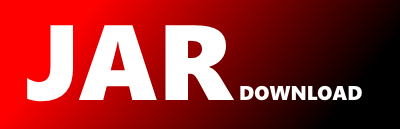
software.amazon.awssdk.services.fms.model.ThirdPartyFirewallMissingExpectedRouteTableViolation Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of fms Show documentation
Show all versions of fms Show documentation
The AWS Java SDK for FMS module holds the client classes that are used for
communicating with FMS.
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.fms.model;
import java.io.Serializable;
import java.util.Arrays;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* The violation details for a third-party firewall that's not associated with an Firewall Manager managed route table.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class ThirdPartyFirewallMissingExpectedRouteTableViolation
implements
SdkPojo,
Serializable,
ToCopyableBuilder {
private static final SdkField VIOLATION_TARGET_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("ViolationTarget").getter(getter(ThirdPartyFirewallMissingExpectedRouteTableViolation::violationTarget))
.setter(setter(Builder::violationTarget))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ViolationTarget").build()).build();
private static final SdkField VPC_FIELD = SdkField. builder(MarshallingType.STRING).memberName("VPC")
.getter(getter(ThirdPartyFirewallMissingExpectedRouteTableViolation::vpc)).setter(setter(Builder::vpc))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("VPC").build()).build();
private static final SdkField AVAILABILITY_ZONE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("AvailabilityZone")
.getter(getter(ThirdPartyFirewallMissingExpectedRouteTableViolation::availabilityZone))
.setter(setter(Builder::availabilityZone))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("AvailabilityZone").build()).build();
private static final SdkField CURRENT_ROUTE_TABLE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("CurrentRouteTable")
.getter(getter(ThirdPartyFirewallMissingExpectedRouteTableViolation::currentRouteTable))
.setter(setter(Builder::currentRouteTable))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("CurrentRouteTable").build()).build();
private static final SdkField EXPECTED_ROUTE_TABLE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("ExpectedRouteTable")
.getter(getter(ThirdPartyFirewallMissingExpectedRouteTableViolation::expectedRouteTable))
.setter(setter(Builder::expectedRouteTable))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ExpectedRouteTable").build())
.build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(VIOLATION_TARGET_FIELD,
VPC_FIELD, AVAILABILITY_ZONE_FIELD, CURRENT_ROUTE_TABLE_FIELD, EXPECTED_ROUTE_TABLE_FIELD));
private static final long serialVersionUID = 1L;
private final String violationTarget;
private final String vpc;
private final String availabilityZone;
private final String currentRouteTable;
private final String expectedRouteTable;
private ThirdPartyFirewallMissingExpectedRouteTableViolation(BuilderImpl builder) {
this.violationTarget = builder.violationTarget;
this.vpc = builder.vpc;
this.availabilityZone = builder.availabilityZone;
this.currentRouteTable = builder.currentRouteTable;
this.expectedRouteTable = builder.expectedRouteTable;
}
/**
*
* The ID of the third-party firewall or VPC resource that's causing the violation.
*
*
* @return The ID of the third-party firewall or VPC resource that's causing the violation.
*/
public final String violationTarget() {
return violationTarget;
}
/**
*
* The resource ID of the VPC associated with a fireawll subnet that's causing the violation.
*
*
* @return The resource ID of the VPC associated with a fireawll subnet that's causing the violation.
*/
public final String vpc() {
return vpc;
}
/**
*
* The Availability Zone of the firewall subnet that's causing the violation.
*
*
* @return The Availability Zone of the firewall subnet that's causing the violation.
*/
public final String availabilityZone() {
return availabilityZone;
}
/**
*
* The resource ID of the current route table that's associated with the subnet, if one is available.
*
*
* @return The resource ID of the current route table that's associated with the subnet, if one is available.
*/
public final String currentRouteTable() {
return currentRouteTable;
}
/**
*
* The resource ID of the route table that should be associated with the subnet.
*
*
* @return The resource ID of the route table that should be associated with the subnet.
*/
public final String expectedRouteTable() {
return expectedRouteTable;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(violationTarget());
hashCode = 31 * hashCode + Objects.hashCode(vpc());
hashCode = 31 * hashCode + Objects.hashCode(availabilityZone());
hashCode = 31 * hashCode + Objects.hashCode(currentRouteTable());
hashCode = 31 * hashCode + Objects.hashCode(expectedRouteTable());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof ThirdPartyFirewallMissingExpectedRouteTableViolation)) {
return false;
}
ThirdPartyFirewallMissingExpectedRouteTableViolation other = (ThirdPartyFirewallMissingExpectedRouteTableViolation) obj;
return Objects.equals(violationTarget(), other.violationTarget()) && Objects.equals(vpc(), other.vpc())
&& Objects.equals(availabilityZone(), other.availabilityZone())
&& Objects.equals(currentRouteTable(), other.currentRouteTable())
&& Objects.equals(expectedRouteTable(), other.expectedRouteTable());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("ThirdPartyFirewallMissingExpectedRouteTableViolation").add("ViolationTarget", violationTarget())
.add("VPC", vpc()).add("AvailabilityZone", availabilityZone()).add("CurrentRouteTable", currentRouteTable())
.add("ExpectedRouteTable", expectedRouteTable()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "ViolationTarget":
return Optional.ofNullable(clazz.cast(violationTarget()));
case "VPC":
return Optional.ofNullable(clazz.cast(vpc()));
case "AvailabilityZone":
return Optional.ofNullable(clazz.cast(availabilityZone()));
case "CurrentRouteTable":
return Optional.ofNullable(clazz.cast(currentRouteTable()));
case "ExpectedRouteTable":
return Optional.ofNullable(clazz.cast(expectedRouteTable()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
private static Function
© 2015 - 2025 Weber Informatics LLC | Privacy Policy