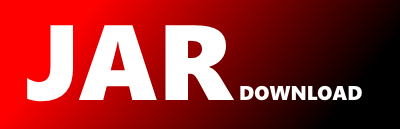
software.amazon.awssdk.services.fms.FmsAsyncClient Maven / Gradle / Ivy
Show all versions of fms Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.fms;
import java.util.concurrent.CompletableFuture;
import java.util.function.Consumer;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkClient;
import software.amazon.awssdk.services.fms.model.AssociateAdminAccountRequest;
import software.amazon.awssdk.services.fms.model.AssociateAdminAccountResponse;
import software.amazon.awssdk.services.fms.model.DeleteNotificationChannelRequest;
import software.amazon.awssdk.services.fms.model.DeleteNotificationChannelResponse;
import software.amazon.awssdk.services.fms.model.DeletePolicyRequest;
import software.amazon.awssdk.services.fms.model.DeletePolicyResponse;
import software.amazon.awssdk.services.fms.model.DisassociateAdminAccountRequest;
import software.amazon.awssdk.services.fms.model.DisassociateAdminAccountResponse;
import software.amazon.awssdk.services.fms.model.GetAdminAccountRequest;
import software.amazon.awssdk.services.fms.model.GetAdminAccountResponse;
import software.amazon.awssdk.services.fms.model.GetComplianceDetailRequest;
import software.amazon.awssdk.services.fms.model.GetComplianceDetailResponse;
import software.amazon.awssdk.services.fms.model.GetNotificationChannelRequest;
import software.amazon.awssdk.services.fms.model.GetNotificationChannelResponse;
import software.amazon.awssdk.services.fms.model.GetPolicyRequest;
import software.amazon.awssdk.services.fms.model.GetPolicyResponse;
import software.amazon.awssdk.services.fms.model.GetProtectionStatusRequest;
import software.amazon.awssdk.services.fms.model.GetProtectionStatusResponse;
import software.amazon.awssdk.services.fms.model.ListComplianceStatusRequest;
import software.amazon.awssdk.services.fms.model.ListComplianceStatusResponse;
import software.amazon.awssdk.services.fms.model.ListMemberAccountsRequest;
import software.amazon.awssdk.services.fms.model.ListMemberAccountsResponse;
import software.amazon.awssdk.services.fms.model.ListPoliciesRequest;
import software.amazon.awssdk.services.fms.model.ListPoliciesResponse;
import software.amazon.awssdk.services.fms.model.ListTagsForResourceRequest;
import software.amazon.awssdk.services.fms.model.ListTagsForResourceResponse;
import software.amazon.awssdk.services.fms.model.PutNotificationChannelRequest;
import software.amazon.awssdk.services.fms.model.PutNotificationChannelResponse;
import software.amazon.awssdk.services.fms.model.PutPolicyRequest;
import software.amazon.awssdk.services.fms.model.PutPolicyResponse;
import software.amazon.awssdk.services.fms.model.TagResourceRequest;
import software.amazon.awssdk.services.fms.model.TagResourceResponse;
import software.amazon.awssdk.services.fms.model.UntagResourceRequest;
import software.amazon.awssdk.services.fms.model.UntagResourceResponse;
import software.amazon.awssdk.services.fms.paginators.ListComplianceStatusPublisher;
import software.amazon.awssdk.services.fms.paginators.ListMemberAccountsPublisher;
import software.amazon.awssdk.services.fms.paginators.ListPoliciesPublisher;
/**
* Service client for accessing FMS asynchronously. This can be created using the static {@link #builder()} method.
*
* AWS Firewall Manager
*
* This is the AWS Firewall Manager API Reference. This guide is for developers who need detailed information
* about the AWS Firewall Manager API actions, data types, and errors. For detailed information about AWS Firewall
* Manager features, see the AWS
* Firewall Manager Developer Guide.
*
*/
@Generated("software.amazon.awssdk:codegen")
public interface FmsAsyncClient extends SdkClient {
String SERVICE_NAME = "fms";
/**
* Create a {@link FmsAsyncClient} with the region loaded from the
* {@link software.amazon.awssdk.regions.providers.DefaultAwsRegionProviderChain} and credentials loaded from the
* {@link software.amazon.awssdk.auth.credentials.DefaultCredentialsProvider}.
*/
static FmsAsyncClient create() {
return builder().build();
}
/**
* Create a builder that can be used to configure and create a {@link FmsAsyncClient}.
*/
static FmsAsyncClientBuilder builder() {
return new DefaultFmsAsyncClientBuilder();
}
/**
*
* Sets the AWS Firewall Manager administrator account. AWS Firewall Manager must be associated with the master
* account of your AWS organization or associated with a member account that has the appropriate permissions. If the
* account ID that you submit is not an AWS Organizations master account, AWS Firewall Manager will set the
* appropriate permissions for the given member account.
*
*
* The account that you associate with AWS Firewall Manager is called the AWS Firewall Manager administrator
* account.
*
*
* @param associateAdminAccountRequest
* @return A Java Future containing the result of the AssociateAdminAccount operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InvalidOperationException The operation failed because there was nothing to do. For example, you
* might have submitted an
AssociateAdminAccount
request, but the account ID that you submitted
* was already set as the AWS Firewall Manager administrator.
* - InvalidInputException The parameters of the request were invalid.
* - ResourceNotFoundException The specified resource was not found.
* - InternalErrorException The operation failed because of a system problem, even though the request was
* valid. Retry your request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - FmsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample FmsAsyncClient.AssociateAdminAccount
* @see AWS API
* Documentation
*/
default CompletableFuture associateAdminAccount(
AssociateAdminAccountRequest associateAdminAccountRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Sets the AWS Firewall Manager administrator account. AWS Firewall Manager must be associated with the master
* account of your AWS organization or associated with a member account that has the appropriate permissions. If the
* account ID that you submit is not an AWS Organizations master account, AWS Firewall Manager will set the
* appropriate permissions for the given member account.
*
*
* The account that you associate with AWS Firewall Manager is called the AWS Firewall Manager administrator
* account.
*
*
*
* This is a convenience which creates an instance of the {@link AssociateAdminAccountRequest.Builder} avoiding the
* need to create one manually via {@link AssociateAdminAccountRequest#builder()}
*
*
* @param associateAdminAccountRequest
* A {@link Consumer} that will call methods on {@link AssociateAdminAccountRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the AssociateAdminAccount operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InvalidOperationException The operation failed because there was nothing to do. For example, you
* might have submitted an
AssociateAdminAccount
request, but the account ID that you submitted
* was already set as the AWS Firewall Manager administrator.
* - InvalidInputException The parameters of the request were invalid.
* - ResourceNotFoundException The specified resource was not found.
* - InternalErrorException The operation failed because of a system problem, even though the request was
* valid. Retry your request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - FmsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample FmsAsyncClient.AssociateAdminAccount
* @see AWS API
* Documentation
*/
default CompletableFuture associateAdminAccount(
Consumer associateAdminAccountRequest) {
return associateAdminAccount(AssociateAdminAccountRequest.builder().applyMutation(associateAdminAccountRequest).build());
}
/**
*
* Deletes an AWS Firewall Manager association with the IAM role and the Amazon Simple Notification Service (SNS)
* topic that is used to record AWS Firewall Manager SNS logs.
*
*
* @param deleteNotificationChannelRequest
* @return A Java Future containing the result of the DeleteNotificationChannel operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ResourceNotFoundException The specified resource was not found.
* - InvalidOperationException The operation failed because there was nothing to do. For example, you
* might have submitted an
AssociateAdminAccount
request, but the account ID that you submitted
* was already set as the AWS Firewall Manager administrator.
* - InternalErrorException The operation failed because of a system problem, even though the request was
* valid. Retry your request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - FmsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample FmsAsyncClient.DeleteNotificationChannel
* @see AWS
* API Documentation
*/
default CompletableFuture deleteNotificationChannel(
DeleteNotificationChannelRequest deleteNotificationChannelRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Deletes an AWS Firewall Manager association with the IAM role and the Amazon Simple Notification Service (SNS)
* topic that is used to record AWS Firewall Manager SNS logs.
*
*
*
* This is a convenience which creates an instance of the {@link DeleteNotificationChannelRequest.Builder} avoiding
* the need to create one manually via {@link DeleteNotificationChannelRequest#builder()}
*
*
* @param deleteNotificationChannelRequest
* A {@link Consumer} that will call methods on {@link DeleteNotificationChannelRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the DeleteNotificationChannel operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ResourceNotFoundException The specified resource was not found.
* - InvalidOperationException The operation failed because there was nothing to do. For example, you
* might have submitted an
AssociateAdminAccount
request, but the account ID that you submitted
* was already set as the AWS Firewall Manager administrator.
* - InternalErrorException The operation failed because of a system problem, even though the request was
* valid. Retry your request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - FmsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample FmsAsyncClient.DeleteNotificationChannel
* @see AWS
* API Documentation
*/
default CompletableFuture deleteNotificationChannel(
Consumer deleteNotificationChannelRequest) {
return deleteNotificationChannel(DeleteNotificationChannelRequest.builder()
.applyMutation(deleteNotificationChannelRequest).build());
}
/**
*
* Permanently deletes an AWS Firewall Manager policy.
*
*
* @param deletePolicyRequest
* @return A Java Future containing the result of the DeletePolicy operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ResourceNotFoundException The specified resource was not found.
* - InvalidOperationException The operation failed because there was nothing to do. For example, you
* might have submitted an
AssociateAdminAccount
request, but the account ID that you submitted
* was already set as the AWS Firewall Manager administrator.
* - InternalErrorException The operation failed because of a system problem, even though the request was
* valid. Retry your request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - FmsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample FmsAsyncClient.DeletePolicy
* @see AWS API
* Documentation
*/
default CompletableFuture deletePolicy(DeletePolicyRequest deletePolicyRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Permanently deletes an AWS Firewall Manager policy.
*
*
*
* This is a convenience which creates an instance of the {@link DeletePolicyRequest.Builder} avoiding the need to
* create one manually via {@link DeletePolicyRequest#builder()}
*
*
* @param deletePolicyRequest
* A {@link Consumer} that will call methods on {@link DeletePolicyRequest.Builder} to create a request.
* @return A Java Future containing the result of the DeletePolicy operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ResourceNotFoundException The specified resource was not found.
* - InvalidOperationException The operation failed because there was nothing to do. For example, you
* might have submitted an
AssociateAdminAccount
request, but the account ID that you submitted
* was already set as the AWS Firewall Manager administrator.
* - InternalErrorException The operation failed because of a system problem, even though the request was
* valid. Retry your request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - FmsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample FmsAsyncClient.DeletePolicy
* @see AWS API
* Documentation
*/
default CompletableFuture deletePolicy(Consumer deletePolicyRequest) {
return deletePolicy(DeletePolicyRequest.builder().applyMutation(deletePolicyRequest).build());
}
/**
*
* Disassociates the account that has been set as the AWS Firewall Manager administrator account. To set a different
* account as the administrator account, you must submit an AssociateAdminAccount
request.
*
*
* @param disassociateAdminAccountRequest
* @return A Java Future containing the result of the DisassociateAdminAccount operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InvalidOperationException The operation failed because there was nothing to do. For example, you
* might have submitted an
AssociateAdminAccount
request, but the account ID that you submitted
* was already set as the AWS Firewall Manager administrator.
* - ResourceNotFoundException The specified resource was not found.
* - InternalErrorException The operation failed because of a system problem, even though the request was
* valid. Retry your request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - FmsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample FmsAsyncClient.DisassociateAdminAccount
* @see AWS
* API Documentation
*/
default CompletableFuture disassociateAdminAccount(
DisassociateAdminAccountRequest disassociateAdminAccountRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Disassociates the account that has been set as the AWS Firewall Manager administrator account. To set a different
* account as the administrator account, you must submit an AssociateAdminAccount
request.
*
*
*
* This is a convenience which creates an instance of the {@link DisassociateAdminAccountRequest.Builder} avoiding
* the need to create one manually via {@link DisassociateAdminAccountRequest#builder()}
*
*
* @param disassociateAdminAccountRequest
* A {@link Consumer} that will call methods on {@link DisassociateAdminAccountRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the DisassociateAdminAccount operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InvalidOperationException The operation failed because there was nothing to do. For example, you
* might have submitted an
AssociateAdminAccount
request, but the account ID that you submitted
* was already set as the AWS Firewall Manager administrator.
* - ResourceNotFoundException The specified resource was not found.
* - InternalErrorException The operation failed because of a system problem, even though the request was
* valid. Retry your request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - FmsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample FmsAsyncClient.DisassociateAdminAccount
* @see AWS
* API Documentation
*/
default CompletableFuture disassociateAdminAccount(
Consumer disassociateAdminAccountRequest) {
return disassociateAdminAccount(DisassociateAdminAccountRequest.builder().applyMutation(disassociateAdminAccountRequest)
.build());
}
/**
*
* Returns the AWS Organizations master account that is associated with AWS Firewall Manager as the AWS Firewall
* Manager administrator.
*
*
* @param getAdminAccountRequest
* @return A Java Future containing the result of the GetAdminAccount operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InvalidOperationException The operation failed because there was nothing to do. For example, you
* might have submitted an
AssociateAdminAccount
request, but the account ID that you submitted
* was already set as the AWS Firewall Manager administrator.
* - ResourceNotFoundException The specified resource was not found.
* - InternalErrorException The operation failed because of a system problem, even though the request was
* valid. Retry your request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - FmsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample FmsAsyncClient.GetAdminAccount
* @see AWS API
* Documentation
*/
default CompletableFuture getAdminAccount(GetAdminAccountRequest getAdminAccountRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Returns the AWS Organizations master account that is associated with AWS Firewall Manager as the AWS Firewall
* Manager administrator.
*
*
*
* This is a convenience which creates an instance of the {@link GetAdminAccountRequest.Builder} avoiding the need
* to create one manually via {@link GetAdminAccountRequest#builder()}
*
*
* @param getAdminAccountRequest
* A {@link Consumer} that will call methods on {@link GetAdminAccountRequest.Builder} to create a request.
* @return A Java Future containing the result of the GetAdminAccount operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InvalidOperationException The operation failed because there was nothing to do. For example, you
* might have submitted an
AssociateAdminAccount
request, but the account ID that you submitted
* was already set as the AWS Firewall Manager administrator.
* - ResourceNotFoundException The specified resource was not found.
* - InternalErrorException The operation failed because of a system problem, even though the request was
* valid. Retry your request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - FmsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample FmsAsyncClient.GetAdminAccount
* @see AWS API
* Documentation
*/
default CompletableFuture getAdminAccount(
Consumer getAdminAccountRequest) {
return getAdminAccount(GetAdminAccountRequest.builder().applyMutation(getAdminAccountRequest).build());
}
/**
*
* Returns detailed compliance information about the specified member account. Details include resources that are in
* and out of compliance with the specified policy. Resources are considered noncompliant for AWS WAF and Shield
* Advanced policies if the specified policy has not been applied to them. Resources are considered noncompliant for
* security group policies if they are in scope of the policy, they violate one or more of the policy rules, and
* remediation is disabled or not possible.
*
*
* @param getComplianceDetailRequest
* @return A Java Future containing the result of the GetComplianceDetail operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ResourceNotFoundException The specified resource was not found.
* - InternalErrorException The operation failed because of a system problem, even though the request was
* valid. Retry your request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - FmsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample FmsAsyncClient.GetComplianceDetail
* @see AWS API
* Documentation
*/
default CompletableFuture getComplianceDetail(
GetComplianceDetailRequest getComplianceDetailRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Returns detailed compliance information about the specified member account. Details include resources that are in
* and out of compliance with the specified policy. Resources are considered noncompliant for AWS WAF and Shield
* Advanced policies if the specified policy has not been applied to them. Resources are considered noncompliant for
* security group policies if they are in scope of the policy, they violate one or more of the policy rules, and
* remediation is disabled or not possible.
*
*
*
* This is a convenience which creates an instance of the {@link GetComplianceDetailRequest.Builder} avoiding the
* need to create one manually via {@link GetComplianceDetailRequest#builder()}
*
*
* @param getComplianceDetailRequest
* A {@link Consumer} that will call methods on {@link GetComplianceDetailRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the GetComplianceDetail operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ResourceNotFoundException The specified resource was not found.
* - InternalErrorException The operation failed because of a system problem, even though the request was
* valid. Retry your request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - FmsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample FmsAsyncClient.GetComplianceDetail
* @see AWS API
* Documentation
*/
default CompletableFuture getComplianceDetail(
Consumer getComplianceDetailRequest) {
return getComplianceDetail(GetComplianceDetailRequest.builder().applyMutation(getComplianceDetailRequest).build());
}
/**
*
* Information about the Amazon Simple Notification Service (SNS) topic that is used to record AWS Firewall Manager
* SNS logs.
*
*
* @param getNotificationChannelRequest
* @return A Java Future containing the result of the GetNotificationChannel operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ResourceNotFoundException The specified resource was not found.
* - InvalidOperationException The operation failed because there was nothing to do. For example, you
* might have submitted an
AssociateAdminAccount
request, but the account ID that you submitted
* was already set as the AWS Firewall Manager administrator.
* - InternalErrorException The operation failed because of a system problem, even though the request was
* valid. Retry your request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - FmsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample FmsAsyncClient.GetNotificationChannel
* @see AWS API
* Documentation
*/
default CompletableFuture getNotificationChannel(
GetNotificationChannelRequest getNotificationChannelRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Information about the Amazon Simple Notification Service (SNS) topic that is used to record AWS Firewall Manager
* SNS logs.
*
*
*
* This is a convenience which creates an instance of the {@link GetNotificationChannelRequest.Builder} avoiding the
* need to create one manually via {@link GetNotificationChannelRequest#builder()}
*
*
* @param getNotificationChannelRequest
* A {@link Consumer} that will call methods on {@link GetNotificationChannelRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the GetNotificationChannel operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ResourceNotFoundException The specified resource was not found.
* - InvalidOperationException The operation failed because there was nothing to do. For example, you
* might have submitted an
AssociateAdminAccount
request, but the account ID that you submitted
* was already set as the AWS Firewall Manager administrator.
* - InternalErrorException The operation failed because of a system problem, even though the request was
* valid. Retry your request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - FmsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample FmsAsyncClient.GetNotificationChannel
* @see AWS API
* Documentation
*/
default CompletableFuture getNotificationChannel(
Consumer getNotificationChannelRequest) {
return getNotificationChannel(GetNotificationChannelRequest.builder().applyMutation(getNotificationChannelRequest)
.build());
}
/**
*
* Returns information about the specified AWS Firewall Manager policy.
*
*
* @param getPolicyRequest
* @return A Java Future containing the result of the GetPolicy operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ResourceNotFoundException The specified resource was not found.
* - InvalidOperationException The operation failed because there was nothing to do. For example, you
* might have submitted an
AssociateAdminAccount
request, but the account ID that you submitted
* was already set as the AWS Firewall Manager administrator.
* - InternalErrorException The operation failed because of a system problem, even though the request was
* valid. Retry your request.
* - InvalidTypeException The value of the
Type
parameter is invalid.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - FmsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample FmsAsyncClient.GetPolicy
* @see AWS API
* Documentation
*/
default CompletableFuture getPolicy(GetPolicyRequest getPolicyRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Returns information about the specified AWS Firewall Manager policy.
*
*
*
* This is a convenience which creates an instance of the {@link GetPolicyRequest.Builder} avoiding the need to
* create one manually via {@link GetPolicyRequest#builder()}
*
*
* @param getPolicyRequest
* A {@link Consumer} that will call methods on {@link GetPolicyRequest.Builder} to create a request.
* @return A Java Future containing the result of the GetPolicy operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ResourceNotFoundException The specified resource was not found.
* - InvalidOperationException The operation failed because there was nothing to do. For example, you
* might have submitted an
AssociateAdminAccount
request, but the account ID that you submitted
* was already set as the AWS Firewall Manager administrator.
* - InternalErrorException The operation failed because of a system problem, even though the request was
* valid. Retry your request.
* - InvalidTypeException The value of the
Type
parameter is invalid.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - FmsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample FmsAsyncClient.GetPolicy
* @see AWS API
* Documentation
*/
default CompletableFuture getPolicy(Consumer getPolicyRequest) {
return getPolicy(GetPolicyRequest.builder().applyMutation(getPolicyRequest).build());
}
/**
*
* If you created a Shield Advanced policy, returns policy-level attack summary information in the event of a
* potential DDoS attack. Other policy types are currently unsupported.
*
*
* @param getProtectionStatusRequest
* @return A Java Future containing the result of the GetProtectionStatus operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InvalidInputException The parameters of the request were invalid.
* - ResourceNotFoundException The specified resource was not found.
* - InternalErrorException The operation failed because of a system problem, even though the request was
* valid. Retry your request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - FmsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample FmsAsyncClient.GetProtectionStatus
* @see AWS API
* Documentation
*/
default CompletableFuture getProtectionStatus(
GetProtectionStatusRequest getProtectionStatusRequest) {
throw new UnsupportedOperationException();
}
/**
*
* If you created a Shield Advanced policy, returns policy-level attack summary information in the event of a
* potential DDoS attack. Other policy types are currently unsupported.
*
*
*
* This is a convenience which creates an instance of the {@link GetProtectionStatusRequest.Builder} avoiding the
* need to create one manually via {@link GetProtectionStatusRequest#builder()}
*
*
* @param getProtectionStatusRequest
* A {@link Consumer} that will call methods on {@link GetProtectionStatusRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the GetProtectionStatus operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InvalidInputException The parameters of the request were invalid.
* - ResourceNotFoundException The specified resource was not found.
* - InternalErrorException The operation failed because of a system problem, even though the request was
* valid. Retry your request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - FmsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample FmsAsyncClient.GetProtectionStatus
* @see AWS API
* Documentation
*/
default CompletableFuture getProtectionStatus(
Consumer getProtectionStatusRequest) {
return getProtectionStatus(GetProtectionStatusRequest.builder().applyMutation(getProtectionStatusRequest).build());
}
/**
*
* Returns an array of PolicyComplianceStatus
objects in the response. Use
* PolicyComplianceStatus
to get a summary of which member accounts are protected by the specified
* policy.
*
*
* @param listComplianceStatusRequest
* @return A Java Future containing the result of the ListComplianceStatus operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ResourceNotFoundException The specified resource was not found.
* - InternalErrorException The operation failed because of a system problem, even though the request was
* valid. Retry your request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - FmsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample FmsAsyncClient.ListComplianceStatus
* @see AWS API
* Documentation
*/
default CompletableFuture listComplianceStatus(
ListComplianceStatusRequest listComplianceStatusRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Returns an array of PolicyComplianceStatus
objects in the response. Use
* PolicyComplianceStatus
to get a summary of which member accounts are protected by the specified
* policy.
*
*
*
* This is a convenience which creates an instance of the {@link ListComplianceStatusRequest.Builder} avoiding the
* need to create one manually via {@link ListComplianceStatusRequest#builder()}
*
*
* @param listComplianceStatusRequest
* A {@link Consumer} that will call methods on {@link ListComplianceStatusRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the ListComplianceStatus operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ResourceNotFoundException The specified resource was not found.
* - InternalErrorException The operation failed because of a system problem, even though the request was
* valid. Retry your request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - FmsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample FmsAsyncClient.ListComplianceStatus
* @see AWS API
* Documentation
*/
default CompletableFuture listComplianceStatus(
Consumer listComplianceStatusRequest) {
return listComplianceStatus(ListComplianceStatusRequest.builder().applyMutation(listComplianceStatusRequest).build());
}
/**
*
* Returns an array of PolicyComplianceStatus
objects in the response. Use
* PolicyComplianceStatus
to get a summary of which member accounts are protected by the specified
* policy.
*
*
*
* This is a variant of
* {@link #listComplianceStatus(software.amazon.awssdk.services.fms.model.ListComplianceStatusRequest)} operation.
* The return type is a custom publisher that can be subscribed to request a stream of response pages. SDK will
* internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.fms.paginators.ListComplianceStatusPublisher publisher = client.listComplianceStatusPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.fms.paginators.ListComplianceStatusPublisher publisher = client.listComplianceStatusPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.fms.model.ListComplianceStatusResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Note: If you prefer to have control on service calls, use the
* {@link #listComplianceStatus(software.amazon.awssdk.services.fms.model.ListComplianceStatusRequest)}
* operation.
*
*
* @param listComplianceStatusRequest
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ResourceNotFoundException The specified resource was not found.
* - InternalErrorException The operation failed because of a system problem, even though the request was
* valid. Retry your request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - FmsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample FmsAsyncClient.ListComplianceStatus
* @see AWS API
* Documentation
*/
default ListComplianceStatusPublisher listComplianceStatusPaginator(ListComplianceStatusRequest listComplianceStatusRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Returns an array of PolicyComplianceStatus
objects in the response. Use
* PolicyComplianceStatus
to get a summary of which member accounts are protected by the specified
* policy.
*
*
*
* This is a variant of
* {@link #listComplianceStatus(software.amazon.awssdk.services.fms.model.ListComplianceStatusRequest)} operation.
* The return type is a custom publisher that can be subscribed to request a stream of response pages. SDK will
* internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.fms.paginators.ListComplianceStatusPublisher publisher = client.listComplianceStatusPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.fms.paginators.ListComplianceStatusPublisher publisher = client.listComplianceStatusPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.fms.model.ListComplianceStatusResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Note: If you prefer to have control on service calls, use the
* {@link #listComplianceStatus(software.amazon.awssdk.services.fms.model.ListComplianceStatusRequest)}
* operation.
*
*
* This is a convenience which creates an instance of the {@link ListComplianceStatusRequest.Builder} avoiding the
* need to create one manually via {@link ListComplianceStatusRequest#builder()}
*
*
* @param listComplianceStatusRequest
* A {@link Consumer} that will call methods on {@link ListComplianceStatusRequest.Builder} to create a
* request.
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ResourceNotFoundException The specified resource was not found.
* - InternalErrorException The operation failed because of a system problem, even though the request was
* valid. Retry your request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - FmsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample FmsAsyncClient.ListComplianceStatus
* @see AWS API
* Documentation
*/
default ListComplianceStatusPublisher listComplianceStatusPaginator(
Consumer listComplianceStatusRequest) {
return listComplianceStatusPaginator(ListComplianceStatusRequest.builder().applyMutation(listComplianceStatusRequest)
.build());
}
/**
*
* Returns a MemberAccounts
object that lists the member accounts in the administrator's AWS
* organization.
*
*
* The ListMemberAccounts
must be submitted by the account that is set as the AWS Firewall Manager
* administrator.
*
*
* @param listMemberAccountsRequest
* @return A Java Future containing the result of the ListMemberAccounts operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ResourceNotFoundException The specified resource was not found.
* - InternalErrorException The operation failed because of a system problem, even though the request was
* valid. Retry your request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - FmsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample FmsAsyncClient.ListMemberAccounts
* @see AWS API
* Documentation
*/
default CompletableFuture listMemberAccounts(ListMemberAccountsRequest listMemberAccountsRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Returns a MemberAccounts
object that lists the member accounts in the administrator's AWS
* organization.
*
*
* The ListMemberAccounts
must be submitted by the account that is set as the AWS Firewall Manager
* administrator.
*
*
*
* This is a convenience which creates an instance of the {@link ListMemberAccountsRequest.Builder} avoiding the
* need to create one manually via {@link ListMemberAccountsRequest#builder()}
*
*
* @param listMemberAccountsRequest
* A {@link Consumer} that will call methods on {@link ListMemberAccountsRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the ListMemberAccounts operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ResourceNotFoundException The specified resource was not found.
* - InternalErrorException The operation failed because of a system problem, even though the request was
* valid. Retry your request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - FmsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample FmsAsyncClient.ListMemberAccounts
* @see AWS API
* Documentation
*/
default CompletableFuture listMemberAccounts(
Consumer listMemberAccountsRequest) {
return listMemberAccounts(ListMemberAccountsRequest.builder().applyMutation(listMemberAccountsRequest).build());
}
/**
*
* Returns a MemberAccounts
object that lists the member accounts in the administrator's AWS
* organization.
*
*
* The ListMemberAccounts
must be submitted by the account that is set as the AWS Firewall Manager
* administrator.
*
*
*
* This is a variant of
* {@link #listMemberAccounts(software.amazon.awssdk.services.fms.model.ListMemberAccountsRequest)} operation. The
* return type is a custom publisher that can be subscribed to request a stream of response pages. SDK will
* internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.fms.paginators.ListMemberAccountsPublisher publisher = client.listMemberAccountsPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.fms.paginators.ListMemberAccountsPublisher publisher = client.listMemberAccountsPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.fms.model.ListMemberAccountsResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Note: If you prefer to have control on service calls, use the
* {@link #listMemberAccounts(software.amazon.awssdk.services.fms.model.ListMemberAccountsRequest)} operation.
*
*
* @param listMemberAccountsRequest
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ResourceNotFoundException The specified resource was not found.
* - InternalErrorException The operation failed because of a system problem, even though the request was
* valid. Retry your request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - FmsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample FmsAsyncClient.ListMemberAccounts
* @see AWS API
* Documentation
*/
default ListMemberAccountsPublisher listMemberAccountsPaginator(ListMemberAccountsRequest listMemberAccountsRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Returns a MemberAccounts
object that lists the member accounts in the administrator's AWS
* organization.
*
*
* The ListMemberAccounts
must be submitted by the account that is set as the AWS Firewall Manager
* administrator.
*
*
*
* This is a variant of
* {@link #listMemberAccounts(software.amazon.awssdk.services.fms.model.ListMemberAccountsRequest)} operation. The
* return type is a custom publisher that can be subscribed to request a stream of response pages. SDK will
* internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.fms.paginators.ListMemberAccountsPublisher publisher = client.listMemberAccountsPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.fms.paginators.ListMemberAccountsPublisher publisher = client.listMemberAccountsPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.fms.model.ListMemberAccountsResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Note: If you prefer to have control on service calls, use the
* {@link #listMemberAccounts(software.amazon.awssdk.services.fms.model.ListMemberAccountsRequest)} operation.
*
*
* This is a convenience which creates an instance of the {@link ListMemberAccountsRequest.Builder} avoiding the
* need to create one manually via {@link ListMemberAccountsRequest#builder()}
*
*
* @param listMemberAccountsRequest
* A {@link Consumer} that will call methods on {@link ListMemberAccountsRequest.Builder} to create a
* request.
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ResourceNotFoundException The specified resource was not found.
* - InternalErrorException The operation failed because of a system problem, even though the request was
* valid. Retry your request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - FmsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample FmsAsyncClient.ListMemberAccounts
* @see AWS API
* Documentation
*/
default ListMemberAccountsPublisher listMemberAccountsPaginator(
Consumer listMemberAccountsRequest) {
return listMemberAccountsPaginator(ListMemberAccountsRequest.builder().applyMutation(listMemberAccountsRequest).build());
}
/**
*
* Returns an array of PolicySummary
objects in the response.
*
*
* @param listPoliciesRequest
* @return A Java Future containing the result of the ListPolicies operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ResourceNotFoundException The specified resource was not found.
* - InvalidOperationException The operation failed because there was nothing to do. For example, you
* might have submitted an
AssociateAdminAccount
request, but the account ID that you submitted
* was already set as the AWS Firewall Manager administrator.
* - LimitExceededException The operation exceeds a resource limit, for example, the maximum number of
*
policy
objects that you can create for an AWS account. For more information, see Firewall Manager Limits
* in the AWS WAF Developer Guide.
* - InternalErrorException The operation failed because of a system problem, even though the request was
* valid. Retry your request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - FmsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample FmsAsyncClient.ListPolicies
* @see AWS API
* Documentation
*/
default CompletableFuture listPolicies(ListPoliciesRequest listPoliciesRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Returns an array of PolicySummary
objects in the response.
*
*
*
* This is a convenience which creates an instance of the {@link ListPoliciesRequest.Builder} avoiding the need to
* create one manually via {@link ListPoliciesRequest#builder()}
*
*
* @param listPoliciesRequest
* A {@link Consumer} that will call methods on {@link ListPoliciesRequest.Builder} to create a request.
* @return A Java Future containing the result of the ListPolicies operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ResourceNotFoundException The specified resource was not found.
* - InvalidOperationException The operation failed because there was nothing to do. For example, you
* might have submitted an
AssociateAdminAccount
request, but the account ID that you submitted
* was already set as the AWS Firewall Manager administrator.
* - LimitExceededException The operation exceeds a resource limit, for example, the maximum number of
*
policy
objects that you can create for an AWS account. For more information, see Firewall Manager Limits
* in the AWS WAF Developer Guide.
* - InternalErrorException The operation failed because of a system problem, even though the request was
* valid. Retry your request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - FmsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample FmsAsyncClient.ListPolicies
* @see AWS API
* Documentation
*/
default CompletableFuture listPolicies(Consumer listPoliciesRequest) {
return listPolicies(ListPoliciesRequest.builder().applyMutation(listPoliciesRequest).build());
}
/**
*
* Returns an array of PolicySummary
objects in the response.
*
*
*
* This is a variant of {@link #listPolicies(software.amazon.awssdk.services.fms.model.ListPoliciesRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.fms.paginators.ListPoliciesPublisher publisher = client.listPoliciesPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.fms.paginators.ListPoliciesPublisher publisher = client.listPoliciesPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.fms.model.ListPoliciesResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Note: If you prefer to have control on service calls, use the
* {@link #listPolicies(software.amazon.awssdk.services.fms.model.ListPoliciesRequest)} operation.
*
*
* @param listPoliciesRequest
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ResourceNotFoundException The specified resource was not found.
* - InvalidOperationException The operation failed because there was nothing to do. For example, you
* might have submitted an
AssociateAdminAccount
request, but the account ID that you submitted
* was already set as the AWS Firewall Manager administrator.
* - LimitExceededException The operation exceeds a resource limit, for example, the maximum number of
*
policy
objects that you can create for an AWS account. For more information, see Firewall Manager Limits
* in the AWS WAF Developer Guide.
* - InternalErrorException The operation failed because of a system problem, even though the request was
* valid. Retry your request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - FmsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample FmsAsyncClient.ListPolicies
* @see AWS API
* Documentation
*/
default ListPoliciesPublisher listPoliciesPaginator(ListPoliciesRequest listPoliciesRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Returns an array of PolicySummary
objects in the response.
*
*
*
* This is a variant of {@link #listPolicies(software.amazon.awssdk.services.fms.model.ListPoliciesRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.fms.paginators.ListPoliciesPublisher publisher = client.listPoliciesPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.fms.paginators.ListPoliciesPublisher publisher = client.listPoliciesPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.fms.model.ListPoliciesResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Note: If you prefer to have control on service calls, use the
* {@link #listPolicies(software.amazon.awssdk.services.fms.model.ListPoliciesRequest)} operation.
*
*
* This is a convenience which creates an instance of the {@link ListPoliciesRequest.Builder} avoiding the need to
* create one manually via {@link ListPoliciesRequest#builder()}
*
*
* @param listPoliciesRequest
* A {@link Consumer} that will call methods on {@link ListPoliciesRequest.Builder} to create a request.
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ResourceNotFoundException The specified resource was not found.
* - InvalidOperationException The operation failed because there was nothing to do. For example, you
* might have submitted an
AssociateAdminAccount
request, but the account ID that you submitted
* was already set as the AWS Firewall Manager administrator.
* - LimitExceededException The operation exceeds a resource limit, for example, the maximum number of
*
policy
objects that you can create for an AWS account. For more information, see Firewall Manager Limits
* in the AWS WAF Developer Guide.
* - InternalErrorException The operation failed because of a system problem, even though the request was
* valid. Retry your request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - FmsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample FmsAsyncClient.ListPolicies
* @see AWS API
* Documentation
*/
default ListPoliciesPublisher listPoliciesPaginator(Consumer listPoliciesRequest) {
return listPoliciesPaginator(ListPoliciesRequest.builder().applyMutation(listPoliciesRequest).build());
}
/**
*
* Retrieves the list of tags for the specified AWS resource.
*
*
* @param listTagsForResourceRequest
* @return A Java Future containing the result of the ListTagsForResource operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ResourceNotFoundException The specified resource was not found.
* - InvalidOperationException The operation failed because there was nothing to do. For example, you
* might have submitted an
AssociateAdminAccount
request, but the account ID that you submitted
* was already set as the AWS Firewall Manager administrator.
* - InternalErrorException The operation failed because of a system problem, even though the request was
* valid. Retry your request.
* - InvalidInputException The parameters of the request were invalid.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - FmsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample FmsAsyncClient.ListTagsForResource
* @see AWS API
* Documentation
*/
default CompletableFuture listTagsForResource(
ListTagsForResourceRequest listTagsForResourceRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Retrieves the list of tags for the specified AWS resource.
*
*
*
* This is a convenience which creates an instance of the {@link ListTagsForResourceRequest.Builder} avoiding the
* need to create one manually via {@link ListTagsForResourceRequest#builder()}
*
*
* @param listTagsForResourceRequest
* A {@link Consumer} that will call methods on {@link ListTagsForResourceRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the ListTagsForResource operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ResourceNotFoundException The specified resource was not found.
* - InvalidOperationException The operation failed because there was nothing to do. For example, you
* might have submitted an
AssociateAdminAccount
request, but the account ID that you submitted
* was already set as the AWS Firewall Manager administrator.
* - InternalErrorException The operation failed because of a system problem, even though the request was
* valid. Retry your request.
* - InvalidInputException The parameters of the request were invalid.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - FmsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample FmsAsyncClient.ListTagsForResource
* @see AWS API
* Documentation
*/
default CompletableFuture listTagsForResource(
Consumer listTagsForResourceRequest) {
return listTagsForResource(ListTagsForResourceRequest.builder().applyMutation(listTagsForResourceRequest).build());
}
/**
*
* Designates the IAM role and Amazon Simple Notification Service (SNS) topic that AWS Firewall Manager uses to
* record SNS logs.
*
*
* @param putNotificationChannelRequest
* @return A Java Future containing the result of the PutNotificationChannel operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ResourceNotFoundException The specified resource was not found.
* - InvalidOperationException The operation failed because there was nothing to do. For example, you
* might have submitted an
AssociateAdminAccount
request, but the account ID that you submitted
* was already set as the AWS Firewall Manager administrator.
* - InternalErrorException The operation failed because of a system problem, even though the request was
* valid. Retry your request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - FmsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample FmsAsyncClient.PutNotificationChannel
* @see AWS API
* Documentation
*/
default CompletableFuture putNotificationChannel(
PutNotificationChannelRequest putNotificationChannelRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Designates the IAM role and Amazon Simple Notification Service (SNS) topic that AWS Firewall Manager uses to
* record SNS logs.
*
*
*
* This is a convenience which creates an instance of the {@link PutNotificationChannelRequest.Builder} avoiding the
* need to create one manually via {@link PutNotificationChannelRequest#builder()}
*
*
* @param putNotificationChannelRequest
* A {@link Consumer} that will call methods on {@link PutNotificationChannelRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the PutNotificationChannel operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ResourceNotFoundException The specified resource was not found.
* - InvalidOperationException The operation failed because there was nothing to do. For example, you
* might have submitted an
AssociateAdminAccount
request, but the account ID that you submitted
* was already set as the AWS Firewall Manager administrator.
* - InternalErrorException The operation failed because of a system problem, even though the request was
* valid. Retry your request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - FmsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample FmsAsyncClient.PutNotificationChannel
* @see AWS API
* Documentation
*/
default CompletableFuture putNotificationChannel(
Consumer putNotificationChannelRequest) {
return putNotificationChannel(PutNotificationChannelRequest.builder().applyMutation(putNotificationChannelRequest)
.build());
}
/**
*
* Creates an AWS Firewall Manager policy.
*
*
* Firewall Manager provides the following types of policies:
*
*
* -
*
* A Shield Advanced policy, which applies Shield Advanced protection to specified accounts and resources
*
*
* -
*
* An AWS WAF policy, which contains a rule group and defines which resources are to be protected by that rule group
*
*
* -
*
* A security group policy, which manages VPC security groups across your AWS organization.
*
*
*
*
* Each policy is specific to one of the three types. If you want to enforce more than one policy type across
* accounts, you can create multiple policies. You can create multiple policies for each type.
*
*
* You must be subscribed to Shield Advanced to create a Shield Advanced policy. For more information about
* subscribing to Shield Advanced, see CreateSubscription.
*
*
* @param putPolicyRequest
* @return A Java Future containing the result of the PutPolicy operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ResourceNotFoundException The specified resource was not found.
* - InvalidOperationException The operation failed because there was nothing to do. For example, you
* might have submitted an
AssociateAdminAccount
request, but the account ID that you submitted
* was already set as the AWS Firewall Manager administrator.
* - InvalidInputException The parameters of the request were invalid.
* - LimitExceededException The operation exceeds a resource limit, for example, the maximum number of
*
policy
objects that you can create for an AWS account. For more information, see Firewall Manager Limits
* in the AWS WAF Developer Guide.
* - InternalErrorException The operation failed because of a system problem, even though the request was
* valid. Retry your request.
* - InvalidTypeException The value of the
Type
parameter is invalid.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - FmsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample FmsAsyncClient.PutPolicy
* @see AWS API
* Documentation
*/
default CompletableFuture putPolicy(PutPolicyRequest putPolicyRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Creates an AWS Firewall Manager policy.
*
*
* Firewall Manager provides the following types of policies:
*
*
* -
*
* A Shield Advanced policy, which applies Shield Advanced protection to specified accounts and resources
*
*
* -
*
* An AWS WAF policy, which contains a rule group and defines which resources are to be protected by that rule group
*
*
* -
*
* A security group policy, which manages VPC security groups across your AWS organization.
*
*
*
*
* Each policy is specific to one of the three types. If you want to enforce more than one policy type across
* accounts, you can create multiple policies. You can create multiple policies for each type.
*
*
* You must be subscribed to Shield Advanced to create a Shield Advanced policy. For more information about
* subscribing to Shield Advanced, see CreateSubscription.
*
*
*
* This is a convenience which creates an instance of the {@link PutPolicyRequest.Builder} avoiding the need to
* create one manually via {@link PutPolicyRequest#builder()}
*
*
* @param putPolicyRequest
* A {@link Consumer} that will call methods on {@link PutPolicyRequest.Builder} to create a request.
* @return A Java Future containing the result of the PutPolicy operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ResourceNotFoundException The specified resource was not found.
* - InvalidOperationException The operation failed because there was nothing to do. For example, you
* might have submitted an
AssociateAdminAccount
request, but the account ID that you submitted
* was already set as the AWS Firewall Manager administrator.
* - InvalidInputException The parameters of the request were invalid.
* - LimitExceededException The operation exceeds a resource limit, for example, the maximum number of
*
policy
objects that you can create for an AWS account. For more information, see Firewall Manager Limits
* in the AWS WAF Developer Guide.
* - InternalErrorException The operation failed because of a system problem, even though the request was
* valid. Retry your request.
* - InvalidTypeException The value of the
Type
parameter is invalid.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - FmsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample FmsAsyncClient.PutPolicy
* @see AWS API
* Documentation
*/
default CompletableFuture putPolicy(Consumer putPolicyRequest) {
return putPolicy(PutPolicyRequest.builder().applyMutation(putPolicyRequest).build());
}
/**
*
* Adds one or more tags to an AWS resource.
*
*
* @param tagResourceRequest
* @return A Java Future containing the result of the TagResource operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ResourceNotFoundException The specified resource was not found.
* - InvalidOperationException The operation failed because there was nothing to do. For example, you
* might have submitted an
AssociateAdminAccount
request, but the account ID that you submitted
* was already set as the AWS Firewall Manager administrator.
* - InternalErrorException The operation failed because of a system problem, even though the request was
* valid. Retry your request.
* - InvalidInputException The parameters of the request were invalid.
* - LimitExceededException The operation exceeds a resource limit, for example, the maximum number of
*
policy
objects that you can create for an AWS account. For more information, see Firewall Manager Limits
* in the AWS WAF Developer Guide.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - FmsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample FmsAsyncClient.TagResource
* @see AWS API
* Documentation
*/
default CompletableFuture tagResource(TagResourceRequest tagResourceRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Adds one or more tags to an AWS resource.
*
*
*
* This is a convenience which creates an instance of the {@link TagResourceRequest.Builder} avoiding the need to
* create one manually via {@link TagResourceRequest#builder()}
*
*
* @param tagResourceRequest
* A {@link Consumer} that will call methods on {@link TagResourceRequest.Builder} to create a request.
* @return A Java Future containing the result of the TagResource operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ResourceNotFoundException The specified resource was not found.
* - InvalidOperationException The operation failed because there was nothing to do. For example, you
* might have submitted an
AssociateAdminAccount
request, but the account ID that you submitted
* was already set as the AWS Firewall Manager administrator.
* - InternalErrorException The operation failed because of a system problem, even though the request was
* valid. Retry your request.
* - InvalidInputException The parameters of the request were invalid.
* - LimitExceededException The operation exceeds a resource limit, for example, the maximum number of
*
policy
objects that you can create for an AWS account. For more information, see Firewall Manager Limits
* in the AWS WAF Developer Guide.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - FmsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample FmsAsyncClient.TagResource
* @see AWS API
* Documentation
*/
default CompletableFuture tagResource(Consumer tagResourceRequest) {
return tagResource(TagResourceRequest.builder().applyMutation(tagResourceRequest).build());
}
/**
*
* Removes one or more tags from an AWS resource.
*
*
* @param untagResourceRequest
* @return A Java Future containing the result of the UntagResource operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ResourceNotFoundException The specified resource was not found.
* - InvalidOperationException The operation failed because there was nothing to do. For example, you
* might have submitted an
AssociateAdminAccount
request, but the account ID that you submitted
* was already set as the AWS Firewall Manager administrator.
* - InternalErrorException The operation failed because of a system problem, even though the request was
* valid. Retry your request.
* - InvalidInputException The parameters of the request were invalid.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - FmsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample FmsAsyncClient.UntagResource
* @see AWS API
* Documentation
*/
default CompletableFuture untagResource(UntagResourceRequest untagResourceRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Removes one or more tags from an AWS resource.
*
*
*
* This is a convenience which creates an instance of the {@link UntagResourceRequest.Builder} avoiding the need to
* create one manually via {@link UntagResourceRequest#builder()}
*
*
* @param untagResourceRequest
* A {@link Consumer} that will call methods on {@link UntagResourceRequest.Builder} to create a request.
* @return A Java Future containing the result of the UntagResource operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ResourceNotFoundException The specified resource was not found.
* - InvalidOperationException The operation failed because there was nothing to do. For example, you
* might have submitted an
AssociateAdminAccount
request, but the account ID that you submitted
* was already set as the AWS Firewall Manager administrator.
* - InternalErrorException The operation failed because of a system problem, even though the request was
* valid. Retry your request.
* - InvalidInputException The parameters of the request were invalid.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - FmsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample FmsAsyncClient.UntagResource
* @see AWS API
* Documentation
*/
default CompletableFuture untagResource(Consumer untagResourceRequest) {
return untagResource(UntagResourceRequest.builder().applyMutation(untagResourceRequest).build());
}
}