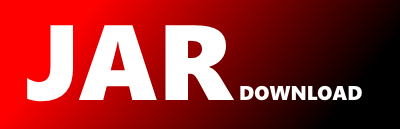
software.amazon.awssdk.services.fms.model.NetworkFirewallInternetTrafficNotInspectedViolation Maven / Gradle / Ivy
Show all versions of fms Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.fms.model;
import java.io.Serializable;
import java.util.Arrays;
import java.util.Collection;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import java.util.stream.Collectors;
import java.util.stream.Stream;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.ListTrait;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.core.util.DefaultSdkAutoConstructList;
import software.amazon.awssdk.core.util.SdkAutoConstructList;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* Violation detail for the subnet for which internet traffic that hasn't been inspected.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class NetworkFirewallInternetTrafficNotInspectedViolation
implements
SdkPojo,
Serializable,
ToCopyableBuilder {
private static final SdkField SUBNET_ID_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("SubnetId").getter(getter(NetworkFirewallInternetTrafficNotInspectedViolation::subnetId))
.setter(setter(Builder::subnetId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("SubnetId").build()).build();
private static final SdkField SUBNET_AVAILABILITY_ZONE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("SubnetAvailabilityZone")
.getter(getter(NetworkFirewallInternetTrafficNotInspectedViolation::subnetAvailabilityZone))
.setter(setter(Builder::subnetAvailabilityZone))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("SubnetAvailabilityZone").build())
.build();
private static final SdkField ROUTE_TABLE_ID_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("RouteTableId").getter(getter(NetworkFirewallInternetTrafficNotInspectedViolation::routeTableId))
.setter(setter(Builder::routeTableId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("RouteTableId").build()).build();
private static final SdkField> VIOLATING_ROUTES_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("ViolatingRoutes")
.getter(getter(NetworkFirewallInternetTrafficNotInspectedViolation::violatingRoutes))
.setter(setter(Builder::violatingRoutes))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ViolatingRoutes").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(Route::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField IS_ROUTE_TABLE_USED_IN_DIFFERENT_AZ_FIELD = SdkField
. builder(MarshallingType.BOOLEAN)
.memberName("IsRouteTableUsedInDifferentAZ")
.getter(getter(NetworkFirewallInternetTrafficNotInspectedViolation::isRouteTableUsedInDifferentAZ))
.setter(setter(Builder::isRouteTableUsedInDifferentAZ))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("IsRouteTableUsedInDifferentAZ")
.build()).build();
private static final SdkField CURRENT_FIREWALL_SUBNET_ROUTE_TABLE_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("CurrentFirewallSubnetRouteTable")
.getter(getter(NetworkFirewallInternetTrafficNotInspectedViolation::currentFirewallSubnetRouteTable))
.setter(setter(Builder::currentFirewallSubnetRouteTable))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("CurrentFirewallSubnetRouteTable")
.build()).build();
private static final SdkField EXPECTED_FIREWALL_ENDPOINT_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("ExpectedFirewallEndpoint")
.getter(getter(NetworkFirewallInternetTrafficNotInspectedViolation::expectedFirewallEndpoint))
.setter(setter(Builder::expectedFirewallEndpoint))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ExpectedFirewallEndpoint").build())
.build();
private static final SdkField FIREWALL_SUBNET_ID_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("FirewallSubnetId").getter(getter(NetworkFirewallInternetTrafficNotInspectedViolation::firewallSubnetId))
.setter(setter(Builder::firewallSubnetId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("FirewallSubnetId").build()).build();
private static final SdkField> EXPECTED_FIREWALL_SUBNET_ROUTES_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("ExpectedFirewallSubnetRoutes")
.getter(getter(NetworkFirewallInternetTrafficNotInspectedViolation::expectedFirewallSubnetRoutes))
.setter(setter(Builder::expectedFirewallSubnetRoutes))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ExpectedFirewallSubnetRoutes")
.build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(ExpectedRoute::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField> ACTUAL_FIREWALL_SUBNET_ROUTES_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("ActualFirewallSubnetRoutes")
.getter(getter(NetworkFirewallInternetTrafficNotInspectedViolation::actualFirewallSubnetRoutes))
.setter(setter(Builder::actualFirewallSubnetRoutes))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ActualFirewallSubnetRoutes").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(Route::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField INTERNET_GATEWAY_ID_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("InternetGatewayId")
.getter(getter(NetworkFirewallInternetTrafficNotInspectedViolation::internetGatewayId))
.setter(setter(Builder::internetGatewayId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("InternetGatewayId").build()).build();
private static final SdkField CURRENT_INTERNET_GATEWAY_ROUTE_TABLE_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("CurrentInternetGatewayRouteTable")
.getter(getter(NetworkFirewallInternetTrafficNotInspectedViolation::currentInternetGatewayRouteTable))
.setter(setter(Builder::currentInternetGatewayRouteTable))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("CurrentInternetGatewayRouteTable")
.build()).build();
private static final SdkField> EXPECTED_INTERNET_GATEWAY_ROUTES_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("ExpectedInternetGatewayRoutes")
.getter(getter(NetworkFirewallInternetTrafficNotInspectedViolation::expectedInternetGatewayRoutes))
.setter(setter(Builder::expectedInternetGatewayRoutes))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ExpectedInternetGatewayRoutes")
.build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(ExpectedRoute::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField> ACTUAL_INTERNET_GATEWAY_ROUTES_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("ActualInternetGatewayRoutes")
.getter(getter(NetworkFirewallInternetTrafficNotInspectedViolation::actualInternetGatewayRoutes))
.setter(setter(Builder::actualInternetGatewayRoutes))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ActualInternetGatewayRoutes")
.build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(Route::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField VPC_ID_FIELD = SdkField. builder(MarshallingType.STRING).memberName("VpcId")
.getter(getter(NetworkFirewallInternetTrafficNotInspectedViolation::vpcId)).setter(setter(Builder::vpcId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("VpcId").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(SUBNET_ID_FIELD,
SUBNET_AVAILABILITY_ZONE_FIELD, ROUTE_TABLE_ID_FIELD, VIOLATING_ROUTES_FIELD,
IS_ROUTE_TABLE_USED_IN_DIFFERENT_AZ_FIELD, CURRENT_FIREWALL_SUBNET_ROUTE_TABLE_FIELD,
EXPECTED_FIREWALL_ENDPOINT_FIELD, FIREWALL_SUBNET_ID_FIELD, EXPECTED_FIREWALL_SUBNET_ROUTES_FIELD,
ACTUAL_FIREWALL_SUBNET_ROUTES_FIELD, INTERNET_GATEWAY_ID_FIELD, CURRENT_INTERNET_GATEWAY_ROUTE_TABLE_FIELD,
EXPECTED_INTERNET_GATEWAY_ROUTES_FIELD, ACTUAL_INTERNET_GATEWAY_ROUTES_FIELD, VPC_ID_FIELD));
private static final long serialVersionUID = 1L;
private final String subnetId;
private final String subnetAvailabilityZone;
private final String routeTableId;
private final List violatingRoutes;
private final Boolean isRouteTableUsedInDifferentAZ;
private final String currentFirewallSubnetRouteTable;
private final String expectedFirewallEndpoint;
private final String firewallSubnetId;
private final List expectedFirewallSubnetRoutes;
private final List actualFirewallSubnetRoutes;
private final String internetGatewayId;
private final String currentInternetGatewayRouteTable;
private final List expectedInternetGatewayRoutes;
private final List actualInternetGatewayRoutes;
private final String vpcId;
private NetworkFirewallInternetTrafficNotInspectedViolation(BuilderImpl builder) {
this.subnetId = builder.subnetId;
this.subnetAvailabilityZone = builder.subnetAvailabilityZone;
this.routeTableId = builder.routeTableId;
this.violatingRoutes = builder.violatingRoutes;
this.isRouteTableUsedInDifferentAZ = builder.isRouteTableUsedInDifferentAZ;
this.currentFirewallSubnetRouteTable = builder.currentFirewallSubnetRouteTable;
this.expectedFirewallEndpoint = builder.expectedFirewallEndpoint;
this.firewallSubnetId = builder.firewallSubnetId;
this.expectedFirewallSubnetRoutes = builder.expectedFirewallSubnetRoutes;
this.actualFirewallSubnetRoutes = builder.actualFirewallSubnetRoutes;
this.internetGatewayId = builder.internetGatewayId;
this.currentInternetGatewayRouteTable = builder.currentInternetGatewayRouteTable;
this.expectedInternetGatewayRoutes = builder.expectedInternetGatewayRoutes;
this.actualInternetGatewayRoutes = builder.actualInternetGatewayRoutes;
this.vpcId = builder.vpcId;
}
/**
*
* The subnet ID.
*
*
* @return The subnet ID.
*/
public final String subnetId() {
return subnetId;
}
/**
*
* The subnet Availability Zone.
*
*
* @return The subnet Availability Zone.
*/
public final String subnetAvailabilityZone() {
return subnetAvailabilityZone;
}
/**
*
* Information about the route table ID.
*
*
* @return Information about the route table ID.
*/
public final String routeTableId() {
return routeTableId;
}
/**
* For responses, this returns true if the service returned a value for the ViolatingRoutes property. This DOES NOT
* check that the value is non-empty (for which, you should check the {@code isEmpty()} method on the property).
* This is useful because the SDK will never return a null collection or map, but you may need to differentiate
* between the service returning nothing (or null) and the service returning an empty collection or map. For
* requests, this returns true if a value for the property was specified in the request builder, and false if a
* value was not specified.
*/
public final boolean hasViolatingRoutes() {
return violatingRoutes != null && !(violatingRoutes instanceof SdkAutoConstructList);
}
/**
*
* The route or routes that are in violation.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasViolatingRoutes} method.
*
*
* @return The route or routes that are in violation.
*/
public final List violatingRoutes() {
return violatingRoutes;
}
/**
*
* Information about whether the route table is used in another Availability Zone.
*
*
* @return Information about whether the route table is used in another Availability Zone.
*/
public final Boolean isRouteTableUsedInDifferentAZ() {
return isRouteTableUsedInDifferentAZ;
}
/**
*
* Information about the subnet route table for the current firewall.
*
*
* @return Information about the subnet route table for the current firewall.
*/
public final String currentFirewallSubnetRouteTable() {
return currentFirewallSubnetRouteTable;
}
/**
*
* The expected endpoint for the current firewall.
*
*
* @return The expected endpoint for the current firewall.
*/
public final String expectedFirewallEndpoint() {
return expectedFirewallEndpoint;
}
/**
*
* The firewall subnet ID.
*
*
* @return The firewall subnet ID.
*/
public final String firewallSubnetId() {
return firewallSubnetId;
}
/**
* For responses, this returns true if the service returned a value for the ExpectedFirewallSubnetRoutes property.
* This DOES NOT check that the value is non-empty (for which, you should check the {@code isEmpty()} method on the
* property). This is useful because the SDK will never return a null collection or map, but you may need to
* differentiate between the service returning nothing (or null) and the service returning an empty collection or
* map. For requests, this returns true if a value for the property was specified in the request builder, and false
* if a value was not specified.
*/
public final boolean hasExpectedFirewallSubnetRoutes() {
return expectedFirewallSubnetRoutes != null && !(expectedFirewallSubnetRoutes instanceof SdkAutoConstructList);
}
/**
*
* The firewall subnet routes that are expected.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasExpectedFirewallSubnetRoutes} method.
*
*
* @return The firewall subnet routes that are expected.
*/
public final List expectedFirewallSubnetRoutes() {
return expectedFirewallSubnetRoutes;
}
/**
* For responses, this returns true if the service returned a value for the ActualFirewallSubnetRoutes property.
* This DOES NOT check that the value is non-empty (for which, you should check the {@code isEmpty()} method on the
* property). This is useful because the SDK will never return a null collection or map, but you may need to
* differentiate between the service returning nothing (or null) and the service returning an empty collection or
* map. For requests, this returns true if a value for the property was specified in the request builder, and false
* if a value was not specified.
*/
public final boolean hasActualFirewallSubnetRoutes() {
return actualFirewallSubnetRoutes != null && !(actualFirewallSubnetRoutes instanceof SdkAutoConstructList);
}
/**
*
* The actual firewall subnet routes.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasActualFirewallSubnetRoutes} method.
*
*
* @return The actual firewall subnet routes.
*/
public final List actualFirewallSubnetRoutes() {
return actualFirewallSubnetRoutes;
}
/**
*
* The internet gateway ID.
*
*
* @return The internet gateway ID.
*/
public final String internetGatewayId() {
return internetGatewayId;
}
/**
*
* The current route table for the internet gateway.
*
*
* @return The current route table for the internet gateway.
*/
public final String currentInternetGatewayRouteTable() {
return currentInternetGatewayRouteTable;
}
/**
* For responses, this returns true if the service returned a value for the ExpectedInternetGatewayRoutes property.
* This DOES NOT check that the value is non-empty (for which, you should check the {@code isEmpty()} method on the
* property). This is useful because the SDK will never return a null collection or map, but you may need to
* differentiate between the service returning nothing (or null) and the service returning an empty collection or
* map. For requests, this returns true if a value for the property was specified in the request builder, and false
* if a value was not specified.
*/
public final boolean hasExpectedInternetGatewayRoutes() {
return expectedInternetGatewayRoutes != null && !(expectedInternetGatewayRoutes instanceof SdkAutoConstructList);
}
/**
*
* The internet gateway routes that are expected.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasExpectedInternetGatewayRoutes} method.
*
*
* @return The internet gateway routes that are expected.
*/
public final List expectedInternetGatewayRoutes() {
return expectedInternetGatewayRoutes;
}
/**
* For responses, this returns true if the service returned a value for the ActualInternetGatewayRoutes property.
* This DOES NOT check that the value is non-empty (for which, you should check the {@code isEmpty()} method on the
* property). This is useful because the SDK will never return a null collection or map, but you may need to
* differentiate between the service returning nothing (or null) and the service returning an empty collection or
* map. For requests, this returns true if a value for the property was specified in the request builder, and false
* if a value was not specified.
*/
public final boolean hasActualInternetGatewayRoutes() {
return actualInternetGatewayRoutes != null && !(actualInternetGatewayRoutes instanceof SdkAutoConstructList);
}
/**
*
* The actual internet gateway routes.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasActualInternetGatewayRoutes} method.
*
*
* @return The actual internet gateway routes.
*/
public final List actualInternetGatewayRoutes() {
return actualInternetGatewayRoutes;
}
/**
*
* Information about the VPC ID.
*
*
* @return Information about the VPC ID.
*/
public final String vpcId() {
return vpcId;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(subnetId());
hashCode = 31 * hashCode + Objects.hashCode(subnetAvailabilityZone());
hashCode = 31 * hashCode + Objects.hashCode(routeTableId());
hashCode = 31 * hashCode + Objects.hashCode(hasViolatingRoutes() ? violatingRoutes() : null);
hashCode = 31 * hashCode + Objects.hashCode(isRouteTableUsedInDifferentAZ());
hashCode = 31 * hashCode + Objects.hashCode(currentFirewallSubnetRouteTable());
hashCode = 31 * hashCode + Objects.hashCode(expectedFirewallEndpoint());
hashCode = 31 * hashCode + Objects.hashCode(firewallSubnetId());
hashCode = 31 * hashCode + Objects.hashCode(hasExpectedFirewallSubnetRoutes() ? expectedFirewallSubnetRoutes() : null);
hashCode = 31 * hashCode + Objects.hashCode(hasActualFirewallSubnetRoutes() ? actualFirewallSubnetRoutes() : null);
hashCode = 31 * hashCode + Objects.hashCode(internetGatewayId());
hashCode = 31 * hashCode + Objects.hashCode(currentInternetGatewayRouteTable());
hashCode = 31 * hashCode + Objects.hashCode(hasExpectedInternetGatewayRoutes() ? expectedInternetGatewayRoutes() : null);
hashCode = 31 * hashCode + Objects.hashCode(hasActualInternetGatewayRoutes() ? actualInternetGatewayRoutes() : null);
hashCode = 31 * hashCode + Objects.hashCode(vpcId());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof NetworkFirewallInternetTrafficNotInspectedViolation)) {
return false;
}
NetworkFirewallInternetTrafficNotInspectedViolation other = (NetworkFirewallInternetTrafficNotInspectedViolation) obj;
return Objects.equals(subnetId(), other.subnetId())
&& Objects.equals(subnetAvailabilityZone(), other.subnetAvailabilityZone())
&& Objects.equals(routeTableId(), other.routeTableId()) && hasViolatingRoutes() == other.hasViolatingRoutes()
&& Objects.equals(violatingRoutes(), other.violatingRoutes())
&& Objects.equals(isRouteTableUsedInDifferentAZ(), other.isRouteTableUsedInDifferentAZ())
&& Objects.equals(currentFirewallSubnetRouteTable(), other.currentFirewallSubnetRouteTable())
&& Objects.equals(expectedFirewallEndpoint(), other.expectedFirewallEndpoint())
&& Objects.equals(firewallSubnetId(), other.firewallSubnetId())
&& hasExpectedFirewallSubnetRoutes() == other.hasExpectedFirewallSubnetRoutes()
&& Objects.equals(expectedFirewallSubnetRoutes(), other.expectedFirewallSubnetRoutes())
&& hasActualFirewallSubnetRoutes() == other.hasActualFirewallSubnetRoutes()
&& Objects.equals(actualFirewallSubnetRoutes(), other.actualFirewallSubnetRoutes())
&& Objects.equals(internetGatewayId(), other.internetGatewayId())
&& Objects.equals(currentInternetGatewayRouteTable(), other.currentInternetGatewayRouteTable())
&& hasExpectedInternetGatewayRoutes() == other.hasExpectedInternetGatewayRoutes()
&& Objects.equals(expectedInternetGatewayRoutes(), other.expectedInternetGatewayRoutes())
&& hasActualInternetGatewayRoutes() == other.hasActualInternetGatewayRoutes()
&& Objects.equals(actualInternetGatewayRoutes(), other.actualInternetGatewayRoutes())
&& Objects.equals(vpcId(), other.vpcId());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString
.builder("NetworkFirewallInternetTrafficNotInspectedViolation")
.add("SubnetId", subnetId())
.add("SubnetAvailabilityZone", subnetAvailabilityZone())
.add("RouteTableId", routeTableId())
.add("ViolatingRoutes", hasViolatingRoutes() ? violatingRoutes() : null)
.add("IsRouteTableUsedInDifferentAZ", isRouteTableUsedInDifferentAZ())
.add("CurrentFirewallSubnetRouteTable", currentFirewallSubnetRouteTable())
.add("ExpectedFirewallEndpoint", expectedFirewallEndpoint())
.add("FirewallSubnetId", firewallSubnetId())
.add("ExpectedFirewallSubnetRoutes", hasExpectedFirewallSubnetRoutes() ? expectedFirewallSubnetRoutes() : null)
.add("ActualFirewallSubnetRoutes", hasActualFirewallSubnetRoutes() ? actualFirewallSubnetRoutes() : null)
.add("InternetGatewayId", internetGatewayId())
.add("CurrentInternetGatewayRouteTable", currentInternetGatewayRouteTable())
.add("ExpectedInternetGatewayRoutes", hasExpectedInternetGatewayRoutes() ? expectedInternetGatewayRoutes() : null)
.add("ActualInternetGatewayRoutes", hasActualInternetGatewayRoutes() ? actualInternetGatewayRoutes() : null)
.add("VpcId", vpcId()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "SubnetId":
return Optional.ofNullable(clazz.cast(subnetId()));
case "SubnetAvailabilityZone":
return Optional.ofNullable(clazz.cast(subnetAvailabilityZone()));
case "RouteTableId":
return Optional.ofNullable(clazz.cast(routeTableId()));
case "ViolatingRoutes":
return Optional.ofNullable(clazz.cast(violatingRoutes()));
case "IsRouteTableUsedInDifferentAZ":
return Optional.ofNullable(clazz.cast(isRouteTableUsedInDifferentAZ()));
case "CurrentFirewallSubnetRouteTable":
return Optional.ofNullable(clazz.cast(currentFirewallSubnetRouteTable()));
case "ExpectedFirewallEndpoint":
return Optional.ofNullable(clazz.cast(expectedFirewallEndpoint()));
case "FirewallSubnetId":
return Optional.ofNullable(clazz.cast(firewallSubnetId()));
case "ExpectedFirewallSubnetRoutes":
return Optional.ofNullable(clazz.cast(expectedFirewallSubnetRoutes()));
case "ActualFirewallSubnetRoutes":
return Optional.ofNullable(clazz.cast(actualFirewallSubnetRoutes()));
case "InternetGatewayId":
return Optional.ofNullable(clazz.cast(internetGatewayId()));
case "CurrentInternetGatewayRouteTable":
return Optional.ofNullable(clazz.cast(currentInternetGatewayRouteTable()));
case "ExpectedInternetGatewayRoutes":
return Optional.ofNullable(clazz.cast(expectedInternetGatewayRoutes()));
case "ActualInternetGatewayRoutes":
return Optional.ofNullable(clazz.cast(actualInternetGatewayRoutes()));
case "VpcId":
return Optional.ofNullable(clazz.cast(vpcId()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
private static Function