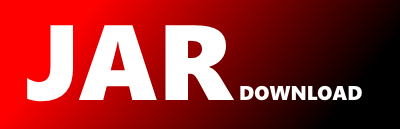
software.amazon.awssdk.services.fms.model.PolicySummary Maven / Gradle / Ivy
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.fms.model;
import java.io.Serializable;
import java.util.Arrays;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* Details of the AWS Firewall Manager policy.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class PolicySummary implements SdkPojo, Serializable, ToCopyableBuilder {
private static final SdkField POLICY_ARN_FIELD = SdkField. builder(MarshallingType.STRING)
.getter(getter(PolicySummary::policyArn)).setter(setter(Builder::policyArn))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("PolicyArn").build()).build();
private static final SdkField POLICY_ID_FIELD = SdkField. builder(MarshallingType.STRING)
.getter(getter(PolicySummary::policyId)).setter(setter(Builder::policyId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("PolicyId").build()).build();
private static final SdkField POLICY_NAME_FIELD = SdkField. builder(MarshallingType.STRING)
.getter(getter(PolicySummary::policyName)).setter(setter(Builder::policyName))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("PolicyName").build()).build();
private static final SdkField RESOURCE_TYPE_FIELD = SdkField. builder(MarshallingType.STRING)
.getter(getter(PolicySummary::resourceType)).setter(setter(Builder::resourceType))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ResourceType").build()).build();
private static final SdkField SECURITY_SERVICE_TYPE_FIELD = SdkField. builder(MarshallingType.STRING)
.getter(getter(PolicySummary::securityServiceTypeAsString)).setter(setter(Builder::securityServiceType))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("SecurityServiceType").build())
.build();
private static final SdkField REMEDIATION_ENABLED_FIELD = SdkField. builder(MarshallingType.BOOLEAN)
.getter(getter(PolicySummary::remediationEnabled)).setter(setter(Builder::remediationEnabled))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("RemediationEnabled").build())
.build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(POLICY_ARN_FIELD,
POLICY_ID_FIELD, POLICY_NAME_FIELD, RESOURCE_TYPE_FIELD, SECURITY_SERVICE_TYPE_FIELD, REMEDIATION_ENABLED_FIELD));
private static final long serialVersionUID = 1L;
private final String policyArn;
private final String policyId;
private final String policyName;
private final String resourceType;
private final String securityServiceType;
private final Boolean remediationEnabled;
private PolicySummary(BuilderImpl builder) {
this.policyArn = builder.policyArn;
this.policyId = builder.policyId;
this.policyName = builder.policyName;
this.resourceType = builder.resourceType;
this.securityServiceType = builder.securityServiceType;
this.remediationEnabled = builder.remediationEnabled;
}
/**
*
* The Amazon Resource Name (ARN) of the specified policy.
*
*
* @return The Amazon Resource Name (ARN) of the specified policy.
*/
public String policyArn() {
return policyArn;
}
/**
*
* The ID of the specified policy.
*
*
* @return The ID of the specified policy.
*/
public String policyId() {
return policyId;
}
/**
*
* The friendly name of the specified policy.
*
*
* @return The friendly name of the specified policy.
*/
public String policyName() {
return policyName;
}
/**
*
* The type of resource protected by or in scope of the policy. This is in the format shown in the AWS
* Resource Types Reference. For AWS WAF and Shield Advanced, examples include
* AWS::ElasticLoadBalancingV2::LoadBalancer
and AWS::CloudFront::Distribution
. For a
* security group common policy, valid values are AWS::EC2::NetworkInterface
and
* AWS::EC2::Instance
. For a security group content audit policy, valid values are
* AWS::EC2::SecurityGroup
, AWS::EC2::NetworkInterface
, and
* AWS::EC2::Instance
. For a security group usage audit policy, the value is
* AWS::EC2::SecurityGroup
.
*
*
* @return The type of resource protected by or in scope of the policy. This is in the format shown in the AWS Resource Types Reference. For AWS WAF and Shield Advanced, examples include
* AWS::ElasticLoadBalancingV2::LoadBalancer
and AWS::CloudFront::Distribution
.
* For a security group common policy, valid values are AWS::EC2::NetworkInterface
and
* AWS::EC2::Instance
. For a security group content audit policy, valid values are
* AWS::EC2::SecurityGroup
, AWS::EC2::NetworkInterface
, and
* AWS::EC2::Instance
. For a security group usage audit policy, the value is
* AWS::EC2::SecurityGroup
.
*/
public String resourceType() {
return resourceType;
}
/**
*
* The service that the policy is using to protect the resources. This specifies the type of policy that is created,
* either an AWS WAF policy, a Shield Advanced policy, or a security group policy.
*
*
* If the service returns an enum value that is not available in the current SDK version,
* {@link #securityServiceType} will return {@link SecurityServiceType#UNKNOWN_TO_SDK_VERSION}. The raw value
* returned by the service is available from {@link #securityServiceTypeAsString}.
*
*
* @return The service that the policy is using to protect the resources. This specifies the type of policy that is
* created, either an AWS WAF policy, a Shield Advanced policy, or a security group policy.
* @see SecurityServiceType
*/
public SecurityServiceType securityServiceType() {
return SecurityServiceType.fromValue(securityServiceType);
}
/**
*
* The service that the policy is using to protect the resources. This specifies the type of policy that is created,
* either an AWS WAF policy, a Shield Advanced policy, or a security group policy.
*
*
* If the service returns an enum value that is not available in the current SDK version,
* {@link #securityServiceType} will return {@link SecurityServiceType#UNKNOWN_TO_SDK_VERSION}. The raw value
* returned by the service is available from {@link #securityServiceTypeAsString}.
*
*
* @return The service that the policy is using to protect the resources. This specifies the type of policy that is
* created, either an AWS WAF policy, a Shield Advanced policy, or a security group policy.
* @see SecurityServiceType
*/
public String securityServiceTypeAsString() {
return securityServiceType;
}
/**
*
* Indicates if the policy should be automatically applied to new resources.
*
*
* @return Indicates if the policy should be automatically applied to new resources.
*/
public Boolean remediationEnabled() {
return remediationEnabled;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(policyArn());
hashCode = 31 * hashCode + Objects.hashCode(policyId());
hashCode = 31 * hashCode + Objects.hashCode(policyName());
hashCode = 31 * hashCode + Objects.hashCode(resourceType());
hashCode = 31 * hashCode + Objects.hashCode(securityServiceTypeAsString());
hashCode = 31 * hashCode + Objects.hashCode(remediationEnabled());
return hashCode;
}
@Override
public boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof PolicySummary)) {
return false;
}
PolicySummary other = (PolicySummary) obj;
return Objects.equals(policyArn(), other.policyArn()) && Objects.equals(policyId(), other.policyId())
&& Objects.equals(policyName(), other.policyName()) && Objects.equals(resourceType(), other.resourceType())
&& Objects.equals(securityServiceTypeAsString(), other.securityServiceTypeAsString())
&& Objects.equals(remediationEnabled(), other.remediationEnabled());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public String toString() {
return ToString.builder("PolicySummary").add("PolicyArn", policyArn()).add("PolicyId", policyId())
.add("PolicyName", policyName()).add("ResourceType", resourceType())
.add("SecurityServiceType", securityServiceTypeAsString()).add("RemediationEnabled", remediationEnabled())
.build();
}
public Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "PolicyArn":
return Optional.ofNullable(clazz.cast(policyArn()));
case "PolicyId":
return Optional.ofNullable(clazz.cast(policyId()));
case "PolicyName":
return Optional.ofNullable(clazz.cast(policyName()));
case "ResourceType":
return Optional.ofNullable(clazz.cast(resourceType()));
case "SecurityServiceType":
return Optional.ofNullable(clazz.cast(securityServiceTypeAsString()));
case "RemediationEnabled":
return Optional.ofNullable(clazz.cast(remediationEnabled()));
default:
return Optional.empty();
}
}
@Override
public List> sdkFields() {
return SDK_FIELDS;
}
private static Function