer createUserRequest) {
return createUser(CreateUserRequest.builder().applyMutation(createUserRequest).build());
}
/**
*
* Deletes a FinSpace Dataset.
*
*
* @param deleteDatasetRequest
* The request for a DeleteDataset operation.
* @return A Java Future containing the result of the DeleteDataset operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InternalServerException The request processing has failed because of an unknown error, exception or
* failure.
* - ValidationException The input fails to satisfy the constraints specified by an AWS service.
* - ThrottlingException The request was denied due to request throttling.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ResourceNotFoundException One or more resources can't be found.
* - LimitExceededException A limit has exceeded.
* - ConflictException The request conflicts with an existing resource.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - FinspaceDataException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample FinspaceDataAsyncClient.DeleteDataset
* @see AWS API
* Documentation
*/
default CompletableFuture deleteDataset(DeleteDatasetRequest deleteDatasetRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Deletes a FinSpace Dataset.
*
*
*
* This is a convenience which creates an instance of the {@link DeleteDatasetRequest.Builder} avoiding the need to
* create one manually via {@link DeleteDatasetRequest#builder()}
*
*
* @param deleteDatasetRequest
* A {@link Consumer} that will call methods on {@link DeleteDatasetRequest.Builder} to create a request. The
* request for a DeleteDataset operation.
* @return A Java Future containing the result of the DeleteDataset operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InternalServerException The request processing has failed because of an unknown error, exception or
* failure.
* - ValidationException The input fails to satisfy the constraints specified by an AWS service.
* - ThrottlingException The request was denied due to request throttling.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ResourceNotFoundException One or more resources can't be found.
* - LimitExceededException A limit has exceeded.
* - ConflictException The request conflicts with an existing resource.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - FinspaceDataException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample FinspaceDataAsyncClient.DeleteDataset
* @see AWS API
* Documentation
*/
default CompletableFuture deleteDataset(Consumer deleteDatasetRequest) {
return deleteDataset(DeleteDatasetRequest.builder().applyMutation(deleteDatasetRequest).build());
}
/**
*
* Deletes a permission group. This action is irreversible.
*
*
* @param deletePermissionGroupRequest
* @return A Java Future containing the result of the DeletePermissionGroup operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InternalServerException The request processing has failed because of an unknown error, exception or
* failure.
* - ValidationException The input fails to satisfy the constraints specified by an AWS service.
* - ThrottlingException The request was denied due to request throttling.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ResourceNotFoundException One or more resources can't be found.
* - LimitExceededException A limit has exceeded.
* - ConflictException The request conflicts with an existing resource.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - FinspaceDataException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample FinspaceDataAsyncClient.DeletePermissionGroup
* @see AWS API Documentation
*/
default CompletableFuture deletePermissionGroup(
DeletePermissionGroupRequest deletePermissionGroupRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Deletes a permission group. This action is irreversible.
*
*
*
* This is a convenience which creates an instance of the {@link DeletePermissionGroupRequest.Builder} avoiding the
* need to create one manually via {@link DeletePermissionGroupRequest#builder()}
*
*
* @param deletePermissionGroupRequest
* A {@link Consumer} that will call methods on {@link DeletePermissionGroupRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the DeletePermissionGroup operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InternalServerException The request processing has failed because of an unknown error, exception or
* failure.
* - ValidationException The input fails to satisfy the constraints specified by an AWS service.
* - ThrottlingException The request was denied due to request throttling.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ResourceNotFoundException One or more resources can't be found.
* - LimitExceededException A limit has exceeded.
* - ConflictException The request conflicts with an existing resource.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - FinspaceDataException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample FinspaceDataAsyncClient.DeletePermissionGroup
* @see AWS API Documentation
*/
default CompletableFuture deletePermissionGroup(
Consumer deletePermissionGroupRequest) {
return deletePermissionGroup(DeletePermissionGroupRequest.builder().applyMutation(deletePermissionGroupRequest).build());
}
/**
*
* Denies access to the FinSpace web application and API for the specified user.
*
*
* @param disableUserRequest
* @return A Java Future containing the result of the DisableUser operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InternalServerException The request processing has failed because of an unknown error, exception or
* failure.
* - ValidationException The input fails to satisfy the constraints specified by an AWS service.
* - ThrottlingException The request was denied due to request throttling.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ResourceNotFoundException One or more resources can't be found.
* - ConflictException The request conflicts with an existing resource.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - FinspaceDataException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample FinspaceDataAsyncClient.DisableUser
* @see AWS API
* Documentation
*/
default CompletableFuture disableUser(DisableUserRequest disableUserRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Denies access to the FinSpace web application and API for the specified user.
*
*
*
* This is a convenience which creates an instance of the {@link DisableUserRequest.Builder} avoiding the need to
* create one manually via {@link DisableUserRequest#builder()}
*
*
* @param disableUserRequest
* A {@link Consumer} that will call methods on {@link DisableUserRequest.Builder} to create a request.
* @return A Java Future containing the result of the DisableUser operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InternalServerException The request processing has failed because of an unknown error, exception or
* failure.
* - ValidationException The input fails to satisfy the constraints specified by an AWS service.
* - ThrottlingException The request was denied due to request throttling.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ResourceNotFoundException One or more resources can't be found.
* - ConflictException The request conflicts with an existing resource.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - FinspaceDataException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample FinspaceDataAsyncClient.DisableUser
* @see AWS API
* Documentation
*/
default CompletableFuture disableUser(Consumer disableUserRequest) {
return disableUser(DisableUserRequest.builder().applyMutation(disableUserRequest).build());
}
/**
*
* Removes a user account from a permission group.
*
*
* @param disassociateUserFromPermissionGroupRequest
* @return A Java Future containing the result of the DisassociateUserFromPermissionGroup operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InternalServerException The request processing has failed because of an unknown error, exception or
* failure.
* - ValidationException The input fails to satisfy the constraints specified by an AWS service.
* - ThrottlingException The request was denied due to request throttling.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ResourceNotFoundException One or more resources can't be found.
* - ConflictException The request conflicts with an existing resource.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - FinspaceDataException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample FinspaceDataAsyncClient.DisassociateUserFromPermissionGroup
* @see AWS API Documentation
*/
default CompletableFuture disassociateUserFromPermissionGroup(
DisassociateUserFromPermissionGroupRequest disassociateUserFromPermissionGroupRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Removes a user account from a permission group.
*
*
*
* This is a convenience which creates an instance of the {@link DisassociateUserFromPermissionGroupRequest.Builder}
* avoiding the need to create one manually via {@link DisassociateUserFromPermissionGroupRequest#builder()}
*
*
* @param disassociateUserFromPermissionGroupRequest
* A {@link Consumer} that will call methods on {@link DisassociateUserFromPermissionGroupRequest.Builder} to
* create a request.
* @return A Java Future containing the result of the DisassociateUserFromPermissionGroup operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InternalServerException The request processing has failed because of an unknown error, exception or
* failure.
* - ValidationException The input fails to satisfy the constraints specified by an AWS service.
* - ThrottlingException The request was denied due to request throttling.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ResourceNotFoundException One or more resources can't be found.
* - ConflictException The request conflicts with an existing resource.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - FinspaceDataException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample FinspaceDataAsyncClient.DisassociateUserFromPermissionGroup
* @see AWS API Documentation
*/
default CompletableFuture disassociateUserFromPermissionGroup(
Consumer disassociateUserFromPermissionGroupRequest) {
return disassociateUserFromPermissionGroup(DisassociateUserFromPermissionGroupRequest.builder()
.applyMutation(disassociateUserFromPermissionGroupRequest).build());
}
/**
*
* Allows the specified user to access the FinSpace web application and API.
*
*
* @param enableUserRequest
* @return A Java Future containing the result of the EnableUser operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InternalServerException The request processing has failed because of an unknown error, exception or
* failure.
* - ValidationException The input fails to satisfy the constraints specified by an AWS service.
* - ThrottlingException The request was denied due to request throttling.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ResourceNotFoundException One or more resources can't be found.
* - LimitExceededException A limit has exceeded.
* - ConflictException The request conflicts with an existing resource.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - FinspaceDataException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample FinspaceDataAsyncClient.EnableUser
* @see AWS API
* Documentation
*/
default CompletableFuture enableUser(EnableUserRequest enableUserRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Allows the specified user to access the FinSpace web application and API.
*
*
*
* This is a convenience which creates an instance of the {@link EnableUserRequest.Builder} avoiding the need to
* create one manually via {@link EnableUserRequest#builder()}
*
*
* @param enableUserRequest
* A {@link Consumer} that will call methods on {@link EnableUserRequest.Builder} to create a request.
* @return A Java Future containing the result of the EnableUser operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InternalServerException The request processing has failed because of an unknown error, exception or
* failure.
* - ValidationException The input fails to satisfy the constraints specified by an AWS service.
* - ThrottlingException The request was denied due to request throttling.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ResourceNotFoundException One or more resources can't be found.
* - LimitExceededException A limit has exceeded.
* - ConflictException The request conflicts with an existing resource.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - FinspaceDataException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample FinspaceDataAsyncClient.EnableUser
* @see AWS API
* Documentation
*/
default CompletableFuture enableUser(Consumer enableUserRequest) {
return enableUser(EnableUserRequest.builder().applyMutation(enableUserRequest).build());
}
/**
*
* Get information about a Changeset.
*
*
* @param getChangesetRequest
* Request to describe a changeset.
* @return A Java Future containing the result of the GetChangeset operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ResourceNotFoundException One or more resources can't be found.
* - InternalServerException The request processing has failed because of an unknown error, exception or
* failure.
* - ValidationException The input fails to satisfy the constraints specified by an AWS service.
* - ThrottlingException The request was denied due to request throttling.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ConflictException The request conflicts with an existing resource.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - FinspaceDataException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample FinspaceDataAsyncClient.GetChangeset
* @see AWS API
* Documentation
*/
default CompletableFuture getChangeset(GetChangesetRequest getChangesetRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Get information about a Changeset.
*
*
*
* This is a convenience which creates an instance of the {@link GetChangesetRequest.Builder} avoiding the need to
* create one manually via {@link GetChangesetRequest#builder()}
*
*
* @param getChangesetRequest
* A {@link Consumer} that will call methods on {@link GetChangesetRequest.Builder} to create a request.
* Request to describe a changeset.
* @return A Java Future containing the result of the GetChangeset operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ResourceNotFoundException One or more resources can't be found.
* - InternalServerException The request processing has failed because of an unknown error, exception or
* failure.
* - ValidationException The input fails to satisfy the constraints specified by an AWS service.
* - ThrottlingException The request was denied due to request throttling.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ConflictException The request conflicts with an existing resource.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - FinspaceDataException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample FinspaceDataAsyncClient.GetChangeset
* @see AWS API
* Documentation
*/
default CompletableFuture getChangeset(Consumer getChangesetRequest) {
return getChangeset(GetChangesetRequest.builder().applyMutation(getChangesetRequest).build());
}
/**
*
* Gets information about a Dataview.
*
*
* @param getDataViewRequest
* Request for retrieving a data view detail. Grouped / accessible within a dataset by its dataset id.
* @return A Java Future containing the result of the GetDataView operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ResourceNotFoundException One or more resources can't be found.
* - InternalServerException The request processing has failed because of an unknown error, exception or
* failure.
* - ThrottlingException The request was denied due to request throttling.
* - ValidationException The input fails to satisfy the constraints specified by an AWS service.
* - ConflictException The request conflicts with an existing resource.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - FinspaceDataException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample FinspaceDataAsyncClient.GetDataView
* @see AWS API
* Documentation
*/
default CompletableFuture getDataView(GetDataViewRequest getDataViewRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Gets information about a Dataview.
*
*
*
* This is a convenience which creates an instance of the {@link GetDataViewRequest.Builder} avoiding the need to
* create one manually via {@link GetDataViewRequest#builder()}
*
*
* @param getDataViewRequest
* A {@link Consumer} that will call methods on {@link GetDataViewRequest.Builder} to create a request.
* Request for retrieving a data view detail. Grouped / accessible within a dataset by its dataset id.
* @return A Java Future containing the result of the GetDataView operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ResourceNotFoundException One or more resources can't be found.
* - InternalServerException The request processing has failed because of an unknown error, exception or
* failure.
* - ThrottlingException The request was denied due to request throttling.
* - ValidationException The input fails to satisfy the constraints specified by an AWS service.
* - ConflictException The request conflicts with an existing resource.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - FinspaceDataException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample FinspaceDataAsyncClient.GetDataView
* @see AWS API
* Documentation
*/
default CompletableFuture getDataView(Consumer getDataViewRequest) {
return getDataView(GetDataViewRequest.builder().applyMutation(getDataViewRequest).build());
}
/**
*
* Returns information about a Dataset.
*
*
* @param getDatasetRequest
* Request for the GetDataset operation.
* @return A Java Future containing the result of the GetDataset operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InternalServerException The request processing has failed because of an unknown error, exception or
* failure.
* - ValidationException The input fails to satisfy the constraints specified by an AWS service.
* - ThrottlingException The request was denied due to request throttling.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ResourceNotFoundException One or more resources can't be found.
* - ConflictException The request conflicts with an existing resource.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - FinspaceDataException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample FinspaceDataAsyncClient.GetDataset
* @see AWS API
* Documentation
*/
default CompletableFuture getDataset(GetDatasetRequest getDatasetRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Returns information about a Dataset.
*
*
*
* This is a convenience which creates an instance of the {@link GetDatasetRequest.Builder} avoiding the need to
* create one manually via {@link GetDatasetRequest#builder()}
*
*
* @param getDatasetRequest
* A {@link Consumer} that will call methods on {@link GetDatasetRequest.Builder} to create a request.
* Request for the GetDataset operation.
* @return A Java Future containing the result of the GetDataset operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InternalServerException The request processing has failed because of an unknown error, exception or
* failure.
* - ValidationException The input fails to satisfy the constraints specified by an AWS service.
* - ThrottlingException The request was denied due to request throttling.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ResourceNotFoundException One or more resources can't be found.
* - ConflictException The request conflicts with an existing resource.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - FinspaceDataException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample FinspaceDataAsyncClient.GetDataset
* @see AWS API
* Documentation
*/
default CompletableFuture getDataset(Consumer getDatasetRequest) {
return getDataset(GetDatasetRequest.builder().applyMutation(getDatasetRequest).build());
}
/**
*
* Returns the credentials to access the external Dataview from an S3 location. To call this API:
*
*
* -
*
* You must retrieve the programmatic credentials.
*
*
* -
*
* You must be a member of a FinSpace user group, where the dataset that you want to access has
* Read Dataset Data
permissions.
*
*
*
*
* @param getExternalDataViewAccessDetailsRequest
* @return A Java Future containing the result of the GetExternalDataViewAccessDetails operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ResourceNotFoundException One or more resources can't be found.
* - InternalServerException The request processing has failed because of an unknown error, exception or
* failure.
* - ThrottlingException The request was denied due to request throttling.
* - ValidationException The input fails to satisfy the constraints specified by an AWS service.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - FinspaceDataException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample FinspaceDataAsyncClient.GetExternalDataViewAccessDetails
* @see AWS API Documentation
*/
default CompletableFuture getExternalDataViewAccessDetails(
GetExternalDataViewAccessDetailsRequest getExternalDataViewAccessDetailsRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Returns the credentials to access the external Dataview from an S3 location. To call this API:
*
*
* -
*
* You must retrieve the programmatic credentials.
*
*
* -
*
* You must be a member of a FinSpace user group, where the dataset that you want to access has
* Read Dataset Data
permissions.
*
*
*
*
*
* This is a convenience which creates an instance of the {@link GetExternalDataViewAccessDetailsRequest.Builder}
* avoiding the need to create one manually via {@link GetExternalDataViewAccessDetailsRequest#builder()}
*
*
* @param getExternalDataViewAccessDetailsRequest
* A {@link Consumer} that will call methods on {@link GetExternalDataViewAccessDetailsRequest.Builder} to
* create a request.
* @return A Java Future containing the result of the GetExternalDataViewAccessDetails operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ResourceNotFoundException One or more resources can't be found.
* - InternalServerException The request processing has failed because of an unknown error, exception or
* failure.
* - ThrottlingException The request was denied due to request throttling.
* - ValidationException The input fails to satisfy the constraints specified by an AWS service.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - FinspaceDataException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample FinspaceDataAsyncClient.GetExternalDataViewAccessDetails
* @see AWS API Documentation
*/
default CompletableFuture getExternalDataViewAccessDetails(
Consumer getExternalDataViewAccessDetailsRequest) {
return getExternalDataViewAccessDetails(GetExternalDataViewAccessDetailsRequest.builder()
.applyMutation(getExternalDataViewAccessDetailsRequest).build());
}
/**
*
* Retrieves the details of a specific permission group.
*
*
* @param getPermissionGroupRequest
* @return A Java Future containing the result of the GetPermissionGroup operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InternalServerException The request processing has failed because of an unknown error, exception or
* failure.
* - ValidationException The input fails to satisfy the constraints specified by an AWS service.
* - ThrottlingException The request was denied due to request throttling.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ResourceNotFoundException One or more resources can't be found.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - FinspaceDataException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample FinspaceDataAsyncClient.GetPermissionGroup
* @see AWS
* API Documentation
*/
default CompletableFuture getPermissionGroup(GetPermissionGroupRequest getPermissionGroupRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Retrieves the details of a specific permission group.
*
*
*
* This is a convenience which creates an instance of the {@link GetPermissionGroupRequest.Builder} avoiding the
* need to create one manually via {@link GetPermissionGroupRequest#builder()}
*
*
* @param getPermissionGroupRequest
* A {@link Consumer} that will call methods on {@link GetPermissionGroupRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the GetPermissionGroup operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InternalServerException The request processing has failed because of an unknown error, exception or
* failure.
* - ValidationException The input fails to satisfy the constraints specified by an AWS service.
* - ThrottlingException The request was denied due to request throttling.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ResourceNotFoundException One or more resources can't be found.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - FinspaceDataException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample FinspaceDataAsyncClient.GetPermissionGroup
* @see AWS
* API Documentation
*/
default CompletableFuture getPermissionGroup(
Consumer getPermissionGroupRequest) {
return getPermissionGroup(GetPermissionGroupRequest.builder().applyMutation(getPermissionGroupRequest).build());
}
/**
*
* Request programmatic credentials to use with FinSpace SDK.
*
*
* @param getProgrammaticAccessCredentialsRequest
* Request for GetProgrammaticAccessCredentials operation
* @return A Java Future containing the result of the GetProgrammaticAccessCredentials operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InternalServerException The request processing has failed because of an unknown error, exception or
* failure.
* - ValidationException The input fails to satisfy the constraints specified by an AWS service.
* - ThrottlingException The request was denied due to request throttling.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - FinspaceDataException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample FinspaceDataAsyncClient.GetProgrammaticAccessCredentials
* @see AWS API Documentation
*/
default CompletableFuture getProgrammaticAccessCredentials(
GetProgrammaticAccessCredentialsRequest getProgrammaticAccessCredentialsRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Request programmatic credentials to use with FinSpace SDK.
*
*
*
* This is a convenience which creates an instance of the {@link GetProgrammaticAccessCredentialsRequest.Builder}
* avoiding the need to create one manually via {@link GetProgrammaticAccessCredentialsRequest#builder()}
*
*
* @param getProgrammaticAccessCredentialsRequest
* A {@link Consumer} that will call methods on {@link GetProgrammaticAccessCredentialsRequest.Builder} to
* create a request. Request for GetProgrammaticAccessCredentials operation
* @return A Java Future containing the result of the GetProgrammaticAccessCredentials operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InternalServerException The request processing has failed because of an unknown error, exception or
* failure.
* - ValidationException The input fails to satisfy the constraints specified by an AWS service.
* - ThrottlingException The request was denied due to request throttling.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - FinspaceDataException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample FinspaceDataAsyncClient.GetProgrammaticAccessCredentials
* @see AWS API Documentation
*/
default CompletableFuture getProgrammaticAccessCredentials(
Consumer getProgrammaticAccessCredentialsRequest) {
return getProgrammaticAccessCredentials(GetProgrammaticAccessCredentialsRequest.builder()
.applyMutation(getProgrammaticAccessCredentialsRequest).build());
}
/**
*
* Retrieves details for a specific user.
*
*
* @param getUserRequest
* @return A Java Future containing the result of the GetUser operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InternalServerException The request processing has failed because of an unknown error, exception or
* failure.
* - ValidationException The input fails to satisfy the constraints specified by an AWS service.
* - ThrottlingException The request was denied due to request throttling.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ResourceNotFoundException One or more resources can't be found.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - FinspaceDataException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample FinspaceDataAsyncClient.GetUser
* @see AWS API
* Documentation
*/
default CompletableFuture getUser(GetUserRequest getUserRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Retrieves details for a specific user.
*
*
*
* This is a convenience which creates an instance of the {@link GetUserRequest.Builder} avoiding the need to create
* one manually via {@link GetUserRequest#builder()}
*
*
* @param getUserRequest
* A {@link Consumer} that will call methods on {@link GetUserRequest.Builder} to create a request.
* @return A Java Future containing the result of the GetUser operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InternalServerException The request processing has failed because of an unknown error, exception or
* failure.
* - ValidationException The input fails to satisfy the constraints specified by an AWS service.
* - ThrottlingException The request was denied due to request throttling.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ResourceNotFoundException One or more resources can't be found.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - FinspaceDataException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample FinspaceDataAsyncClient.GetUser
* @see AWS API
* Documentation
*/
default CompletableFuture getUser(Consumer getUserRequest) {
return getUser(GetUserRequest.builder().applyMutation(getUserRequest).build());
}
/**
*
* A temporary Amazon S3 location, where you can copy your files from a source location to stage or use as a scratch
* space in FinSpace notebook.
*
*
* @param getWorkingLocationRequest
* @return A Java Future containing the result of the GetWorkingLocation operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InternalServerException The request processing has failed because of an unknown error, exception or
* failure.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ThrottlingException The request was denied due to request throttling.
* - ValidationException The input fails to satisfy the constraints specified by an AWS service.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - FinspaceDataException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample FinspaceDataAsyncClient.GetWorkingLocation
* @see AWS
* API Documentation
*/
default CompletableFuture getWorkingLocation(GetWorkingLocationRequest getWorkingLocationRequest) {
throw new UnsupportedOperationException();
}
/**
*
* A temporary Amazon S3 location, where you can copy your files from a source location to stage or use as a scratch
* space in FinSpace notebook.
*
*
*
* This is a convenience which creates an instance of the {@link GetWorkingLocationRequest.Builder} avoiding the
* need to create one manually via {@link GetWorkingLocationRequest#builder()}
*
*
* @param getWorkingLocationRequest
* A {@link Consumer} that will call methods on {@link GetWorkingLocationRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the GetWorkingLocation operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InternalServerException The request processing has failed because of an unknown error, exception or
* failure.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ThrottlingException The request was denied due to request throttling.
* - ValidationException The input fails to satisfy the constraints specified by an AWS service.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - FinspaceDataException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample FinspaceDataAsyncClient.GetWorkingLocation
* @see AWS
* API Documentation
*/
default CompletableFuture getWorkingLocation(
Consumer getWorkingLocationRequest) {
return getWorkingLocation(GetWorkingLocationRequest.builder().applyMutation(getWorkingLocationRequest).build());
}
/**
*
* Lists the FinSpace Changesets for a Dataset.
*
*
* @param listChangesetsRequest
* Request to ListChangesetsRequest. It exposes minimal query filters.
* @return A Java Future containing the result of the ListChangesets operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ResourceNotFoundException One or more resources can't be found.
* - InternalServerException The request processing has failed because of an unknown error, exception or
* failure.
* - ValidationException The input fails to satisfy the constraints specified by an AWS service.
* - ThrottlingException The request was denied due to request throttling.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ConflictException The request conflicts with an existing resource.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - FinspaceDataException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample FinspaceDataAsyncClient.ListChangesets
* @see AWS API
* Documentation
*/
default CompletableFuture listChangesets(ListChangesetsRequest listChangesetsRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Lists the FinSpace Changesets for a Dataset.
*
*
*
* This is a convenience which creates an instance of the {@link ListChangesetsRequest.Builder} avoiding the need to
* create one manually via {@link ListChangesetsRequest#builder()}
*
*
* @param listChangesetsRequest
* A {@link Consumer} that will call methods on {@link ListChangesetsRequest.Builder} to create a request.
* Request to ListChangesetsRequest. It exposes minimal query filters.
* @return A Java Future containing the result of the ListChangesets operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ResourceNotFoundException One or more resources can't be found.
* - InternalServerException The request processing has failed because of an unknown error, exception or
* failure.
* - ValidationException The input fails to satisfy the constraints specified by an AWS service.
* - ThrottlingException The request was denied due to request throttling.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ConflictException The request conflicts with an existing resource.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - FinspaceDataException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample FinspaceDataAsyncClient.ListChangesets
* @see AWS API
* Documentation
*/
default CompletableFuture listChangesets(Consumer listChangesetsRequest) {
return listChangesets(ListChangesetsRequest.builder().applyMutation(listChangesetsRequest).build());
}
/**
*
* Lists the FinSpace Changesets for a Dataset.
*
*
*
* This is a variant of
* {@link #listChangesets(software.amazon.awssdk.services.finspacedata.model.ListChangesetsRequest)} operation. The
* return type is a custom publisher that can be subscribed to request a stream of response pages. SDK will
* internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.finspacedata.paginators.ListChangesetsPublisher publisher = client.listChangesetsPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.finspacedata.paginators.ListChangesetsPublisher publisher = client.listChangesetsPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.finspacedata.model.ListChangesetsResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of maxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listChangesets(software.amazon.awssdk.services.finspacedata.model.ListChangesetsRequest)} operation.
*
*
* @param listChangesetsRequest
* Request to ListChangesetsRequest. It exposes minimal query filters.
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ResourceNotFoundException One or more resources can't be found.
* - InternalServerException The request processing has failed because of an unknown error, exception or
* failure.
* - ValidationException The input fails to satisfy the constraints specified by an AWS service.
* - ThrottlingException The request was denied due to request throttling.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ConflictException The request conflicts with an existing resource.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - FinspaceDataException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample FinspaceDataAsyncClient.ListChangesets
* @see AWS API
* Documentation
*/
default ListChangesetsPublisher listChangesetsPaginator(ListChangesetsRequest listChangesetsRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Lists the FinSpace Changesets for a Dataset.
*
*
*
* This is a variant of
* {@link #listChangesets(software.amazon.awssdk.services.finspacedata.model.ListChangesetsRequest)} operation. The
* return type is a custom publisher that can be subscribed to request a stream of response pages. SDK will
* internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.finspacedata.paginators.ListChangesetsPublisher publisher = client.listChangesetsPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.finspacedata.paginators.ListChangesetsPublisher publisher = client.listChangesetsPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.finspacedata.model.ListChangesetsResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of maxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listChangesets(software.amazon.awssdk.services.finspacedata.model.ListChangesetsRequest)} operation.
*
*
* This is a convenience which creates an instance of the {@link ListChangesetsRequest.Builder} avoiding the need to
* create one manually via {@link ListChangesetsRequest#builder()}
*
*
* @param listChangesetsRequest
* A {@link Consumer} that will call methods on {@link ListChangesetsRequest.Builder} to create a request.
* Request to ListChangesetsRequest. It exposes minimal query filters.
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ResourceNotFoundException One or more resources can't be found.
* - InternalServerException The request processing has failed because of an unknown error, exception or
* failure.
* - ValidationException The input fails to satisfy the constraints specified by an AWS service.
* - ThrottlingException The request was denied due to request throttling.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ConflictException The request conflicts with an existing resource.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - FinspaceDataException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample FinspaceDataAsyncClient.ListChangesets
* @see AWS API
* Documentation
*/
default ListChangesetsPublisher listChangesetsPaginator(Consumer listChangesetsRequest) {
return listChangesetsPaginator(ListChangesetsRequest.builder().applyMutation(listChangesetsRequest).build());
}
/**
*
* Lists all available Dataviews for a Dataset.
*
*
* @param listDataViewsRequest
* Request for a list data views.
* @return A Java Future containing the result of the ListDataViews operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ResourceNotFoundException One or more resources can't be found.
* - InternalServerException The request processing has failed because of an unknown error, exception or
* failure.
* - ThrottlingException The request was denied due to request throttling.
* - ValidationException The input fails to satisfy the constraints specified by an AWS service.
* - ConflictException The request conflicts with an existing resource.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - FinspaceDataException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample FinspaceDataAsyncClient.ListDataViews
* @see AWS API
* Documentation
*/
default CompletableFuture listDataViews(ListDataViewsRequest listDataViewsRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Lists all available Dataviews for a Dataset.
*
*
*
* This is a convenience which creates an instance of the {@link ListDataViewsRequest.Builder} avoiding the need to
* create one manually via {@link ListDataViewsRequest#builder()}
*
*
* @param listDataViewsRequest
* A {@link Consumer} that will call methods on {@link ListDataViewsRequest.Builder} to create a request.
* Request for a list data views.
* @return A Java Future containing the result of the ListDataViews operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ResourceNotFoundException One or more resources can't be found.
* - InternalServerException The request processing has failed because of an unknown error, exception or
* failure.
* - ThrottlingException The request was denied due to request throttling.
* - ValidationException The input fails to satisfy the constraints specified by an AWS service.
* - ConflictException The request conflicts with an existing resource.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - FinspaceDataException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample FinspaceDataAsyncClient.ListDataViews
* @see AWS API
* Documentation
*/
default CompletableFuture listDataViews(Consumer listDataViewsRequest) {
return listDataViews(ListDataViewsRequest.builder().applyMutation(listDataViewsRequest).build());
}
/**
*
* Lists all available Dataviews for a Dataset.
*
*
*
* This is a variant of
* {@link #listDataViews(software.amazon.awssdk.services.finspacedata.model.ListDataViewsRequest)} operation. The
* return type is a custom publisher that can be subscribed to request a stream of response pages. SDK will
* internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.finspacedata.paginators.ListDataViewsPublisher publisher = client.listDataViewsPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.finspacedata.paginators.ListDataViewsPublisher publisher = client.listDataViewsPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.finspacedata.model.ListDataViewsResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of maxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listDataViews(software.amazon.awssdk.services.finspacedata.model.ListDataViewsRequest)} operation.
*
*
* @param listDataViewsRequest
* Request for a list data views.
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ResourceNotFoundException One or more resources can't be found.
* - InternalServerException The request processing has failed because of an unknown error, exception or
* failure.
* - ThrottlingException The request was denied due to request throttling.
* - ValidationException The input fails to satisfy the constraints specified by an AWS service.
* - ConflictException The request conflicts with an existing resource.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - FinspaceDataException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample FinspaceDataAsyncClient.ListDataViews
* @see AWS API
* Documentation
*/
default ListDataViewsPublisher listDataViewsPaginator(ListDataViewsRequest listDataViewsRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Lists all available Dataviews for a Dataset.
*
*
*
* This is a variant of
* {@link #listDataViews(software.amazon.awssdk.services.finspacedata.model.ListDataViewsRequest)} operation. The
* return type is a custom publisher that can be subscribed to request a stream of response pages. SDK will
* internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.finspacedata.paginators.ListDataViewsPublisher publisher = client.listDataViewsPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.finspacedata.paginators.ListDataViewsPublisher publisher = client.listDataViewsPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.finspacedata.model.ListDataViewsResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of maxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listDataViews(software.amazon.awssdk.services.finspacedata.model.ListDataViewsRequest)} operation.
*
*
* This is a convenience which creates an instance of the {@link ListDataViewsRequest.Builder} avoiding the need to
* create one manually via {@link ListDataViewsRequest#builder()}
*
*
* @param listDataViewsRequest
* A {@link Consumer} that will call methods on {@link ListDataViewsRequest.Builder} to create a request.
* Request for a list data views.
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ResourceNotFoundException One or more resources can't be found.
* - InternalServerException The request processing has failed because of an unknown error, exception or
* failure.
* - ThrottlingException The request was denied due to request throttling.
* - ValidationException The input fails to satisfy the constraints specified by an AWS service.
* - ConflictException The request conflicts with an existing resource.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - FinspaceDataException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample FinspaceDataAsyncClient.ListDataViews
* @see AWS API
* Documentation
*/
default ListDataViewsPublisher listDataViewsPaginator(Consumer listDataViewsRequest) {
return listDataViewsPaginator(ListDataViewsRequest.builder().applyMutation(listDataViewsRequest).build());
}
/**
*
* Lists all of the active Datasets that a user has access to.
*
*
* @param listDatasetsRequest
* Request for the ListDatasets operation.
* @return A Java Future containing the result of the ListDatasets operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InternalServerException The request processing has failed because of an unknown error, exception or
* failure.
* - ThrottlingException The request was denied due to request throttling.
* - ValidationException The input fails to satisfy the constraints specified by an AWS service.
* - ConflictException The request conflicts with an existing resource.
* - ResourceNotFoundException One or more resources can't be found.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - FinspaceDataException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample FinspaceDataAsyncClient.ListDatasets
* @see AWS API
* Documentation
*/
default CompletableFuture listDatasets(ListDatasetsRequest listDatasetsRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Lists all of the active Datasets that a user has access to.
*
*
*
* This is a convenience which creates an instance of the {@link ListDatasetsRequest.Builder} avoiding the need to
* create one manually via {@link ListDatasetsRequest#builder()}
*
*
* @param listDatasetsRequest
* A {@link Consumer} that will call methods on {@link ListDatasetsRequest.Builder} to create a request.
* Request for the ListDatasets operation.
* @return A Java Future containing the result of the ListDatasets operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InternalServerException The request processing has failed because of an unknown error, exception or
* failure.
* - ThrottlingException The request was denied due to request throttling.
* - ValidationException The input fails to satisfy the constraints specified by an AWS service.
* - ConflictException The request conflicts with an existing resource.
* - ResourceNotFoundException One or more resources can't be found.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - FinspaceDataException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample FinspaceDataAsyncClient.ListDatasets
* @see AWS API
* Documentation
*/
default CompletableFuture listDatasets(Consumer listDatasetsRequest) {
return listDatasets(ListDatasetsRequest.builder().applyMutation(listDatasetsRequest).build());
}
/**
*
* Lists all of the active Datasets that a user has access to.
*
*
*
* This is a variant of
* {@link #listDatasets(software.amazon.awssdk.services.finspacedata.model.ListDatasetsRequest)} operation. The
* return type is a custom publisher that can be subscribed to request a stream of response pages. SDK will
* internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.finspacedata.paginators.ListDatasetsPublisher publisher = client.listDatasetsPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.finspacedata.paginators.ListDatasetsPublisher publisher = client.listDatasetsPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.finspacedata.model.ListDatasetsResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of maxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listDatasets(software.amazon.awssdk.services.finspacedata.model.ListDatasetsRequest)} operation.
*
*
* @param listDatasetsRequest
* Request for the ListDatasets operation.
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InternalServerException The request processing has failed because of an unknown error, exception or
* failure.
* - ThrottlingException The request was denied due to request throttling.
* - ValidationException The input fails to satisfy the constraints specified by an AWS service.
* - ConflictException The request conflicts with an existing resource.
* - ResourceNotFoundException One or more resources can't be found.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - FinspaceDataException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample FinspaceDataAsyncClient.ListDatasets
* @see AWS API
* Documentation
*/
default ListDatasetsPublisher listDatasetsPaginator(ListDatasetsRequest listDatasetsRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Lists all of the active Datasets that a user has access to.
*
*
*
* This is a variant of
* {@link #listDatasets(software.amazon.awssdk.services.finspacedata.model.ListDatasetsRequest)} operation. The
* return type is a custom publisher that can be subscribed to request a stream of response pages. SDK will
* internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.finspacedata.paginators.ListDatasetsPublisher publisher = client.listDatasetsPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.finspacedata.paginators.ListDatasetsPublisher publisher = client.listDatasetsPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.finspacedata.model.ListDatasetsResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of maxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listDatasets(software.amazon.awssdk.services.finspacedata.model.ListDatasetsRequest)} operation.
*
*
* This is a convenience which creates an instance of the {@link ListDatasetsRequest.Builder} avoiding the need to
* create one manually via {@link ListDatasetsRequest#builder()}
*
*
* @param listDatasetsRequest
* A {@link Consumer} that will call methods on {@link ListDatasetsRequest.Builder} to create a request.
* Request for the ListDatasets operation.
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InternalServerException The request processing has failed because of an unknown error, exception or
* failure.
* - ThrottlingException The request was denied due to request throttling.
* - ValidationException The input fails to satisfy the constraints specified by an AWS service.
* - ConflictException The request conflicts with an existing resource.
* - ResourceNotFoundException One or more resources can't be found.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - FinspaceDataException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample FinspaceDataAsyncClient.ListDatasets
* @see AWS API
* Documentation
*/
default ListDatasetsPublisher listDatasetsPaginator(Consumer listDatasetsRequest) {
return listDatasetsPaginator(ListDatasetsRequest.builder().applyMutation(listDatasetsRequest).build());
}
/**
*
* Lists all available permission groups in FinSpace.
*
*
* @param listPermissionGroupsRequest
* @return A Java Future containing the result of the ListPermissionGroups operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InternalServerException The request processing has failed because of an unknown error, exception or
* failure.
* - ValidationException The input fails to satisfy the constraints specified by an AWS service.
* - ThrottlingException The request was denied due to request throttling.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - FinspaceDataException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample FinspaceDataAsyncClient.ListPermissionGroups
* @see AWS
* API Documentation
*/
default CompletableFuture listPermissionGroups(
ListPermissionGroupsRequest listPermissionGroupsRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Lists all available permission groups in FinSpace.
*
*
*
* This is a convenience which creates an instance of the {@link ListPermissionGroupsRequest.Builder} avoiding the
* need to create one manually via {@link ListPermissionGroupsRequest#builder()}
*
*
* @param listPermissionGroupsRequest
* A {@link Consumer} that will call methods on {@link ListPermissionGroupsRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the ListPermissionGroups operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InternalServerException The request processing has failed because of an unknown error, exception or
* failure.
* - ValidationException The input fails to satisfy the constraints specified by an AWS service.
* - ThrottlingException The request was denied due to request throttling.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - FinspaceDataException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample FinspaceDataAsyncClient.ListPermissionGroups
* @see AWS
* API Documentation
*/
default CompletableFuture listPermissionGroups(
Consumer listPermissionGroupsRequest) {
return listPermissionGroups(ListPermissionGroupsRequest.builder().applyMutation(listPermissionGroupsRequest).build());
}
/**
*
* Lists all the permission groups that are associated with a specific user account.
*
*
* @param listPermissionGroupsByUserRequest
* @return A Java Future containing the result of the ListPermissionGroupsByUser operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InternalServerException The request processing has failed because of an unknown error, exception or
* failure.
* - ValidationException The input fails to satisfy the constraints specified by an AWS service.
* - ThrottlingException The request was denied due to request throttling.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ResourceNotFoundException One or more resources can't be found.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - FinspaceDataException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample FinspaceDataAsyncClient.ListPermissionGroupsByUser
* @see AWS API Documentation
*/
default CompletableFuture listPermissionGroupsByUser(
ListPermissionGroupsByUserRequest listPermissionGroupsByUserRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Lists all the permission groups that are associated with a specific user account.
*
*
*
* This is a convenience which creates an instance of the {@link ListPermissionGroupsByUserRequest.Builder} avoiding
* the need to create one manually via {@link ListPermissionGroupsByUserRequest#builder()}
*
*
* @param listPermissionGroupsByUserRequest
* A {@link Consumer} that will call methods on {@link ListPermissionGroupsByUserRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the ListPermissionGroupsByUser operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InternalServerException The request processing has failed because of an unknown error, exception or
* failure.
* - ValidationException The input fails to satisfy the constraints specified by an AWS service.
* - ThrottlingException The request was denied due to request throttling.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ResourceNotFoundException One or more resources can't be found.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - FinspaceDataException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample FinspaceDataAsyncClient.ListPermissionGroupsByUser
* @see AWS API Documentation
*/
default CompletableFuture listPermissionGroupsByUser(
Consumer listPermissionGroupsByUserRequest) {
return listPermissionGroupsByUser(ListPermissionGroupsByUserRequest.builder()
.applyMutation(listPermissionGroupsByUserRequest).build());
}
/**
*
* Lists all available permission groups in FinSpace.
*
*
*
* This is a variant of
* {@link #listPermissionGroups(software.amazon.awssdk.services.finspacedata.model.ListPermissionGroupsRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.finspacedata.paginators.ListPermissionGroupsPublisher publisher = client.listPermissionGroupsPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.finspacedata.paginators.ListPermissionGroupsPublisher publisher = client.listPermissionGroupsPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.finspacedata.model.ListPermissionGroupsResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of maxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listPermissionGroups(software.amazon.awssdk.services.finspacedata.model.ListPermissionGroupsRequest)}
* operation.
*
*
* @param listPermissionGroupsRequest
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InternalServerException The request processing has failed because of an unknown error, exception or
* failure.
* - ValidationException The input fails to satisfy the constraints specified by an AWS service.
* - ThrottlingException The request was denied due to request throttling.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - FinspaceDataException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample FinspaceDataAsyncClient.ListPermissionGroups
* @see AWS
* API Documentation
*/
default ListPermissionGroupsPublisher listPermissionGroupsPaginator(ListPermissionGroupsRequest listPermissionGroupsRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Lists all available permission groups in FinSpace.
*
*
*
* This is a variant of
* {@link #listPermissionGroups(software.amazon.awssdk.services.finspacedata.model.ListPermissionGroupsRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.finspacedata.paginators.ListPermissionGroupsPublisher publisher = client.listPermissionGroupsPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.finspacedata.paginators.ListPermissionGroupsPublisher publisher = client.listPermissionGroupsPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.finspacedata.model.ListPermissionGroupsResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of maxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listPermissionGroups(software.amazon.awssdk.services.finspacedata.model.ListPermissionGroupsRequest)}
* operation.
*
*
* This is a convenience which creates an instance of the {@link ListPermissionGroupsRequest.Builder} avoiding the
* need to create one manually via {@link ListPermissionGroupsRequest#builder()}
*
*
* @param listPermissionGroupsRequest
* A {@link Consumer} that will call methods on {@link ListPermissionGroupsRequest.Builder} to create a
* request.
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InternalServerException The request processing has failed because of an unknown error, exception or
* failure.
* - ValidationException The input fails to satisfy the constraints specified by an AWS service.
* - ThrottlingException The request was denied due to request throttling.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - FinspaceDataException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample FinspaceDataAsyncClient.ListPermissionGroups
* @see AWS
* API Documentation
*/
default ListPermissionGroupsPublisher listPermissionGroupsPaginator(
Consumer listPermissionGroupsRequest) {
return listPermissionGroupsPaginator(ListPermissionGroupsRequest.builder().applyMutation(listPermissionGroupsRequest)
.build());
}
/**
*
* Lists all available user accounts in FinSpace.
*
*
* @param listUsersRequest
* @return A Java Future containing the result of the ListUsers operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InternalServerException The request processing has failed because of an unknown error, exception or
* failure.
* - ValidationException The input fails to satisfy the constraints specified by an AWS service.
* - ThrottlingException The request was denied due to request throttling.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - FinspaceDataException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample FinspaceDataAsyncClient.ListUsers
* @see AWS API
* Documentation
*/
default CompletableFuture listUsers(ListUsersRequest listUsersRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Lists all available user accounts in FinSpace.
*
*
*
* This is a convenience which creates an instance of the {@link ListUsersRequest.Builder} avoiding the need to
* create one manually via {@link ListUsersRequest#builder()}
*
*
* @param listUsersRequest
* A {@link Consumer} that will call methods on {@link ListUsersRequest.Builder} to create a request.
* @return A Java Future containing the result of the ListUsers operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InternalServerException The request processing has failed because of an unknown error, exception or
* failure.
* - ValidationException The input fails to satisfy the constraints specified by an AWS service.
* - ThrottlingException The request was denied due to request throttling.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - FinspaceDataException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample FinspaceDataAsyncClient.ListUsers
* @see AWS API
* Documentation
*/
default CompletableFuture listUsers(Consumer listUsersRequest) {
return listUsers(ListUsersRequest.builder().applyMutation(listUsersRequest).build());
}
/**
*
* Lists details of all the users in a specific permission group.
*
*
* @param listUsersByPermissionGroupRequest
* @return A Java Future containing the result of the ListUsersByPermissionGroup operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InternalServerException The request processing has failed because of an unknown error, exception or
* failure.
* - ValidationException The input fails to satisfy the constraints specified by an AWS service.
* - ThrottlingException The request was denied due to request throttling.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ResourceNotFoundException One or more resources can't be found.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - FinspaceDataException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample FinspaceDataAsyncClient.ListUsersByPermissionGroup
* @see AWS API Documentation
*/
default CompletableFuture listUsersByPermissionGroup(
ListUsersByPermissionGroupRequest listUsersByPermissionGroupRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Lists details of all the users in a specific permission group.
*
*
*
* This is a convenience which creates an instance of the {@link ListUsersByPermissionGroupRequest.Builder} avoiding
* the need to create one manually via {@link ListUsersByPermissionGroupRequest#builder()}
*
*
* @param listUsersByPermissionGroupRequest
* A {@link Consumer} that will call methods on {@link ListUsersByPermissionGroupRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the ListUsersByPermissionGroup operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InternalServerException The request processing has failed because of an unknown error, exception or
* failure.
* - ValidationException The input fails to satisfy the constraints specified by an AWS service.
* - ThrottlingException The request was denied due to request throttling.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ResourceNotFoundException One or more resources can't be found.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - FinspaceDataException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample FinspaceDataAsyncClient.ListUsersByPermissionGroup
* @see AWS API Documentation
*/
default CompletableFuture listUsersByPermissionGroup(
Consumer listUsersByPermissionGroupRequest) {
return listUsersByPermissionGroup(ListUsersByPermissionGroupRequest.builder()
.applyMutation(listUsersByPermissionGroupRequest).build());
}
/**
*
* Lists all available user accounts in FinSpace.
*
*
*
* This is a variant of {@link #listUsers(software.amazon.awssdk.services.finspacedata.model.ListUsersRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.finspacedata.paginators.ListUsersPublisher publisher = client.listUsersPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.finspacedata.paginators.ListUsersPublisher publisher = client.listUsersPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.finspacedata.model.ListUsersResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of maxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listUsers(software.amazon.awssdk.services.finspacedata.model.ListUsersRequest)} operation.
*
*
* @param listUsersRequest
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InternalServerException The request processing has failed because of an unknown error, exception or
* failure.
* - ValidationException The input fails to satisfy the constraints specified by an AWS service.
* - ThrottlingException The request was denied due to request throttling.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - FinspaceDataException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample FinspaceDataAsyncClient.ListUsers
* @see AWS API
* Documentation
*/
default ListUsersPublisher listUsersPaginator(ListUsersRequest listUsersRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Lists all available user accounts in FinSpace.
*
*
*
* This is a variant of {@link #listUsers(software.amazon.awssdk.services.finspacedata.model.ListUsersRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.finspacedata.paginators.ListUsersPublisher publisher = client.listUsersPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.finspacedata.paginators.ListUsersPublisher publisher = client.listUsersPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.finspacedata.model.ListUsersResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of maxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listUsers(software.amazon.awssdk.services.finspacedata.model.ListUsersRequest)} operation.
*
*
* This is a convenience which creates an instance of the {@link ListUsersRequest.Builder} avoiding the need to
* create one manually via {@link ListUsersRequest#builder()}
*
*
* @param listUsersRequest
* A {@link Consumer} that will call methods on {@link ListUsersRequest.Builder} to create a request.
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InternalServerException The request processing has failed because of an unknown error, exception or
* failure.
* - ValidationException The input fails to satisfy the constraints specified by an AWS service.
* - ThrottlingException The request was denied due to request throttling.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - FinspaceDataException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample FinspaceDataAsyncClient.ListUsers
* @see AWS API
* Documentation
*/
default ListUsersPublisher listUsersPaginator(Consumer listUsersRequest) {
return listUsersPaginator(ListUsersRequest.builder().applyMutation(listUsersRequest).build());
}
/**
*
* Resets the password for a specified user ID and generates a temporary one. Only a superuser can reset password
* for other users. Resetting the password immediately invalidates the previous password associated with the user.
*
*
* @param resetUserPasswordRequest
* @return A Java Future containing the result of the ResetUserPassword operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InternalServerException The request processing has failed because of an unknown error, exception or
* failure.
* - ValidationException The input fails to satisfy the constraints specified by an AWS service.
* - ThrottlingException The request was denied due to request throttling.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ResourceNotFoundException One or more resources can't be found.
* - ConflictException The request conflicts with an existing resource.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - FinspaceDataException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample FinspaceDataAsyncClient.ResetUserPassword
* @see AWS
* API Documentation
*/
default CompletableFuture resetUserPassword(ResetUserPasswordRequest resetUserPasswordRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Resets the password for a specified user ID and generates a temporary one. Only a superuser can reset password
* for other users. Resetting the password immediately invalidates the previous password associated with the user.
*
*
*
* This is a convenience which creates an instance of the {@link ResetUserPasswordRequest.Builder} avoiding the need
* to create one manually via {@link ResetUserPasswordRequest#builder()}
*
*
* @param resetUserPasswordRequest
* A {@link Consumer} that will call methods on {@link ResetUserPasswordRequest.Builder} to create a request.
* @return A Java Future containing the result of the ResetUserPassword operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InternalServerException The request processing has failed because of an unknown error, exception or
* failure.
* - ValidationException The input fails to satisfy the constraints specified by an AWS service.
* - ThrottlingException The request was denied due to request throttling.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ResourceNotFoundException One or more resources can't be found.
* - ConflictException The request conflicts with an existing resource.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - FinspaceDataException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample FinspaceDataAsyncClient.ResetUserPassword
* @see AWS
* API Documentation
*/
default CompletableFuture resetUserPassword(
Consumer resetUserPasswordRequest) {
return resetUserPassword(ResetUserPasswordRequest.builder().applyMutation(resetUserPasswordRequest).build());
}
/**
*
* Updates a FinSpace Changeset.
*
*
* @param updateChangesetRequest
* Request to update an existing changeset.
* @return A Java Future containing the result of the UpdateChangeset operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ResourceNotFoundException One or more resources can't be found.
* - InternalServerException The request processing has failed because of an unknown error, exception or
* failure.
* - ValidationException The input fails to satisfy the constraints specified by an AWS service.
* - ThrottlingException The request was denied due to request throttling.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ConflictException The request conflicts with an existing resource.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - FinspaceDataException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample FinspaceDataAsyncClient.UpdateChangeset
* @see AWS API
* Documentation
*/
default CompletableFuture updateChangeset(UpdateChangesetRequest updateChangesetRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Updates a FinSpace Changeset.
*
*
*
* This is a convenience which creates an instance of the {@link UpdateChangesetRequest.Builder} avoiding the need
* to create one manually via {@link UpdateChangesetRequest#builder()}
*
*
* @param updateChangesetRequest
* A {@link Consumer} that will call methods on {@link UpdateChangesetRequest.Builder} to create a request.
* Request to update an existing changeset.
* @return A Java Future containing the result of the UpdateChangeset operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ResourceNotFoundException One or more resources can't be found.
* - InternalServerException The request processing has failed because of an unknown error, exception or
* failure.
* - ValidationException The input fails to satisfy the constraints specified by an AWS service.
* - ThrottlingException The request was denied due to request throttling.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ConflictException The request conflicts with an existing resource.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - FinspaceDataException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample FinspaceDataAsyncClient.UpdateChangeset
* @see AWS API
* Documentation
*/
default CompletableFuture updateChangeset(
Consumer updateChangesetRequest) {
return updateChangeset(UpdateChangesetRequest.builder().applyMutation(updateChangesetRequest).build());
}
/**
*
* Updates a FinSpace Dataset.
*
*
* @param updateDatasetRequest
* The request for an UpdateDataset operation
* @return A Java Future containing the result of the UpdateDataset operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InternalServerException The request processing has failed because of an unknown error, exception or
* failure.
* - ValidationException The input fails to satisfy the constraints specified by an AWS service.
* - ThrottlingException The request was denied due to request throttling.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ConflictException The request conflicts with an existing resource.
* - ResourceNotFoundException One or more resources can't be found.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - FinspaceDataException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample FinspaceDataAsyncClient.UpdateDataset
* @see AWS API
* Documentation
*/
default CompletableFuture updateDataset(UpdateDatasetRequest updateDatasetRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Updates a FinSpace Dataset.
*
*
*
* This is a convenience which creates an instance of the {@link UpdateDatasetRequest.Builder} avoiding the need to
* create one manually via {@link UpdateDatasetRequest#builder()}
*
*
* @param updateDatasetRequest
* A {@link Consumer} that will call methods on {@link UpdateDatasetRequest.Builder} to create a request. The
* request for an UpdateDataset operation
* @return A Java Future containing the result of the UpdateDataset operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InternalServerException The request processing has failed because of an unknown error, exception or
* failure.
* - ValidationException The input fails to satisfy the constraints specified by an AWS service.
* - ThrottlingException The request was denied due to request throttling.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ConflictException The request conflicts with an existing resource.
* - ResourceNotFoundException One or more resources can't be found.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - FinspaceDataException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample FinspaceDataAsyncClient.UpdateDataset
* @see AWS API
* Documentation
*/
default CompletableFuture updateDataset(Consumer updateDatasetRequest) {
return updateDataset(UpdateDatasetRequest.builder().applyMutation(updateDatasetRequest).build());
}
/**
*
* Modifies the details of a permission group. You cannot modify a permissionGroupID
.
*
*
* @param updatePermissionGroupRequest
* @return A Java Future containing the result of the UpdatePermissionGroup operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InternalServerException The request processing has failed because of an unknown error, exception or
* failure.
* - ValidationException The input fails to satisfy the constraints specified by an AWS service.
* - ThrottlingException The request was denied due to request throttling.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ResourceNotFoundException One or more resources can't be found.
* - ConflictException The request conflicts with an existing resource.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - FinspaceDataException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample FinspaceDataAsyncClient.UpdatePermissionGroup
* @see AWS API Documentation
*/
default CompletableFuture updatePermissionGroup(
UpdatePermissionGroupRequest updatePermissionGroupRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Modifies the details of a permission group. You cannot modify a permissionGroupID
.
*
*
*
* This is a convenience which creates an instance of the {@link UpdatePermissionGroupRequest.Builder} avoiding the
* need to create one manually via {@link UpdatePermissionGroupRequest#builder()}
*
*
* @param updatePermissionGroupRequest
* A {@link Consumer} that will call methods on {@link UpdatePermissionGroupRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the UpdatePermissionGroup operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InternalServerException The request processing has failed because of an unknown error, exception or
* failure.
* - ValidationException The input fails to satisfy the constraints specified by an AWS service.
* - ThrottlingException The request was denied due to request throttling.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ResourceNotFoundException One or more resources can't be found.
* - ConflictException The request conflicts with an existing resource.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - FinspaceDataException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample FinspaceDataAsyncClient.UpdatePermissionGroup
* @see AWS API Documentation
*/
default CompletableFuture updatePermissionGroup(
Consumer updatePermissionGroupRequest) {
return updatePermissionGroup(UpdatePermissionGroupRequest.builder().applyMutation(updatePermissionGroupRequest).build());
}
/**
*
* Modifies the details of the specified user account. You cannot update the userId
for a user.
*
*
* @param updateUserRequest
* @return A Java Future containing the result of the UpdateUser operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InternalServerException The request processing has failed because of an unknown error, exception or
* failure.
* - ValidationException The input fails to satisfy the constraints specified by an AWS service.
* - ThrottlingException The request was denied due to request throttling.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ResourceNotFoundException One or more resources can't be found.
* - ConflictException The request conflicts with an existing resource.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - FinspaceDataException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample FinspaceDataAsyncClient.UpdateUser
* @see AWS API
* Documentation
*/
default CompletableFuture updateUser(UpdateUserRequest updateUserRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Modifies the details of the specified user account. You cannot update the userId
for a user.
*
*
*
* This is a convenience which creates an instance of the {@link UpdateUserRequest.Builder} avoiding the need to
* create one manually via {@link UpdateUserRequest#builder()}
*
*
* @param updateUserRequest
* A {@link Consumer} that will call methods on {@link UpdateUserRequest.Builder} to create a request.
* @return A Java Future containing the result of the UpdateUser operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InternalServerException The request processing has failed because of an unknown error, exception or
* failure.
* - ValidationException The input fails to satisfy the constraints specified by an AWS service.
* - ThrottlingException The request was denied due to request throttling.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ResourceNotFoundException One or more resources can't be found.
* - ConflictException The request conflicts with an existing resource.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - FinspaceDataException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample FinspaceDataAsyncClient.UpdateUser
* @see AWS API
* Documentation
*/
default CompletableFuture updateUser(Consumer updateUserRequest) {
return updateUser(UpdateUserRequest.builder().applyMutation(updateUserRequest).build());
}
/**
* Create a {@link FinspaceDataAsyncClient} with the region loaded from the
* {@link software.amazon.awssdk.regions.providers.DefaultAwsRegionProviderChain} and credentials loaded from the
* {@link software.amazon.awssdk.auth.credentials.DefaultCredentialsProvider}.
*/
static FinspaceDataAsyncClient create() {
return builder().build();
}
/**
* Create a builder that can be used to configure and create a {@link FinspaceDataAsyncClient}.
*/
static FinspaceDataAsyncClientBuilder builder() {
return new DefaultFinspaceDataAsyncClientBuilder();
}
}