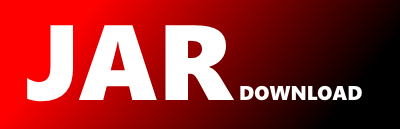
software.amazon.awssdk.services.fms.model.NetworkFirewallInvalidRouteConfigurationViolation Maven / Gradle / Ivy
Show all versions of fms Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.fms.model;
import java.beans.Transient;
import java.io.Serializable;
import java.util.Arrays;
import java.util.Collection;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import java.util.stream.Collectors;
import java.util.stream.Stream;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.ListTrait;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.core.util.DefaultSdkAutoConstructList;
import software.amazon.awssdk.core.util.SdkAutoConstructList;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* Violation detail for the improperly configured subnet route. It's possible there is a missing route table route, or a
* configuration that causes traffic to cross an Availability Zone boundary.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class NetworkFirewallInvalidRouteConfigurationViolation
implements
SdkPojo,
Serializable,
ToCopyableBuilder {
private static final SdkField> AFFECTED_SUBNETS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("AffectedSubnets")
.getter(getter(NetworkFirewallInvalidRouteConfigurationViolation::affectedSubnets))
.setter(setter(Builder::affectedSubnets))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("AffectedSubnets").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.STRING)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField ROUTE_TABLE_ID_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("RouteTableId").getter(getter(NetworkFirewallInvalidRouteConfigurationViolation::routeTableId))
.setter(setter(Builder::routeTableId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("RouteTableId").build()).build();
private static final SdkField IS_ROUTE_TABLE_USED_IN_DIFFERENT_AZ_FIELD = SdkField
. builder(MarshallingType.BOOLEAN)
.memberName("IsRouteTableUsedInDifferentAZ")
.getter(getter(NetworkFirewallInvalidRouteConfigurationViolation::isRouteTableUsedInDifferentAZ))
.setter(setter(Builder::isRouteTableUsedInDifferentAZ))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("IsRouteTableUsedInDifferentAZ")
.build()).build();
private static final SdkField VIOLATING_ROUTE_FIELD = SdkField. builder(MarshallingType.SDK_POJO)
.memberName("ViolatingRoute").getter(getter(NetworkFirewallInvalidRouteConfigurationViolation::violatingRoute))
.setter(setter(Builder::violatingRoute)).constructor(Route::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ViolatingRoute").build()).build();
private static final SdkField CURRENT_FIREWALL_SUBNET_ROUTE_TABLE_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("CurrentFirewallSubnetRouteTable")
.getter(getter(NetworkFirewallInvalidRouteConfigurationViolation::currentFirewallSubnetRouteTable))
.setter(setter(Builder::currentFirewallSubnetRouteTable))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("CurrentFirewallSubnetRouteTable")
.build()).build();
private static final SdkField EXPECTED_FIREWALL_ENDPOINT_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("ExpectedFirewallEndpoint")
.getter(getter(NetworkFirewallInvalidRouteConfigurationViolation::expectedFirewallEndpoint))
.setter(setter(Builder::expectedFirewallEndpoint))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ExpectedFirewallEndpoint").build())
.build();
private static final SdkField ACTUAL_FIREWALL_ENDPOINT_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("ActualFirewallEndpoint")
.getter(getter(NetworkFirewallInvalidRouteConfigurationViolation::actualFirewallEndpoint))
.setter(setter(Builder::actualFirewallEndpoint))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ActualFirewallEndpoint").build())
.build();
private static final SdkField EXPECTED_FIREWALL_SUBNET_ID_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("ExpectedFirewallSubnetId")
.getter(getter(NetworkFirewallInvalidRouteConfigurationViolation::expectedFirewallSubnetId))
.setter(setter(Builder::expectedFirewallSubnetId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ExpectedFirewallSubnetId").build())
.build();
private static final SdkField ACTUAL_FIREWALL_SUBNET_ID_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("ActualFirewallSubnetId")
.getter(getter(NetworkFirewallInvalidRouteConfigurationViolation::actualFirewallSubnetId))
.setter(setter(Builder::actualFirewallSubnetId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ActualFirewallSubnetId").build())
.build();
private static final SdkField> EXPECTED_FIREWALL_SUBNET_ROUTES_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("ExpectedFirewallSubnetRoutes")
.getter(getter(NetworkFirewallInvalidRouteConfigurationViolation::expectedFirewallSubnetRoutes))
.setter(setter(Builder::expectedFirewallSubnetRoutes))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ExpectedFirewallSubnetRoutes")
.build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(ExpectedRoute::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField> ACTUAL_FIREWALL_SUBNET_ROUTES_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("ActualFirewallSubnetRoutes")
.getter(getter(NetworkFirewallInvalidRouteConfigurationViolation::actualFirewallSubnetRoutes))
.setter(setter(Builder::actualFirewallSubnetRoutes))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ActualFirewallSubnetRoutes").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(Route::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField INTERNET_GATEWAY_ID_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("InternetGatewayId").getter(getter(NetworkFirewallInvalidRouteConfigurationViolation::internetGatewayId))
.setter(setter(Builder::internetGatewayId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("InternetGatewayId").build()).build();
private static final SdkField CURRENT_INTERNET_GATEWAY_ROUTE_TABLE_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("CurrentInternetGatewayRouteTable")
.getter(getter(NetworkFirewallInvalidRouteConfigurationViolation::currentInternetGatewayRouteTable))
.setter(setter(Builder::currentInternetGatewayRouteTable))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("CurrentInternetGatewayRouteTable")
.build()).build();
private static final SdkField> EXPECTED_INTERNET_GATEWAY_ROUTES_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("ExpectedInternetGatewayRoutes")
.getter(getter(NetworkFirewallInvalidRouteConfigurationViolation::expectedInternetGatewayRoutes))
.setter(setter(Builder::expectedInternetGatewayRoutes))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ExpectedInternetGatewayRoutes")
.build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(ExpectedRoute::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField> ACTUAL_INTERNET_GATEWAY_ROUTES_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("ActualInternetGatewayRoutes")
.getter(getter(NetworkFirewallInvalidRouteConfigurationViolation::actualInternetGatewayRoutes))
.setter(setter(Builder::actualInternetGatewayRoutes))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ActualInternetGatewayRoutes")
.build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(Route::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField VPC_ID_FIELD = SdkField. builder(MarshallingType.STRING).memberName("VpcId")
.getter(getter(NetworkFirewallInvalidRouteConfigurationViolation::vpcId)).setter(setter(Builder::vpcId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("VpcId").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(AFFECTED_SUBNETS_FIELD,
ROUTE_TABLE_ID_FIELD, IS_ROUTE_TABLE_USED_IN_DIFFERENT_AZ_FIELD, VIOLATING_ROUTE_FIELD,
CURRENT_FIREWALL_SUBNET_ROUTE_TABLE_FIELD, EXPECTED_FIREWALL_ENDPOINT_FIELD, ACTUAL_FIREWALL_ENDPOINT_FIELD,
EXPECTED_FIREWALL_SUBNET_ID_FIELD, ACTUAL_FIREWALL_SUBNET_ID_FIELD, EXPECTED_FIREWALL_SUBNET_ROUTES_FIELD,
ACTUAL_FIREWALL_SUBNET_ROUTES_FIELD, INTERNET_GATEWAY_ID_FIELD, CURRENT_INTERNET_GATEWAY_ROUTE_TABLE_FIELD,
EXPECTED_INTERNET_GATEWAY_ROUTES_FIELD, ACTUAL_INTERNET_GATEWAY_ROUTES_FIELD, VPC_ID_FIELD));
private static final long serialVersionUID = 1L;
private final List affectedSubnets;
private final String routeTableId;
private final Boolean isRouteTableUsedInDifferentAZ;
private final Route violatingRoute;
private final String currentFirewallSubnetRouteTable;
private final String expectedFirewallEndpoint;
private final String actualFirewallEndpoint;
private final String expectedFirewallSubnetId;
private final String actualFirewallSubnetId;
private final List expectedFirewallSubnetRoutes;
private final List actualFirewallSubnetRoutes;
private final String internetGatewayId;
private final String currentInternetGatewayRouteTable;
private final List expectedInternetGatewayRoutes;
private final List actualInternetGatewayRoutes;
private final String vpcId;
private NetworkFirewallInvalidRouteConfigurationViolation(BuilderImpl builder) {
this.affectedSubnets = builder.affectedSubnets;
this.routeTableId = builder.routeTableId;
this.isRouteTableUsedInDifferentAZ = builder.isRouteTableUsedInDifferentAZ;
this.violatingRoute = builder.violatingRoute;
this.currentFirewallSubnetRouteTable = builder.currentFirewallSubnetRouteTable;
this.expectedFirewallEndpoint = builder.expectedFirewallEndpoint;
this.actualFirewallEndpoint = builder.actualFirewallEndpoint;
this.expectedFirewallSubnetId = builder.expectedFirewallSubnetId;
this.actualFirewallSubnetId = builder.actualFirewallSubnetId;
this.expectedFirewallSubnetRoutes = builder.expectedFirewallSubnetRoutes;
this.actualFirewallSubnetRoutes = builder.actualFirewallSubnetRoutes;
this.internetGatewayId = builder.internetGatewayId;
this.currentInternetGatewayRouteTable = builder.currentInternetGatewayRouteTable;
this.expectedInternetGatewayRoutes = builder.expectedInternetGatewayRoutes;
this.actualInternetGatewayRoutes = builder.actualInternetGatewayRoutes;
this.vpcId = builder.vpcId;
}
/**
* For responses, this returns true if the service returned a value for the AffectedSubnets property. This DOES NOT
* check that the value is non-empty (for which, you should check the {@code isEmpty()} method on the property).
* This is useful because the SDK will never return a null collection or map, but you may need to differentiate
* between the service returning nothing (or null) and the service returning an empty collection or map. For
* requests, this returns true if a value for the property was specified in the request builder, and false if a
* value was not specified.
*/
public final boolean hasAffectedSubnets() {
return affectedSubnets != null && !(affectedSubnets instanceof SdkAutoConstructList);
}
/**
*
* The subnets that are affected.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasAffectedSubnets} method.
*
*
* @return The subnets that are affected.
*/
public final List affectedSubnets() {
return affectedSubnets;
}
/**
*
* The route table ID.
*
*
* @return The route table ID.
*/
public final String routeTableId() {
return routeTableId;
}
/**
*
* Information about whether the route table is used in another Availability Zone.
*
*
* @return Information about whether the route table is used in another Availability Zone.
*/
public final Boolean isRouteTableUsedInDifferentAZ() {
return isRouteTableUsedInDifferentAZ;
}
/**
*
* The route that's in violation.
*
*
* @return The route that's in violation.
*/
public final Route violatingRoute() {
return violatingRoute;
}
/**
*
* The subnet route table for the current firewall.
*
*
* @return The subnet route table for the current firewall.
*/
public final String currentFirewallSubnetRouteTable() {
return currentFirewallSubnetRouteTable;
}
/**
*
* The firewall endpoint that's expected.
*
*
* @return The firewall endpoint that's expected.
*/
public final String expectedFirewallEndpoint() {
return expectedFirewallEndpoint;
}
/**
*
* The actual firewall endpoint.
*
*
* @return The actual firewall endpoint.
*/
public final String actualFirewallEndpoint() {
return actualFirewallEndpoint;
}
/**
*
* The expected subnet ID for the firewall.
*
*
* @return The expected subnet ID for the firewall.
*/
public final String expectedFirewallSubnetId() {
return expectedFirewallSubnetId;
}
/**
*
* The actual subnet ID for the firewall.
*
*
* @return The actual subnet ID for the firewall.
*/
public final String actualFirewallSubnetId() {
return actualFirewallSubnetId;
}
/**
* For responses, this returns true if the service returned a value for the ExpectedFirewallSubnetRoutes property.
* This DOES NOT check that the value is non-empty (for which, you should check the {@code isEmpty()} method on the
* property). This is useful because the SDK will never return a null collection or map, but you may need to
* differentiate between the service returning nothing (or null) and the service returning an empty collection or
* map. For requests, this returns true if a value for the property was specified in the request builder, and false
* if a value was not specified.
*/
public final boolean hasExpectedFirewallSubnetRoutes() {
return expectedFirewallSubnetRoutes != null && !(expectedFirewallSubnetRoutes instanceof SdkAutoConstructList);
}
/**
*
* The firewall subnet routes that are expected.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasExpectedFirewallSubnetRoutes} method.
*
*
* @return The firewall subnet routes that are expected.
*/
public final List expectedFirewallSubnetRoutes() {
return expectedFirewallSubnetRoutes;
}
/**
* For responses, this returns true if the service returned a value for the ActualFirewallSubnetRoutes property.
* This DOES NOT check that the value is non-empty (for which, you should check the {@code isEmpty()} method on the
* property). This is useful because the SDK will never return a null collection or map, but you may need to
* differentiate between the service returning nothing (or null) and the service returning an empty collection or
* map. For requests, this returns true if a value for the property was specified in the request builder, and false
* if a value was not specified.
*/
public final boolean hasActualFirewallSubnetRoutes() {
return actualFirewallSubnetRoutes != null && !(actualFirewallSubnetRoutes instanceof SdkAutoConstructList);
}
/**
*
* The actual firewall subnet routes that are expected.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasActualFirewallSubnetRoutes} method.
*
*
* @return The actual firewall subnet routes that are expected.
*/
public final List actualFirewallSubnetRoutes() {
return actualFirewallSubnetRoutes;
}
/**
*
* The internet gateway ID.
*
*
* @return The internet gateway ID.
*/
public final String internetGatewayId() {
return internetGatewayId;
}
/**
*
* The route table for the current internet gateway.
*
*
* @return The route table for the current internet gateway.
*/
public final String currentInternetGatewayRouteTable() {
return currentInternetGatewayRouteTable;
}
/**
* For responses, this returns true if the service returned a value for the ExpectedInternetGatewayRoutes property.
* This DOES NOT check that the value is non-empty (for which, you should check the {@code isEmpty()} method on the
* property). This is useful because the SDK will never return a null collection or map, but you may need to
* differentiate between the service returning nothing (or null) and the service returning an empty collection or
* map. For requests, this returns true if a value for the property was specified in the request builder, and false
* if a value was not specified.
*/
public final boolean hasExpectedInternetGatewayRoutes() {
return expectedInternetGatewayRoutes != null && !(expectedInternetGatewayRoutes instanceof SdkAutoConstructList);
}
/**
*
* The expected routes for the internet gateway.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasExpectedInternetGatewayRoutes} method.
*
*
* @return The expected routes for the internet gateway.
*/
public final List expectedInternetGatewayRoutes() {
return expectedInternetGatewayRoutes;
}
/**
* For responses, this returns true if the service returned a value for the ActualInternetGatewayRoutes property.
* This DOES NOT check that the value is non-empty (for which, you should check the {@code isEmpty()} method on the
* property). This is useful because the SDK will never return a null collection or map, but you may need to
* differentiate between the service returning nothing (or null) and the service returning an empty collection or
* map. For requests, this returns true if a value for the property was specified in the request builder, and false
* if a value was not specified.
*/
public final boolean hasActualInternetGatewayRoutes() {
return actualInternetGatewayRoutes != null && !(actualInternetGatewayRoutes instanceof SdkAutoConstructList);
}
/**
*
* The actual internet gateway routes.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasActualInternetGatewayRoutes} method.
*
*
* @return The actual internet gateway routes.
*/
public final List actualInternetGatewayRoutes() {
return actualInternetGatewayRoutes;
}
/**
*
* Information about the VPC ID.
*
*
* @return Information about the VPC ID.
*/
public final String vpcId() {
return vpcId;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(hasAffectedSubnets() ? affectedSubnets() : null);
hashCode = 31 * hashCode + Objects.hashCode(routeTableId());
hashCode = 31 * hashCode + Objects.hashCode(isRouteTableUsedInDifferentAZ());
hashCode = 31 * hashCode + Objects.hashCode(violatingRoute());
hashCode = 31 * hashCode + Objects.hashCode(currentFirewallSubnetRouteTable());
hashCode = 31 * hashCode + Objects.hashCode(expectedFirewallEndpoint());
hashCode = 31 * hashCode + Objects.hashCode(actualFirewallEndpoint());
hashCode = 31 * hashCode + Objects.hashCode(expectedFirewallSubnetId());
hashCode = 31 * hashCode + Objects.hashCode(actualFirewallSubnetId());
hashCode = 31 * hashCode + Objects.hashCode(hasExpectedFirewallSubnetRoutes() ? expectedFirewallSubnetRoutes() : null);
hashCode = 31 * hashCode + Objects.hashCode(hasActualFirewallSubnetRoutes() ? actualFirewallSubnetRoutes() : null);
hashCode = 31 * hashCode + Objects.hashCode(internetGatewayId());
hashCode = 31 * hashCode + Objects.hashCode(currentInternetGatewayRouteTable());
hashCode = 31 * hashCode + Objects.hashCode(hasExpectedInternetGatewayRoutes() ? expectedInternetGatewayRoutes() : null);
hashCode = 31 * hashCode + Objects.hashCode(hasActualInternetGatewayRoutes() ? actualInternetGatewayRoutes() : null);
hashCode = 31 * hashCode + Objects.hashCode(vpcId());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof NetworkFirewallInvalidRouteConfigurationViolation)) {
return false;
}
NetworkFirewallInvalidRouteConfigurationViolation other = (NetworkFirewallInvalidRouteConfigurationViolation) obj;
return hasAffectedSubnets() == other.hasAffectedSubnets() && Objects.equals(affectedSubnets(), other.affectedSubnets())
&& Objects.equals(routeTableId(), other.routeTableId())
&& Objects.equals(isRouteTableUsedInDifferentAZ(), other.isRouteTableUsedInDifferentAZ())
&& Objects.equals(violatingRoute(), other.violatingRoute())
&& Objects.equals(currentFirewallSubnetRouteTable(), other.currentFirewallSubnetRouteTable())
&& Objects.equals(expectedFirewallEndpoint(), other.expectedFirewallEndpoint())
&& Objects.equals(actualFirewallEndpoint(), other.actualFirewallEndpoint())
&& Objects.equals(expectedFirewallSubnetId(), other.expectedFirewallSubnetId())
&& Objects.equals(actualFirewallSubnetId(), other.actualFirewallSubnetId())
&& hasExpectedFirewallSubnetRoutes() == other.hasExpectedFirewallSubnetRoutes()
&& Objects.equals(expectedFirewallSubnetRoutes(), other.expectedFirewallSubnetRoutes())
&& hasActualFirewallSubnetRoutes() == other.hasActualFirewallSubnetRoutes()
&& Objects.equals(actualFirewallSubnetRoutes(), other.actualFirewallSubnetRoutes())
&& Objects.equals(internetGatewayId(), other.internetGatewayId())
&& Objects.equals(currentInternetGatewayRouteTable(), other.currentInternetGatewayRouteTable())
&& hasExpectedInternetGatewayRoutes() == other.hasExpectedInternetGatewayRoutes()
&& Objects.equals(expectedInternetGatewayRoutes(), other.expectedInternetGatewayRoutes())
&& hasActualInternetGatewayRoutes() == other.hasActualInternetGatewayRoutes()
&& Objects.equals(actualInternetGatewayRoutes(), other.actualInternetGatewayRoutes())
&& Objects.equals(vpcId(), other.vpcId());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString
.builder("NetworkFirewallInvalidRouteConfigurationViolation")
.add("AffectedSubnets", hasAffectedSubnets() ? affectedSubnets() : null)
.add("RouteTableId", routeTableId())
.add("IsRouteTableUsedInDifferentAZ", isRouteTableUsedInDifferentAZ())
.add("ViolatingRoute", violatingRoute())
.add("CurrentFirewallSubnetRouteTable", currentFirewallSubnetRouteTable())
.add("ExpectedFirewallEndpoint", expectedFirewallEndpoint())
.add("ActualFirewallEndpoint", actualFirewallEndpoint())
.add("ExpectedFirewallSubnetId", expectedFirewallSubnetId())
.add("ActualFirewallSubnetId", actualFirewallSubnetId())
.add("ExpectedFirewallSubnetRoutes", hasExpectedFirewallSubnetRoutes() ? expectedFirewallSubnetRoutes() : null)
.add("ActualFirewallSubnetRoutes", hasActualFirewallSubnetRoutes() ? actualFirewallSubnetRoutes() : null)
.add("InternetGatewayId", internetGatewayId())
.add("CurrentInternetGatewayRouteTable", currentInternetGatewayRouteTable())
.add("ExpectedInternetGatewayRoutes", hasExpectedInternetGatewayRoutes() ? expectedInternetGatewayRoutes() : null)
.add("ActualInternetGatewayRoutes", hasActualInternetGatewayRoutes() ? actualInternetGatewayRoutes() : null)
.add("VpcId", vpcId()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "AffectedSubnets":
return Optional.ofNullable(clazz.cast(affectedSubnets()));
case "RouteTableId":
return Optional.ofNullable(clazz.cast(routeTableId()));
case "IsRouteTableUsedInDifferentAZ":
return Optional.ofNullable(clazz.cast(isRouteTableUsedInDifferentAZ()));
case "ViolatingRoute":
return Optional.ofNullable(clazz.cast(violatingRoute()));
case "CurrentFirewallSubnetRouteTable":
return Optional.ofNullable(clazz.cast(currentFirewallSubnetRouteTable()));
case "ExpectedFirewallEndpoint":
return Optional.ofNullable(clazz.cast(expectedFirewallEndpoint()));
case "ActualFirewallEndpoint":
return Optional.ofNullable(clazz.cast(actualFirewallEndpoint()));
case "ExpectedFirewallSubnetId":
return Optional.ofNullable(clazz.cast(expectedFirewallSubnetId()));
case "ActualFirewallSubnetId":
return Optional.ofNullable(clazz.cast(actualFirewallSubnetId()));
case "ExpectedFirewallSubnetRoutes":
return Optional.ofNullable(clazz.cast(expectedFirewallSubnetRoutes()));
case "ActualFirewallSubnetRoutes":
return Optional.ofNullable(clazz.cast(actualFirewallSubnetRoutes()));
case "InternetGatewayId":
return Optional.ofNullable(clazz.cast(internetGatewayId()));
case "CurrentInternetGatewayRouteTable":
return Optional.ofNullable(clazz.cast(currentInternetGatewayRouteTable()));
case "ExpectedInternetGatewayRoutes":
return Optional.ofNullable(clazz.cast(expectedInternetGatewayRoutes()));
case "ActualInternetGatewayRoutes":
return Optional.ofNullable(clazz.cast(actualInternetGatewayRoutes()));
case "VpcId":
return Optional.ofNullable(clazz.cast(vpcId()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
private static Function