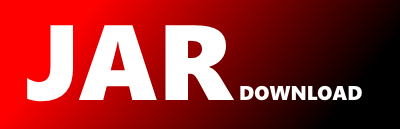
software.amazon.awssdk.services.firehose.model.DatabaseSourceDescription Maven / Gradle / Ivy
Show all versions of firehose Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.firehose.model;
import java.io.Serializable;
import java.util.Arrays;
import java.util.Collection;
import java.util.Collections;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import java.util.stream.Collectors;
import java.util.stream.Stream;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.ListTrait;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.core.util.DefaultSdkAutoConstructList;
import software.amazon.awssdk.core.util.SdkAutoConstructList;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
*
*
* Amazon Data Firehose is in preview release and is subject to change.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class DatabaseSourceDescription implements SdkPojo, Serializable,
ToCopyableBuilder {
private static final SdkField TYPE_FIELD = SdkField. builder(MarshallingType.STRING).memberName("Type")
.getter(getter(DatabaseSourceDescription::typeAsString)).setter(setter(Builder::type))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Type").build()).build();
private static final SdkField ENDPOINT_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("Endpoint").getter(getter(DatabaseSourceDescription::endpoint)).setter(setter(Builder::endpoint))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Endpoint").build()).build();
private static final SdkField PORT_FIELD = SdkField. builder(MarshallingType.INTEGER).memberName("Port")
.getter(getter(DatabaseSourceDescription::port)).setter(setter(Builder::port))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Port").build()).build();
private static final SdkField SSL_MODE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("SSLMode").getter(getter(DatabaseSourceDescription::sslModeAsString)).setter(setter(Builder::sslMode))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("SSLMode").build()).build();
private static final SdkField DATABASES_FIELD = SdkField. builder(MarshallingType.SDK_POJO)
.memberName("Databases").getter(getter(DatabaseSourceDescription::databases)).setter(setter(Builder::databases))
.constructor(DatabaseList::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Databases").build()).build();
private static final SdkField TABLES_FIELD = SdkField
. builder(MarshallingType.SDK_POJO).memberName("Tables")
.getter(getter(DatabaseSourceDescription::tables)).setter(setter(Builder::tables))
.constructor(DatabaseTableList::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Tables").build()).build();
private static final SdkField COLUMNS_FIELD = SdkField
. builder(MarshallingType.SDK_POJO).memberName("Columns")
.getter(getter(DatabaseSourceDescription::columns)).setter(setter(Builder::columns))
.constructor(DatabaseColumnList::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Columns").build()).build();
private static final SdkField> SURROGATE_KEYS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("SurrogateKeys")
.getter(getter(DatabaseSourceDescription::surrogateKeys))
.setter(setter(Builder::surrogateKeys))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("SurrogateKeys").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.STRING)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField SNAPSHOT_WATERMARK_TABLE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("SnapshotWatermarkTable").getter(getter(DatabaseSourceDescription::snapshotWatermarkTable))
.setter(setter(Builder::snapshotWatermarkTable))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("SnapshotWatermarkTable").build())
.build();
private static final SdkField> SNAPSHOT_INFO_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("SnapshotInfo")
.getter(getter(DatabaseSourceDescription::snapshotInfo))
.setter(setter(Builder::snapshotInfo))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("SnapshotInfo").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(DatabaseSnapshotInfo::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField DATABASE_SOURCE_AUTHENTICATION_CONFIGURATION_FIELD = SdkField
. builder(MarshallingType.SDK_POJO)
.memberName("DatabaseSourceAuthenticationConfiguration")
.getter(getter(DatabaseSourceDescription::databaseSourceAuthenticationConfiguration))
.setter(setter(Builder::databaseSourceAuthenticationConfiguration))
.constructor(DatabaseSourceAuthenticationConfiguration::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("DatabaseSourceAuthenticationConfiguration").build()).build();
private static final SdkField DATABASE_SOURCE_VPC_CONFIGURATION_FIELD = SdkField
. builder(MarshallingType.SDK_POJO)
.memberName("DatabaseSourceVPCConfiguration")
.getter(getter(DatabaseSourceDescription::databaseSourceVPCConfiguration))
.setter(setter(Builder::databaseSourceVPCConfiguration))
.constructor(DatabaseSourceVPCConfiguration::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("DatabaseSourceVPCConfiguration")
.build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(TYPE_FIELD, ENDPOINT_FIELD,
PORT_FIELD, SSL_MODE_FIELD, DATABASES_FIELD, TABLES_FIELD, COLUMNS_FIELD, SURROGATE_KEYS_FIELD,
SNAPSHOT_WATERMARK_TABLE_FIELD, SNAPSHOT_INFO_FIELD, DATABASE_SOURCE_AUTHENTICATION_CONFIGURATION_FIELD,
DATABASE_SOURCE_VPC_CONFIGURATION_FIELD));
private static final Map> SDK_NAME_TO_FIELD = Collections
.unmodifiableMap(new HashMap>() {
{
put("Type", TYPE_FIELD);
put("Endpoint", ENDPOINT_FIELD);
put("Port", PORT_FIELD);
put("SSLMode", SSL_MODE_FIELD);
put("Databases", DATABASES_FIELD);
put("Tables", TABLES_FIELD);
put("Columns", COLUMNS_FIELD);
put("SurrogateKeys", SURROGATE_KEYS_FIELD);
put("SnapshotWatermarkTable", SNAPSHOT_WATERMARK_TABLE_FIELD);
put("SnapshotInfo", SNAPSHOT_INFO_FIELD);
put("DatabaseSourceAuthenticationConfiguration", DATABASE_SOURCE_AUTHENTICATION_CONFIGURATION_FIELD);
put("DatabaseSourceVPCConfiguration", DATABASE_SOURCE_VPC_CONFIGURATION_FIELD);
}
});
private static final long serialVersionUID = 1L;
private final String type;
private final String endpoint;
private final Integer port;
private final String sslMode;
private final DatabaseList databases;
private final DatabaseTableList tables;
private final DatabaseColumnList columns;
private final List surrogateKeys;
private final String snapshotWatermarkTable;
private final List snapshotInfo;
private final DatabaseSourceAuthenticationConfiguration databaseSourceAuthenticationConfiguration;
private final DatabaseSourceVPCConfiguration databaseSourceVPCConfiguration;
private DatabaseSourceDescription(BuilderImpl builder) {
this.type = builder.type;
this.endpoint = builder.endpoint;
this.port = builder.port;
this.sslMode = builder.sslMode;
this.databases = builder.databases;
this.tables = builder.tables;
this.columns = builder.columns;
this.surrogateKeys = builder.surrogateKeys;
this.snapshotWatermarkTable = builder.snapshotWatermarkTable;
this.snapshotInfo = builder.snapshotInfo;
this.databaseSourceAuthenticationConfiguration = builder.databaseSourceAuthenticationConfiguration;
this.databaseSourceVPCConfiguration = builder.databaseSourceVPCConfiguration;
}
/**
*
*
*
* Amazon Data Firehose is in preview release and is subject to change.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #type} will return
* {@link DatabaseType#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #typeAsString}.
*
*
* @return
*
* Amazon Data Firehose is in preview release and is subject to change.
* @see DatabaseType
*/
public final DatabaseType type() {
return DatabaseType.fromValue(type);
}
/**
*
*
*
* Amazon Data Firehose is in preview release and is subject to change.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #type} will return
* {@link DatabaseType#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #typeAsString}.
*
*
* @return
*
* Amazon Data Firehose is in preview release and is subject to change.
* @see DatabaseType
*/
public final String typeAsString() {
return type;
}
/**
*
*
*
* Amazon Data Firehose is in preview release and is subject to change.
*
*
* @return
*
* Amazon Data Firehose is in preview release and is subject to change.
*/
public final String endpoint() {
return endpoint;
}
/**
*
*
*
* Amazon Data Firehose is in preview release and is subject to change.
*
*
* @return
*
* Amazon Data Firehose is in preview release and is subject to change.
*/
public final Integer port() {
return port;
}
/**
*
*
*
* Amazon Data Firehose is in preview release and is subject to change.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #sslMode} will
* return {@link SSLMode#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #sslModeAsString}.
*
*
* @return
*
* Amazon Data Firehose is in preview release and is subject to change.
* @see SSLMode
*/
public final SSLMode sslMode() {
return SSLMode.fromValue(sslMode);
}
/**
*
*
*
* Amazon Data Firehose is in preview release and is subject to change.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #sslMode} will
* return {@link SSLMode#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #sslModeAsString}.
*
*
* @return
*
* Amazon Data Firehose is in preview release and is subject to change.
* @see SSLMode
*/
public final String sslModeAsString() {
return sslMode;
}
/**
*
*
*
* Amazon Data Firehose is in preview release and is subject to change.
*
*
* @return
*
* Amazon Data Firehose is in preview release and is subject to change.
*/
public final DatabaseList databases() {
return databases;
}
/**
*
*
*
* Amazon Data Firehose is in preview release and is subject to change.
*
*
* @return
*
* Amazon Data Firehose is in preview release and is subject to change.
*/
public final DatabaseTableList tables() {
return tables;
}
/**
*
*
*
* Amazon Data Firehose is in preview release and is subject to change.
*
*
* @return
*
* Amazon Data Firehose is in preview release and is subject to change.
*/
public final DatabaseColumnList columns() {
return columns;
}
/**
* For responses, this returns true if the service returned a value for the SurrogateKeys property. This DOES NOT
* check that the value is non-empty (for which, you should check the {@code isEmpty()} method on the property).
* This is useful because the SDK will never return a null collection or map, but you may need to differentiate
* between the service returning nothing (or null) and the service returning an empty collection or map. For
* requests, this returns true if a value for the property was specified in the request builder, and false if a
* value was not specified.
*/
public final boolean hasSurrogateKeys() {
return surrogateKeys != null && !(surrogateKeys instanceof SdkAutoConstructList);
}
/**
*
*
*
* Amazon Data Firehose is in preview release and is subject to change.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasSurrogateKeys} method.
*
*
* @return
*
* Amazon Data Firehose is in preview release and is subject to change.
*/
public final List surrogateKeys() {
return surrogateKeys;
}
/**
*
*
*
* Amazon Data Firehose is in preview release and is subject to change.
*
*
* @return
*
* Amazon Data Firehose is in preview release and is subject to change.
*/
public final String snapshotWatermarkTable() {
return snapshotWatermarkTable;
}
/**
* For responses, this returns true if the service returned a value for the SnapshotInfo property. This DOES NOT
* check that the value is non-empty (for which, you should check the {@code isEmpty()} method on the property).
* This is useful because the SDK will never return a null collection or map, but you may need to differentiate
* between the service returning nothing (or null) and the service returning an empty collection or map. For
* requests, this returns true if a value for the property was specified in the request builder, and false if a
* value was not specified.
*/
public final boolean hasSnapshotInfo() {
return snapshotInfo != null && !(snapshotInfo instanceof SdkAutoConstructList);
}
/**
*
*
*
* Amazon Data Firehose is in preview release and is subject to change.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasSnapshotInfo} method.
*
*
* @return
*
* Amazon Data Firehose is in preview release and is subject to change.
*/
public final List snapshotInfo() {
return snapshotInfo;
}
/**
*
*
*
* Amazon Data Firehose is in preview release and is subject to change.
*
*
* @return
*
* Amazon Data Firehose is in preview release and is subject to change.
*/
public final DatabaseSourceAuthenticationConfiguration databaseSourceAuthenticationConfiguration() {
return databaseSourceAuthenticationConfiguration;
}
/**
*
*
*
* Amazon Data Firehose is in preview release and is subject to change.
*
*
* @return
*
* Amazon Data Firehose is in preview release and is subject to change.
*/
public final DatabaseSourceVPCConfiguration databaseSourceVPCConfiguration() {
return databaseSourceVPCConfiguration;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(typeAsString());
hashCode = 31 * hashCode + Objects.hashCode(endpoint());
hashCode = 31 * hashCode + Objects.hashCode(port());
hashCode = 31 * hashCode + Objects.hashCode(sslModeAsString());
hashCode = 31 * hashCode + Objects.hashCode(databases());
hashCode = 31 * hashCode + Objects.hashCode(tables());
hashCode = 31 * hashCode + Objects.hashCode(columns());
hashCode = 31 * hashCode + Objects.hashCode(hasSurrogateKeys() ? surrogateKeys() : null);
hashCode = 31 * hashCode + Objects.hashCode(snapshotWatermarkTable());
hashCode = 31 * hashCode + Objects.hashCode(hasSnapshotInfo() ? snapshotInfo() : null);
hashCode = 31 * hashCode + Objects.hashCode(databaseSourceAuthenticationConfiguration());
hashCode = 31 * hashCode + Objects.hashCode(databaseSourceVPCConfiguration());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof DatabaseSourceDescription)) {
return false;
}
DatabaseSourceDescription other = (DatabaseSourceDescription) obj;
return Objects.equals(typeAsString(), other.typeAsString()) && Objects.equals(endpoint(), other.endpoint())
&& Objects.equals(port(), other.port()) && Objects.equals(sslModeAsString(), other.sslModeAsString())
&& Objects.equals(databases(), other.databases()) && Objects.equals(tables(), other.tables())
&& Objects.equals(columns(), other.columns()) && hasSurrogateKeys() == other.hasSurrogateKeys()
&& Objects.equals(surrogateKeys(), other.surrogateKeys())
&& Objects.equals(snapshotWatermarkTable(), other.snapshotWatermarkTable())
&& hasSnapshotInfo() == other.hasSnapshotInfo() && Objects.equals(snapshotInfo(), other.snapshotInfo())
&& Objects.equals(databaseSourceAuthenticationConfiguration(), other.databaseSourceAuthenticationConfiguration())
&& Objects.equals(databaseSourceVPCConfiguration(), other.databaseSourceVPCConfiguration());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("DatabaseSourceDescription").add("Type", typeAsString()).add("Endpoint", endpoint())
.add("Port", port()).add("SSLMode", sslModeAsString()).add("Databases", databases()).add("Tables", tables())
.add("Columns", columns()).add("SurrogateKeys", hasSurrogateKeys() ? surrogateKeys() : null)
.add("SnapshotWatermarkTable", snapshotWatermarkTable())
.add("SnapshotInfo", hasSnapshotInfo() ? snapshotInfo() : null)
.add("DatabaseSourceAuthenticationConfiguration", databaseSourceAuthenticationConfiguration())
.add("DatabaseSourceVPCConfiguration", databaseSourceVPCConfiguration()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "Type":
return Optional.ofNullable(clazz.cast(typeAsString()));
case "Endpoint":
return Optional.ofNullable(clazz.cast(endpoint()));
case "Port":
return Optional.ofNullable(clazz.cast(port()));
case "SSLMode":
return Optional.ofNullable(clazz.cast(sslModeAsString()));
case "Databases":
return Optional.ofNullable(clazz.cast(databases()));
case "Tables":
return Optional.ofNullable(clazz.cast(tables()));
case "Columns":
return Optional.ofNullable(clazz.cast(columns()));
case "SurrogateKeys":
return Optional.ofNullable(clazz.cast(surrogateKeys()));
case "SnapshotWatermarkTable":
return Optional.ofNullable(clazz.cast(snapshotWatermarkTable()));
case "SnapshotInfo":
return Optional.ofNullable(clazz.cast(snapshotInfo()));
case "DatabaseSourceAuthenticationConfiguration":
return Optional.ofNullable(clazz.cast(databaseSourceAuthenticationConfiguration()));
case "DatabaseSourceVPCConfiguration":
return Optional.ofNullable(clazz.cast(databaseSourceVPCConfiguration()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
@Override
public final Map> sdkFieldNameToField() {
return SDK_NAME_TO_FIELD;
}
private static Function
*
* Amazon Data Firehose is in preview release and is subject to change.
* @see DatabaseType
* @return Returns a reference to this object so that method calls can be chained together.
* @see DatabaseType
*/
Builder type(String type);
/**
*
*
*
* Amazon Data Firehose is in preview release and is subject to change.
*
*
* @param type
*
*
* Amazon Data Firehose is in preview release and is subject to change.
* @see DatabaseType
* @return Returns a reference to this object so that method calls can be chained together.
* @see DatabaseType
*/
Builder type(DatabaseType type);
/**
*
*
*
* Amazon Data Firehose is in preview release and is subject to change.
*
*
* @param endpoint
*
*
* Amazon Data Firehose is in preview release and is subject to change.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder endpoint(String endpoint);
/**
*
*
*
* Amazon Data Firehose is in preview release and is subject to change.
*
*
* @param port
*
*
* Amazon Data Firehose is in preview release and is subject to change.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder port(Integer port);
/**
*
*
*
* Amazon Data Firehose is in preview release and is subject to change.
*
*
* @param sslMode
*
*
* Amazon Data Firehose is in preview release and is subject to change.
* @see SSLMode
* @return Returns a reference to this object so that method calls can be chained together.
* @see SSLMode
*/
Builder sslMode(String sslMode);
/**
*
*
*
* Amazon Data Firehose is in preview release and is subject to change.
*
*
* @param sslMode
*
*
* Amazon Data Firehose is in preview release and is subject to change.
* @see SSLMode
* @return Returns a reference to this object so that method calls can be chained together.
* @see SSLMode
*/
Builder sslMode(SSLMode sslMode);
/**
*
*
*
* Amazon Data Firehose is in preview release and is subject to change.
*
*
* @param databases
*
*
* Amazon Data Firehose is in preview release and is subject to change.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder databases(DatabaseList databases);
/**
*
*
*
* Amazon Data Firehose is in preview release and is subject to change.
*
* This is a convenience method that creates an instance of the {@link DatabaseList.Builder} avoiding the need
* to create one manually via {@link DatabaseList#builder()}.
*
*
* When the {@link Consumer} completes, {@link DatabaseList.Builder#build()} is called immediately and its
* result is passed to {@link #databases(DatabaseList)}.
*
* @param databases
* a consumer that will call methods on {@link DatabaseList.Builder}
* @return Returns a reference to this object so that method calls can be chained together.
* @see #databases(DatabaseList)
*/
default Builder databases(Consumer databases) {
return databases(DatabaseList.builder().applyMutation(databases).build());
}
/**
*
*
*
* Amazon Data Firehose is in preview release and is subject to change.
*
*
* @param tables
*
*
* Amazon Data Firehose is in preview release and is subject to change.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder tables(DatabaseTableList tables);
/**
*
*
*
* Amazon Data Firehose is in preview release and is subject to change.
*
* This is a convenience method that creates an instance of the {@link DatabaseTableList.Builder} avoiding the
* need to create one manually via {@link DatabaseTableList#builder()}.
*
*
* When the {@link Consumer} completes, {@link DatabaseTableList.Builder#build()} is called immediately and its
* result is passed to {@link #tables(DatabaseTableList)}.
*
* @param tables
* a consumer that will call methods on {@link DatabaseTableList.Builder}
* @return Returns a reference to this object so that method calls can be chained together.
* @see #tables(DatabaseTableList)
*/
default Builder tables(Consumer tables) {
return tables(DatabaseTableList.builder().applyMutation(tables).build());
}
/**
*
*
*
* Amazon Data Firehose is in preview release and is subject to change.
*
*
* @param columns
*
*
* Amazon Data Firehose is in preview release and is subject to change.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder columns(DatabaseColumnList columns);
/**
*
*
*
* Amazon Data Firehose is in preview release and is subject to change.
*
* This is a convenience method that creates an instance of the {@link DatabaseColumnList.Builder} avoiding the
* need to create one manually via {@link DatabaseColumnList#builder()}.
*
*
* When the {@link Consumer} completes, {@link DatabaseColumnList.Builder#build()} is called immediately and its
* result is passed to {@link #columns(DatabaseColumnList)}.
*
* @param columns
* a consumer that will call methods on {@link DatabaseColumnList.Builder}
* @return Returns a reference to this object so that method calls can be chained together.
* @see #columns(DatabaseColumnList)
*/
default Builder columns(Consumer columns) {
return columns(DatabaseColumnList.builder().applyMutation(columns).build());
}
/**
*
*
*
* Amazon Data Firehose is in preview release and is subject to change.
*
*
* @param surrogateKeys
*
*
* Amazon Data Firehose is in preview release and is subject to change.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder surrogateKeys(Collection surrogateKeys);
/**
*
*
*
* Amazon Data Firehose is in preview release and is subject to change.
*
*
* @param surrogateKeys
*
*
* Amazon Data Firehose is in preview release and is subject to change.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder surrogateKeys(String... surrogateKeys);
/**
*
*
*
* Amazon Data Firehose is in preview release and is subject to change.
*
*
* @param snapshotWatermarkTable
*
*
* Amazon Data Firehose is in preview release and is subject to change.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder snapshotWatermarkTable(String snapshotWatermarkTable);
/**
*
*
*
* Amazon Data Firehose is in preview release and is subject to change.
*
*
* @param snapshotInfo
*
*
* Amazon Data Firehose is in preview release and is subject to change.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder snapshotInfo(Collection snapshotInfo);
/**
*
*
*
* Amazon Data Firehose is in preview release and is subject to change.
*
*
* @param snapshotInfo
*
*
* Amazon Data Firehose is in preview release and is subject to change.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder snapshotInfo(DatabaseSnapshotInfo... snapshotInfo);
/**
*
*
*
* Amazon Data Firehose is in preview release and is subject to change.
*
* This is a convenience method that creates an instance of the
* {@link software.amazon.awssdk.services.firehose.model.DatabaseSnapshotInfo.Builder} avoiding the need to
* create one manually via {@link software.amazon.awssdk.services.firehose.model.DatabaseSnapshotInfo#builder()}
* .
*
*
* When the {@link Consumer} completes,
* {@link software.amazon.awssdk.services.firehose.model.DatabaseSnapshotInfo.Builder#build()} is called
* immediately and its result is passed to {@link #snapshotInfo(List)}.
*
* @param snapshotInfo
* a consumer that will call methods on
* {@link software.amazon.awssdk.services.firehose.model.DatabaseSnapshotInfo.Builder}
* @return Returns a reference to this object so that method calls can be chained together.
* @see #snapshotInfo(java.util.Collection)
*/
Builder snapshotInfo(Consumer... snapshotInfo);
/**
*
*
*
* Amazon Data Firehose is in preview release and is subject to change.
*
*
* @param databaseSourceAuthenticationConfiguration
*
*
* Amazon Data Firehose is in preview release and is subject to change.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder databaseSourceAuthenticationConfiguration(
DatabaseSourceAuthenticationConfiguration databaseSourceAuthenticationConfiguration);
/**
*
*
*
* Amazon Data Firehose is in preview release and is subject to change.
*
* This is a convenience method that creates an instance of the
* {@link DatabaseSourceAuthenticationConfiguration.Builder} avoiding the need to create one manually via
* {@link DatabaseSourceAuthenticationConfiguration#builder()}.
*
*
* When the {@link Consumer} completes, {@link DatabaseSourceAuthenticationConfiguration.Builder#build()} is
* called immediately and its result is passed to
* {@link #databaseSourceAuthenticationConfiguration(DatabaseSourceAuthenticationConfiguration)}.
*
* @param databaseSourceAuthenticationConfiguration
* a consumer that will call methods on {@link DatabaseSourceAuthenticationConfiguration.Builder}
* @return Returns a reference to this object so that method calls can be chained together.
* @see #databaseSourceAuthenticationConfiguration(DatabaseSourceAuthenticationConfiguration)
*/
default Builder databaseSourceAuthenticationConfiguration(
Consumer databaseSourceAuthenticationConfiguration) {
return databaseSourceAuthenticationConfiguration(DatabaseSourceAuthenticationConfiguration.builder()
.applyMutation(databaseSourceAuthenticationConfiguration).build());
}
/**
*
*
*
* Amazon Data Firehose is in preview release and is subject to change.
*
*
* @param databaseSourceVPCConfiguration
*
*
* Amazon Data Firehose is in preview release and is subject to change.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder databaseSourceVPCConfiguration(DatabaseSourceVPCConfiguration databaseSourceVPCConfiguration);
/**
*
*
*
* Amazon Data Firehose is in preview release and is subject to change.
*
* This is a convenience method that creates an instance of the {@link DatabaseSourceVPCConfiguration.Builder}
* avoiding the need to create one manually via {@link DatabaseSourceVPCConfiguration#builder()}.
*
*
* When the {@link Consumer} completes, {@link DatabaseSourceVPCConfiguration.Builder#build()} is called
* immediately and its result is passed to
* {@link #databaseSourceVPCConfiguration(DatabaseSourceVPCConfiguration)}.
*
* @param databaseSourceVPCConfiguration
* a consumer that will call methods on {@link DatabaseSourceVPCConfiguration.Builder}
* @return Returns a reference to this object so that method calls can be chained together.
* @see #databaseSourceVPCConfiguration(DatabaseSourceVPCConfiguration)
*/
default Builder databaseSourceVPCConfiguration(
Consumer databaseSourceVPCConfiguration) {
return databaseSourceVPCConfiguration(DatabaseSourceVPCConfiguration.builder()
.applyMutation(databaseSourceVPCConfiguration).build());
}
}
static final class BuilderImpl implements Builder {
private String type;
private String endpoint;
private Integer port;
private String sslMode;
private DatabaseList databases;
private DatabaseTableList tables;
private DatabaseColumnList columns;
private List surrogateKeys = DefaultSdkAutoConstructList.getInstance();
private String snapshotWatermarkTable;
private List snapshotInfo = DefaultSdkAutoConstructList.getInstance();
private DatabaseSourceAuthenticationConfiguration databaseSourceAuthenticationConfiguration;
private DatabaseSourceVPCConfiguration databaseSourceVPCConfiguration;
private BuilderImpl() {
}
private BuilderImpl(DatabaseSourceDescription model) {
type(model.type);
endpoint(model.endpoint);
port(model.port);
sslMode(model.sslMode);
databases(model.databases);
tables(model.tables);
columns(model.columns);
surrogateKeys(model.surrogateKeys);
snapshotWatermarkTable(model.snapshotWatermarkTable);
snapshotInfo(model.snapshotInfo);
databaseSourceAuthenticationConfiguration(model.databaseSourceAuthenticationConfiguration);
databaseSourceVPCConfiguration(model.databaseSourceVPCConfiguration);
}
public final String getType() {
return type;
}
public final void setType(String type) {
this.type = type;
}
@Override
public final Builder type(String type) {
this.type = type;
return this;
}
@Override
public final Builder type(DatabaseType type) {
this.type(type == null ? null : type.toString());
return this;
}
public final String getEndpoint() {
return endpoint;
}
public final void setEndpoint(String endpoint) {
this.endpoint = endpoint;
}
@Override
public final Builder endpoint(String endpoint) {
this.endpoint = endpoint;
return this;
}
public final Integer getPort() {
return port;
}
public final void setPort(Integer port) {
this.port = port;
}
@Override
public final Builder port(Integer port) {
this.port = port;
return this;
}
public final String getSslMode() {
return sslMode;
}
public final void setSslMode(String sslMode) {
this.sslMode = sslMode;
}
@Override
public final Builder sslMode(String sslMode) {
this.sslMode = sslMode;
return this;
}
@Override
public final Builder sslMode(SSLMode sslMode) {
this.sslMode(sslMode == null ? null : sslMode.toString());
return this;
}
public final DatabaseList.Builder getDatabases() {
return databases != null ? databases.toBuilder() : null;
}
public final void setDatabases(DatabaseList.BuilderImpl databases) {
this.databases = databases != null ? databases.build() : null;
}
@Override
public final Builder databases(DatabaseList databases) {
this.databases = databases;
return this;
}
public final DatabaseTableList.Builder getTables() {
return tables != null ? tables.toBuilder() : null;
}
public final void setTables(DatabaseTableList.BuilderImpl tables) {
this.tables = tables != null ? tables.build() : null;
}
@Override
public final Builder tables(DatabaseTableList tables) {
this.tables = tables;
return this;
}
public final DatabaseColumnList.Builder getColumns() {
return columns != null ? columns.toBuilder() : null;
}
public final void setColumns(DatabaseColumnList.BuilderImpl columns) {
this.columns = columns != null ? columns.build() : null;
}
@Override
public final Builder columns(DatabaseColumnList columns) {
this.columns = columns;
return this;
}
public final Collection getSurrogateKeys() {
if (surrogateKeys instanceof SdkAutoConstructList) {
return null;
}
return surrogateKeys;
}
public final void setSurrogateKeys(Collection surrogateKeys) {
this.surrogateKeys = DatabaseColumnIncludeOrExcludeListCopier.copy(surrogateKeys);
}
@Override
public final Builder surrogateKeys(Collection surrogateKeys) {
this.surrogateKeys = DatabaseColumnIncludeOrExcludeListCopier.copy(surrogateKeys);
return this;
}
@Override
@SafeVarargs
public final Builder surrogateKeys(String... surrogateKeys) {
surrogateKeys(Arrays.asList(surrogateKeys));
return this;
}
public final String getSnapshotWatermarkTable() {
return snapshotWatermarkTable;
}
public final void setSnapshotWatermarkTable(String snapshotWatermarkTable) {
this.snapshotWatermarkTable = snapshotWatermarkTable;
}
@Override
public final Builder snapshotWatermarkTable(String snapshotWatermarkTable) {
this.snapshotWatermarkTable = snapshotWatermarkTable;
return this;
}
public final List getSnapshotInfo() {
List result = DatabaseSnapshotInfoListCopier.copyToBuilder(this.snapshotInfo);
if (result instanceof SdkAutoConstructList) {
return null;
}
return result;
}
public final void setSnapshotInfo(Collection snapshotInfo) {
this.snapshotInfo = DatabaseSnapshotInfoListCopier.copyFromBuilder(snapshotInfo);
}
@Override
public final Builder snapshotInfo(Collection snapshotInfo) {
this.snapshotInfo = DatabaseSnapshotInfoListCopier.copy(snapshotInfo);
return this;
}
@Override
@SafeVarargs
public final Builder snapshotInfo(DatabaseSnapshotInfo... snapshotInfo) {
snapshotInfo(Arrays.asList(snapshotInfo));
return this;
}
@Override
@SafeVarargs
public final Builder snapshotInfo(Consumer... snapshotInfo) {
snapshotInfo(Stream.of(snapshotInfo).map(c -> DatabaseSnapshotInfo.builder().applyMutation(c).build())
.collect(Collectors.toList()));
return this;
}
public final DatabaseSourceAuthenticationConfiguration.Builder getDatabaseSourceAuthenticationConfiguration() {
return databaseSourceAuthenticationConfiguration != null ? databaseSourceAuthenticationConfiguration.toBuilder()
: null;
}
public final void setDatabaseSourceAuthenticationConfiguration(
DatabaseSourceAuthenticationConfiguration.BuilderImpl databaseSourceAuthenticationConfiguration) {
this.databaseSourceAuthenticationConfiguration = databaseSourceAuthenticationConfiguration != null ? databaseSourceAuthenticationConfiguration
.build() : null;
}
@Override
public final Builder databaseSourceAuthenticationConfiguration(
DatabaseSourceAuthenticationConfiguration databaseSourceAuthenticationConfiguration) {
this.databaseSourceAuthenticationConfiguration = databaseSourceAuthenticationConfiguration;
return this;
}
public final DatabaseSourceVPCConfiguration.Builder getDatabaseSourceVPCConfiguration() {
return databaseSourceVPCConfiguration != null ? databaseSourceVPCConfiguration.toBuilder() : null;
}
public final void setDatabaseSourceVPCConfiguration(
DatabaseSourceVPCConfiguration.BuilderImpl databaseSourceVPCConfiguration) {
this.databaseSourceVPCConfiguration = databaseSourceVPCConfiguration != null ? databaseSourceVPCConfiguration.build()
: null;
}
@Override
public final Builder databaseSourceVPCConfiguration(DatabaseSourceVPCConfiguration databaseSourceVPCConfiguration) {
this.databaseSourceVPCConfiguration = databaseSourceVPCConfiguration;
return this;
}
@Override
public DatabaseSourceDescription build() {
return new DatabaseSourceDescription(this);
}
@Override
public List> sdkFields() {
return SDK_FIELDS;
}
@Override
public Map> sdkFieldNameToField() {
return SDK_NAME_TO_FIELD;
}
}
}