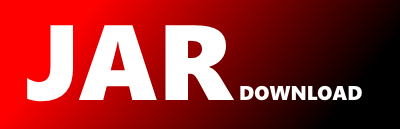
software.amazon.awssdk.services.finspacedata.model.UserByPermissionGroup Maven / Gradle / Ivy
Show all versions of finspacedata Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.finspacedata.model;
import java.io.Serializable;
import java.util.Arrays;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* The structure of a user associated with a permission group.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class UserByPermissionGroup implements SdkPojo, Serializable,
ToCopyableBuilder {
private static final SdkField USER_ID_FIELD = SdkField. builder(MarshallingType.STRING).memberName("userId")
.getter(getter(UserByPermissionGroup::userId)).setter(setter(Builder::userId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("userId").build()).build();
private static final SdkField STATUS_FIELD = SdkField. builder(MarshallingType.STRING).memberName("status")
.getter(getter(UserByPermissionGroup::statusAsString)).setter(setter(Builder::status))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("status").build()).build();
private static final SdkField FIRST_NAME_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("firstName").getter(getter(UserByPermissionGroup::firstName)).setter(setter(Builder::firstName))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("firstName").build()).build();
private static final SdkField LAST_NAME_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("lastName").getter(getter(UserByPermissionGroup::lastName)).setter(setter(Builder::lastName))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("lastName").build()).build();
private static final SdkField EMAIL_ADDRESS_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("emailAddress").getter(getter(UserByPermissionGroup::emailAddress)).setter(setter(Builder::emailAddress))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("emailAddress").build()).build();
private static final SdkField TYPE_FIELD = SdkField. builder(MarshallingType.STRING).memberName("type")
.getter(getter(UserByPermissionGroup::typeAsString)).setter(setter(Builder::type))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("type").build()).build();
private static final SdkField API_ACCESS_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("apiAccess").getter(getter(UserByPermissionGroup::apiAccessAsString)).setter(setter(Builder::apiAccess))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("apiAccess").build()).build();
private static final SdkField API_ACCESS_PRINCIPAL_ARN_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("apiAccessPrincipalArn").getter(getter(UserByPermissionGroup::apiAccessPrincipalArn))
.setter(setter(Builder::apiAccessPrincipalArn))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("apiAccessPrincipalArn").build())
.build();
private static final SdkField MEMBERSHIP_STATUS_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("membershipStatus").getter(getter(UserByPermissionGroup::membershipStatusAsString))
.setter(setter(Builder::membershipStatus))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("membershipStatus").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(USER_ID_FIELD, STATUS_FIELD,
FIRST_NAME_FIELD, LAST_NAME_FIELD, EMAIL_ADDRESS_FIELD, TYPE_FIELD, API_ACCESS_FIELD, API_ACCESS_PRINCIPAL_ARN_FIELD,
MEMBERSHIP_STATUS_FIELD));
private static final long serialVersionUID = 1L;
private final String userId;
private final String status;
private final String firstName;
private final String lastName;
private final String emailAddress;
private final String type;
private final String apiAccess;
private final String apiAccessPrincipalArn;
private final String membershipStatus;
private UserByPermissionGroup(BuilderImpl builder) {
this.userId = builder.userId;
this.status = builder.status;
this.firstName = builder.firstName;
this.lastName = builder.lastName;
this.emailAddress = builder.emailAddress;
this.type = builder.type;
this.apiAccess = builder.apiAccess;
this.apiAccessPrincipalArn = builder.apiAccessPrincipalArn;
this.membershipStatus = builder.membershipStatus;
}
/**
*
* The unique identifier for the user.
*
*
* @return The unique identifier for the user.
*/
public final String userId() {
return userId;
}
/**
*
* The current status of the user.
*
*
* -
*
* CREATING
– The user creation is in progress.
*
*
* -
*
* ENABLED
– The user is created and is currently active.
*
*
* -
*
* DISABLED
– The user is currently inactive.
*
*
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #status} will
* return {@link UserStatus#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #statusAsString}.
*
*
* @return The current status of the user.
*
* -
*
* CREATING
– The user creation is in progress.
*
*
* -
*
* ENABLED
– The user is created and is currently active.
*
*
* -
*
* DISABLED
– The user is currently inactive.
*
*
* @see UserStatus
*/
public final UserStatus status() {
return UserStatus.fromValue(status);
}
/**
*
* The current status of the user.
*
*
* -
*
* CREATING
– The user creation is in progress.
*
*
* -
*
* ENABLED
– The user is created and is currently active.
*
*
* -
*
* DISABLED
– The user is currently inactive.
*
*
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #status} will
* return {@link UserStatus#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #statusAsString}.
*
*
* @return The current status of the user.
*
* -
*
* CREATING
– The user creation is in progress.
*
*
* -
*
* ENABLED
– The user is created and is currently active.
*
*
* -
*
* DISABLED
– The user is currently inactive.
*
*
* @see UserStatus
*/
public final String statusAsString() {
return status;
}
/**
*
* The first name of the user.
*
*
* @return The first name of the user.
*/
public final String firstName() {
return firstName;
}
/**
*
* The last name of the user.
*
*
* @return The last name of the user.
*/
public final String lastName() {
return lastName;
}
/**
*
* The email address of the user. The email address serves as a unique identifier for each user and cannot be
* changed after it's created.
*
*
* @return The email address of the user. The email address serves as a unique identifier for each user and cannot
* be changed after it's created.
*/
public final String emailAddress() {
return emailAddress;
}
/**
*
* Indicates the type of user.
*
*
* -
*
* SUPER_USER
– A user with permission to all the functionality and data in FinSpace.
*
*
* -
*
* APP_USER
– A user with specific permissions in FinSpace. The users are assigned permissions by
* adding them to a permission group.
*
*
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #type} will return
* {@link UserType#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #typeAsString}.
*
*
* @return Indicates the type of user.
*
* -
*
* SUPER_USER
– A user with permission to all the functionality and data in FinSpace.
*
*
* -
*
* APP_USER
– A user with specific permissions in FinSpace. The users are assigned permissions
* by adding them to a permission group.
*
*
* @see UserType
*/
public final UserType type() {
return UserType.fromValue(type);
}
/**
*
* Indicates the type of user.
*
*
* -
*
* SUPER_USER
– A user with permission to all the functionality and data in FinSpace.
*
*
* -
*
* APP_USER
– A user with specific permissions in FinSpace. The users are assigned permissions by
* adding them to a permission group.
*
*
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #type} will return
* {@link UserType#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #typeAsString}.
*
*
* @return Indicates the type of user.
*
* -
*
* SUPER_USER
– A user with permission to all the functionality and data in FinSpace.
*
*
* -
*
* APP_USER
– A user with specific permissions in FinSpace. The users are assigned permissions
* by adding them to a permission group.
*
*
* @see UserType
*/
public final String typeAsString() {
return type;
}
/**
*
* Indicates whether the user can access FinSpace API operations.
*
*
* -
*
* ENABLED
– The user has permissions to use the API operations.
*
*
* -
*
* DISABLED
– The user does not have permissions to use any API operations.
*
*
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #apiAccess} will
* return {@link ApiAccess#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #apiAccessAsString}.
*
*
* @return Indicates whether the user can access FinSpace API operations.
*
* -
*
* ENABLED
– The user has permissions to use the API operations.
*
*
* -
*
* DISABLED
– The user does not have permissions to use any API operations.
*
*
* @see ApiAccess
*/
public final ApiAccess apiAccess() {
return ApiAccess.fromValue(apiAccess);
}
/**
*
* Indicates whether the user can access FinSpace API operations.
*
*
* -
*
* ENABLED
– The user has permissions to use the API operations.
*
*
* -
*
* DISABLED
– The user does not have permissions to use any API operations.
*
*
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #apiAccess} will
* return {@link ApiAccess#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #apiAccessAsString}.
*
*
* @return Indicates whether the user can access FinSpace API operations.
*
* -
*
* ENABLED
– The user has permissions to use the API operations.
*
*
* -
*
* DISABLED
– The user does not have permissions to use any API operations.
*
*
* @see ApiAccess
*/
public final String apiAccessAsString() {
return apiAccess;
}
/**
*
* The IAM ARN identifier that is attached to FinSpace API calls.
*
*
* @return The IAM ARN identifier that is attached to FinSpace API calls.
*/
public final String apiAccessPrincipalArn() {
return apiAccessPrincipalArn;
}
/**
*
* Indicates the status of the user within a permission group.
*
*
* -
*
* ADDITION_IN_PROGRESS
– The user is currently being added to the permission group.
*
*
* -
*
* ADDITION_SUCCESS
– The user is successfully added to the permission group.
*
*
* -
*
* REMOVAL_IN_PROGRESS
– The user is currently being removed from the permission group.
*
*
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #membershipStatus}
* will return {@link PermissionGroupMembershipStatus#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service
* is available from {@link #membershipStatusAsString}.
*
*
* @return Indicates the status of the user within a permission group.
*
* -
*
* ADDITION_IN_PROGRESS
– The user is currently being added to the permission group.
*
*
* -
*
* ADDITION_SUCCESS
– The user is successfully added to the permission group.
*
*
* -
*
* REMOVAL_IN_PROGRESS
– The user is currently being removed from the permission group.
*
*
* @see PermissionGroupMembershipStatus
*/
public final PermissionGroupMembershipStatus membershipStatus() {
return PermissionGroupMembershipStatus.fromValue(membershipStatus);
}
/**
*
* Indicates the status of the user within a permission group.
*
*
* -
*
* ADDITION_IN_PROGRESS
– The user is currently being added to the permission group.
*
*
* -
*
* ADDITION_SUCCESS
– The user is successfully added to the permission group.
*
*
* -
*
* REMOVAL_IN_PROGRESS
– The user is currently being removed from the permission group.
*
*
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #membershipStatus}
* will return {@link PermissionGroupMembershipStatus#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service
* is available from {@link #membershipStatusAsString}.
*
*
* @return Indicates the status of the user within a permission group.
*
* -
*
* ADDITION_IN_PROGRESS
– The user is currently being added to the permission group.
*
*
* -
*
* ADDITION_SUCCESS
– The user is successfully added to the permission group.
*
*
* -
*
* REMOVAL_IN_PROGRESS
– The user is currently being removed from the permission group.
*
*
* @see PermissionGroupMembershipStatus
*/
public final String membershipStatusAsString() {
return membershipStatus;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(userId());
hashCode = 31 * hashCode + Objects.hashCode(statusAsString());
hashCode = 31 * hashCode + Objects.hashCode(firstName());
hashCode = 31 * hashCode + Objects.hashCode(lastName());
hashCode = 31 * hashCode + Objects.hashCode(emailAddress());
hashCode = 31 * hashCode + Objects.hashCode(typeAsString());
hashCode = 31 * hashCode + Objects.hashCode(apiAccessAsString());
hashCode = 31 * hashCode + Objects.hashCode(apiAccessPrincipalArn());
hashCode = 31 * hashCode + Objects.hashCode(membershipStatusAsString());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof UserByPermissionGroup)) {
return false;
}
UserByPermissionGroup other = (UserByPermissionGroup) obj;
return Objects.equals(userId(), other.userId()) && Objects.equals(statusAsString(), other.statusAsString())
&& Objects.equals(firstName(), other.firstName()) && Objects.equals(lastName(), other.lastName())
&& Objects.equals(emailAddress(), other.emailAddress()) && Objects.equals(typeAsString(), other.typeAsString())
&& Objects.equals(apiAccessAsString(), other.apiAccessAsString())
&& Objects.equals(apiAccessPrincipalArn(), other.apiAccessPrincipalArn())
&& Objects.equals(membershipStatusAsString(), other.membershipStatusAsString());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("UserByPermissionGroup").add("UserId", userId()).add("Status", statusAsString())
.add("FirstName", firstName() == null ? null : "*** Sensitive Data Redacted ***")
.add("LastName", lastName() == null ? null : "*** Sensitive Data Redacted ***")
.add("EmailAddress", emailAddress() == null ? null : "*** Sensitive Data Redacted ***")
.add("Type", typeAsString()).add("ApiAccess", apiAccessAsString())
.add("ApiAccessPrincipalArn", apiAccessPrincipalArn()).add("MembershipStatus", membershipStatusAsString())
.build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "userId":
return Optional.ofNullable(clazz.cast(userId()));
case "status":
return Optional.ofNullable(clazz.cast(statusAsString()));
case "firstName":
return Optional.ofNullable(clazz.cast(firstName()));
case "lastName":
return Optional.ofNullable(clazz.cast(lastName()));
case "emailAddress":
return Optional.ofNullable(clazz.cast(emailAddress()));
case "type":
return Optional.ofNullable(clazz.cast(typeAsString()));
case "apiAccess":
return Optional.ofNullable(clazz.cast(apiAccessAsString()));
case "apiAccessPrincipalArn":
return Optional.ofNullable(clazz.cast(apiAccessPrincipalArn()));
case "membershipStatus":
return Optional.ofNullable(clazz.cast(membershipStatusAsString()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
private static Function