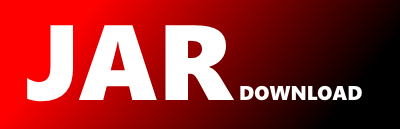
software.amazon.awssdk.services.fms.model.SecurityServicePolicyData Maven / Gradle / Ivy
Show all versions of fms Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.fms.model;
import java.io.Serializable;
import java.util.Arrays;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* Details about the security service that is being used to protect the resources.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class SecurityServicePolicyData implements SdkPojo, Serializable,
ToCopyableBuilder {
private static final SdkField TYPE_FIELD = SdkField. builder(MarshallingType.STRING).memberName("Type")
.getter(getter(SecurityServicePolicyData::typeAsString)).setter(setter(Builder::type))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Type").build()).build();
private static final SdkField MANAGED_SERVICE_DATA_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("ManagedServiceData").getter(getter(SecurityServicePolicyData::managedServiceData))
.setter(setter(Builder::managedServiceData))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ManagedServiceData").build())
.build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(TYPE_FIELD,
MANAGED_SERVICE_DATA_FIELD));
private static final long serialVersionUID = 1L;
private final String type;
private final String managedServiceData;
private SecurityServicePolicyData(BuilderImpl builder) {
this.type = builder.type;
this.managedServiceData = builder.managedServiceData;
}
/**
*
* The service that the policy is using to protect the resources. This specifies the type of policy that is created,
* either an AWS WAF policy, a Shield Advanced policy, or a security group policy. For security group policies,
* Firewall Manager supports one security group for each common policy and for each content audit policy. This is an
* adjustable limit that you can increase by contacting AWS Support.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #type} will return
* {@link SecurityServiceType#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #typeAsString}.
*
*
* @return The service that the policy is using to protect the resources. This specifies the type of policy that is
* created, either an AWS WAF policy, a Shield Advanced policy, or a security group policy. For security
* group policies, Firewall Manager supports one security group for each common policy and for each content
* audit policy. This is an adjustable limit that you can increase by contacting AWS Support.
* @see SecurityServiceType
*/
public final SecurityServiceType type() {
return SecurityServiceType.fromValue(type);
}
/**
*
* The service that the policy is using to protect the resources. This specifies the type of policy that is created,
* either an AWS WAF policy, a Shield Advanced policy, or a security group policy. For security group policies,
* Firewall Manager supports one security group for each common policy and for each content audit policy. This is an
* adjustable limit that you can increase by contacting AWS Support.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #type} will return
* {@link SecurityServiceType#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #typeAsString}.
*
*
* @return The service that the policy is using to protect the resources. This specifies the type of policy that is
* created, either an AWS WAF policy, a Shield Advanced policy, or a security group policy. For security
* group policies, Firewall Manager supports one security group for each common policy and for each content
* audit policy. This is an adjustable limit that you can increase by contacting AWS Support.
* @see SecurityServiceType
*/
public final String typeAsString() {
return type;
}
/**
*
* Details about the service that are specific to the service type, in JSON format. For service type
* SHIELD_ADVANCED
, this is an empty string.
*
*
* -
*
* Example: NETWORK_FIREWALL
*
*
* "{\"type\":\"NETWORK_FIREWALL\",\"networkFirewallStatelessRuleGroupReferences\":[{\"resourceARN\":\"arn:aws:network-firewall:us-west-1:1234567891011:stateless-rulegroup/rulegroup2\",\"priority\":10}],\"networkFirewallStatelessDefaultActions\":[\"aws:pass\",\"custom1\"],\"networkFirewallStatelessFragmentDefaultActions\":[\"custom2\",\"aws:pass\"],\"networkFirewallStatelessCustomActions\":[{\"actionName\":\"custom1\",\"actionDefinition\":{\"publishMetricAction\":{\"dimensions\":[{\"value\":\"dimension1\"}]}}},{\"actionName\":\"custom2\",\"actionDefinition\":{\"publishMetricAction\":{\"dimensions\":[{\"value\":\"dimension2\"}]}}}],\"networkFirewallStatefulRuleGroupReferences\":[{\"resourceARN\":\"arn:aws:network-firewall:us-west-1:1234567891011:stateful-rulegroup/rulegroup1\"}],\"networkFirewallOrchestrationConfig\":{\"singleFirewallEndpointPerVPC\":true,\"allowedIPV4CidrList\":[\"10.24.34.0/28\"]} }"
*
*
* -
*
* Example: WAFV2
*
*
* "{\"type\":\"WAFV2\",\"preProcessRuleGroups\":[{\"ruleGroupArn\":null,\"overrideAction\":{\"type\":\"NONE\"},\"managedRuleGroupIdentifier\":{\"version\":null,\"vendorName\":\"AWS\",\"managedRuleGroupName\":\"AWSManagedRulesAmazonIpReputationList\"},\"ruleGroupType\":\"ManagedRuleGroup\",\"excludeRules\":[]}],\"postProcessRuleGroups\":[],\"defaultAction\":{\"type\":\"ALLOW\"},\"overrideCustomerWebACLAssociation\":false,\"loggingConfiguration\":{\"logDestinationConfigs\":[\"arn:aws:firehose:us-west-2:12345678912:deliverystream/aws-waf-logs-fms-admin-destination\"],\"redactedFields\":[{\"redactedFieldType\":\"SingleHeader\",\"redactedFieldValue\":\"Cookies\"},{\"redactedFieldType\":\"Method\"}]}}"
*
*
* In the loggingConfiguration
, you can specify one logDestinationConfigs
, you can
* optionally provide up to 20 redactedFields
, and the RedactedFieldType
must be one of
* URI
, QUERY_STRING
, HEADER
, or METHOD
.
*
*
* -
*
* Example: WAF Classic
*
*
* "{\"type\": \"WAF\", \"ruleGroups\": [{\"id\":\"12345678-1bcd-9012-efga-0987654321ab\", \"overrideAction\" : {\"type\": \"COUNT\"}}], \"defaultAction\": {\"type\": \"BLOCK\"}}"
*
*
* -
*
* Example: SECURITY_GROUPS_COMMON
*
*
* "{\"type\":\"SECURITY_GROUPS_COMMON\",\"revertManualSecurityGroupChanges\":false,\"exclusiveResourceSecurityGroupManagement\":false, \"applyToAllEC2InstanceENIs\":false,\"securityGroups\":[{\"id\":\" sg-000e55995d61a06bd\"}]}"
*
*
* -
*
* Example: SECURITY_GROUPS_CONTENT_AUDIT
*
*
* "{\"type\":\"SECURITY_GROUPS_CONTENT_AUDIT\",\"securityGroups\":[{\"id\":\"sg-000e55995d61a06bd\"}],\"securityGroupAction\":{\"type\":\"ALLOW\"}}"
*
*
* The security group action for content audit can be ALLOW
or DENY
. For
* ALLOW
, all in-scope security group rules must be within the allowed range of the policy's security
* group rules. For DENY
, all in-scope security group rules must not contain a value or a range that
* matches a rule value or range in the policy security group.
*
*
* -
*
* Example: SECURITY_GROUPS_USAGE_AUDIT
*
*
* "{\"type\":\"SECURITY_GROUPS_USAGE_AUDIT\",\"deleteUnusedSecurityGroups\":true,\"coalesceRedundantSecurityGroups\":true}"
*
*
*
*
* @return Details about the service that are specific to the service type, in JSON format. For service type
* SHIELD_ADVANCED
, this is an empty string.
*
* -
*
* Example: NETWORK_FIREWALL
*
*
* "{\"type\":\"NETWORK_FIREWALL\",\"networkFirewallStatelessRuleGroupReferences\":[{\"resourceARN\":\"arn:aws:network-firewall:us-west-1:1234567891011:stateless-rulegroup/rulegroup2\",\"priority\":10}],\"networkFirewallStatelessDefaultActions\":[\"aws:pass\",\"custom1\"],\"networkFirewallStatelessFragmentDefaultActions\":[\"custom2\",\"aws:pass\"],\"networkFirewallStatelessCustomActions\":[{\"actionName\":\"custom1\",\"actionDefinition\":{\"publishMetricAction\":{\"dimensions\":[{\"value\":\"dimension1\"}]}}},{\"actionName\":\"custom2\",\"actionDefinition\":{\"publishMetricAction\":{\"dimensions\":[{\"value\":\"dimension2\"}]}}}],\"networkFirewallStatefulRuleGroupReferences\":[{\"resourceARN\":\"arn:aws:network-firewall:us-west-1:1234567891011:stateful-rulegroup/rulegroup1\"}],\"networkFirewallOrchestrationConfig\":{\"singleFirewallEndpointPerVPC\":true,\"allowedIPV4CidrList\":[\"10.24.34.0/28\"]} }"
*
*
* -
*
* Example: WAFV2
*
*
* "{\"type\":\"WAFV2\",\"preProcessRuleGroups\":[{\"ruleGroupArn\":null,\"overrideAction\":{\"type\":\"NONE\"},\"managedRuleGroupIdentifier\":{\"version\":null,\"vendorName\":\"AWS\",\"managedRuleGroupName\":\"AWSManagedRulesAmazonIpReputationList\"},\"ruleGroupType\":\"ManagedRuleGroup\",\"excludeRules\":[]}],\"postProcessRuleGroups\":[],\"defaultAction\":{\"type\":\"ALLOW\"},\"overrideCustomerWebACLAssociation\":false,\"loggingConfiguration\":{\"logDestinationConfigs\":[\"arn:aws:firehose:us-west-2:12345678912:deliverystream/aws-waf-logs-fms-admin-destination\"],\"redactedFields\":[{\"redactedFieldType\":\"SingleHeader\",\"redactedFieldValue\":\"Cookies\"},{\"redactedFieldType\":\"Method\"}]}}"
*
*
* In the loggingConfiguration
, you can specify one logDestinationConfigs
, you can
* optionally provide up to 20 redactedFields
, and the RedactedFieldType
must be
* one of URI
, QUERY_STRING
, HEADER
, or METHOD
.
*
*
* -
*
* Example: WAF Classic
*
*
* "{\"type\": \"WAF\", \"ruleGroups\": [{\"id\":\"12345678-1bcd-9012-efga-0987654321ab\", \"overrideAction\" : {\"type\": \"COUNT\"}}], \"defaultAction\": {\"type\": \"BLOCK\"}}"
*
*
* -
*
* Example: SECURITY_GROUPS_COMMON
*
*
* "{\"type\":\"SECURITY_GROUPS_COMMON\",\"revertManualSecurityGroupChanges\":false,\"exclusiveResourceSecurityGroupManagement\":false, \"applyToAllEC2InstanceENIs\":false,\"securityGroups\":[{\"id\":\" sg-000e55995d61a06bd\"}]}"
*
*
* -
*
* Example: SECURITY_GROUPS_CONTENT_AUDIT
*
*
* "{\"type\":\"SECURITY_GROUPS_CONTENT_AUDIT\",\"securityGroups\":[{\"id\":\"sg-000e55995d61a06bd\"}],\"securityGroupAction\":{\"type\":\"ALLOW\"}}"
*
*
* The security group action for content audit can be ALLOW
or DENY
. For
* ALLOW
, all in-scope security group rules must be within the allowed range of the policy's
* security group rules. For DENY
, all in-scope security group rules must not contain a value
* or a range that matches a rule value or range in the policy security group.
*
*
* -
*
* Example: SECURITY_GROUPS_USAGE_AUDIT
*
*
* "{\"type\":\"SECURITY_GROUPS_USAGE_AUDIT\",\"deleteUnusedSecurityGroups\":true,\"coalesceRedundantSecurityGroups\":true}"
*
*
*/
public final String managedServiceData() {
return managedServiceData;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(typeAsString());
hashCode = 31 * hashCode + Objects.hashCode(managedServiceData());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof SecurityServicePolicyData)) {
return false;
}
SecurityServicePolicyData other = (SecurityServicePolicyData) obj;
return Objects.equals(typeAsString(), other.typeAsString())
&& Objects.equals(managedServiceData(), other.managedServiceData());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("SecurityServicePolicyData").add("Type", typeAsString())
.add("ManagedServiceData", managedServiceData()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "Type":
return Optional.ofNullable(clazz.cast(typeAsString()));
case "ManagedServiceData":
return Optional.ofNullable(clazz.cast(managedServiceData()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
private static Function