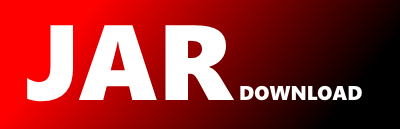
software.amazon.awssdk.services.finspacedata.model.ColumnDefinition Maven / Gradle / Ivy
Show all versions of finspacedata Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.finspacedata.model;
import java.io.Serializable;
import java.util.Arrays;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* The definition of a column in a tabular Dataset.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class ColumnDefinition implements SdkPojo, Serializable,
ToCopyableBuilder {
private static final SdkField DATA_TYPE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("dataType").getter(getter(ColumnDefinition::dataTypeAsString)).setter(setter(Builder::dataType))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("dataType").build()).build();
private static final SdkField COLUMN_NAME_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("columnName").getter(getter(ColumnDefinition::columnName)).setter(setter(Builder::columnName))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("columnName").build()).build();
private static final SdkField COLUMN_DESCRIPTION_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("columnDescription").getter(getter(ColumnDefinition::columnDescription))
.setter(setter(Builder::columnDescription))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("columnDescription").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(DATA_TYPE_FIELD,
COLUMN_NAME_FIELD, COLUMN_DESCRIPTION_FIELD));
private static final long serialVersionUID = 1L;
private final String dataType;
private final String columnName;
private final String columnDescription;
private ColumnDefinition(BuilderImpl builder) {
this.dataType = builder.dataType;
this.columnName = builder.columnName;
this.columnDescription = builder.columnDescription;
}
/**
*
* Data type of a column.
*
*
* -
*
* STRING
– A String data type.
*
*
* CHAR
– A char data type.
*
*
* INTEGER
– An integer data type.
*
*
* TINYINT
– A tinyint data type.
*
*
* SMALLINT
– A smallint data type.
*
*
* BIGINT
– A bigint data type.
*
*
* FLOAT
– A float data type.
*
*
* DOUBLE
– A double data type.
*
*
* DATE
– A date data type.
*
*
* DATETIME
– A datetime data type.
*
*
* BOOLEAN
– A boolean data type.
*
*
* BINARY
– A binary data type.
*
*
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #dataType} will
* return {@link ColumnDataType#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #dataTypeAsString}.
*
*
* @return Data type of a column.
*
* -
*
* STRING
– A String data type.
*
*
* CHAR
– A char data type.
*
*
* INTEGER
– An integer data type.
*
*
* TINYINT
– A tinyint data type.
*
*
* SMALLINT
– A smallint data type.
*
*
* BIGINT
– A bigint data type.
*
*
* FLOAT
– A float data type.
*
*
* DOUBLE
– A double data type.
*
*
* DATE
– A date data type.
*
*
* DATETIME
– A datetime data type.
*
*
* BOOLEAN
– A boolean data type.
*
*
* BINARY
– A binary data type.
*
*
* @see ColumnDataType
*/
public final ColumnDataType dataType() {
return ColumnDataType.fromValue(dataType);
}
/**
*
* Data type of a column.
*
*
* -
*
* STRING
– A String data type.
*
*
* CHAR
– A char data type.
*
*
* INTEGER
– An integer data type.
*
*
* TINYINT
– A tinyint data type.
*
*
* SMALLINT
– A smallint data type.
*
*
* BIGINT
– A bigint data type.
*
*
* FLOAT
– A float data type.
*
*
* DOUBLE
– A double data type.
*
*
* DATE
– A date data type.
*
*
* DATETIME
– A datetime data type.
*
*
* BOOLEAN
– A boolean data type.
*
*
* BINARY
– A binary data type.
*
*
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #dataType} will
* return {@link ColumnDataType#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #dataTypeAsString}.
*
*
* @return Data type of a column.
*
* -
*
* STRING
– A String data type.
*
*
* CHAR
– A char data type.
*
*
* INTEGER
– An integer data type.
*
*
* TINYINT
– A tinyint data type.
*
*
* SMALLINT
– A smallint data type.
*
*
* BIGINT
– A bigint data type.
*
*
* FLOAT
– A float data type.
*
*
* DOUBLE
– A double data type.
*
*
* DATE
– A date data type.
*
*
* DATETIME
– A datetime data type.
*
*
* BOOLEAN
– A boolean data type.
*
*
* BINARY
– A binary data type.
*
*
* @see ColumnDataType
*/
public final String dataTypeAsString() {
return dataType;
}
/**
*
* The name of a column.
*
*
* @return The name of a column.
*/
public final String columnName() {
return columnName;
}
/**
*
* Description for a column.
*
*
* @return Description for a column.
*/
public final String columnDescription() {
return columnDescription;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(dataTypeAsString());
hashCode = 31 * hashCode + Objects.hashCode(columnName());
hashCode = 31 * hashCode + Objects.hashCode(columnDescription());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof ColumnDefinition)) {
return false;
}
ColumnDefinition other = (ColumnDefinition) obj;
return Objects.equals(dataTypeAsString(), other.dataTypeAsString()) && Objects.equals(columnName(), other.columnName())
&& Objects.equals(columnDescription(), other.columnDescription());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("ColumnDefinition").add("DataType", dataTypeAsString()).add("ColumnName", columnName())
.add("ColumnDescription", columnDescription()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "dataType":
return Optional.ofNullable(clazz.cast(dataTypeAsString()));
case "columnName":
return Optional.ofNullable(clazz.cast(columnName()));
case "columnDescription":
return Optional.ofNullable(clazz.cast(columnDescription()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
private static Function