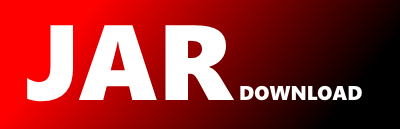
software.amazon.awssdk.services.fis.FisAsyncClient Maven / Gradle / Ivy
Show all versions of fis Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.fis;
import java.util.concurrent.CompletableFuture;
import java.util.function.Consumer;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.annotations.SdkPublicApi;
import software.amazon.awssdk.annotations.ThreadSafe;
import software.amazon.awssdk.awscore.AwsClient;
import software.amazon.awssdk.services.fis.model.CreateExperimentTemplateRequest;
import software.amazon.awssdk.services.fis.model.CreateExperimentTemplateResponse;
import software.amazon.awssdk.services.fis.model.DeleteExperimentTemplateRequest;
import software.amazon.awssdk.services.fis.model.DeleteExperimentTemplateResponse;
import software.amazon.awssdk.services.fis.model.GetActionRequest;
import software.amazon.awssdk.services.fis.model.GetActionResponse;
import software.amazon.awssdk.services.fis.model.GetExperimentRequest;
import software.amazon.awssdk.services.fis.model.GetExperimentResponse;
import software.amazon.awssdk.services.fis.model.GetExperimentTemplateRequest;
import software.amazon.awssdk.services.fis.model.GetExperimentTemplateResponse;
import software.amazon.awssdk.services.fis.model.GetTargetResourceTypeRequest;
import software.amazon.awssdk.services.fis.model.GetTargetResourceTypeResponse;
import software.amazon.awssdk.services.fis.model.ListActionsRequest;
import software.amazon.awssdk.services.fis.model.ListActionsResponse;
import software.amazon.awssdk.services.fis.model.ListExperimentTemplatesRequest;
import software.amazon.awssdk.services.fis.model.ListExperimentTemplatesResponse;
import software.amazon.awssdk.services.fis.model.ListExperimentsRequest;
import software.amazon.awssdk.services.fis.model.ListExperimentsResponse;
import software.amazon.awssdk.services.fis.model.ListTagsForResourceRequest;
import software.amazon.awssdk.services.fis.model.ListTagsForResourceResponse;
import software.amazon.awssdk.services.fis.model.ListTargetResourceTypesRequest;
import software.amazon.awssdk.services.fis.model.ListTargetResourceTypesResponse;
import software.amazon.awssdk.services.fis.model.StartExperimentRequest;
import software.amazon.awssdk.services.fis.model.StartExperimentResponse;
import software.amazon.awssdk.services.fis.model.StopExperimentRequest;
import software.amazon.awssdk.services.fis.model.StopExperimentResponse;
import software.amazon.awssdk.services.fis.model.TagResourceRequest;
import software.amazon.awssdk.services.fis.model.TagResourceResponse;
import software.amazon.awssdk.services.fis.model.UntagResourceRequest;
import software.amazon.awssdk.services.fis.model.UntagResourceResponse;
import software.amazon.awssdk.services.fis.model.UpdateExperimentTemplateRequest;
import software.amazon.awssdk.services.fis.model.UpdateExperimentTemplateResponse;
import software.amazon.awssdk.services.fis.paginators.ListActionsPublisher;
import software.amazon.awssdk.services.fis.paginators.ListExperimentTemplatesPublisher;
import software.amazon.awssdk.services.fis.paginators.ListExperimentsPublisher;
import software.amazon.awssdk.services.fis.paginators.ListTargetResourceTypesPublisher;
/**
* Service client for accessing FIS asynchronously. This can be created using the static {@link #builder()} method.
*
*
* Fault Injection Simulator is a managed service that enables you to perform fault injection experiments on your Amazon
* Web Services workloads. For more information, see the Fault Injection Simulator User Guide.
*
*/
@Generated("software.amazon.awssdk:codegen")
@SdkPublicApi
@ThreadSafe
public interface FisAsyncClient extends AwsClient {
String SERVICE_NAME = "fis";
/**
* Value for looking up the service's metadata from the
* {@link software.amazon.awssdk.regions.ServiceMetadataProvider}.
*/
String SERVICE_METADATA_ID = "fis";
/**
*
* Creates an experiment template.
*
*
* An experiment template includes the following components:
*
*
* -
*
* Targets: A target can be a specific resource in your Amazon Web Services environment, or one or more
* resources that match criteria that you specify, for example, resources that have specific tags.
*
*
* -
*
* Actions: The actions to carry out on the target. You can specify multiple actions, the duration of each
* action, and when to start each action during an experiment.
*
*
* -
*
* Stop conditions: If a stop condition is triggered while an experiment is running, the experiment is
* automatically stopped. You can define a stop condition as a CloudWatch alarm.
*
*
*
*
* For more information, see Experiment templates in the
* Fault Injection Simulator User Guide.
*
*
* @param createExperimentTemplateRequest
* @return A Java Future containing the result of the CreateExperimentTemplate operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException The specified input is not valid, or fails to satisfy the constraints for the
* request.
* - ConflictException The request could not be processed because of a conflict.
* - ResourceNotFoundException The specified resource cannot be found.
* - ServiceQuotaExceededException You have exceeded your service quota.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - FisException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample FisAsyncClient.CreateExperimentTemplate
* @see AWS
* API Documentation
*/
default CompletableFuture createExperimentTemplate(
CreateExperimentTemplateRequest createExperimentTemplateRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Creates an experiment template.
*
*
* An experiment template includes the following components:
*
*
* -
*
* Targets: A target can be a specific resource in your Amazon Web Services environment, or one or more
* resources that match criteria that you specify, for example, resources that have specific tags.
*
*
* -
*
* Actions: The actions to carry out on the target. You can specify multiple actions, the duration of each
* action, and when to start each action during an experiment.
*
*
* -
*
* Stop conditions: If a stop condition is triggered while an experiment is running, the experiment is
* automatically stopped. You can define a stop condition as a CloudWatch alarm.
*
*
*
*
* For more information, see Experiment templates in the
* Fault Injection Simulator User Guide.
*
*
*
* This is a convenience which creates an instance of the {@link CreateExperimentTemplateRequest.Builder} avoiding
* the need to create one manually via {@link CreateExperimentTemplateRequest#builder()}
*
*
* @param createExperimentTemplateRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.fis.model.CreateExperimentTemplateRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the CreateExperimentTemplate operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException The specified input is not valid, or fails to satisfy the constraints for the
* request.
* - ConflictException The request could not be processed because of a conflict.
* - ResourceNotFoundException The specified resource cannot be found.
* - ServiceQuotaExceededException You have exceeded your service quota.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - FisException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample FisAsyncClient.CreateExperimentTemplate
* @see AWS
* API Documentation
*/
default CompletableFuture createExperimentTemplate(
Consumer createExperimentTemplateRequest) {
return createExperimentTemplate(CreateExperimentTemplateRequest.builder().applyMutation(createExperimentTemplateRequest)
.build());
}
/**
*
* Deletes the specified experiment template.
*
*
* @param deleteExperimentTemplateRequest
* @return A Java Future containing the result of the DeleteExperimentTemplate operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException The specified input is not valid, or fails to satisfy the constraints for the
* request.
* - ResourceNotFoundException The specified resource cannot be found.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - FisException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample FisAsyncClient.DeleteExperimentTemplate
* @see AWS
* API Documentation
*/
default CompletableFuture deleteExperimentTemplate(
DeleteExperimentTemplateRequest deleteExperimentTemplateRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Deletes the specified experiment template.
*
*
*
* This is a convenience which creates an instance of the {@link DeleteExperimentTemplateRequest.Builder} avoiding
* the need to create one manually via {@link DeleteExperimentTemplateRequest#builder()}
*
*
* @param deleteExperimentTemplateRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.fis.model.DeleteExperimentTemplateRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the DeleteExperimentTemplate operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException The specified input is not valid, or fails to satisfy the constraints for the
* request.
* - ResourceNotFoundException The specified resource cannot be found.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - FisException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample FisAsyncClient.DeleteExperimentTemplate
* @see AWS
* API Documentation
*/
default CompletableFuture deleteExperimentTemplate(
Consumer deleteExperimentTemplateRequest) {
return deleteExperimentTemplate(DeleteExperimentTemplateRequest.builder().applyMutation(deleteExperimentTemplateRequest)
.build());
}
/**
*
* Gets information about the specified FIS action.
*
*
* @param getActionRequest
* @return A Java Future containing the result of the GetAction operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException The specified input is not valid, or fails to satisfy the constraints for the
* request.
* - ResourceNotFoundException The specified resource cannot be found.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - FisException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample FisAsyncClient.GetAction
* @see AWS API
* Documentation
*/
default CompletableFuture getAction(GetActionRequest getActionRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Gets information about the specified FIS action.
*
*
*
* This is a convenience which creates an instance of the {@link GetActionRequest.Builder} avoiding the need to
* create one manually via {@link GetActionRequest#builder()}
*
*
* @param getActionRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.fis.model.GetActionRequest.Builder} to create a request.
* @return A Java Future containing the result of the GetAction operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException The specified input is not valid, or fails to satisfy the constraints for the
* request.
* - ResourceNotFoundException The specified resource cannot be found.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - FisException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample FisAsyncClient.GetAction
* @see AWS API
* Documentation
*/
default CompletableFuture getAction(Consumer getActionRequest) {
return getAction(GetActionRequest.builder().applyMutation(getActionRequest).build());
}
/**
*
* Gets information about the specified experiment.
*
*
* @param getExperimentRequest
* @return A Java Future containing the result of the GetExperiment operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException The specified input is not valid, or fails to satisfy the constraints for the
* request.
* - ResourceNotFoundException The specified resource cannot be found.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - FisException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample FisAsyncClient.GetExperiment
* @see AWS API
* Documentation
*/
default CompletableFuture getExperiment(GetExperimentRequest getExperimentRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Gets information about the specified experiment.
*
*
*
* This is a convenience which creates an instance of the {@link GetExperimentRequest.Builder} avoiding the need to
* create one manually via {@link GetExperimentRequest#builder()}
*
*
* @param getExperimentRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.fis.model.GetExperimentRequest.Builder} to create a request.
* @return A Java Future containing the result of the GetExperiment operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException The specified input is not valid, or fails to satisfy the constraints for the
* request.
* - ResourceNotFoundException The specified resource cannot be found.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - FisException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample FisAsyncClient.GetExperiment
* @see AWS API
* Documentation
*/
default CompletableFuture getExperiment(Consumer getExperimentRequest) {
return getExperiment(GetExperimentRequest.builder().applyMutation(getExperimentRequest).build());
}
/**
*
* Gets information about the specified experiment template.
*
*
* @param getExperimentTemplateRequest
* @return A Java Future containing the result of the GetExperimentTemplate operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException The specified input is not valid, or fails to satisfy the constraints for the
* request.
* - ResourceNotFoundException The specified resource cannot be found.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - FisException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample FisAsyncClient.GetExperimentTemplate
* @see AWS API
* Documentation
*/
default CompletableFuture getExperimentTemplate(
GetExperimentTemplateRequest getExperimentTemplateRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Gets information about the specified experiment template.
*
*
*
* This is a convenience which creates an instance of the {@link GetExperimentTemplateRequest.Builder} avoiding the
* need to create one manually via {@link GetExperimentTemplateRequest#builder()}
*
*
* @param getExperimentTemplateRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.fis.model.GetExperimentTemplateRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the GetExperimentTemplate operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException The specified input is not valid, or fails to satisfy the constraints for the
* request.
* - ResourceNotFoundException The specified resource cannot be found.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - FisException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample FisAsyncClient.GetExperimentTemplate
* @see AWS API
* Documentation
*/
default CompletableFuture getExperimentTemplate(
Consumer getExperimentTemplateRequest) {
return getExperimentTemplate(GetExperimentTemplateRequest.builder().applyMutation(getExperimentTemplateRequest).build());
}
/**
*
* Gets information about the specified resource type.
*
*
* @param getTargetResourceTypeRequest
* @return A Java Future containing the result of the GetTargetResourceType operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException The specified input is not valid, or fails to satisfy the constraints for the
* request.
* - ResourceNotFoundException The specified resource cannot be found.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - FisException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample FisAsyncClient.GetTargetResourceType
* @see AWS API
* Documentation
*/
default CompletableFuture getTargetResourceType(
GetTargetResourceTypeRequest getTargetResourceTypeRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Gets information about the specified resource type.
*
*
*
* This is a convenience which creates an instance of the {@link GetTargetResourceTypeRequest.Builder} avoiding the
* need to create one manually via {@link GetTargetResourceTypeRequest#builder()}
*
*
* @param getTargetResourceTypeRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.fis.model.GetTargetResourceTypeRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the GetTargetResourceType operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException The specified input is not valid, or fails to satisfy the constraints for the
* request.
* - ResourceNotFoundException The specified resource cannot be found.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - FisException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample FisAsyncClient.GetTargetResourceType
* @see AWS API
* Documentation
*/
default CompletableFuture getTargetResourceType(
Consumer getTargetResourceTypeRequest) {
return getTargetResourceType(GetTargetResourceTypeRequest.builder().applyMutation(getTargetResourceTypeRequest).build());
}
/**
*
* Lists the available FIS actions.
*
*
* @param listActionsRequest
* @return A Java Future containing the result of the ListActions operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException The specified input is not valid, or fails to satisfy the constraints for the
* request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - FisException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample FisAsyncClient.ListActions
* @see AWS API
* Documentation
*/
default CompletableFuture listActions(ListActionsRequest listActionsRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Lists the available FIS actions.
*
*
*
* This is a convenience which creates an instance of the {@link ListActionsRequest.Builder} avoiding the need to
* create one manually via {@link ListActionsRequest#builder()}
*
*
* @param listActionsRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.fis.model.ListActionsRequest.Builder} to create a request.
* @return A Java Future containing the result of the ListActions operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException The specified input is not valid, or fails to satisfy the constraints for the
* request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - FisException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample FisAsyncClient.ListActions
* @see AWS API
* Documentation
*/
default CompletableFuture listActions(Consumer listActionsRequest) {
return listActions(ListActionsRequest.builder().applyMutation(listActionsRequest).build());
}
/**
*
* Lists the available FIS actions.
*
*
*
* This is a variant of {@link #listActions(software.amazon.awssdk.services.fis.model.ListActionsRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.fis.paginators.ListActionsPublisher publisher = client.listActionsPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.fis.paginators.ListActionsPublisher publisher = client.listActionsPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.fis.model.ListActionsResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of maxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listActions(software.amazon.awssdk.services.fis.model.ListActionsRequest)} operation.
*
*
* @param listActionsRequest
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException The specified input is not valid, or fails to satisfy the constraints for the
* request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - FisException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample FisAsyncClient.ListActions
* @see AWS API
* Documentation
*/
default ListActionsPublisher listActionsPaginator(ListActionsRequest listActionsRequest) {
return new ListActionsPublisher(this, listActionsRequest);
}
/**
*
* Lists the available FIS actions.
*
*
*
* This is a variant of {@link #listActions(software.amazon.awssdk.services.fis.model.ListActionsRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.fis.paginators.ListActionsPublisher publisher = client.listActionsPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.fis.paginators.ListActionsPublisher publisher = client.listActionsPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.fis.model.ListActionsResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of maxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listActions(software.amazon.awssdk.services.fis.model.ListActionsRequest)} operation.
*
*
* This is a convenience which creates an instance of the {@link ListActionsRequest.Builder} avoiding the need to
* create one manually via {@link ListActionsRequest#builder()}
*
*
* @param listActionsRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.fis.model.ListActionsRequest.Builder} to create a request.
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException The specified input is not valid, or fails to satisfy the constraints for the
* request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - FisException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample FisAsyncClient.ListActions
* @see AWS API
* Documentation
*/
default ListActionsPublisher listActionsPaginator(Consumer listActionsRequest) {
return listActionsPaginator(ListActionsRequest.builder().applyMutation(listActionsRequest).build());
}
/**
*
* Lists your experiment templates.
*
*
* @param listExperimentTemplatesRequest
* @return A Java Future containing the result of the ListExperimentTemplates operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException The specified input is not valid, or fails to satisfy the constraints for the
* request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - FisException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample FisAsyncClient.ListExperimentTemplates
* @see AWS
* API Documentation
*/
default CompletableFuture listExperimentTemplates(
ListExperimentTemplatesRequest listExperimentTemplatesRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Lists your experiment templates.
*
*
*
* This is a convenience which creates an instance of the {@link ListExperimentTemplatesRequest.Builder} avoiding
* the need to create one manually via {@link ListExperimentTemplatesRequest#builder()}
*
*
* @param listExperimentTemplatesRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.fis.model.ListExperimentTemplatesRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the ListExperimentTemplates operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException The specified input is not valid, or fails to satisfy the constraints for the
* request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - FisException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample FisAsyncClient.ListExperimentTemplates
* @see AWS
* API Documentation
*/
default CompletableFuture listExperimentTemplates(
Consumer listExperimentTemplatesRequest) {
return listExperimentTemplates(ListExperimentTemplatesRequest.builder().applyMutation(listExperimentTemplatesRequest)
.build());
}
/**
*
* Lists your experiment templates.
*
*
*
* This is a variant of
* {@link #listExperimentTemplates(software.amazon.awssdk.services.fis.model.ListExperimentTemplatesRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.fis.paginators.ListExperimentTemplatesPublisher publisher = client.listExperimentTemplatesPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.fis.paginators.ListExperimentTemplatesPublisher publisher = client.listExperimentTemplatesPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.fis.model.ListExperimentTemplatesResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of maxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listExperimentTemplates(software.amazon.awssdk.services.fis.model.ListExperimentTemplatesRequest)}
* operation.
*
*
* @param listExperimentTemplatesRequest
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException The specified input is not valid, or fails to satisfy the constraints for the
* request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - FisException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample FisAsyncClient.ListExperimentTemplates
* @see AWS
* API Documentation
*/
default ListExperimentTemplatesPublisher listExperimentTemplatesPaginator(
ListExperimentTemplatesRequest listExperimentTemplatesRequest) {
return new ListExperimentTemplatesPublisher(this, listExperimentTemplatesRequest);
}
/**
*
* Lists your experiment templates.
*
*
*
* This is a variant of
* {@link #listExperimentTemplates(software.amazon.awssdk.services.fis.model.ListExperimentTemplatesRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.fis.paginators.ListExperimentTemplatesPublisher publisher = client.listExperimentTemplatesPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.fis.paginators.ListExperimentTemplatesPublisher publisher = client.listExperimentTemplatesPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.fis.model.ListExperimentTemplatesResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of maxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listExperimentTemplates(software.amazon.awssdk.services.fis.model.ListExperimentTemplatesRequest)}
* operation.
*
*
* This is a convenience which creates an instance of the {@link ListExperimentTemplatesRequest.Builder} avoiding
* the need to create one manually via {@link ListExperimentTemplatesRequest#builder()}
*
*
* @param listExperimentTemplatesRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.fis.model.ListExperimentTemplatesRequest.Builder} to create a
* request.
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException The specified input is not valid, or fails to satisfy the constraints for the
* request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - FisException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample FisAsyncClient.ListExperimentTemplates
* @see AWS
* API Documentation
*/
default ListExperimentTemplatesPublisher listExperimentTemplatesPaginator(
Consumer listExperimentTemplatesRequest) {
return listExperimentTemplatesPaginator(ListExperimentTemplatesRequest.builder()
.applyMutation(listExperimentTemplatesRequest).build());
}
/**
*
* Lists your experiments.
*
*
* @param listExperimentsRequest
* @return A Java Future containing the result of the ListExperiments operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException The specified input is not valid, or fails to satisfy the constraints for the
* request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - FisException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample FisAsyncClient.ListExperiments
* @see AWS API
* Documentation
*/
default CompletableFuture listExperiments(ListExperimentsRequest listExperimentsRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Lists your experiments.
*
*
*
* This is a convenience which creates an instance of the {@link ListExperimentsRequest.Builder} avoiding the need
* to create one manually via {@link ListExperimentsRequest#builder()}
*
*
* @param listExperimentsRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.fis.model.ListExperimentsRequest.Builder} to create a request.
* @return A Java Future containing the result of the ListExperiments operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException The specified input is not valid, or fails to satisfy the constraints for the
* request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - FisException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample FisAsyncClient.ListExperiments
* @see AWS API
* Documentation
*/
default CompletableFuture listExperiments(
Consumer listExperimentsRequest) {
return listExperiments(ListExperimentsRequest.builder().applyMutation(listExperimentsRequest).build());
}
/**
*
* Lists your experiments.
*
*
*
* This is a variant of {@link #listExperiments(software.amazon.awssdk.services.fis.model.ListExperimentsRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.fis.paginators.ListExperimentsPublisher publisher = client.listExperimentsPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.fis.paginators.ListExperimentsPublisher publisher = client.listExperimentsPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.fis.model.ListExperimentsResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of maxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listExperiments(software.amazon.awssdk.services.fis.model.ListExperimentsRequest)} operation.
*
*
* @param listExperimentsRequest
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException The specified input is not valid, or fails to satisfy the constraints for the
* request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - FisException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample FisAsyncClient.ListExperiments
* @see AWS API
* Documentation
*/
default ListExperimentsPublisher listExperimentsPaginator(ListExperimentsRequest listExperimentsRequest) {
return new ListExperimentsPublisher(this, listExperimentsRequest);
}
/**
*
* Lists your experiments.
*
*
*
* This is a variant of {@link #listExperiments(software.amazon.awssdk.services.fis.model.ListExperimentsRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.fis.paginators.ListExperimentsPublisher publisher = client.listExperimentsPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.fis.paginators.ListExperimentsPublisher publisher = client.listExperimentsPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.fis.model.ListExperimentsResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of maxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listExperiments(software.amazon.awssdk.services.fis.model.ListExperimentsRequest)} operation.
*
*
* This is a convenience which creates an instance of the {@link ListExperimentsRequest.Builder} avoiding the need
* to create one manually via {@link ListExperimentsRequest#builder()}
*
*
* @param listExperimentsRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.fis.model.ListExperimentsRequest.Builder} to create a request.
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException The specified input is not valid, or fails to satisfy the constraints for the
* request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - FisException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample FisAsyncClient.ListExperiments
* @see AWS API
* Documentation
*/
default ListExperimentsPublisher listExperimentsPaginator(Consumer listExperimentsRequest) {
return listExperimentsPaginator(ListExperimentsRequest.builder().applyMutation(listExperimentsRequest).build());
}
/**
*
* Lists the tags for the specified resource.
*
*
* @param listTagsForResourceRequest
* @return A Java Future containing the result of the ListTagsForResource operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - FisException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample FisAsyncClient.ListTagsForResource
* @see AWS API
* Documentation
*/
default CompletableFuture listTagsForResource(
ListTagsForResourceRequest listTagsForResourceRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Lists the tags for the specified resource.
*
*
*
* This is a convenience which creates an instance of the {@link ListTagsForResourceRequest.Builder} avoiding the
* need to create one manually via {@link ListTagsForResourceRequest#builder()}
*
*
* @param listTagsForResourceRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.fis.model.ListTagsForResourceRequest.Builder} to create a request.
* @return A Java Future containing the result of the ListTagsForResource operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - FisException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample FisAsyncClient.ListTagsForResource
* @see AWS API
* Documentation
*/
default CompletableFuture listTagsForResource(
Consumer listTagsForResourceRequest) {
return listTagsForResource(ListTagsForResourceRequest.builder().applyMutation(listTagsForResourceRequest).build());
}
/**
*
* Lists the target resource types.
*
*
* @param listTargetResourceTypesRequest
* @return A Java Future containing the result of the ListTargetResourceTypes operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException The specified input is not valid, or fails to satisfy the constraints for the
* request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - FisException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample FisAsyncClient.ListTargetResourceTypes
* @see AWS
* API Documentation
*/
default CompletableFuture listTargetResourceTypes(
ListTargetResourceTypesRequest listTargetResourceTypesRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Lists the target resource types.
*
*
*
* This is a convenience which creates an instance of the {@link ListTargetResourceTypesRequest.Builder} avoiding
* the need to create one manually via {@link ListTargetResourceTypesRequest#builder()}
*
*
* @param listTargetResourceTypesRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.fis.model.ListTargetResourceTypesRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the ListTargetResourceTypes operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException The specified input is not valid, or fails to satisfy the constraints for the
* request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - FisException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample FisAsyncClient.ListTargetResourceTypes
* @see AWS
* API Documentation
*/
default CompletableFuture listTargetResourceTypes(
Consumer listTargetResourceTypesRequest) {
return listTargetResourceTypes(ListTargetResourceTypesRequest.builder().applyMutation(listTargetResourceTypesRequest)
.build());
}
/**
*
* Lists the target resource types.
*
*
*
* This is a variant of
* {@link #listTargetResourceTypes(software.amazon.awssdk.services.fis.model.ListTargetResourceTypesRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.fis.paginators.ListTargetResourceTypesPublisher publisher = client.listTargetResourceTypesPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.fis.paginators.ListTargetResourceTypesPublisher publisher = client.listTargetResourceTypesPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.fis.model.ListTargetResourceTypesResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of maxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listTargetResourceTypes(software.amazon.awssdk.services.fis.model.ListTargetResourceTypesRequest)}
* operation.
*
*
* @param listTargetResourceTypesRequest
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException The specified input is not valid, or fails to satisfy the constraints for the
* request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - FisException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample FisAsyncClient.ListTargetResourceTypes
* @see AWS
* API Documentation
*/
default ListTargetResourceTypesPublisher listTargetResourceTypesPaginator(
ListTargetResourceTypesRequest listTargetResourceTypesRequest) {
return new ListTargetResourceTypesPublisher(this, listTargetResourceTypesRequest);
}
/**
*
* Lists the target resource types.
*
*
*
* This is a variant of
* {@link #listTargetResourceTypes(software.amazon.awssdk.services.fis.model.ListTargetResourceTypesRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.fis.paginators.ListTargetResourceTypesPublisher publisher = client.listTargetResourceTypesPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.fis.paginators.ListTargetResourceTypesPublisher publisher = client.listTargetResourceTypesPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.fis.model.ListTargetResourceTypesResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of maxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listTargetResourceTypes(software.amazon.awssdk.services.fis.model.ListTargetResourceTypesRequest)}
* operation.
*
*
* This is a convenience which creates an instance of the {@link ListTargetResourceTypesRequest.Builder} avoiding
* the need to create one manually via {@link ListTargetResourceTypesRequest#builder()}
*
*
* @param listTargetResourceTypesRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.fis.model.ListTargetResourceTypesRequest.Builder} to create a
* request.
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException The specified input is not valid, or fails to satisfy the constraints for the
* request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - FisException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample FisAsyncClient.ListTargetResourceTypes
* @see AWS
* API Documentation
*/
default ListTargetResourceTypesPublisher listTargetResourceTypesPaginator(
Consumer listTargetResourceTypesRequest) {
return listTargetResourceTypesPaginator(ListTargetResourceTypesRequest.builder()
.applyMutation(listTargetResourceTypesRequest).build());
}
/**
*
* Starts running an experiment from the specified experiment template.
*
*
* @param startExperimentRequest
* @return A Java Future containing the result of the StartExperiment operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException The specified input is not valid, or fails to satisfy the constraints for the
* request.
* - ConflictException The request could not be processed because of a conflict.
* - ResourceNotFoundException The specified resource cannot be found.
* - ServiceQuotaExceededException You have exceeded your service quota.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - FisException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample FisAsyncClient.StartExperiment
* @see AWS API
* Documentation
*/
default CompletableFuture startExperiment(StartExperimentRequest startExperimentRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Starts running an experiment from the specified experiment template.
*
*
*
* This is a convenience which creates an instance of the {@link StartExperimentRequest.Builder} avoiding the need
* to create one manually via {@link StartExperimentRequest#builder()}
*
*
* @param startExperimentRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.fis.model.StartExperimentRequest.Builder} to create a request.
* @return A Java Future containing the result of the StartExperiment operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException The specified input is not valid, or fails to satisfy the constraints for the
* request.
* - ConflictException The request could not be processed because of a conflict.
* - ResourceNotFoundException The specified resource cannot be found.
* - ServiceQuotaExceededException You have exceeded your service quota.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - FisException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample FisAsyncClient.StartExperiment
* @see AWS API
* Documentation
*/
default CompletableFuture startExperiment(
Consumer startExperimentRequest) {
return startExperiment(StartExperimentRequest.builder().applyMutation(startExperimentRequest).build());
}
/**
*
* Stops the specified experiment.
*
*
* @param stopExperimentRequest
* @return A Java Future containing the result of the StopExperiment operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException The specified input is not valid, or fails to satisfy the constraints for the
* request.
* - ResourceNotFoundException The specified resource cannot be found.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - FisException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample FisAsyncClient.StopExperiment
* @see AWS API
* Documentation
*/
default CompletableFuture stopExperiment(StopExperimentRequest stopExperimentRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Stops the specified experiment.
*
*
*
* This is a convenience which creates an instance of the {@link StopExperimentRequest.Builder} avoiding the need to
* create one manually via {@link StopExperimentRequest#builder()}
*
*
* @param stopExperimentRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.fis.model.StopExperimentRequest.Builder} to create a request.
* @return A Java Future containing the result of the StopExperiment operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException The specified input is not valid, or fails to satisfy the constraints for the
* request.
* - ResourceNotFoundException The specified resource cannot be found.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - FisException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample FisAsyncClient.StopExperiment
* @see AWS API
* Documentation
*/
default CompletableFuture stopExperiment(Consumer stopExperimentRequest) {
return stopExperiment(StopExperimentRequest.builder().applyMutation(stopExperimentRequest).build());
}
/**
*
* Applies the specified tags to the specified resource.
*
*
* @param tagResourceRequest
* @return A Java Future containing the result of the TagResource operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - FisException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample FisAsyncClient.TagResource
* @see AWS API
* Documentation
*/
default CompletableFuture tagResource(TagResourceRequest tagResourceRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Applies the specified tags to the specified resource.
*
*
*
* This is a convenience which creates an instance of the {@link TagResourceRequest.Builder} avoiding the need to
* create one manually via {@link TagResourceRequest#builder()}
*
*
* @param tagResourceRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.fis.model.TagResourceRequest.Builder} to create a request.
* @return A Java Future containing the result of the TagResource operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - FisException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample FisAsyncClient.TagResource
* @see AWS API
* Documentation
*/
default CompletableFuture tagResource(Consumer tagResourceRequest) {
return tagResource(TagResourceRequest.builder().applyMutation(tagResourceRequest).build());
}
/**
*
* Removes the specified tags from the specified resource.
*
*
* @param untagResourceRequest
* @return A Java Future containing the result of the UntagResource operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - FisException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample FisAsyncClient.UntagResource
* @see AWS API
* Documentation
*/
default CompletableFuture untagResource(UntagResourceRequest untagResourceRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Removes the specified tags from the specified resource.
*
*
*
* This is a convenience which creates an instance of the {@link UntagResourceRequest.Builder} avoiding the need to
* create one manually via {@link UntagResourceRequest#builder()}
*
*
* @param untagResourceRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.fis.model.UntagResourceRequest.Builder} to create a request.
* @return A Java Future containing the result of the UntagResource operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - FisException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample FisAsyncClient.UntagResource
* @see AWS API
* Documentation
*/
default CompletableFuture untagResource(Consumer untagResourceRequest) {
return untagResource(UntagResourceRequest.builder().applyMutation(untagResourceRequest).build());
}
/**
*
* Updates the specified experiment template.
*
*
* @param updateExperimentTemplateRequest
* @return A Java Future containing the result of the UpdateExperimentTemplate operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException The specified input is not valid, or fails to satisfy the constraints for the
* request.
* - ResourceNotFoundException The specified resource cannot be found.
* - ServiceQuotaExceededException You have exceeded your service quota.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - FisException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample FisAsyncClient.UpdateExperimentTemplate
* @see AWS
* API Documentation
*/
default CompletableFuture updateExperimentTemplate(
UpdateExperimentTemplateRequest updateExperimentTemplateRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Updates the specified experiment template.
*
*
*
* This is a convenience which creates an instance of the {@link UpdateExperimentTemplateRequest.Builder} avoiding
* the need to create one manually via {@link UpdateExperimentTemplateRequest#builder()}
*
*
* @param updateExperimentTemplateRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.fis.model.UpdateExperimentTemplateRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the UpdateExperimentTemplate operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException The specified input is not valid, or fails to satisfy the constraints for the
* request.
* - ResourceNotFoundException The specified resource cannot be found.
* - ServiceQuotaExceededException You have exceeded your service quota.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - FisException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample FisAsyncClient.UpdateExperimentTemplate
* @see AWS
* API Documentation
*/
default CompletableFuture updateExperimentTemplate(
Consumer updateExperimentTemplateRequest) {
return updateExperimentTemplate(UpdateExperimentTemplateRequest.builder().applyMutation(updateExperimentTemplateRequest)
.build());
}
@Override
default FisServiceClientConfiguration serviceClientConfiguration() {
throw new UnsupportedOperationException();
}
/**
* Create a {@link FisAsyncClient} with the region loaded from the
* {@link software.amazon.awssdk.regions.providers.DefaultAwsRegionProviderChain} and credentials loaded from the
* {@link software.amazon.awssdk.auth.credentials.DefaultCredentialsProvider}.
*/
static FisAsyncClient create() {
return builder().build();
}
/**
* Create a builder that can be used to configure and create a {@link FisAsyncClient}.
*/
static FisAsyncClientBuilder builder() {
return new DefaultFisAsyncClientBuilder();
}
}